In C++, a string is a sequence of characters used to represent textual data, which can be manipulated using the `std::string` class from the Standard Library.
Here’s a simple example of how to use strings in C++:
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello, World!";
std::cout << greeting << std::endl;
return 0;
}
Understanding Strings in C++
What is a String?
In C++, a string is a sequence of characters used to represent text. Strings can be categorized into two types: C-style strings and C++ strings (std::string).
C-style strings are essentially arrays of characters ending with a null terminator (`\0`). For example:
char str[] = "Hello";
On the other hand, C++ strings are implemented as the `std::string` class from the Standard Template Library (STL). This class offers a more robust and user-friendly way to handle strings, with built-in memory management and useful methods for string manipulation.
Why Use std::string?
Using `std::string` instead of C-style strings provides several advantages:
- Memory Management: `std::string` automatically handles memory allocation and deallocation, reducing the risk of memory leaks.
- Dynamic Sizing: Unlike C-style strings, which require manual sizing, `std::string` can grow as needed.
- Rich Functionality: The `std::string` class includes numerous member functions that facilitate manipulation, comparison, and conversion.
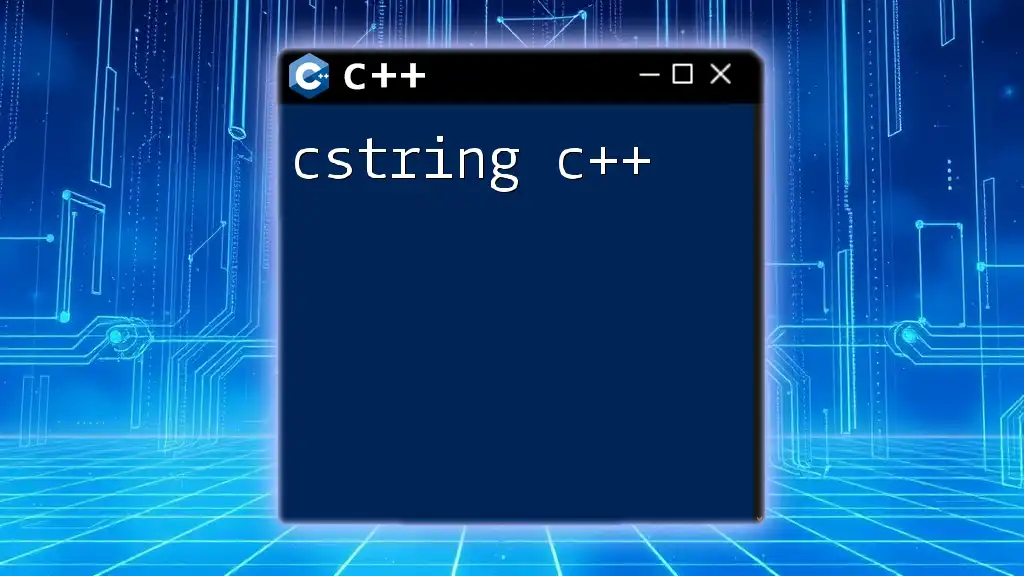
Creating Strings
Initializing a String
Strings can be initialized in various ways:
-
Using String Literals:
std::string str1 = "Hello, World!";
-
Using the String Constructor:
std::string str2("Hello, World!");
These methods create a string object that can be used throughout your program.
Assigning Values to Strings
You can easily assign new values to a string after its creation using the assignment operator:
std::string str = "Initial value";
str = "New value";
This method of string assignment allows for dynamic text updates throughout your code.
