A min-heap in C++ is a complete binary tree where the value of each node is less than or equal to the values of its children, allowing for efficient retrieval of the minimum element.
#include <iostream>
#include <queue>
int main() {
std::priority_queue<int, std::vector<int>, std::greater<int>> minHeap;
minHeap.push(10);
minHeap.push(15);
minHeap.push(5);
std::cout << "The minimum element is: " << minHeap.top() << std::endl; // Outputs: 5
return 0;
}
Understanding the Basics of MinHeap
Heap Data Structure Overview
To understand a MinHeap, we need to start with the concept of a heap: a binary tree that satisfies the heap property, where the parent node is always less than or equal to its child nodes in the case of a MinHeap. This leads us to distinguish between two types of heaps: MaxHeap, where the parent is greater than or equal to its children, and MinHeap, where the opposite holds true.
Properties of a MinHeap
A MinHeap has key characteristics:
- It is a complete binary tree: This means every level of the tree is fully filled except possibly for the last level, which is filled from left to right.
- It follows the MinHeap property: Each node must be smaller than or equal to its children, ensuring that the smallest element is always at the root.

Benefits of Using MinHeap in C++
Performance Advantages
The performance of a MinHeap can be outstanding, particularly in scenarios where you frequently need to access or modify the smallest element.
- Time complexity analysis: The complexity for insertion and deletion operations is O(log n), making it efficient for managing a dynamic dataset.
- Space complexity benefits: A MinHeap typically uses an array structure that allows for compact memory allocation, with space complexity being O(n).
Use Cases
A MinHeap has a range of practical applications:
- Priority Queues: MinHeaps are often used to implement priority queues, where elements with the highest priority are dequeued first.
- Graph Algorithms: Efficiently implementing algorithms such as Dijkstra's and Prim's relies heavily on the properties of a MinHeap.
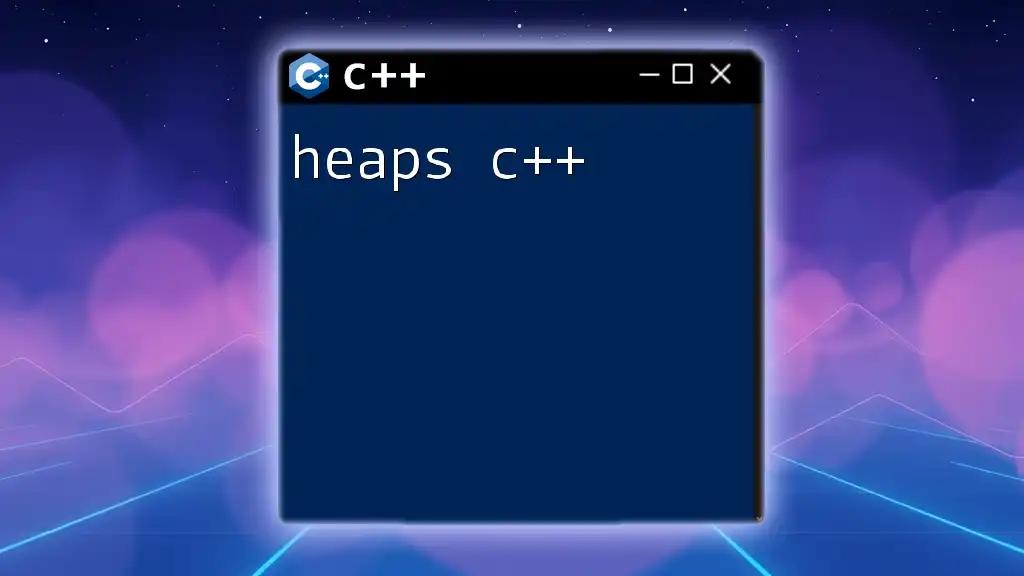
Implementing a MinHeap in C++
Setting Up Your Environment
To begin implementing a MinHeap in C++, ensure you have an appropriate development environment like a C++ compiler (GCC, Clang) and an IDE (Visual Studio, Code::Blocks, etc.).
