C++ algorithms are built-in functions in the Standard Template Library (STL) that perform various operations, such as searching, sorting, and modifying collections of data.
Here’s a simple example of using the `std::sort` algorithm to sort an array of integers:
#include <algorithm>
#include <iostream>
int main() {
int arr[] = {5, 2, 9, 1, 5, 6};
std::sort(arr, arr + 6);
for (int i : arr) {
std::cout << i << " ";
}
return 0;
}
Understanding Algorithms in C++
An algorithm in C++ refers to a well-defined sequence of steps or instructions designed to solve a particular problem. Every algorithm has specific characteristics, including clarity, finiteness, and effectiveness. In the realm of programming, particularly in C++, efficient algorithms are essential as they directly impact the performance and speed of software applications. They are the backbone of operations that manage data and perform critical tasks, making understanding algorithms fundamental for any C++ developer.
The Standard Template Library (STL) in C++ provides a multitude of built-in algorithms and data structures, allowing developers to utilize advanced features without extensive coding. These include sorting, searching, and manipulating collections of data, which are fundamental tasks in software development.
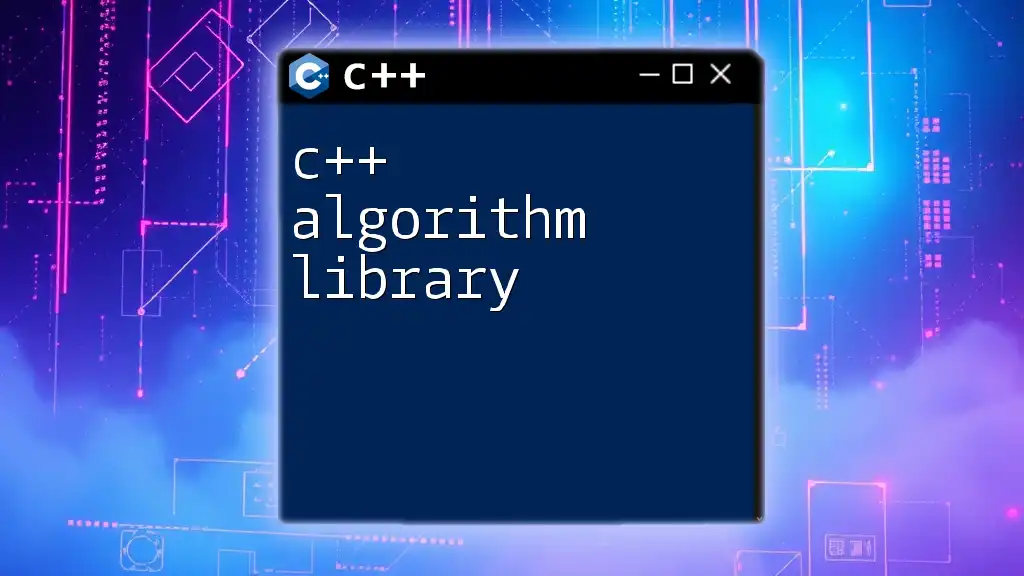
Types of Algorithms in C++
Search Algorithms
Searching algorithms are methods used to retrieve information stored within data structures. Let's delve into two popular searching algorithms:
Linear Search
Linear search is the simplest searching algorithm, iterating through each element in a collection until it finds the target value.
Code snippet of Linear Search:
int linearSearch(int arr[], int size, int target) {
for (int i = 0; i < size; i++) {
if (arr[i] == target) {
return i; // Found the target
}
}
return -1; // Target not found
}
Example Usage:
int main() {
int arr[] = {3, 1, 4, 1, 5, 9};
int target = 4;
int index = linearSearch(arr, 6, target);
if (index != -1) {
std::cout << "Target found at index: " << index << std::endl;
} else {
std::cout << "Target not found." << std::endl;
}
}
Binary Search
Binary search is a more efficient algorithm but requires the input array to be sorted. It divides the array into halves and significantly reduces the search space.
Code snippet of Binary Search:
int binarySearch(int arr[], int size, int target) {
int left = 0, right = size - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid; // Found the target
}
if (arr[mid] < target) {
left = mid + 1; // Search right half
} else {
right = mid - 1; // Search left half
}
}
return -1; // Target not found
}
Example Usage:
int main() {
int arr[] = {1, 1, 3, 4, 5, 9}; // Sorted array
int target = 5;
int index = binarySearch(arr, 6, target);
if (index != -1) {
std::cout << "Target found at index: " << index << std::endl;
} else {
std::cout << "Target not found." << std::endl;
}
}
Sorting Algorithms
Sorting algorithms are crucial for organizing data to ensure efficient access and retrieval. Some notable algorithms include:
Bubble Sort
Bubble sort repeatedly steps through the list, compares adjacent items, and swaps them if they are in the wrong order. This process is repeated until no swaps are needed.
