UML (Unified Modeling Language) is a standardized modeling language that helps in visualizing, constructing, and documenting the artifacts of a software system, including the use of C++ commands.
Here's a simple example of UML representation of a C++ class:
class Animal {
public:
void makeSound();
private:
int age;
};
In this UML example, `Animal` is the class with a public method `makeSound()` and a private attribute `age`.
What is UML?
Unified Modeling Language (UML) is a standardized modeling language that is widely used in software development to document, visualize, and specify the design of software systems. It enables developers and stakeholders to communicate ideas, requirements, and designs effectively through various types of diagrams.
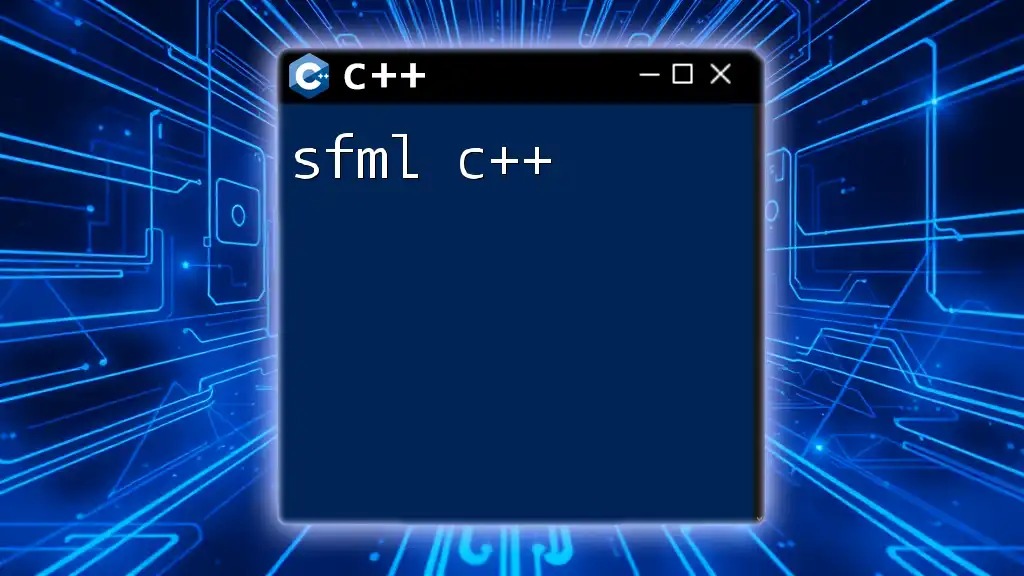
What is C++?
C++ is a powerful programming language that combines both high-level and low-level language features. It is known for its efficiency and performance, making it a preferred choice in various application domains such as systems programming, game development, and high-performance applications. UML provides a valuable framework for designing and communicating C++ software architectures and systems.
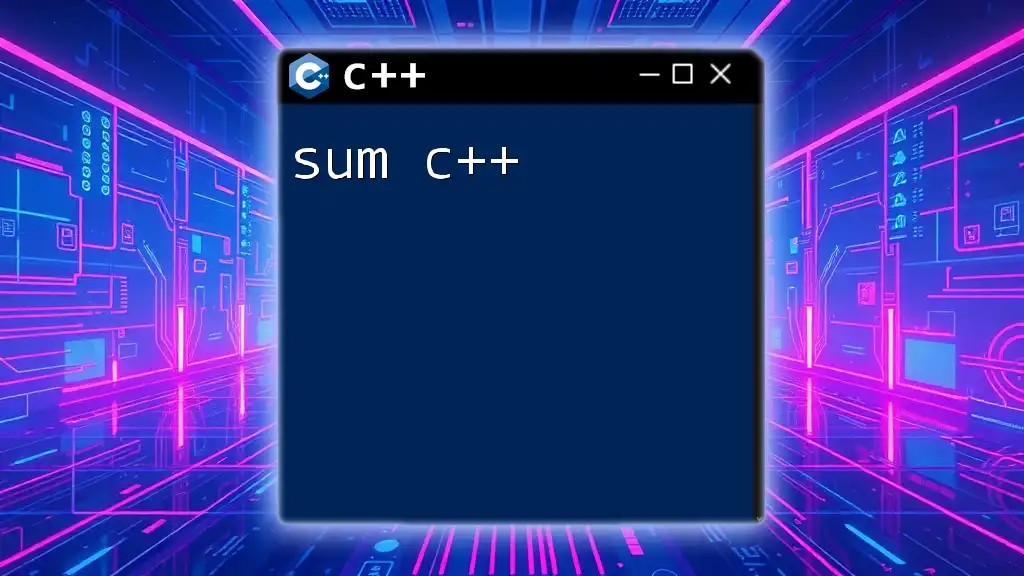
Understanding UML Diagrams
UML encompasses various diagrams, which are classified into two main categories: structural diagrams and behavioral diagrams. Each category serves a specific purpose and provides insights into different aspects of a system.
