The `fabs` function in C++ is used to compute the absolute value of a floating-point number.
Here’s a code snippet demonstrating its use:
#include <iostream>
#include <cmath>
int main() {
double number = -5.7;
double absoluteValue = fabs(number);
std::cout << "The absolute value of " << number << " is " << absoluteValue << std::endl;
return 0;
}
Understanding the fabs Function
Definition and Purpose
The `fabs` function in C++ is a standard library function that is specifically used to compute the absolute value of a floating-point number. When we talk about absolute values, we refer to the non-negative value of a number regardless of its sign. This function is crucial in various mathematical computations where negative values need to be converted to their positive counterparts for analysis, calculations, or conditions.
Key Benefits of Using fabs:
- It handles floating-point values efficiently.
- It ensures that the results maintain precision by returning a double type.
Syntax of fabs
The syntax for the `fabs` function is straightforward:
double fabs(double x);
Parameters:
- `x`: This parameter represents the floating-point number for which you want to find the absolute value. It could be any valid double value, including negative numbers.
Return Value:
- The function returns the absolute value of `x` as a `double`. If `x` is negative, it converts it to a positive value, and if `x` is already positive, it returns it unchanged.
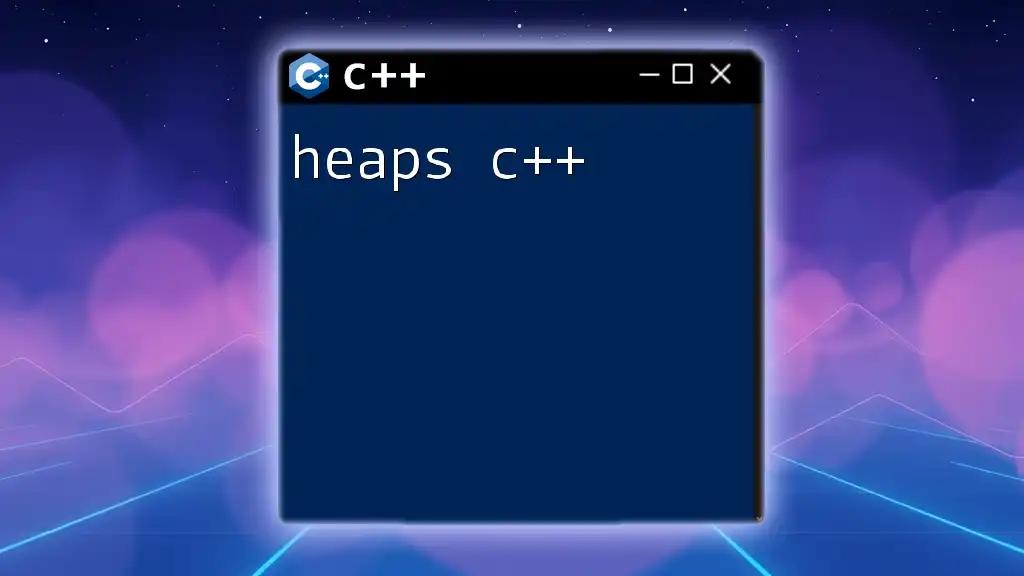
How to Use fabs in C++
Including Required Headers
To use the `fabs` function, you must include the appropriate header file. This can be done by adding the following line at the beginning of your program:
#include <cmath>
Basic Examples of fabs
Let’s start with a simple example to illustrate the use of `fabs`. Here is a basic program that demonstrates how to find the absolute value of a negative number:
#include <iostream>
#include <cmath>
int main() {
double num = -5.5;
double result = fabs(num);
std::cout << "The absolute value of " << num << " is " << result << std::endl;
return 0;
}
In this example:
- We declare a variable `num` with a negative value.
- We then use `fabs(num)` to compute the absolute value, which produces an output of `5.5`.
Advanced Examples
Using fabs in Calculations
The `fabs` function can often be used in calculations where you need to find differences or other mathematical operations. Consider the following example where we calculate the absolute difference between two numbers:
double a = -3.7;
double b = 2.5;
double difference = fabs(a - b);
std::cout << "The absolute difference is " << difference << std::endl;
In this code:
- We compute the difference between `a` and `b`. Since `a` is negative, using `fabs` helps us get the non-negative outcome for the difference, which is useful in various applications, such as physics simulations or real-time data processing.