Code snippet of Bubble Sort:
void bubbleSort(int arr[], int size) {
for (int i = 0; i < size - 1; i++) {
for (int j = 0; j < size - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
std::swap(arr[j], arr[j + 1]); // Swap elements
}
}
}
}
Example Usage:
int main() {
int arr[] = {5, 4, 3, 2, 1};
int size = sizeof(arr) / sizeof(arr[0]);
bubbleSort(arr, size);
// Output sorted array
for (int i : arr) std::cout << i << ' '; // Print sorted array
}
Quick Sort
This is an efficient sorting algorithm that employs a divide-and-conquer strategy. It selects a 'pivot' and partitions the array around it, sorting the elements recursively.
Code snippet of Quick Sort:
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pivot = partition(arr, low, high);
quickSort(arr, low, pivot - 1);
quickSort(arr, pivot + 1, high);
}
}
int partition(int arr[], int low, int high) {
int pivot = arr[high]; // Choosing the last element as pivot
int i = (low - 1); // Index of smaller element
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
std::swap(arr[i], arr[j]); // Swap elements
}
}
std::swap(arr[i + 1], arr[high]); // Put pivot in correct position
return (i + 1);
}
Example Usage:
int main() {
int arr[] = {10, 7, 8, 9, 1, 5};
int size = sizeof(arr) / sizeof(arr[0]);
quickSort(arr, 0, size - 1);
// Output sorted array
for (int i : arr) std::cout << i << ' '; // Print sorted array
}
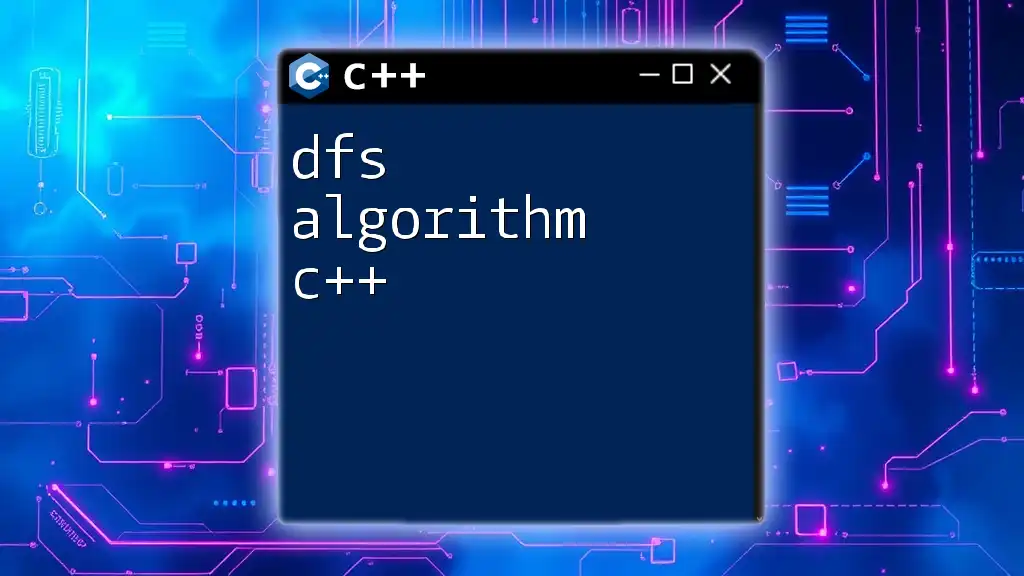
Algorithms in C++: Data Structures
Understanding the Link Between Algorithms and Data Structures
Data structures are essential for organizing and managing data efficiently, and algorithms are employed to perform operations on these data structures. Choosing the right data structure can result in significant performance improvements.
Example Data Structures and Their Algorithms
Arrays
Arrays are fundamental data structures that allow the storage of elements in contiguous memory locations. Algorithms like searching and sorting are often implemented using arrays due to their random access property.
Linked Lists
Linked lists consist of nodes, where each node contains data and a pointer to the next node. Algorithms for insertion, deletion, and traversal are commonly implemented using linked lists.
Trees
Tree structures are non-linear data structures that allow hierarchical storage of data. Algorithms related to trees include traversal algorithms such as pre-order, in-order, and post-order, which are vital for searching and accessing data efficiently.
