In C++, you can reverse a string using the `std::reverse` function from the `<algorithm>` header, which modifies the string in place. Here's a simple example:
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string str = "Hello, World!";
std::reverse(str.begin(), str.end());
std::cout << str << std::endl; // Output: !dlroW ,olleH
return 0;
}
What is a String in C++?
In C++, a string is a sequence of characters used to represent text. C++ provides the `std::string` class as part of its Standard Library, which offers various features for string manipulation. Unlike character arrays, `std::string` manages memory automatically, enabling dynamic resizing and easier text processing.
Understanding strings is crucial since they are foundational in software development, from user input to data storage and manipulation. Mastering string handling can significantly enhance your C++ programming skills.
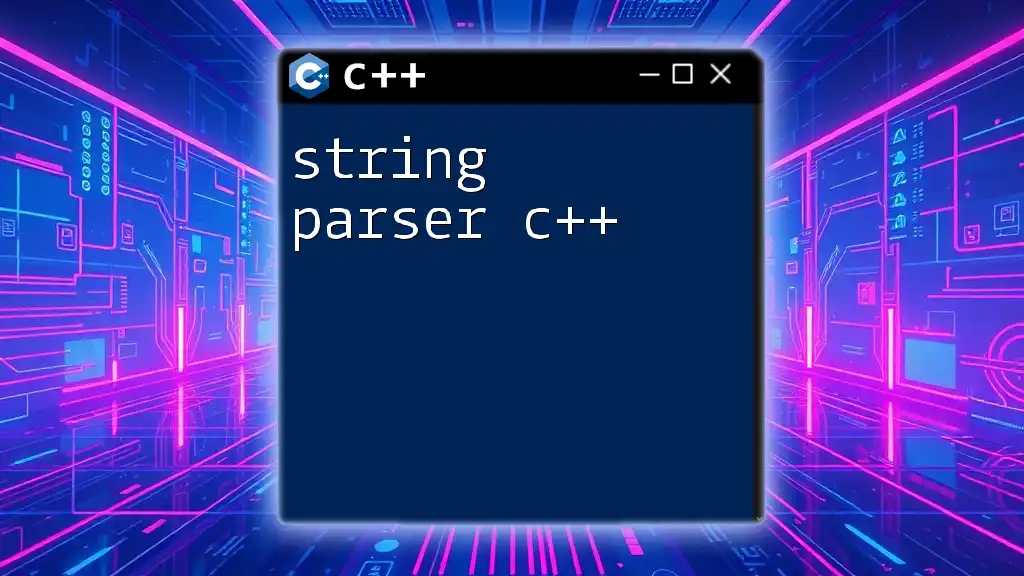
Why Reverse a String?
Reversing a string serves various practical purposes:
- Palindrome checks: To determine if a string reads the same backward as forward.
- Data formatting: Sometimes, strings need to be displayed in reverse order for user interfaces or logging purposes.
- Data processing: In algorithms, reversing strings can be a common operation when manipulating text, like in certain encryption techniques.
These scenarios illustrate why knowing how to reverse a string in C++ is a valuable skill for any programmer.
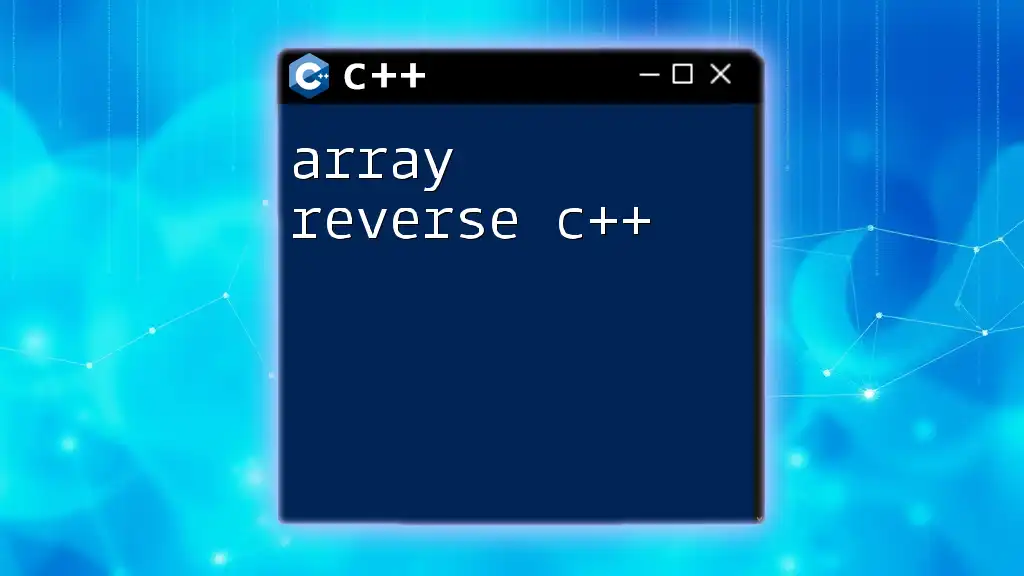
Different Methods to Reverse a String in C++
Using C++ Standard Library Functions
C++ offers powerful tools through its Standard Library, including the `reverse` function found in the `<algorithm>` header. This method is incredibly efficient and concise, making it a preferred approach in many cases.
