Using C++ involves leveraging its powerful syntax and features to write efficient, compiled programs, such as the example below which demonstrates a simple "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++
What is C++?
C++ is a powerful, high-performance programming language developed by Bjarne Stroustrup in the early 1980s. It expands upon the foundational concepts of the C programming language by introducing object-oriented features, which allow for easier data management and manipulation at a higher abstraction level. C++ supports a variety of programming paradigms, making it enormously versatile in its applications, from systems programming to game development.
Why Use C++?
Using C++ provides significant performance benefits, mainly due to its ability to operate close to the hardware level, enabling efficient memory management and optimized execution of code. This efficiency makes C++ a preferred choice in sectors where speed is critical, such as:
- Game Development
- Real-time simulation
- Operating Systems
- High-performance applications
- System Level Access
Moreover, C++ is commonly used for developing complex software systems and applications due to its rich set of libraries and frameworks that can be leveraged effectively.
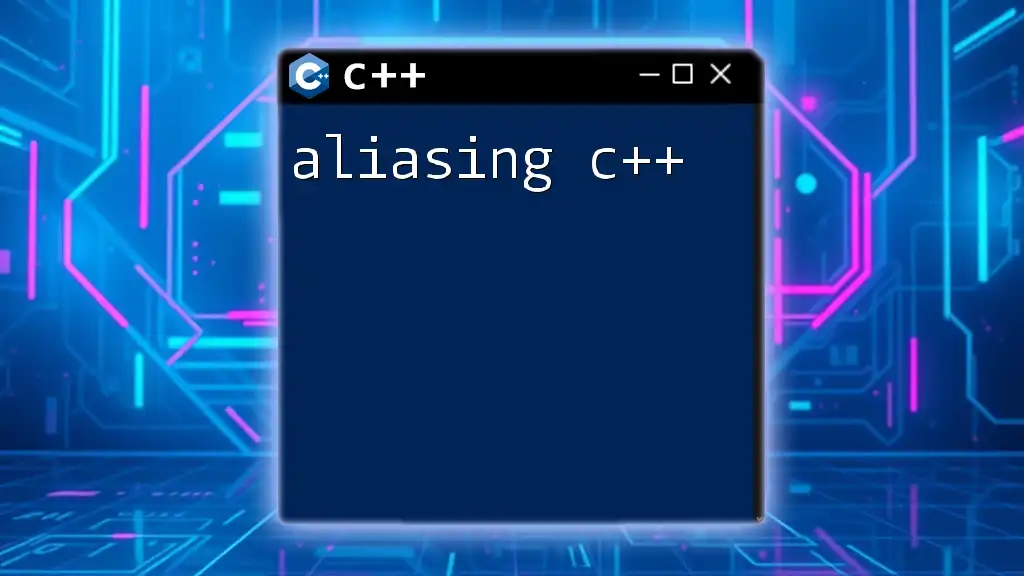
Getting Started with C++
Setting Up Your Environment
To start using C++, you need a suitable development environment. Here are some recommended Integrated Development Environments (IDEs):
- Visual Studio: A powerful IDE for Windows with extensive support for C++.
- Code::Blocks: A free, open-source IDE that’s highly customizable.
- Eclipse: An IDE with C++ support through the CDT (C/C++ Development Tooling).
To install any of these IDEs, follow the official documentation provided on their respective websites, and ensure that you also install a suitable compiler such as MinGW or Microsoft's C++ compiler.
Your First C++ Program
After setting up your environment, it’s time to write your first C++ program. A classic beginner example is the “Hello, World!” program, used to demonstrate the syntax of a language.
Here’s how you would write it in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Explanation:
- `#include <iostream>`: This line includes the Standard Input Output stream library, allowing you to use `std::cout`.
- `int main() { ... }`: This is the main function where program execution begins.
- `std::cout << "Hello, World!" << std::endl;`: This outputs the text "Hello, World!" to the console.
The key takeaway from this snippet is understanding how functions and the main execution flow works in C++.
