Profiling C++ code involves measuring the performance and resource usage of your program to identify bottlenecks and optimize its efficiency. Here's an example of a simple C++ profiling code snippet using `chrono` to measure the execution time of a function:
#include <iostream>
#include <chrono>
void exampleFunction() {
// Simulated workload
for (volatile int i = 0; i < 10000000; ++i);
}
int main() {
auto start = std::chrono::high_resolution_clock::now();
exampleFunction();
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> duration = end - start;
std::cout << "The function took " << duration.count() << " seconds.\n";
return 0;
}
Understanding Performance Bottlenecks
What are Performance Bottlenecks?
Performance bottlenecks refer to points in your code where the execution slows down significantly, preventing the application from achieving its optimal performance. These bottlenecks can arise from various factors such as inefficient algorithms, excessive memory usage, or I/O operations that take longer to complete. Understanding these issues is crucial because they can lead to a poor user experience, particularly in applications that require high performance, such as gaming, financial software, or real-time systems.
Common examples of bottlenecks in C++ code include:
- Inefficient Algorithms: Using algorithms with high time complexity, such as O(n²) instead of O(n log n) for sorting, can significantly increase execution time as data size grows.
- Memory Leaks: Not freeing up memory can lead to exhaustion of available resources, causing the program to slow down or crash.
- Frequent I/O operations: Repeatedly reading from or writing to disk instead of caching data can introduce delays.
Identifying Bottlenecks Before Profiling
Before diving into profiling, it’s beneficial to perform preliminary analyses to identify potential performance issues. Various techniques help you spot these problems efficiently:
- Code Review: Examine your codebase for inefficient loops, recursive calls, and algorithmic complexities.
- Logging and Timing: Use logging messages that track execution time for specific blocks of code. This can provide insight into which parts of your application are consuming the most time.
auto start = std::chrono::high_resolution_clock::now(); // Block of code to time auto end = std::chrono::high_resolution_clock::now(); std::chrono::duration<double> elapsed = end - start; std::cout << "Elapsed time: " << elapsed.count() << " seconds" << std::endl;
- Basic Profiling with Print Statements: Insert print statements to monitor resource usage at various points in your code.
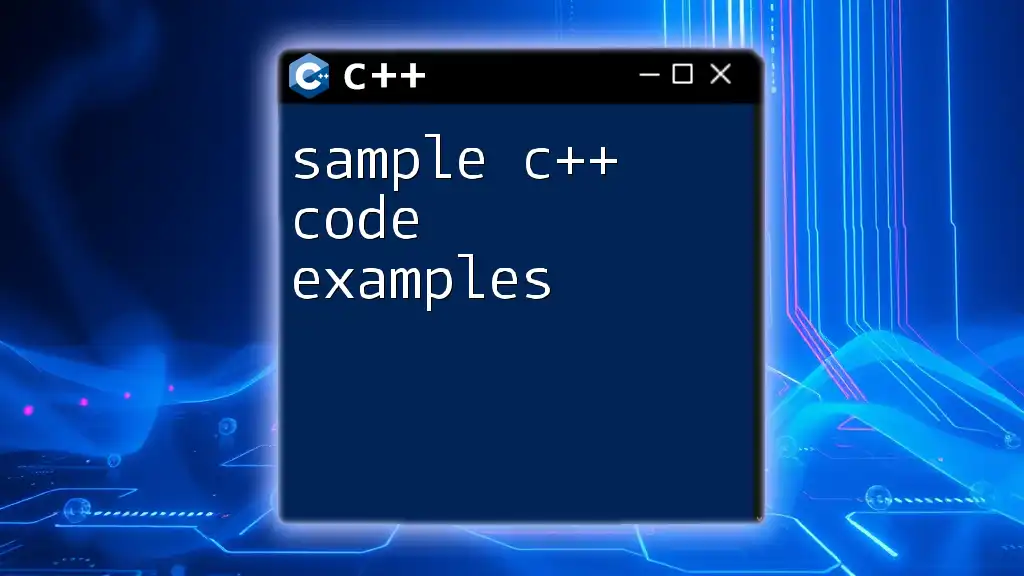
C++ Profiling Tools
Overview of Popular C++ Profiling Tools
Several tools are available for profiling C++ code, each offering unique features that suit different environments and use cases:
- gprof: A powerful GNU profiler that helps visualize function call graphs and execution times. It's suitable for small to medium-sized applications but may struggle with multi-threaded programs.
