A builder in C++ is a design pattern that facilitates the construction of complex objects step-by-step, allowing for greater flexibility and readability in object creation.
Here’s a simple code snippet demonstrating a builder pattern in C++:
#include <string>
#include <iostream>
// Product class
class Pizza {
public:
std::string dough;
std::string sauce;
std::string topping;
void display() {
std::cout << "Pizza with " << dough << ", " << sauce << ", and " << topping << std::endl;
}
};
// Builder class
class PizzaBuilder {
public:
Pizza pizza;
PizzaBuilder& setDough(const std::string& dough) {
pizza.dough = dough;
return *this;
}
PizzaBuilder& setSauce(const std::string& sauce) {
pizza.sauce = sauce;
return *this;
}
PizzaBuilder& setTopping(const std::string& topping) {
pizza.topping = topping;
return *this;
}
Pizza build() {
return pizza;
}
};
// Example usage
int main() {
PizzaBuilder builder;
Pizza pizza = builder.setDough("thin crust")
.setSauce("tomato")
.setTopping("cheese")
.build();
pizza.display();
return 0;
}
Understanding Design Patterns
What Are Design Patterns?
Design patterns are standardized solutions to common programming challenges. They offer a template for solving particular problems in various contexts while adhering to object-oriented design principles. Understanding these patterns allows developers to create more efficient, reusable, and maintainable code.
Types of Design Patterns
Design patterns can be categorized into three main types:
- Creational patterns: These patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. Examples include the Builder, Factory Method, and Singleton patterns.
- Structural patterns: These focus on how objects and classes can be composed to form larger structures. Examples include Adapter, Composite, and Proxy patterns.
- Behavioral patterns: These patterns are concerned with communication between objects, defining how objects interact. Examples include Strategy, Observer, and Command patterns.
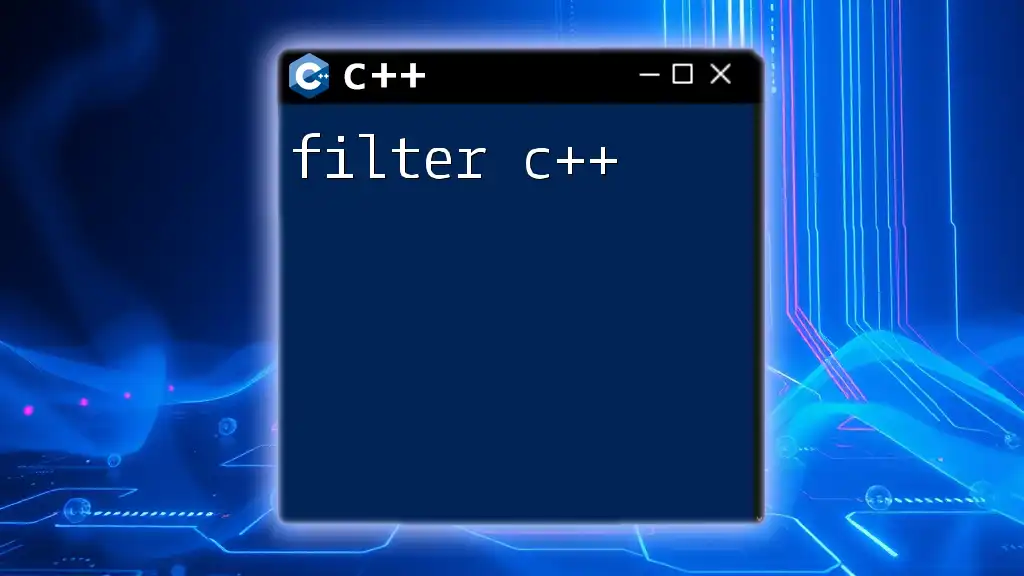
What is the Builder Pattern?
Definition of the Builder Pattern
The Builder pattern is a creational design pattern that allows for the seamless construction of complex objects. Instead of having a single constructor with numerous parameters, the Builder pattern enables the step-by-step creation of an object using method calls. This approach leads to cleaner code, as the construction process is segregated from the representation.
