The C++ Algorithm Library provides a collection of algorithms for operations on containers, allowing developers to write efficient code for tasks such as sorting, searching, and manipulating data with ease.
Here's a simple example demonstrating the use of the `std::sort` algorithm to sort an array:
#include <iostream>
#include <algorithm>
int main() {
int arr[] = {5, 3, 8, 1, 2};
std::sort(arr, arr + 5);
for (int n : arr) {
std::cout << n << ' ';
}
return 0;
}
What is the C++ Algorithm Library?
The C++ Algorithm Library is a part of the Standard Library that provides a collection of algorithms designed to operate on a range of elements, such as arrays and containers. These algorithms are highly efficient, easy to use, and versatile, allowing programmers to handle a wide variety of tasks ranging from sorting and searching to manipulating data.
Using the algorithm library can greatly enhance the performance of your programs. It abstracts complex implementations, allowing you to leverage highly optimized algorithms without needing to understand the intricate details behind them. This means you can focus on solving problems rather than reinventing the wheel.
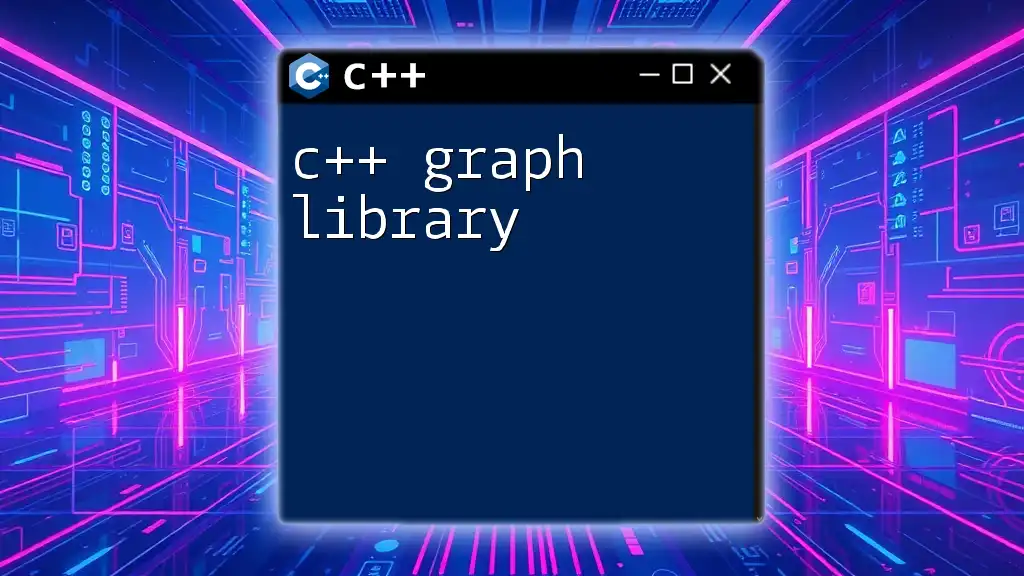
Types of Algorithms in the C++ Library
Sorting Algorithms
Sorting algorithms organize data in a specified order, which is a fundamental operation in programming. The C++ algorithm library includes several efficient sorting methods:
-
std::sort: This is perhaps the most commonly used sorting function. It sorts a range using the QuickSort algorithm (or Introsort, which is a hybrid sort algorithm).
Here's an example of how to use it:
#include <algorithm> #include <vector> std::vector<int> vec = {5, 3, 8, 1, 2}; std::sort(vec.begin(), vec.end());
In this snippet, the `std::sort` function sorts the vector `vec` in ascending order.
-
std::stable_sort: Unlike `std::sort`, this function preserves the relative order of equivalent elements. This can be particularly useful when the order of elements carries significance.
-
std::partial_sort: This function can sort a subset of the elements while leaving the rest unordered, which can be beneficial when you only need the top N elements.