Basic MinHeap Implementation
MinHeap Class Structure
Here is a simple structure for a MinHeap class:
class MinHeap {
private:
std::vector<int> heap; // Container for heap elements
void siftUp(int index);
void siftDown(int index);
public:
MinHeap();
void insert(int value);
int deleteMin();
int getMin() const;
bool isEmpty() const;
int size() const;
};
This class declaration includes essential members, with private methods for maintaining heap properties.
Constructor and Destructor
The MinHeap constructor initializes the underlying data structure:
MinHeap::MinHeap() {
// Constructor logic if any, such as initializing the heap vector
}
Implementing a destructor is generally not necessary in this simple implementation, as the vector will handle memory management automatically.
Key Operations of MinHeap
Inserting an Element
Insertion into a MinHeap requires adding the new element to the end and then "sifting it up" to restore the heap property:
void MinHeap::insert(int value) {
heap.push_back(value); // Add element to the end
siftUp(heap.size() - 1); // Restore heap property
}
void MinHeap::siftUp(int index) {
int parentIndex = (index - 1) / 2;
while (index > 0 && heap[index] < heap[parentIndex]) {
std::swap(heap[index], heap[parentIndex]);
index = parentIndex;
parentIndex = (index - 1) / 2;
}
}
Removing the Minimum Element
To remove the minimum element, which is always at the root, replace it with the last element and then perform the "sift down" operation:
int MinHeap::deleteMin() {
if (heap.empty()) {
throw std::out_of_range("Heap is empty");
}
int minValue = heap[0]; // The minimum value
heap[0] = heap.back(); // Replace root with last element
heap.pop_back(); // Remove last element
siftDown(0); // Restore heap property
return minValue;
}
void MinHeap::siftDown(int index) {
int leftChildIndex = 2 * index + 1;
int rightChildIndex = 2 * index + 2;
int smallest = index;
if (leftChildIndex < heap.size() && heap[leftChildIndex] < heap[smallest]) {
smallest = leftChildIndex;
}
if (rightChildIndex < heap.size() && heap[rightChildIndex] < heap[smallest]) {
smallest = rightChildIndex;
}
if (smallest != index) {
std::swap(heap[index], heap[smallest]);
siftDown(smallest);
}
}
Peeking at the Minimum Element
Peeking allows you to access the minimum element without removing it:
int MinHeap::getMin() const {
if (heap.empty()) {
throw std::out_of_range("Heap is empty");
}
return heap[0]; // The minimum element, without removal