When to Use the Builder Pattern
The Builder pattern is most advantageous in scenarios where:
- An object requires several components that can vary in configuration.
- The construction process is complex and requires several steps, potentially involving multiple methods.
- There’s a need for a more readable and maintainable approach to creating objects.
It's helpful to compare the Builder pattern with other creational patterns, such as the Factory Method, which is better suited for returning previously defined types without complex construction logic.
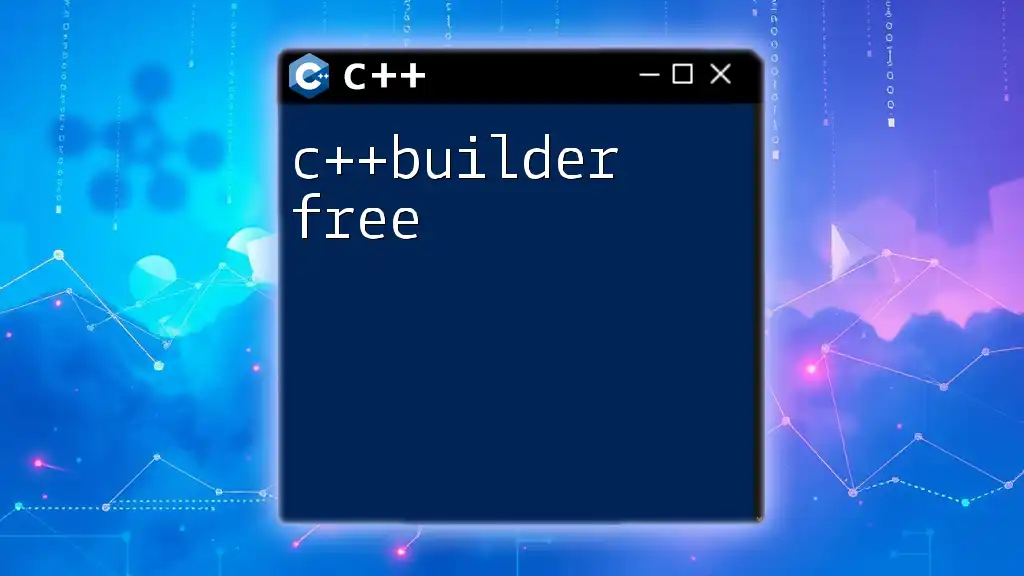
Components of the Builder Pattern
Components Overview
The Builder pattern is composed of three essential components:
- Builder
- Product
- Director
Each of these plays a vital role in the construction process.
The Builder
The Builder defines an interface for creating the parts of the Product object. It ensures that a stepwise construction sequence can be implemented.
Here’s an example code snippet illustrating the Builder interface:
class Builder {
public:
virtual ~Builder() {}
virtual void buildPartA() = 0;
virtual void buildPartB() = 0;
virtual Product* getResult() = 0;
};
The Product
The Product is the complex object that is created by the Builder. It can have various parts assembled together during construction.
Here’s what the Product class implementation might look like:
class Product {
private:
std::string partA;
std::string partB;
public:
void setPartA(const std::string &part) { partA = part; }
void setPartB(const std::string &part) { partB = part; }
};
The Director
The Director is responsible for controlling the building process. It uses the Builder abstraction to construct the final product without being concerned about the details of its assembly.