Searching Algorithms
Searching algorithms are vital for finding specific data within a collection. The C++ algorithm library provides several efficient searching techniques:
-
std::find: This algorithm searches for a specific value in a range.
Example:
#include <vector> #include <algorithm> std::vector<int> vec = {10, 20, 30, 40}; auto it = std::find(vec.begin(), vec.end(), 20);
Here, `std::find` returns an iterator to the first occurrence of the number 20.
-
std::binary_search: This function determines if a value exists inside a sorted range, offering a faster search method than linear searching when data is sorted.
-
std::lower_bound and std::upper_bound: These functions return the position of the first element that is not less than (or greater than) the specified value. They're especially useful in sorted ranges.
Manipulation Algorithms
Manipulating data structures can be essential for effective programming. The algorithm library includes a variety of useful manipulation functions:
-
std::reverse: This function reverses the order of elements in a range.
Usage example:
#include <vector> #include <algorithm> std::vector<int> vec = {1, 2, 3, 4}; std::reverse(vec.begin(), vec.end());
After calling `std::reverse`, the vector `vec` will contain the elements in reverse order.
-
std::transform: It applies a given function to each element in a range and stores the result in another range, making it perfect for transforming data.
-
std::copy: This function copies elements from one range to another, which can be useful when you need to create duplicates of data.
Numeric Algorithms
Numeric algorithms in the C++ Algorithm Library are particularly useful in mathematical computations and data analysis:
-
std::accumulate: This function sums up the elements in a range, making it easy to compute totals and averages.
Here's how you can use it:
#include <numeric> #include <vector> std::vector<int> vec = {1, 2, 3, 4}; int sum = std::accumulate(vec.begin(), vec.end(), 0);
In this case, `std::accumulate` computes the total of all elements in `vec`, resulting in a sum of 10.
-
std::inner_product: This function calculates the inner product of two ranges, which is commonly used in mathematical applications involving vectors.
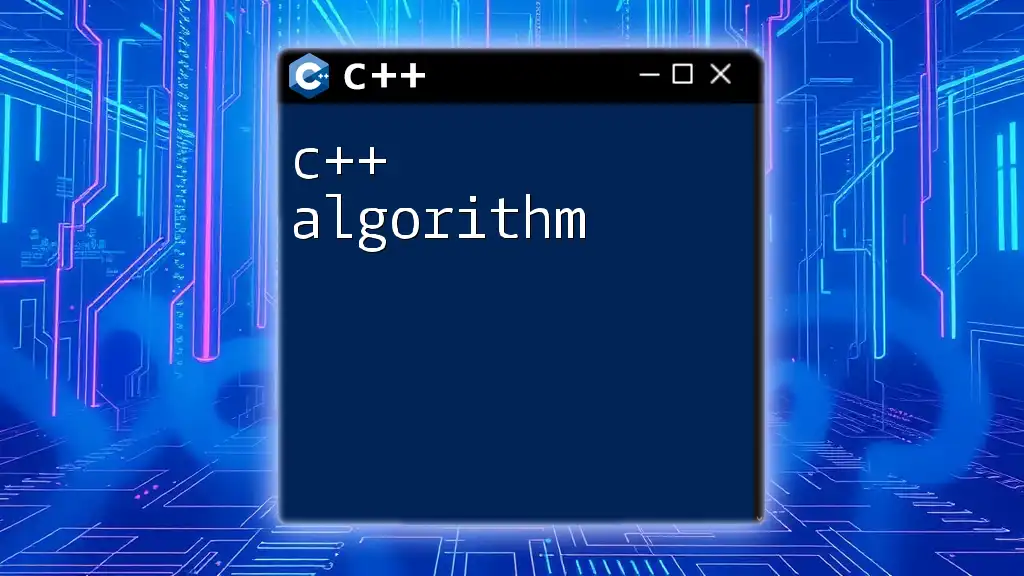
How to Use the C++ Algorithm Library Effectively
Best Practices for Implementing Algorithms
When using the C++ Algorithm Library, adhering to best practices can significantly enhance your programming efficiency. Here are some tips:
-
Choosing the Right Algorithm: Understand your data and the problem you're solving. For example, if you're dealing with a sorted dataset, consider using binary search methods for improved performance.
