"Minimum C++ refers to the essential components needed to create a basic C++ program, typically including a main function and a simple output statement."
Here’s a minimal example in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Minimum C++?
Minimum C++ is the practice of writing concise and efficient code that captures the essential functionality without unnecessary complexity. Unlike verbose coding practices that may lead to bloated and hard-to-read code, minimum C++ focuses on clarity and brevity while maintaining performance. This coding philosophy promotes simplicity and effectiveness, enabling developers to produce more maintainable code.
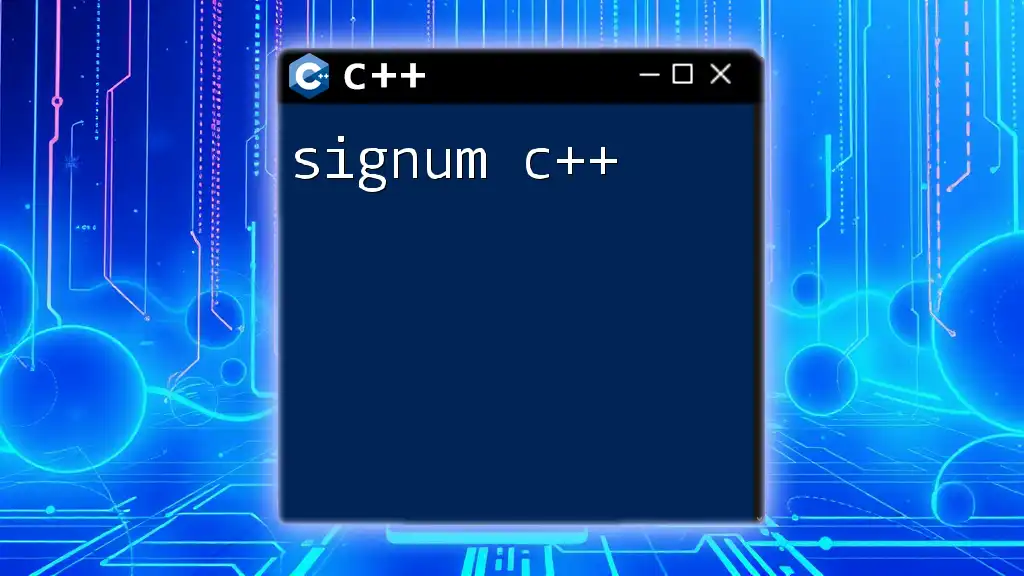
Benefits of Using Minimum C++
When applied correctly, minimum C++ brings several advantages:
-
Performance: By reducing the amount of code to be executed, you can improve runtime performance. Less code often translates to fewer resources consumed, leading to faster execution times.
-
Readability: Writing in a minimal style ensures that your code is easier to read and understand. When fewer lines are cluttered with unnecessary syntax, it becomes more approachable for you and others who may work with it later.
-
Maintainability: Code that is clear and concise is easier to maintain. It is simpler to identify and fix bugs, add new features, or modify existing functionality when the codebase isn't overloaded with superfluous elements.
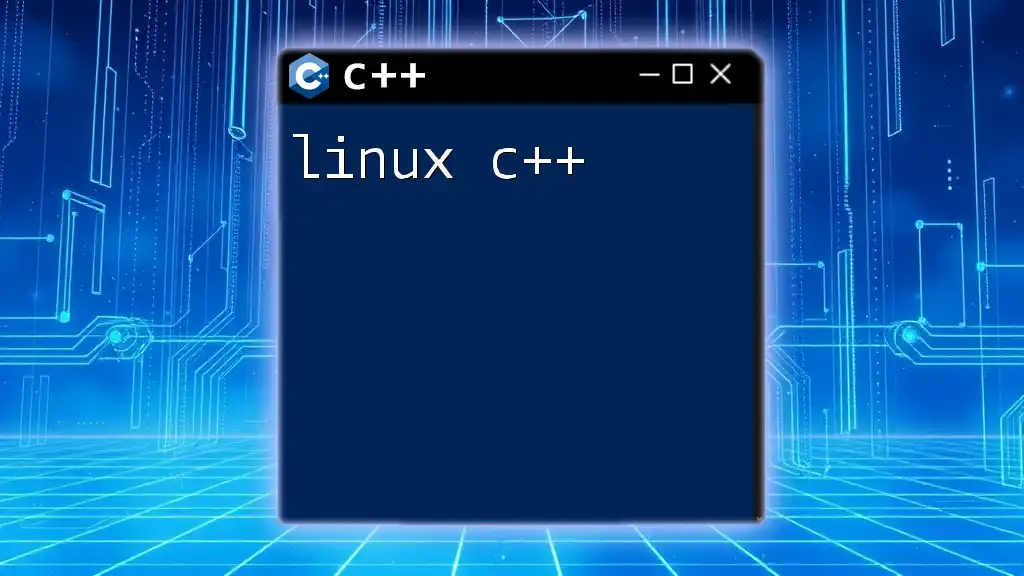
Core Principles of Minimum C++
Eliminate Redundancy
Redundancy is a common pitfall in coding. Minimizing redundancy not only cleans up your code but also enhances its efficiency. By refactoring duplicated code into reusable functions, you can simplify your approach considerably.
