A web server in C++ can be created using simple socket programming to handle HTTP requests and serve responses to clients.
Here’s a basic example of a C++ web server that listens for incoming connections:
#include <iostream>
#include <string>
#include <netinet/in.h>
#include <unistd.h>
int main() {
int server_fd = socket(AF_INET, SOCK_STREAM, 0);
struct sockaddr_in address;
int opt = 1;
int addrlen = sizeof(address);
setsockopt(server_fd, SOL_SOCKET, SO_REUSEADDR, &opt, sizeof(opt));
address.sin_family = AF_INET;
address.sin_addr.s_addr = INADDR_ANY;
address.sin_port = htons(8080);
bind(server_fd, (struct sockaddr *)&address, sizeof(address));
listen(server_fd, 3);
while (true) {
int new_socket = accept(server_fd, (struct sockaddr *)&address, (socklen_t*)&addrlen);
const char *hello = "HTTP/1.1 200 OK\n\nHello, World!";
send(new_socket, hello, strlen(hello), 0);
close(new_socket);
}
return 0;
}
Introduction to C++ Web Servers
Understanding Web Servers
A web server is a software or hardware that stores, processes, and delivers web pages to clients. When you access a website, your browser sends a request to a server, which processes the request and returns the relevant data (HTML, CSS, JavaScript, images, etc.). This interaction forms the backbone of the World Wide Web.
The significance of web servers is immeasurable; they enable users to access information and services over the internet. In this digital age, having a solid understanding of how to create and manage your own web server can unlock numerous possibilities, from hosting personal projects to running complex applications.
Why Use C++ for a Web Server?
C++ is renowned for its performance and efficiency, making it an excellent choice for web server development. Unlike higher-level languages, C++ provides fine-grained control over system resources, enabling optimized performance. When high-throughput and low-latency are crucial—think streaming services or real-time applications—C++ becomes a preferred option.
Comparison with Other Languages:
- Python: Easier to build with but slower performance.
- Java: Good performance but can be more cumbersome and requires more setup.
- Node.js: Great for quick prototyping but can be less performant under heavy loads.
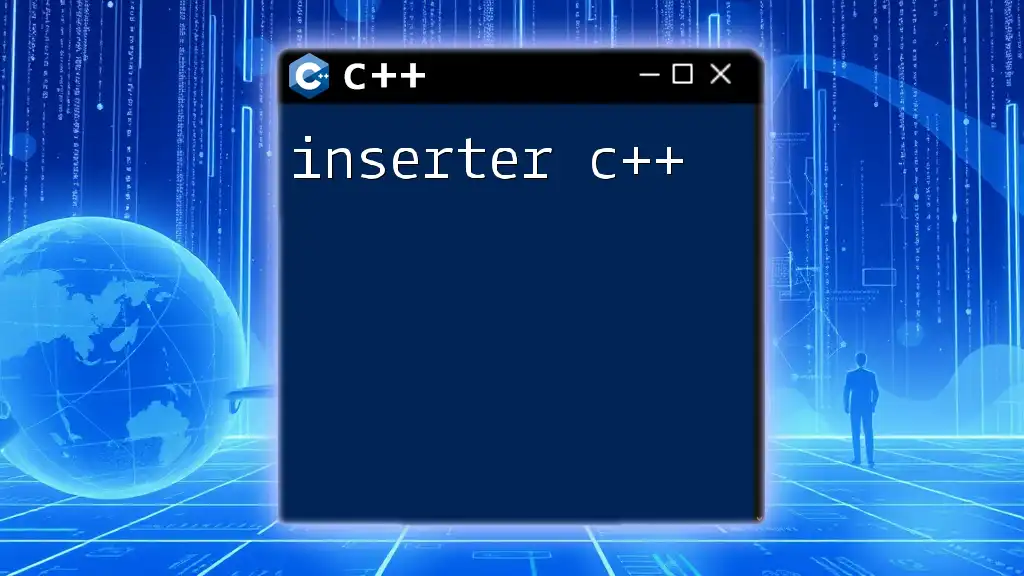
Setting Up Your C++ Environment
Required Tools and Libraries
Before diving into code, it's crucial to set up your environment effectively. Here are the essential components:
- Compiler: Install a modern C++ compiler such as GCC or Clang.
