The `to_string` function in C++ converts various data types, including integers and floating-point numbers, into their string representation. Here's a code snippet demonstrating its usage:
#include <iostream>
#include <string>
int main() {
int number = 42;
std::string strNumber = std::to_string(number);
std::cout << "The string representation is: " << strNumber << std::endl;
return 0;
}
Understanding `to_string`
The `to_string` function is a powerful tool in C++ that converts various built-in data types into their string representation. This capability is essential for tasks such as user interface development, data logging, and exporting data to files. Converting numbers, booleans, or any other type to a string makes it easier to manipulate, display, or store data for later use.
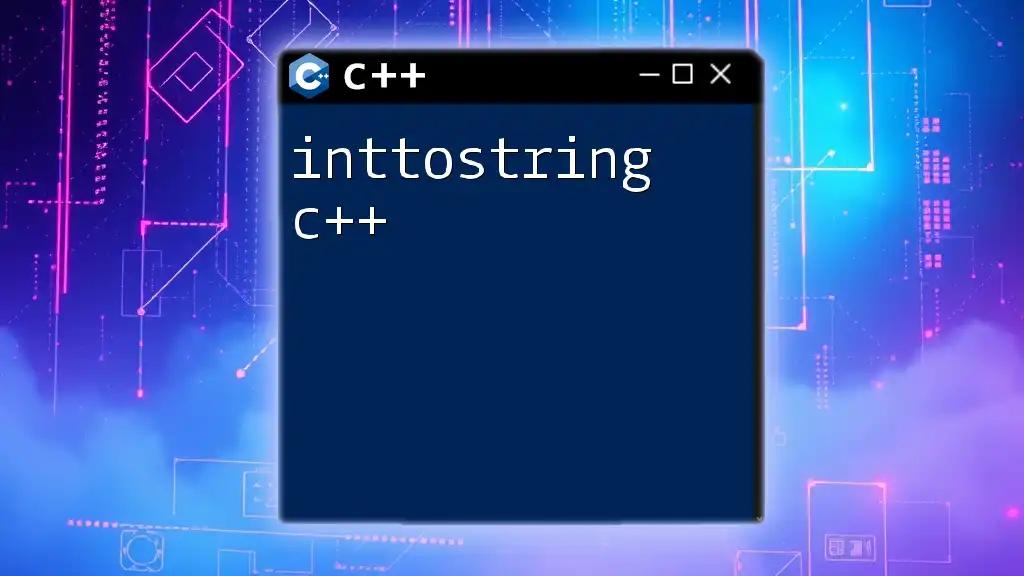
Syntax of `to_string`
The syntax for `to_string` is quite straightforward. The function signature looks like this:
std::string to_string(int val);
std::string to_string(long val);
std::string to_string(long long val);
std::string to_string(unsigned val);
std::string to_string(unsigned long val);
std::string to_string(unsigned long long val);
std::string to_string(float val);
std::string to_string(double val);
std::string to_string(long double val);
std::string to_string(bool val);
Here, you can see that `to_string` accepts multiple parameter types, including integers, floating-point numbers, and booleans, conveniently returning a `std::string`.

Data Types Supported by `to_string`
Overview of Supported Types
-
Integer Types: C++ supports all of the standard integer types, allowing you to convert them easily to strings.
int number = 123; std::string strNumber = std::to_string(number); // "123"
-
Floating-point Types: `to_string` also works seamlessly with floating-point data types.
double pi = 3.14159; std::string strPi = std::to_string(pi); // "3.141590"
-
Boolean Type: When a boolean is converted, `true` becomes `"1"` and `false` becomes `"0"`.
bool isAlive = true; std::string strBool = std::to_string(isAlive); // "1"

Detailed Walkthrough of `to_string` Examples
Basic Usage of `to_string`
To illustrate the basic usage of `to_string`, let's look at how to convert integers and floating-point numbers.
int number = 123;
std::string strNumber = std::to_string(number); // Converts integer to string
double pi = 3.14159;
std::string strPi = std::to_string(pi); // Converts the double to string
Using `to_string`, developers can easily integrate numbers into strings for concatenation or display.
