`mmap` in C++ is a system call that maps files or devices into memory, allowing for file manipulation through memory operations, which can enhance performance compared to traditional file I/O methods.
Here's a simple code snippet demonstrating the use of `mmap`:
#include <sys/mman.h>
#include <fcntl.h>
#include <unistd.h>
#include <iostream>
int main() {
int fd = open("example.txt", O_RDONLY);
if (fd == -1) {
perror("open");
return 1;
}
size_t length = 100; // Length to map
char *mapped = (char *)mmap(nullptr, length, PROT_READ, MAP_PRIVATE, fd, 0);
if (mapped == MAP_FAILED) {
perror("mmap");
close(fd);
return 1;
}
std::cout << "Mapped content: " << mapped << std::endl;
munmap(mapped, length);
close(fd);
return 0;
}
What is mmap?
Definition and Purpose
mmap (memory map) is a powerful mechanism used in C++ that allows developers to map files or devices into memory. By creating a direct link between a file on disk and a location in memory, it enables efficient input/output operations and allows the processor to manipulate file data as if it were part of the program’s memory space. This is especially useful for applications that require large data sets or need high-performance file operations.
How mmap Works
When a file is mapped into memory using `mmap`, the operating system creates a memory address that can be used to read or write data directly to and from that file. This process not only speeds up file access but also eliminates the need for explicit read and write system calls. Rather than moving data between user space and kernel space, `mmap` can directly interface with the file. As a result, any modifications made to the memory region are directly reflected in the underlying file.
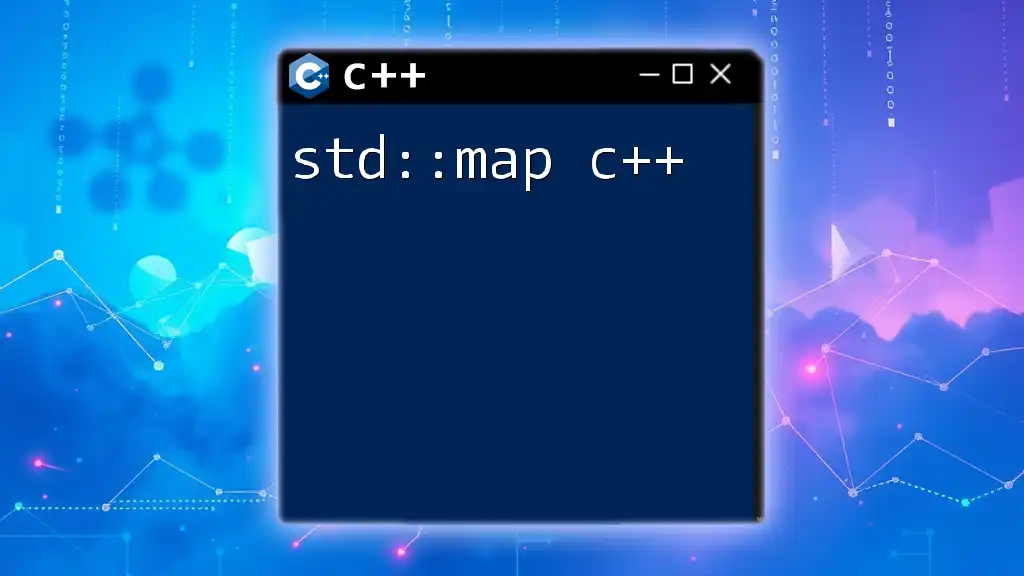
Key Concepts of mmap in C++
The mmap Function Prototype
The basic structure of the mmap function call is as follows:
void* mmap(void *addr, size_t length, int prot, int flags, int fd, off_t offset);
Understanding each parameter is crucial for effective usage:
- addr: This is the starting address for the mapping. If set to `NULL`, the kernel chooses the address.
- length: Specifies the size of the mapping in bytes.
- prot: Defines allowable memory protection. Options include:
- `PROT_READ`: Pages may be read.
- `PROT_WRITE`: Pages may be written.
- `PROT_EXEC`: Pages may be executed.
