The `make` command in C++ is commonly used to automate the building process of C++ programs from source code using a Makefile, which defines the rules and dependencies for compiling the code.
Here's a basic example of a Makefile for a simple C++ project:
# Makefile
# Compiler
CXX = g++
# Source files
SRCS = main.cpp utils.cpp
# Output executable
OUT = my_program
# Build rule
all: $(OUT)
$(OUT): $(SRCS)
$(CXX) -o $(OUT) $(SRCS)
# Clean rule
clean:
rm -f $(OUT)
To build the program, simply run the command `make` in the terminal.
Understanding Build Automation
What is Build Automation?
Build automation refers to the process of automating the tasks involved in building software from source code. It streamlines the compilation, linking, and packaging tasks required to turn raw code into an executable or library. Without build automation, developers often have to run multiple commands manually, which can be error-prone and time-consuming.
Why Use `make`?
Using `make` in your C++ projects provides several advantages:
- Efficiency: `make` only recompiles files that have changed, speeding up the build process significantly.
- Consistency: By using a Makefile, you ensure that builds are reproducible across different environments.
- Simplification: `make` handles the complexity of dependency management, allowing developers to focus on writing code rather than managing builds.
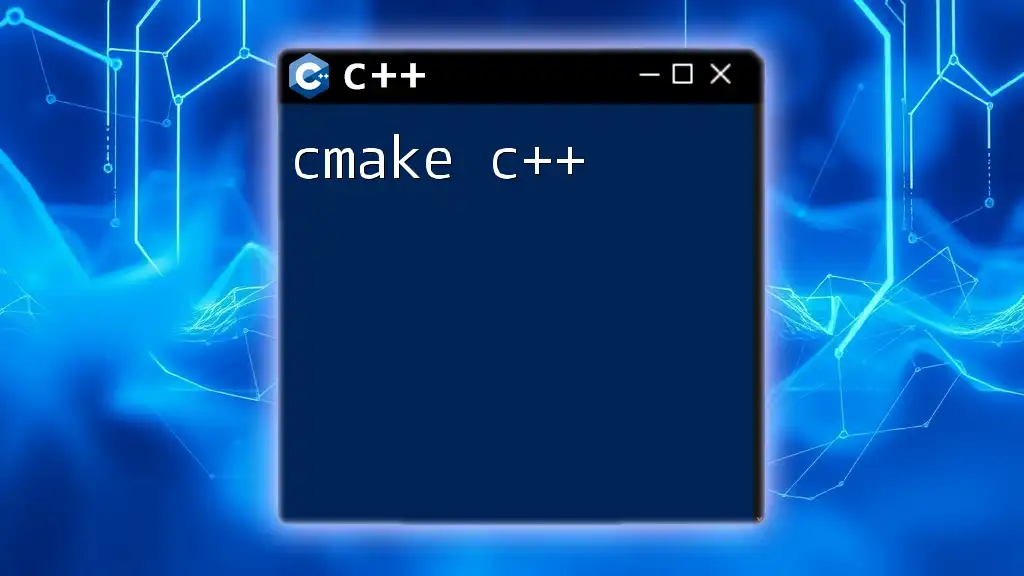
The Basics of the `Makefile`
What is a Makefile?
A Makefile is a special file that defines a set of tasks to be executed. It contains the rules about how to build different targets such as executable programs or libraries. A Makefile is organized into targets, prerequisites, and recipes.
- Targets: The output files you want to create (e.g., `.o` files, executables).
- Prerequisites: Files required to create the target (e.g., source files).
- Recipes: Commands to build the target (e.g., compiler commands).
Creating a Simple Makefile
To get started, you need to write a simple Makefile. Here’s a basic example that compiles a main program alongside a helper file:
all: main
main: main.o helper.o
g++ -o main main.o helper.o
main.o: main.cpp
g++ -c main.cpp
helper.o: helper.cpp
g++ -c helper.cpp
In this example:
- The `all` target depends on the `main` target.