Example: Refactoring duplicated code into functions.
// Before (redundant code)
int add(int a, int b) {
return a + b;
}
int sum = add(5, 10);
int total = add(5, 20);
// After (reduced redundancy)
int add(int a, int b); // Function declaration
int sum = add(5, 10);
int total = add(5, 20);
In the example above, the function `add` is defined once and can be reused, minimizing redundancy in code.
Use Standard Library Effectively
Importance of STL (Standard Template Library)
The Standard Template Library (STL) is a powerful feature of C++ that provides a range of ready-to-use classes and functions. By effectively leveraging STL, developers can accomplish tasks with minimal effort.
Common STL Containers
Key containers, such as vectors, lists, and maps, allow you to handle data structures efficiently.
Example: Employing a vector for dynamic array needs.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " "; // Output: 1 2 3 4 5
}
return 0;
}
Using a vector provides automatic memory management and resizes the container as needed, promoting minimal code.
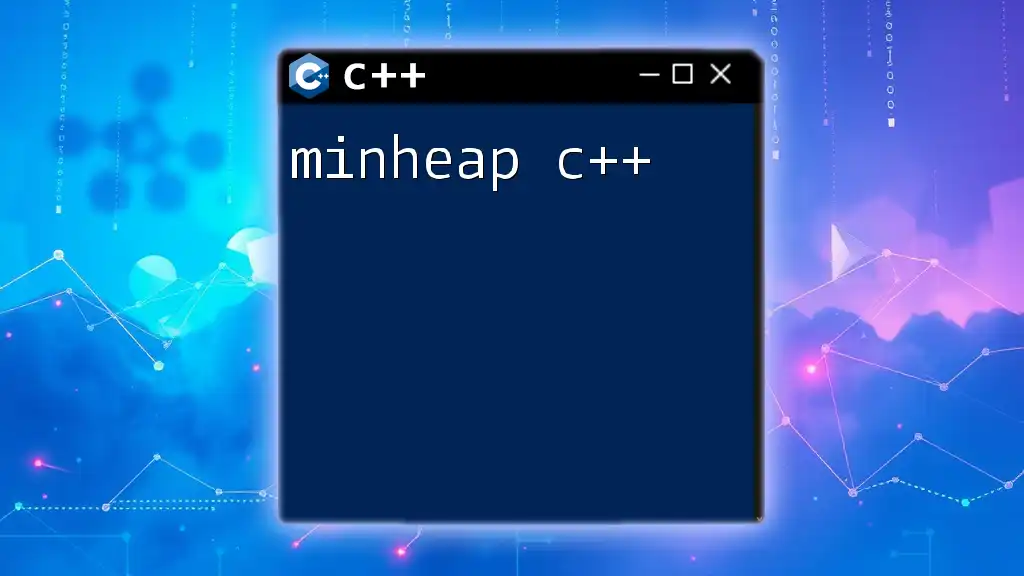
Minimal Syntax and Constructs
Leveraging Type Inference
C++11 introduced the `auto` keyword, allowing you to specify types automatically. This not only shortens your code but enhances readability.
