In C++, an empty string can be created using the `std::string` class with no characters, which is often used to initialize strings or represent a lack of content.
#include <string>
std::string emptyString = ""; // Creating an empty string in C++
Understanding Strings in C++
What is a String?
In C++, a string is a sequence of characters that can hold text. The `std::string` class provides a flexible way to handle strings, allowing for dynamic resizing and operations on the character data. Unlike C-style strings, which use character arrays and are null-terminated, `std::string` manages its own memory, making it safer and easier to use.
Characteristics of C++ Strings
C++ strings are dynamic and can grow or shrink in size automatically based on the content they hold. This flexibility eliminates many common pitfalls associated with fixed-size C-style strings, such as buffer overflows or memory leaks. Also, because `std::string` manages memory internally, it simplifies string manipulation while providing functionality for string comparison, concatenation, and searching.
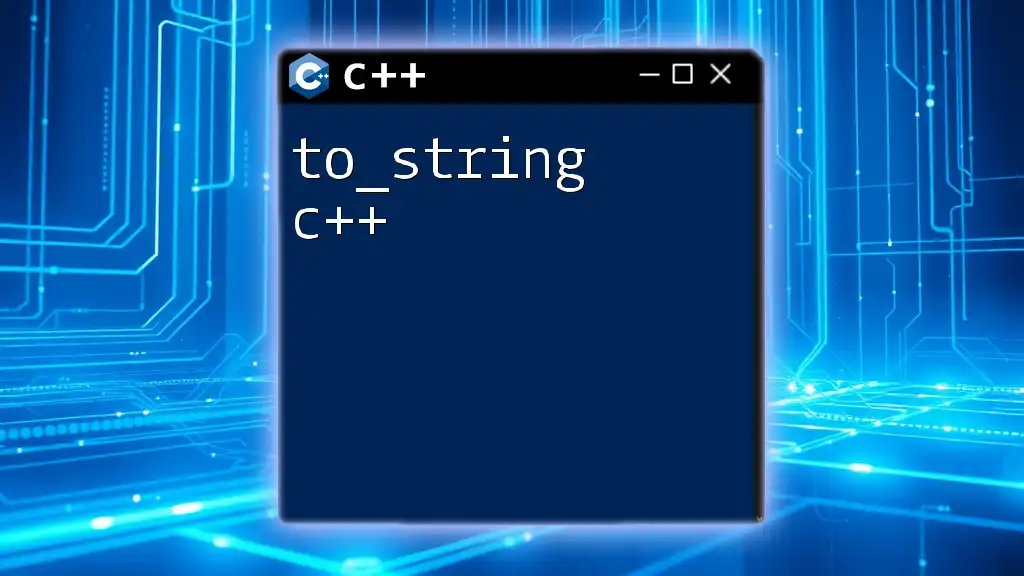
The Concept of an Empty String in C++
What is an Empty String?
An empty string in C++ is a string with no characters: it has a length of zero. It is important to distinguish an empty string from a null pointer (which refers to no string at all). The representation of an empty string is `""`, and its significance lies in how it can affect program logic and data handling.
Creation of an Empty String
An empty string can be easily created during the initialization of a `std::string` variable. Here’s how:
std::string emptyString = "";
In this example, `emptyString` is a valid string object, but it contains no characters. It's useful when you want to initialize a string variable but are not yet ready to assign it a meaningful value.
Checking if a String is Empty
Using `empty()` Method
One of the most straightforward ways to check if a string is empty is through the `empty()` method of the `std::string` class. This method returns a boolean value: `true` if the string is empty and `false` otherwise.
if (emptyString.empty()) {
std::cout << "The string is empty." << std::endl;
}
This method is efficient and directly communicates the intent of checking for emptiness.
Using `size()` or `length()` Method
Another way to determine if a string is empty is by checking its size using the `size()` or `length()` methods. Both methods yield the same result, returning the number of characters in the string. If the value is zero, the string is empty.
if (emptyString.size() == 0) {
std::cout << "The string is empty." << std::endl;
}
Both `size()` and `length()` are viable options, but it is essential to choose based on code readability and the specific context in which you are working.