- flags: Provide options for the mapping, with `MAP_SHARED` (modifications are visible to other processes) and `MAP_PRIVATE` (modifications are not visible to others) being the most common.
- fd: This is the file descriptor referring to the file being mapped.
- offset: The starting point in the file from which mapping begins.
Memory Protection Levels
Memory protection is a vital concept when using mmap. It ensures that areas of memory are accessed properly, preventing unintended modifications that can lead to data corruption or application crashes. Depending on the combination of protection flags used, mmap can permit different types of access to the mapped memory, making it essential to choose these flags wisely.
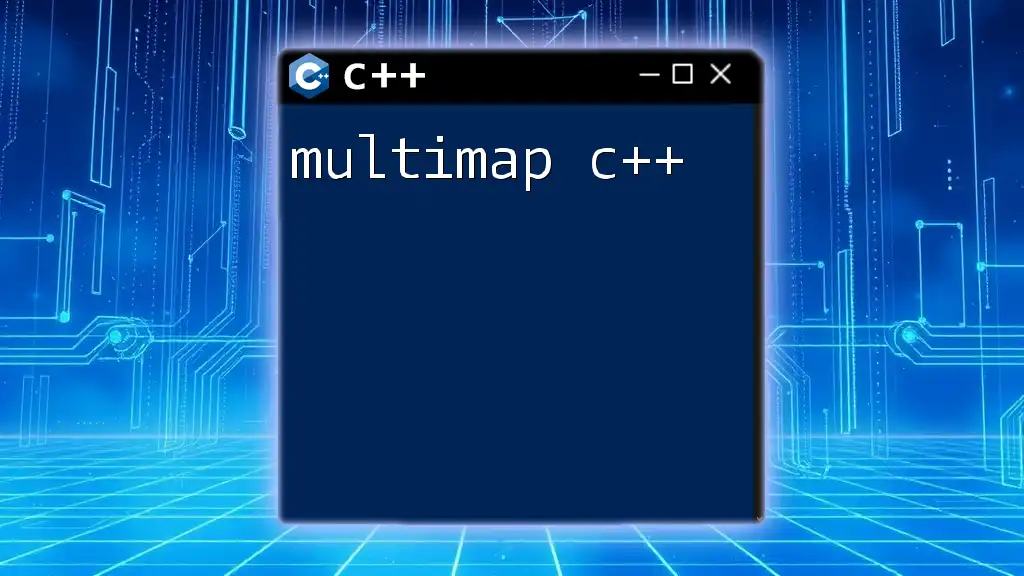
Setting Up mmap in C++
Required Headers
To utilize mmap correctly, you'll need to include specific headers in your C++ program, like so:
#include <sys/mman.h>
#include <fcntl.h>
#include <unistd.h>
Sample Code - Opening a File for Mapping
Before mapping a file, it is essential to open it correctly to obtain a valid file descriptor. Here’s how you can do that:
int fd = open("example.txt", O_RDWR);
if (fd == -1) {
perror("Error opening file");
return -1;
}
This code attempts to open "example.txt" in read/write mode with `O_RDWR`. If the open call fails, it prints an error message using perror and exits.
