Dynamic memory in C++ allows programmers to allocate and deallocate memory at runtime using pointers, enabling more flexible memory management for complex data structures.
#include <iostream>
int main() {
int* ptr = new int; // allocate memory
*ptr = 42; // assign value
std::cout << *ptr << std::endl; // output: 42
delete ptr; // deallocate memory
return 0;
}
Understanding Dynamic Memory in C++
What is Dynamic Memory?
Dynamic memory refers to the allocation of memory during the execution of a program, as opposed to at compile time. In C++, this is mainly achieved using the `new` and `delete` operators. Dynamic memory is crucial for tasks that require variable-sized data structures or memory whose lifetime needs to exceed the scope of its creating function.
Why Use Dynamic Memory in C++?
Using dynamic memory has several advantages, particularly when dealing with large data sets or data structures that require flexibility:
- Flexibility: Unlike static memory allocation, dynamic memory allows you to allocate more or less memory depending on the needs of your application at runtime.
- Resource Efficiency: Only the required amount of memory is allocated, which helps in optimizing the use of system resources.
- Lifetime Management: Dynamically allocated memory can persist beyond the scope of the function that created it, enabling more complex data management strategies.
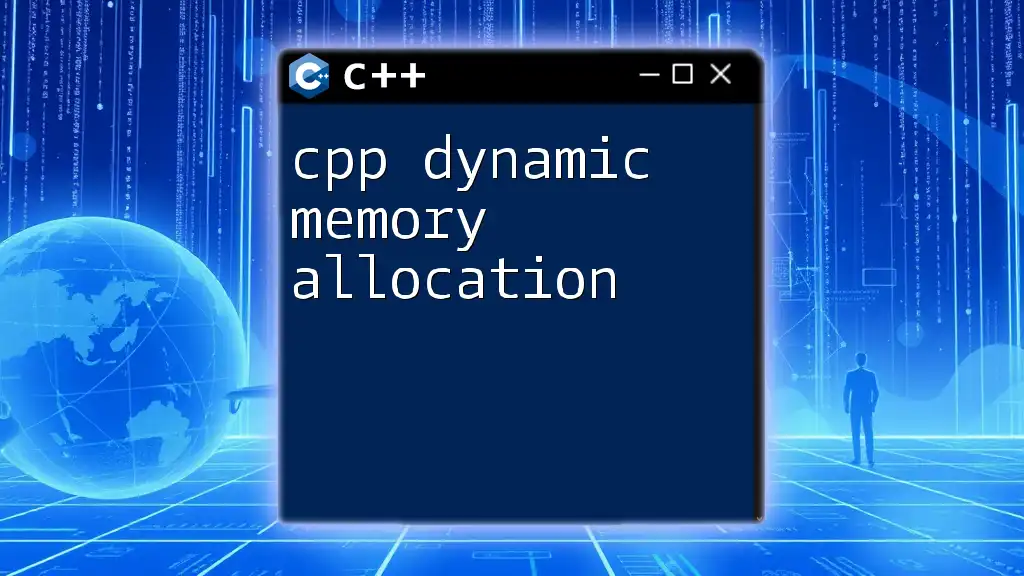
Basic Concepts of Dynamic Memory Allocation in C++
Memory Management
In C++, memory is managed in different segments, primarily the stack and the heap. The stack is used for static memory allocation where memory is reserved during compile time, while the heap is reserved for dynamic memory allocation where memory is allocated during runtime. Understanding the distinction is essential for effective memory management.
Key Terms to Know
- Pointers: These are variables that store the memory address of another variable. They are fundamental to dynamic memory allocation, as they allow you to reference and manipulate dynamically allocated entities directly.
- Memory Address: Each byte in memory has a unique address. Understanding how memory is accessed by address is vital for debugging and efficient memory management.