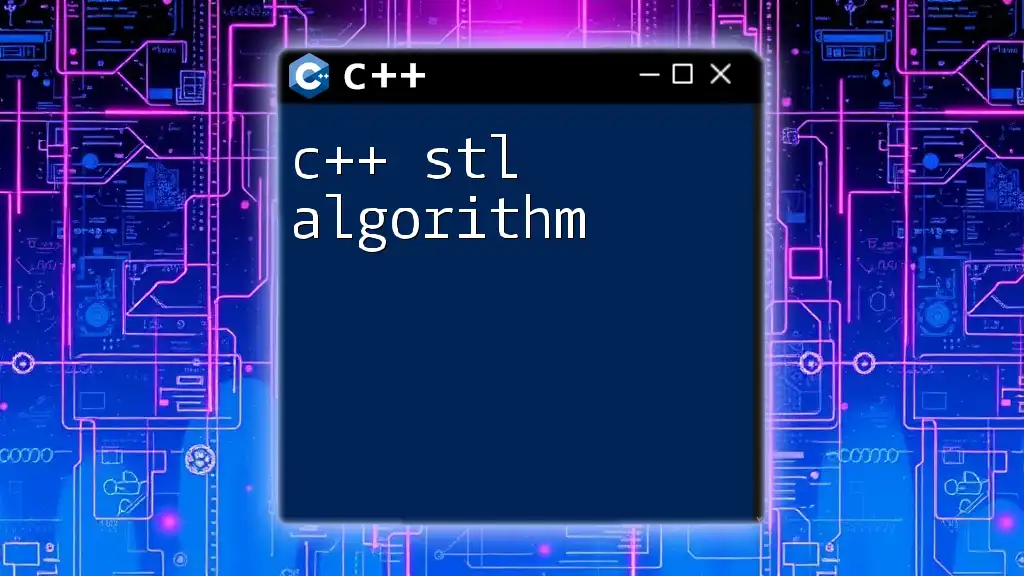
Analyzing Algorithm Efficiency
Time Complexity
Understanding the efficiency of an algorithm is crucial for selecting the right one for your application. Time complexity measures the amount of time an algorithm takes to complete as a function of the length of the input.
Big O Notation
Big O notation is a mathematical expression that describes the upper limit of an algorithm's runtime, providing insights into worst-case scenarios. For instance, the time complexity of linear search is O(n), while that of binary search is O(log n) — highlighting the efficiency of binary search over linear search in sorted collections.
Space Complexity
Space complexity analyzes the total memory space required by an algorithm, including both the space needed for input values and auxiliary space. Developers must balance time and space complexity; an efficient algorithm should ideally use minimal space without sacrificing performance.
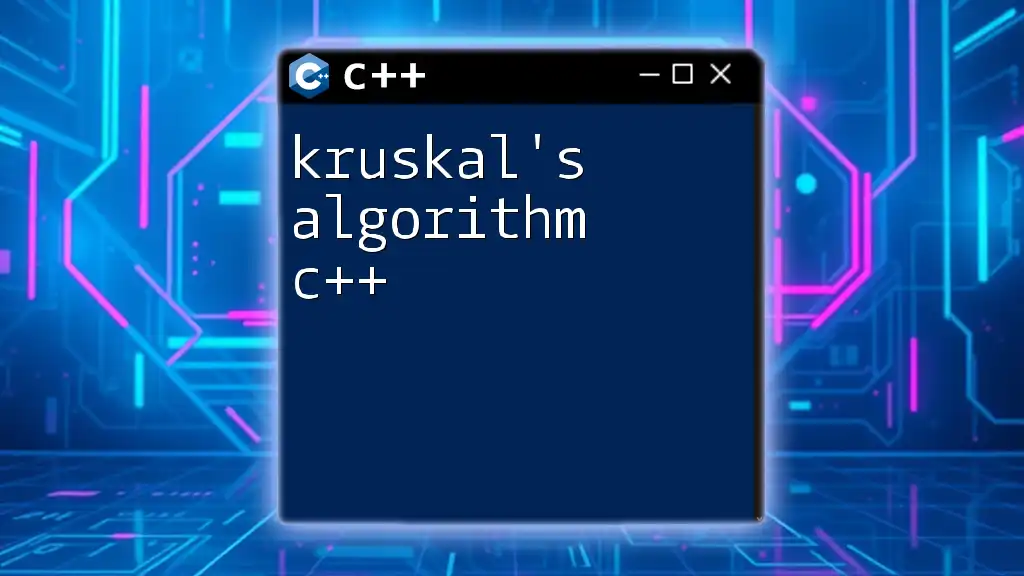
Practical Applications of C++ Algorithms
C++ algorithms are employed in various domains including software engineering, data processing, cryptography, AI, and many more. Real-world applications commonly involve sorting data for efficient retrieval, searching databases, and building complex data structures, all of which rely on effective C++ algorithms.
