A data member in C++ is a variable that is declared within a class and is used to store the attributes of an object.
Here’s an example of a class with a data member:
class Car {
public:
int speed; // data member to store the speed of the car
};
What are Data Members in C++?
Definition of Data Members
In C++, a data member is a variable that is associated with a class or an object. Data members hold the state or attributes of the class, while methods (member functions) define the behavior. A fundamental understanding of data members is essential in object-oriented programming, as they allow encapsulation of data within the class.
Types of Data Members
Data members in C++ can be categorized into two main types:
Instance Data Members: These members are unique to each instance (object) of a class. Each object has its own copy of the instance data member.
Static Data Members: Unlike instance members, static data members are shared among all instances of a class. There's only one copy of a static data member, which belongs to the class rather than any individual object.
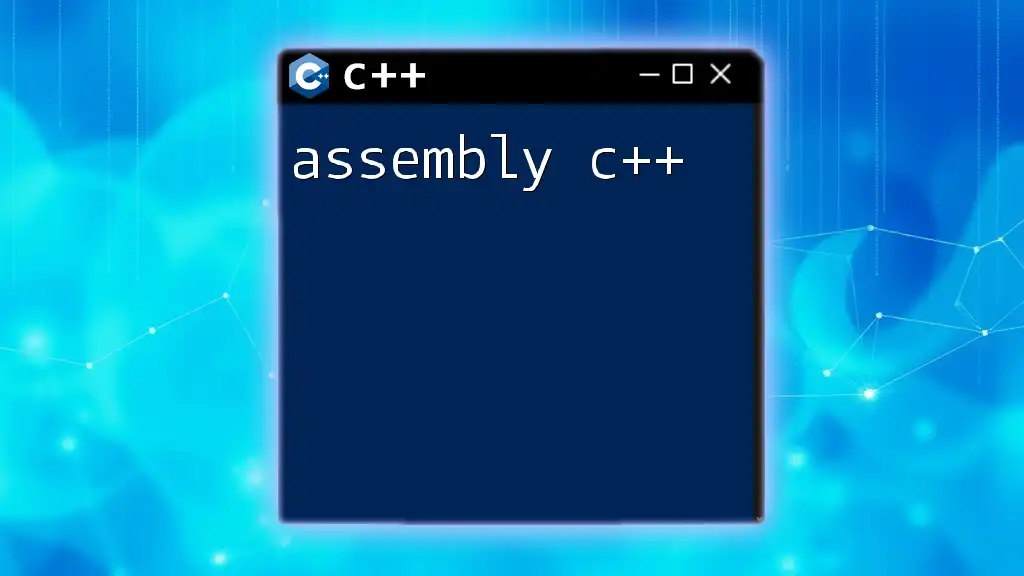
Declaring Data Members in C++
Syntax for Declaring Data Members
Declaring data members involves specifying the access level (public, private, or protected) and the data type. The following example demonstrates how to declare instance and static data members within a class:
class Example {
public:
int instanceData; // Instance Data Member
static int staticData; // Static Data Member
};
Access Specifiers: Public, Private, and Protected
Access specifiers control the visibility of data members:
Public Data Members
Public members are accessible from outside the class. For example:
class PublicExample {
public:
int value; // Public data member
};
Here, `value` can be accessed from any function that has visibility of the `PublicExample` class.
Private Data Members
Private members cannot be accessed directly from outside the class. They are typically used to enforce encapsulation. Example:
class PrivateExample {
private:
int value; // Private data member
};
To access `value`, you must use public methods, known as getters and setters.