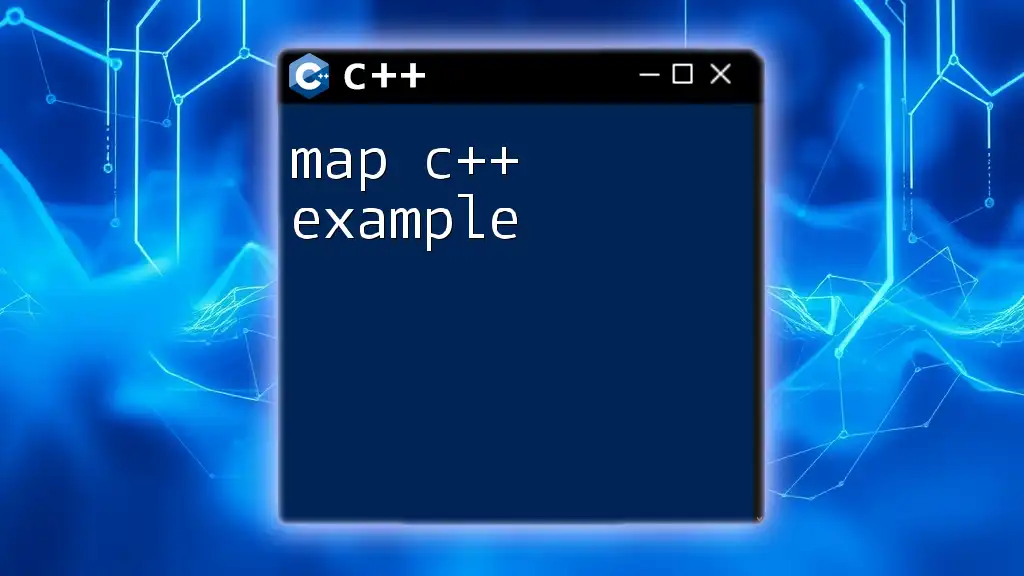
Creating a Memory Mapping
The mmap Call
After opening a file, you can create a memory mapping. Below is a simple example of how to do this:
size_t length = /* size of the file */;
void* map = mmap(NULL, length, PROT_READ | PROT_WRITE, MAP_SHARED, fd, 0);
if (map == MAP_FAILED) {
perror("mmap failed");
close(fd);
return -1;
}
Explanation of the Code
In this snippet:
- length should be set to the size of the file you want to map.
- The call to `mmap` requests mapping, with permissions set to allow both reading and writing in a shared manner.
- If `mmap` returns `MAP_FAILED`, an error occurred, and appropriate handling is required.
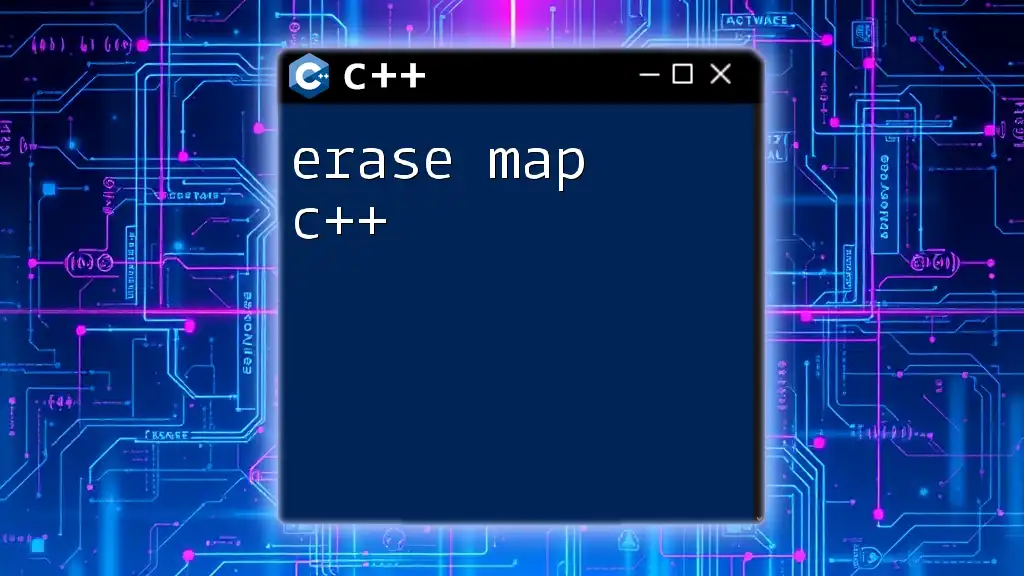
Using the Mapped Memory
Reading from the Mapped Space
Once the file is successfully mapped, reading data is straightforward. Here’s how you can access the data:
char* data = static_cast<char*>(map);
printf("Data: %s\n", data);
This code casts the mapped memory to a `char*`, enabling direct access to the contents. The `printf` function prints the data stored in the mapped memory.