-
Performance Considerations: It's crucial to consider the time and space complexities of the algorithms. Select algorithms based on the size of your datasets and the operations you need to perform.
-
Using Iterators: The C++ Standard Library algorithms utilize iterators, allowing you to operate on any data structure that supports them, leading to more flexible and reusable code.
Common Mistakes and How to Avoid Them
Even experienced programmers can fall into pitfalls while using the C++ Algorithm Library. Being aware of common mistakes can help you avoid them:
-
Understanding Input Requirements: Many algorithms have specific requirements for the types of iterators they accept. Always consult the documentation to ensure compatibility.
-
Considering Return Types: Be mindful of what each function returns. For example, searching algorithms return iterators that need to be checked before dereferencing.
-
Overusing Algorithms: While the library provides powerful functionality, over-relying on algorithms for simple problems can lead to unnecessary complexity and performance bottlenecks. Striking a balance is essential.
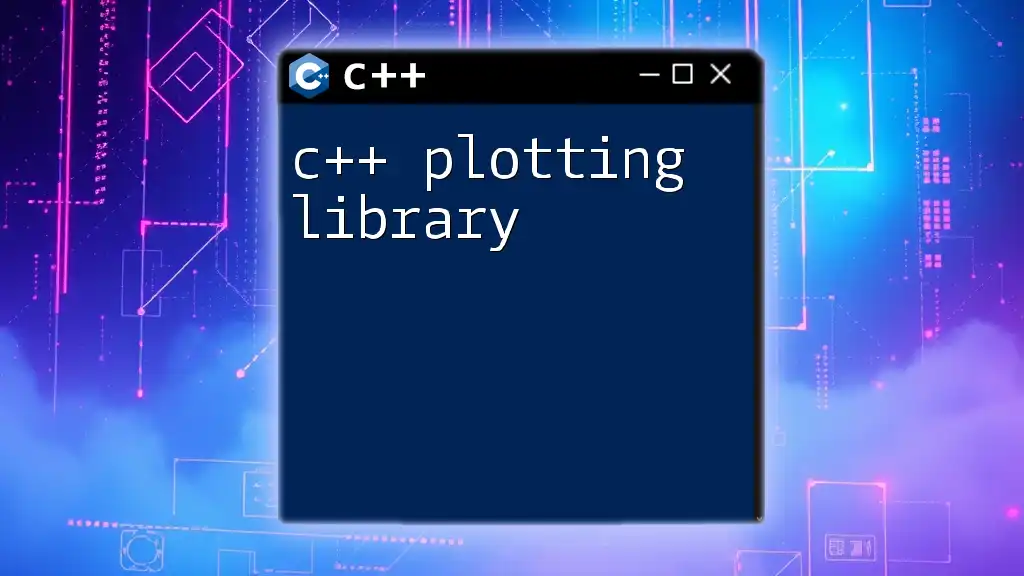
Real-World Applications of the C++ Algorithm Library
Case Study: Sorting Large Datasets
Sorting algorithms in C++ can be employed to efficiently process large datasets. For instance, if you have a file containing thousands of records, using `std::sort` can help quickly organize this data before executing further operations, such as filtering or searching.
Consider a scenario where you need to sort a CSV file of user data based on user IDs. The following code snippet illustrates how you could accomplish this:
#include <iostream>
#include <fstream>
#include <vector>
#include <algorithm>
#include <string>
// Assume User is a struct defined to hold user data
struct User {
int id;
std::string name;
// ... Other fields
};
// Function to sort users by ID
void sortUsersById(std::vector<User>& users) {
std::sort(users.begin(), users.end(), [](const User& a, const User& b) {
return a.id < b.id;
});
}
Case Study: Searching in Databases
Searching algorithms are crucial in database management systems. For example, employing binary search can improve the performance of record retrievals vastly. When combined with indexing techniques, it optimally locates entries.