- IDE/Text Editor: Use IDEs like Visual Studio, Code::Blocks, or a lightweight editor like Visual Studio Code.
- Libraries: Integrate libraries that simplify HTTP server functionalities. Popular choices include:
- Boost.Asio: Excellent for asynchronous network programming.
- POCO: Comprehensive libraries for network programming.
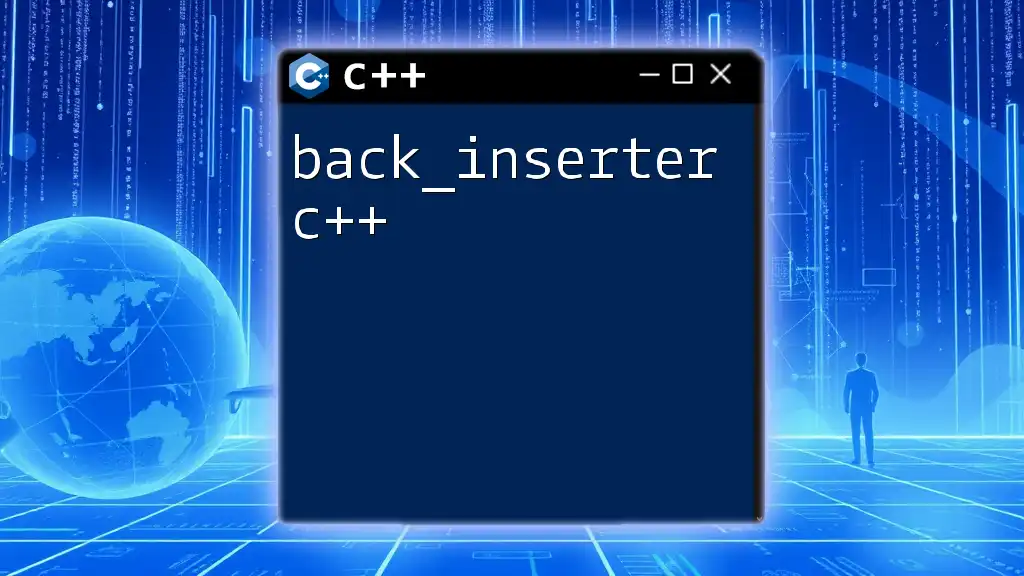
Building a Simple HTTP C++ Server
Creating the Basic Structure
A successful HTTP server relies on a clear structured architecture that handles the incoming requests efficiently. This involves initializing network sockets, setting them to listen for incoming connections, and processing these connections.
Code Snippet: Basic C++ HTTP Server
Below is an example of a simple HTTP server written in C++. It listens on port 8080 and responds with a basic HTML page.
#include <iostream>
#include <boost/asio.hpp>
using boost::asio::ip::tcp;
int main() {
try {
boost::asio::io_context io_context;
tcp::acceptor acceptor(io_context, tcp::endpoint(tcp::v4(), 8080));
while (true) {
tcp::socket socket(io_context);
acceptor.accept(socket);
std::string response = "HTTP/1.1 200 OK\r\nContent-Type: text/html\r\n\r\n<html><h1>Hello, World!</h1></html>";
boost::asio::write(socket, boost::asio::buffer(response));
}
} catch (std::exception& e) {
std::cerr << "Exception: " << e.what() << "\n";
}
}
In this code:
- We initialize the `io_context` and `acceptor` to manage incoming connections.
- A loop continuously waits for requests, and upon acceptance, sends a simple HTML response back to the client.
