The `minicap_34.cpp` file is used in Android development to capture and stream screen content on devices running Android 4.4 (KitKat) or higher.
Here's a simple example snippet demonstrating how to include the necessary headers and set up a basic minicap functionality:
#include <iostream>
#include <cstdio>
#include <cstdlib>
int main() {
system("adb shell screenrecord /sdcard/video.mp4");
std::cout << "Screen recording started..." << std::endl;
return 0;
}
What is minicap?
`minicap` is a utility that captures the screen of an Android device. It is essential for developers needing to record or display live screen activity. Its origins lie in the need for efficient and lightweight screen capturing methods that run directly on Android without the overhead of a complete video recording solution. Developers often use it in testing scenarios, debugging, and even in creating tutorials by capturing the device's UI.
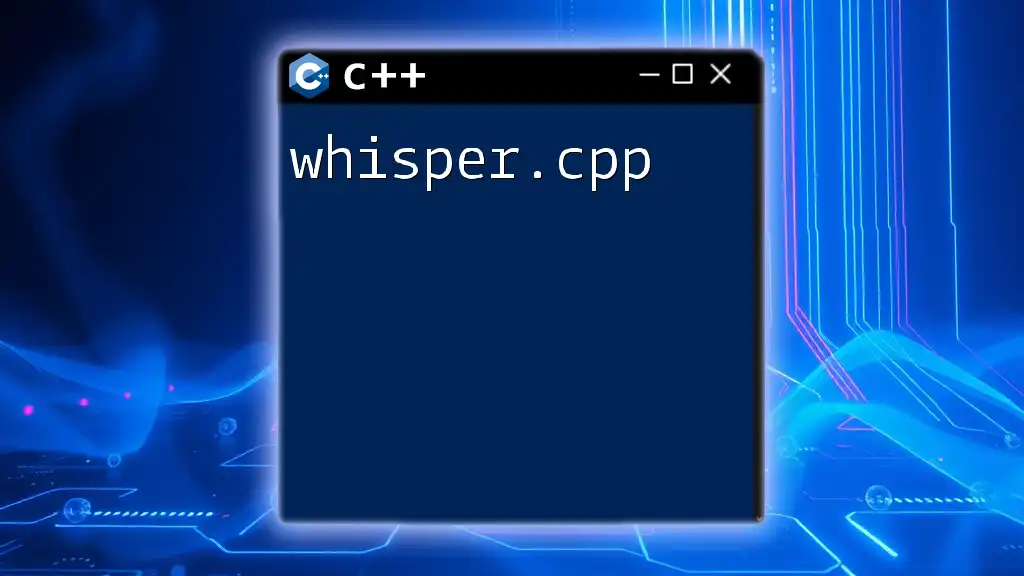
Understanding minicap_34.cpp
The `minicap_34.cpp` file is a crucial part of the `minicap` utility. It serves as the backbone for capturing and processing screens in an Android environment. This file is structured in a way that allows seamless interaction between the Android framework and C++ code, providing developers with the flexibility needed for performance optimization and easy modification.
Key components of minicap_34.cpp
Header Files
`minicap_34.cpp` includes various header files that are essential for its functionality. Understanding these files helps in navigating the code better. For instance, the inclusion of `<jni.h>` is vital as it allows the communication between Java and native C++ code, enabling developers to invoke C++ functions from Java directly. Similarly, `<android/log.h>` supports logging functionality, allowing developers to write logs, which is crucial for debugging and monitoring the application's behavior.
Namespaces
Namespaces in C++ help to organize code and avoid naming conflicts. In `minicap_34.cpp`, the use of `namespace` helps encapsulate the functions and classes, keeping the global scope clean. This organizational aspect becomes crucial when merging several libraries or frameworks in larger projects.
Function Declarations
Function declarations indicate what functions are defined in the file. They provide insight into the functionalities offered by `minicap_34.cpp`. Understanding these declarations helps in creating more effective calls within your application.