Types of UML Diagrams
Structural Diagrams
Structural diagrams represent the static aspects of the system, showcasing the different components, their attributes, and relationships.
- Class Diagram
A class diagram is a fundamental structural diagram that illustrates the classes, their attributes, methods, and relationships among them. In C++, classes are the blueprint for creating objects, and a clear understanding of class diagrams helps in the structured design of systems.
For example, consider a simple vehicle class in C++:
class Vehicle {
protected:
int wheels;
public:
void setWheels(int w) { wheels = w; }
};
This class demonstrates the encapsulation of the wheel attribute. The UML class diagram would represent the class with its respective attributes and methods, detailing the structure that C++ will implement.
- Component Diagram
Component diagrams illustrate the organization and dependencies among a set of components. They delve into the components' interactions but do not worry about the finer details of their implementation.
- Deployment Diagram
Deployment diagrams show the physical deployment of artifacts on nodes. They highlight the environment within which the software will operate, showing the distribution of components across hardware nodes.
Behavioral Diagrams
Behavioral diagrams depict the dynamic aspects of the system, focusing on how components interact and behave over time.
- Use Case Diagram
A use case diagram captures the functional requirements of a system, showcasing the interactions between actors (users or other systems) and use cases (functionalities). This provides a high-level view of what the system should do.
For example, a use case diagram might involve a user interacting with a vehicle system to set wheels, reflecting the functionality that will be implemented in C++.
- Sequence Diagram
Sequence diagrams display how objects interact with each other in a time sequence, allowing insight into the flow of messages between objects.
Consider the following scenario where a vehicle's wheel is set:
void executeProcess() {
Vehicle v;
v.setWheels(4);
// additional calls
}
In a sequence diagram representing this process, you would illustrate the method calls and the order they occur, providing clarity on how interactions unfold over time.
- Activity Diagram
Activity diagrams are another critical tool in UML that serve to visualize the flow of control or data among activities. They represent the sequence of operations and potential decision paths and are incredibly helpful for understanding complex workflows within C++ applications.
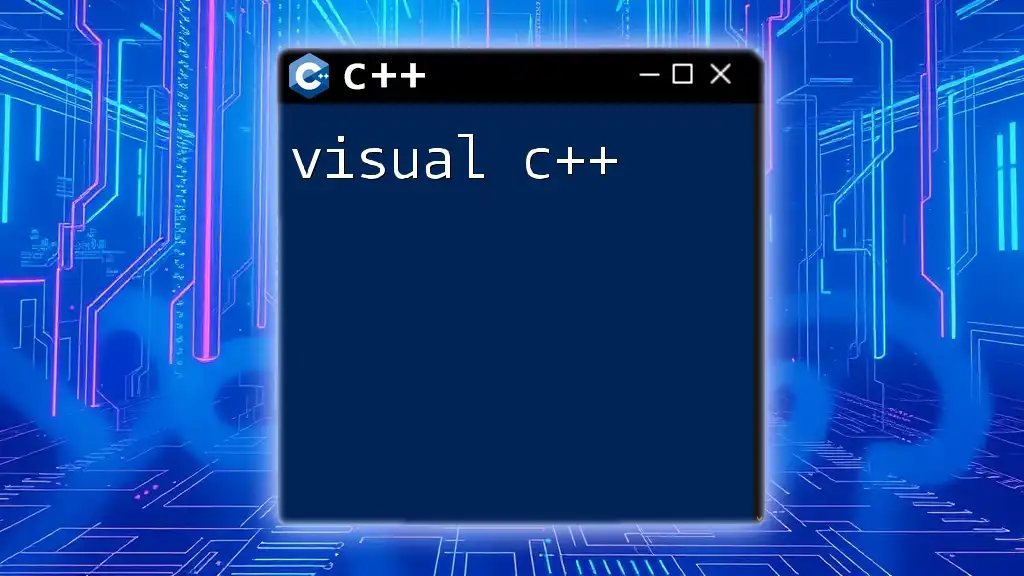
How UML Enhances C++ Development
Using UML in C++ projects offers multiple advantages, significantly improving both the design and implementation phases of software development.
