Async C++ allows you to run operations concurrently, improving the performance of your applications by utilizing asynchronous functions and future objects.
Here's a simple example using `std::async`:
#include <iostream>
#include <future>
int asyncFunction() {
return 42;
}
int main() {
std::future<int> result = std::async(asyncFunction);
std::cout << "The answer is: " << result.get() << std::endl;
return 0;
}
What is Asynchronous Programming?
Asynchronous programming is a programming paradigm that allows a program to initiate a task and move on to another task before that initial task is completed. This contrasts with synchronous programming, where tasks must be completed one after the other. The significance of asynchronous programming lies in its ability to enhance performance and responsiveness in applications, especially for tasks that involve waiting, such as I/O operations or network requests.
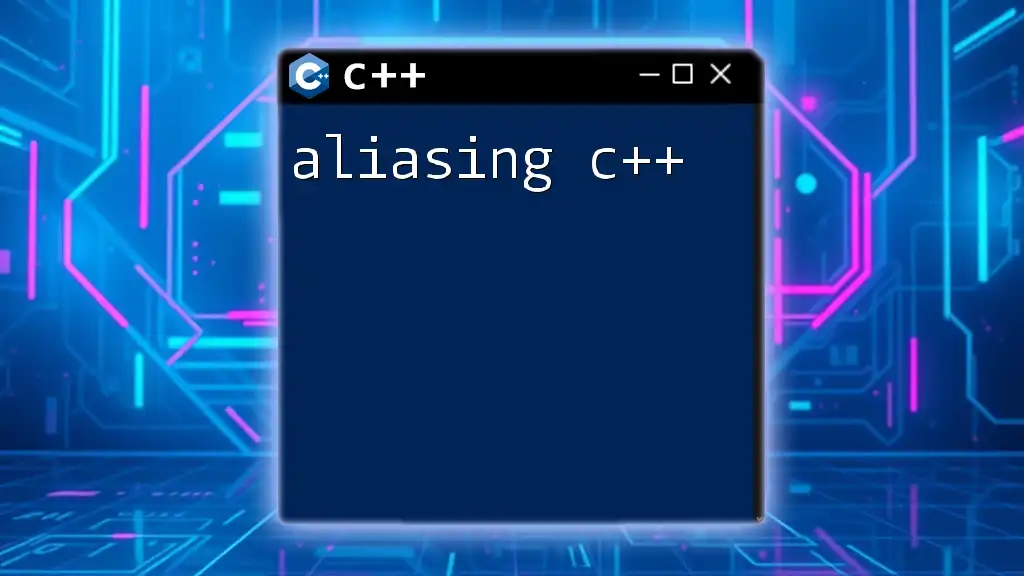
Why Use Async in C++?
Incorporating `async` in C++ allows developers to maximize resource utilization and improve the responsiveness of applications. Key benefits include:
- Non-blocking Execution: Functions can run concurrently without stopping the main program flow.
- Improved Performance in I/O-bound Applications: These applications can benefit substantially from asynchronous operations, reducing wait times.
- Enhanced User Experience: Applications remain responsive, making software feel faster and more fluid.
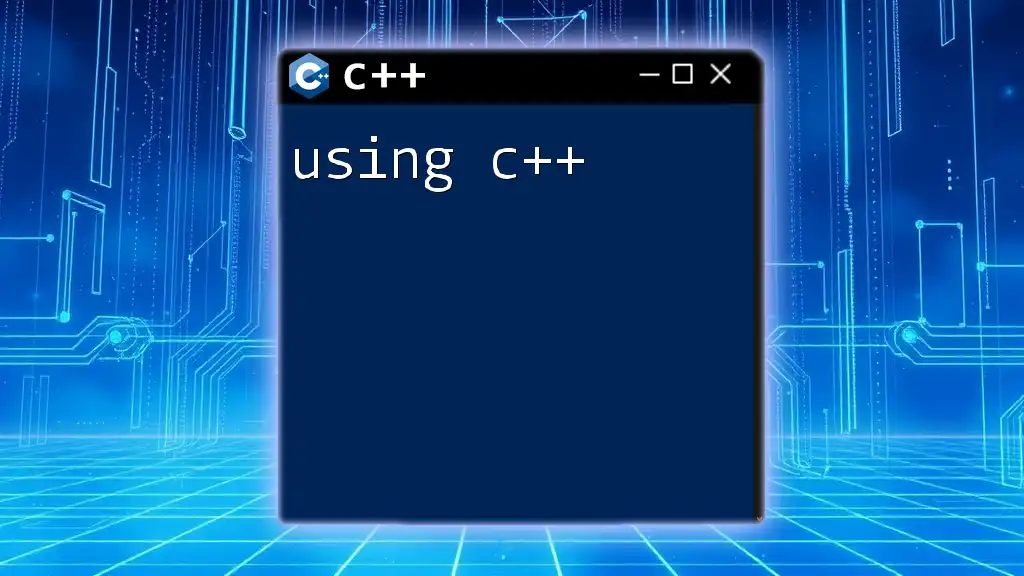
Defining C++ Async
C++ provides the `std::async` function, introduced in C++11, as a means to launch asynchronous tasks. The `std::async` function allows a developer to create a task that may execute in parallel on another thread or defer execution until explicitly called.