}
Heapify Operations
Building a MinHeap from an Array
You can create a MinHeap directly from an array with a function called `buildHeap`:
void buildHeap(const std::vector<int>& elements) {
heap = elements; // Initializing the heap
for (int i = (heap.size() / 2) - 1; i >= 0; --i) {
siftDown(i); // Sift down each element to establish heap property
}
}
Heap Sort using MinHeap
Heap sort can utilize the MinHeap to sort an array of elements efficiently:
void heapSort(std::vector<int>& elements) {
buildHeap(elements); // Create the MinHeap
for (int i = elements.size() - 1; i > 0; --i) {
std::swap(elements[0], elements[i]);
heap.pop_back(); // Remove the last element from the heap
siftDown(0); // Restore heap property from the root
}
}
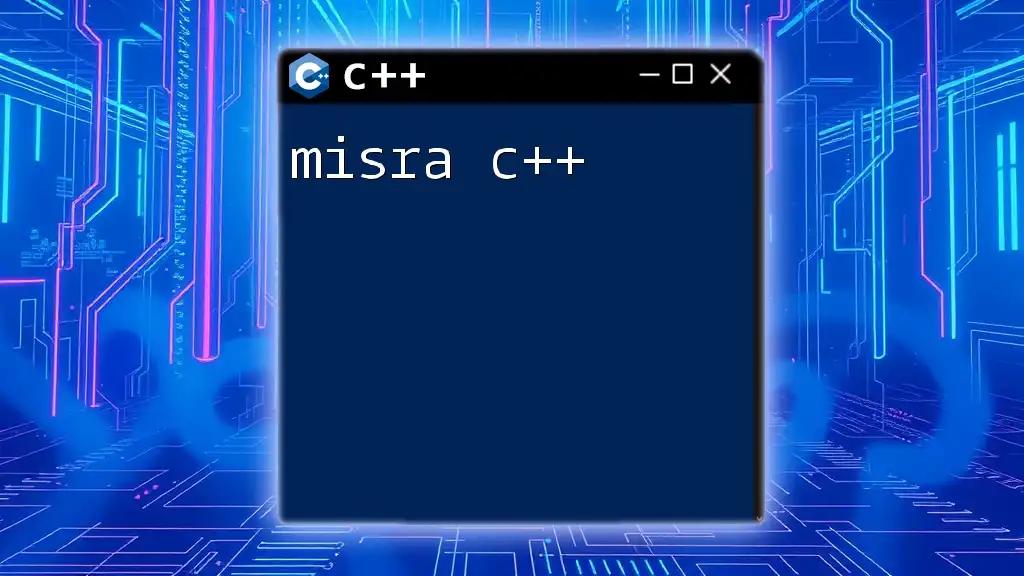
Advanced MinHeap Operations
Decrease Key Operation
In some scenarios, you may need to decrease the value of an element within the heap. This requires updating the value and then sifting it up:
void MinHeap::decreaseKey(int index, int newValue) {
if (index >= heap.size() || newValue > heap[index]) {
throw std::invalid_argument("Invalid operation");
}
heap[index] = newValue; // Update the value
siftUp(index); // Restore heap property
}
Delete Operation
Deleting an arbitrary element ensures that you maintain the heap structure:
void MinHeap::deleteKey(int index) {
if (index >= heap.size()) {
throw std::out_of_range("Index out of range");
}
decreaseKey(index, INT_MIN); // Decrease value to minimum
deleteMin(); // Remove the minimum element
}

Common Mistakes and How to Avoid Them
When implementing a MinHeap, it is easy to overlook fundamental details, like index calculations. Always remember that in a binary heap representation in an array:
- Left Child = `2 * index + 1`
- Right Child = `2 * index + 2`
- Parent = `(index - 1) / 2`
Also, be cautious about maintaining the heap property during element insertions and deletions. Regularly debug with simple test cases to catch these errors early.
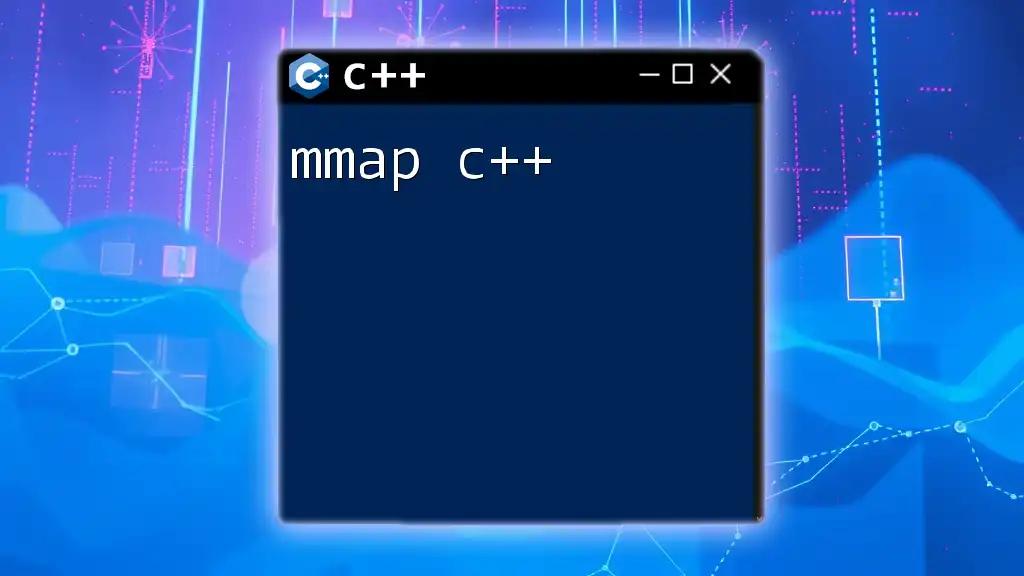
Performance Analysis of MinHeap
Time Complexity Summary
Understanding the complexities helps in selecting the right data structure for your needs:
- Insert: O(log n)
- Delete: O(log n)
- Peek: O(1)
- Heapify: O(n)
Space Complexity Considerations
The overall space complexity is O(n) as we need to store the heap elements, and the size grows with the number of elements you manipulate.