- Valgrind: Primarily a memory debugging tool, Valgrind can profile code by monitoring actual resource usage, making it effective for identifying memory leaks as well as performance bottlenecks.
- Perf: A command-line tool that provides a wealth of information on CPU performance and can assist in profiling specific sections of your C++ code on Linux.
- Visual Studio Profiler: Integrated into Visual Studio, it provides rich performance insights, including CPU and memory utilization, and is ideal for Windows applications.
Choosing the Right Tool for Your Needs
Choosing the right profiling tool requires considering various factors:
- Platform: Ensure compatibility with your operating system (Windows, Linux, etc.).
- Complexity of the Application: For small projects, simpler tools like gprof could suffice, while larger applications may necessitate more comprehensive tools like Valgrind or the Visual Studio Profiler.
- Specific Needs: If you're primarily concerned about memory management, Valgrind would be the best choice. For CPU utilization, tools like Perf or gprof are more appropriate.
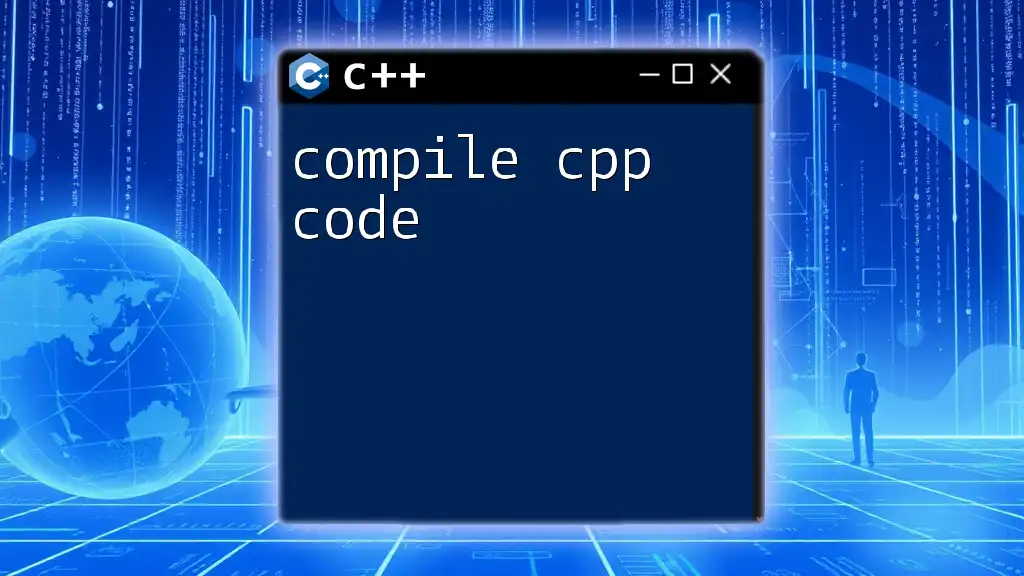
Setting Up Your C++ Profiler
Installation and Configuration
Setting up your profiling tool usually involves installing the software and preparing your environment. Each profiler has its guide, but the basic steps often include:
- Download the tool: Make sure to use the official sites or trusted repositories.
- Follow installation instructions: Be mindful of dependencies, especially when installing on Linux systems.
Sample Setup for gprof
To set up and run gprof, follow these steps:
-
Compile your C++ code with the `-pg` flag:
g++ -pg your_code.cpp -o your_program
-
Execute your program to generate the profiling data:
./your_program
-
Analyze the generated output file:
gprof ./your_program gmon.out > profile.txt
In this example, gprof produces output that summarizes the execution time of functions, helping pinpoint which functions consume the most resources.