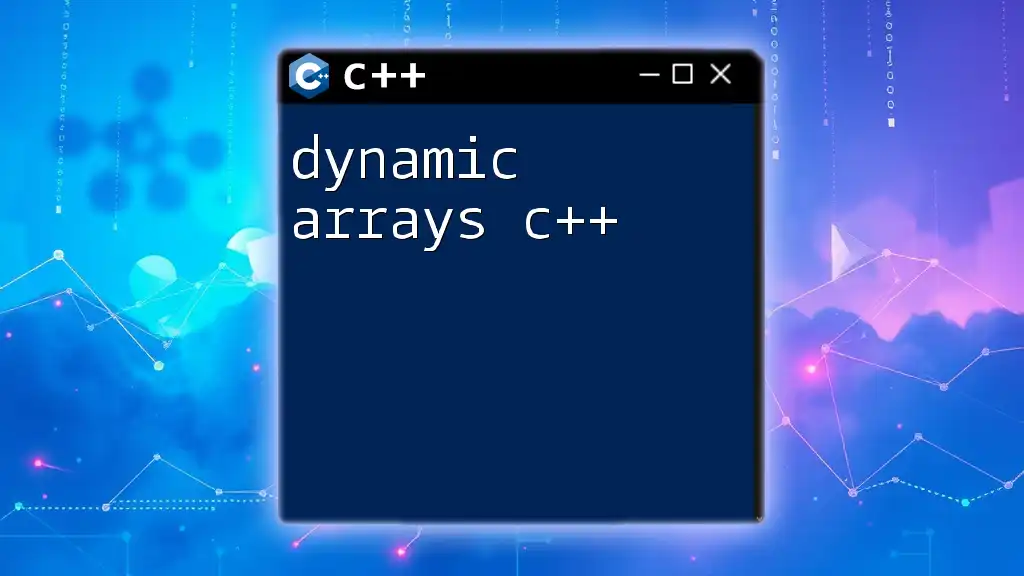
Dynamic Memory Allocation in C++
The `new` Operator
Basic Syntax and Usage
The `new` operator in C++ is used to allocate memory. Its syntax is straightforward:
int* ptr = new int; // Allocating a single integer
This line allocates memory for an integer on the heap and returns its memory address, which is stored in the pointer variable `ptr`.
Allocating Arrays
You can also allocate arrays dynamically:
int* arr = new int[10]; // Allocating an array of 10 integers
In this case, memory for ten integers is allocated, and the starting address is assigned to `arr`. You can access individual elements using array indexing, e.g., `arr[0]`, `arr[1]`, etc.
The `delete` Operator
Syntax and Usage
The importance of deallocating memory cannot be overstated. The `delete` operator is used to free the memory allocated for single objects:
delete ptr; // Deleting a single integer
For arrays, the syntax is:
delete[] arr; // Deleting a dynamically allocated array
Properly using `delete` prevents memory leaks, which can lead to resource exhaustion in long-running applications.
Common Mistakes
When working with dynamic memory, be aware of potential pitfalls, such as memory leaks and dangling pointers. A memory leak occurs when dynamically allocated memory is not released, leading to wasted memory. A dangling pointer refers to a pointer that points to memory that has already been freed. Always ensure that every `new` has a corresponding `delete`.
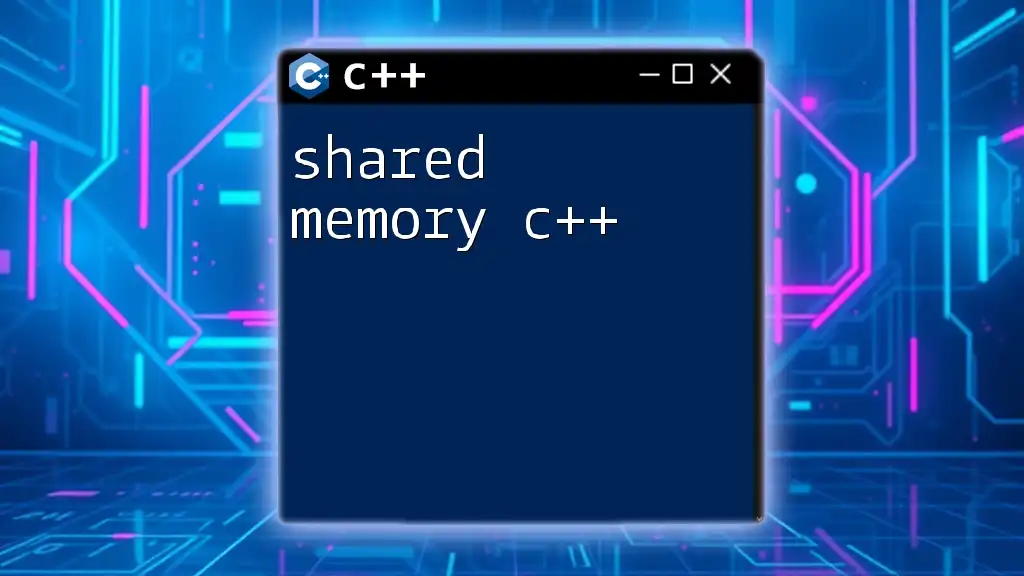
Exploring Advanced Dynamic Memory Concepts
Smart Pointers in C++
Introduction to Smart Pointers
Smart pointers simplify memory management and significantly reduce the chances of memory leaks. In modern C++, `std::unique_ptr`, `std::shared_ptr`, and `std::weak_ptr` are included in the Standard Template Library (STL). They automatically manage memory, ensuring proper deallocation when the object goes out of scope or is no longer needed.