Integrating fabs with Other Functions
You can easily integrate `fabs` into more extensive mathematical formulas. Here’s an example that calculates the product of the absolute values of two numbers:
double x = -4.2;
double y = 3.1;
double product = fabs(x) * fabs(y);
std::cout << "The product of absolute values is " << product << std::endl;
In this case:
- Both `x` and `y` are passed through `fabs` to ensure the product is calculated with their absolute values, which is particularly useful in operations where direction does not matter, such as calculating distances.
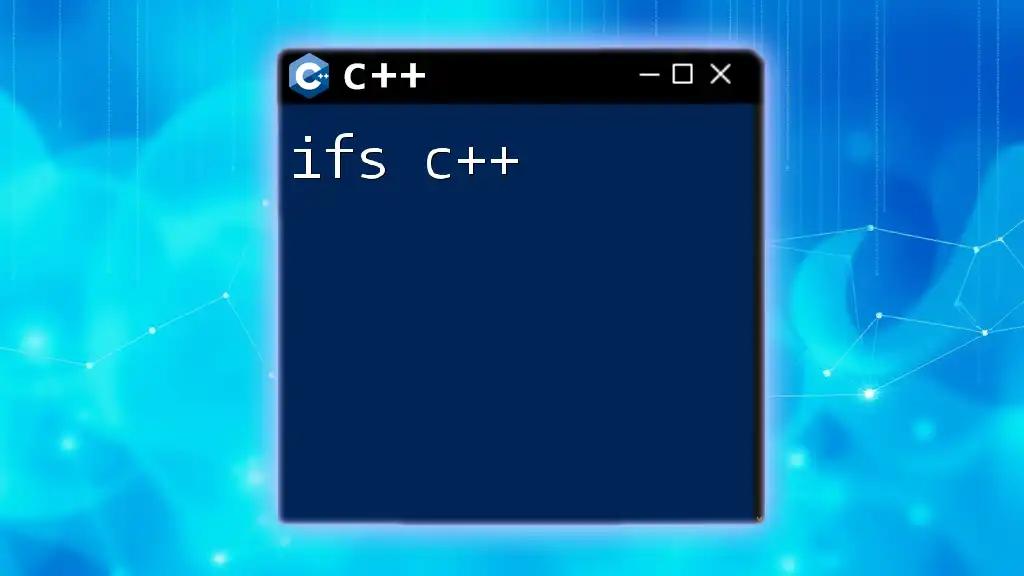
Common Mistakes When Using fabs
Misinterpretation of Results
One common mistake when using the `fabs` function is misinterpreting the results, especially if you're new to C++. Floating-point calculations can sometimes lead to unexpected results due to precision issues inherent to binary representation. It’s important to ensure you understand how types are stored and computed in C++ to avoid unnecessary confusion.
Performance Considerations
While `fabs` is optimized for floating-point operations, there are scenarios where other algorithms may provide better performance for specific types of calculations. Understanding the context in which you’re working will help you decide whether to use `fabs` or consider other methods. For instance, in high-performance computing, you might perform benchmarking to see if using `fabs` is efficient for your specific application.