#include <future>
#include <iostream>
int exampleFunction() {
return 42;
}
int main() {
std::future<int> result = std::async(std::launch::async, exampleFunction);
std::cout << "Result: " << result.get() << std::endl;
return 0;
}
In this example, `exampleFunction` runs asynchronously, allowing the main program to await its result without blocking.
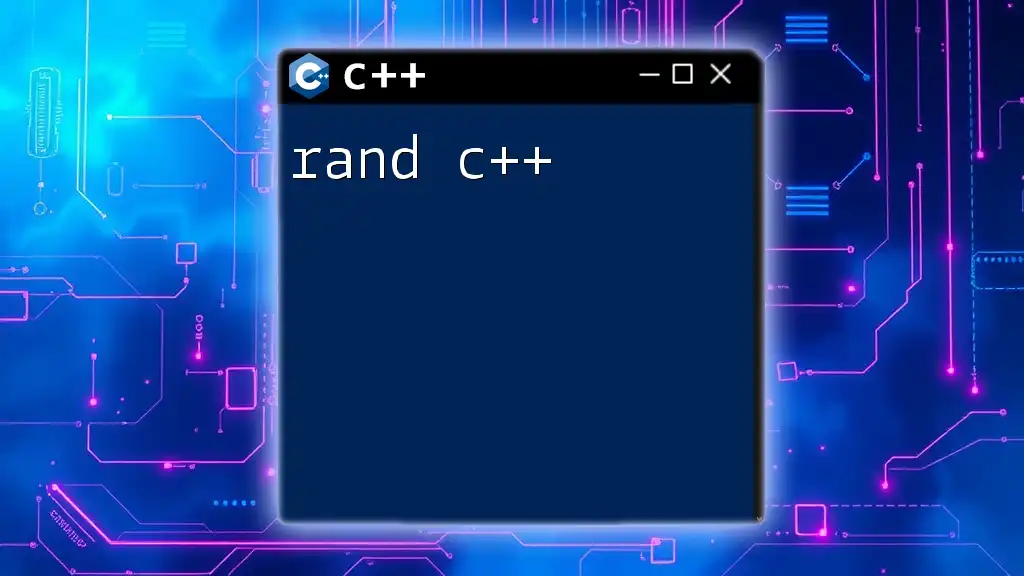
How Async Works in C++
Async in C++ leverages the thread management capabilities of the C++ standard library. When you call `std::async`, the library manages the underlying thread creation and execution, providing a cleaner interface for multi-threaded programming. Understanding the C++ memory model and ensuring proper handling of shared resources is critical for writing effective asynchronous code.