Example: Simplifying declarations with `auto`.
auto x = 10; // Automatically deduced as int
auto y = 2.5; // Automatically deduced as double
By using `auto`, you eliminate redundancy in type declarations, making your code cleaner.
Smart Pointers Over Raw Pointers
Memory management in C++ can be challenging, especially with raw pointers. Switching to smart pointers, such as `std::unique_ptr` and `std::shared_ptr`, not only simplifies management but also improves resource safety.
Example: Using `std::unique_ptr` for automatic resource management.
#include <iostream>
#include <memory>
int main() {
std::unique_ptr<int> ptr(new int(5));
std::cout << *ptr << std::endl; // Output: 5
return 0;
}
By adopting smart pointers, you significantly reduce the likelihood of memory leaks and enhance the overall integrity of your code.
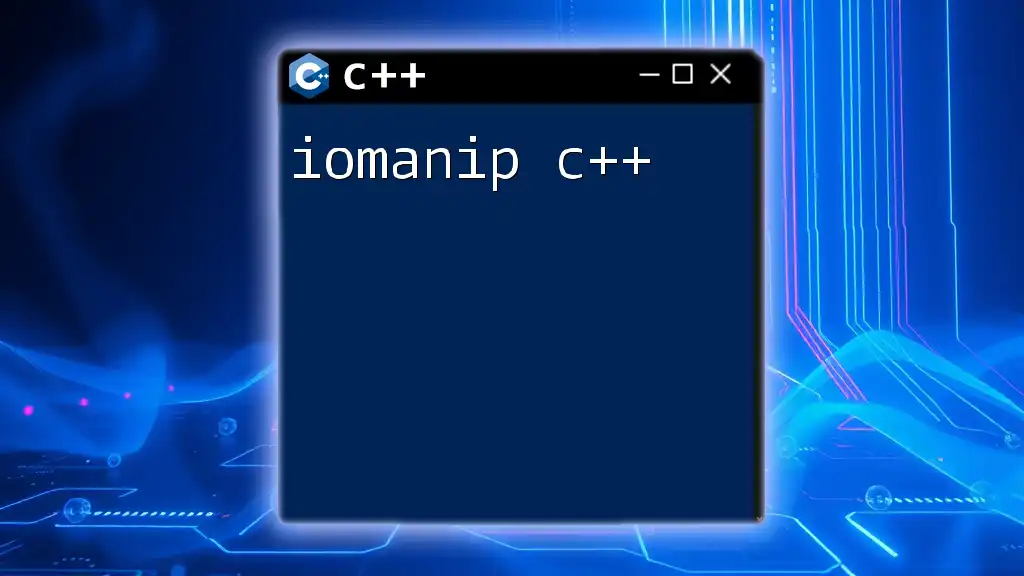
Writing Minimum C++ Functions
Function Signature Simplification
The signature of a function can be made more concise through the use of default arguments. This approach effectively declutters your code.
Example: Using default arguments for simpler signatures.
int multiply(int a, int b = 1); // b is optional
This allows for versatility without cluttering your function definitions.
Overloading Functions
Function overloading enables you to create multiple versions of a function with different parameter types, reducing the need for additional functions and enhancing code clarity.
Example: Demonstrating basic overloading.
int add(int a, int b) { return a + b; }
double add(double a, double b) { return a + b; }
Overloading keeps related functionality together while avoiding redundancy, which is a core tenet of minimum C++.
