To create a folder in C++, you can use the `mkdir` function from the `<sys/stat.h>` library, as shown in the following code snippet:
#include <sys/stat.h>
int main() {
mkdir("NewFolder", 0777); // Creates a folder named "NewFolder" with full permissions
return 0;
}
Understanding the C++ Standard Library
Overview of the `<filesystem>` Library
The `<filesystem>` library in C++ is a versatile and powerful tool designed to simplify file system operations. Introduced in C++17, this library allows developers to interact with the file system in a more intuitive manner. Its capabilities extend beyond merely reading and writing files; it provides the means to navigate, manipulate, and manage directories and files within the file system.
One of the main benefits of using the `<filesystem>` library is its ability to handle cross-platform operations seamlessly. This means you can write code that will work on various operating systems such as Windows, macOS, and Linux without needing to worry about specific system calls.
Key Features
The `<filesystem>` library offers a structured way to perform a wide range of operations, including:
- Navigating through directories and subdirectories
- Creating, renaming, and removing files and folders
- Checking for file existence and status (like permissions and modification time)
This library not only streamlines directory operations but also abstracts away the complexity involved in dealing with different file system architectures across platforms.

Creating a Folder Using C++
Basic Syntax of `std::filesystem::create_directory`
To create a folder in C++, you primarily utilize the `std::filesystem::create_directory` function. The basic syntax looks like this:
std::filesystem::create_directory(path);
This function takes a single argument—the path of the directory you want to create. It's important to note that this function returns a boolean value. If the directory is created successfully, it returns `true`; if the directory already exists or cannot be created, it returns `false`.
Example: Creating a Single Folder
Let's explore a simple example for creating a folder:
#include <iostream>
#include <filesystem>
int main() {
std::string path = "NewFolder";
// Create a directory
if (std::filesystem::create_directory(path)) {
std::cout << "Directory created successfully!" << std::endl;
} else {
std::cout << "Directory already exists or cannot be created." << std::endl;
}
return 0;
}
In this example, we declare a string `path` with the value `"NewFolder"`. When executing `create_directory`, if the directory does not already exist, we successfully create it, and the program will output "Directory created successfully!". However, if the directory already exists or there are permission issues, the program will inform us accordingly.