Writing to the Mapped Space
Writing to the memory-mapped area is just as simple. You can assign new data directly to the mapped region like so:
sprintf(data, "Hello, mmap!");
In this case, `sprintf` is used to write a string into the memory space. This operation directly affects the underlying file without needing a separate write syscall.
Synchronizing Changes with msync
To ensure that any changes made to the mapped memory are written back to the file, you should use `msync`. Here’s an example:
if (msync(map, length, MS_SYNC) == -1) {
perror("msync failed");
}
This step is crucial when working with shared memory scenarios as it ensures data consistency and integrity.
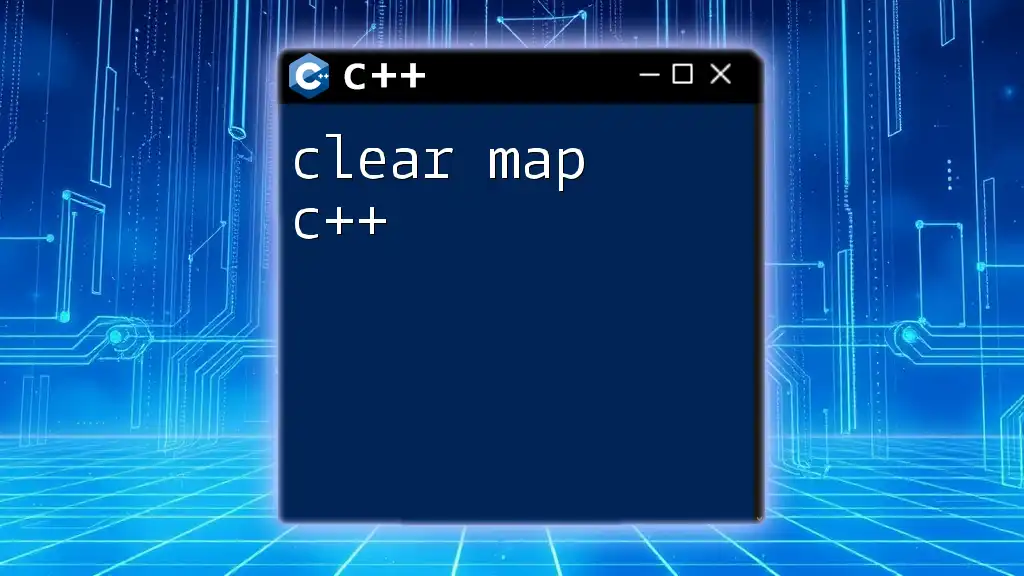
Unmapping Memory
Cleaning Up After Yourself
After finishing operations on the mapped memory, you must unmap it to release resources:
if (munmap(map, length) == -1) {
perror("munmap failed");
}
Calling `munmap` informs the OS that you no longer need the mapped region, preventing memory leaks.
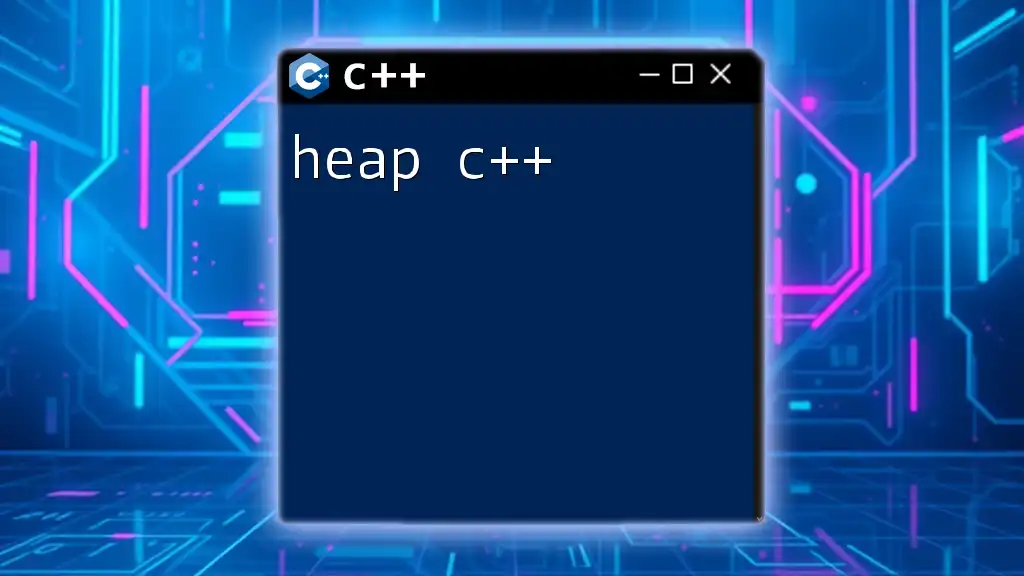
Error Handling in Using mmap
Common Errors and Their Solutions
Using mmap comes with its own set of challenges. Common errors include:
- mmap failures: If `mmap` returns `MAP_FAILED`, you should check the reasons such as invalid file descriptor or insufficient permissions.