An example implementation of the Director may look like this:
class Director {
public:
void construct(Builder &builder) {
builder.buildPartA();
builder.buildPartB();
}
};
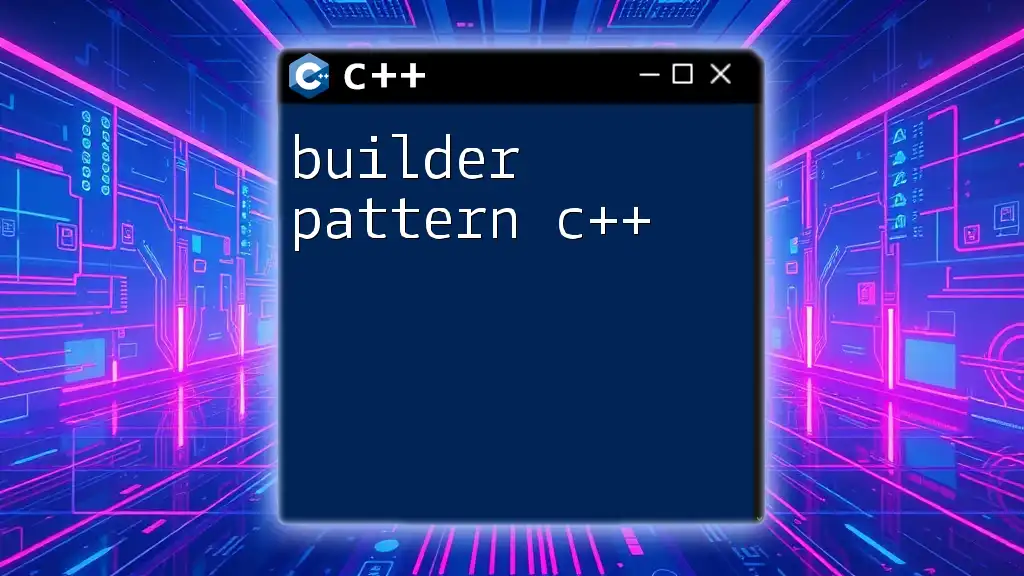
Implementing the Builder Pattern in C++
Step-by-Step Implementation Guide
To implement the Builder pattern, you need to establish the structure consisting of the Product class, Builder interface, Concrete Builders, and the Director.
Creating the Product Class
You start by defining the components of the Product:
class Product {
private:
std::string partA;
std::string partB;
public:
void setPartA(const std::string &part) { partA = part; }
void setPartB(const std::string &part) { partB = part; }
};
Creating the Builder Interface
Next, the builder interface needs to be defined, stating the responsibilities of the Builder:
class Builder {
public:
virtual ~Builder() {}
virtual void buildPartA() = 0;
virtual void buildPartB() = 0;
virtual Product* getResult() = 0;
};
Creating Concrete Builders
Once the interface is ready, you can implement a Concrete Builder that creates the Product via defined methods:
class ConcreteBuilder : public Builder {
private:
Product* product;
public:
ConcreteBuilder() { product = new Product(); }
void buildPartA() override { product->setPartA("Part A"); }
void buildPartB() override { product->setPartB("Part B"); }
Product* getResult() override { return product; }
};
Creating the Director
Finally, the Director uses the Builder to manage the construction of the Product:
class Director {
public:
void construct(Builder &builder) {
builder.buildPartA();
builder.buildPartB();
}
};
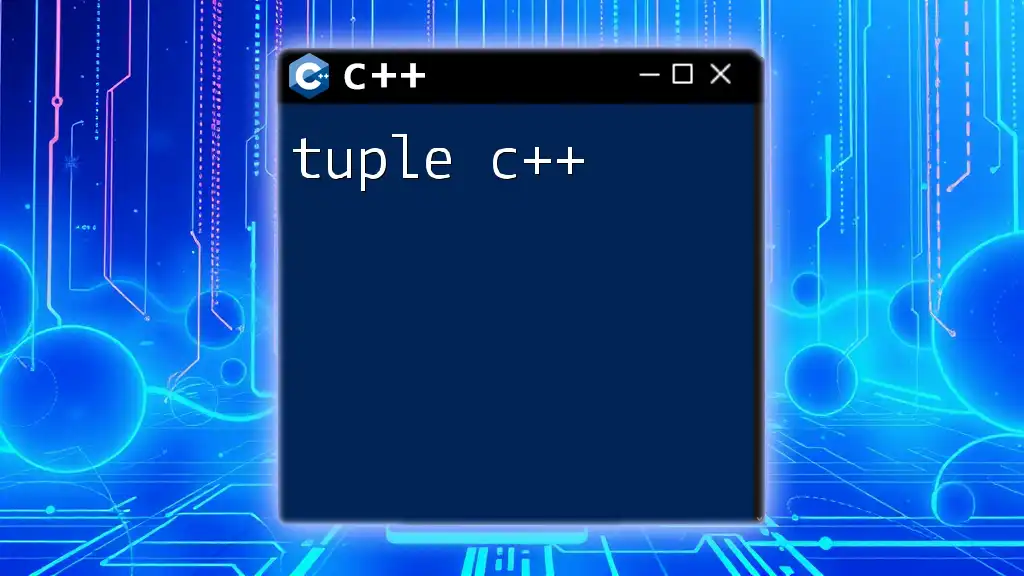
Using the Builder Pattern
Creating and Utilizing Builders
With all components in place, you can now utilize the Builder pattern effectively. Here's an example of how to use the Builder in a main context:
int main() {
ConcreteBuilder builder;
Director director;
director.construct(builder);
Product* product = builder.getResult();
// Use the product...
