Min heapify in C++ is a process to maintain the min-heap property of a binary tree, ensuring that each parent node is smaller than its child nodes, typically used in heap sort and priority queues. Here's a code snippet demonstrating the min-heapify function:
void minHeapify(int arr[], int n, int i) {
int smallest = i; // Initialize smallest as root
int left = 2 * i + 1; // left child index
int right = 2 * i + 2; // right child index
// If left child is smaller than root
if (left < n && arr[left] < arr[smallest])
smallest = left;
// If right child is smaller than smallest so far
if (right < n && arr[right] < arr[smallest])
smallest = right;
// If smallest is not root
if (smallest != i) {
std::swap(arr[i], arr[smallest]); // Swap
minHeapify(arr, n, smallest); // Recursively heapify the affected sub-tree
}
}
What is a Min-Heap?
A min-heap is a specialized tree-based data structure that satisfies the min-heap property. This means that for any given node, its value is less than or equal to the values of its children. Min-heaps are commonly used to implement priority queues, where the highest priority element (the smallest element in the case of a min-heap) can be fetched in constant time.
Characteristics of Min-Heaps
- Complete Binary Tree: A min-heap is structured as a complete binary tree, meaning all levels are fully filled except possibly for the last level, which is filled from left to right.
- Heap Property: The hierarchical structure enforces that each parent node's value is smaller than that of its children, which facilitates efficient retrieval, insertion, and deletion operations.
The significance of the min-heap structure allows for efficient algorithms to be implemented, notably in sorting and priority management.

The Min Heapify Process
What is Min Heapify?
The min heapify operation is fundamental in maintaining the min-heap property whenever the structure is altered, such as during insertion, deletion, or array construction. The main objective of this operation is to adjust a binary tree to ensure that the min-heap property is satisfied starting from a certain node downwards.
When to Use Min Heapify
Min heapify becomes essential in several scenarios, including:
- During the construction of a min-heap from an unordered array.
- After removing the root element from the heap, to maintain the min-heap property.
- Any time data structures are modified that may potentially violate the min-heap property.

Understanding the Algorithm
Steps of the Min Heapify Algorithm
- Identify the Parent Node: Start with the parent node assigned to the variable.
- Compare with Children: Identify left and right child nodes and compare their values with the parent's value.
- Swap if Necessary: If either child has a smaller value than the parent, swap the parent with the smaller child.
- Recursive Call: Continue the process for the subtree where the swap occurred until the min-heap property is restored.
Visual Representation of Min Heapify
While not visualized here, consider a binary tree where the parent node has been violated due to a larger value than its children. When we apply min heapify, the process ensures that the smallest value bubbles to the parent position, maintaining the min-heap structure efficiently.

C++ Implementation of Min Heapify
Code Snippet for Min Heapify
void minHeapify(int arr[], int n, int i) {
int smallest = i; // Initialize the smallest as the root
int left = 2 * i + 1; // Calculate the left child index
int right = 2 * i + 2; // Calculate the right child index
// If the left child exists and is smaller than the root
if (left < n && arr[left] < arr[smallest]) {
smallest = left;
}
// If the right child exists and is smaller than the smallest element found so far
if (right < n && arr[right] < arr[smallest]) {
smallest = right;
}
// If the smallest is not the root
if (smallest != i) {
std::swap(arr[i], arr[smallest]); // Swap the root with the smallest
// Recursively heapify the affected subtree
minHeapify(arr, n, smallest);
}
}
Explanation of the Code
- The function `minHeapify` accepts an array `arr[]`, the size of the heap `n`, and the current index `i`.
- It initializes `smallest` to the index of the parent node and calculates the indices of its left and right children.
- The algorithm checks if the left child exists and is smaller than the current smallest. If it is, `smallest` is updated to the left child's index.
- A similar check is performed for the right child.
- If the smallest value is not at the root, the algorithm swaps the values at the indices, ensuring that the smaller child takes the parent's place.
- Finally, the `minHeapify` operation is recursively called on the affected subtree to ensure the min-heap structure is restored throughout.
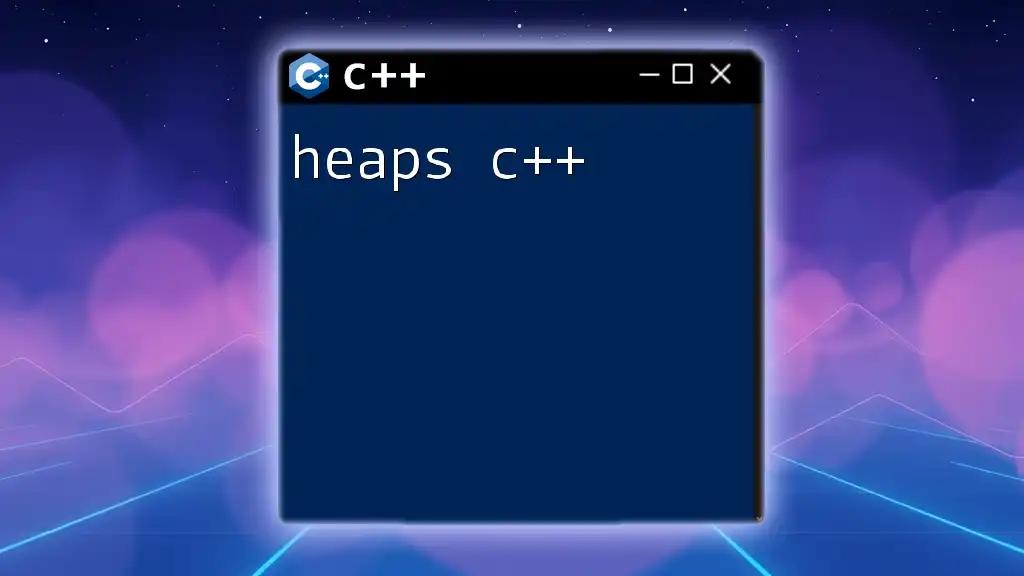
Using Min Heapify in Practice
Building a Min Heap
To effectively build a min-heap from an unordered array, we can utilize the `minHeapify` function iteratively starting from the last non-leaf node down to the root. This operation ensures that the entire heap maintains its properties by fixing any violations encountered along the way.
Code Snippet for Building a Min Heap
void buildMinHeap(int arr[], int n) {
// Starting from the last non-leaf node and heapify each node
for (int i = n / 2 - 1; i >= 0; i--) {
minHeapify(arr, n, i);
}
}
Complete Example: Min Heap Construction
#include <iostream>
using namespace std;
void minHeapify(int arr[], int n, int i);
void buildMinHeap(int arr[], int n);
int main() {
int arr[] = {3, 9, 2, 1, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
buildMinHeap(arr, n);
cout << "Min heap constructed from the array: ";
for (int i = 0; i < n; ++i)
cout << arr[i] << " ";
return 0;
}
In this example, the unordered array `{3, 9, 2, 1, 4, 5}` is transformed into a min-heap. The `buildMinHeap` function iterates from the last non-leaf node index to the root node, ensuring that each part of the tree adheres to the min-heap property.