Protected Data Members
Protected members are similar to private members but are accessible in derived classes. For instance:
class Base {
protected:
int value; // Protected data member
};
class Derived : public Base {
public:
void setValue(int v) { value = v; } // Accessing protected member
};
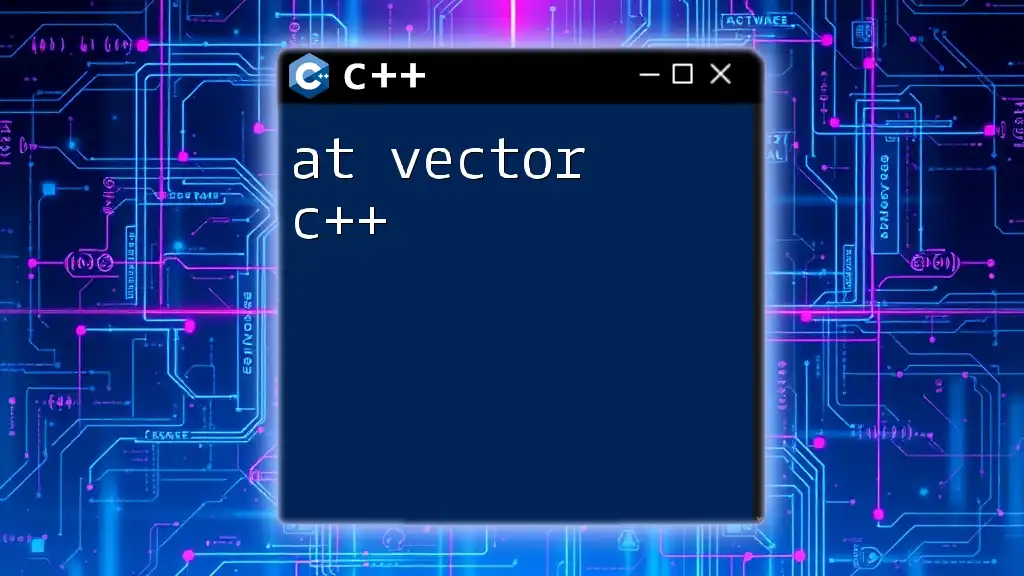
Accessing Data Members
Accessing Public Data Members
Public data members can be accessed directly using the object of the class. Example:
PublicExample obj;
obj.value = 10; // Accessing public member
Accessing Private Data Members
Private members must be accessed through public methods. Example:
class PrivateExample {
private:
int value;
public:
void setValue(int v) { value = v; } // Setter method
int getValue() { return value; } // Getter method
};
PrivateExample obj;
obj.setValue(5); // Setting value via method
int val = obj.getValue(); // Getting value via method
Accessing Static Data Members
Static data members are accessed using the class name or through an object of the class. Example:
class StaticExample {
public:
static int count; // Declaration
};
// Definition outside the class
int StaticExample::count = 0;
// Accessing static data member
int main() {
StaticExample::count = 5; // Access via class name
StaticExample obj;
obj.count = 10; // Access via object
}
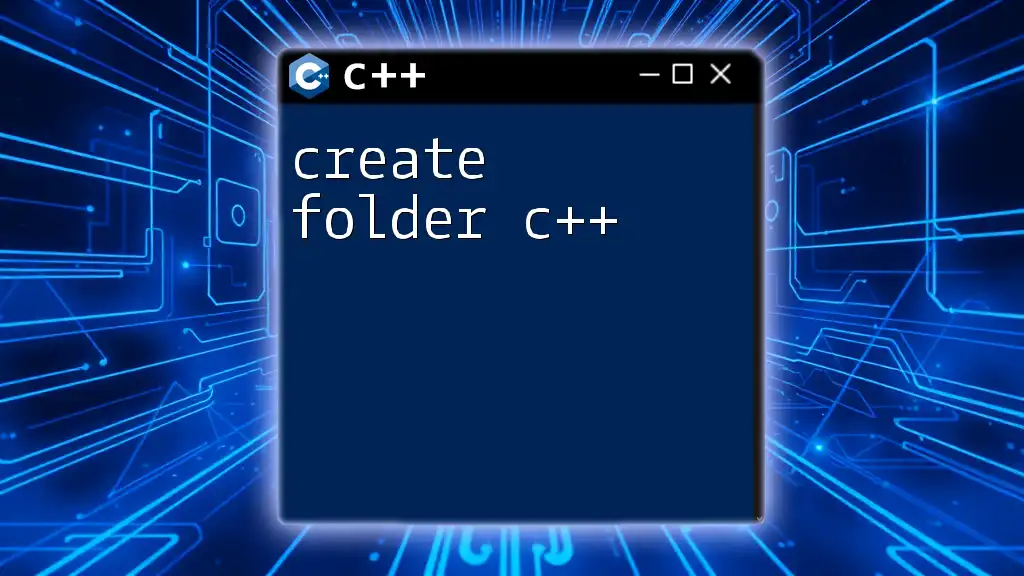
Initializing Data Members
Default Initialization
Data members can be default initialized when defined. Consider the following example:
class DefaultInit {
public:
int a{0}; // Automatically initialized to 0
};
Constructor Initialization
You can initialize data members using constructors, providing values at the time of object creation. Example:
