MFC (Microsoft Foundation Class) is a library that encapsulates the Windows API in C++, enabling the rapid development of GUI applications for Windows.
Here's a simple example of creating a basic MFC application:
#include <afxwin.h>
class CMyApp : public CWinApp {
public:
virtual BOOL InitInstance() {
CWnd *pFrame = new CFrameWnd();
pFrame->Create(NULL, _T("Hello MFC World!"));
pFrame->ShowWindow(SW_SHOW);
m_pMainWnd = pFrame;
return TRUE;
}
};
CMyApp theApp;
What is MFC?
Microsoft Foundation Class Library (MFC) is a powerful framework for building Windows applications using C++. It simplifies the process of developing Windows applications by providing a set of classes that encapsulate Microsoft's Win32 API, making it easier for developers to create rich user interfaces, handle events, and manage application states. MFC has a significant history, having evolved alongside Windows itself, which has made it a stable choice for developing desktop applications.
Key features of MFC include a wide range of user interface components, a robust event-handling mechanism, and a well-defined architecture that helps in maintaining the separation of application logic and UI design.
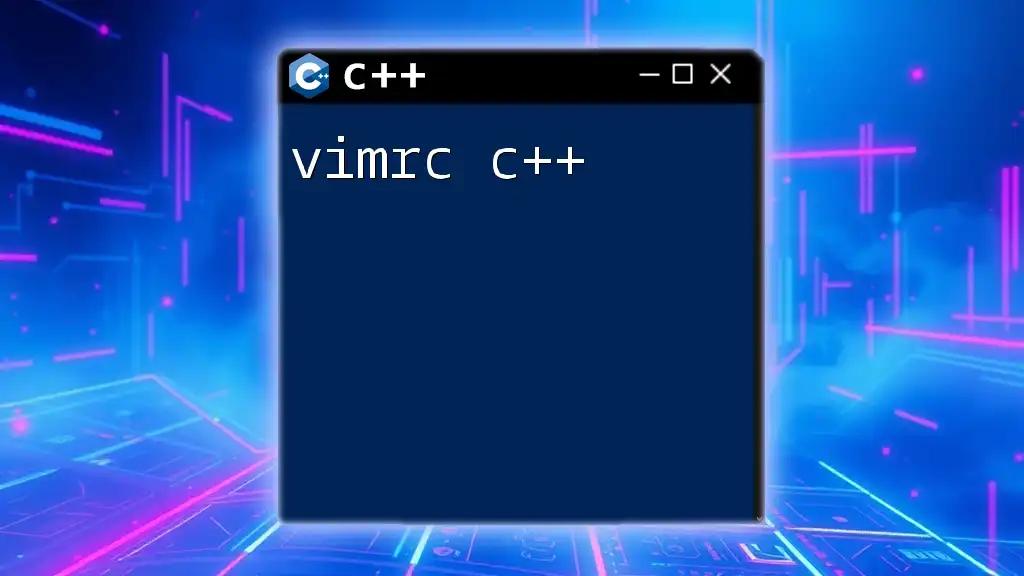
Why Use MFC?
There are several advantages to using mfc c++ for application development:
-
Rich set of classes: MFC provides a comprehensive library of classes to handle everything from basic controls to complex dialogs.
-
Native Windows applications: Applications developed using MFC are true Windows applications that offer better performance when compared to applications built with web-based frameworks.
-
Extensive documentation and community support: Due to its long history, MFC comes with extensive documentation and a large community which can be very helpful for new developers.
When comparing MFC with other frameworks, like Win32 API and .NET, MFC offers a higher level of abstraction, making it easier to develop user interfaces without delving too deeply into the intricacies of the operating system.
