In C++, a hash function is used to map data of arbitrary size to fixed-size values, often utilized in hash tables to enable fast data retrieval.
Here's a simple example of a hash function using `std::hash`:
#include <iostream>
#include <string>
#include <functional>
int main() {
std::string key = "example";
std::hash<std::string> hashFn;
size_t hashValue = hashFn(key);
std::cout << "Hash value of \"" << key << "\": " << hashValue << std::endl;
return 0;
}
What is a Hash Function?
A hash function is a computational algorithm that transforms input data of any size into a fixed-size string of characters. This transformation helps map data in a seemingly random manner. In programming, hash functions are critical for organizing data efficiently, allowing for quicker access and retrieval in data structures like hash tables.
Properties of Hash Functions
When working with hash functions, it's crucial to understand their key properties:
- Deterministic: A hash function produces the same output consistently for the same input. This reliability is vital for data integrity.
- Fast Computation: The function must compute the hash value quickly, ensuring efficient data access and manipulation.
- Pre-image Resistance: It should be computationally infeasible to retrieve the original data from its hash representation.
- Collision Resistance: A good hash function minimizes the chances of two different inputs generating the same output, known as a collision.

Common Use Cases for Hashing in C++
Hashing finds applications in numerous fields within C++ programming, particularly in data structures and cryptography.
Data Structures
Hash Tables
A hash table is a data structure that implements an associative array, allowing for fast data insertion, deletion, and retrieval. The efficiency of hash tables stems from their ability to use a hash function to compute an index into an array of buckets or slots, where the desired value is stored.
Here’s a simple implementation of a hash table in C++:
#include <iostream>
#include <unordered_map>
#include <string>
class HashTable {
private:
std::unordered_map<std::string, int> table;
public:
void insert(const std::string &key, int value) {
table[key] = value;
}
int search(const std::string &key) {
if (table.find(key) != table.end()) {
return table[key];
}
return -1; // or some not-found indicator
}
void remove(const std::string &key) {
table.erase(key);
}
};
int main() {
HashTable ht;
ht.insert("example", 1);
std::cout << "Value for 'example': " << ht.search("example") << std::endl;
ht.remove("example");
return 0;
}
This example demonstrates inserting, searching, and removing elements from a hash table using `std::unordered_map`.
Sets and Maps
The C++ Standard Library offers data structures such as `std::unordered_set` and `std::unordered_map`, which utilize hash functions under the hood for efficient O(1) average-time complexity for search, insert, and delete operations.
#include <iostream>
#include <unordered_set>
#include <unordered_map>
int main() {
std::unordered_set<std::string> mySet;
mySet.insert("apple");
mySet.insert("banana");
if (mySet.find("banana") != mySet.end()) {
std::cout << "Banana found in the set." << std::endl;
}
std::unordered_map<std::string, int> myMap;
myMap["orange"] = 5;
std::cout << "Count of oranges: " << myMap["orange"] << std::endl;
return 0;
}
In this example, `std::unordered_set` checks for membership, while `std::unordered_map` efficiently associates a key with a value.
Cryptography
Hashing Algorithms
Cryptography relies heavily on hashing algorithms. Common algorithms include:
- MD5: While fast, it is no longer considered secure.
- SHA-256: Part of the SHA-2 family, it offers a higher level of security and is widely used in various applications.
When choosing a hashing algorithm, consider the security requirements and performance implications.
Example: Implementing SHA-256 in C++
Here’s a simplified view of how one might implement SHA-256 in C++. Though actual implementations are extensive, this snippet serves to illustrate the core concept:
#include <iostream>
#include <cstring>
#include <openssl/sha.h>
void SHA256Hash(const std::string &input) {
unsigned char hash[SHA256_DIGEST_LENGTH];
SHA256_CTX sha256;
SHA256_Init(&sha256);
SHA256_Update(&sha256, input.c_str(), input.size());
SHA256_Final(hash, &sha256);
std::cout << "SHA-256 hash: ";
for (int i = 0; i < SHA256_DIGEST_LENGTH; i++) {
printf("%02x", hash[i]);
}
std::cout << std::endl;
}
int main() {
SHA256Hash("Hello, World!");
return 0;
}
This code uses OpenSSL to compute the SHA-256 hash of the given input string, producing a hex representation of the hash value.