- Clarity in Design and Planning Stages
By modeling systems using UML, developers can visualize complex architectures clearly before diving into code. This preemptive step can reveal potential design flaws early on, saving time and resources later.
- Improved Communication Amongst Team Members
UML diagrams serve as a common language for developers, designers, and stakeholders. By utilizing these diagrams, teams can bridge gaps in understanding, ensuring everyone is aligned on project objectives.
- Better Alignment Between Design and Implementation
UML acts as a blueprint for programmers, making it easier to translate designs into C++ code accurately. This direct correlation helps maintain consistency and integrity between high-level design and actual execution.
Case Studies
Reflecting on real-life projects, several C++ applications across various domains have successfully benefitted from UML integration. For instance, a logistics software application used UML to model its class structures and interactions, which significantly increased the team's efficiency and effectiveness in developing features on time.
In another case, a gaming application utilized UML to create a detailed use case and sequence diagrams, allowing developers to understand complex interactions between game characters and the environment better.
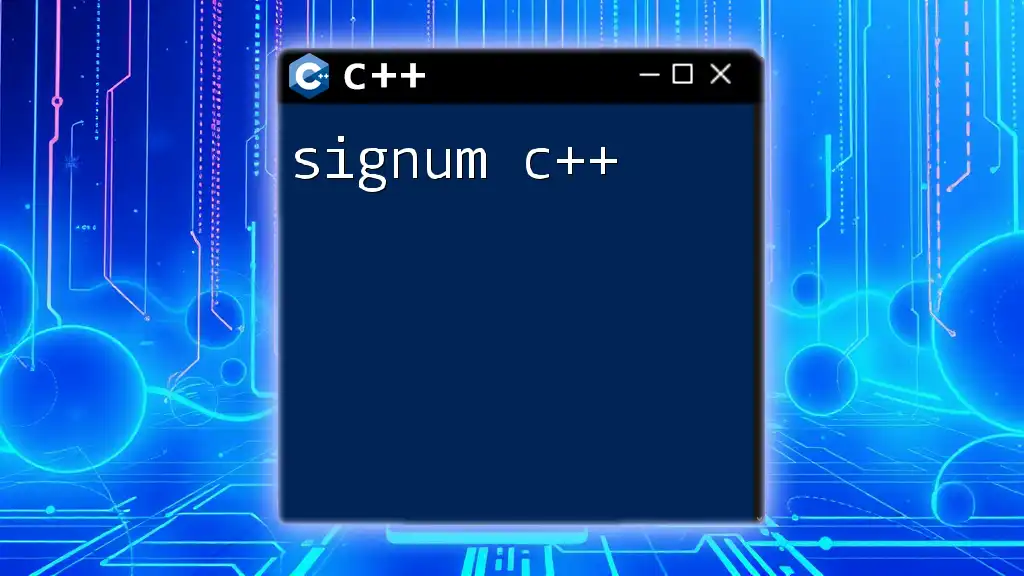
Best Practices for Integrating UML with C++
To maximize the benefits of UML in C++ development, consider the following best practices:
Choosing the Right UML Diagram for Your Project
Determining which UML diagrams to use depends on your project size and complexity. Smaller projects may require just a class diagram, while larger systems might necessitate a combination of use case, sequence, and activity diagrams to convey the full picture.
Tools for UML and C++ Integration
There are various tools available that support UML modeling alongside C++ code generation. Some of the notable ones include:
- Visual Paradigm: This tool offers comprehensive UML capabilities while allowing integration with C++ code.
- StarUML: Supports various UML diagrams and provides an intuitive user interface for modeling.
- Microsoft Visio: While not solely a UML tool, it provides functionalities to create UML diagrams effectively.