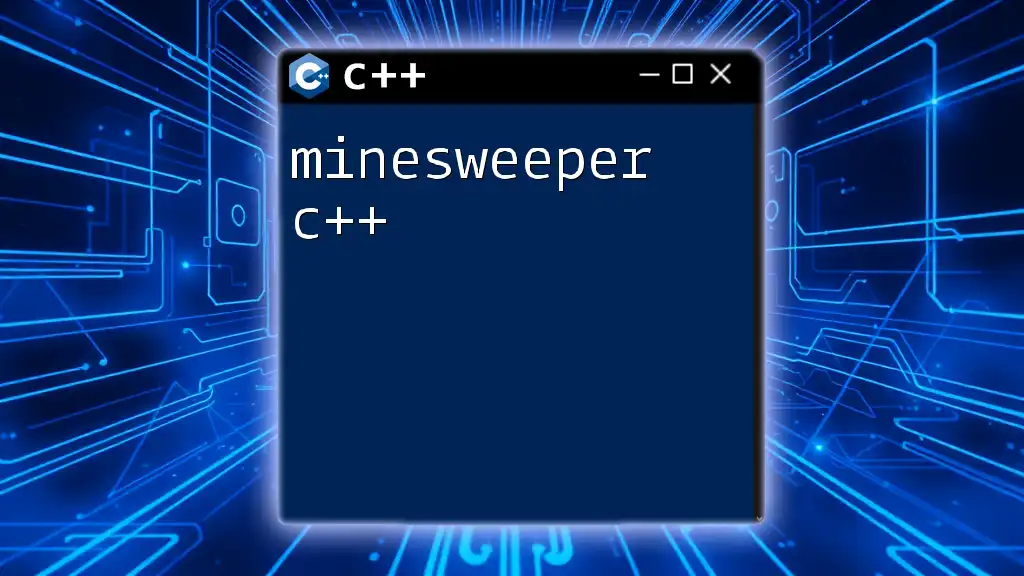
Understanding HTTP Protocol
What is HTTP?
HTTP (Hypertext Transfer Protocol) is the foundation of data communication on the web. It’s a stateless protocol used for transmitting hypermedia documents. The protocol has evolved significantly, with major versions including HTTP/1.1, HTTP/2, and the emerging HTTP/3.
Common HTTP methods include:
- GET: Retrieve data from the server.
- POST: Send data to the server.
- PUT: Update existing data.
- DELETE: Remove data.
Handling HTTP Requests in C++
Handling requests involves parsing incoming HTTP requests and structuring response messages. This can initially be simple, but as the server grows, it may require a more sophisticated approach to parsing.
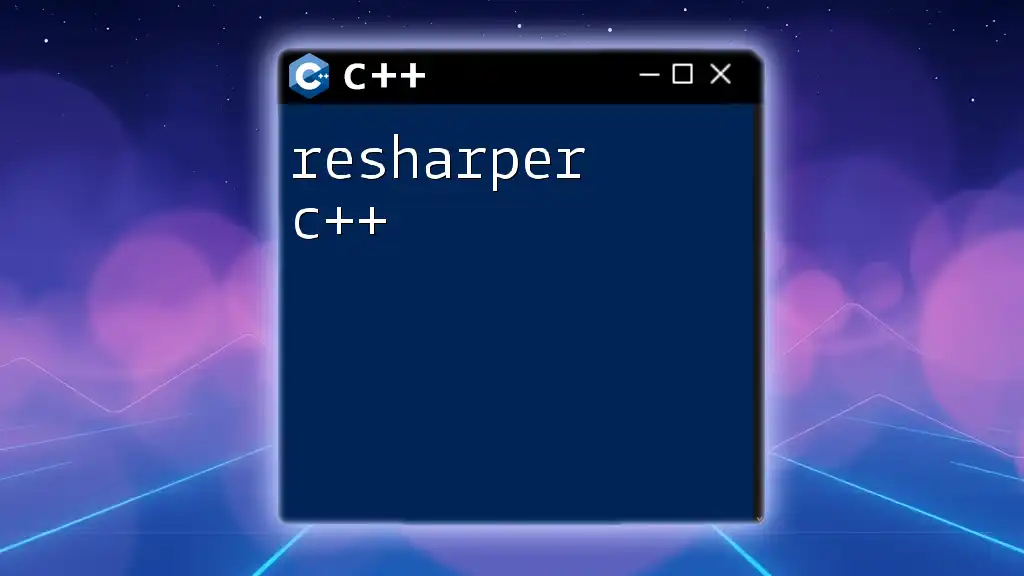
Enhancing Your C++ HTTP Server
Adding Multi-threading Support
Concurrency is critical for any web server that expects multiple requests simultaneously. C++11 introduced threading capabilities which can be leveraged to run each connection in separate threads.
Example code snippet demonstrating multi-threading:
#include <thread>
// existing includes...
void handle_request(tcp::socket socket) {
// Process request
// Similar code as shown earlier
}
int main() {
// Set up acceptor and context
while (true) {
tcp::socket socket(io_context);
acceptor.accept(socket);
std::thread(handle_request, std::move(socket)).detach();
}
}
This approach allows your server to handle multiple requests concurrently, ensuring responsiveness no matter the load.
