"VS C++ refers to the C++ programming language as utilized within Microsoft's Visual Studio, providing a robust integrated development environment (IDE) for building applications."
Here’s an example code snippet to illustrate a simple "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Visual Studio?
Visual Studio is an integrated development environment (IDE) created by Microsoft. It provides a comprehensive suite of tools that facilitate the development of applications across various platforms, including desktop, mobile, and web. With a focus on C++, Visual Studio enhances productivity through features that support coding, debugging, project management, and teamwork.
Advantages of Using Visual Studio for C++
Using Visual Studio for C++ development brings several benefits:
- User-Friendly Interface: Visual Studio's layout is intuitive, allowing developers to focus on coding without being overwhelmed by complexity.
- Integrated Debugging Tools: It provides robust debugging capabilities that help identify and resolve errors efficiently.
- Built-In Compiler and Code Editor: With a powerful editor and compiler, developers can write, test, and debug their C++ code seamlessly.

Setting Up Visual Studio for C++ Development
System Requirements
Before installing Visual Studio, ensure that your system meets the following minimum requirements:
- Operating System: Windows 10 (version 1909 or later) or Windows Server 2016.
- Processor: 1.8 GHz or faster; x64 architecture.
- RAM: At least 4 GB of RAM (8 GB recommended).
- Storage: Minimum of 6 GB available space, more for additional features.
Installation Steps
To get started with Visual Studio for C++, follow these steps:
- Download the Visual Studio installer from the official website.
- Run the installer and select the Desktop development with C++ workload, which includes all the necessary tools and libraries for C++ development.
- Choose any additional components that you may need, then click on Install.
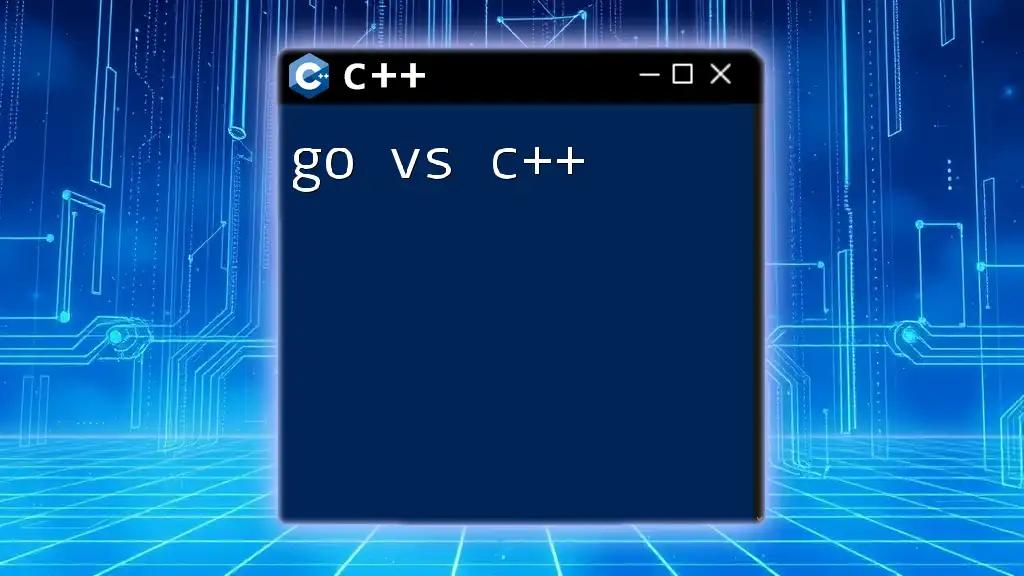
Navigating the Visual Studio Interface
The Main Components of Visual Studio
Upon launching Visual Studio, you'll notice several key components in the interface:
- Solution Explorer: Displays the files in your project and allows you to manage them easily.
- Editor Window: Where you write your C++ code. It features syntax highlighting and formatting.
- Output Window: Shows the results of build processes, errors, and output from running your program.