- The `main` target depends on two object files: `main.o` and `helper.o`.
- Each object file has its own rule specifying how to compile it.
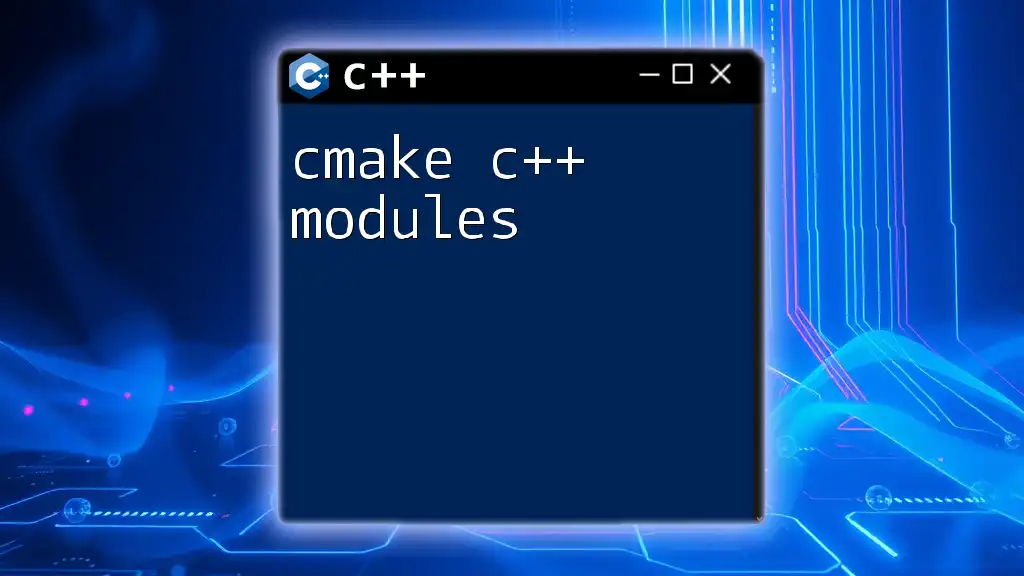
Common `make` Commands
Running Make
To execute a Makefile, you simply type the `make` command in your terminal. By default, it builds the first target defined in the Makefile. For example:
make
This command will build the `all` target in our previous example. Similarly, you can run:
make clean
This would remove any generated object files or executables, assuming you've defined a `clean` target.
Specifying Targets
If you want to build a specific target, you can specify it in the command line. For instance:
make main
This command will trigger the build process for the `main` target specifically.
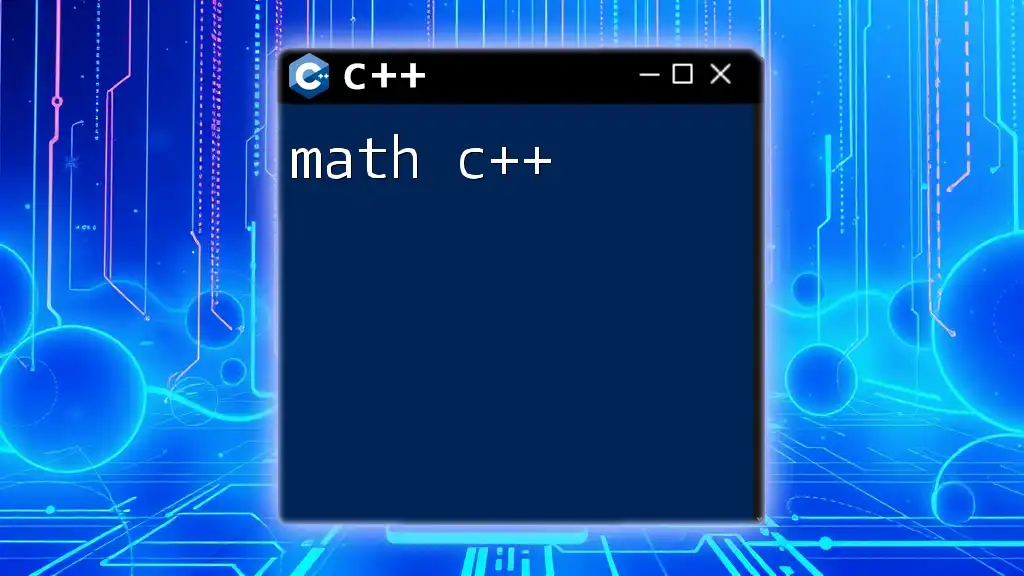
Advanced Makefile Features
Variables in Makefiles
Makefiles support variables, which allow you to define reusable values, enhancing flexibility and maintainability. Here's an example that makes use of variables for the compiler and compiler flags:
CC = g++
CFLAGS = -c -Wall
all: main
main: main.o helper.o
$(CC) -o main main.o helper.o
main.o: main.cpp
$(CC) $(CFLAGS) main.cpp