Implementing Hash Functions in C++
Creating a Custom Hash Function
To create a custom hash function in C++, ensure it adheres to the properties outlined earlier. Here’s how you can define a hash function for a user-defined type:
#include <iostream>
#include <functional>
struct User {
std::string name;
int age;
bool operator==(const User &other) const {
return name == other.name && age == other.age;
}
};
namespace std {
template <>
struct hash<User> {
size_t operator()(const User &user) const {
return hash<string>()(user.name) ^ hash<int>()(user.age);
}
};
}
int main() {
User user1{"Alice", 30};
User user2{"Bob", 25};
std::unordered_map<User, std::string> userMap;
userMap[user1] = "Software Engineer";
userMap[user2] = "Data Scientist";
std::cout << user1.name << " is a " << userMap[user1] << std::endl;
return 0;
}
In this example, we create a custom hash function for the `User` structure, allowing it to be used in hash tables.
Overloading the Hash Function
Using `std::hash`
C++ provides a built-in `std::hash` class which can be overloaded for user-defined types, offering a straightforward method to create a hash function.
Code Example: Overloading for Custom Class
Here's how to leverage `std::hash` for a custom class:
#include <iostream>
#include <string>
#include <unordered_map>
class Person {
public:
std::string name;
int age;
Person(std::string n, int a) : name(n), age(a) {}
};
namespace std {
template <>
struct hash<Person> {
size_t operator()(const Person &p) const {
return hash<string>()(p.name) ^ hash<int>()(p.age);
}
};
}
int main() {
std::unordered_map<Person, std::string> people;
people[Person("Alice", 30)] = "Developer";
std::cout << "Alice: " << people[Person("Alice", 30)] << std::endl;
return 0;
}
This overload allows instances of `Person` to be used as keys in hash maps, leveraging our custom hash function.
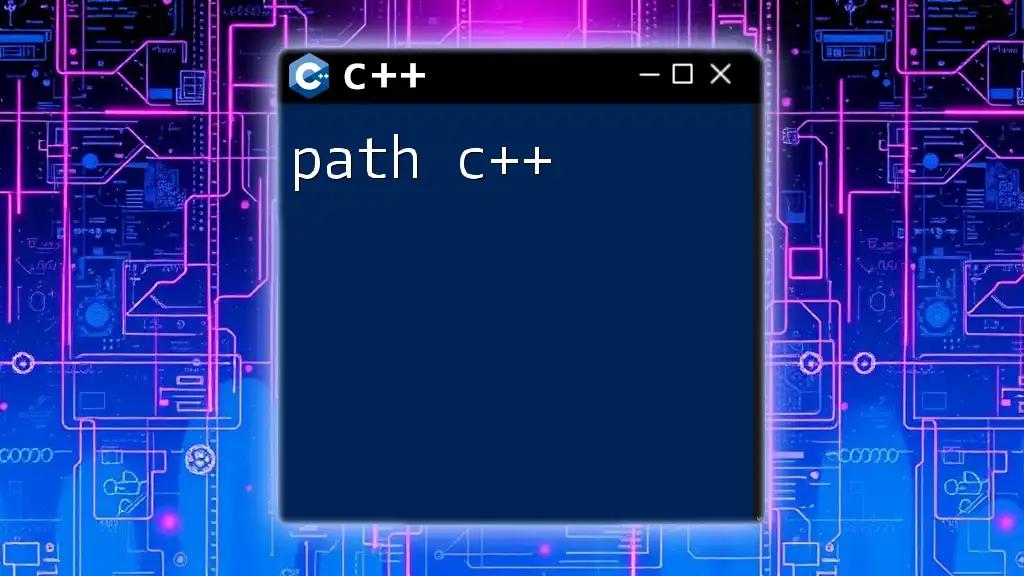
Performance Considerations
When implementing hashing solutions, consider both time and space complexity to ensure efficiency in your applications.