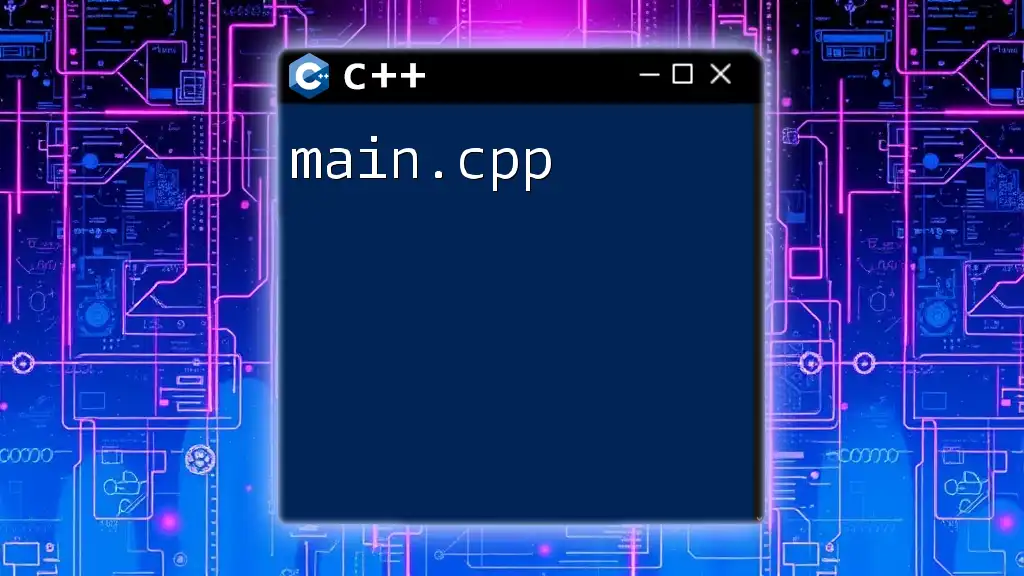
Key Functions in minicap_34.cpp
Main Capturing Function
The main function responsible for capturing the screen is at the heart of the `minicap` utility. Its purpose is to grab the current display buffer, typically involving direct access to framebuffer data.
Function Purpose: This function is crucial as it initiates the capturing process of the screen.
Here’s an example code snippet:
void capture() {
// logic to capture screen
}
In this function, various setups happen, like initializing services and finding the framebuffer that holds the display content. Understanding each step enhances comprehension of how the screen capture process works.
Buffer Handling
Buffer management is critical in `minicap_34.cpp`, primarily for storing the captured image data temporarily before processing it further. Buffers hold pixel data in a format compatible with the intended output format, such as PNG or JPEG.
Purpose of Buffering: Buffers help in managing memory efficiently by temporarily holding the image data, allowing the program to process this data without overloading memory resources.
For instance, initializing a buffer might look like this:
char* buffer = new char[buffer_size];
Properly managing memory by ensuring that buffers are deleted after usage prevents memory leaks and enhances application reliability.
Data Processing
Data processing in `minicap_34.cpp` involves converting the raw framebuffer data into a usable image format. This step is vital as it makes the captured data user-friendly.
How Data is Processed: The pipeline typically includes reading the buffer, processing pixel data, and encoding it in a smartphone-compatible format.
An example of image conversion looks like:
convertToPNG(buffer, width, height);
In this function, the pixel data held within the buffer is manipulated and encoded into a PNG file format. This transformation ensures the captured content is displayed correctly across various applications.
