The `strftime` function in C++ is used to format time and date information according to specified format specifiers, enabling developers to create human-readable date and time strings.
Here's an example code snippet:
#include <iostream>
#include <iomanip>
#include <ctime>
int main() {
std::time_t t = std::time(nullptr);
char buffer[100];
std::strftime(buffer, sizeof(buffer), "%Y-%m-%d %H:%M:%S", std::localtime(&t));
std::cout << "Current date and time: " << buffer << std::endl;
return 0;
}
What is strftime?
`strftime` is a powerful function available in C and C++ programming that formats date and time information into a string. It stands out as an essential tool for developers who need to present date and time in a user-friendly manner. By utilizing `strftime`, programmers can convert the internal representation of time into human-readable formats, which is crucial for applications ranging from logging systems to user interfaces.
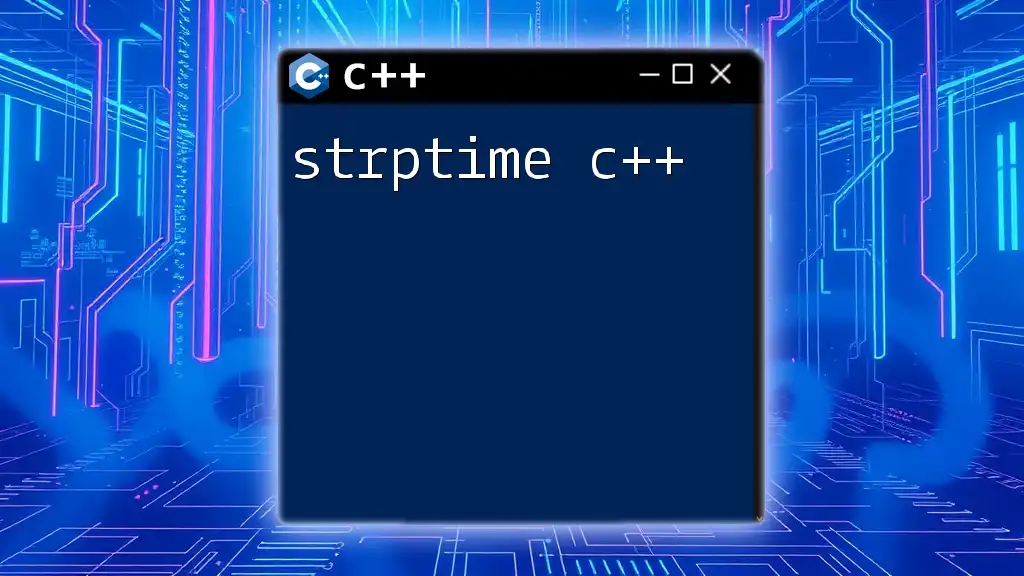
History and Purpose
The `strftime` function is part of the C standard library and has been widely adopted in C++ for its ability to create formatted date and time strings effortlessly. Its versatility and ease of use have made it a staple in many applications where datetime representation is necessary.
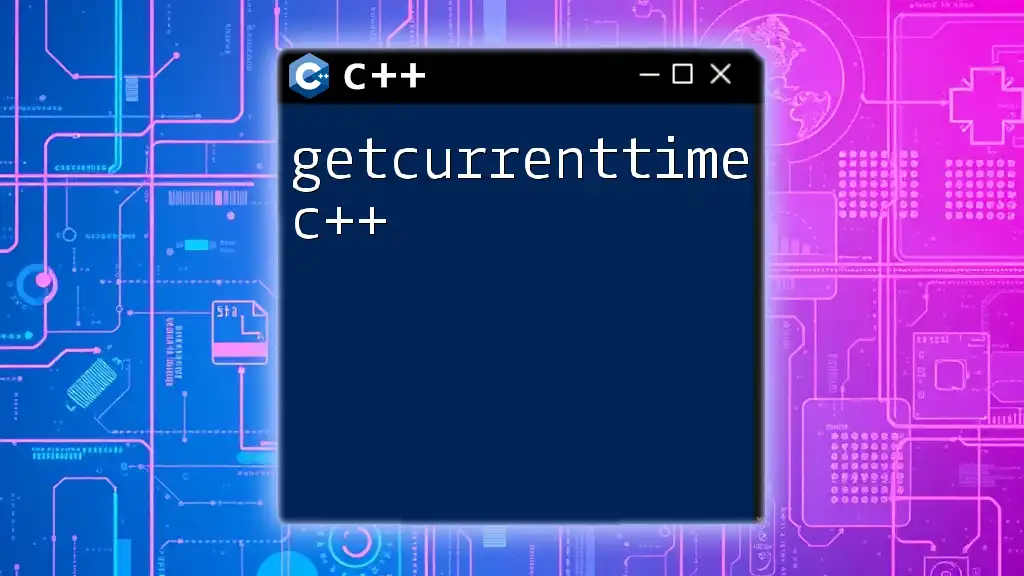
Understanding the Basics of Time Representation
Date and Time in C++
In C++, date and time are primarily managed using the standard library's `<ctime>` header, which provides various functions and types for handling time. Compared to other programming languages, C++ offers a robust set of facilities for datetime manipulations, bridging the gap between raw time values and human-readable formats.