Customizing Visual Studio Environment
You can optimize your workflow by customizing Visual Studio to fit your preferences:
- Theme and Layout: Change the color scheme and layout based on your visual preferences. This can reduce eye strain and enhance focus.
- Workspace: Arrange panels and toolbars according to how you work best. You can even save different workspace configurations.
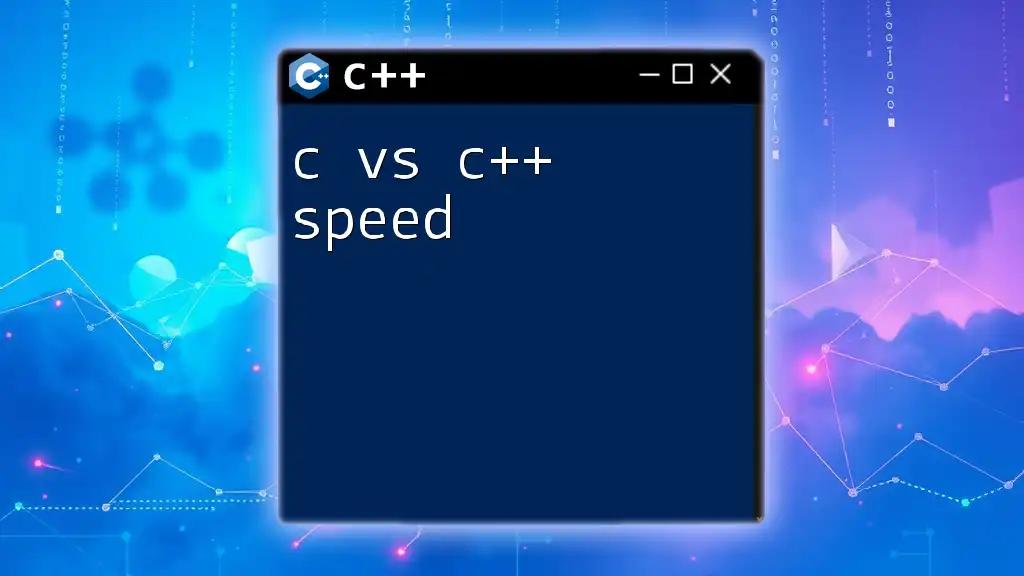
Creating Your First C++ Project in Visual Studio
Starting a New Project
Once your Visual Studio setup is complete, it's time to create your first C++ project:
- Open Visual Studio and select Create a new project.
- Choose Console App under the C++ category.
- Name your project and select a location to save it.
Here's a simple console application to get you started:
#include <iostream>
int main() {
std::cout << "Hello, Visual Studio C++!" << std::endl;
return 0;
}
Building and Running Your Project
After writing your code, it's essential to build and run the project:
- Building the Project: Click on Build in the menu and select Build Solution. Make sure there are no errors displayed.
- Running the Application: Once built, press `Ctrl + F5` to run your application. The output should display the message "Hello, Visual Studio C++!".