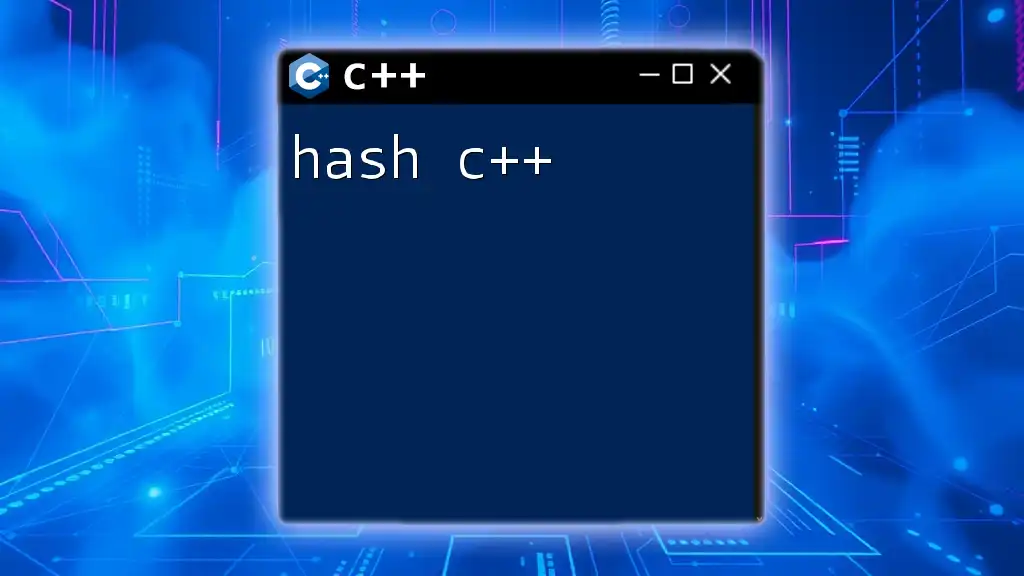
Launch Policies in C++ Async
`std::async` can accept different launch policies that influence how tasks are executed. There are two primary options:
- std::launch::async: Forces the task to run on a new thread. This ensures that the function executes immediately in parallel.
- std::launch::deferred: The function execution is deferred until the `future` object’s `get()` method is called. In this case, the task runs in the same thread that calls `get()`.
Choosing between these options depends on your specific use case. For CPU-bound tasks, `std::launch::async` is often preferred, while for lightweight or I/O-bound tasks, `std::launch::deferred` might be more efficient.
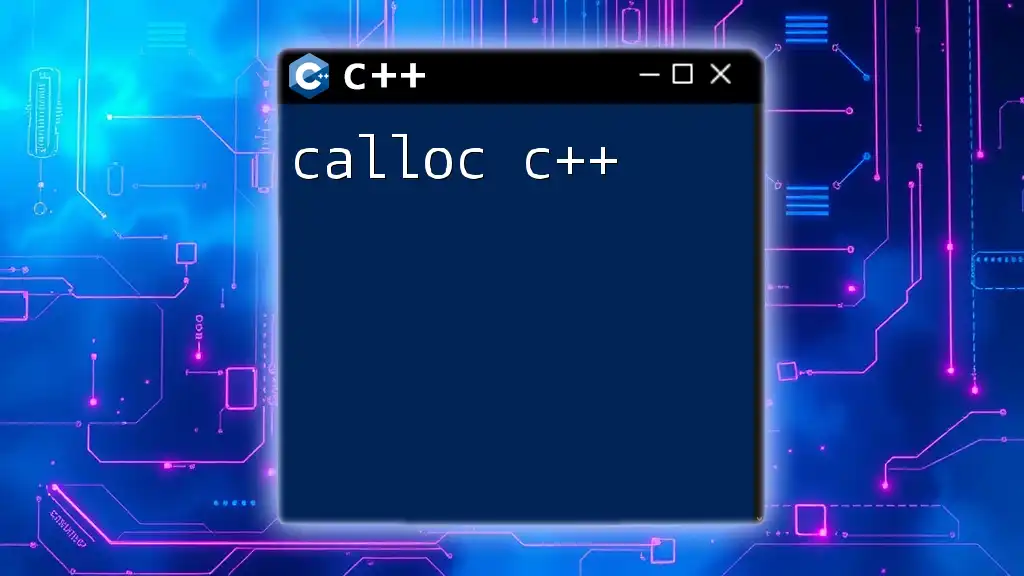
Error Handling with Async
Handling errors correctly in asynchronous tasks is vital. If a function executed through `std::async` throws an exception, it is captured in the associated `future` and rethrown when you call `get()`.
std::future<int> safeAsyncFunction() {
throw std::runtime_error("An error occurred!");
}
try {
auto result = safeAsyncFunction().get();
} catch (const std::exception& e) {
std::cout << "Error: " << e.what() << std::endl;
}
In this snippet, invoking `.get()` on the `future` throws the captured exception, which can be caught and handled gracefully. This is an essential aspect of building robust asynchronous applications.