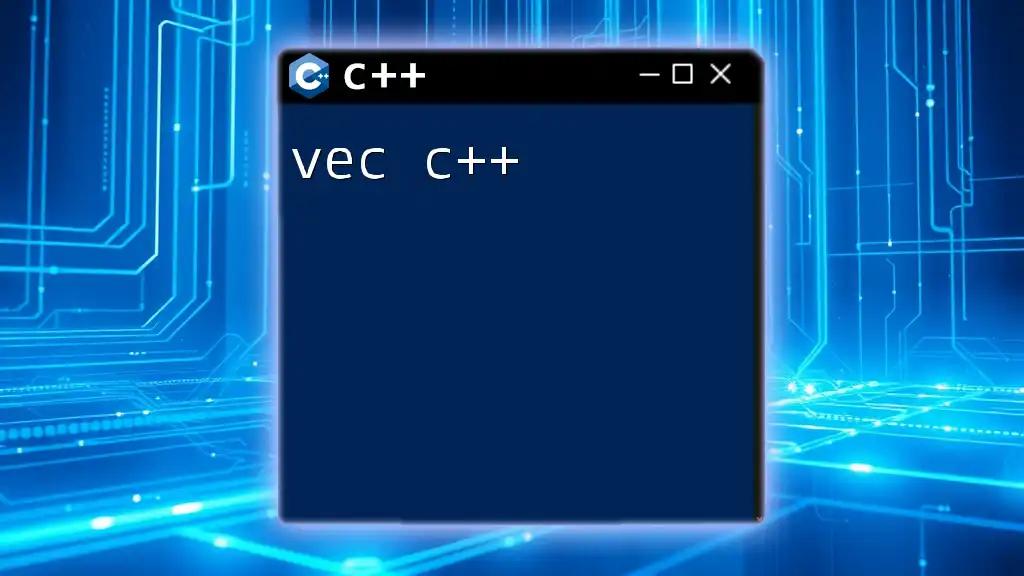
Setting Up the MFC Environment
Prerequisites for MFC Development
Before diving into mfc c++, you will need to ensure that you have the right tools installed:
- Visual Studio: The primary IDE for MFC development which comes equipped with the necessary libraries and tools.
- Windows SDK: Make sure you have the Windows development workload installed in Visual Studio to access the required SDK.
Installing MFC in Visual Studio
To create your first MFC application, follow these steps in Visual Studio:
- Open Visual Studio and select "Create a new project."
- Choose "MFC Application" from the available templates.
- Follow the prompts in the MFC Application Wizard. This will guide you through selecting an application type and configuration settings.
- Once set up, you can click Finish and Visual Studio will generate the basic framework for an MFC application.
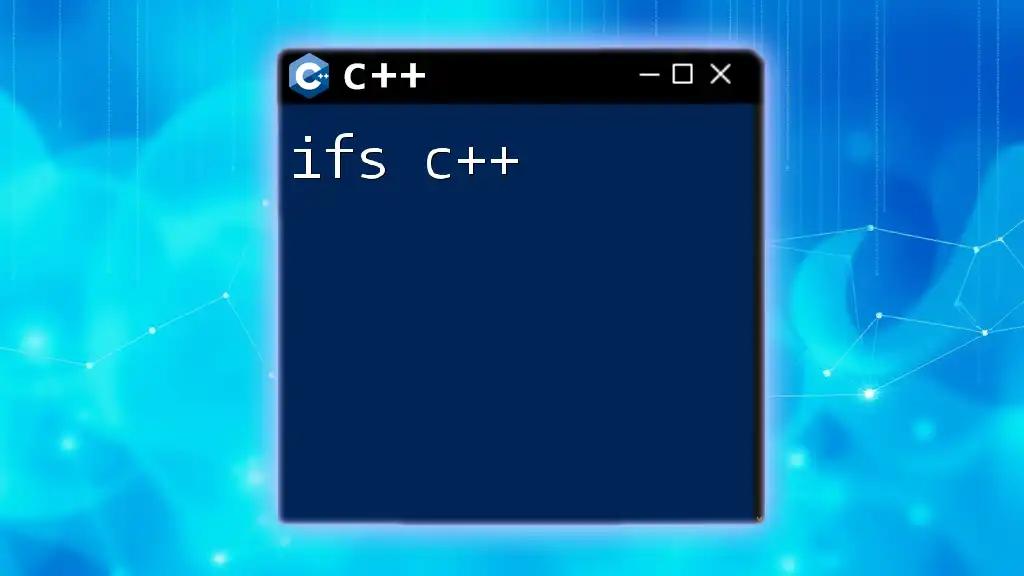
Architectural Overview of MFC
MFC Application Structure
An MFC application has a specific architecture centered around key components that drive its function:
- CWinApp: Represents the application itself, initializes application resources, and manages the application’s execution.
- CWnd: This class is responsible for the creation of windows, allowing you to manage the interface.
- CView and CDocument: These classes implement the Document/View architecture, separating the data (document) from the presentation layer (view).
Message Mapping in MFC
MFC applications operate by responding to messages, which are events that occur in the system (like keystrokes or mouse clicks). MFC uses a mechanism called message mapping. To utilize message handling in your MFC application, you define message maps in your classes.
Here’s a simple example of the message map process:
BEGIN_MESSAGE_MAP(CMyWnd, CWnd)
ON_WM_PAINT()
END_MESSAGE_MAP()
void CMyWnd::OnPaint() {
// Handle drawing here
}
In this example, the application maps the `WM_PAINT` message to the `OnPaint` function, which will define how the window should be drawn.