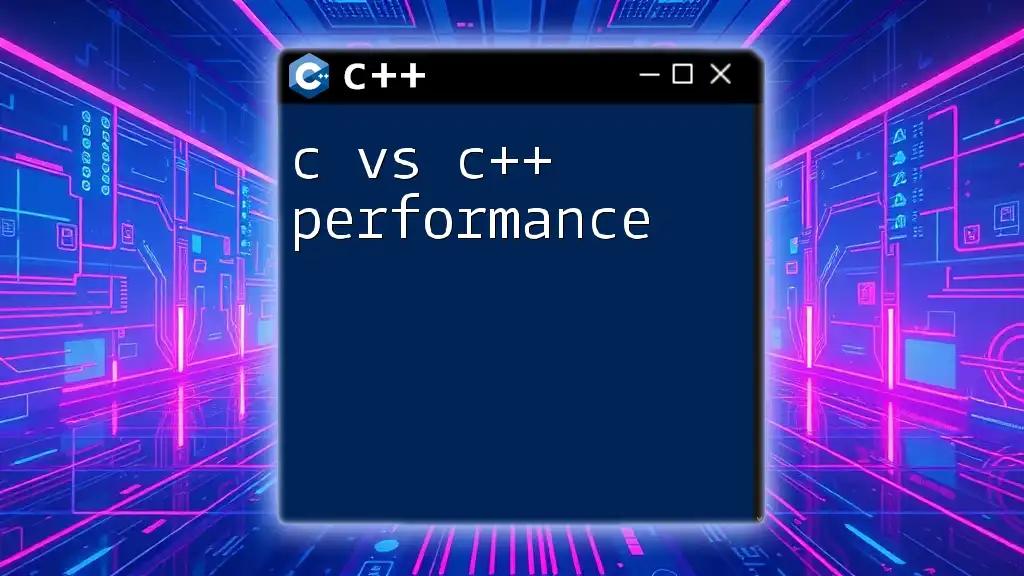
Understanding Key Features of Visual Studio for C++
IntelliSense
IntelliSense is one of the standout features of Visual Studio:
- Code Completion: As you start typing, IntelliSense provides suggestions, making coding faster and reducing typographical errors.
- Parameter Info: When calling functions, hovering over them reveals parameter information, making it easier to understand how to use them correctly.
Debugging Tools
Debugging is crucial in software development, and Visual Studio offers powerful debugging tools:
- Breakpoints: You can set breakpoints by clicking in the margin of your code window. This pauses execution at a specific line, allowing you to inspect values.
- Variable Inspection: During a debugging session, hover over variables to see their current values, helping you identify issues effectively.
Code Refactoring
Refactoring tools in Visual Studio help maintain clean and efficient code:
- Renaming Variables: Easily rename variables by right-clicking and selecting Rename. Visual Studio updates all references automatically, preventing errors.
- Extracting Methods: You can extract a block of code into a separate method, improving the organization and readability of your code.
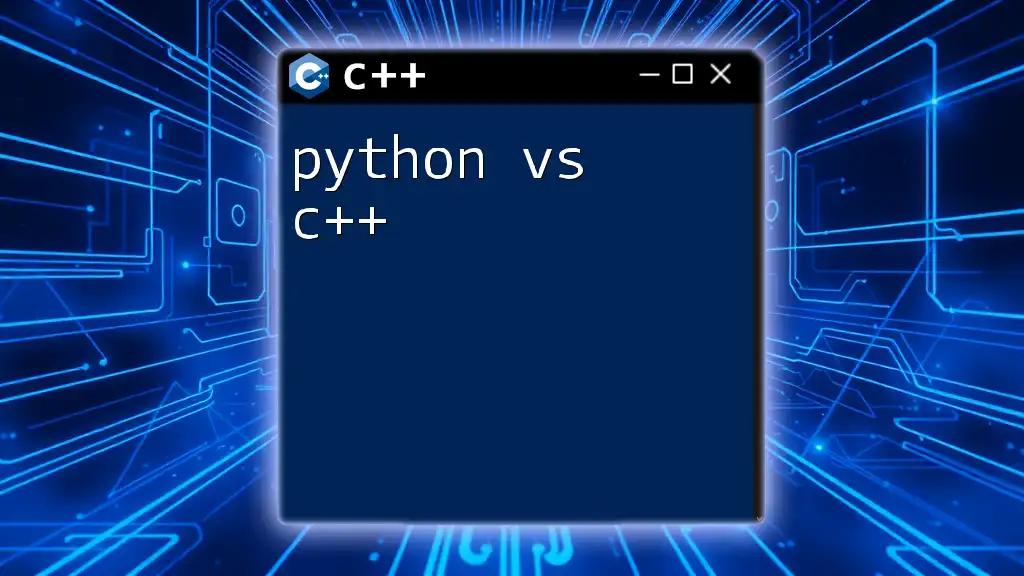
Advanced Features of Visual Studio for C++
Integration with Git
Visual Studio smoothly integrates with Git, enabling efficient version control management:
- You can initialize a new Git repository directly from the IDE, making it easier to manage code changes.