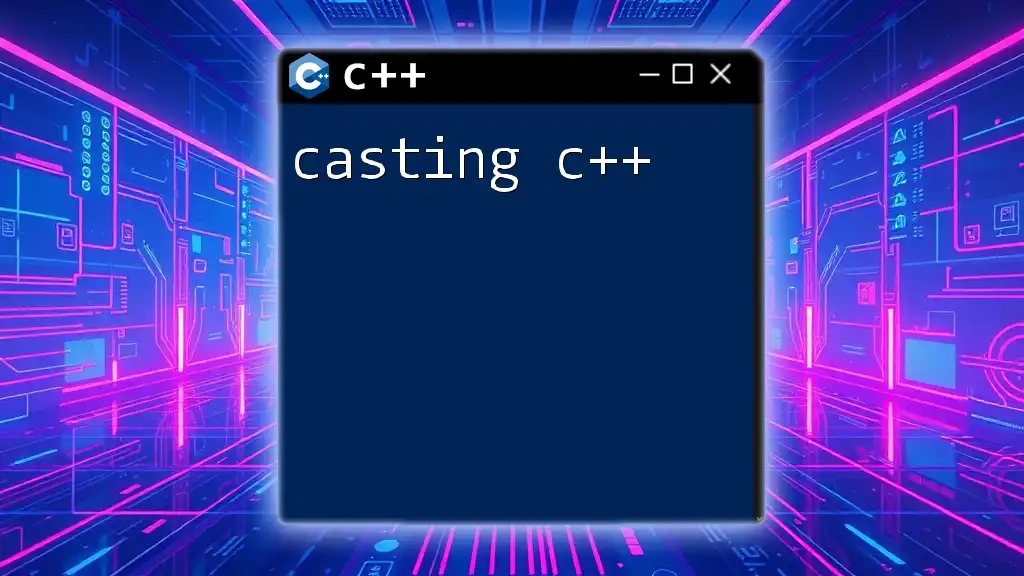
Basic Syntax and Structure
Understanding the Syntax
C++ has a structured syntax that emphasizes readability and organization. At the core, we have:
- Variables: Used to store data.
- Data Types: Such as `int`, `float`, `char`, etc.
- Semicolons: Denote the end of a statement.
Example of variable declaration:
int age = 30;
Control Structures
Control structures allow you to dictate the flow of your program.
Conditional Statements
Using conditional statements helps in making decisions in your code. The `if`, `else if`, and `else` statements are fundamental.
Here’s an example:
if (age >= 18) {
std::cout << "Adult";
} else {
std::cout << "Minor";
}
Explanation:
- The program checks the `age` variable and outputs either "Adult" or "Minor" based on the condition evaluated.
Loops
Loops enable you to execute code repeatedly. The two primary types are for loops and while loops.
Example of a for loop:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
This snippet will print the numbers 0 through 4.
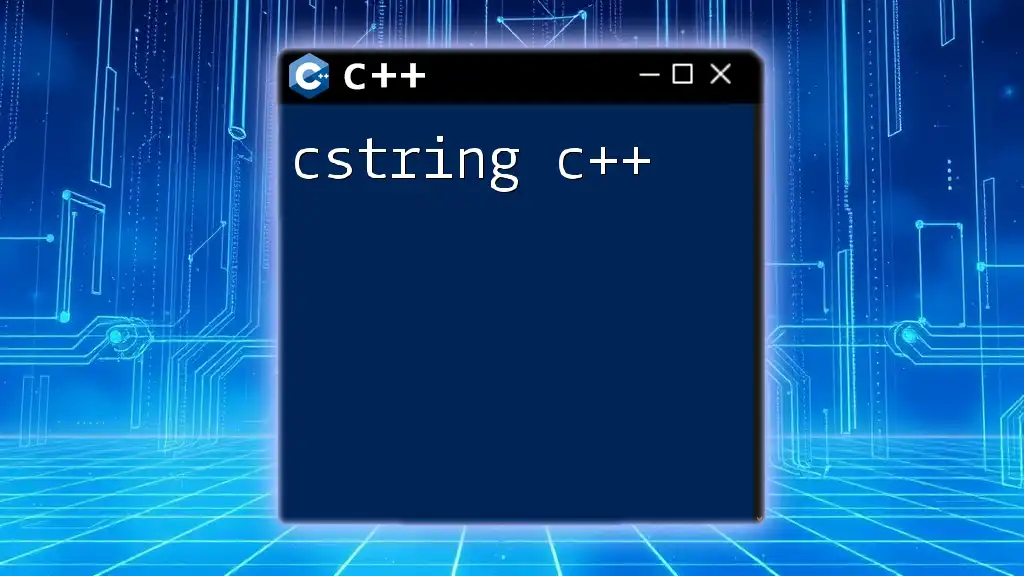
Functions in C++
Definition and Importance
Functions are blocks of code that perform a specific task, making programs more modular and easier to read. By breaking down code into functions, it aids in managing complexity.
