The `push` operation in C++ is commonly associated with adding elements to a data structure, such as a stack or a vector.
Here's a code snippet demonstrating how to use `push_back` with a `std::vector` in C++:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers;
numbers.push_back(10); // Adds 10 to the end of the vector
numbers.push_back(20); // Adds 20 to the end of the vector
for (int num : numbers) {
std::cout << num << " "; // Outputs: 10 20
}
return 0;
}
What is `push`?
In C++, the term `push` typically refers to the operation that adds an element to a data structure, such as a stack or vector. Understanding how this operation works in these contexts is crucial for effective data manipulation and management.
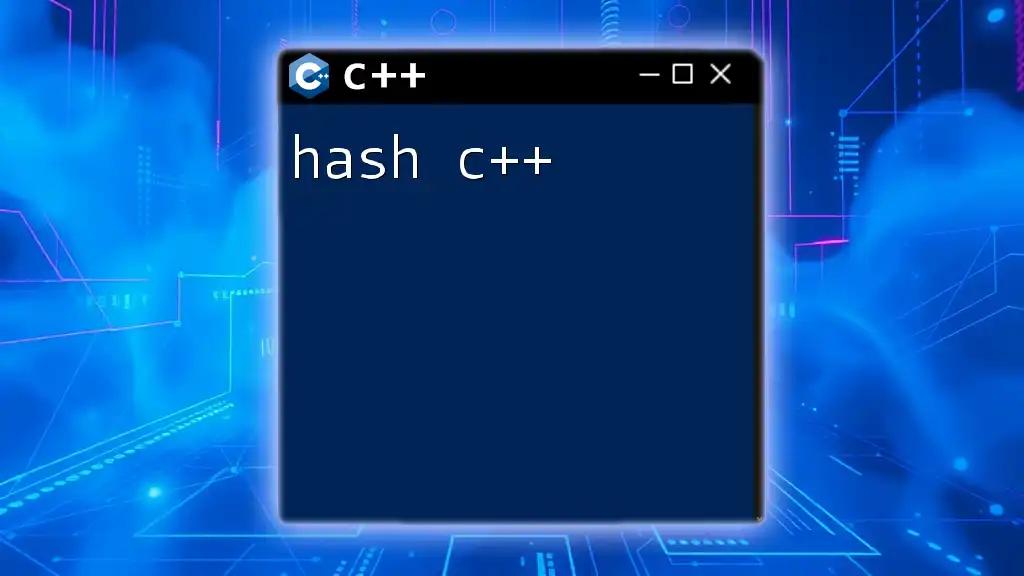
Importance of Understanding `push` in C++ Programming
Mastering the `push` operation is vital for any programmer working with data structures in C++. It allows you to efficiently manage collections of data, perform algorithmic processes, and maintain optimal performance across various applications.
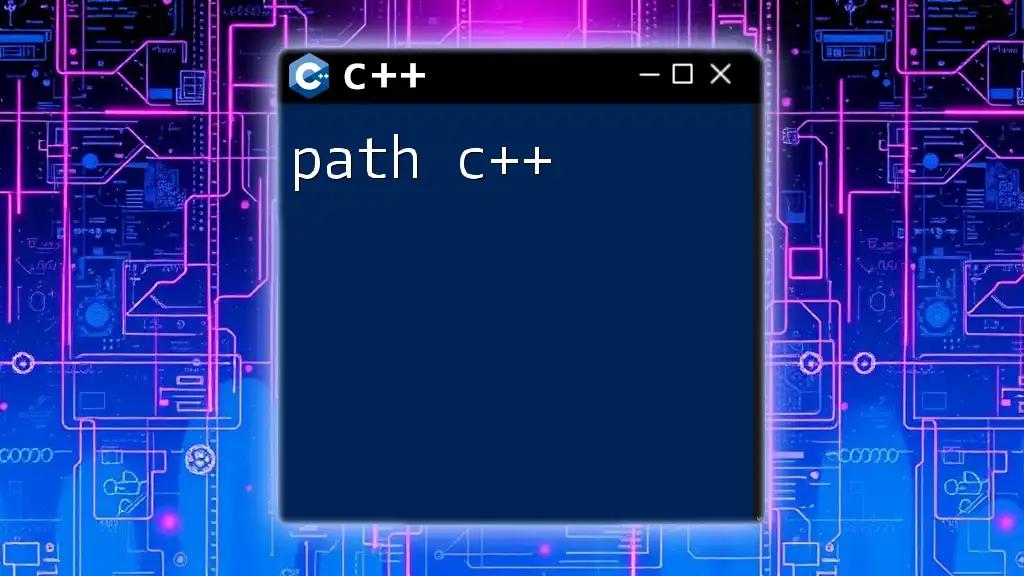
Understanding the Data Structures Involved
Stacks in C++
Definition and Properties of Stacks
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle, meaning the last element added is the first to be removed. This behavior makes stacks ideal for scenarios like reversing strings or parsing expressions.