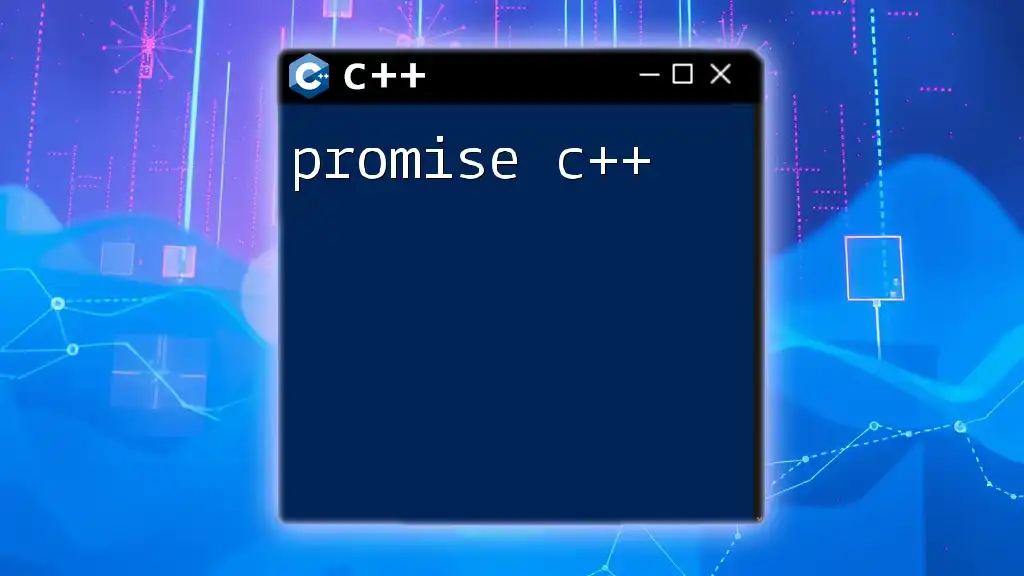
Running the Profiler
Preparing Your Code for Profiling
Before you run the profiler, structure your code with profiling in mind:
- Avoid global variables when possible, as they can introduce hidden dependencies that may skew results.
- Implement modular design by allowing profilers to track distinct functions or classes easily.
- Keep optimization flags minimal during profiling to get a clearer picture of how your code performs without optimizations.
Executing a Profiling Session
During profiling, execute the following to gather data effectively:
- Run your application under typical operating conditions to simulate real usage scenarios. This could involve loading large datasets or executing common user commands.
- Ensure to note any unusual behaviors during the profiling session, as they can give context to the data generated.
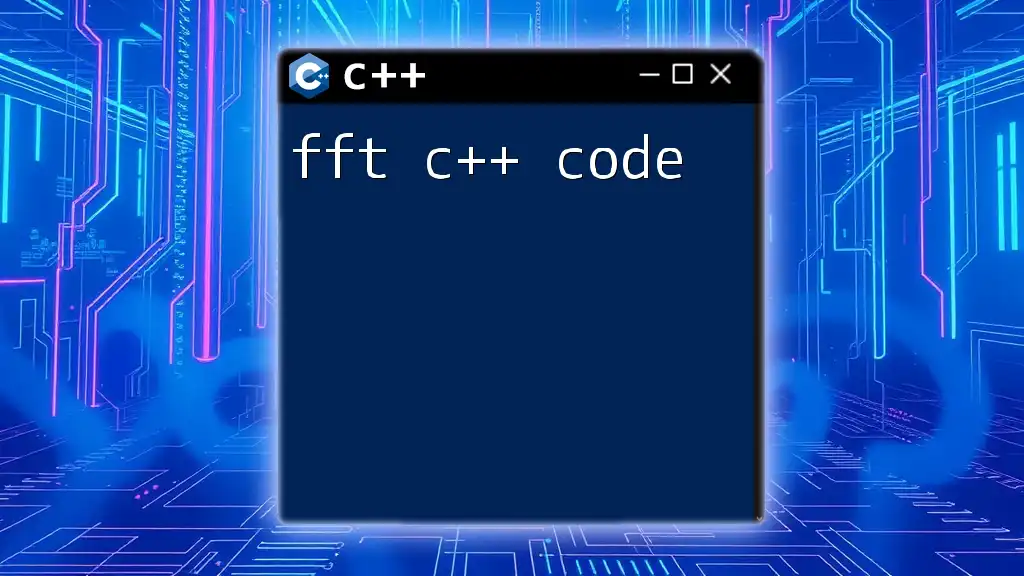
Analyzing Profiling Results
Understanding Profiling Output
Profiling tools like gprof generate a report summarizing resource utilization. Here’s how to interpret it:
- Flat Profile: Lists functions in descending order of execution time.