- File descriptor errors: Ensure the file was successfully opened before attempting to map it.
- Memory protection mismatches: Ensure the specified protection levels match your application requirements. For instance, trying to write to a read-only mapped area will lead to segmentation faults.
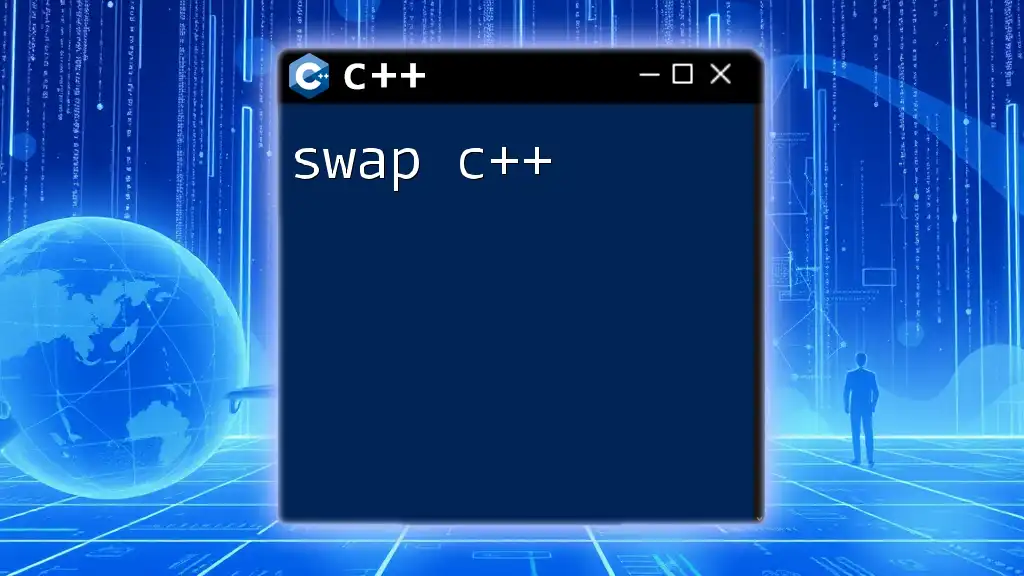
Advanced mmap Techniques
Using mmap for Shared Memory
The `mmap` function is invaluable for inter-process communication (IPC). When using the `MAP_SHARED` flag, multiple processes can access the same memory region, allowing them to communicate seamlessly.
Here’s an example scenario of using mmap for shared memory:
void* sharedMap = mmap(NULL, length, PROT_READ | PROT_WRITE, MAP_SHARED, fd, 0);
Memory-Mapped I/O
In performance-critical applications like databases or large-scale file processing, memory-mapped I/O can be used to enhance speed by minimizing kernel-user space transitions. It allows programs to manipulate hardware devices directly through memory access rather than traditional I/O functions.