class InitExample {
public:
int x;
// Constructor with initialization list
InitExample(int val) : x(val) {}
};
InitExample obj(10); // x is initialized to 10
Static Data Members Initialization
Static data members must be defined outside the class. Here's how it works:
int StaticExample::count = 0; // Definition of static member
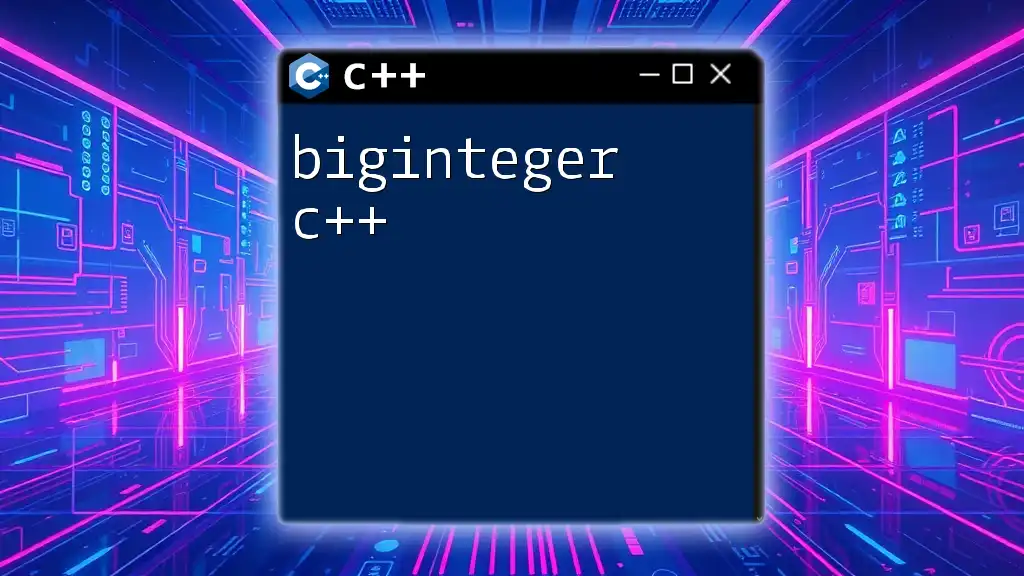
Best Practices for Using Data Members in C++
Encapsulation and Data Hiding
By limiting access to members, you enforce encapsulation, allowing the internal state of an object to be hidden from the outside. This encapsulation aids in maintaining integrity and reduces dependencies, which can lead to more maintainable code. Always prefer private data members with public getters and setters.
Consistency in Naming Conventions
Adhering to consistent naming conventions greatly enhances code readability. Using camelCase or snake_case helps differentiate between variables and data types, improving clarity. For instance, using `firstName` for an instance member can make the code intuitive.
Minimizing Data Exposure
Exposing too many data members publicly can lead to unintended modifications. Aim to keep data members private and provide public interfaces to control access. This practice enhances the robustness of your classes.
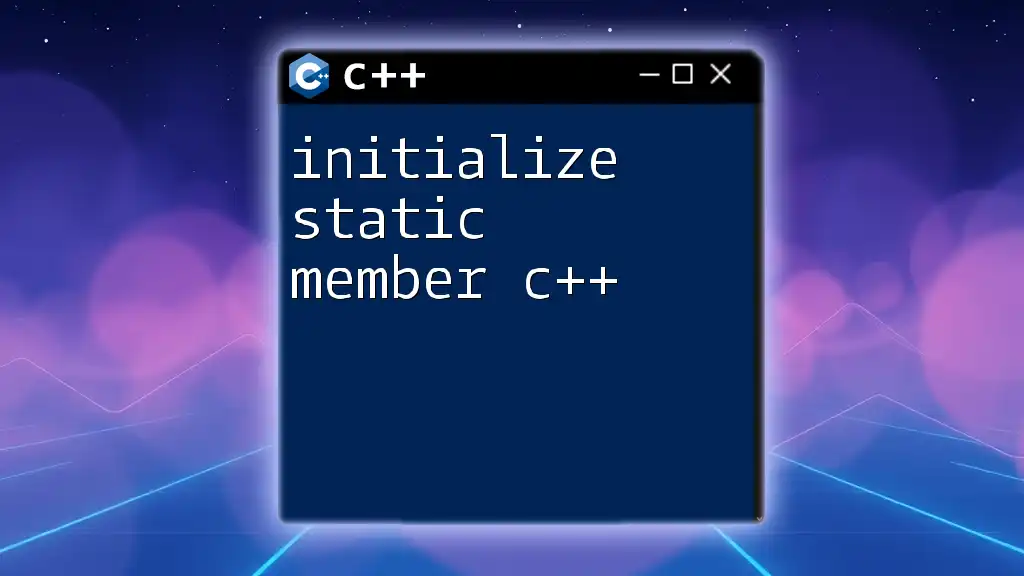
Common Mistakes to Avoid
Uninitialized Data Members
One common pitfall is leaving data members uninitialized. Failing to initialize members can lead to unpredictable behavior and hard-to-track bugs. Always ensure that each member has a defined initial value, preferably through constructors or default initializations.