- Call Graph: Shows relationships between functions and how often they call one another. This visual can be invaluable in understanding complex interactions.
For instance:
Flat profile:
Each sample counts as 1 in this table.
% cumulative self self
time seconds seconds calls ms/call name
25.00 0.15 0.15 125 1.20 someFunction
20.00 0.25 0.10 50 2.00 anotherFunction
In this report, someFunction and anotherFunction consume substantial execution time, indicating they may be targets for optimization.
Visualizing Performance Bottlenecks
Visualization aids in comprehending data extracted from profiling. Various software, such as Perf report or Graphviz, can convert profiling data into graphical representations:
- Graphical Call Graphs: Visualize function calls and nested execution times.
- Heat Maps: Show resource distribution across various code segments.
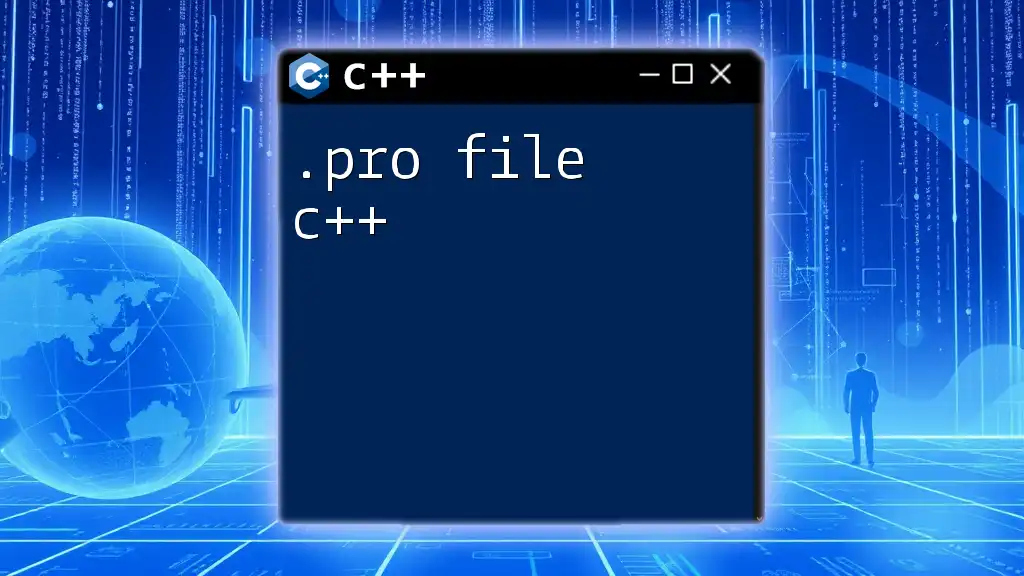
Optimizing Your C++ Code Based on Profiling
Techniques for Performance Improvement
Once bottlenecks are identified, several optimization techniques can enhance your C++ code's performance:
- Refactoring for Speed: Replace slow algorithms with faster alternatives. For instance, consider using quicksort instead of bubble sort where applicable.