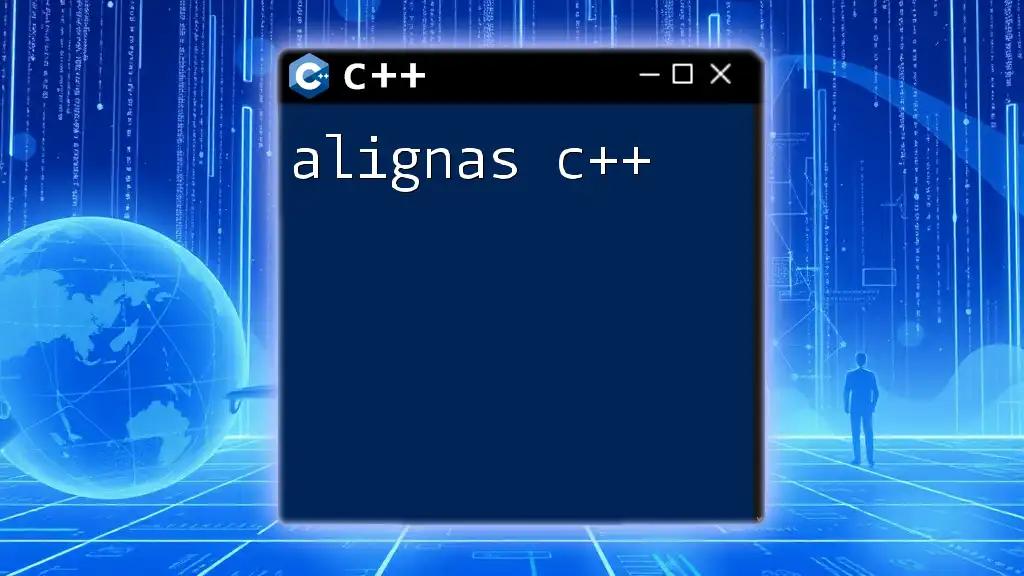
Best Practices for Using fabs in C++
Readability and Maintainability
To write clean and maintainable code, always ensure that your use of `fabs` does not compromise the readability of your program. Make it clear why you rely on `fabs`, especially when the logic might be complex. Commenting on the rationale behind using `fabs` can help others (or even yourself later on) understand the intent of the code.
Debugging Tips
Debugging issues related to the `fabs` function can be straightforward but requires attention to detail. If you face unexpected results, consider:
- Checking the input values carefully to ensure they are what you expect.
- Using print statements to monitor the values at each step of your calculations.
- Understanding how floating-point arithmetic works in C++ can help you grasp the results produced by the `fabs` function.
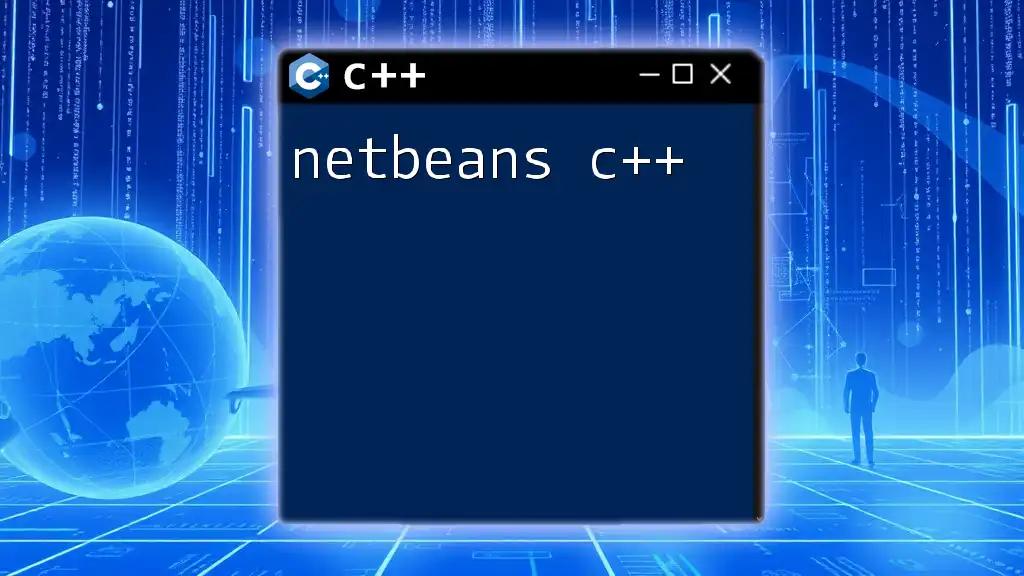
Conclusion
In summary, the `fabs` function in C++ is an essential tool for working with floating-point numbers, providing a reliable means to compute absolute values. Its straightforward syntax and powerful application in calculations make it a must-know for any programmer working with numerical data. By understanding its purpose, potential pitfalls, and best practices, you can effectively harness the capabilities of `fabs` in your C++ projects.