Using std::lower_bound for record lookups in an ordered dataset can demonstrate this concept effectively. By ensuring your dataset is sorted and leveraging this algorithm, you can achieve logging retrieval times that are logarithmic rather than linear.
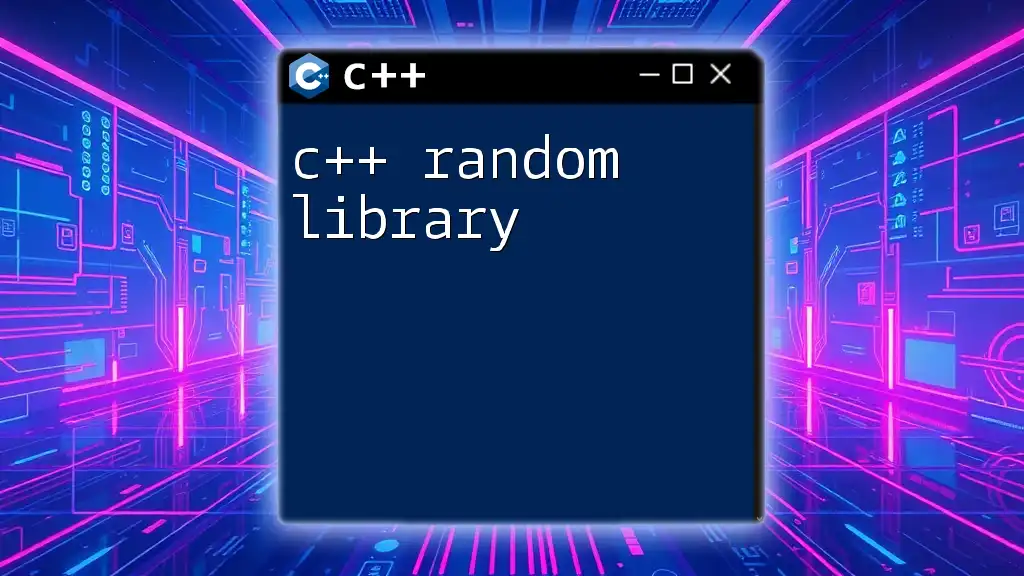
Additional Resources
To extend your knowledge of the C++ Algorithm Library, several resources can help:
-
Books: Titles like The C++ Standard Library by Nicolai M. Josuttis offer thorough insights into standard practices.
-
Online Courses: Platforms like Coursera and Udemy have C++ courses that include sections focused on the algorithm library.
-
Official Documentation: The official C++ documentation contains comprehensive details and examples regarding all the algorithms available in the library.
-
Community Forums: Websites like Stack Overflow and Reddit’s r/cpp can provide support and discussion on best practices.
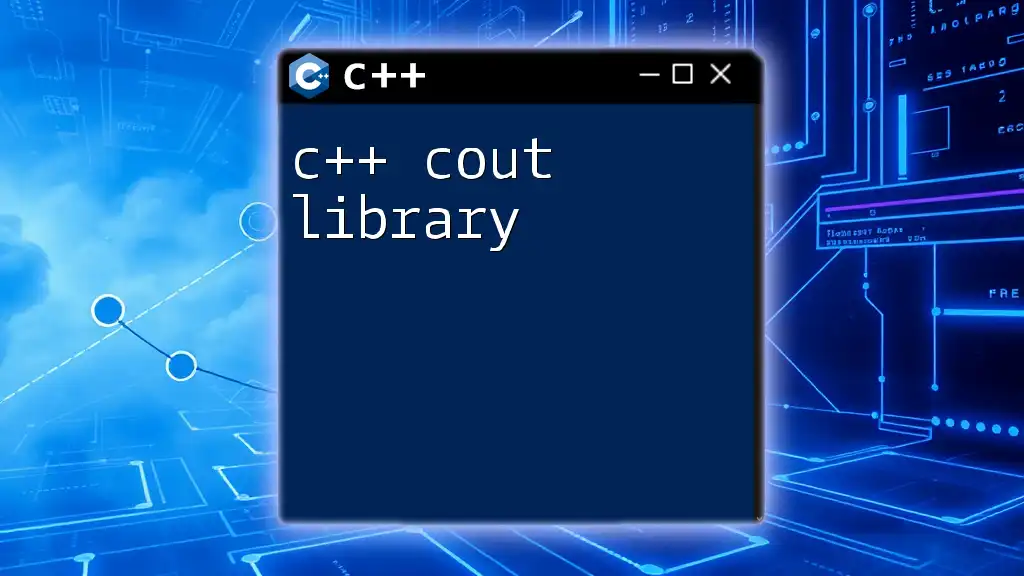
Conclusion
The C++ Algorithm Library is a powerful suite of tools that can immensely improve the efficiency of your C++ programs. By understanding the various types of algorithms available, their optimal use cases, and common pitfalls, you can harness their capabilities to produce clean, efficient, and effective code.