Creating and Calling Functions
Here’s how to create and use a function in C++:
int add(int a, int b) {
return a + b;
}
// Calling the function
std::cout << add(3, 4);
In this example, the `add` function takes two integers, adds them, and returns the result. The output will be `7`.
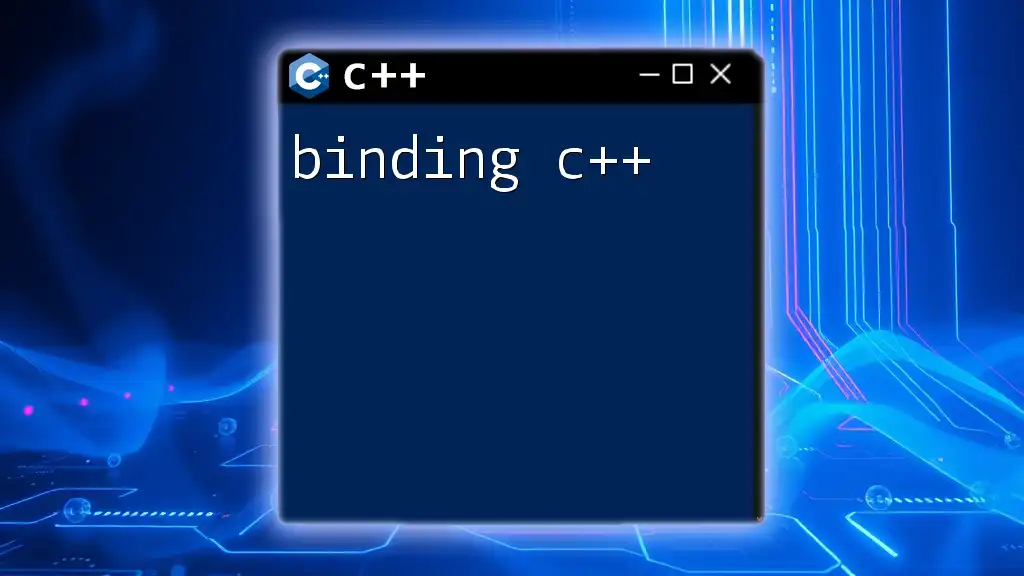
Leveraging Classes and Objects
Object-Oriented Programming in C++
C++ is built supporting the Object-Oriented Programming (OOP) paradigm. OOP allows for the creation of reusable code through the concepts of classes and objects, which help in structuring programs efficiently.
Creating a Class
Here’s how you define a class in C++:
class Car {
public:
std::string brand;
void drive() {
std::cout << "Driving " << brand << std::endl;
}
};
Instance Creation and Usage
After defining your class, you can create an object (or instance) of this class:
Car myCar;
myCar.brand = "Toyota";
myCar.drive();
This example shows how to create an instance of the `Car` class, set the `brand`, and call the `drive` method which outputs "Driving Toyota".