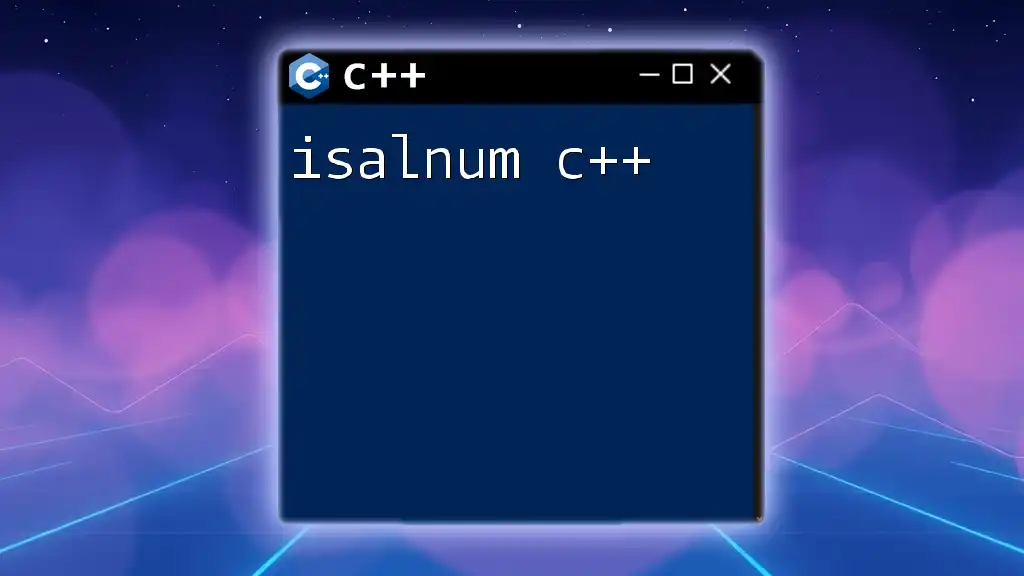
Creating UML Diagrams for C++ Classes
Step-by-Step Guide
Creating UML diagrams for C++ classes can be simplified into a few steps:
- Identify the Classes: Outline all classes that will be part of your system.
- Define Attributes and Methods: Determine the key attributes and the methods for each class, reflecting their responsibilities.
- Establish Relationships: Identify how classes relate to each other, whether through inheritance, associations, or dependencies.
For instance, let’s take a look at a simple class for a `Car`, which inherits from the `Vehicle` class:
class Car : public Vehicle {
private:
string model;
public:
Car(string mod) { model = mod; }
};
In the corresponding UML class diagram, you would depict the `Car` class linked to the `Vehicle` class, showing inheritance, including the `model` attribute, and any specific methods, rendering the blueprint clearer for C++ implementation.
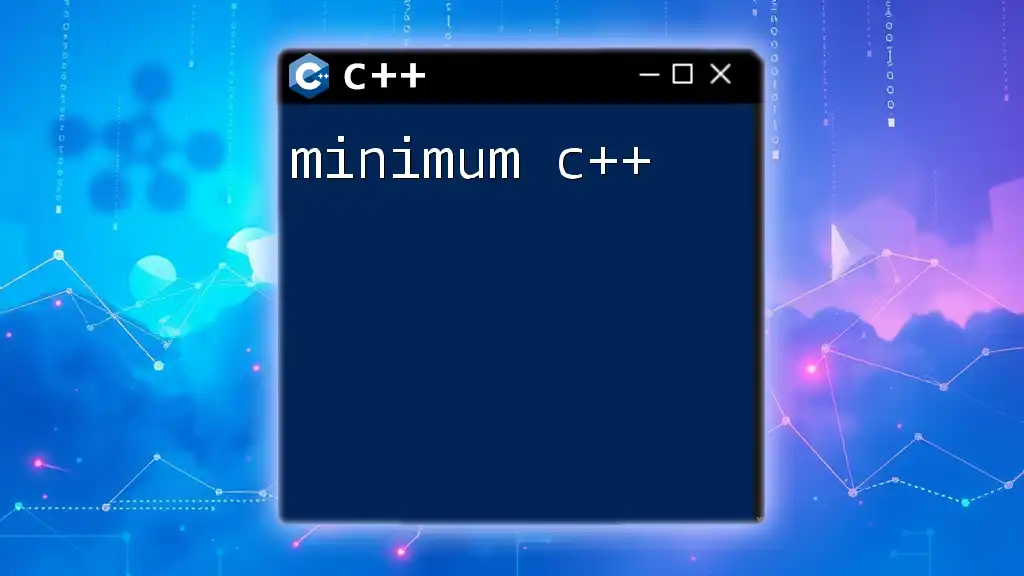
Conclusion
Integrating UML with C++ plays a crucial role in enhancing the clarity, communication, and alignment within software development processes. By using different types of UML diagrams, developers can create comprehensive and understandable designs that guide implementation. Embracing UML as a part of your C++ project will lead to increased efficiency and improved software quality, encouraging developers to leverage this powerful modeling language in their coding practices.