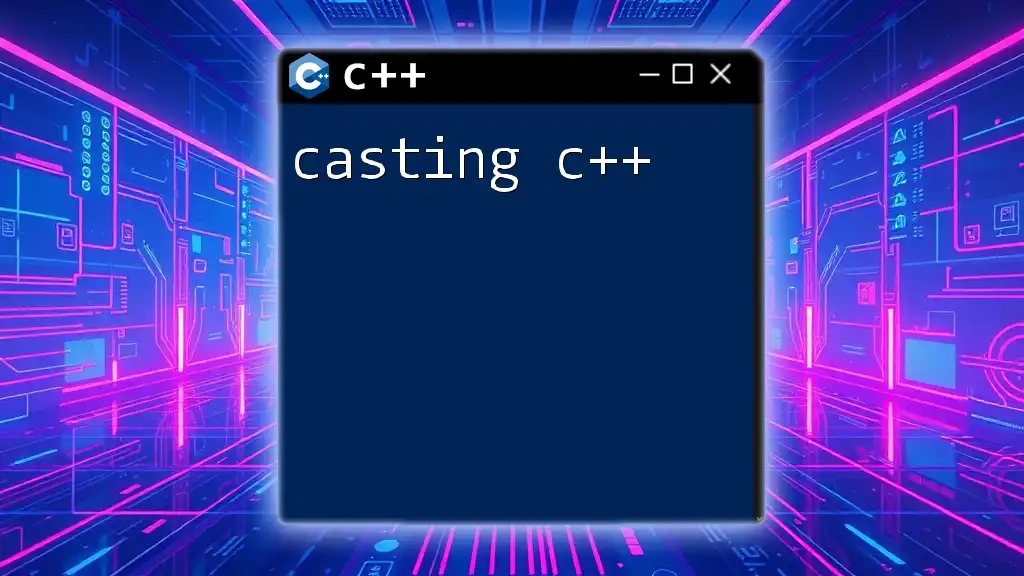
Asynchronous I/O Operations
Async programming can significantly enhance I/O-bound operations, such as network calls or file reading. When utilizing async in these scenarios, the application can continue processing other tasks while waiting for the I/O operations to complete. This non-blocking behavior is crucial in modern applications, where user interactivity is a priority.
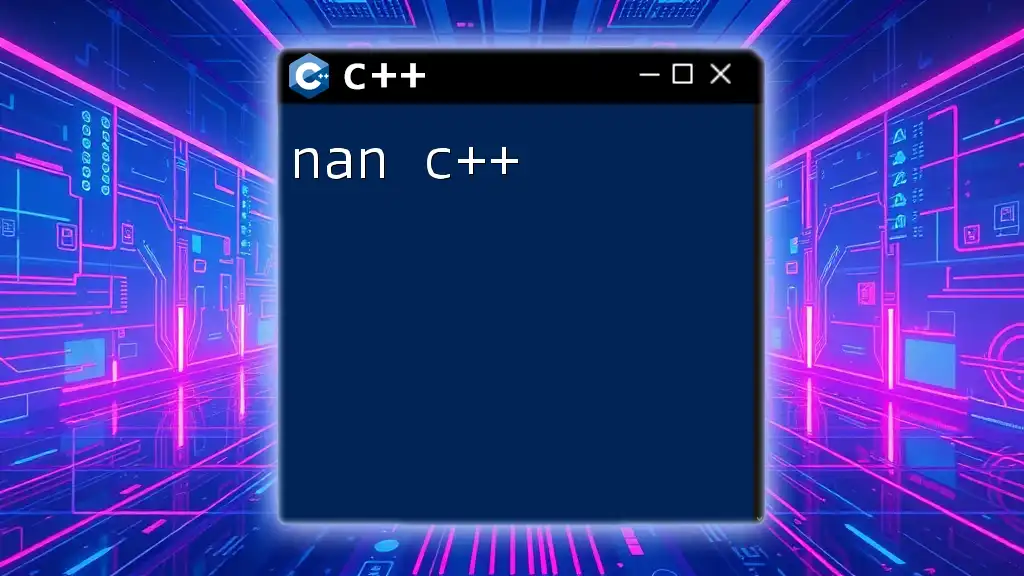
Parallel Computing with Async
Parallel computing can be simplified using `async`. Running multiple tasks concurrently allows for performance improvements, particularly when each task is independent and can be computed simultaneously.
auto task1 = std::async([] { return "Task 1 completed"; });
auto task2 = std::async([] { return "Task 2 completed"; });
std::cout << task1.get() << std::endl;
std::cout << task2.get() << std::endl;
In this example, two separate tasks run concurrently, enhancing overall throughput and performance.