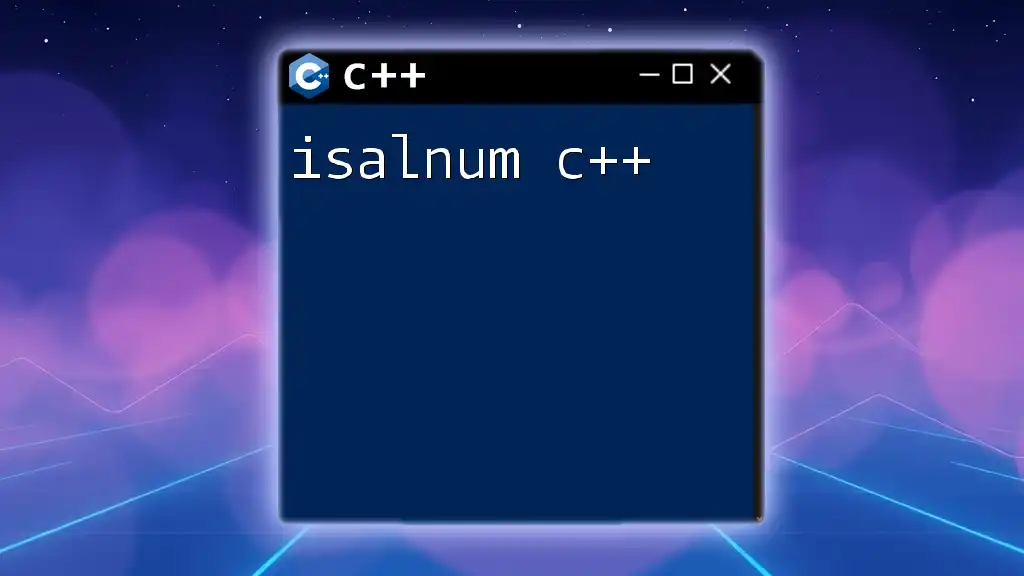
Practice Examples
Example Application: Simple Calculator
Building a simple command-line calculator is an excellent way to implement principles of minimum C++. By focusing on core functionality, you can create an efficient and readable piece of software that highlights your understanding of minimalism in C++.
Example Application: To-Do List
Creating a basic to-do list application using STL is another practice opportunity. For instance, you can use vectors to manage tasks dynamically while minimizing unnecessary complexity. This example serves to underscore the principles of maintaining clarity and efficiency in your code.
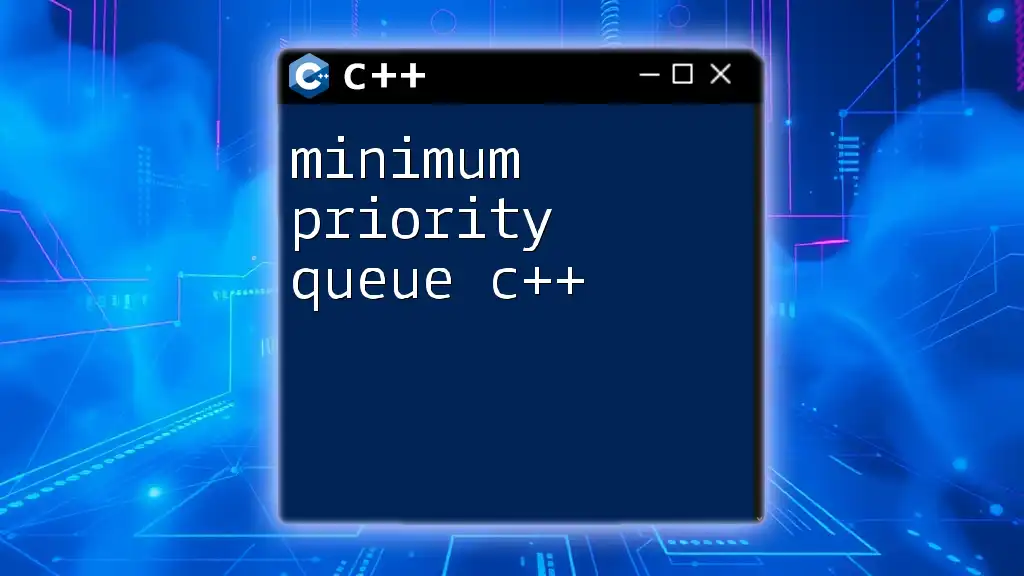
Conclusion
Minimum C++ exemplifies the strength in simplicity and clarity. By embracing this philosophy, you can significantly enhance the performance, readability, and maintainability of your code. Implementing these principles requires practice and a continual commitment to striving for efficiency, but the rewards are substantial. The more you apply minimum C++, the more intuitive and beneficial it will become in your programming journey.