Advanced Usage Scenarios
Formatting Output with `to_string`
One common issue arises when converting floating-point values—the default precision might not always meet your needs. For example, `to_string` tends to present six digits after the decimal point for floating-point types.
float num = 3.14159f;
std::string strNum = std::to_string(num); // Results in "3.141590"
To ensure your code handles floating-point precision effectively, always be mindful of how `to_string` behaves and the implications for your output.
Conversion of Boolean Values
When converting boolean values, remember that `to_string` will produce `"1"` for `true` and `"0"` for `false`. This is useful for conditional statements and logging.
bool flag = false;
std::string strFlag = std::to_string(flag); // "0"
Common Pitfalls and Best Practices
One common pitfall when using `to_string` is the treatment of floating-point values. Since the function converts them to strings with default precision, it's crucial to understand that this may lead to unexpected results in your application.
Best practices include:
- Use `to_string` for straightforward conversions, but prefer `std::ostringstream` for more complex needs.
- Always validate the precision of your output when dealing with floating-point numbers.
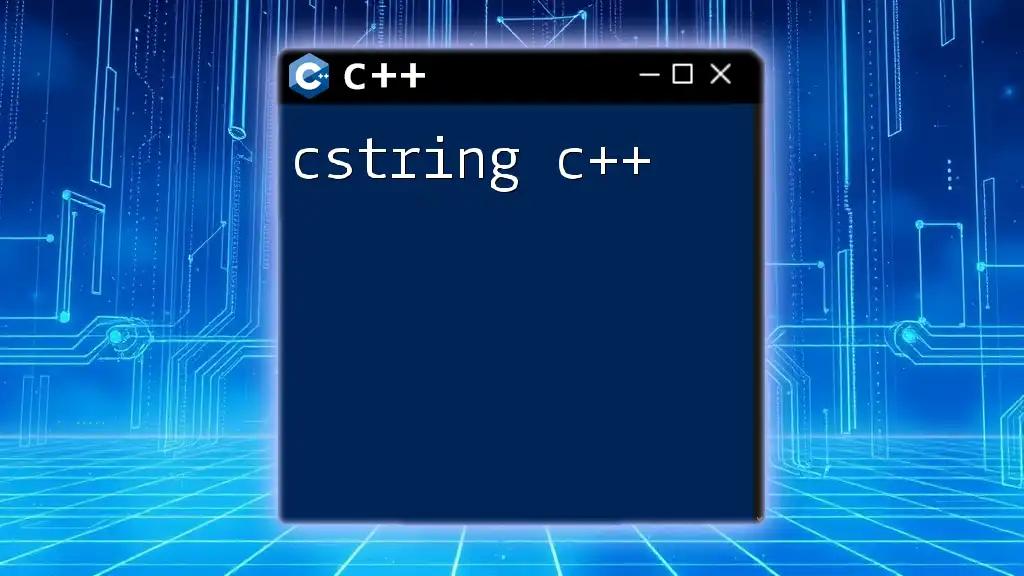
Alternative Functions to Convert to String
Overview of Other Methods
While `to_string` is convenient, there are instances where alternative methods may be preferable, particularly for formatted output or more complex situations.
Using `stringstream` for Complex Conversions
`stringstream` provides more control over the formatting of your output. Here's a simple example demonstrating its use:
#include <sstream>
int number = 456;
std::stringstream ss;
ss << number; // Place the number into the stringstream
std::string strNumber = ss.str(); // Convert to string
This approach allows for greater formatting options and better handling of data types.
Using `std::ostringstream`
Enhancing the formatting even further can be accomplished using `std::ostringstream`. This allows formatted output with specific precision settings:
#include <iomanip>
double value = 9.8765;
std::ostringstream oss;
oss << std::fixed << std::setprecision(2) << value; // Fixed-point notation with precision of 2
std::string strValue = oss.str(); // "9.88"
This method is particularly useful when you need to control how the number appears in your final output.

Common Use Cases for `to_string`
Throughout programming, there are numerous scenarios where you might find `to_string` invaluable:
- Logging and Debugging: Converting numbers and states to strings allows for easier logging and debugging messages which are often formatted as strings.
- User Interface Development: When creating dynamic user interfaces, converting numerical data to strings is essential for display elements.
- CSV and File Handling: Converting numbers for data export in formats like CSV requires an easy transition from numbers to strings.