The Time Structure
The core of time representation in C++ is the `tm` structure, which encapsulates individual components of time, such as year, month, day, hour, minute, and second. Here is a breakdown of its members:
- tm_year: Year since 1900
- tm_mon: Month (0-11; January is 0)
- tm_mday: Day of the month (1-31)
- tm_hour: Hour (0-23)
- tm_min: Minute (0-59)
- tm_sec: Second (0-60, includes leap seconds)
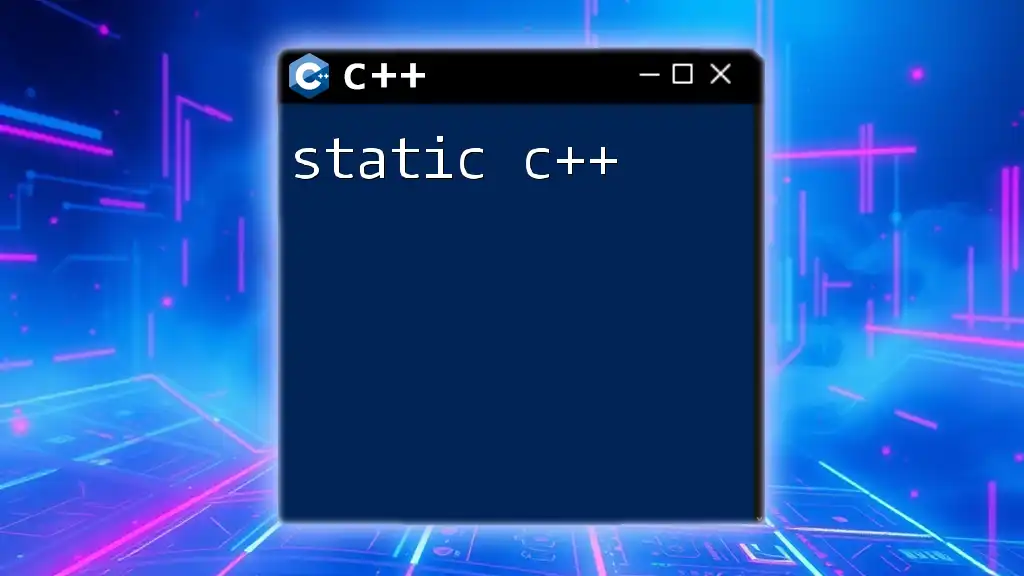
Syntax of strftime
Function Prototype
The basic syntax of the `strftime` function is as follows:
size_t strftime(char *str, size_t maxsize, const char *format, const struct tm *tm);
Parameters:
- str: Pointer to the destination string where the formatted date/time will be stored.
- maxsize: Maximum size of the string to prevent overflow.
- format: Format specifier string.
- tm: Pointer to the `tm` structure containing date and time components.
Return Value
The `strftime` function returns the number of characters placed in the output string, excluding the terminating null character. If the output string cannot hold the result, `strftime` returns zero. Handling the return value properly is essential for ensuring that your program performs as expected without errors.