- This integration allows for streamlined commits, branches, and merges without needing to leave the development environment.
Visual Studio Extensions
Enhance your development experience with additional extensions:
- Popular extensions like ReSharper and Visual Assist provide additional coding assistance, improving productivity.
- Install and manage extensions directly from the Visual Studio Marketplace for a customization that fits your needs.
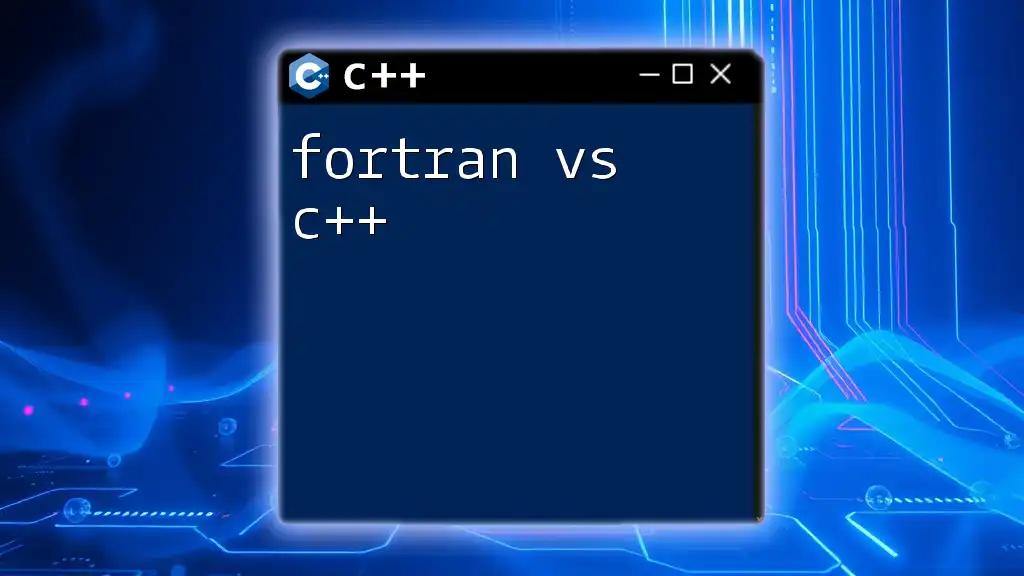
Tips and Tricks for Efficient C++ Development
Best Practices
Adhering to best practices in C++ development is essential for producing high-quality, maintainable code:
- Coding Standards: Follow common C++ coding standards for naming conventions, indentation, and commenting. Consistency in your coding style will make your code more readable.
- Project Organization: Structure your project files logically. Group related files together and keep your codebase tidy.
Troubleshooting Common Issues
As a beginner, you may encounter some common problems:
- Build Errors: If you receive build errors, check for typos or missing semicolons. The Output Window can provide insights into what went wrong.
- Debugger Issues: If breakpoints aren’t working, ensure the project is built in Debug mode instead of Release mode.