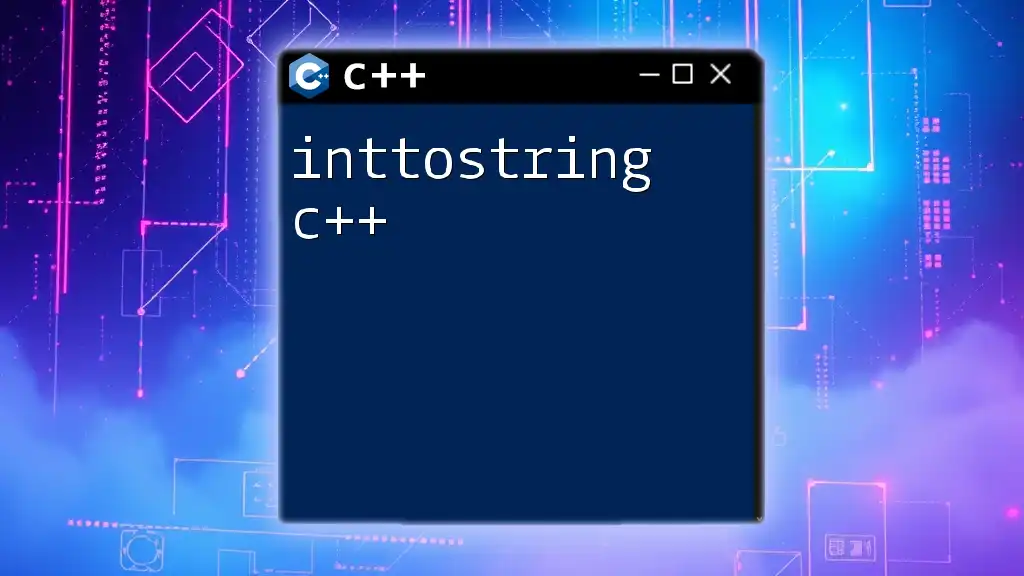
Practical Use Cases for Empty Strings
Input Validation
In many applications, especially those that handle user input, validating that a string is not empty is crucial. If an empty value is submitted where a string is expected, it may lead to unexpected behavior or software errors. Here’s a simple example:
std::string userInput;
std::cout << "Enter some text: ";
std::getline(std::cin, userInput);
if (userInput.empty()) {
std::cout << "No input provided." << std::endl;
}
This code checks if the user’s input is empty and provides feedback accordingly, ensuring that the program behaves correctly.
String Manipulation
Empty strings can also play a significant role in string manipulation. For instance, they can be concatenated, affecting the final string output without altering it.
std::string greeting = "Hello, " + emptyString + "World!";
std::cout << greeting << std::endl; // Outputs: Hello, World!
In this example, the empty string is seamlessly employed in a concatenation operation, demonstrating how it can be used without causing any disruption in string formation.
Data Structures and Algorithms
Empty strings can serve as sentinel values in data structures like queues or stacks, indicating an empty state or the end of data input. This can simplify logic during push/pop operations and facilitate clearer conditions in algorithms.
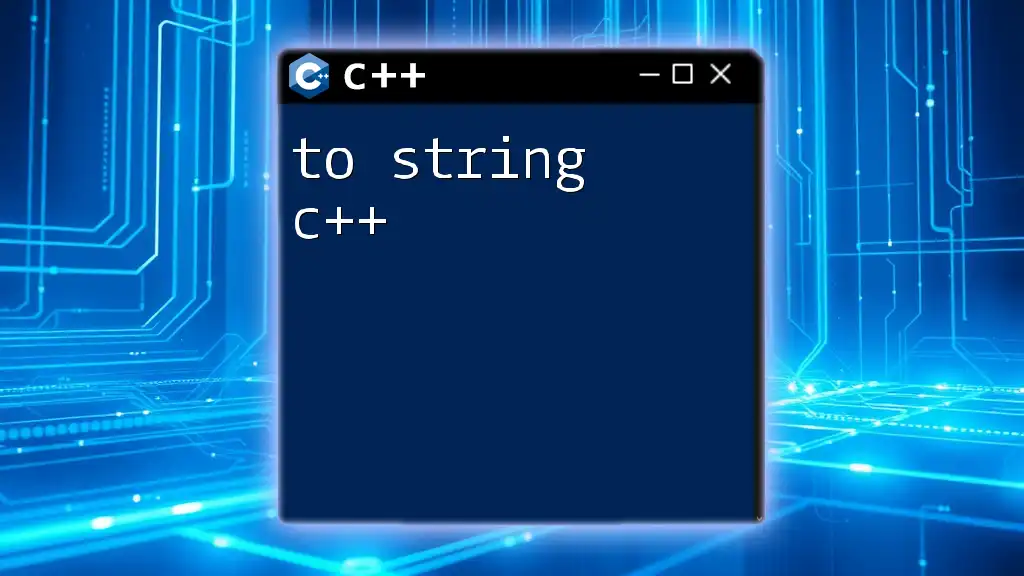
Common Mistakes When Dealing with Empty Strings
Confusing Empty Strings with Null Pointers
A frequent source of confusion arises when programmers confuse empty strings with null pointers. While an empty string is a valid object with a length of zero, a null pointer indicates that no string exists. This distinction is essential for avoiding dereferencing errors and crashes.
std::string* strPtr = nullptr;
// Potentially dangerous if we try to dereference strPtr
Attempting to access a null pointer will result in undefined behavior, highlighting the importance of understanding these differences.