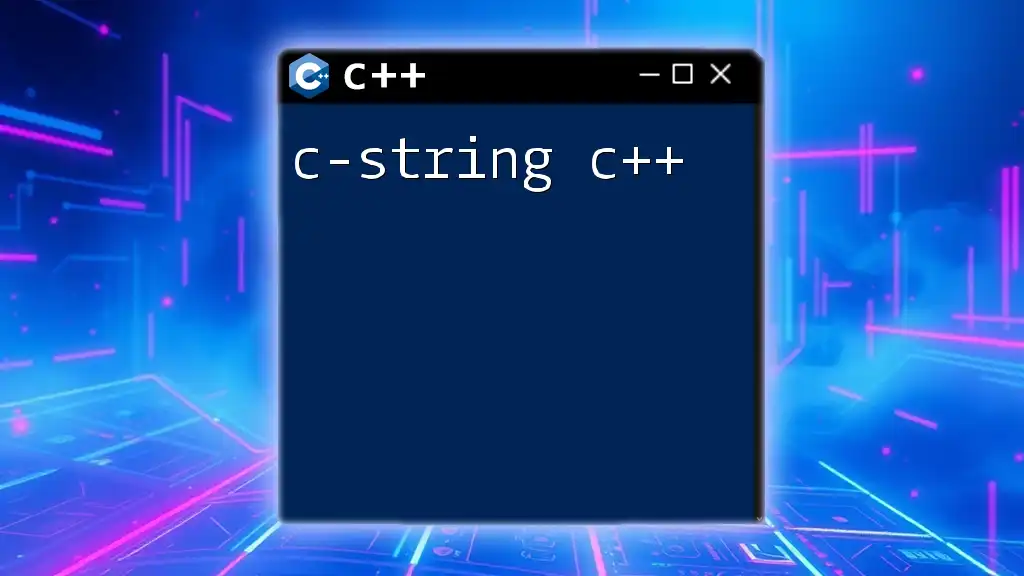
String Operations
Concatenation
Concatenating two or more strings can be done effortlessly using the `+` operator:
std::string str1 = "Hello, ";
std::string str2 = "World!";
std::string result = str1 + str2; // Result: "Hello, World!"
You can also use the `append()` method to achieve the same result:
str1.append(str2);
Length and Size
To determine the number of characters in a string, you can utilize the `length()` or `size()` methods:
std::string str = "Hello";
std::cout << str.length(); // Outputs: 5
Both `length()` and `size()` return the same value, representing the count of characters in the string.
Accessing Characters
C++ strings allow you to access characters individually using the `[]` operator and the `at()` method:
std::string str = "Hello";
char firstChar = str[0]; // H
char secondChar = str.at(1); // e
While both methods work similarly, using `at()` provides bounds checking, throwing an exception if the index is out of range.
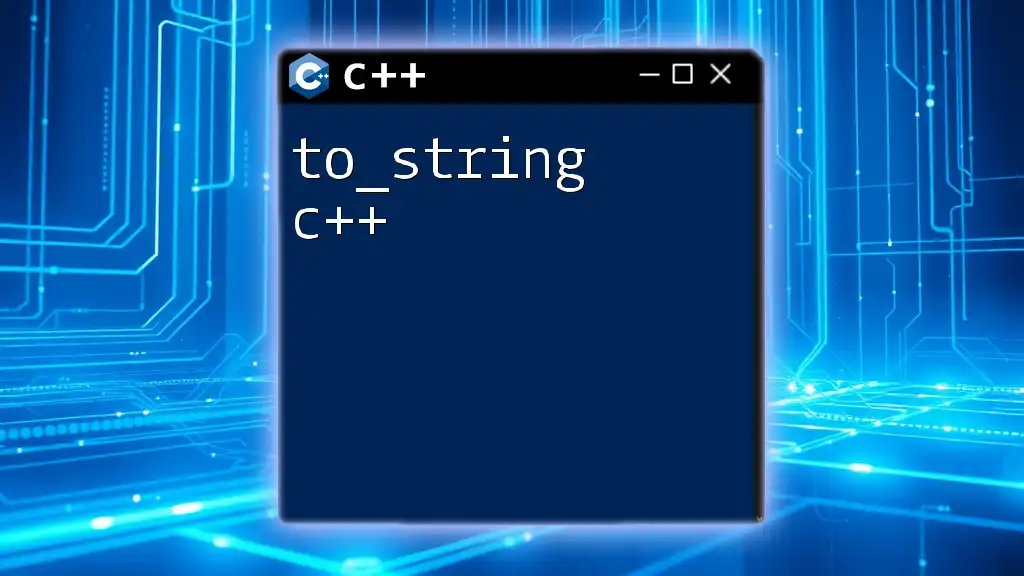
String Manipulation Methods
Inserting and Erasing
You can insert text into a string using the `insert()` method. For example:
std::string str = "Hello";
str.insert(5, " World"); // Now str is "Hello World"
To remove a portion of a string, the `erase()` method is utilized:
str.erase(5, 6); // Removes " World", str is now "Hello"
Replacing Substrings
To replace a portion of a string, `replace()` is the method to use:
str.replace(0, 5, "Hi"); // str is now "Hi"
This method allows for replacing a substring starting from a specified position for a given length.