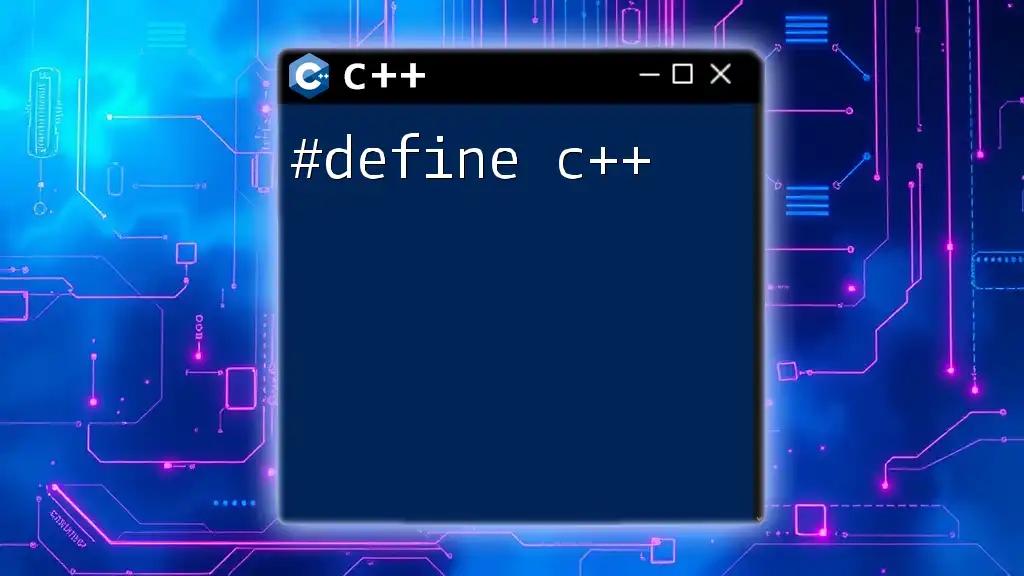
Practical Applications of C++ MinHeap
Handling Priority Queues
A MinHeap can effectively manage a priority queue by allowing you to efficiently insert elements based on priority and retrieve the highest priority items in constant time.
Here’s how you can integrate a MinHeap with a simple task scheduling example:
struct Task {
int priority;
std::string name;
};
bool operator>(const Task& lhs, const Task& rhs) {
return lhs.priority > rhs.priority; // Compare based on priority
}
// Use MinHeap to manage tasks
Graph Algorithms Using MinHeap
Graph algorithms such as Dijkstra's use MinHeap to efficiently retrieve and process the lowest cost nodes. For example, in Dijkstra's algorithm, the MinHeap can offer rapid access to the node with the smallest tentative distance, making the algorithm both efficient and effective.