Misusing String Functions
Another common error is misusing string functions or calling them on uninitialized strings. If you intend to use a string that has not been properly initialized, you might end up with unpredictable results or runtime errors.
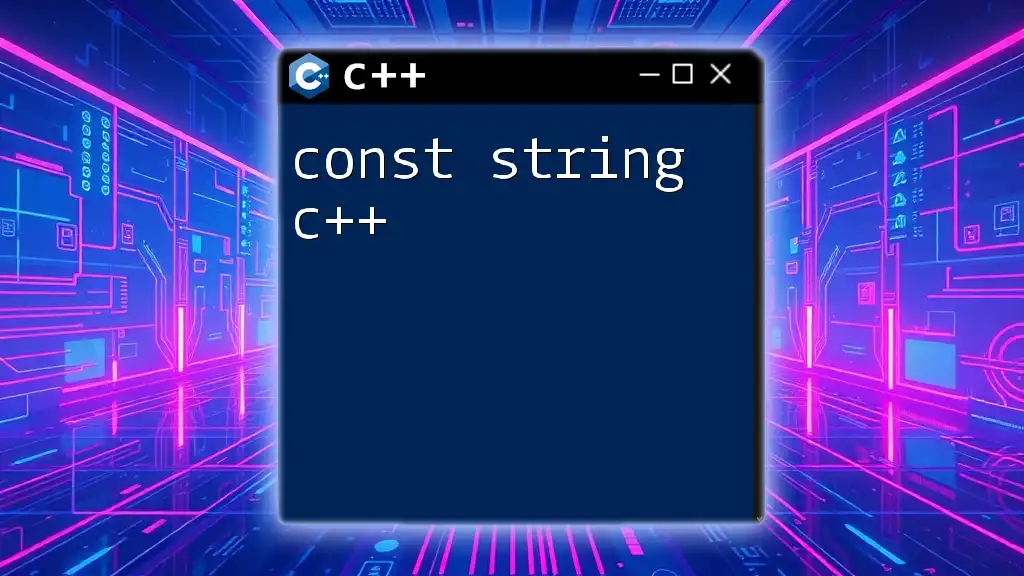
Performance Considerations
Cost of Creating Empty Strings
Creating empty strings does involve some overhead, but it is generally negligible compared to the benefits they offer. Nonetheless, be mindful of unnecessary instantiations in performance-critical sections of your code.
Optimization Tips
To improve efficiency, minimize unnecessary checks for empty strings in performance-sensitive areas. Use logical conditions that enable early exits in your code to avoid overhead from method calls when determining string status isn't crucial.
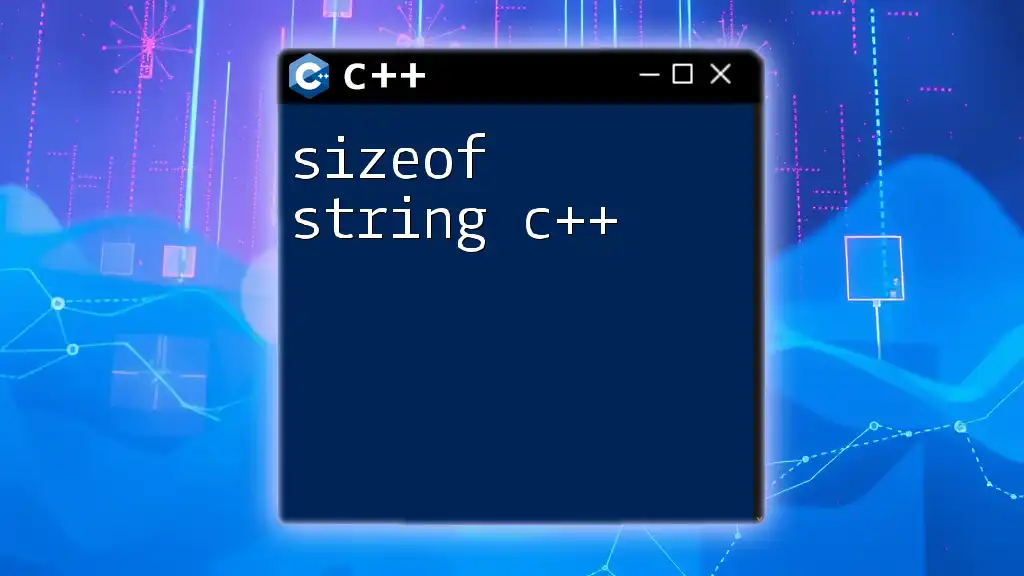
Conclusion
Mastering the concept of an empty string in C++ is essential for writing robust and error-free code. By understanding how to initialize, manage, and check for empty strings, you can ensure your programs handle string data effectively. As you code, remember to implement checks for empty strings to promote safer and more predictable outcomes in user interactions and data handling.