Find and Replace
Finding substrings within a string is achievable with the `find()` method:
std::size_t pos = str.find("lo"); // Returns the position of "lo"
You can chain `find()` with `replace()` to update text based on specific conditions.
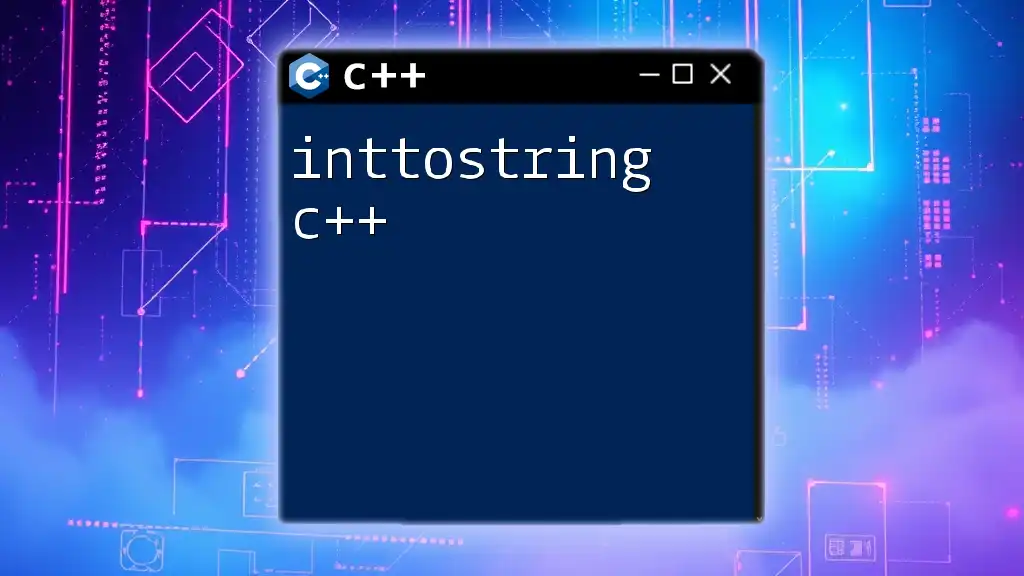
String Comparison
Comparison Operators
String comparison in C++ can leverage the following operators:
- `==` for equality
- `!=` for inequality
- `<`, `>`, `<=`, `>=` for lexicographical comparisons
For example:
if (str1 == str2) {
// Code to execute if strings are equal
}
Comparing with C-style Strings
When working with C-style strings, you can compare them using the `strcmp()` function:
if (strcmp(c_str1, c_str2) == 0) {
// C-style strings are equal
}
This function returns 0 when the strings are equal, which provides an easy way to compare them in combination with std::string.
