A `.pro` file in C++ is a project file used by the Qt framework to define project settings, specify file paths, and configure compilation details for building applications. Here's a simple example of a `.pro` file:
TEMPLATE = app
SOURCES += main.cpp
HEADERS += mainwindow.h
FORMS += mainwindow.ui
What is a .pro File?
A `.pro file` serves as a configuration file primarily used in Qt projects. It acts as a blueprint that outlines how the build system should process your C++ source code, manage resources, and link against libraries. The `.pro file` simplifies the complexities of managing multiple files in larger projects and ensures consistency across different development environments.
Structure of a .pro File
A typical `.pro file` is straightforward in its layout and consists of several key components:
- TEMPLATE: Specifies the type of project, such as an application or library.
- TARGET: Defines the name of the output file generated from the project.
- SOURCES: Lists the C++ source files used in the project.
- HEADERS: Lists the header files that the application will include.
Understanding how these components interact is crucial for successfully managing a Qt-based C++ project.
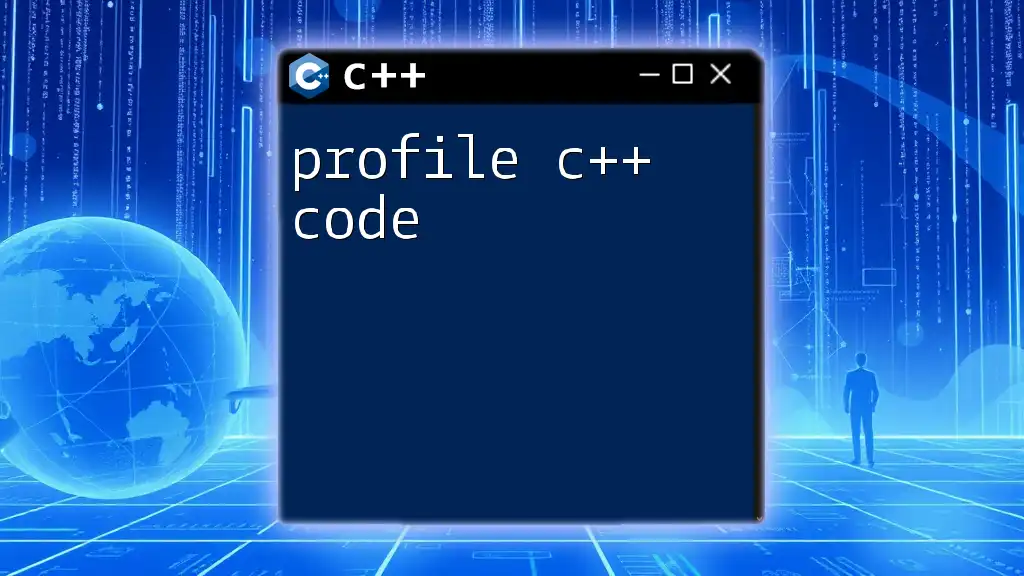
Creating a Basic .pro File
Setting Up Your Environment
Before creating a `.pro file`, ensure that you have the necessary tools installed, such as Qt Creator and the Qt SDK. These tools facilitate the development process and provide an integrated environment for writing, building, and debugging your C++ applications.
Sample Basic .pro File
Here’s an example of a very simple `.pro file`:
TEMPLATE = app
TARGET = MyApp
SOURCES += main.cpp
HEADERS += mainwindow.h
In this example:
- TEMPLATE = app indicates that the project is an application.
- TARGET = MyApp sets the name of the output executable to `MyApp`.
- SOURCES += main.cpp specifies that `main.cpp` is a source file.
- HEADERS += mainwindow.h includes the header file `mainwindow.h`.
Each component is vital for the successful building of the application.