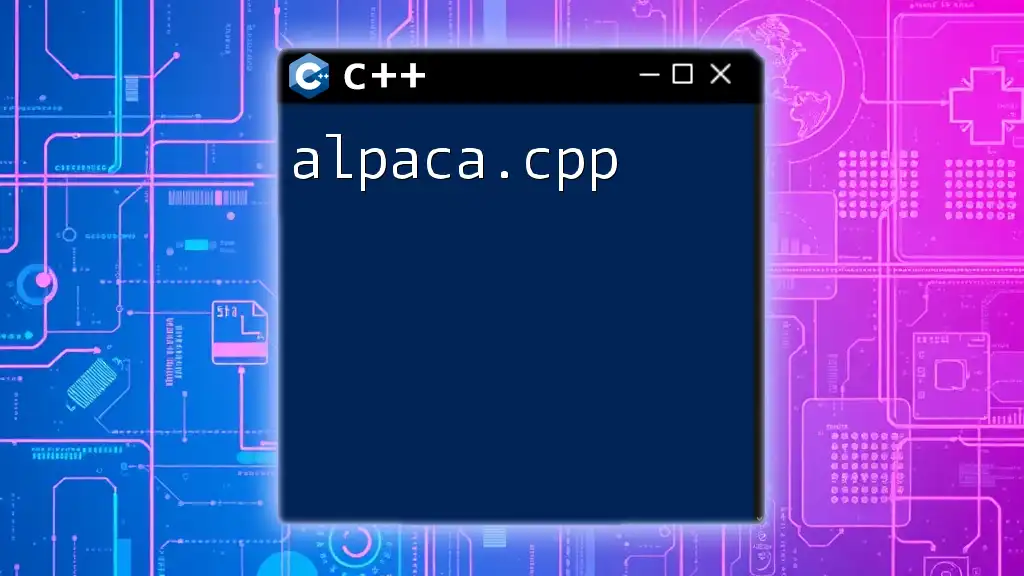
Building minicap_34.cpp
Prerequisites
To successfully compile `minicap_34.cpp`, you need a set of tools and libraries:
- CMake: This is essential for managing the build process.
- Android NDK: It provides the necessary tools and libraries to compile native code for Android.
Ensure that you have these installed and properly configured in your development environment.
Compilation Steps
To compile `minicap_34.cpp`, you can follow these steps:
- Navigate to the directory containing the `minicap` source files.
- Execute the following commands in your terminal:
cmake ..
make
These commands will configure the project and compile the native code, resulting in an executable capable of capturing Android screen data.
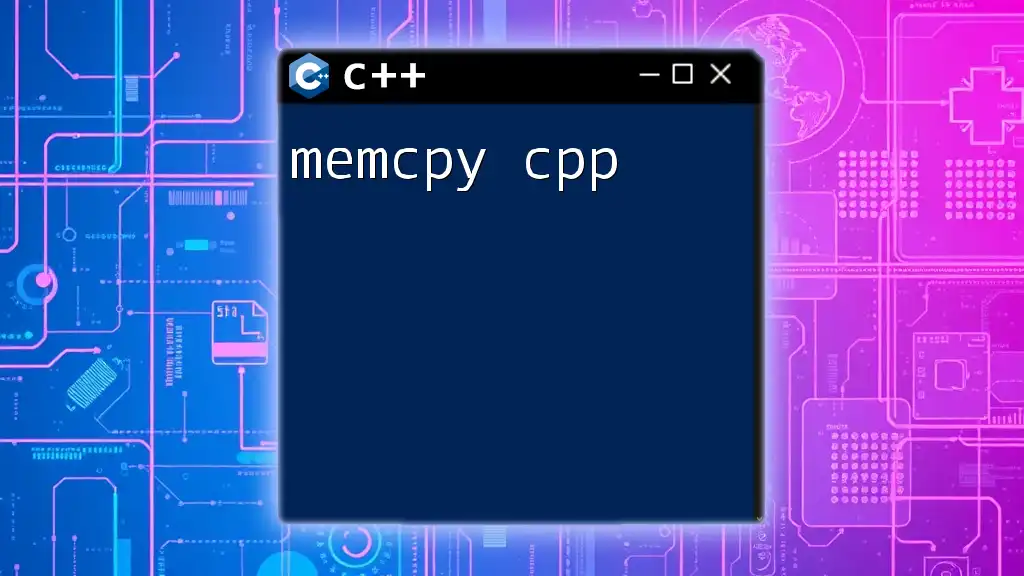
Common Issues and Troubleshooting
While using `minicap_34.cpp`, developers may encounter several common issues that hinder smooth operation:
- Compatibility Issues: Different Android versions or devices may implement changes that could affect how `minicap` functions. Always check for version compatibility.
