Sorting algorithms in C++ are methods used to rearrange elements in a specific order, such as ascending or descending, with a common example being the Quick Sort algorithm. Here's a simple implementation of Quick Sort in C++:
#include <iostream>
#include <vector>
void quickSort(std::vector<int>& arr, int low, int high) {
if (low < high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
std::swap(arr[i], arr[j]);
}
}
std::swap(arr[i + 1], arr[high]);
int pi = i + 1;
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
int main() {
std::vector<int> arr = {10, 7, 8, 9, 1, 5};
int n = arr.size();
quickSort(arr, 0, n - 1);
for (int x : arr) std::cout << x << " ";
return 0;
}
What are Sorting Algorithms?
Sorting algorithms are systematic methods used to arrange the elements of a data structure, often an array or list, in a specific order—typically ascending or descending. Sorting is fundamental in computer science, as it enables efficient data retrieval and processing. Various sorting algorithms cater to different scenarios, each with unique advantages and drawbacks.
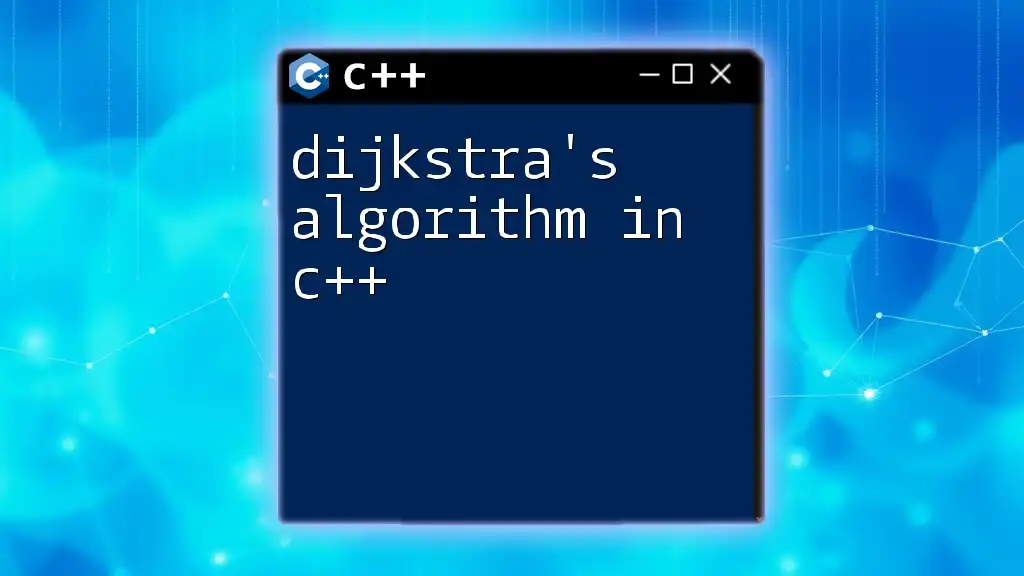
Why Learn Sorting Algorithms in C++?
Understanding sorting algorithms in C++ is crucial for several reasons:
-
Performance Optimization: Different sorting techniques excel under varying conditions. Knowing which to use can significantly enhance performance.
-
Foundation of Data Structures: Many advanced data handling techniques rest on solid sorting foundations.
-
Real-World Applications: Sorting methods are widely used in algorithms like search functions, database management, and even in user interfaces.