Time Complexity
The average-case time complexity for operations in hash tables is O(1). However, in the worst case, where many collisions occur, it can degrade to O(n). Understanding the behavior of your hash function is crucial to maintaining performance.
Space Complexity
Hash tables require additional space for the underlying array and can occasionally require resizing. Memory efficiency is vital, so consider the average size of inputs when designing your hash tables.

Best Practices for Hashing in C++
Choosing the Right Hash Function
Selecting a suitable hash function is fundamental. Factors to consider include:
- Consistency: Ensure that it produces unique outputs for varying inputs.
- Speed: Consider the computational overhead involved.
Avoiding Collisions
To minimize hash collisions, adopt strategies such as:
- Using well-distributed hash functions.
- Implementing a resizing strategy for your hash table when it exceeds load factors.
Security Implications
When handling sensitive data, use secure hashing algorithms, such as SHA-256, to avoid vulnerabilities that can be exploited. Always keep the potential for collisions in mind, especially in applications requiring high data integrity.
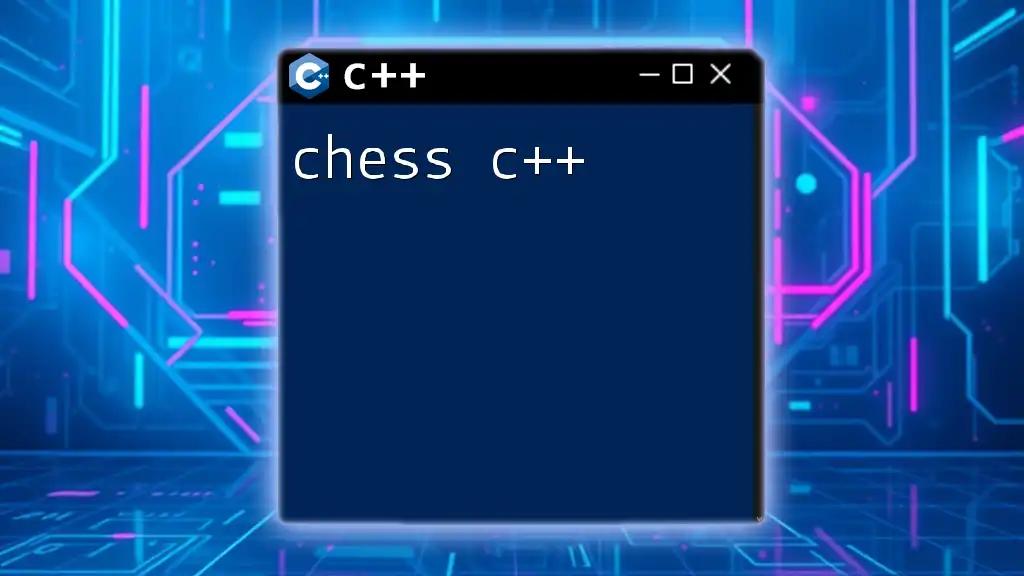
Conclusion
Hashing is a powerful concept in C++ that enhances data retrieval efficiencies and contributes significantly to various applications. By understanding the intricacies of hash functions, programmers can leverage hashing effectively in their code, enhancing both performance and security.