Using the `reverse` function is simple:
#include <iostream>
#include <algorithm>
#include <string>
int main() {
std::string str = "Hello World";
std::reverse(str.begin(), str.end());
std::cout << "Reversed String: " << str << std::endl;
return 0;
}
In this code, `std::reverse` takes two iterators—`str.begin()` and `str.end()`—indicating the range to reverse. This method operates in-place, meaning it doesn't create a new string but modifies the original one. The main advantages here are simplicity and speed, while a key consideration is that it relies on the underlying implementation of the Standard Library.
Using a Traditional Loop
For those who prefer a more manual approach, a traditional loop can be employed to reverse a string. This method gives you a finer level of control and is beneficial for educational purposes.
Here's how it works:
#include <iostream>
#include <string>
std::string reverseString(const std::string &str) {
std::string reversed;
for (int i = str.size() - 1; i >= 0; --i) {
reversed += str[i];
}
return reversed;
}
int main() {
std::string str = "Hello World";
std::cout << "Reversed String: " << reverseString(str) << std::endl;
return 0;
}
This code snippet demonstrates reversing a string by iterating from the end of the string to the beginning, appending each character to a new string. While this method is intuitive, it's essential to consider its time complexity of \(O(n)\) and the additional space complexity since it creates a new string.
Using Recursion
Utilizing recursion can also effectively reverse a string. This approach emphasizes a foundational programming concept whereby a function calls itself.
Here's a recursive solution:
#include <iostream>
#include <string>
std::string reverseString(const std::string &str) {
if (str.empty()) return ""; // base case
return str.back() + reverseString(str.substr(0, str.size() - 1));
}
int main() {
std::string str = "Hello World";
std::cout << "Reversed String: " << reverseString(str) << std::endl;
return 0;
}
In this example, the base case returns an empty string when the input string is empty. The function constructs the reversed string by concatenating the last character of the string with the reversed substring. Though elegant, be cautious about the memory overhead associated with function calls and the creation of substrings.
Using Stack Data Structure
Another interesting method for reversing a string is by utilizing a stack. This approach leverages the LIFO (Last In, First Out) nature of stacks to reverse the order of characters.
Here’s how it’s done:
#include <iostream>
#include <stack>
#include <string>
std::string reverseString(const std::string &str) {
std::stack<char> stack;
for (char ch : str) {
stack.push(ch);
}
std::string reversed;
while (!stack.empty()) {
reversed += stack.top();
stack.pop();
}
return reversed;
}
int main() {
std::string str = "Hello World";
std::cout << "Reversed String: " << reverseString(str) << std::endl;
return 0;
}
In this code, each character of the string is pushed onto the stack. Once all characters are stored, the reversed string is constructed by popping characters from the stack. This method is particularly useful for visualizing how data can be manipulated using data structures; however, it does entail an additional space complexity of \(O(n)\) due to the stack.