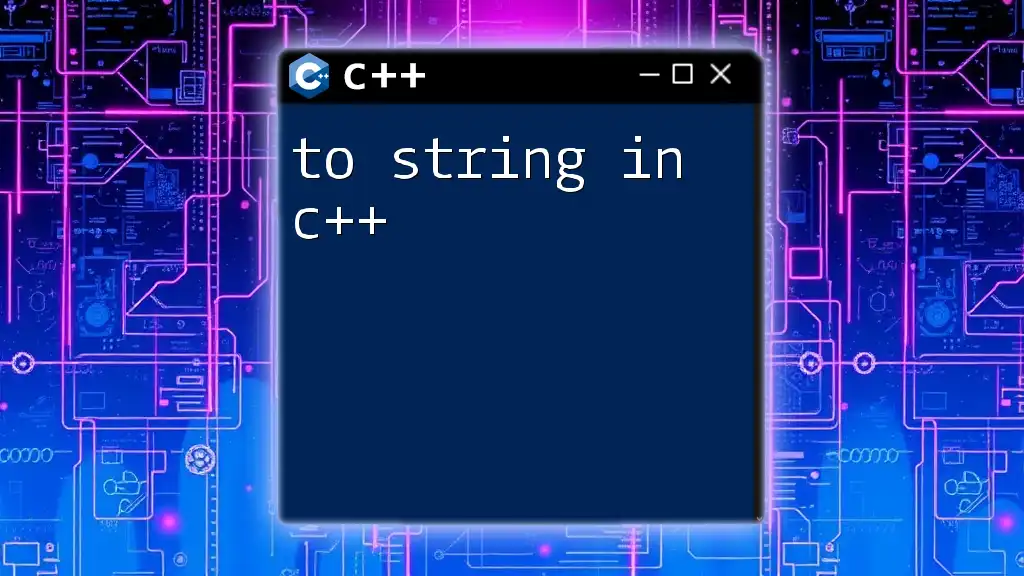
String Formatting
Using `std::to_string()`
To convert numeric types to strings, the `std::to_string()` function can be employed effectively:
int num = 100;
std::string strNum = std::to_string(num); // strNum is now "100"
This method is particularly useful for formatting numbers as strings for output or concatenation.
Using Streams
Formatting strings can also be achieved by using string streams (`std::stringstream`):
std::stringstream ss;
ss << "Number: " << num;
std::string formattedString = ss.str(); // formattedString is now "Number: 100"
This approach allows for complex string formatting with any type of data.
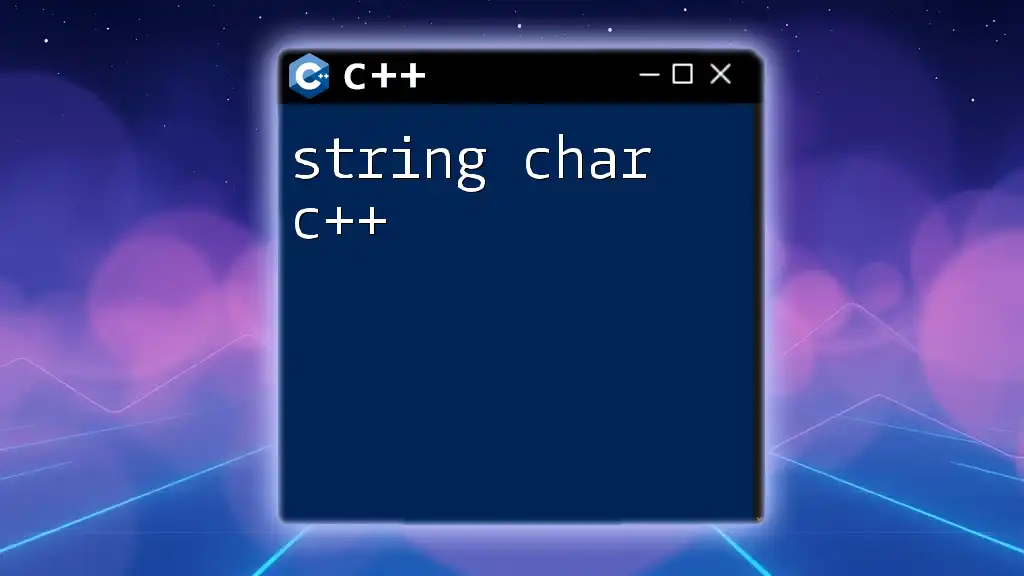
String Iterators
Using Iterators
The `std::string` class provides iterators that can be employed to traverse through the characters of the string. Here's an example of using iterators in a loop:
std::string str = "Hello";
for (auto it = str.begin(); it != str.end(); ++it) {
std::cout << *it; // Outputs each character in "Hello"
}
Using iterators allows for flexible traversing and manipulating string contents.