Examples of Smart Pointer Usage
The use of `unique_ptr` allows you to ensure exclusive ownership of a dynamic object:
std::unique_ptr<int> uptr(new int);
Here, `uptr` manages a pointer to an integer. When `uptr` goes out of scope, the memory is automatically freed.
Similarly, `shared_ptr` allows multiple pointers to share ownership of a single object:
std::shared_ptr<int> sptr = std::make_shared<int>(10);
This `shared_ptr` will automatically release the memory when all references to it are out of scope.
Dynamic Memory Allocation in Data Structures
Creating Linked Lists
Linked lists are classic examples of data structures that heavily utilize dynamic memory allocation. Each node of a linked list typically consists of a data element and a pointer to the next node. Consider the following structure:
struct Node {
int data;
Node* next;
};
In this example, a new node can be dynamically allocated as follows:
Node* newNode = new Node();
newNode->data = 10; // Assigning a value
newNode->next = nullptr; // Default value
Dynamic Arrays
Dynamic arrays allow resizing, which is not possible with statically allocated arrays. When you need to resize, create a new memory allocation and copy the existing data:
int* newArr = new int[newSize];
// Copy existing data
memcpy(newArr, oldArr, oldSize * sizeof(int));
delete[] oldArr; // Free the old array
This process underscores the flexibility of dynamic memory allocation in C++.
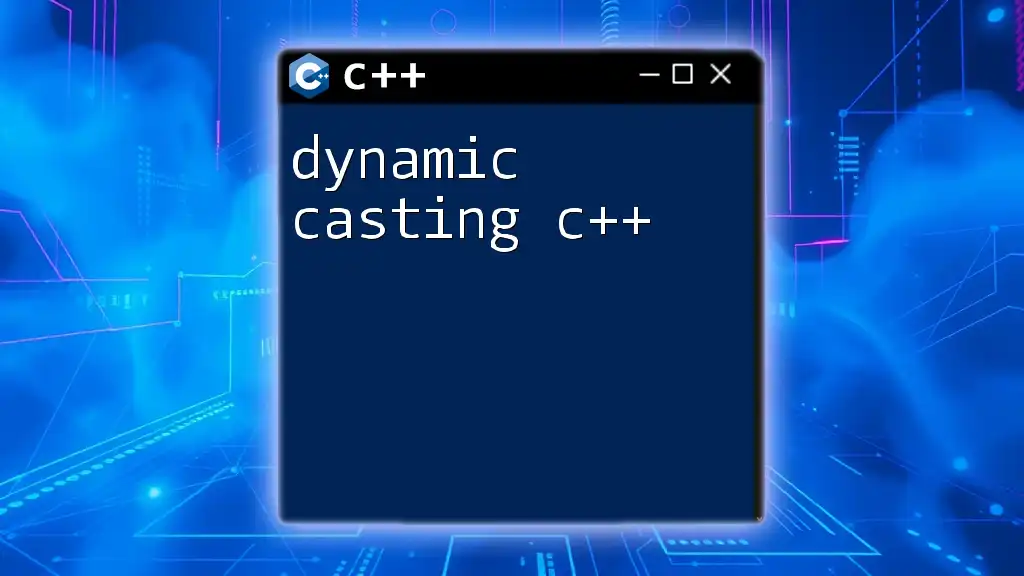
Best Practices for Dynamic Memory Management in C++
Memory Leak Prevention
Avoiding memory leaks is critical for maintaining system performance. Techniques include:
- Always pair every `new` with a `delete`.
- Use smart pointers for automatic memory management.
- Utilize memory management tools to detect leaks during development.
Using RAII (Resource Acquisition Is Initialization)
RAII is a programming idiom in C++ that ties resource management to object lifetime. By constructing objects that manage resources (like memory) and releasing those resources during destruction, you ensure that memory leaks do not occur. This principle enhances the reliability of your software.