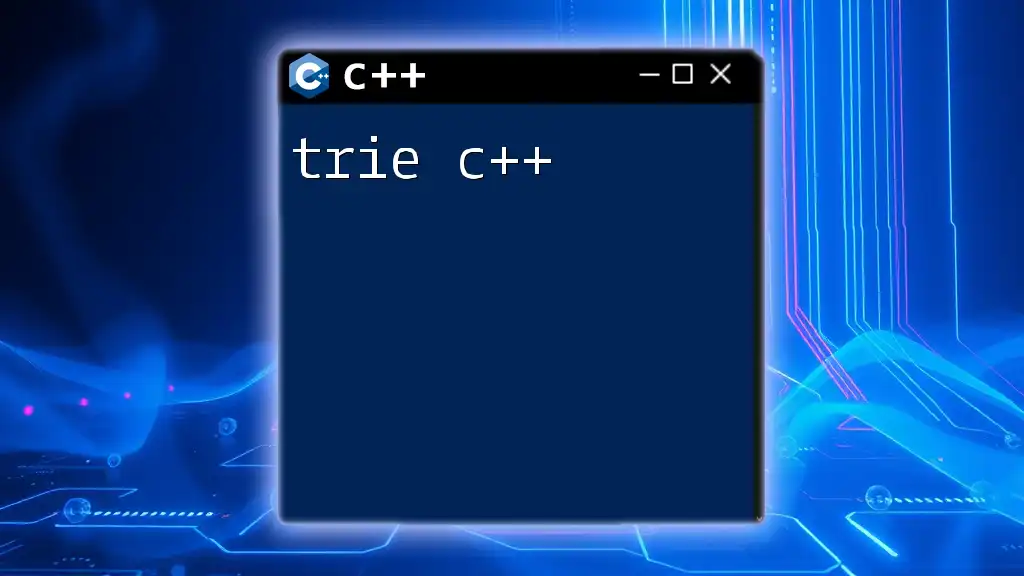
Format Specifiers in strftime
Overview of Format Specifiers
Format specifiers act as placeholders in the format string for specific components of date and time. They allow you to define how you want the output to appear, providing flexibility for various needs.
Commonly Used Specifiers
- %Y: Year with century (e.g., 2023)
- %m: Month as a zero-padded number (01 to 12)
- %d: Day of the month as a zero-padded number (01 to 31)
- %H: Hour in 24-hour format (00 to 23)
- %M: Minute (00 to 59)
- %S: Second (00 to 60, allowing for leap seconds)
Here’s an example of a simple format that incorporates several specifiers:
strftime(buffer, sizeof(buffer), "%Y-%m-%d %H:%M:%S", now);
// Result: "2023-10-13 14:30:00"
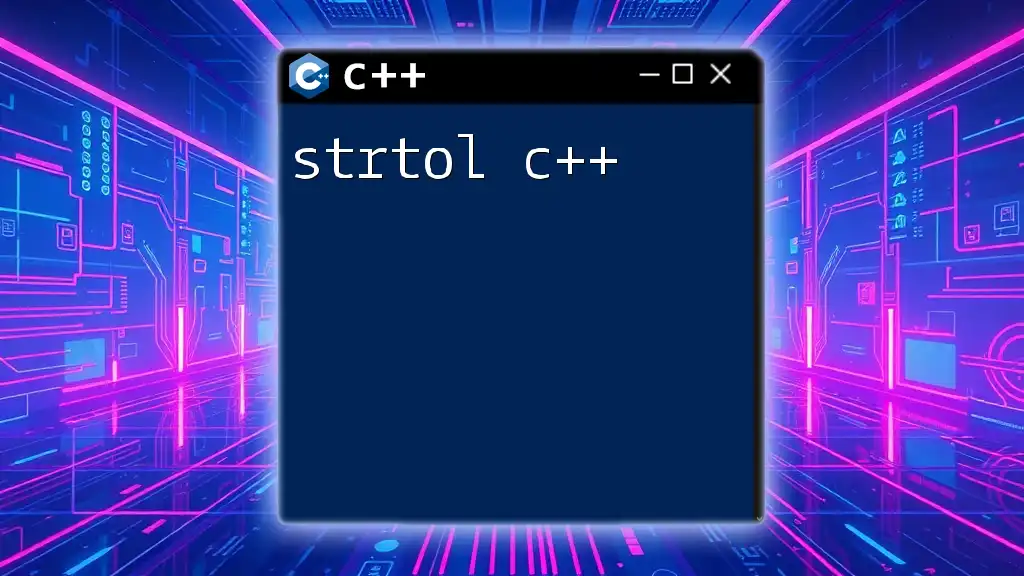
Detailed Examples of Using strftime
Basic Example
To illustrate the basic usage of `strftime`, here is a simple program that formats the current date and time:
#include <iostream>
#include <ctime>
int main() {
time_t t = time(0);
tm* now = localtime(&t);
char buffer[80];
strftime(buffer, sizeof(buffer), "%Y-%m-%d %H:%M:%S", now);
std::cout << "Current Date and Time: " << buffer << std::endl;
return 0;
}
This example captures the current time, formats it using the `strftime` function, and displays it in the format of "YYYY-MM-DD HH:MM:SS".