Misusing Static Data Members
A frequent misstep involves the misuse of static data members. Static members should be used judiciously, as they share a common state across all instances, which can lead to unexpected behavior. Ensure that static members are genuinely shared attributes rather than individual instance properties.
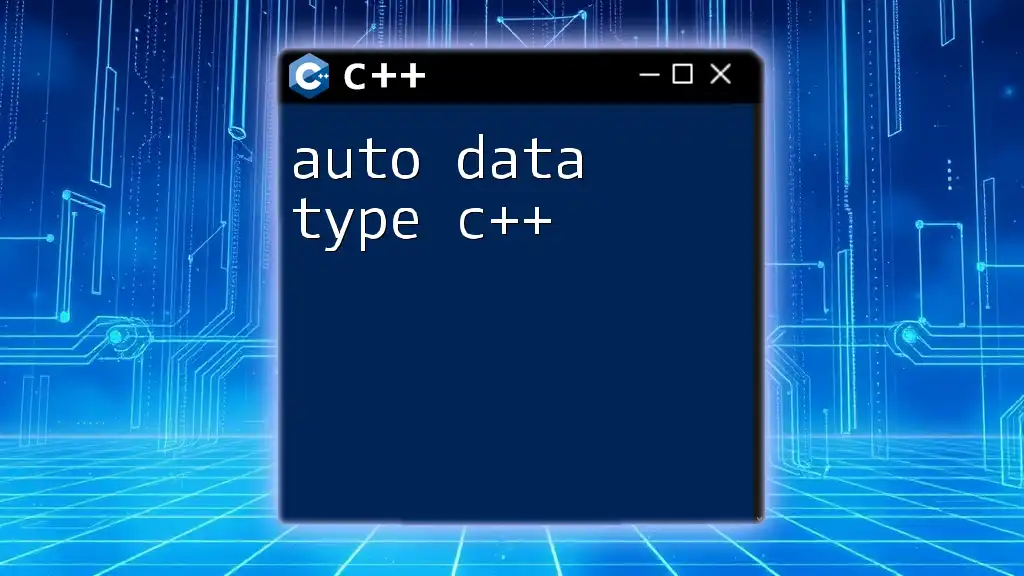
Conclusion
Understanding data members in C++ is crucial for effective object-oriented programming. From their declaration and access mechanisms to best practices for their use, data members form the backbone of any C++ class. By following the guidelines and avoiding common pitfalls, you can write robust, maintainable code that harnesses the full power of object-oriented principles.
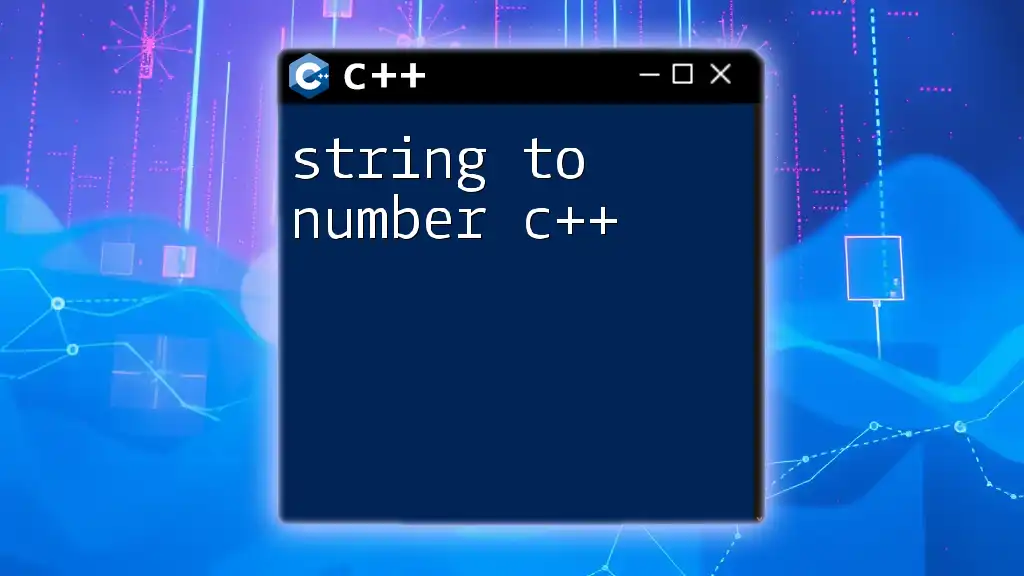
Call to Action
Begin practicing your skills with data members. Experiment with creating classes, defining access specifiers, and utilizing constructors for member initialization. For further learning, consider exploring resources or courses that expand on your C++ knowledge, particularly around object-oriented programming practices.