In this case, `CC` and `CFLAGS` can be easily modified in one location, which automatically updates all relevant commands.
Pattern Rules
Pattern rules enable you to define generic recipes for files that share the same structure. Here’s an example:
%.o: %.cpp
$(CC) $(CFLAGS) $<
This pattern compiles all `.cpp` files into corresponding `.o` files, making your Makefile cleaner and easier to manage.
Conditional Statements
You can control the behavior of your Makefile using conditional statements. This allows you to customize builds, such as enabling debugging:
ifeq ($(DEBUG), 1)
CFLAGS += -g
endif
This snippet adds the `-g` flag to the `CFLAGS` if the `DEBUG` variable is set to `1`.
Phony Targets
Phony targets are not associated with files; they are simply commands you want to invoke. This is particularly useful for commands like `clean`:
.PHONY: clean
clean:
rm -f *.o main
This ensures that the `clean` target will always run the associated commands, even if a file named `clean` exists.
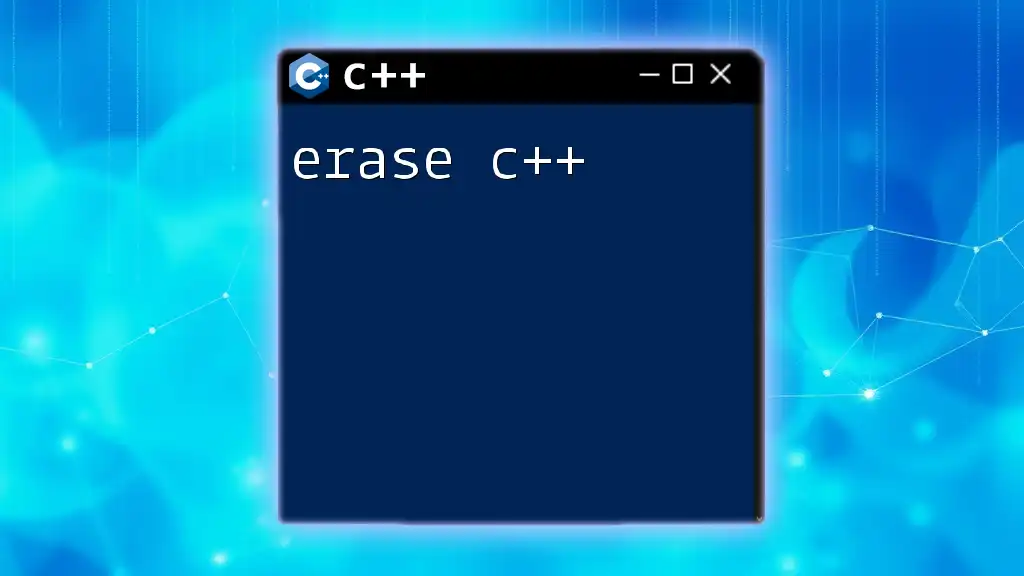
Troubleshooting Common Issues
Debugging Your Makefile
When developing a Makefile, you may encounter errors or unexpected behavior. Common issues include:
- Missing prerequisites: Ensure all required files exist.