How `push` Works in Stacks
When you use the `push` operation on a stack, it adds an element to the top of the stack. The stack grows in size with each `push`, allowing you to manage all kinds of data efficiently.
Example: Implementing a Basic Stack
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack; // Create a stack of integers
myStack.push(1); // Push 1 onto the stack
myStack.push(2); // Push 2 onto the stack
std::cout << "Top element: " << myStack.top(); // Outputs: Top element: 2
return 0;
}
In this example, we create a stack of integers and use the `push` method to add elements to the stack’s top. We then retrieve the top element using `.top()`, which operates directly on the most recently pushed data.
Vectors in C++
Definition and Properties of Vectors
A vector is a dynamic array that can grow and shrink in size. Unlike static arrays, a vector can automatically resize itself upon the addition of elements, representing a more flexible data structure.
How `push_back` Functions in Vectors
Vectors utilize the `push_back` operation, which appends an element to the end of the vector. Although similar to `push` in stacks, it's important to note that `push_back` adds to the back of the vector rather than the top.
Example: Using `push_back` for Vectors
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector; // Create a vector
myVector.push_back(5); // Push_back 5 into the vector
myVector.push_back(10); // Push_back 10 into the vector
std::cout << "Last element: " << myVector.back(); // Outputs: Last element: 10
return 0;
}
In this code snippet, we create a vector and add elements using the `push_back` method. The last element in the vector can be accessed using `.back()`, reflecting the most recently appended data.
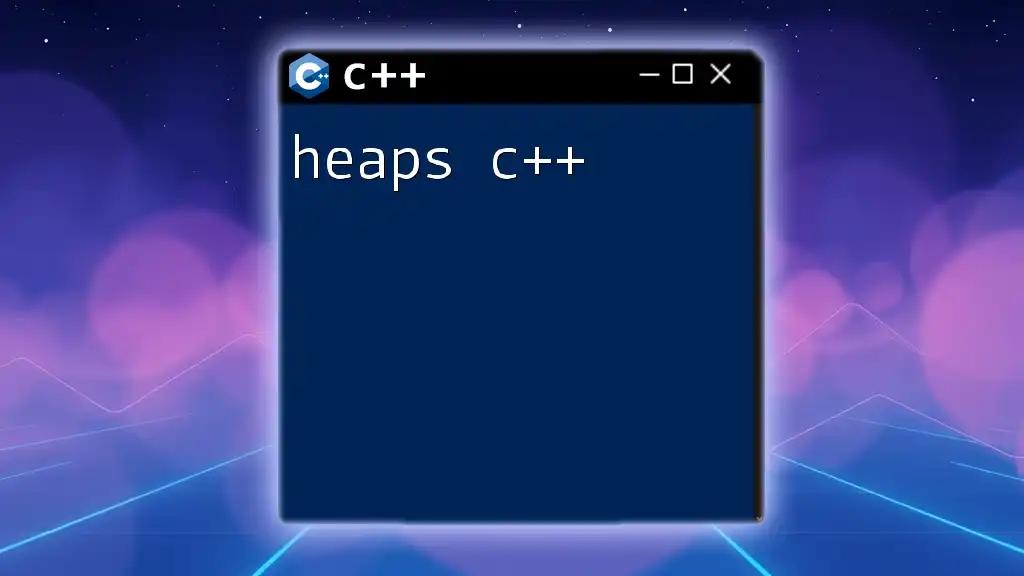
How to Use `push` Effectively
Best Practices for Using `push` in C++
To ensure efficient use of the `push` operation, consider the following best practices:
- Memory Management: Always be aware of how much memory is being allocated. Overusing `push` can lead to unnecessary allocations, especially in vectors.