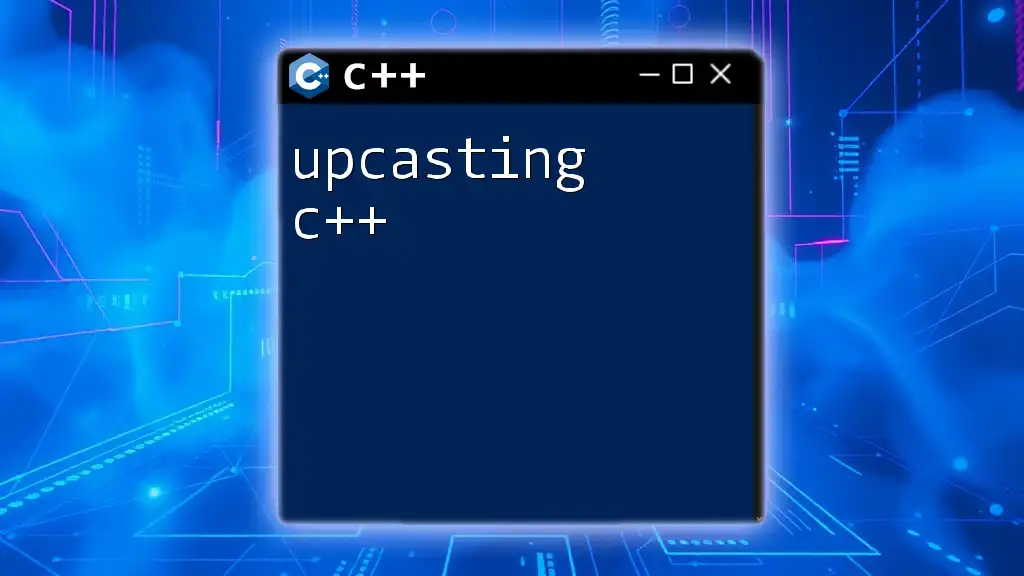
Using Standard Template Library (STL)
Introduction to STL
The Standard Template Library (STL) is a powerful feature of C++ that provides numerous ready-to-use classes and functions for managing collections of data. STL includes several components, such as containers, algorithms, and iterators.
Practical Examples with STL
Using Vectors
Vectors are dynamic arrays that can resize themselves automatically. Here’s how to use them:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4};
for (int num : numbers) {
std::cout << num << std::endl;
}
This snippet initializes a vector of integers and iterates through it, printing each number.
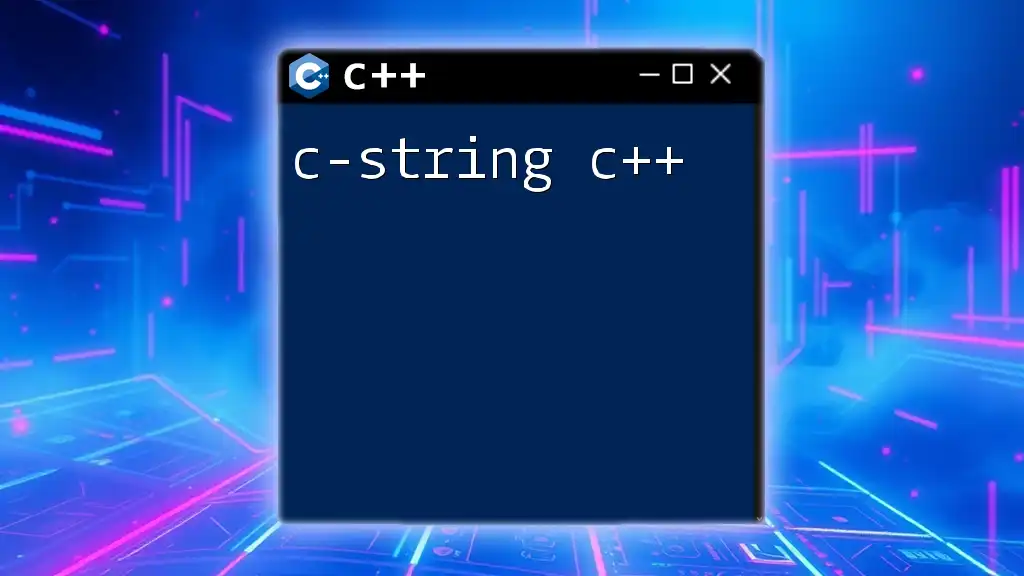
Handling Exceptions
Importance of Exception Handling
Exception handling is critical for maintaining the robustness of your programs. It allows you to manage errors gracefully, preventing your program from crashing unexpectedly.
Example of Exception Handling
Here’s a basic structure for handling exceptions:
try {
throw std::runtime_error("An error occurred");
} catch (const std::runtime_error& e) {
std::cout << e.what() << std::endl;
}
In this case, if an error occurs, the `catch` block will execute, outputting the error message.