Serving Static Files
Serving static files (like HTML, CSS, and JS) adds a layer of complexity to your server. To serve files, you must:
- Parse the requested URL.
- Locate the file on your server.
- Return it as an HTTP response.
Code Example: Setting Up Routes for File Serving
Here’s a simple way to serve static files:
#include <fstream>
// existing includes...
std::string serve_file(const std::string& path) {
std::ifstream file(path);
if (!file) return "404 Not Found";
std::string content((std::istreambuf_iterator<char>(file)), std::istreambuf_iterator<char>());
return "HTTP/1.1 200 OK\r\nContent-Type: text/html\r\n\r\n" + content;
}
In this code:
- `serve_file()` reads a file and prepares the HTTP response.
- Always ensure to handle scenarios where the file might not exist.
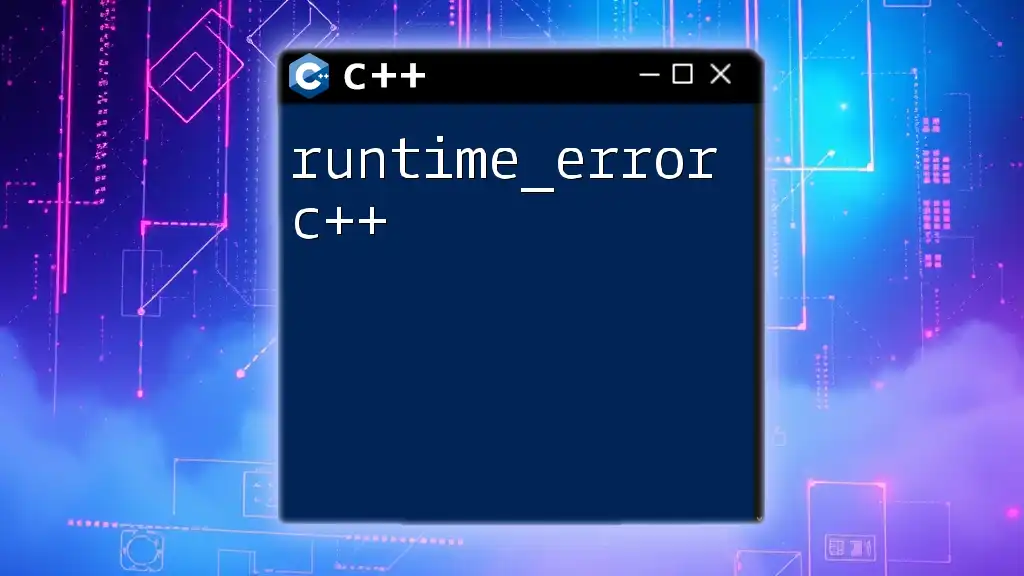
Advanced Features of an HTTP C++ Server
Implementing a RESTful API
Creating a RESTful API involves defining endpoints that clients can interact with. You would typically define your routes based on the CRUD operations.
Code Example: Creating REST Endpoints
Below is a simplified approach to set up a RESTful endpoint:
if (request_method == "GET" && request_path == "/api/data") {
std::string response = "{\"key\":\"value\"}";
socket.send(response);
}
This checks for a specific request method and path, returning JSON data when a client requests the `/api/data` endpoint.
Using Middleware in C++ Server
Middleware refers to software that acts as an intermediary between requests and responses. This can be useful for logging, authentication, etc.
Example of a logging middleware implementation might look like this:
void log_request(const Request& req) {
std::cout << "Request received: " << req.path() << std::endl;
}
Incorporating this into your main request handling would help track day-to-day operations and facilitate debugging.