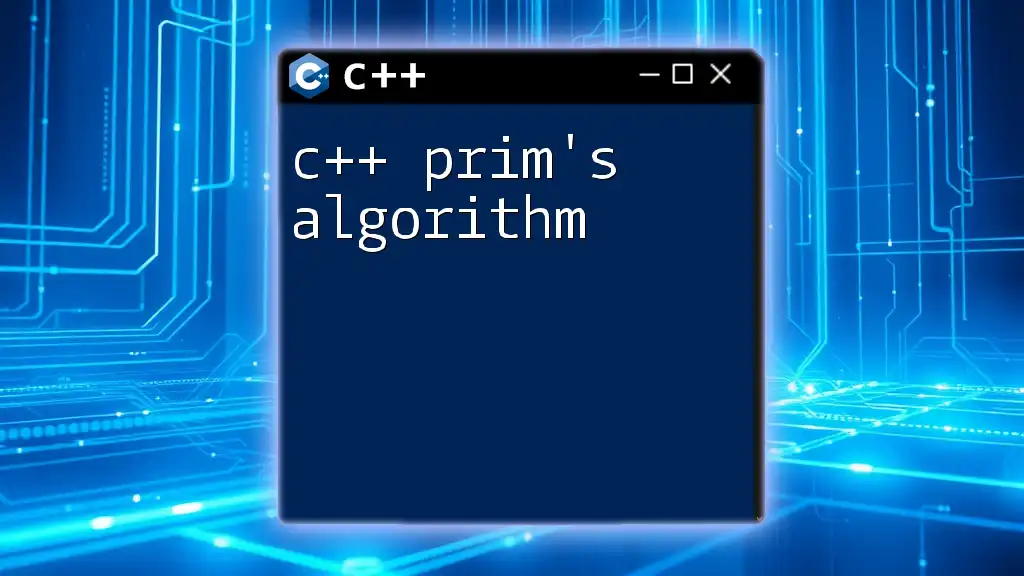
Best Practices for Implementing Algorithms in C++
To ensure your algorithms are efficient, consider these best practices:
- Understand the problem: Before jumping into coding, ensure you thoroughly understand the problem statement and requirements.
- Choose the right data structure: The selection of data structures significantly affects the efficiency of the algorithm.
- Optimize for performance and readability: Write clear and concise code, and comment where necessary to improve maintainability.
- Test and analyze: Implement unit tests to verify the functionality and analyze the performance of your algorithms to refine them.
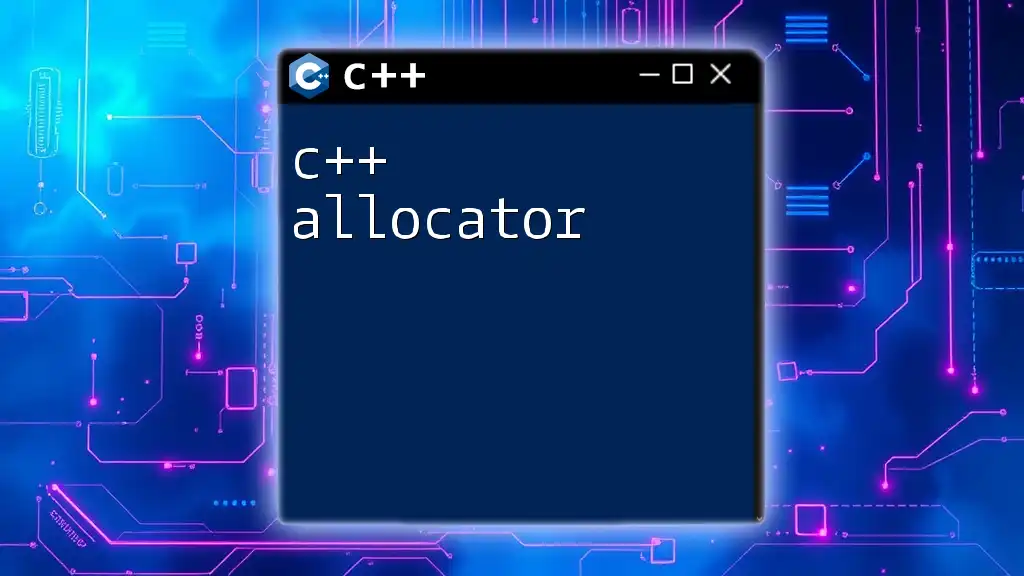
Conclusion
Mastering C++ algorithms is essential for developing high-performance applications. Understanding various types of algorithms, their efficiencies, and their practical applications will significantly enhance your programming skills. Encourage exploration and practice with numerous algorithms to build a strong foundation in C++. For those eager to advance, there are numerous resources available through books, online tutorials, and community forums.