- Runtime Errors: Memory mismanagement often leads to crashes. Ensure all allocated buffers are appropriately handled and deleted.
For each of these problems, reviewing logs generated via the `<android/log.h>` becomes imperative for diagnosing and fixing errors.
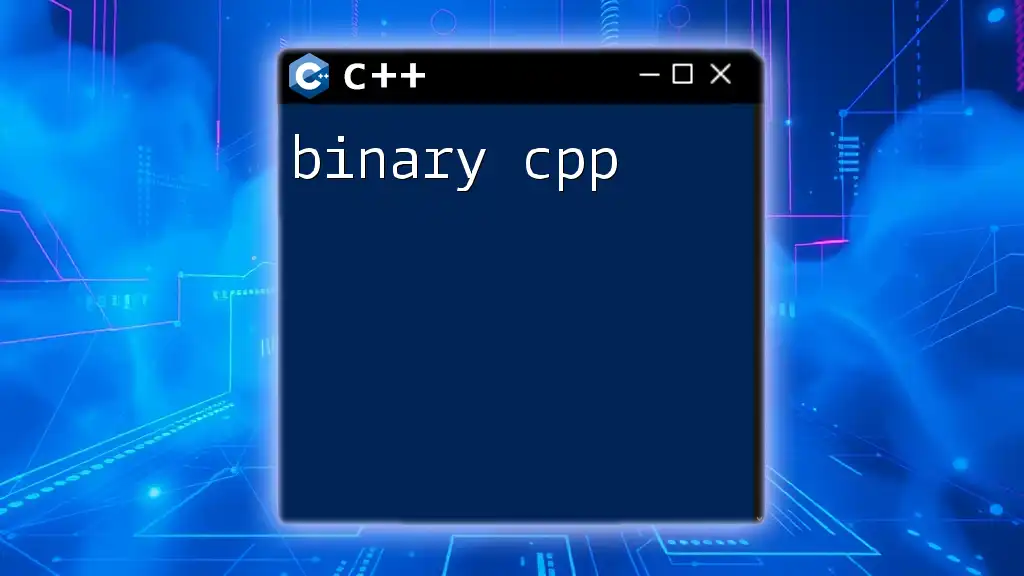
Enhancing minicap_34.cpp
Adding Features
Developers can extend the functionality of `minicap_34.cpp` to include additional features such as video capturing. Enhancements may involve triggering the capture function at regular intervals and stitching images together to produce video output.
Performance Optimizations
Optimizing performance is a crucial part of working with `minicap_34.cpp`. Techniques may include:
- Using more efficient algorithms for data processing.
- Minimizing memory allocations during runtime.
For example, you might implement a strategy to preallocate buffers only when necessary, further reducing memory overhead.