- Memory Management Optimizations: Use smart pointers to avoid memory leaks and minimize memory allocation overhead.
- Reducing Function Call Overhead: Inline functions where appropriate.
inline int add(int a, int b) { return a + b; }
Example Code Optimization
Here's an example of optimizing a loop within a function:
Before Optimization:
for (size_t i = 0; i < n; i++) {
result += slowFunction(data[i]);
}
After Optimization:
auto results = std::vector<double>(n);
std::transform(data.begin(), data.end(), results.begin(), fastFunction);
for (auto value : results) {
result += value;
}
The after version leverages `std::transform`, significantly speeding up the processing time.
Continuously Monitoring Performance
Profiling is not a one-time task. Continuous monitoring allows you to maintain high performance. Regular benchmarks at different stages of development help ensure that code changes don’t introduce new bottlenecks.
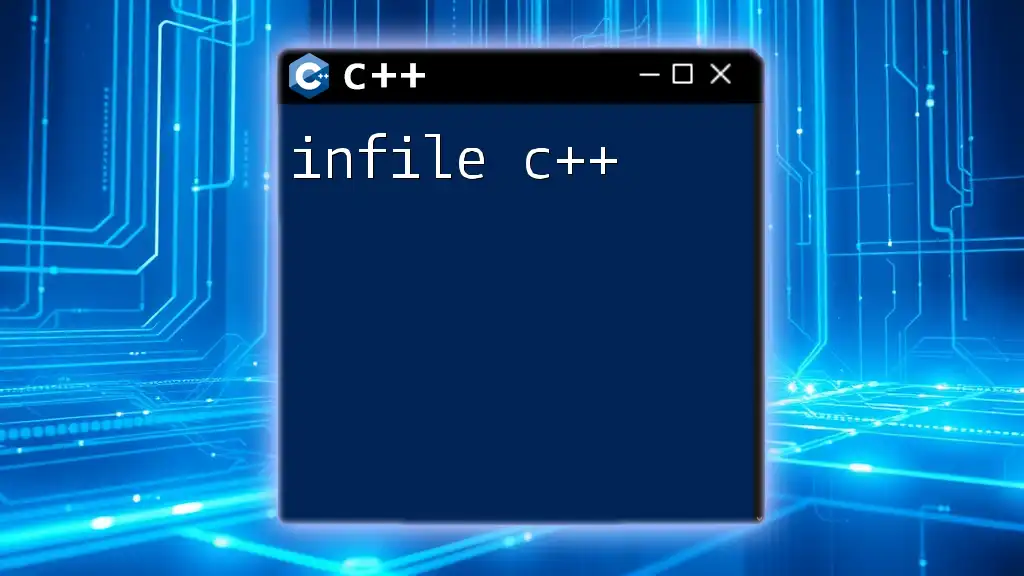
Best Practices for C++ Code Profiling
Regular Profiling Practices
Make profiling an integral part of your development workflow:
- Frequency of Profiling Sessions: Profile your C++ code at regular intervals, especially after significant changes or before release cycles.
- Set up benchmarks: Establish clear benchmarks to evaluate performance improvements.
Common Mistakes to Avoid
Be mindful of these pitfalls:
- Misinterpreting Results: Don’t jump to conclusions based on singular results; consider overall trends over multiple runs.
- Ignoring Context: Understand that performance can vary across different environments. Always replicate scenarios as closely as possible.
