In C++, the `std::copy_if` algorithm from the Standard Template Library (STL) can be used to filter elements from a source container based on a specified condition and copy them to a destination container.
Here's a code snippet demonstrating how to filter elements:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5, 6};
std::vector<int> evenNumbers;
std::copy_if(numbers.begin(), numbers.end(), std::back_inserter(evenNumbers), [](int n) { return n % 2 == 0; });
for (int n : evenNumbers) {
std::cout << n << " ";
}
return 0;
}
Understanding Filters in C++
Filters are an essential aspect of programming, particularly when manipulating data sets. In the context of C++, filtering refers to the selective operation of sorting through data collections, such as arrays or vectors, to retrieve elements that meet specific criteria. Effective filtering enhances code efficiency and makes data handling significantly easier, especially in large applications where data management is crucial.
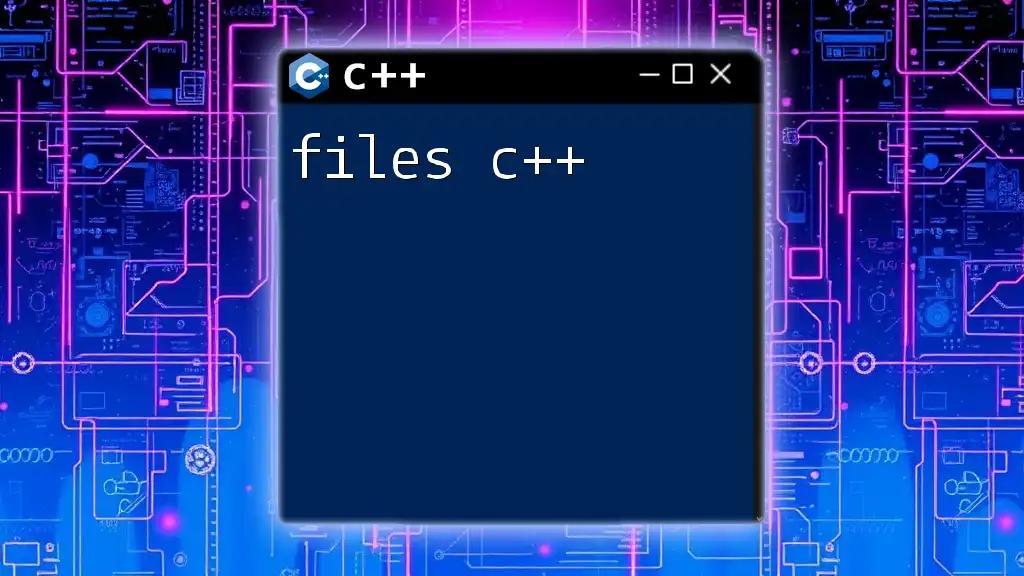
The C++ Standard Library
The C++ Standard Library offers powerful tools that streamline the filtering process. Key components of this library include algorithms and iterators, which can be accessed by including `<algorithm>` and `<iterator>` headers in your code. These standard algorithms provide efficient ways to manipulate and filter collections of data without the need for writing complex loops.
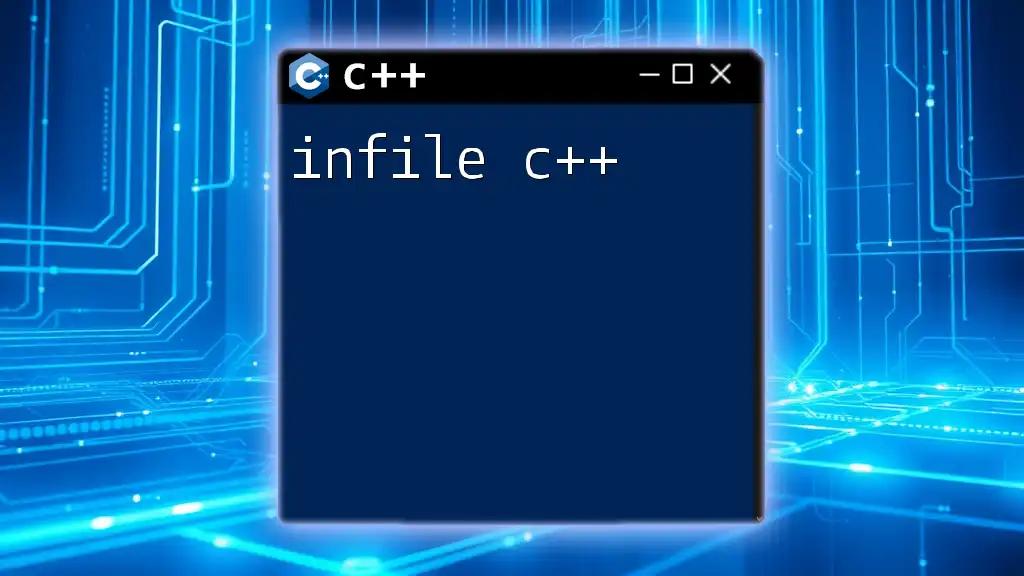
The Concept of Filtering
What is Filtering?