- Performance: When frequently adding elements, consider the most appropriate data structure based on your needs, as linked lists may outperform stacks and vectors in some cases.
Common Pitfalls and How to Avoid Them
Programmers often encounter pitfalls when using `push`, such as:
- Over-pushing: Accidentally adding too many elements can lead to performance bottlenecks. To avoid this, always check the capacity and size of your data structure before pushing.
- Misusing stacks: Remember that stacks are strictly LIFO; using them as a queue could result in unintended behavior.

Alternatives to `push`
Other Data Structure Operations
In addition to `push`, there are other methods to consider depending on the required data structure. Operations like `insert` in vectors or `enqueue` in queues can provide similar functionalities but meet different scenarios.
When to Choose Alternatives
Choosing alternatives often depends on the necessary order of data retrieval. For instance, if FIFO (First In, First Out) behavior is required, using `enqueue` in queues is much more appropriate than `push` in stacks.

Conclusion
Understanding the `push` operation in C++ is crucial for working effectively with stacks and vectors. This knowledge enables programmers to efficiently manage data and choose the right strategies for data manipulation.
By mastering `push` and its alternative operations, programmers can enhance their C++ proficiency and build applications with greater efficiency and reliability.
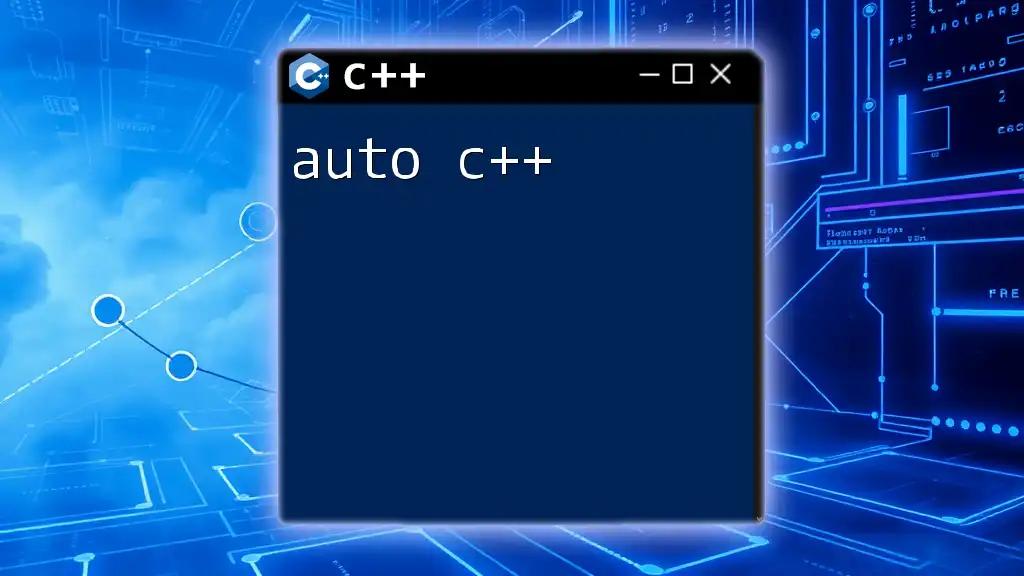
Additional Resources
If you are interested in deepening your understanding of C++ and data structures, consider exploring the following resources:
- Recommended Books: Look for books that focus on C++ data structures and algorithms.
- Online Courses: Platforms like Coursera and Udacity offer excellent courses on C++ programming.
- Community and Support Forums: Engage with communities on sites like Stack Overflow or specialized C++ forums to ask questions and share knowledge.