Advanced Time Formatting
For a more complex example, you can create a formatted string that includes the day of the week and the full month name:
strftime(buffer, sizeof(buffer), "%A, %B %d, %Y %I:%M%p", now);
// Output: "Friday, October 13, 2023 02:30PM"
This output is more descriptive, making it easier for users to understand.
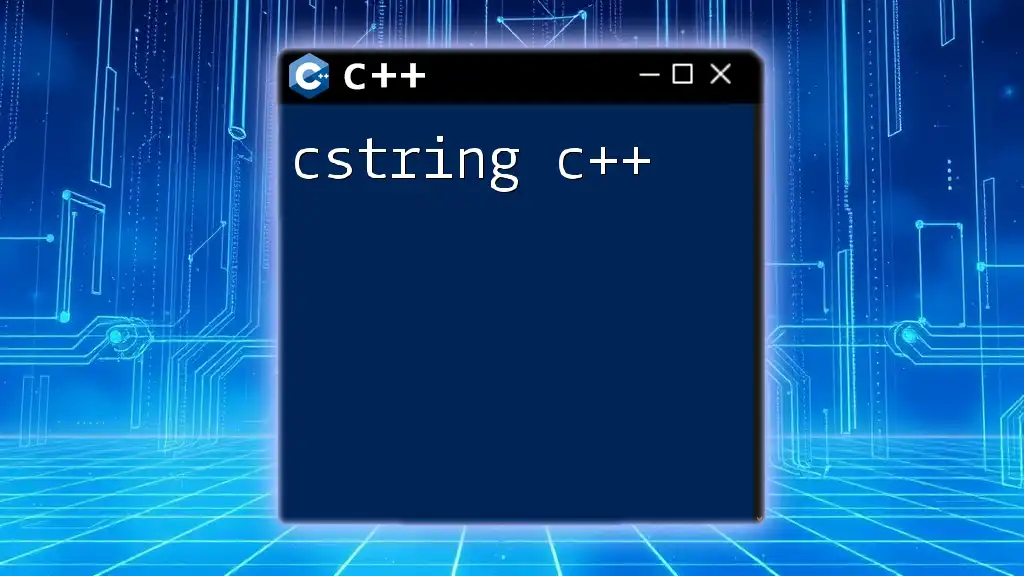
Custom Date and Time Formats
Creating Custom Formats
Developers can define custom formats by combining various specifiers. For example, to represent a date like "13th October 2023", one might use:
strftime(buffer, sizeof(buffer), "%d %B %Y", now);
// Output: "13 October 2023"
Practical Use Cases
Custom formats are particularly valuable in applications that require specific date representations based on local customs or user preferences. Logging systems might also benefit by standardizing date formats across records, making it easier to parse and query data.