In programming, filtering is the process of excluding unwanted data based on defined criteria. Essentially, it allows developers to focus solely on relevant information, which is key for tasks such as data analysis, reporting, and database management.
Use Cases of Filtering
Filtering is commonly applied in myriad scenarios:
- Database Queries: Extracting specific records from a large database.
- User Input Validation: Ensuring that entered data meets particular rules.
- Data Visualization: Displaying only relevant data points on graphs or charts.

Filtering Vectors in C++
Introduction to Vectors
`std::vector` is one of the most widely used data structures in C++. It provides a dynamic array that can automatically resize, making it a flexible choice for storing collections of data. Vectors are an excellent starting point for filtering operations since they can efficiently manage sequences of elements.
How to Filter a Vector
Filtering a vector involves applying specific conditions to extract elements that satisfy the given criteria. One of the most effective ways to filter vectors in C++ is by using the `std::remove_if` algorithm followed by `std::erase`. This combination is both elegant and efficient.
Using `std::remove_if` and `std::erase`
The `std::remove_if` algorithm reorders elements based on a given predicate, effectively moving the elements that do not match the criteria to the end of the container. The `std::erase` method then removes these elements from the vector.
Here is an example:
#include <vector>
#include <algorithm>
std::vector<int> vec = {1, 2, 3, 4, 5, 6};
vec.erase(std::remove_if(vec.begin(), vec.end(), [](int i) { return i % 2 == 0; }), vec.end());
// vec now contains {1, 3, 5}
Explaining the Code Snippet
In the code above, we initialize a vector with integers from 1 to 6. The `std::remove_if` function is used with a lambda function to filter out even numbers. The lambda expression `[](int i) { return i % 2 == 0; }` returns `true` for even numbers, which are then removed from the original vector using `vec.erase()`. The result shows that only the odd numbers are retained.
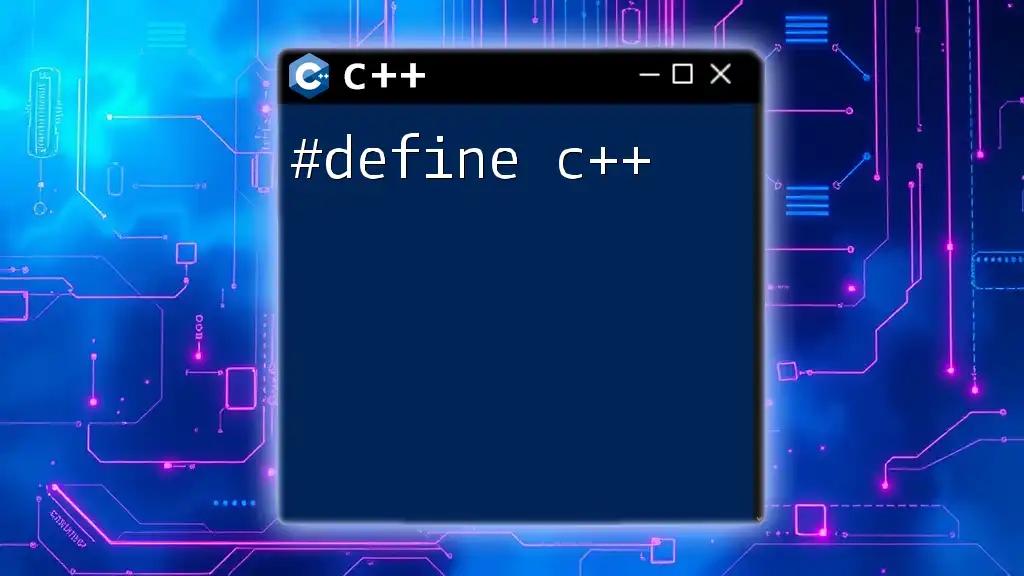
Custom Filtering Function
While built-in algorithms are powerful, creating custom filtering functions allows for greater flexibility tailored to specific needs.