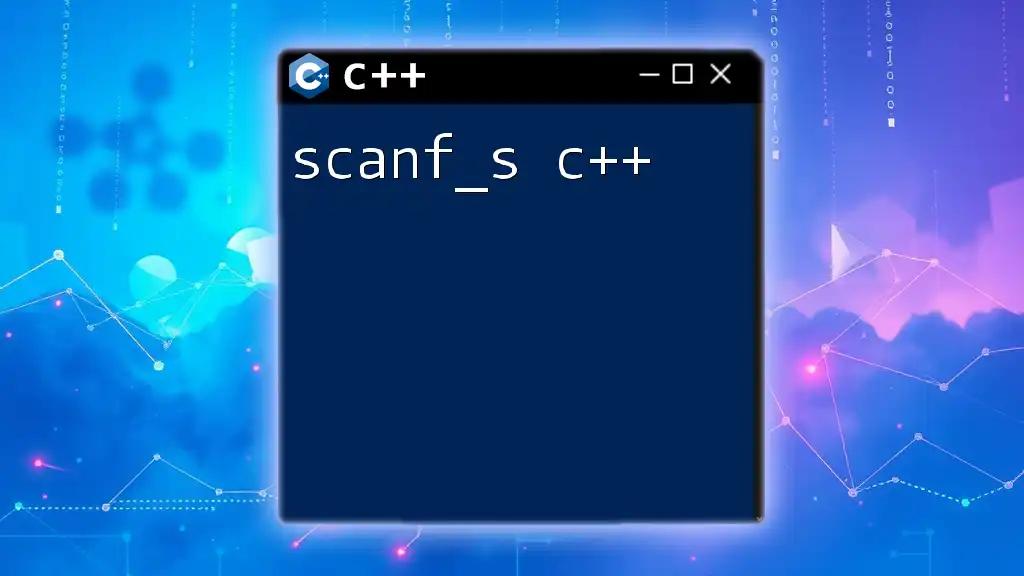
Additional Resources
For further reading on mathematical functions and their applications in C++, it may be beneficial to explore reputable C++ programming books, online forums, and documentation from the C++ standards committee.
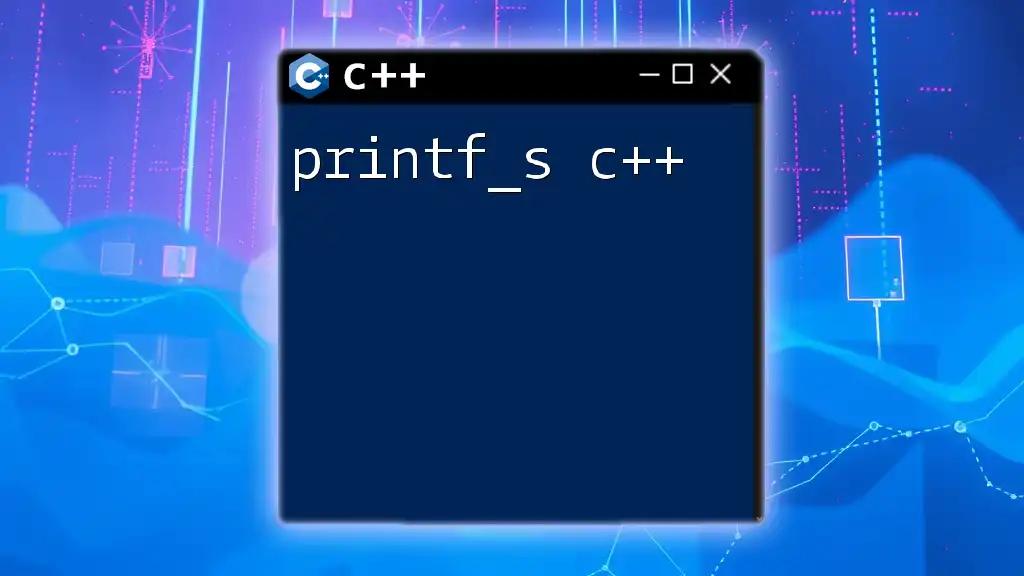
FAQ about fabs in C++
What is the difference between fabs and abs?
The `fabs` function is specifically designed for floating-point numbers, while `abs` can work with both integer and floating-point types. If you use `abs` with a floating-point number, it may result in implicit type conversion, which may lead to performance issues or unexpected behavior.
Can fabs be used with integers?
While `fabs` specifically returns a double, if you pass an integer, C++ will implicitly convert it to a double, making it possible to use `fabs` with integer values. However, if you are looking for the absolute value of integers explicitly, using `abs` is more appropriate.