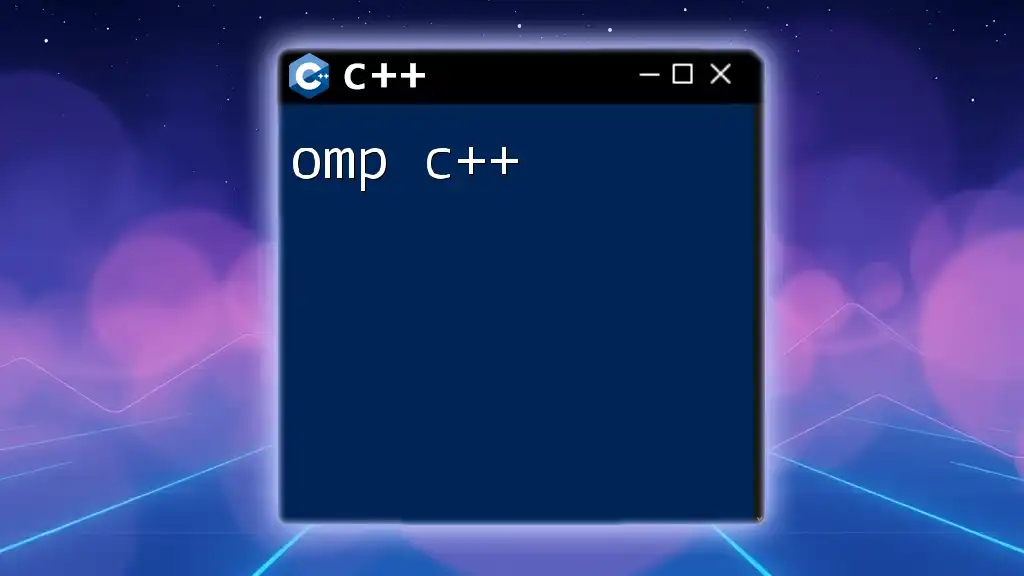
Conclusion
The mmap c++ functionality offers remarkable advantages for applications that require efficient file handling and direct memory access. By utilizing mmap properly, you can achieve substantial performance improvements while simplifying code complexity. Understanding its nuances will empower you to harness its full potential in your C++ projects.
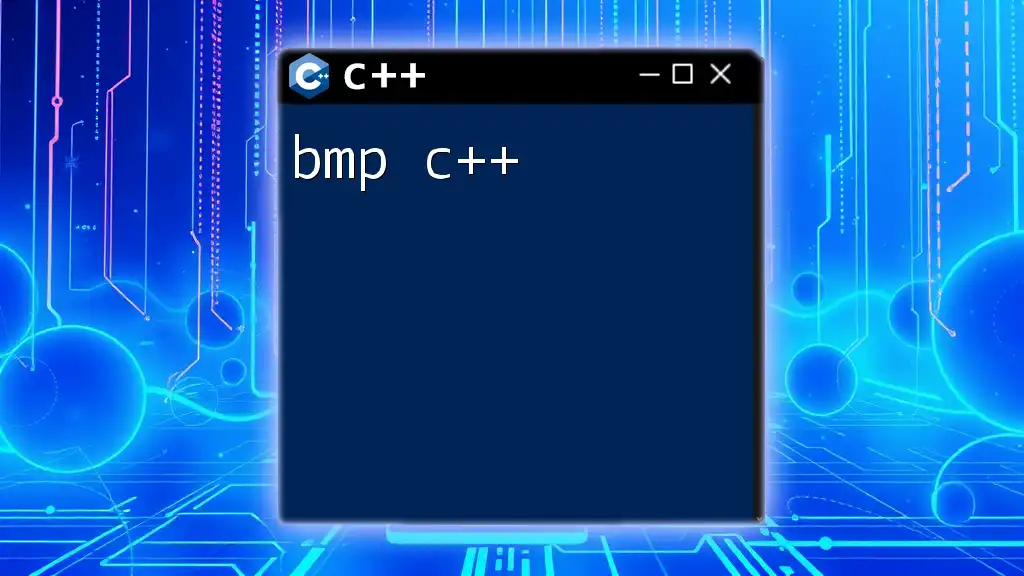
Additional Resources
For further learning, explore the official documentation and resources provided by organizations like The GNU C Library or programming forums that specialize in C++. Additionally, consider libraries that simplify working with mmap for advanced projects.