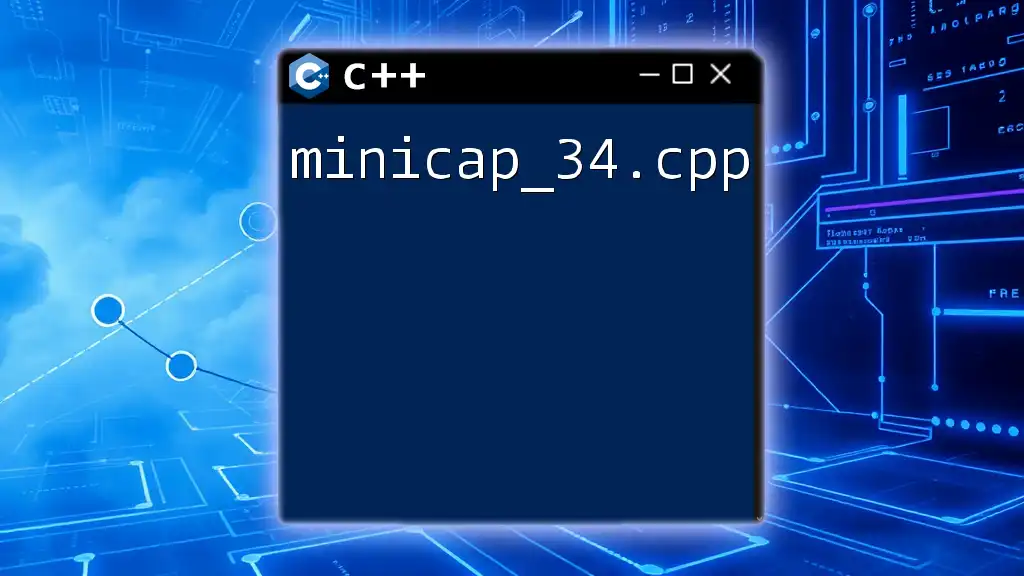
Additional Resources
For those eager to delve deeper into minimum C++, consider exploring recommended C++ books and online resources focused on this philosophy. Tutorial websites, coding communities, and video lessons can also provide valuable insights as you refine your skills.
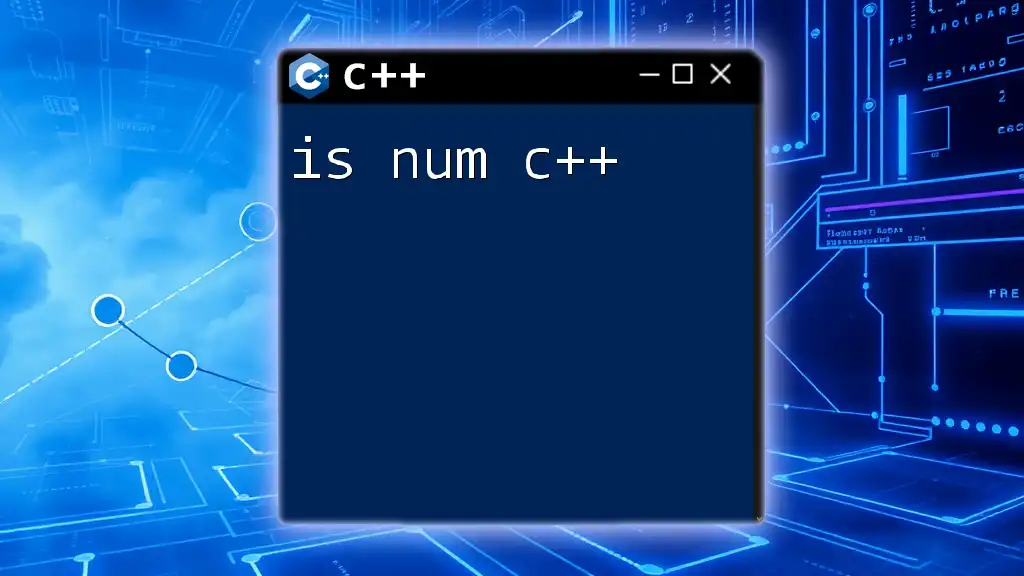
Call to Action
If you found this article helpful, consider subscribing for more content, tutorials, and insider tips on mastering C++. Engage with a community of programmers who are also keen on honing their C++ skills!