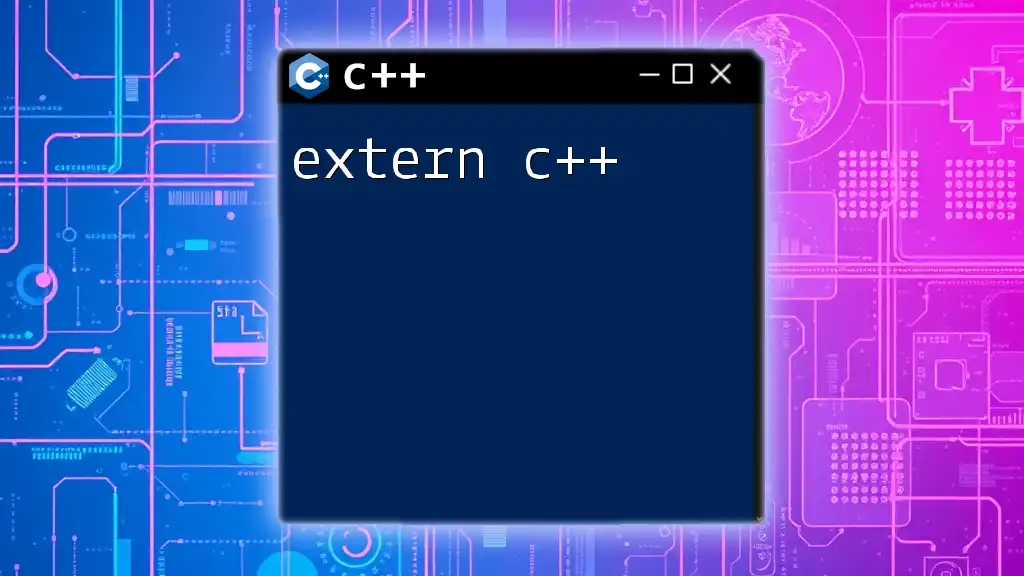
Security Considerations
Understanding Basic Security Practices
Given today’s cybersecurity landscape, it is essential to understand potential threats like Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF). Having basic filtering and validation mechanisms in place can drastically reduce vulnerabilities.
Implementing HTTPS in Your C++ Web Server
To secure communications, enabling HTTPS using SSL/TLS is vital. Transitioning your server from HTTP to HTTPS includes acquiring an SSL certificate and configuring your server to support secure connections.
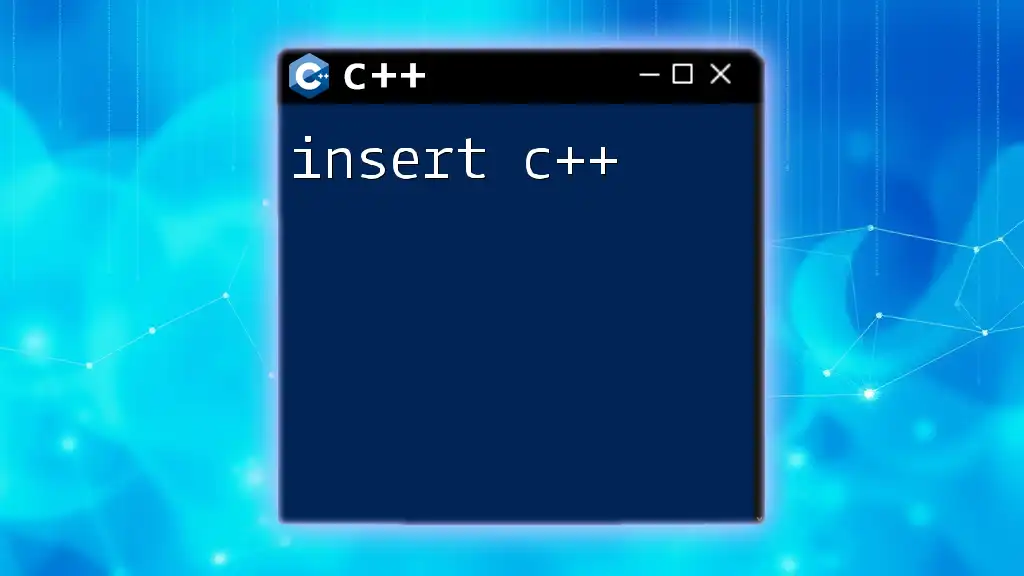
Performance Optimization
Strategies for Enhancing Server Performance
Approaches to enhance your server’s performance can include connection pooling, where connections are reused, and caching static content to minimize redundant file accesses.
Code Examples Demonstrating Optimization Techniques
Caching could be as simple as storing responses in memory after the first request. Use `std::map` or a similar data structure to keep track of served requests.