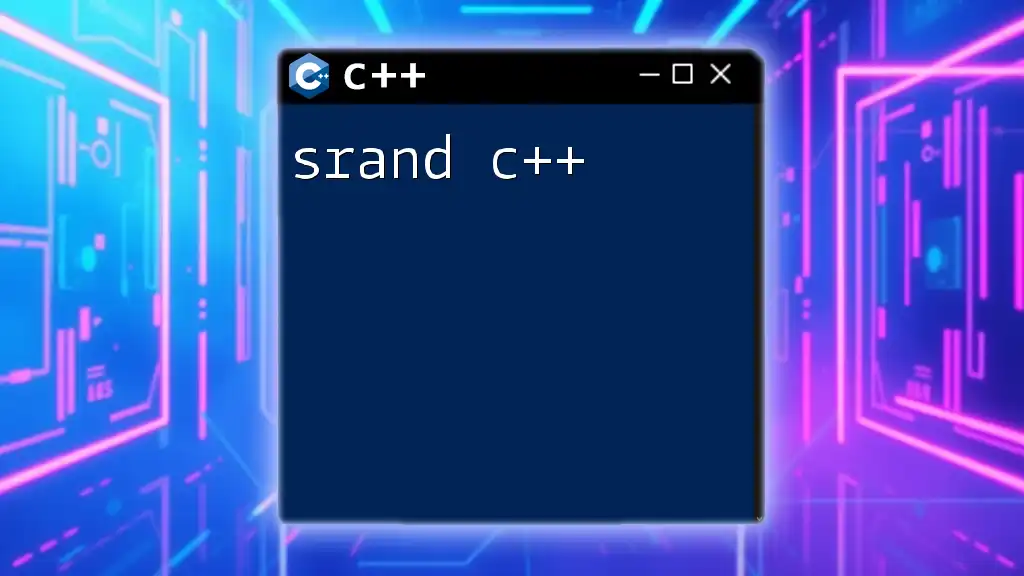
Combining Async with Futures
Using futures in conjunction with async can lead to powerful applications. Futures represent the eventual result of asynchronous operations, allowing further processing without waiting for tasks to complete.
auto future1 = std::async([] { return 5; });
auto future2 = std::async([f1 = std::move(future1)]() { return f1.get() * 2; });
std::cout << "Future Result: " << future2.get() << std::endl;
Here, the second future depends on the completion of the first, demonstrating how futures can be chained together for dependent computations.
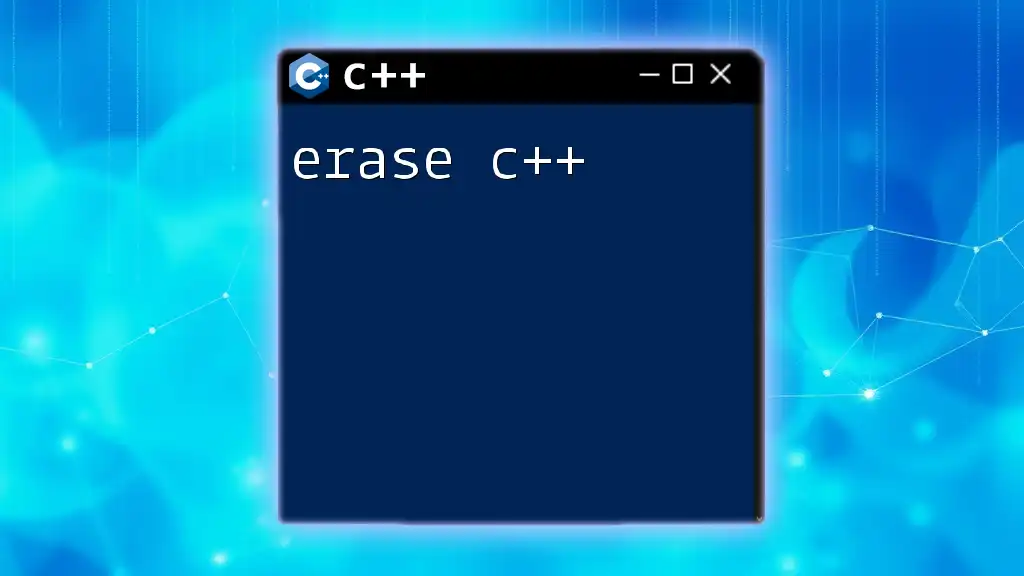
Callbacks and Async
While async functions return futures, callbacks can offer a more flexible approach to handling results. A callback is a function that is passed as an argument to another function and is executed after some computation.