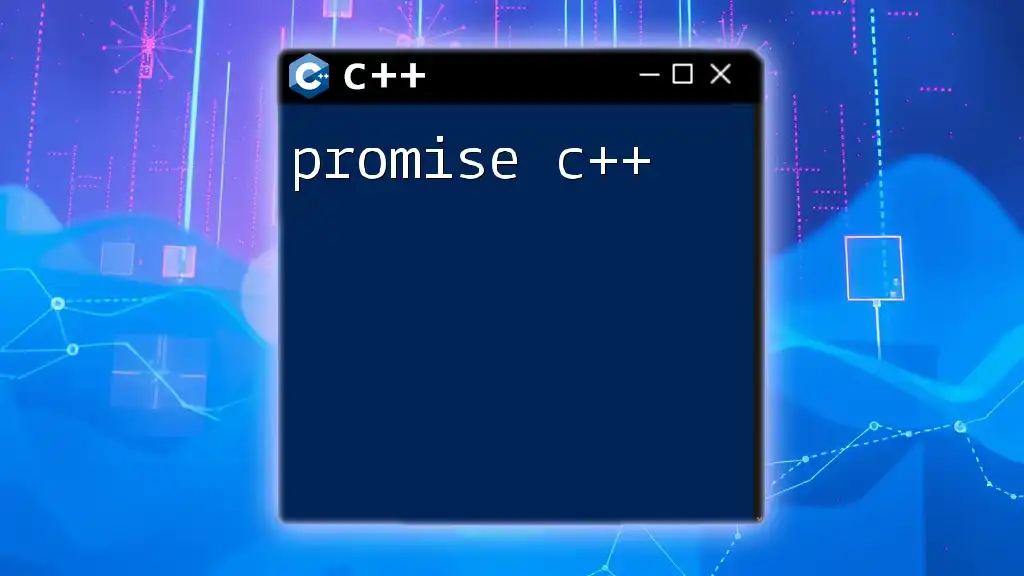
Common Variables in .pro Files
TEMPLATE
The TEMPLATE variable can have several values, including:
- app: For standalone applications.
- lib: For shared or static libraries.
- subdirs: For projects containing multiple sub-projects.
Choosing the correct template is essential for ensuring your project compiles as expected.
TARGET
The TARGET variable indicates what your output file will be called. This is especially crucial for release and debug versions; typically, you want your executable to include some indicator of the configuration it was built in (e.g., `MyApp_debug` for debug builds).
SOURCES and HEADERS
You can specify multiple source and header files in the `.pro file`. For example:
SOURCES += main.cpp helper.cpp utils.cpp
HEADERS += mainwindow.h helper.h utils.h
Additionally, you can utilize wildcards to include files dynamically:
SOURCES += $$wildcard(src/*.cpp)
HEADERS += $$wildcard(include/*.h)
This approach alleviates the need for manual updates when adding new files.
FORMS
In Qt, UI forms created with Qt Designer can also be included in the project configuration. For instance:
FORMS += mainwindow.ui
This command informs the build system to process the `mainwindow.ui` file during compilation, creating the necessary C++ classes automatically.
RESOURCES
To include resources such as images, icons, and translations, utilize the RESOURCES variable:
RESOURCES += resources.qrc
This includes the `resources.qrc` file, allowing you to bundle assets with your executable.
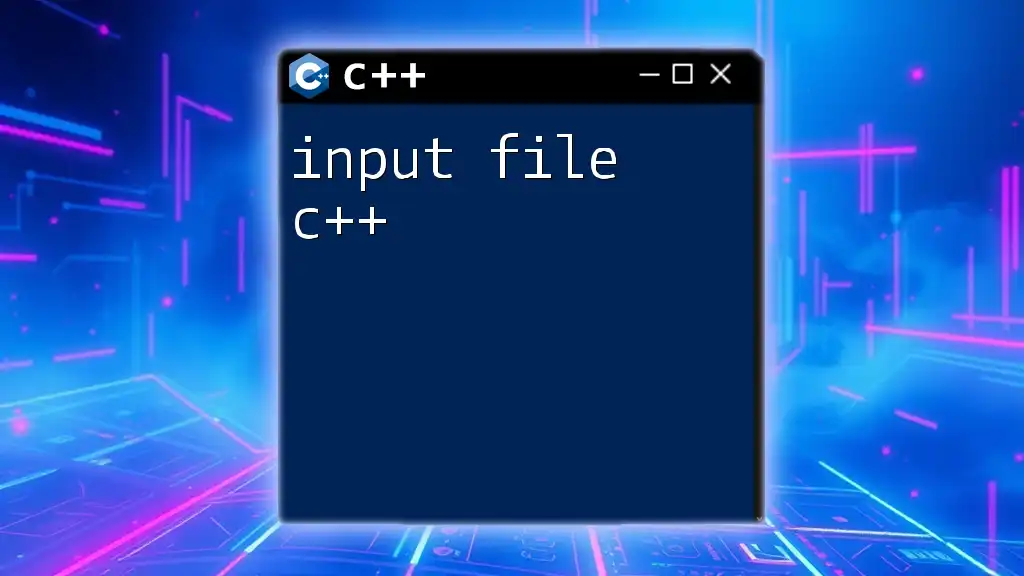
Configuring the Build Environment
INCLUDEPATH
To tell the compiler where to look for additional header files, use INCLUDEPATH:
INCLUDEPATH += /path/to/includes
This line ensures the compiler locates the headers during compilation.