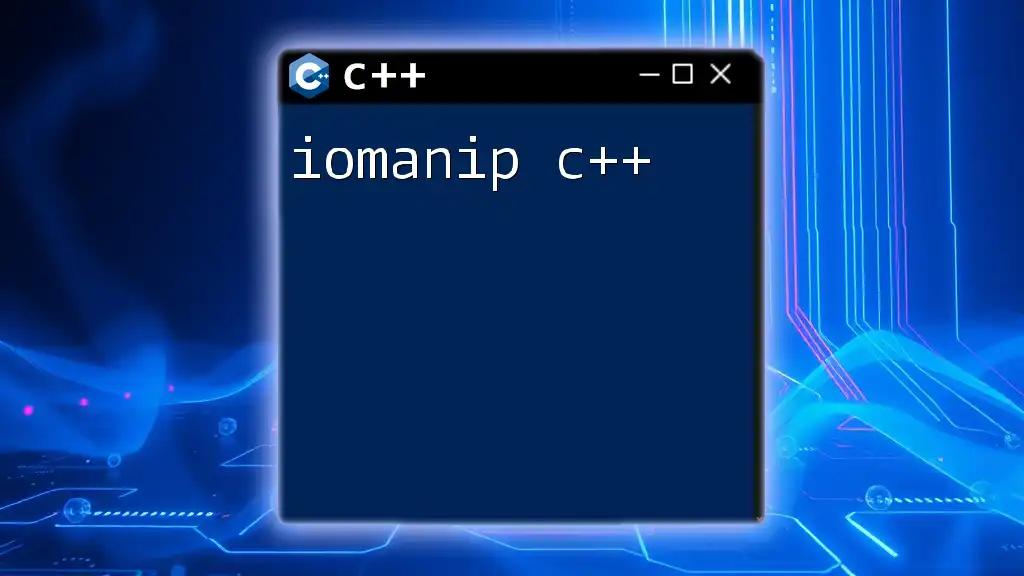
Conclusion
A MinHeap in C++ is a powerful data structure that offers excellent performance for dynamic datasets, particularly for operations involving minimum value access. By understanding its implementation and use cases, you can leverage it to solve a variety of computational problems efficiently.
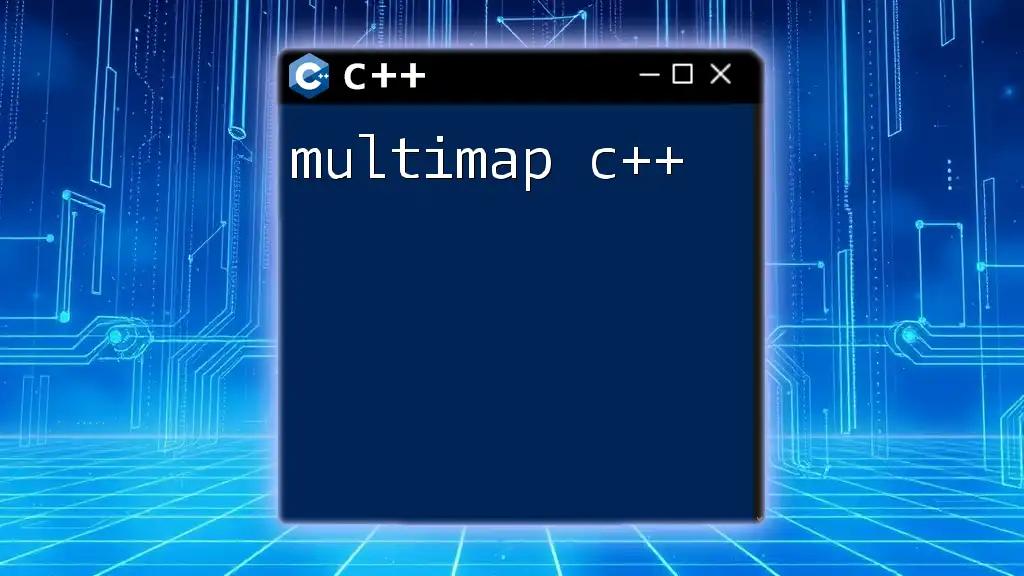
FAQs About MinHeap in C++
What is the difference between a MinHeap and a priority queue?
While both structures manage data based on priority, a MinHeap is specifically a binary tree structure that ensures the smallest element is always accessible. A priority queue can be implemented using various data structures.
How can you implement a MinHeap without using recursion?
By employing iterative methods for both sift-up and sift-down operations, you can avoid recursion and manage memory more explicitly, providing consistent performance.
How does MinHeap handle duplicates?
A MinHeap can store duplicate values without any issue, as long as the heap property is maintained. Duplicates are treated as equivalent elements and can coexist within the heap.
By understanding and utilizing the properties and operations of a MinHeap in C++, you empower yourself to solve complex problems with efficiency and elegance.