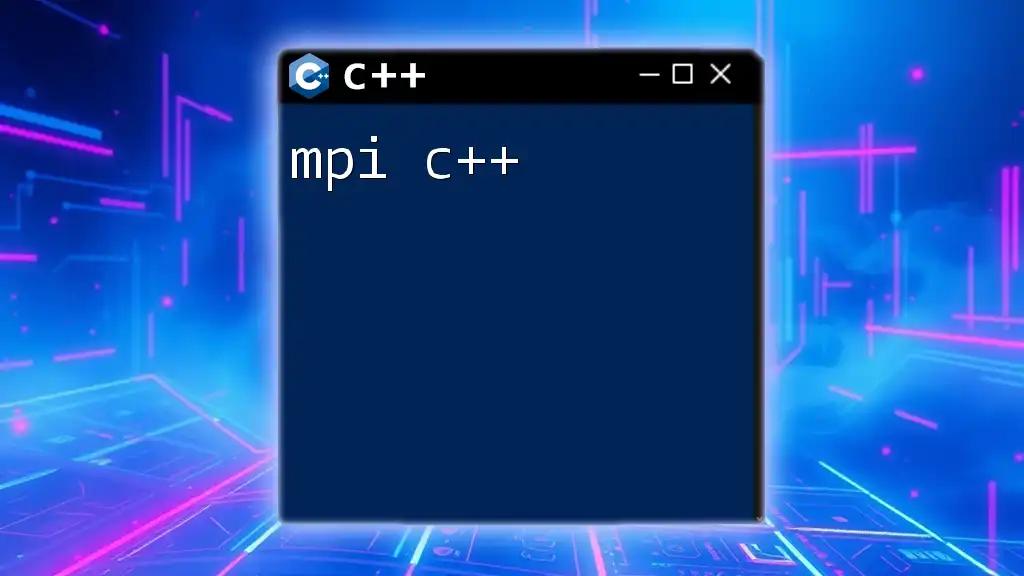
Core MFC Concepts
Basic Controls and Dialogs
Creating dialogs and controls is straightforward in MFC. To create a simple dialog box, you first define a dialog resource in your project, and then in your application, you can call that dialog when needed.
MFC provides a variety of standard controls, such as buttons, list boxes, and edit controls. To create a simple dialog box, you can follow these steps:
- Create a dialog resource using the Visual Studio resource editor.
- Define a class derived from `CDialog` (e.g., `CMyDlg`) to handle its behavior.
Event Handling in MFC
Event handling is a fundamental part of any application, allowing it to respond to user actions. MFC simplifies this through its message mapping capabilities. Here’s an example of handling a button click event:
void CMyDlg::OnBnClickedMyButton() {
// Code to handle button click
AfxMessageBox(_T("Button clicked!"));
}
In this example, when the button is clicked, the application will show a message box with the text "Button clicked!".
Document/View Architecture
The Document/View architecture is a crucial feature of MFC that separates the data model from the presentation, making applications easier to manage and extend. Here’s a simple implementation of a Document/View application.
You define a `CDocument` class to handle data management and a `CView` class to control the presentation. This setup makes it easy to update the UI whenever the underlying data changes.
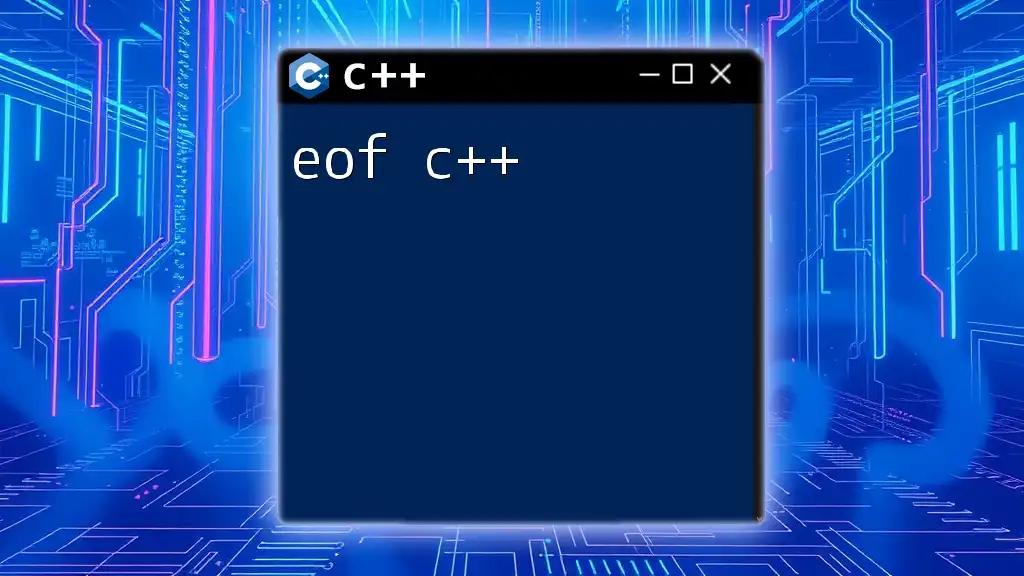
Advanced MFC Topics
Custom Controls and User Interface Design
MFC allows the creation of custom controls tailored to your application’s requirements. Custom controls can significantly enhance user experience when standard controls do not meet your specifications.