LIBS
If your project relies on external libraries, you can link them using the LIBS variable:
LIBS += -L/path/to/library -lname
This tells the compiler where to find the libraries during the linking stage.
Compiler Flags
Customizing compiler settings can be done with QMAKE_CXXFLAGS or QMAKE_CFLAGS. For instance, to enable all compiler warnings:
QMAKE_CXXFLAGS += -Wall
Adjusting compiler flags can help catch issues early in the development cycle.
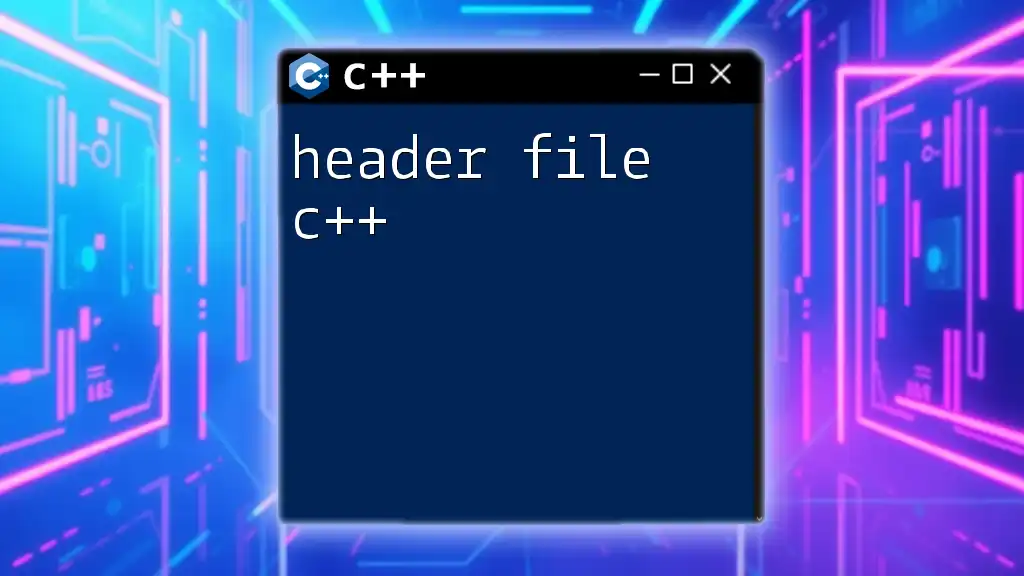
Handling Dependencies
Sub-projects
If your project is large and consists of several components, using the subdirs template is ideal. This allows you to organize related projects efficiently.
Example configuration for a subdirectory project may look like:
TEMPLATE = subdirs
SUBDIRS = projectA projectB projectC
This structure simplifies management and builds for multi-module applications.
Using External Libraries
Integrating third-party libraries into your `.pro file` is necessary for leveraging existing code. When adding libraries, make sure to account for their dependencies and potential versioning issues.
Example:
LIBS += -L/path/to/external/lib -lexternalLib
This allows your application to link against `externalLib`, crucial for extending functionality.