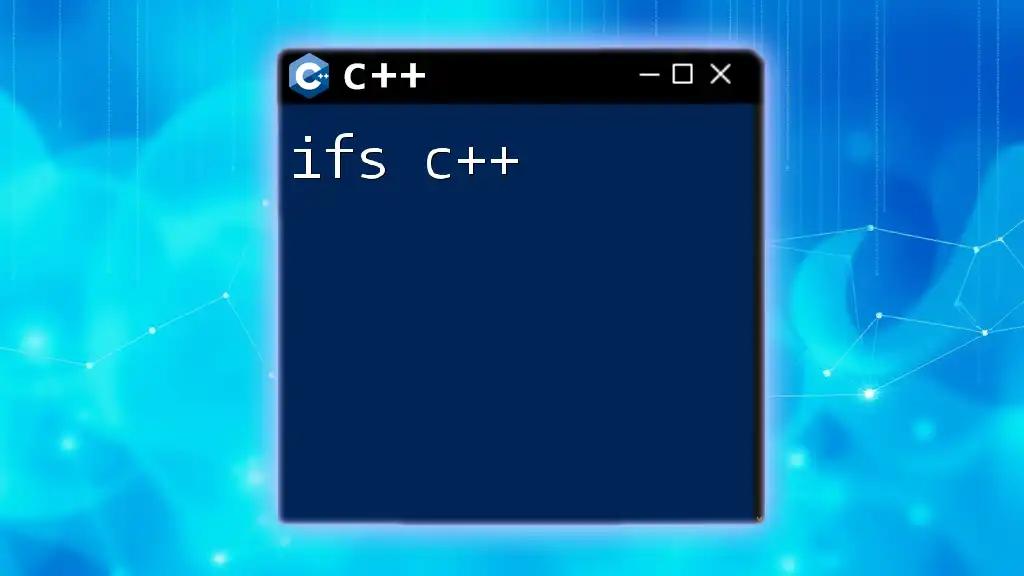
Conclusion
Using Visual Studio for C++ development provides a powerful and intuitive environment that enhances productivity for both beginners and experienced developers. Its robust features, such as integrated debugging, IntelliSense code completion, and easy Git integration, make it an ideal choice for writing efficient C++ code.
As you dive deeper into C++, don’t hesitate to explore the myriad of tools and resources available within Visual Studio. Happy coding!
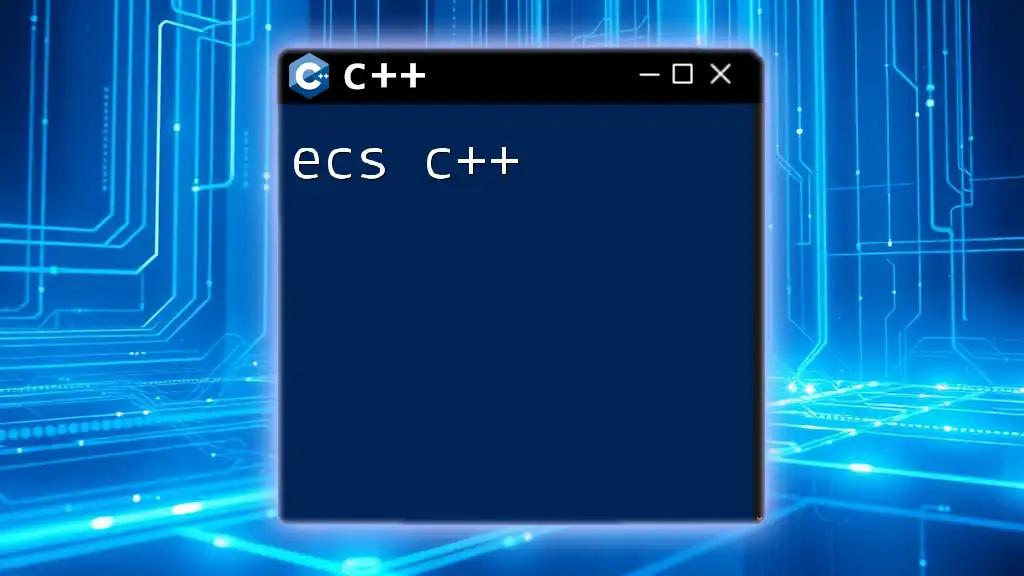
Additional Resources
Learning C++
For those looking to further their C++ knowledge, consider exploring various resources such as:
- Books: Look for titles like "Effective C++" by Scott Meyers.
- Websites: Platforms like Codecademy and Coursera offer excellent C++ courses.
Visual Studio Documentation
To get the most out of Visual Studio, refer to the [official Microsoft documentation](https://docs.microsoft.com/en-us/visualstudio/) for detailed guides and updates.
Community and Support
Engage with community forums, such as Stack Overflow or Microsoft Developer Community, for additional support and to connect with other developers.