Multithreading in MFC
MFC provides built-in support for multithreading, essential for applications that perform long-running tasks while remaining responsive to user input. You can create a thread in MFC as follows:
UINT MyThreadFunction(LPVOID pParam) {
// Thread-related work here
return 0;
}
// Starting the thread
AfxBeginThread(MyThreadFunction);
This capability allows your application to perform background operations without freezing the user interface.
Database Integration with MFC
MFC offers robust support for database operations through ODBC (Open Database Connectivity). You can perform CRUD operations easily, allowing MFC applications to harness the power of relational databases.
An example for a basic database insert might look like:
CDatabase db;
db.OpenEx(_T("DSN=MyDataSource;UID=user;PWD=password;"));
CString strSQL = _T("INSERT INTO MyTable (Column1) VALUES ('Value')");
db.ExecuteSQL(strSQL);
This snippet demonstrates how to connect to a database and execute a straightforward SQL command to insert a record.
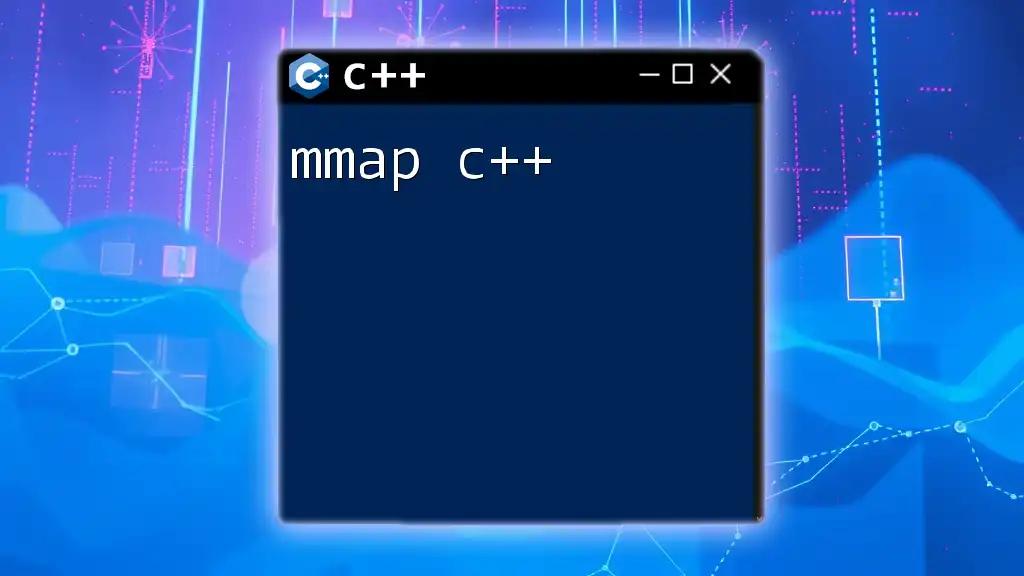
Debugging and Testing MFC Applications
Debugging Techniques in MFC
Debugging is crucial for any software development process. Visual Studio provides an integrated debugger that allows you to set breakpoints, step through code, and inspect variables. Effective use of these tools can significantly enhance your development efficiency.
Testing Strategies
Writing tests for MFC applications may include unit testing specific functionalities. This is essential to maintain code quality and ensure that classes respond as expected under various scenarios.