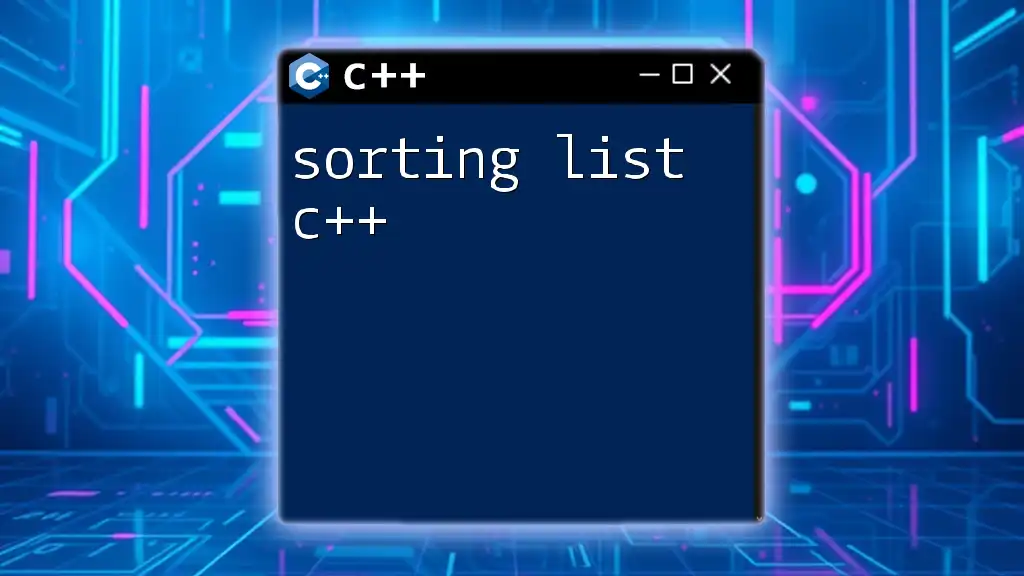
Overview of Common C++ Sorting Algorithms
Sorting algorithms can be broadly categorized into comparison-based and non-comparison-based algorithms. Each category encompasses various sorting methods that have been optimized for different types of data and use cases.
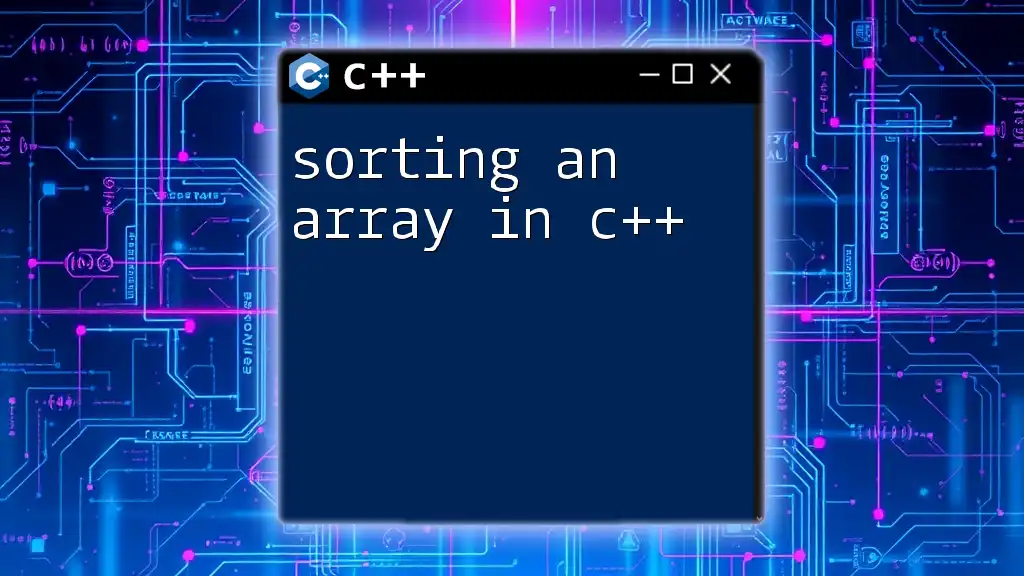
Comparison-based Sorting Algorithms
Bubble Sort
Bubble Sort is one of the simplest sorting algorithms. It repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. Its name comes from the way larger elements "bubble" to the top of the list.