- Typos in commands: Double-check command syntax.
- Invalid paths: Verify that file paths in the Makefile are correct.
Verbose Output
To better understand what `make` is doing, you can enable verbose output. This can be very helpful for debugging:
make --debug
This command provides extensive information about which targets are being built, which prerequisites are missing, and more.
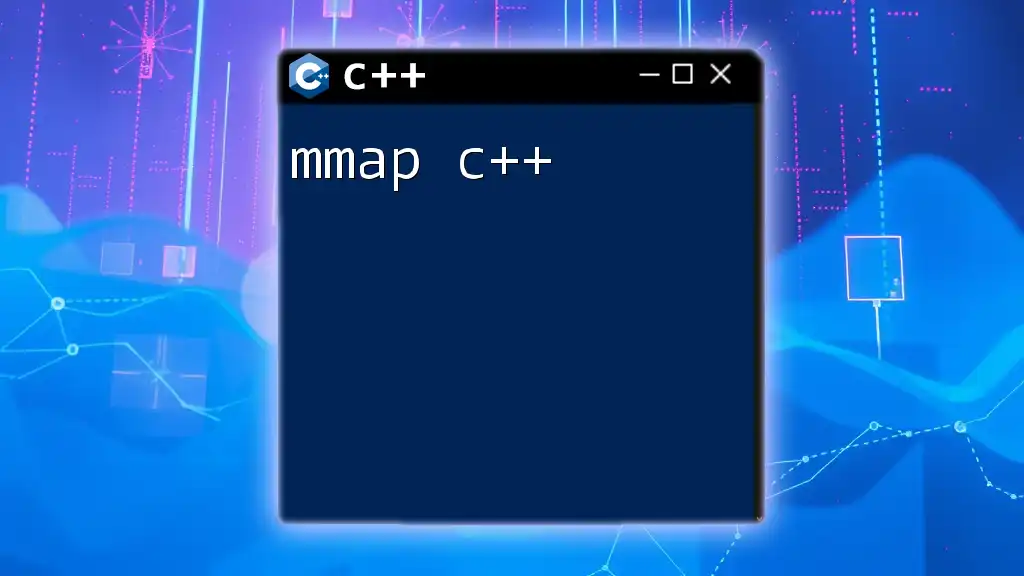
Best Practices for Writing Makefiles
Organizing Your Makefile
For larger projects, keep your Makefile organized. Group related targets logically, and separate sections with comments for clarity. Here's an example of a well-organized Makefile:
# Compilation variables
CC = g++
CFLAGS = -c -Wall
# Targets
.PHONY: all clean
all: main
# Build rules
main: main.o helper.o
$(CC) -o main main.o helper.o
# Object files
%.o: %.cpp
$(CC) $(CFLAGS) $<
# Clean rule
clean:
rm -f *.o main
Documenting Your Makefile