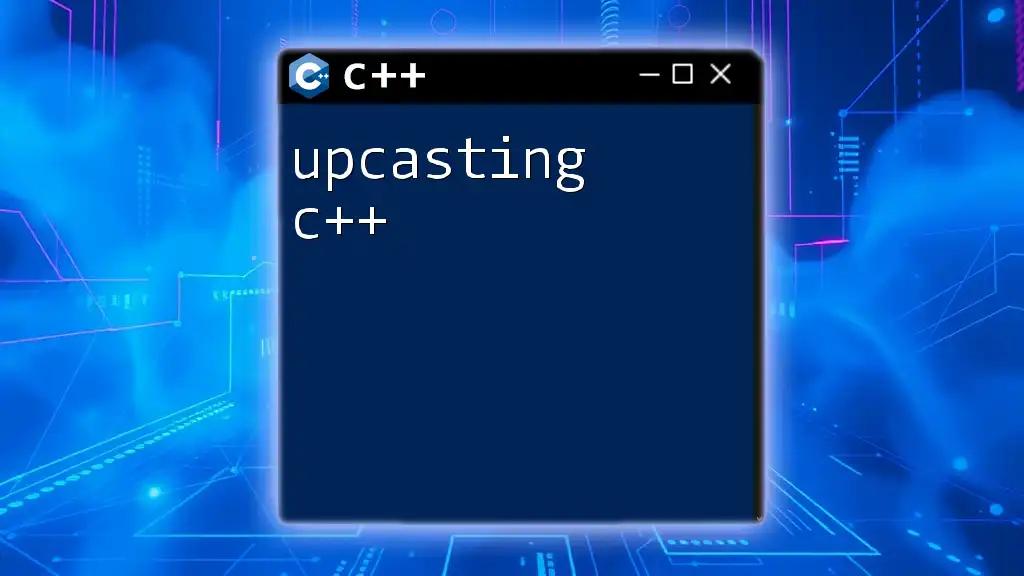
Conclusion
In summary, the `to_string` function in C++ offers an efficient and convenient way to convert various data types into strings. Whether you're debugging, developing user interfaces, or preparing data for export, this function plays a vital role in string manipulation. Practicing its use will enhance your coding skills and allow you to handle string conversions more effectively in your projects.
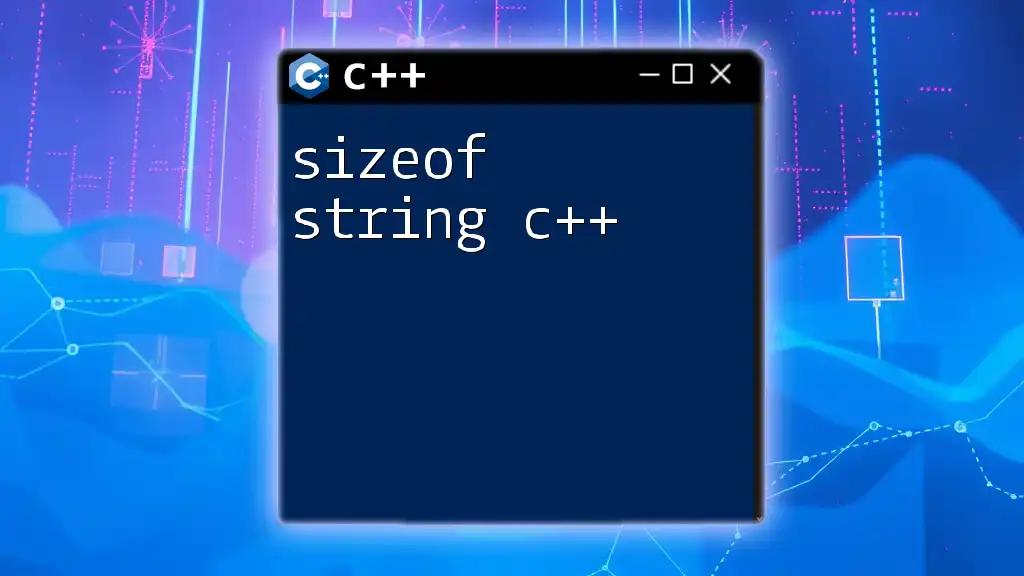
Additional Resources
For further reading on `to_string`, consider diving into the C++ standards documentation or exploring tutorials about string handling techniques. Embrace the versatility of C++ string functions to elevate your programming expertise!