Time Complexity:
- Best Case: \(O(n)\)
- Average Case: \(O(n^2)\)
- Worst Case: \(O(n^2)\)
Code Example:
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n-1; i++)
for (int j = 0; j < n-i-1; j++)
if (arr[j] > arr[j+1])
swap(arr[j], arr[j+1]);
}
Pros and Cons: Pros:
- Simple to understand and implement.
Cons:
- Inefficient for large datasets, making it unsuitable for production use in most cases.
Selection Sort
Selection Sort improves on the Bubble Sort by selecting the minimum element from the unsorted portion and moving it to the sorted portion.
Time Complexity:
- Best Case: \(O(n^2)\)
- Average Case: \(O(n^2)\)
- Worst Case: \(O(n^2)\)
Code Example:
void selectionSort(int arr[], int n) {
for (int i = 0; i < n-1; i++) {
int min_idx = i;
for (int j = i+1; j < n; j++)
if (arr[j] < arr[min_idx])
min_idx = j;
swap(arr[min_idx], arr[i]);
}
}
Pros and Cons: Pros:
- Performs well on small datasets.
Cons:
- Inefficient on larger lists, and it does not adapt as the dataset becomes large.
Insertion Sort
Insertion Sort constructs a sorted array (or list) one item at a time. It maintains a sublist of sorted items and continuously inserts new items into that sublist.
Time Complexity:
- Best Case: \(O(n)\)
- Average Case: \(O(n^2)\)
- Worst Case: \(O(n^2)\)
Code Example:
void insertionSort(int arr[], int n) {
for (int i = 1; i < n; i++) {
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
Pros and Cons: Pros:
- Efficient for small datasets and partially sorted arrays.
Cons:
- Inefficient for large arrays.
Merge Sort
Merge Sort employs a divide-and-conquer strategy. The algorithm divides the input array into two halves, sorts the halves, and then merges them back together.
Time Complexity:
- Best Case: \(O(n \log n)\)
- Average Case: \(O(n \log n)\)
- Worst Case: \(O(n \log n)\)
Code Example:
void merge(int arr[], int left, int mid, int right) {
// Merge two subarrays
}
void mergeSort(int arr[], int left, int right) {
if (left < right) {
int mid = left + (right - left) / 2;
mergeSort(arr, left, mid);
mergeSort(arr, mid + 1, right);
merge(arr, left, mid, right);
}
}
Pros and Cons: Pros:
- Efficient and stable performance, even on large datasets.
Cons:
- Requires extra space for temporary arrays.
Quick Sort
Quick Sort is also a divide-and-conquer algorithm, but it sorts by selecting a "pivot" element from the array and partitioning the other elements into two subarrays.
Time Complexity:
- Best Case: \(O(n \log n)\)
- Average Case: \(O(n \log n)\)
- Worst Case: \(O(n^2)\) (occurs when the smallest or largest element is always picked as the pivot)
Code Example:
int partition(int arr[], int low, int high) {
// Partition logic
}
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
Pros and Cons: Pros:
- Generally faster than other \(O(n \log n)\) algorithms like Merge Sort or Heap Sort.
Cons:
- Worst-case performance can be poor without good pivot choice.