Start your journey with this library today. Explore the algorithms discussed, practice implementing them, and observe how they can elevate your programming projects. Happy coding!
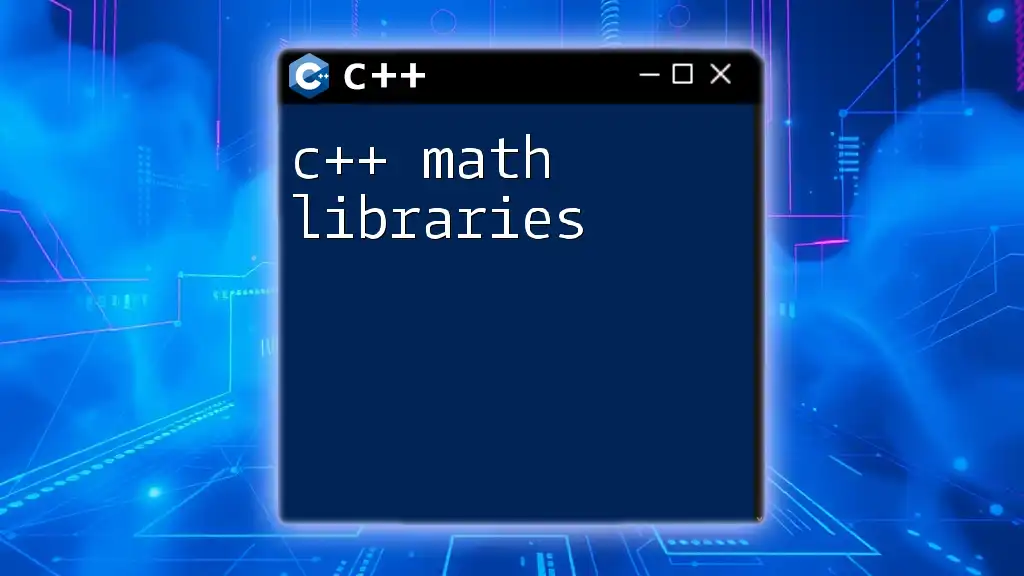
FAQs (Frequently Asked Questions)
What are the differences between `std::sort` and `std::stable_sort`?
The primary difference between these two functions lies in how they handle equivalent elements. While `std::sort` may rearrange equal elements arbitrarily, `std::stable_sort` ensures that their relative order remains unchanged after sorting.
How can I create custom algorithms using the C++ library?
Creating custom algorithms in C++ typically involves defining a function that adheres to the expected input and output types. These functions can then utilize C++ Standard Library features such as iterators and templates for maximum flexibility.
Are all algorithms in the C++ library optimized?
Most algorithms provided in the C++ Standard Library are optimized for efficiency and performance. However, the choice of the correct algorithm for a specific problem can significantly impact overall performance. It's essential to understand the underlying data structures and complexities when selecting an algorithm.