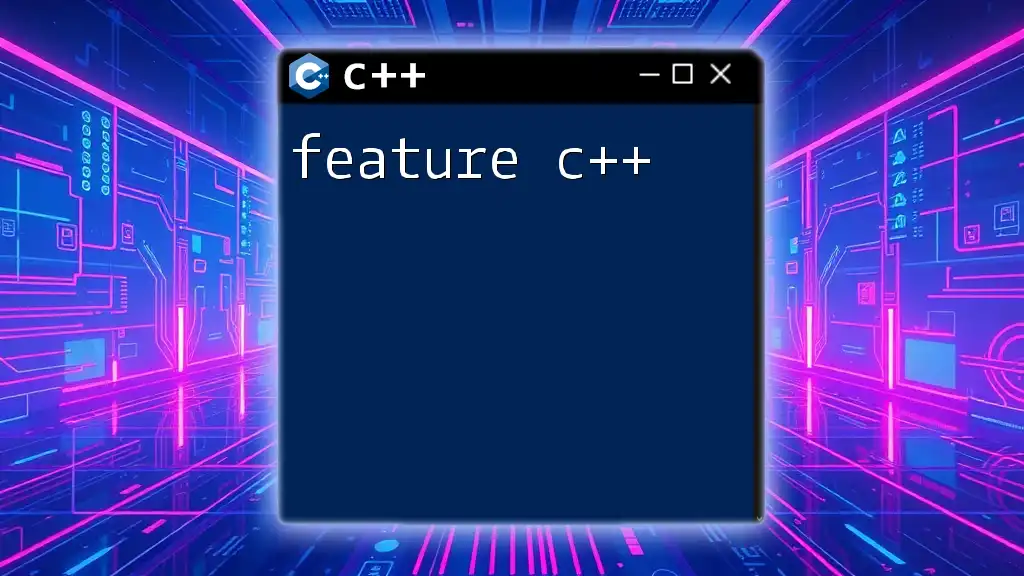
Monitoring and Debugging Tools
Strongly consider incorporating tools for monitoring and error tracking. Software like Prometheus for metrics collection or gdb for debugging your C++ code can be invaluable as your server scales.
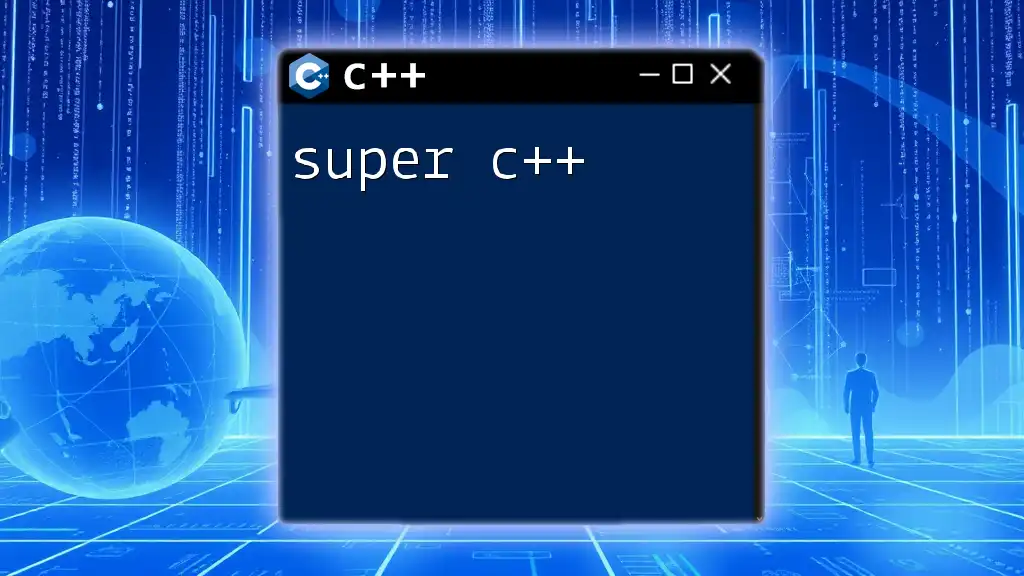
Conclusion
Understanding how to build a web server using C++ opens many doors to potential projects, ranging from simple personal web pages to robust, scalable online applications. Using the methods discussed, anyone can design a high-performance HTTP server tailored to meet specific needs.