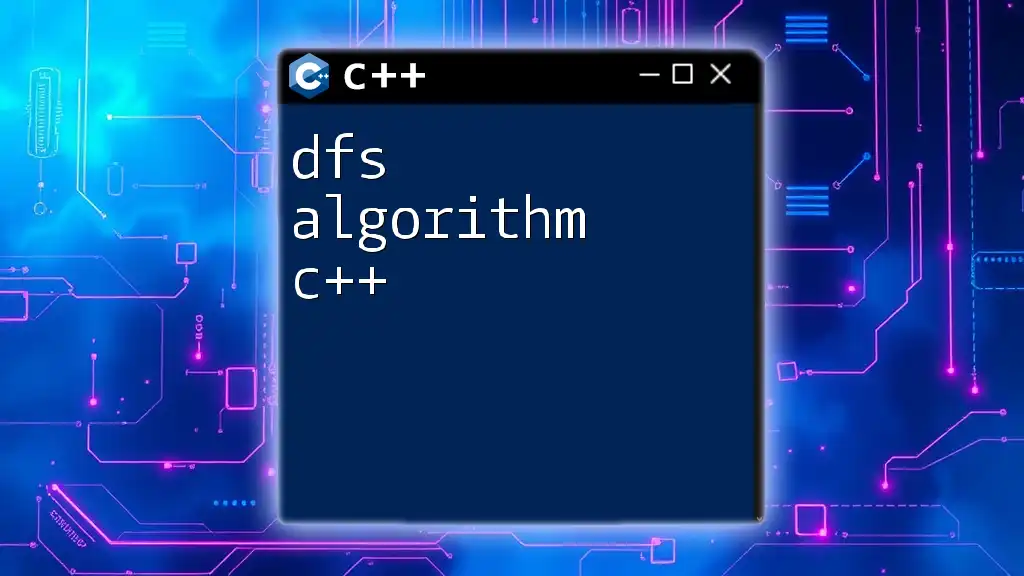
Non-comparison-based Sorting Algorithms
Counting Sort
Counting Sort counts the occurrences of each unique element and determines their positions in the sorted output. It is notably effective when the range of the input data is known.
Time Complexity:
- Best, Average, and Worst Case: \(O(n + k)\), where \(k\) is the range of the input.
Code Example:
void countingSort(int arr[], int n, int range) {
// Initialize count array and sort logic
}
Pros and Cons: Pros:
- Extremely fast for small ranges, often outperforming comparison-based algorithms.
Cons:
- Not suitable for large ranges or for input with floating-point numbers.
Radix Sort
Radix Sort sorts numbers digit by digit, starting from the least significant digit to the most significant digit. It employs a stable sub-sorting algorithm like Counting Sort to sort the numbers.
Time Complexity:
- Best, Average, and Worst Case: \(O(nk)\), where \(k\) is the number of digits in the largest number.
Code Example:
void radixSort(int arr[], int n) {
// Radix sort logic
}
Pros and Cons: Pros:
- Efficient for sorting large sets of integers and can handle datasets where numbers don't vary much in length.
Cons:
- Requires additional space for the counting base, making it less efficient in space-constrained environments.
Bucket Sort
Bucket Sort distributes elements into buckets, each of which is then sorted individually, either using a different sorting algorithm or recursively applying the bucket sort.
Time Complexity:
- Best Case: \(O(n)\)
- Average Case: \(O(n + n^2/k)\), where \(k\) is the number of buckets.
- Worst Case: \(O(n^2)\)
Code Example:
void bucketSort(float arr[], int n) {
// Logic for bucket sort
}
Pros and Cons: Pros:
- Particularly powerful when the input is uniformly distributed over a range.
Cons:
- Performance can degrade significantly if the distribution is not uniform.