delete product;
return 0;
}
Real-world Application Examples
The Builder pattern finds utility in various domains. For instance, in software engineering, you might use it to construct complex UI components, where the structure can change based on user input. In IoT devices, the Builder can aid in creating configurations for devices that require various parameters. In game development, it can manage the construction of complex entities with numerous attributes, enhancing both flexibility and maintainability.
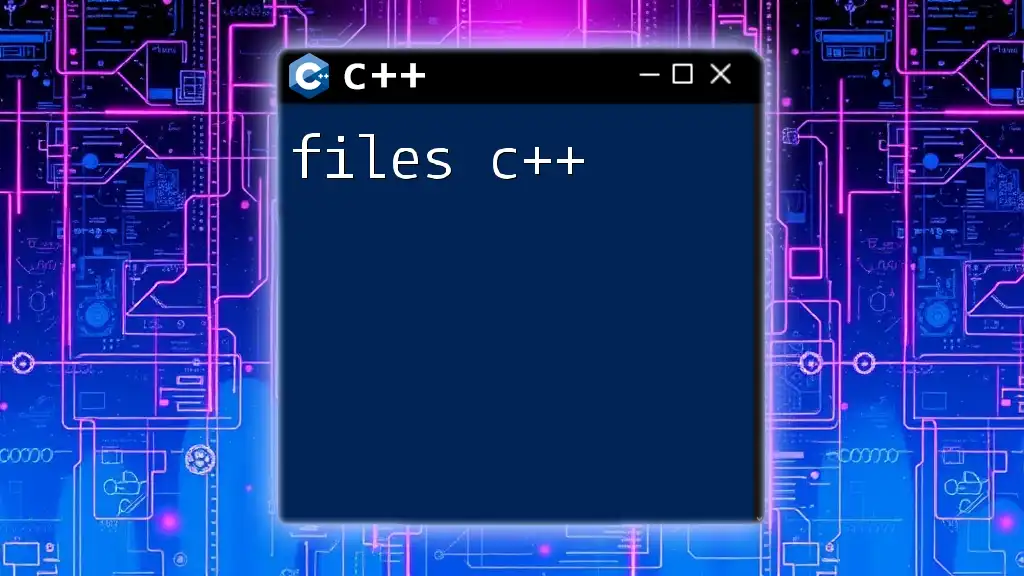
Advantages and Disadvantages of the Builder Pattern
Pros of the Builder Pattern
The Builder pattern presents several advantages:
- It improves readability and maintainability of the code.
- It segregates the construction logic from the object's representation, which is substantial for complex objects.
Cons of the Builder Pattern
Conversely, it also has a few disadvantages:
- The complexity can increase since you must define multiple classes.
- There may be a slight performance overhead due to the additional abstraction layer.