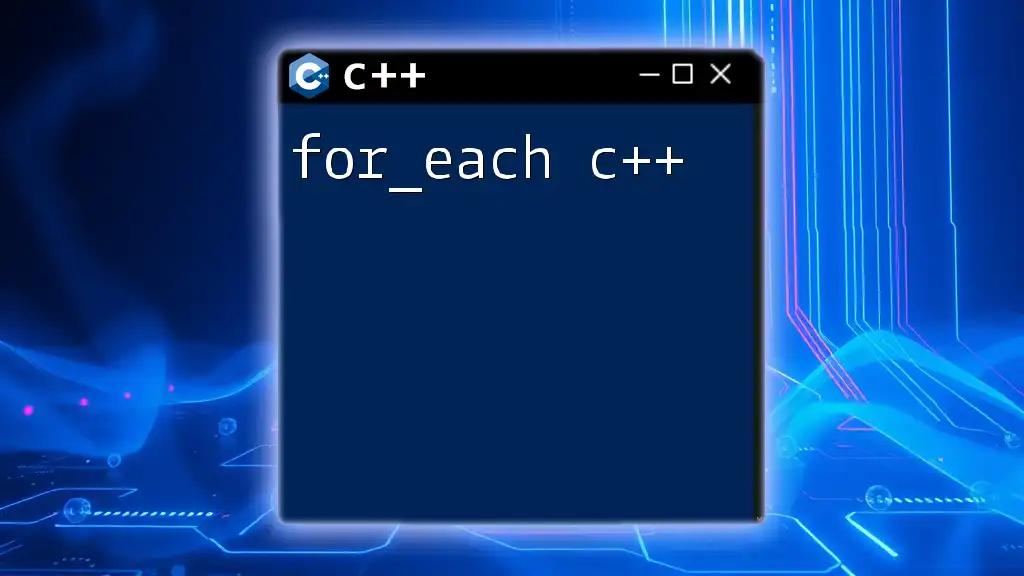
Additional Resources
For those looking to dive deeper into hashing techniques in C++, consider referring to books on advanced data structures, online courses focusing on cryptographic principles, and contributing to communities dedicated to C++ programming.
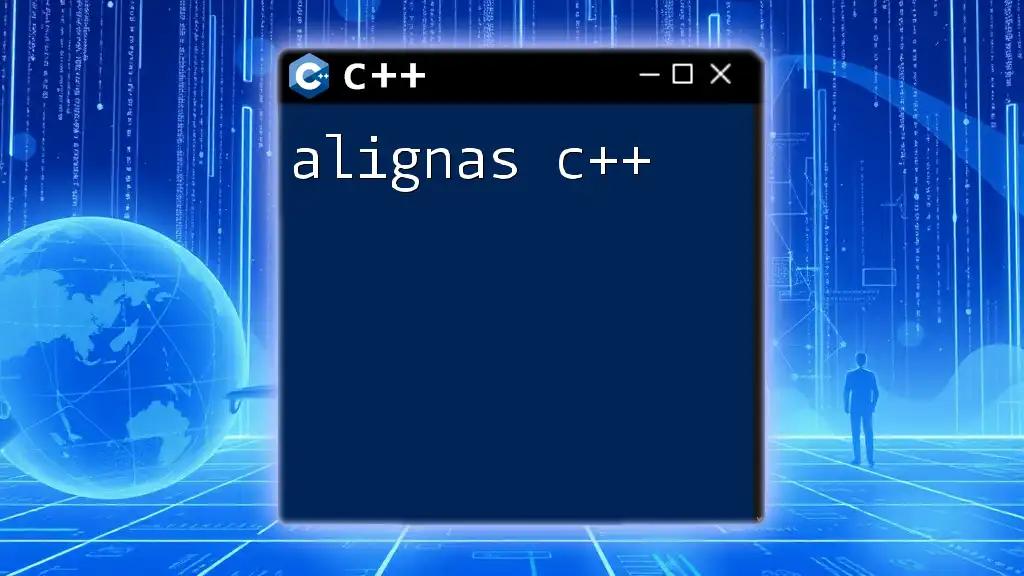
FAQs
-
What is a hash table? A hash table is a data structure that implements an associative array, allowing for efficient key-value pair management.
-
Can I use custom objects in hash tables? Yes, by defining your own hash function and ensuring it adheres to suitable properties, you can use custom objects in hash tables.
-
Are hash functions always secure? Not all hash functions are secure; always use hashing algorithms that are recognized for their security in cryptographic applications.