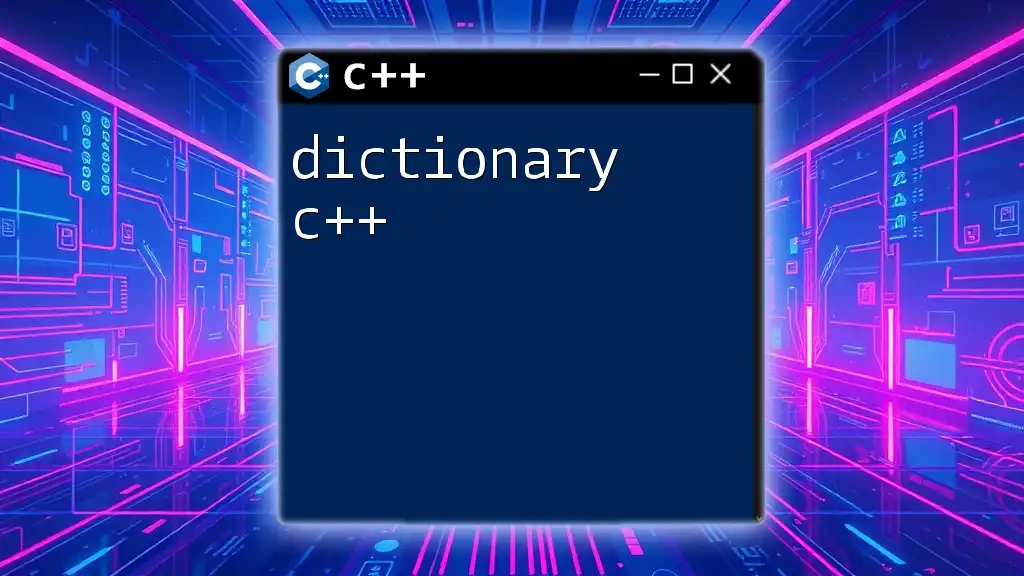
Conclusion
Recap of Key Concepts
Dynamic memory allocation in C++ is a powerful tool that provides flexibility and efficient resource management. Understanding the usage of `new`, `delete`, and smart pointers can significantly enhance your programming capabilities. Following best practices such as RAII will lead to cleaner, more maintainable code.
Further Learning Resources
For those looking to dive deeper, numerous resources are available, including textbooks like "C++ Primer" and online courses on platforms like Udacity and Coursera. The C++ official documentation also serves as an invaluable reference.
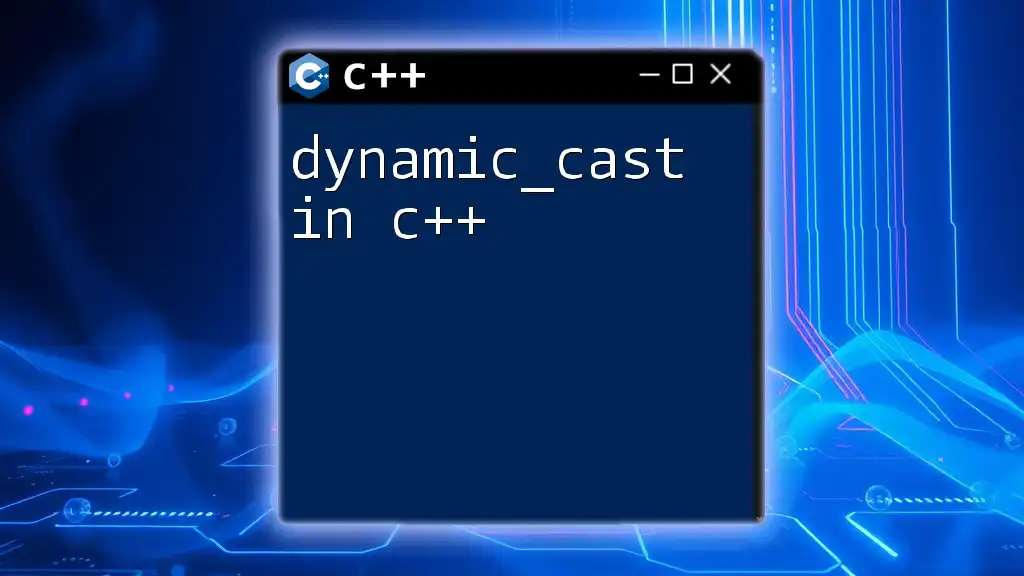
FAQs on Dynamic Memory in C++
Dynamic memory in C++ raises many questions. Common inquiries include:
- What happens if I forget to delete dynamically allocated memory?
- How do I safely share dynamic memory between functions?
- What tools help detect memory leaks in C++?
By addressing these questions, developers can build a robust understanding of dynamic memory allocation in C++.