However, care must be taken with callbacks, particularly concerning exceptions and thread safety. Using `std::async`, you can easily integrate callbacks that execute when a task completes.
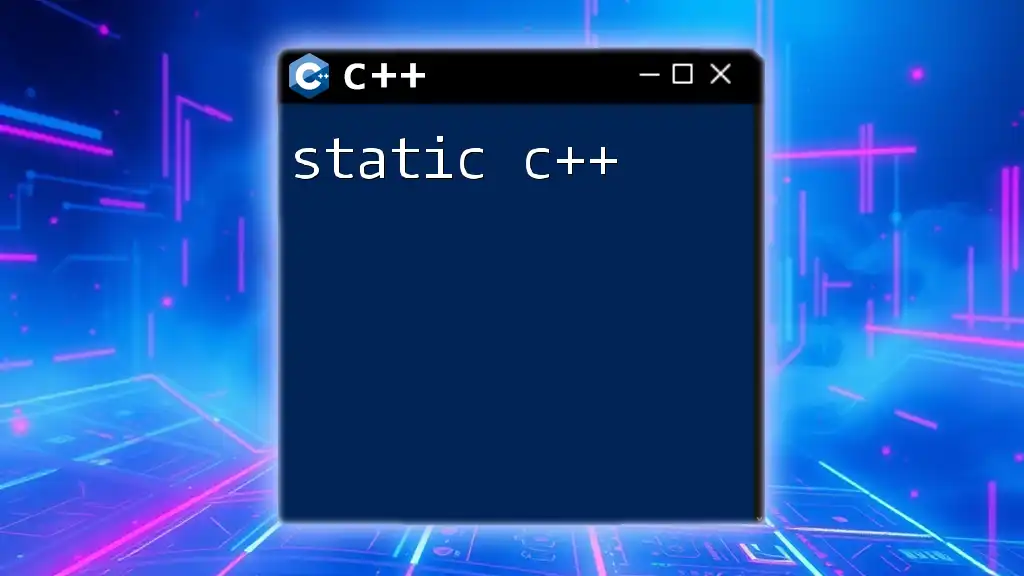
Performance Considerations
When deciding between using async or traditional threading, consider the nature of your tasks:
- I/O-bound tasks: Generally benefit from async as they often involve waiting for external resources.
- CPU-bound tasks: If your tasks are compute-intensive, you might need a more explicit threading approach rather than relying on async.
Measuring performance is crucial. Always benchmark the execution time and resource usage of your async code versus synchronous implementations to ensure you are gaining the expected benefits.