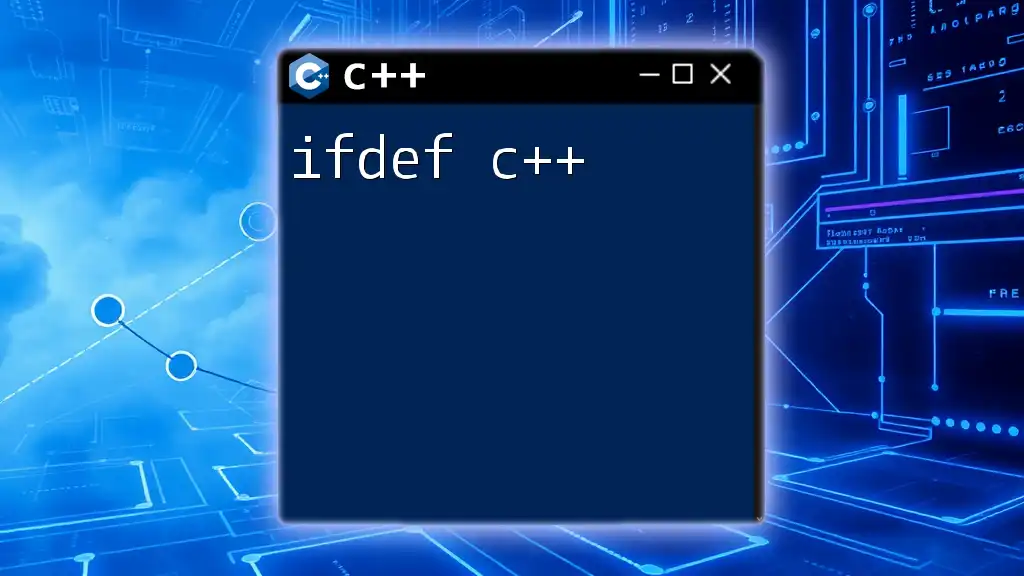
Conclusion
The Builder pattern in C++ is a powerful tool for creating complex objects with clarity and precision. It emphasizes clean, understandable code while managing the intricacies of object assembly. By following the implementation steps outlined in this guide, developers can leverage this pattern effectively in their projects.
Practicing the implementation of the Builder pattern will bolster your ability to construct flexible and maintainable code. I encourage you to experiment with your components using the Builder pattern and explore its potential in various application scenarios.
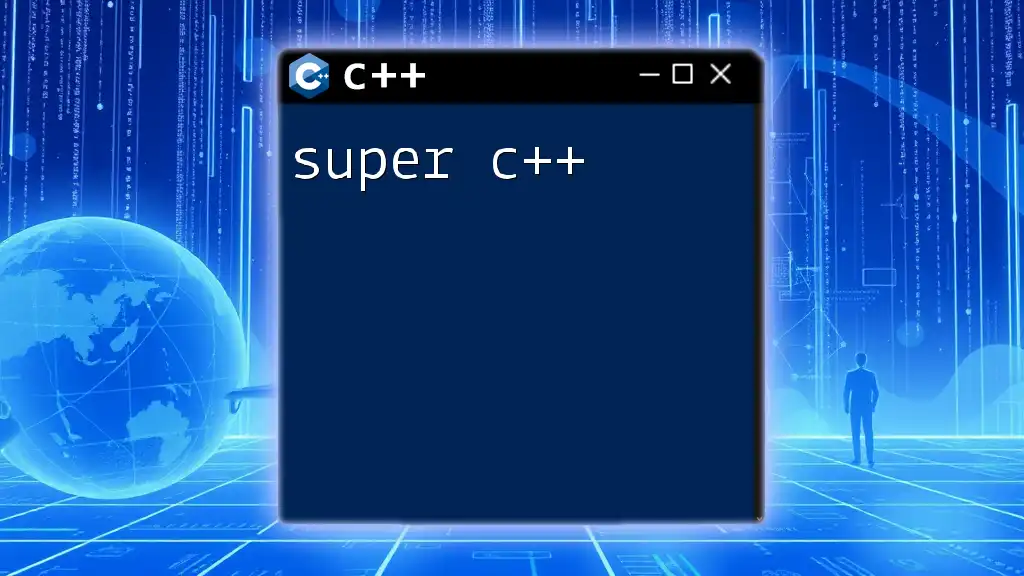
Additional Resources
To expand your knowledge of C++ design patterns further, consider the following resources:
- Books dedicated to C++ Design Patterns.
- Online tutorials or courses focused on advanced C++ concepts.
- Developer communities or forums where you can exchange knowledge and experiences related to C++.