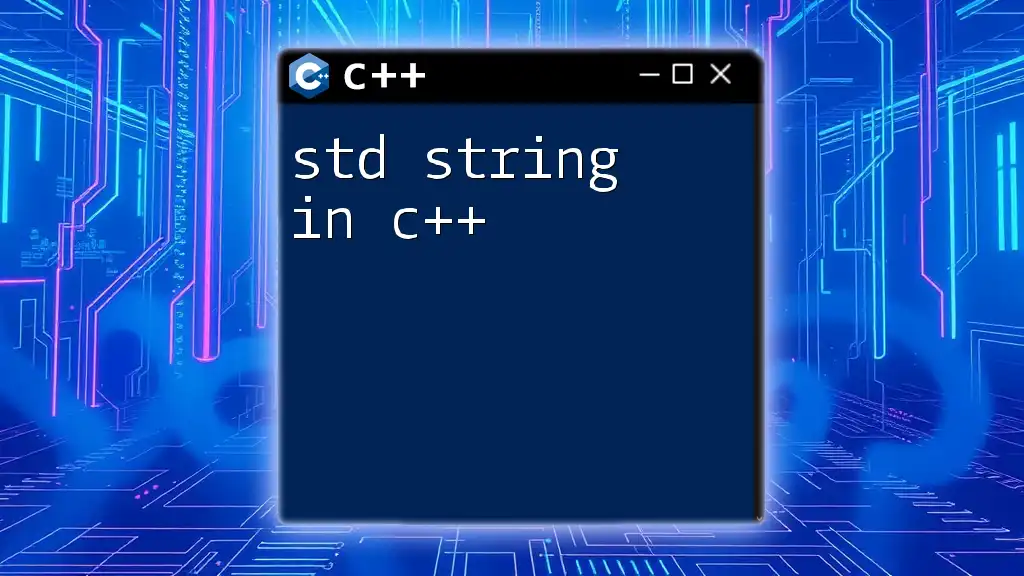
Common Challenges and Solutions
Handling Special Characters
When dealing with strings, especially user-generated input, special characters can pose challenges. In C++, these are represented using escape sequences such as `\"` for a double quote or `\\` for the backslash. Understanding and managing these characters is crucial for ensuring accurate string handling.
Memory Management
When manipulating large strings, it is essential to consider memory implications and potential performance bottlenecks. Optimizing string operations by avoiding unnecessary copying and using `reserve()` to allocate memory can significantly enhance performance in large-scale applications.
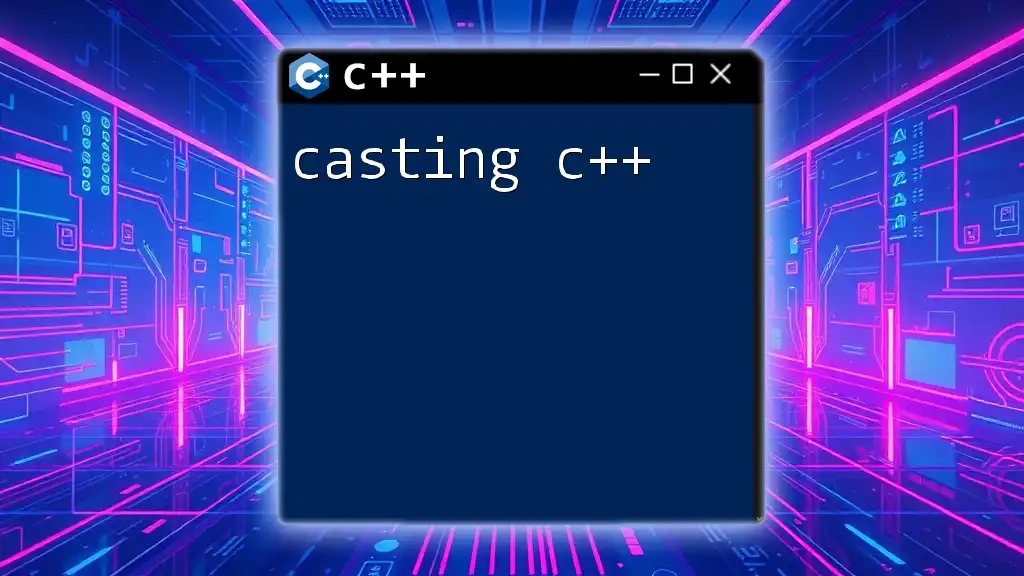
Conclusion
Mastering string manipulation in C++ is crucial for effective programming and can greatly enhance your coding legacies. Strings are integral to handling text data, and understanding their functionalities can make your code simpler and more robust. Practice these concepts to gain confidence and improve your facility with strings in C++.