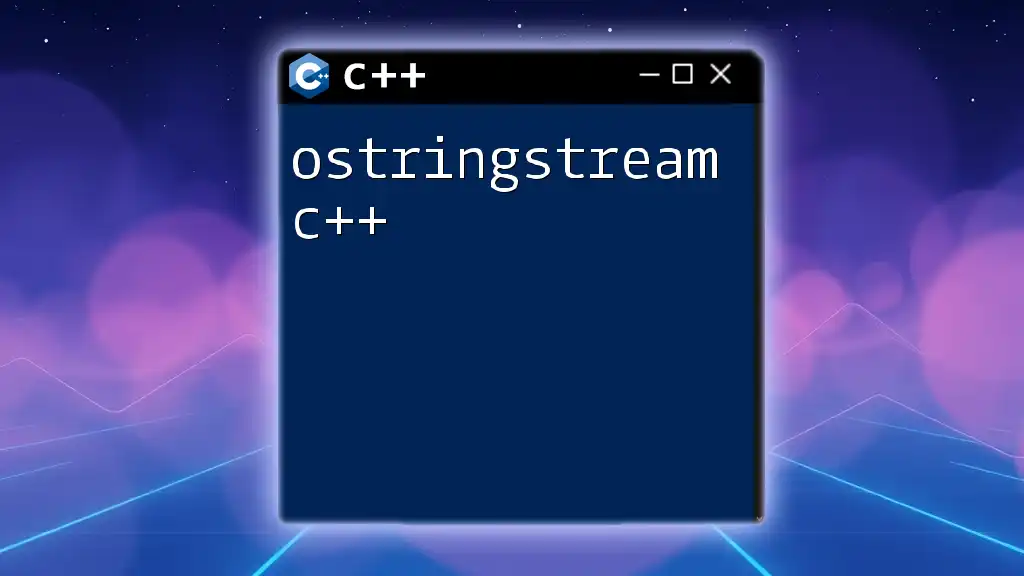
Performance Considerations
When evaluating the different methods to reverse a string in C++, considering the time and space complexity is crucial:
- Standard Library Function: Time complexity is \(O(n)\), and space complexity is \(O(1)\) since it reverses in place.
- Traditional Loop: Both time and space complexity are \(O(n)\).
- Recursion: Time complexity is \(O(n)\), but space complexity is \(O(n)\) due to the call stack.
- Stack Approach: Time complexity remains \(O(n)\), while space complexity is also \(O(n)\) because of stack use.
Understanding these complexities helps developers make informed decisions about which method to use based on the application's requirements.
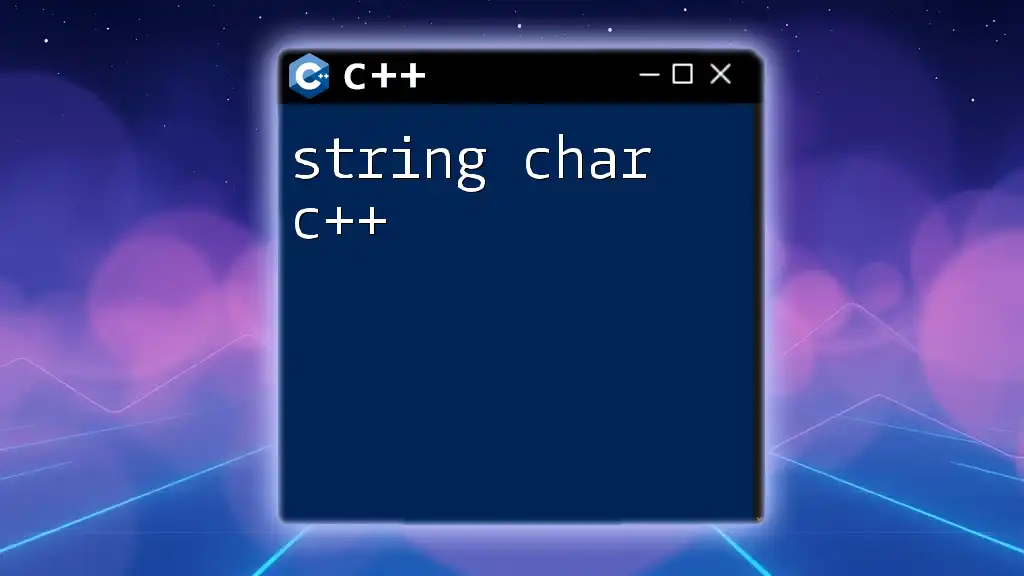
Common Mistakes to Avoid
While reversing a string might seem straightforward, several common pitfalls can arise:
- Failing to handle empty strings: Ensure your code gracefully handles this edge case.
- Memory leaks: Pay attention to dynamically allocated memory, especially when using manual approaches versus STL.
- Assuming immutability of strings: Remember that modifying a string requires understanding how C++ manages memory.
By being aware of these common mistakes, you can enhance the reliability of your code.