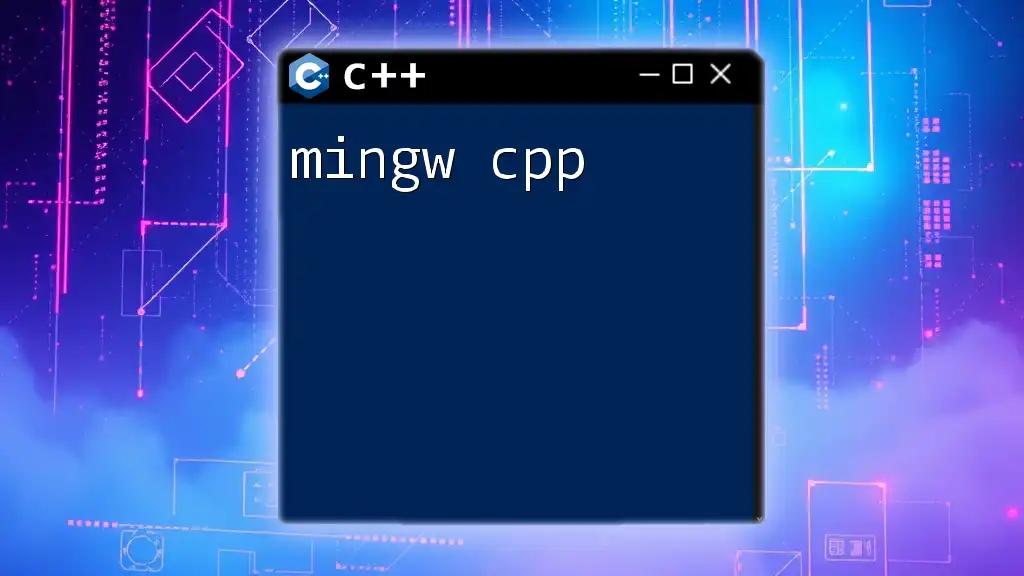
Conclusion
Understanding `minicap_34.cpp` is vital for developers looking to capture Android screens effectively. By mastering this code, you empower yourself to create robust applications capable of live screen capture, which can be immensely helpful in various development scenarios. Don't hesitate to experiment and extend its functionalities, as interactive learning often leads to the best insights and improvements.
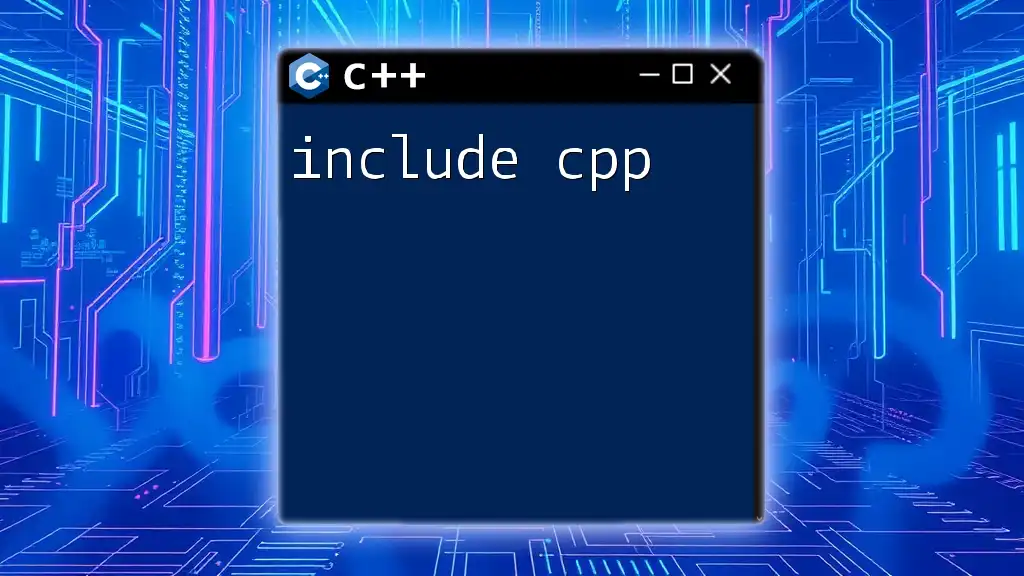
Additional Resources
To further enhance your knowledge of `minicap_34.cpp`, explore the following resources:
- Official Android documentation
- Community forums for troubleshooting and insights
- Tutorials aimed at expanding your skill set in native Android development
For any queries or support, consider joining relevant forums where developers share their experiences and knowledge.