Common Mistakes to Avoid
Misunderstanding Heap Indices
A common pitfall when implementing heap structures in C++ is overlooking the 0-based indexing. For a node at index `i`, its children can be found at indices `2i + 1` (left) and `2i + 2` (right). Failing to account for this can lead to incorrect swapping and structural violations.
Failing to Handle Edge Cases
When implementing min heapify, it is crucial to handle any edge cases, such as:
- Ensuring that the indices for both left and right children do not exceed the heap size (`n`).
- Properly managing the cases where a node might not have children, especially at the lower levels of the tree.
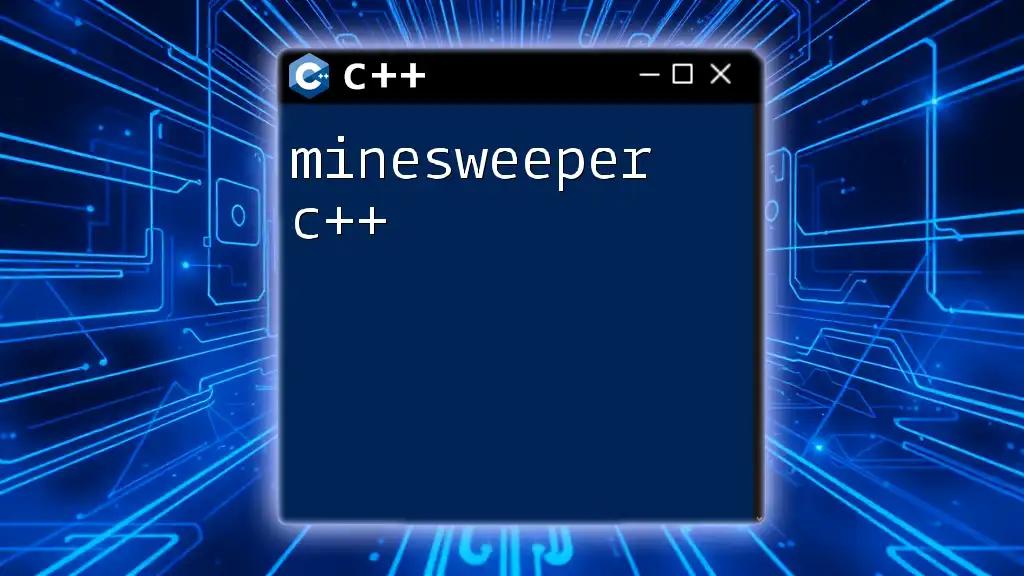
Conclusion
In summary, understanding and implementing min heapify c++ is vital for both managing heaps effectively and constructing algorithms that rely on the min-heap structure. Whether you're building priority queues or performing sorting operations, grasping min heapify will serve you well in your journey through data structures and algorithms.

Additional Resources
For those interested in deepening their understanding, consider exploring online coding environments, participating in coding forums, or checking out recommended textbooks on data structures and algorithms that delve into heaps and their applications.