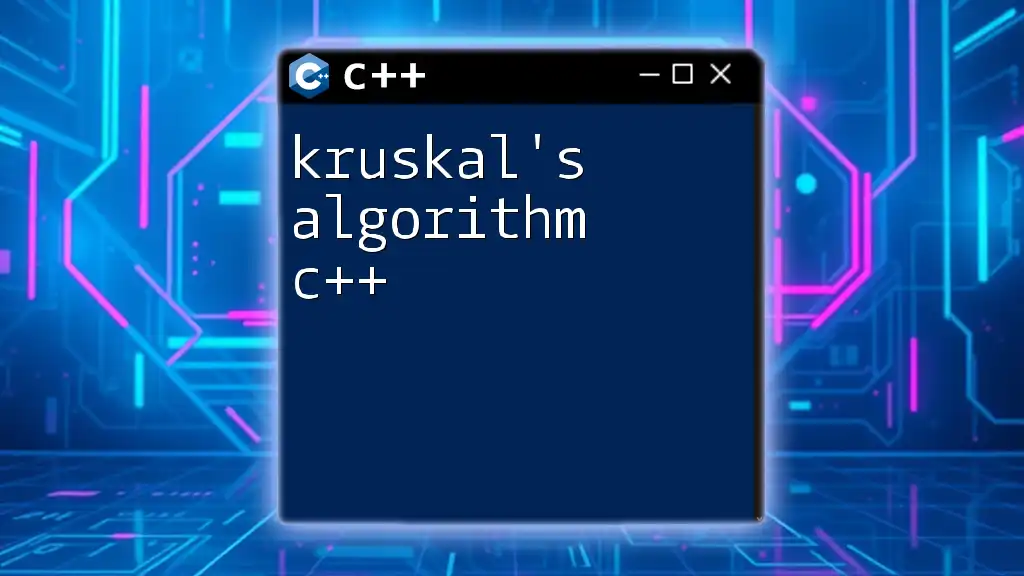
Choosing the Right Sorting Algorithm
When selecting a sorting algorithm, consider the following factors:
-
Dataset Size: Small array? Consider Insertion Sort. Large array? Think Merge or Quick Sort.
-
Data Characteristics: Is the data partially sorted? Insertion Sort may work efficiently.
-
Performance Requirements: Need a stable sort? Merge Sort could be your best choice.
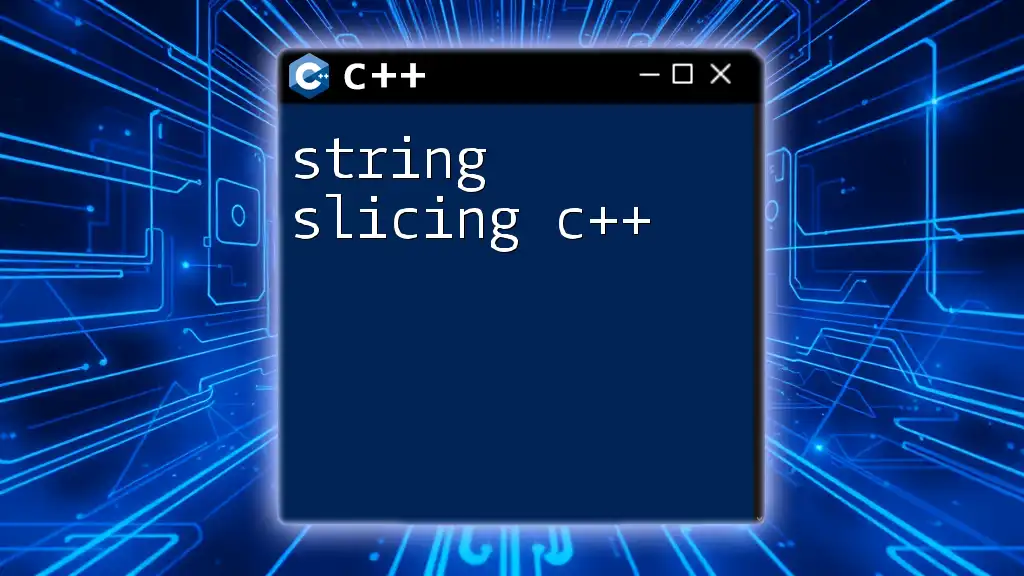
Conclusion
Sorting algorithms in C++ serve as critical tools for data management and organization in programming. From basic methods like Bubble and Selection Sort to more advanced techniques like Quick and Merge Sort, each algorithm has its importance and application. By mastering these algorithms, you position yourself to tackle a variety of challenges in data handling and analysis.
As you grow more comfortable with these sorting techniques, experiment with various datasets to see firsthand how each algorithm performs, and always stay curious for further learning opportunities!