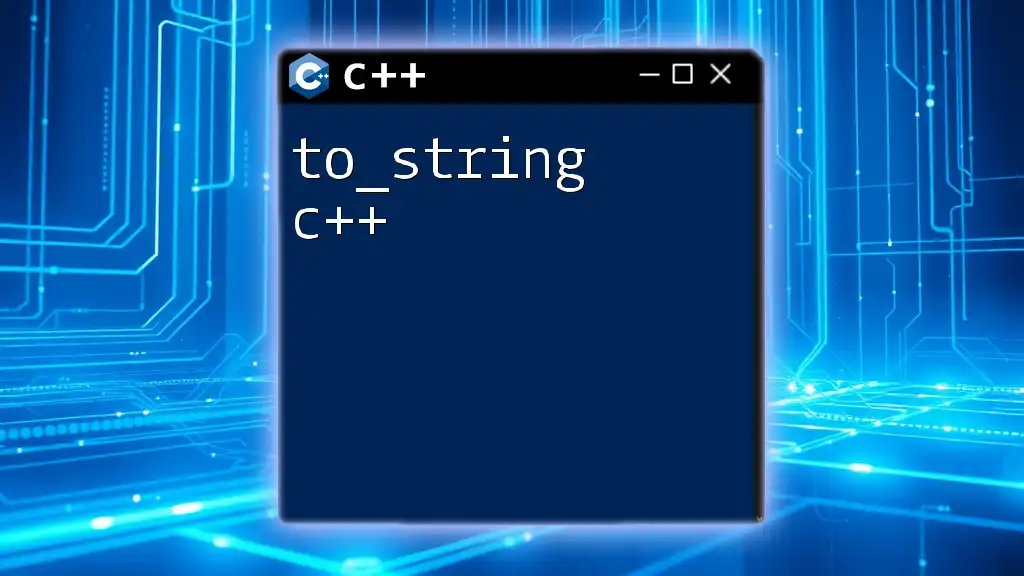
Best Practices for Using C++
Writing Clean Code
Writing readable and maintainable code is paramount in programming. Use meaningful variable names, indentations, and comments effectively. This helps not just you but also others who might read your code in the future.
Common Mistakes to Avoid
- Memory Leaks: Always ensure you’re managing memory properly, especially with dynamic allocations.
- Not using const and explicit: Leverage these keywords to prevent unwanted modifications and improve clarity in your code.
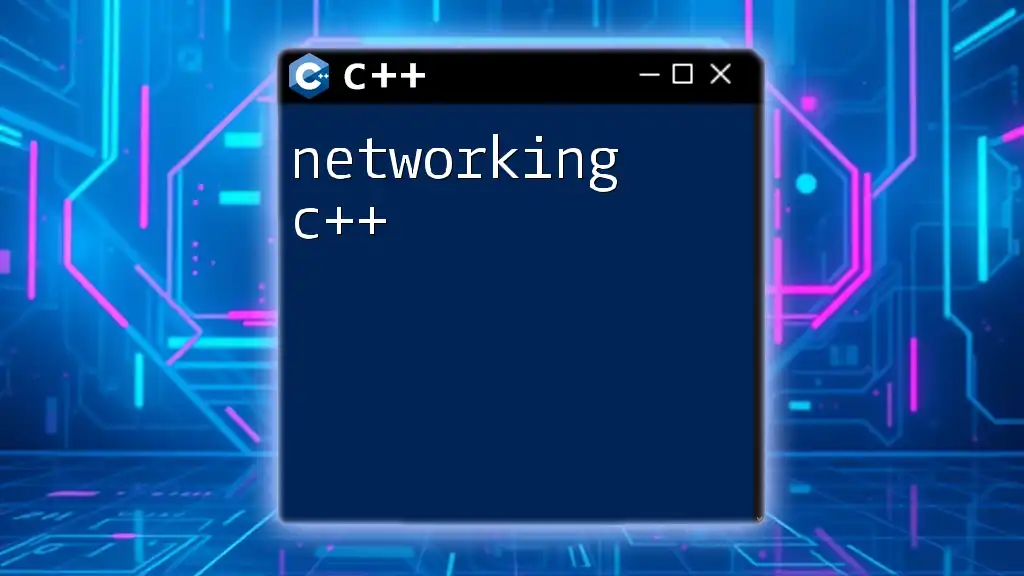
Conclusion
Mastering the art of using C++ opens up a myriad of opportunities in software development. This guide has covered foundational aspects of C++, from basic syntax and functions to object-oriented programming and STL. By practicing regularly and applying these concepts, you will enhance your skills and become proficient in using C++.