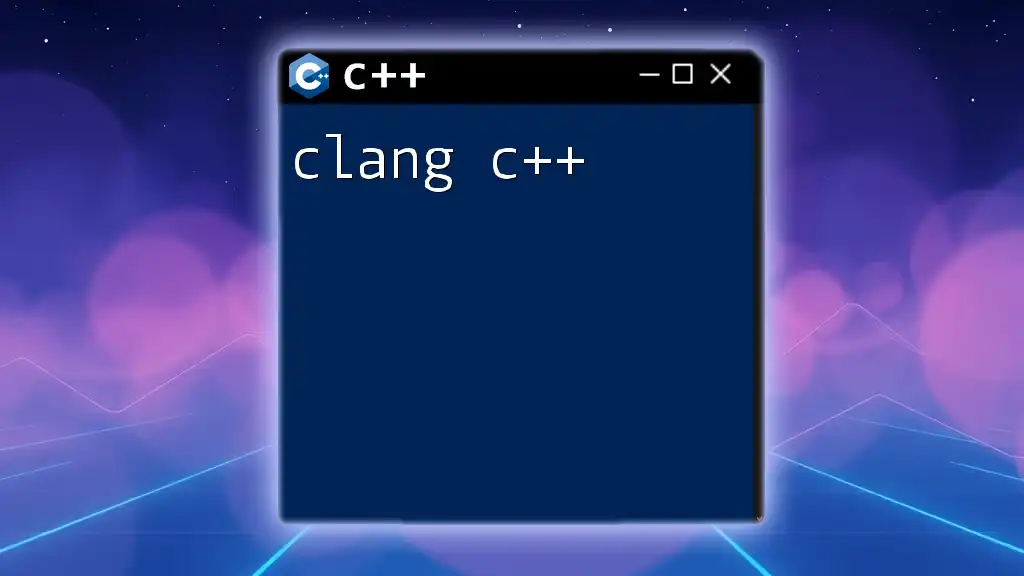
Writing Clean and Maintainable Async Code
Maintaining clarity is fundamental when working with async. Key best practices include:
- Avoid deep nesting of async calls for readability. Use helper functions if necessary.
- Minimize shared state to reduce complexity and the chance of race conditions.
- Use descriptive names for tasks and results to make your code self-documenting.
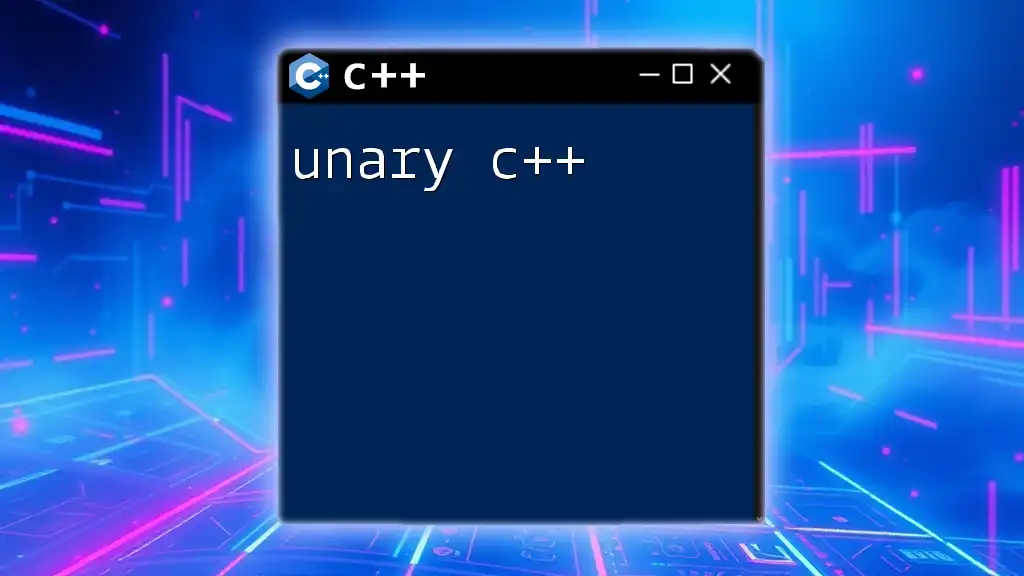
Debugging Async Code
Debugging asynchronous programs can be challenging due to the concurrent nature of task execution. Here are some strategies:
- Use Logging: Log the entry and exit of asynchronous functions to track their execution flow.
- Employ Thread Sanitizers: Utilize tools and sanitizers provided by your development environment to identify race conditions and deadlocks.
- Consider Unit Tests: Write comprehensive tests that check for the correctness of your asynchronous logic.