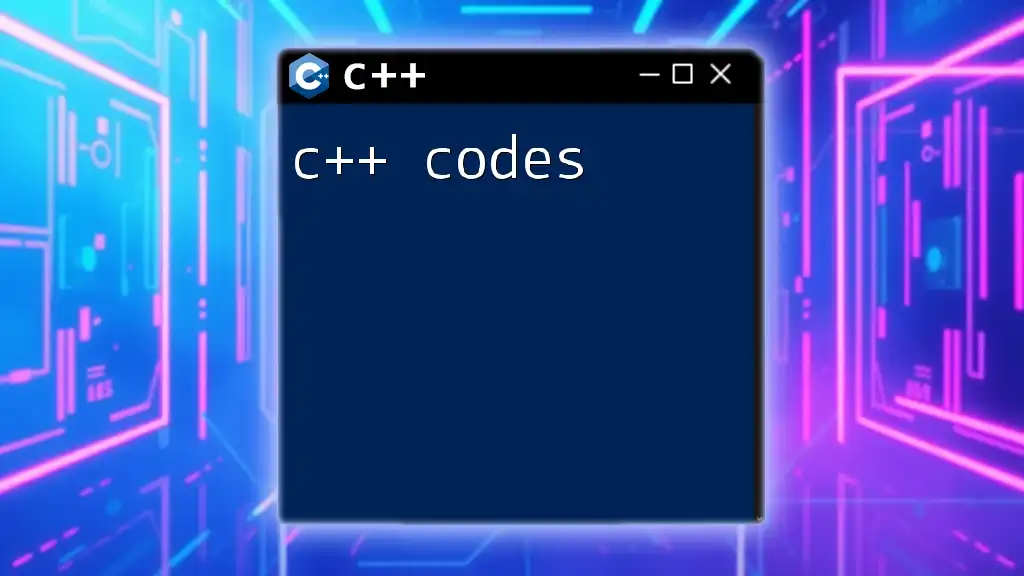
Conclusion
Profiling C++ code is essential for uncovering inefficiencies and enhancing performance. By systematically employing profiling tools, analyzing results thoroughly, and implementing optimizations, you can ensure that your applications run as efficiently as possible. Embrace profiling as a continuous practice that not only solves immediate bottlenecks but also fosters an understanding of your code’s behavior over time. Integrating these insights into your development process will greatly enhance the quality and performance of your C++ projects.
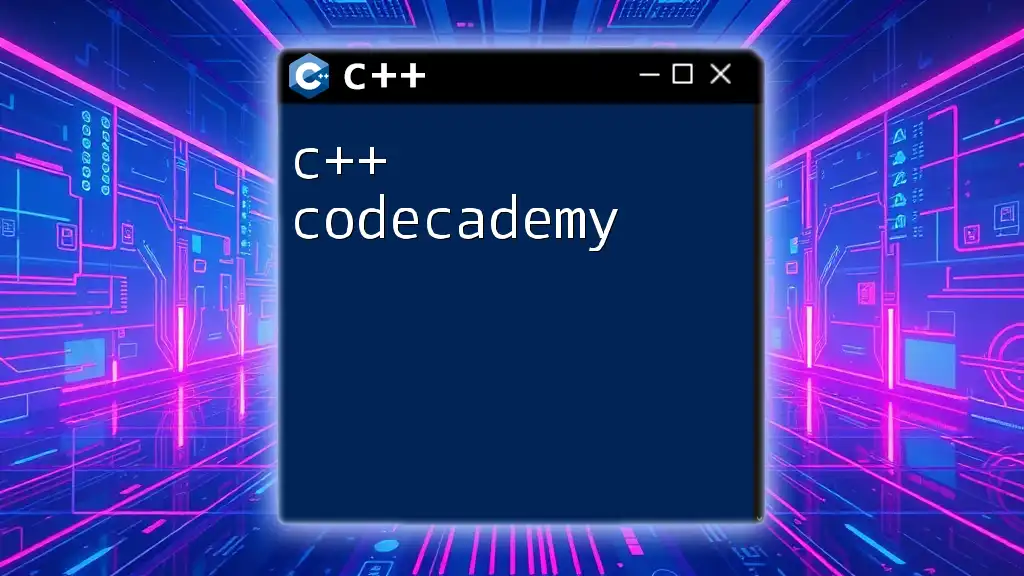
Additional Resources
Explore the wealth of information available in books, online courses, and forums to deepen your understanding of C++ profiling and improve your skills.