Creating Nested Folders
Using `std::filesystem::create_directories`
While `create_directory` allows for creating a single directory, `std::filesystem::create_directories` is designed for creating nested directories in one go. This function will create all directories in the specified path if they do not already exist.
Example: Creating Nested Directories
Here's how you can create nested folders:
#include <iostream>
#include <filesystem>
int main() {
std::string nestedPath = "ParentFolder/ChildFolder";
// Create a nested directory structure
if (std::filesystem::create_directories(nestedPath)) {
std::cout << "Nested directory structure created successfully!" << std::endl;
} else {
std::cout << "Directories already exist or cannot be created." << std::endl;
}
return 0;
}
In this snippet, the variable `nestedPath` specifies a path that includes both a parent and child folder. By using `create_directories`, we ensure that both "ParentFolder" and "ChildFolder" will be created if they do not already exist. This is particularly useful for organizing files and managing project assets effectively.
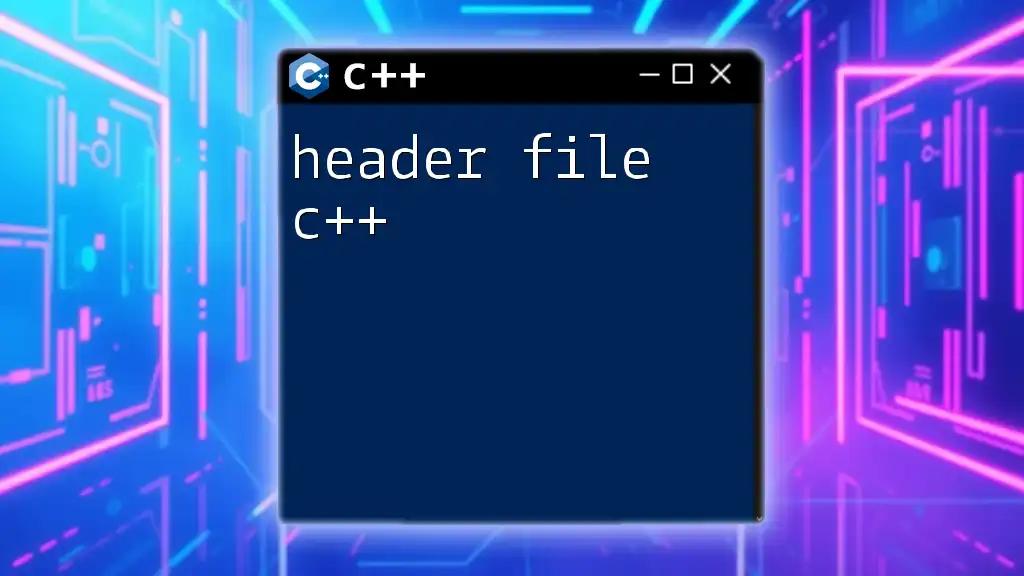
Error Handling while Creating Folders
Common Errors and Exceptions
Creating directories may not always go as planned. Understanding potential errors can enhance your application's robustness. The `std::filesystem::filesystem_error` exception is thrown when the filesystem encounters issues, such as invalid paths or permission problems.
Example: Handling Errors Gracefully
Consider the following enhanced example that integrates error handling:
#include <iostream>
#include <filesystem>
int main() {
std::string path = "AnotherFolder";
try {
std::filesystem::create_directory(path);
std::cout << "Directory created successfully!" << std::endl;
} catch (const std::filesystem::filesystem_error& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
In this case, if an error occurs during the creation of the directory, instead of crashing the program, it catches the `filesystem_error` and prints an error message indicating what went wrong. This allows for smoother user experiences and easier debugging.
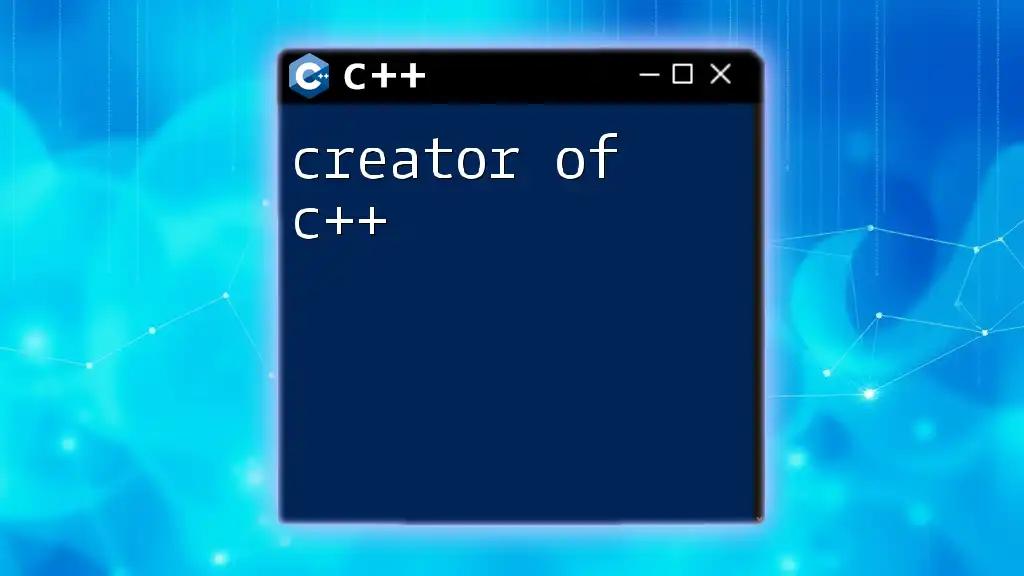
Practical Use Cases for Folder Creation in C++
Organizing Project Files
One of the most practical applications of folder creation in C++ is to organize project files. Creating separate folders for source code, documentation, resources, and binaries helps maintain cleaner project structures and facilitate easier collaboration among teams.
Temporary File Management
Another significant use case is temporary file management. During development, you might need to create temporary folders for storing logs, cache data, or temporary output files. Here's a code snippet demonstrating how to create a temporary folder:
#include <iostream>
#include <filesystem>
#include <string>
#include <chrono>
int main() {
auto timestamp = std::chrono::system_clock::now().time_since_epoch().count();
std::string tempPath = "TempFolder_" + std::to_string(timestamp);
// Create a temporary directory
if (std::filesystem::create_directory(tempPath)) {
std::cout << "Temporary directory created successfully!" << std::endl;
} else {
std::cout << "Error creating temporary directory!" << std::endl;
}
return 0;
}
In this snippet, we generate a unique temporary folder name based on the current timestamp. Such automation is critical for ensuring that temporary files do not collide when multiple operations are quickly executed.

Conclusion
Throughout this guide, you have learned how to create folder c++ using the powerful `<filesystem>` library. From understanding the library's functionality to exploring practical usage scenarios, you've gained insights into managing your directories effectively.
We hope you feel encouraged to experiment with the code and explore further functionalities of the C++ standard library. The versatility of C++ allows you to build robust applications informed by sound directory management practices.
Feel free to delve deeper into the world of C++ and share your experiences with us as you embark on your learning journey!