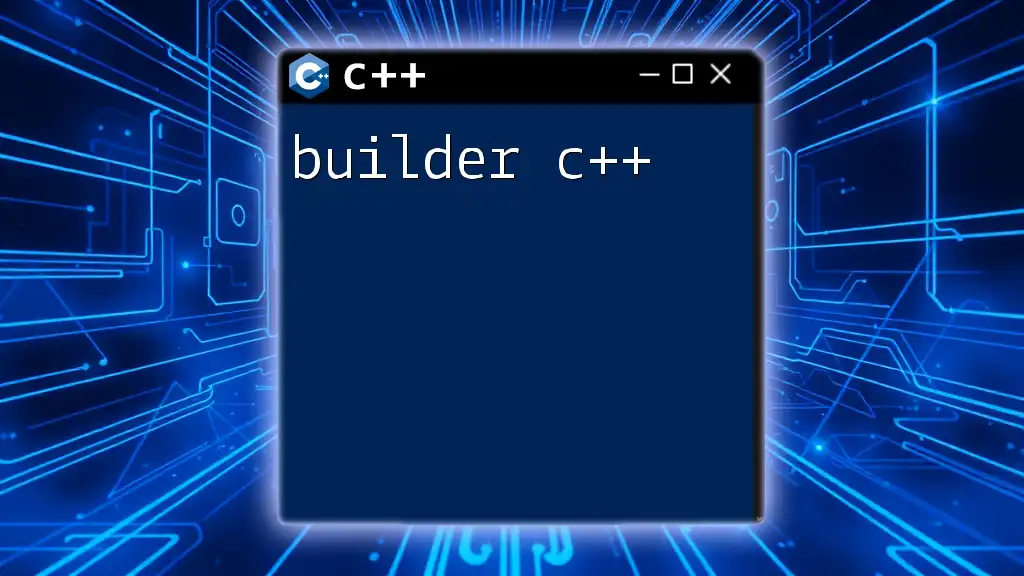
Additional Resources
Choosing additional readings or joining forums that focus on C++ web development can further solidify your understanding and keep you updated with the latest trends. Engage with communities on GitHub, Stack Overflow, or Reddit for collaborative learning.
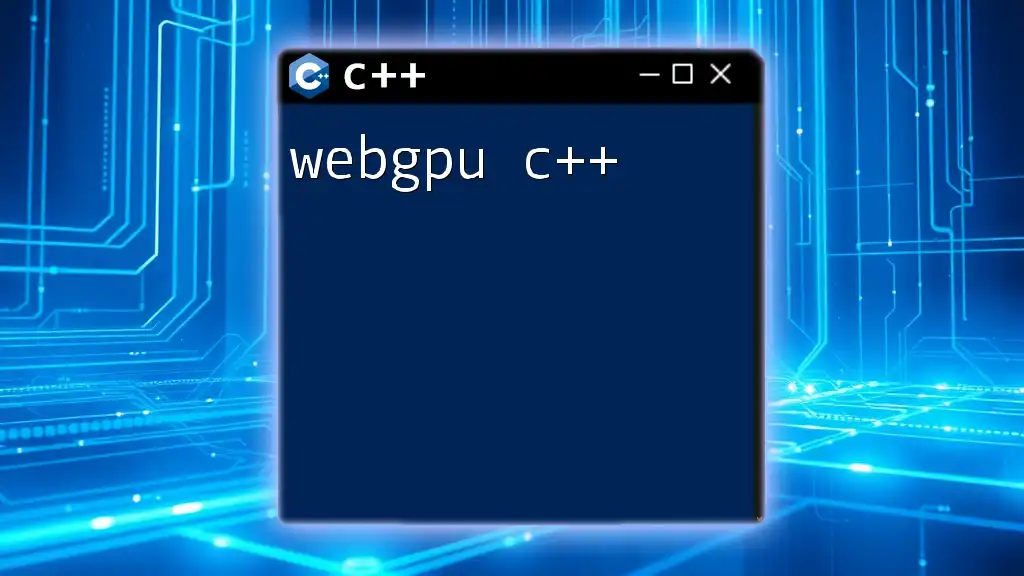
Call to Action
Now that you have the foundational knowledge, it’s time to start building! Begin creating your C++ web server or experiment with the examples presented. Don’t forget to subscribe to newsletters or your favorite blogs to stay informed on future developments in C++.