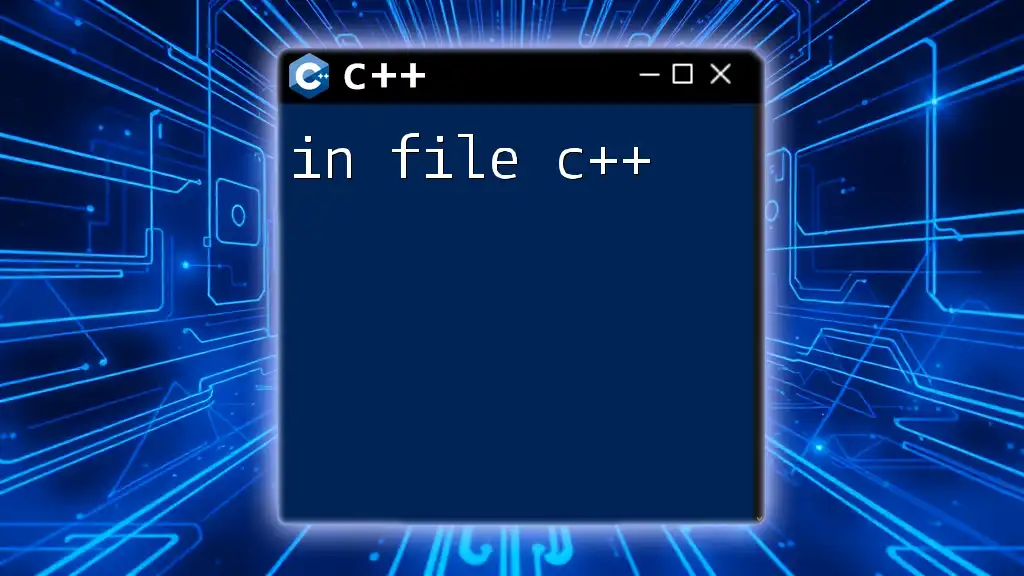
Version Control with .pro Files
Best Practices for Version Control
When using version control systems like Git, it’s essential to track your `.pro file`. Ensure you commit changes periodically to keep the configuration aligned with your source modifications.
Handling Changes
As your project evolves, so will the `.pro file`. Make deliberate changes to the `.pro file`, such as adding new files or changing configurations, and document these changes adequately. This practice aids in troubleshooting and development continuity.
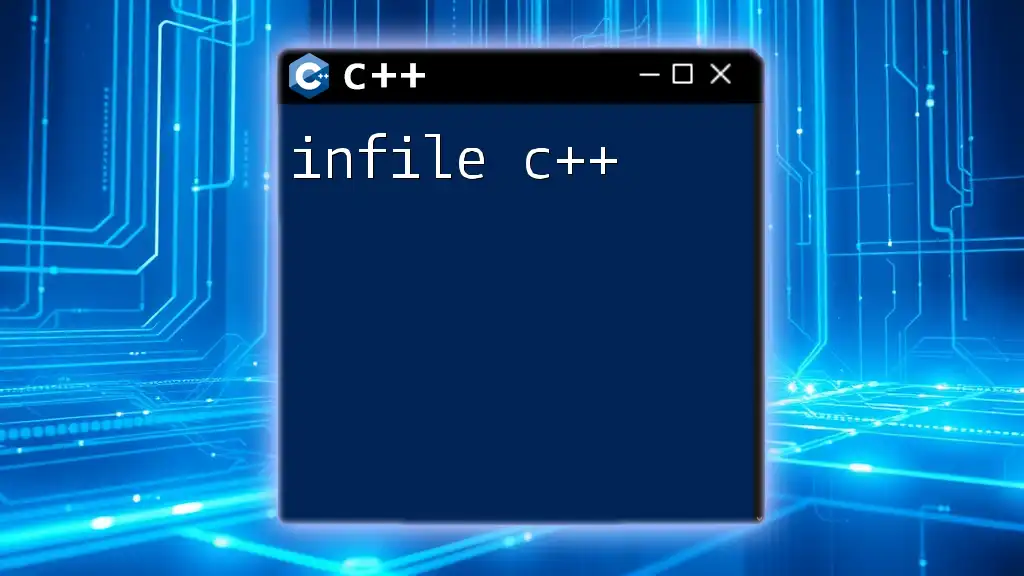
Common Mistakes and Troubleshooting
Errors Related to .pro Files
Common errors in `.pro files` often arise from incorrect paths, variable names, or syntax. Double-check that your file paths are correct and that special characters are avoided unless properly escaped.
Debugging Builds
If your build fails, using verbose output can help identify specific issues. You can enable this by adding the following line:
CONFIG += debug
Verbose output will provide detailed information about what the build system is doing, making it easier to spot errors.