Example of Custom Filter Function
Here’s how to define a custom function for filtering:
#include <vector>
#include <algorithm>
bool isOdd(int number) {
return number % 2 != 0;
}
std::vector<int> filterVector(const std::vector<int>& inputVec) {
std::vector<int> outputVec;
std::copy_if(inputVec.begin(), inputVec.end(), std::back_inserter(outputVec), isOdd);
return outputVec;
}
Explanation of the Example
In this example, we define a function `isOdd` that checks whether a number is odd. The `filterVector` function utilizes `std::copy_if` to copy only the elements satisfying the condition defined by `isOdd`. Using `std::back_inserter` ensures that the new vector is populated without any manual handling of indices, simplifying the coding process.
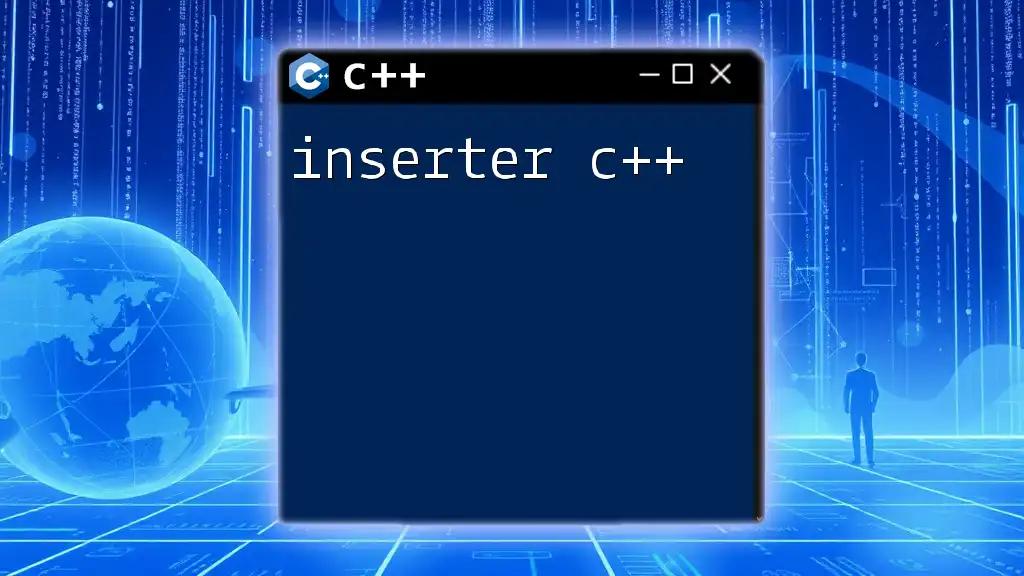
Advanced Filtering Techniques
Using Filters with More Complex Data Structures
Beyond simple types, filtering can also be applied to more complex data structures, such as classes and structures. This technique is invaluable in real-world applications where data is often represented as objects.
Example: Filtering a Vector of Objects
Let’s look at filtering a vector of products based on specific criteria:
struct Product {
std::string name;
float price;
};
std::vector<Product> filterExpensiveProducts(const std::vector<Product>& products, float threshold) {
std::vector<Product> result;
std::copy_if(products.begin(), products.end(), std::back_inserter(result), [threshold](const Product& p) {
return p.price > threshold;
});
return result;
}
Analysis of the Filtering Process
In the above example, we define a `Product` structure with a `name` and a `price`. The `filterExpensiveProducts` function filters out products that cost more than the provided threshold. The lambda function `[](const Product& p) { return p.price > threshold; }` allows for flexible definitions of the filtering criteria, making it easy to adapt to different scenarios.

Best Practices for Filtering in C++
Performance Considerations
When filtering, it is essential to consider performance implications. The combination of `std::remove_if` and `std::erase` is efficient but can become costly with large vectors if not used correctly. Always analyze the time complexity of your filtering operations to avoid potential bottlenecks, particularly in applications dealing with extensive data sets.
Readability and Maintainability
Another crucial aspect of filtering is ensuring that your code remains readable and maintainable. Clear, well-named filter functions improve code comprehension, making it easier for others (or yourself in the future) to understand and modify code.

Conclusion
C++ offers robust capabilities for filtering data through its standard library and custom functions. Through the use of powerful algorithms like `std::remove_if`, `std::copy_if`, and custom predicates, developers can effectively manage and manipulate data in efficient ways. Mastering the art of filtering not only enhances code performance but also leads to cleaner, more maintainable code—an invaluable skill in any developer's toolkit.

Additional Resources
For further exploration and learning:
- Check out the [C++ Standard Library documentation](https://en.cppreference.com/w/cpp) for deeper insights into available algorithms and utilities.
- Consider online courses and tutorials focused on C++ data structures and algorithms for practical applications.