Adding comments throughout your Makefile can improve understanding for others (and yourself) who may modify it in the future. Document important sections and explain complex commands:
# This target builds the main executable
main: main.o helper.o
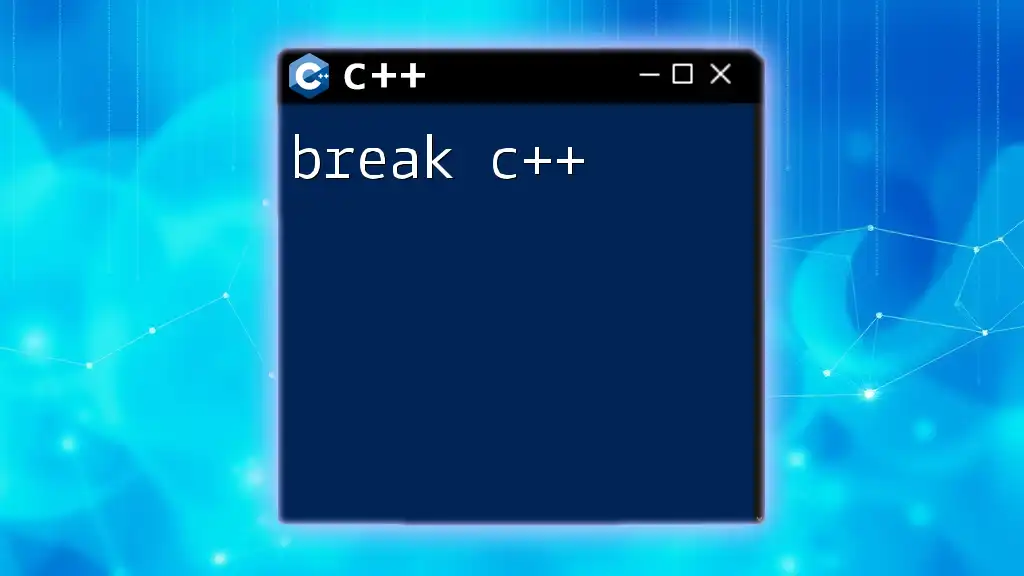
Conclusion
In summary, the `make` command is an invaluable tool in C++ development, simplifying the build process and ensuring consistency. Mastering the art of writing Makefiles can significantly enhance your productivity. As you continue to explore and practice using `make` in your C++ projects, you'll unlock greater efficiency and flexibility in your development workflow.
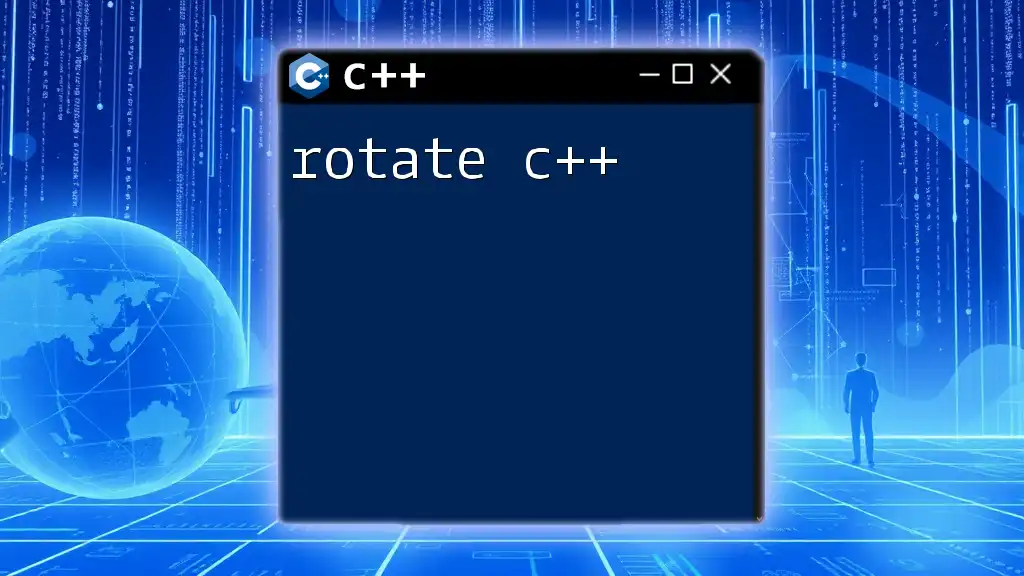
Call to Action
We would love to hear about your experiences with `make` and any tips you have for fellow developers! Be sure to subscribe to our blog for more concise C++ tutorials and practical programming tips.