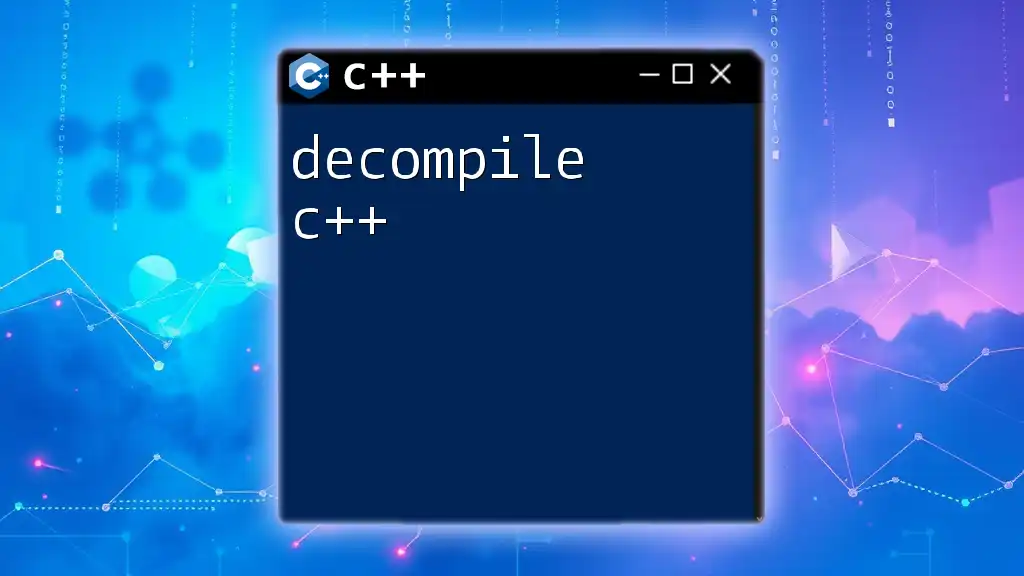
Conclusion
Understanding the significance of a `.pro file` in C++ development, especially within the Qt framework, is crucial for effectively managing your projects. By mastering the structure and common commands used in `.pro files`, you'll enhance your development workflow and simplify the complexities that come with larger applications.
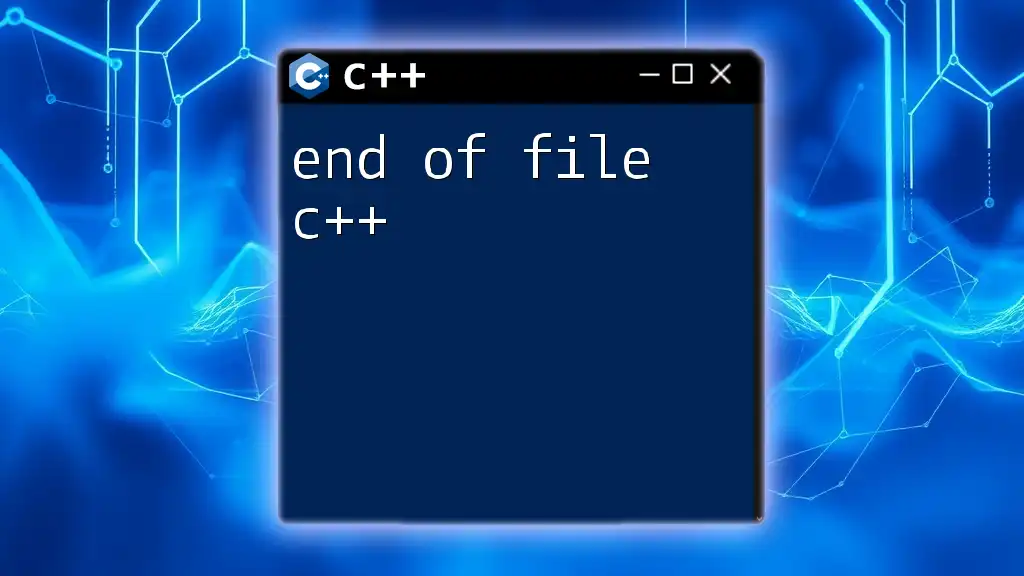
Additional Resources
For further reading and advanced examples, consider exploring the Qt documentation and community forums. Online courses and books that specialize in C++ and Qt development are also excellent resources for those looking to deepen their understanding.