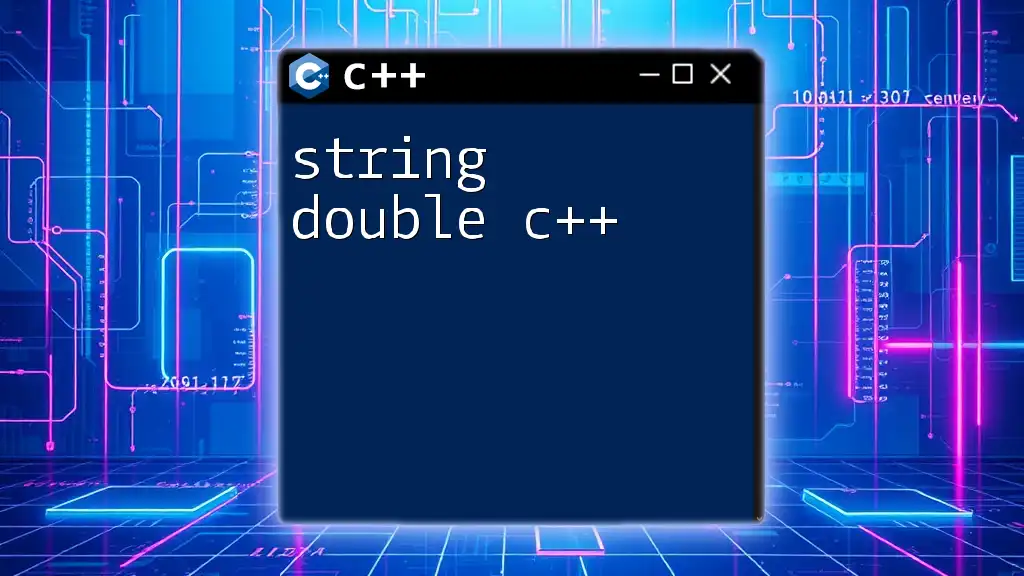
Conclusion
In summary, string reverse in C++ can be accomplished through various methods, each with its advantages and disadvantages. From leveraging the Standard Library to using recursion or data structures like stacks, the options are adaptable to different contexts and needs. By mastering string manipulation, including reversal, you'll not only improve your coding competency but also prepare yourself for more complex challenges ahead.
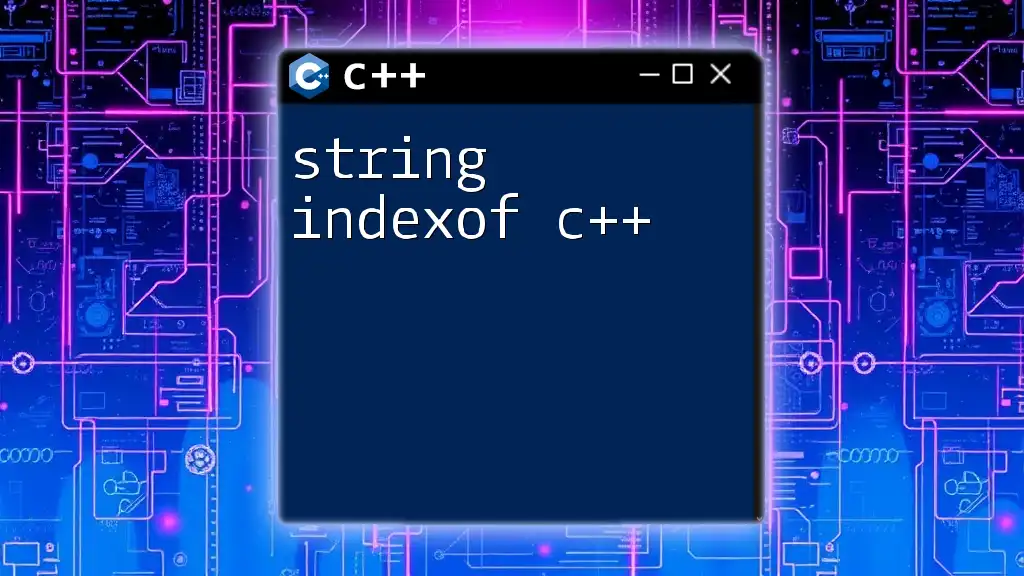
Further Reading and Resources
For more in-depth learning about C++ strings, consider exploring additional resources such as online tutorials, official documentation, and coding practice platforms. Engaging in practical projects will further solidify your knowledge of string manipulation, enhancing both your technical skills and problem-solving abilities.