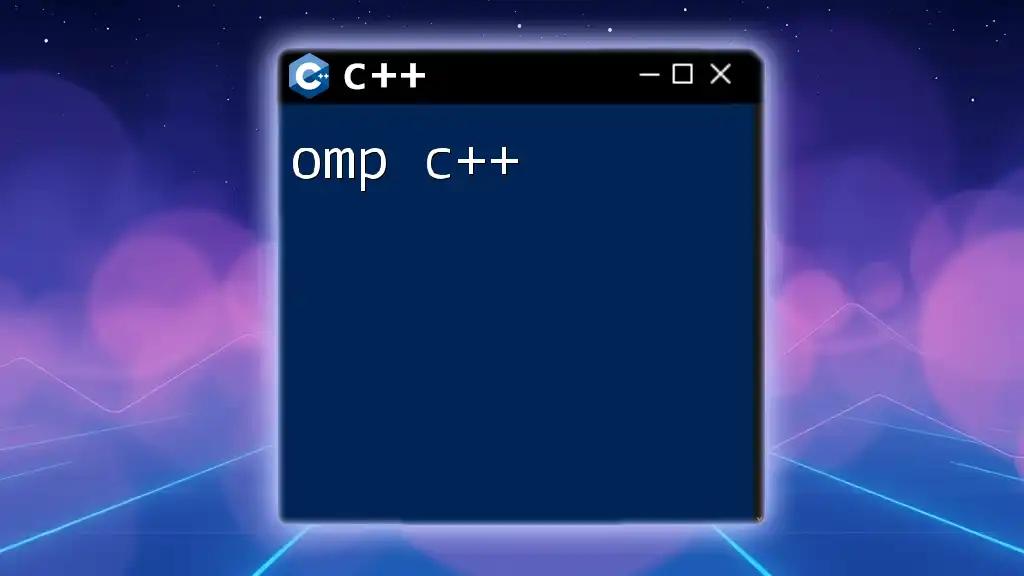
Best Practices for MFC Development
Performance Optimization
To enhance the performance of your MFC application, consider using resources judiciously, minimizing unnecessary redrawing of windows, and releasing resources when no longer needed. Profiling tools can help identify bottlenecks in your application.
Code Maintenance and Organization
A well-organized project structure with clear naming conventions and consistent coding style will facilitate easier maintenance and updates. Documentation, comments, and clear class responsibilities are crucial for sustainability in long-term projects.
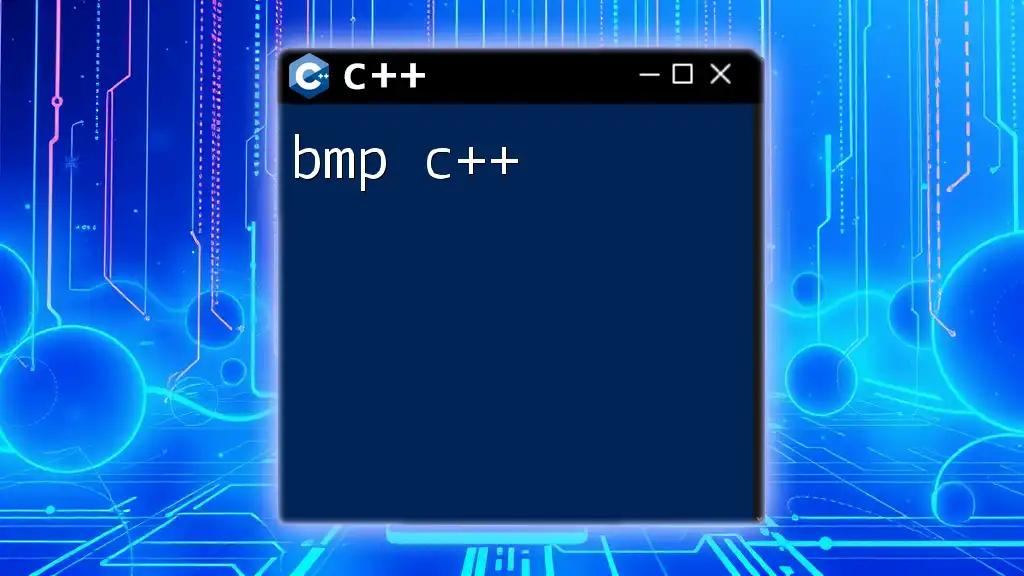
Conclusion
In summary, mfc c++ offers a powerful, flexible framework for developing Windows applications. By leveraging its rich set of features, you can create responsive, robust applications while maintaining a clear separation between data and presentation. The resources available and community support ensure that developers at all skill levels can begin their journey into MFC development with confidence.
Additional Resources
To further your understanding of MFC, consider exploring recommended books, online tutorials, and getting involved in community forums where you can seek help and share experiences with other developers.