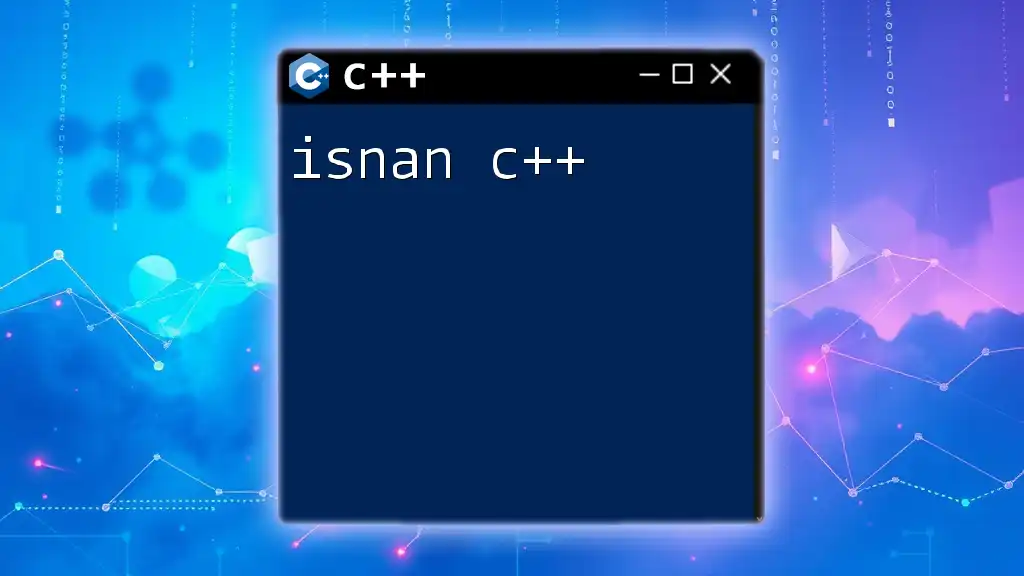
Recap of Async in C++
Throughout this guide, we've explored the fundamentals and applications of `async c++`, delving into launch policies, performance considerations, and best practices for writing clean and maintainable code. The asynchronous paradigm not only enables better performance but also helps create responsive user interfaces.
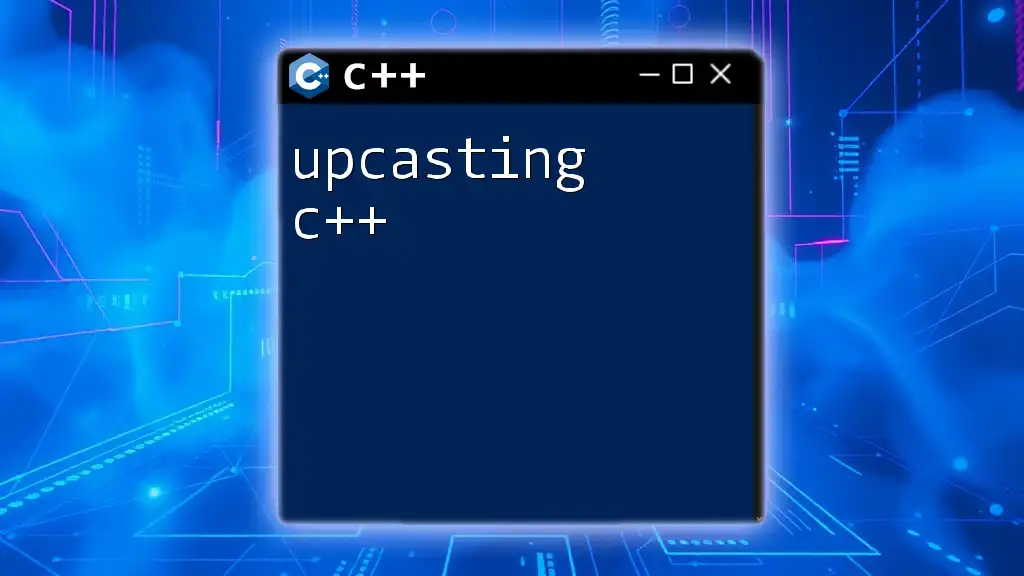
Further Learning Resources
To deepen your knowledge of async programming in C++, consider resources such as:
- Textbooks on C++11 and C++ Concurrency.
- Online tutorials and courses focused specifically on asynchronous programming in C++.
- Community forums and discussion groups for real-world applications and problem-solving.
By experimenting with `std::async` and engaging with the broader C++ community, you can refine your skills and become proficient in implementing asynchronous programming in your applications.