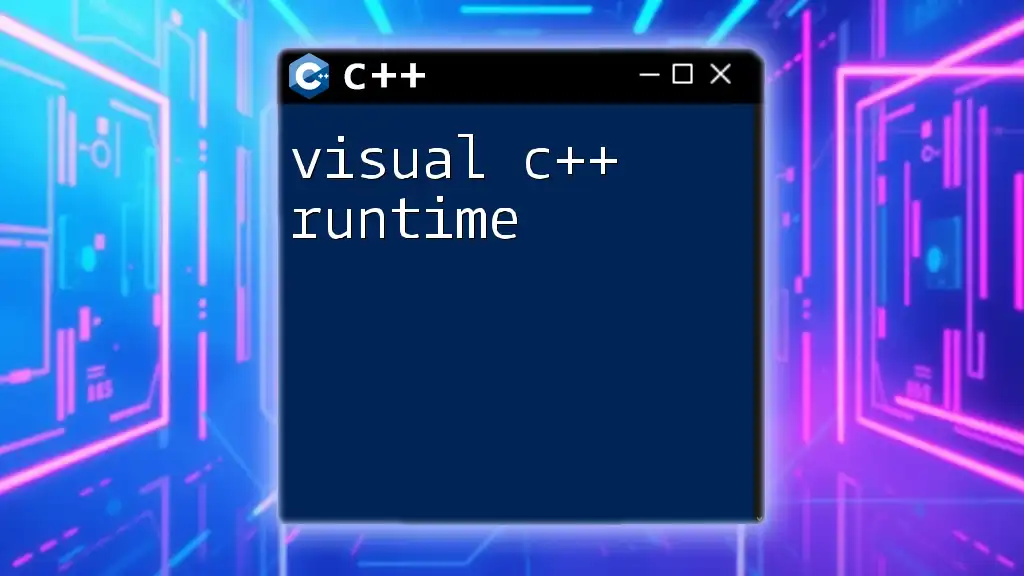
References
For further reading on UML and C++, consider examining resources that delve deeper into both subjects. Recommended books and courses can provide additional insights, and staying updated on UML best practices is vital for effective software engineering.
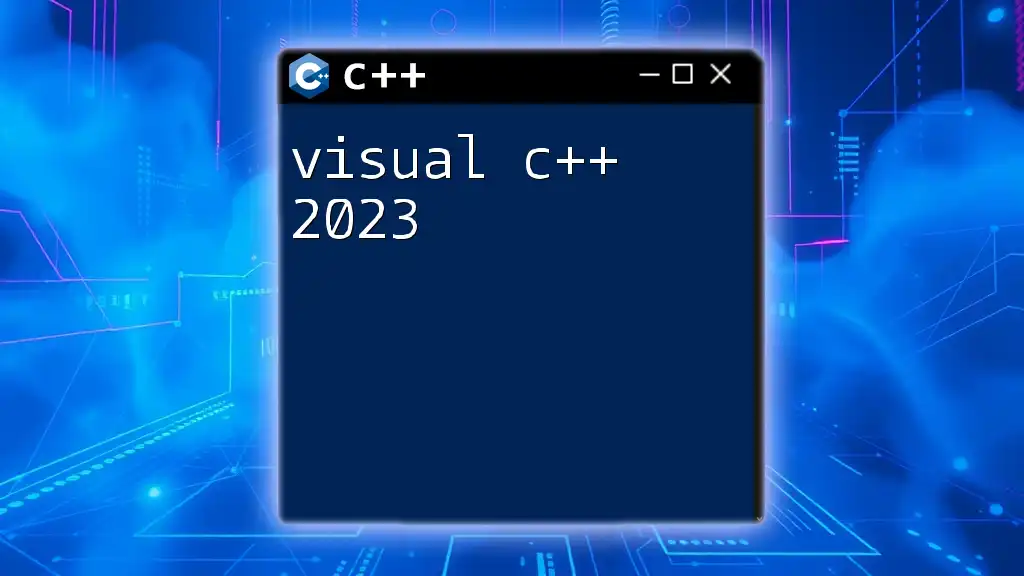
About the Author
With a strong foundation in both UML and C++, the author is committed to sharing knowledge and strategies to help others master these essential tools to enhance their software development journey. Follow for more updates and educational content!