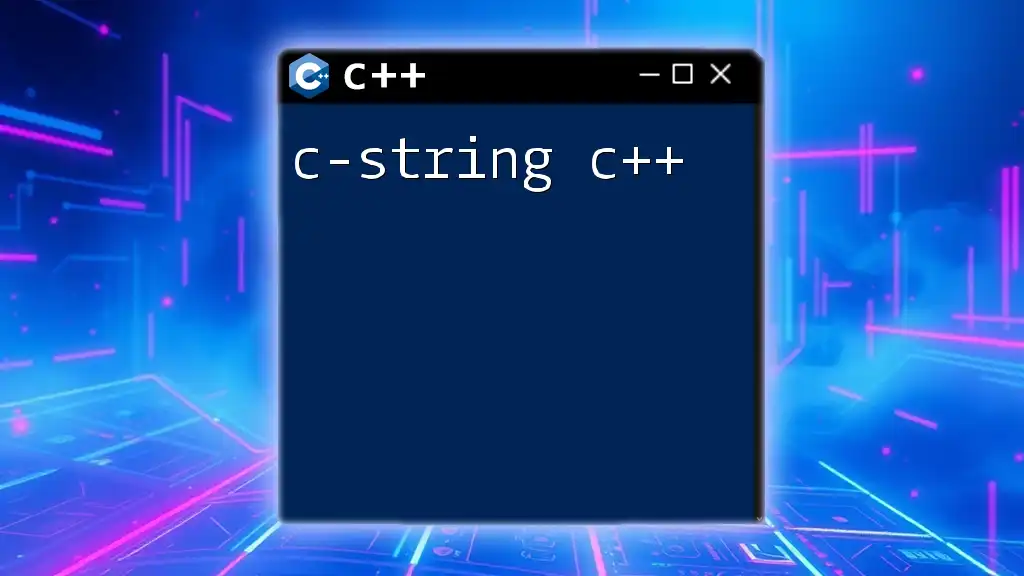
Error Handling with strftime
Common Errors and Exceptions
While `strftime` is generally reliable, it can run into common errors like buffer overflow if the string size is underestimated. Be cautious with the size of the `buffer`.
Checking Result of strftime
It is good practice to check whether `strftime` has successfully formatted the string. For instance:
if(strftime(buffer, sizeof(buffer), "%Y-%m-%d", now) == 0) {
std::cerr << "Error formatting date." << std::endl;
}
This helps ensure graceful handling of errors, contributing to more robust applications.
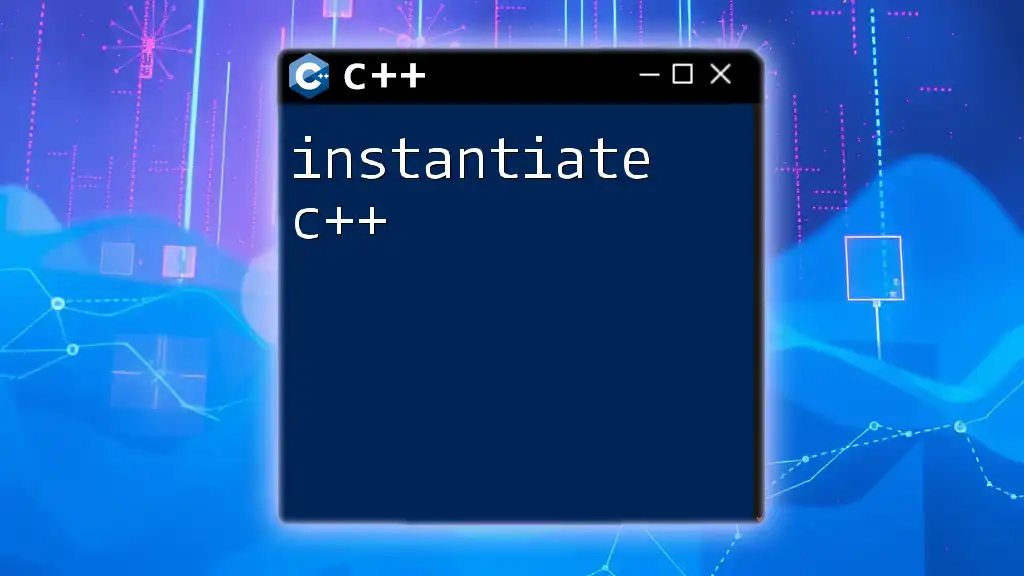
Performance Considerations
Efficiency of strftime
While `strftime` is efficient for typical use cases, developers should be mindful of its limitations in high-performance scenarios where many format calls are made in rapid succession. In cases of heavy repetitive formatting, consider caching results or building your own lightweight formatter.
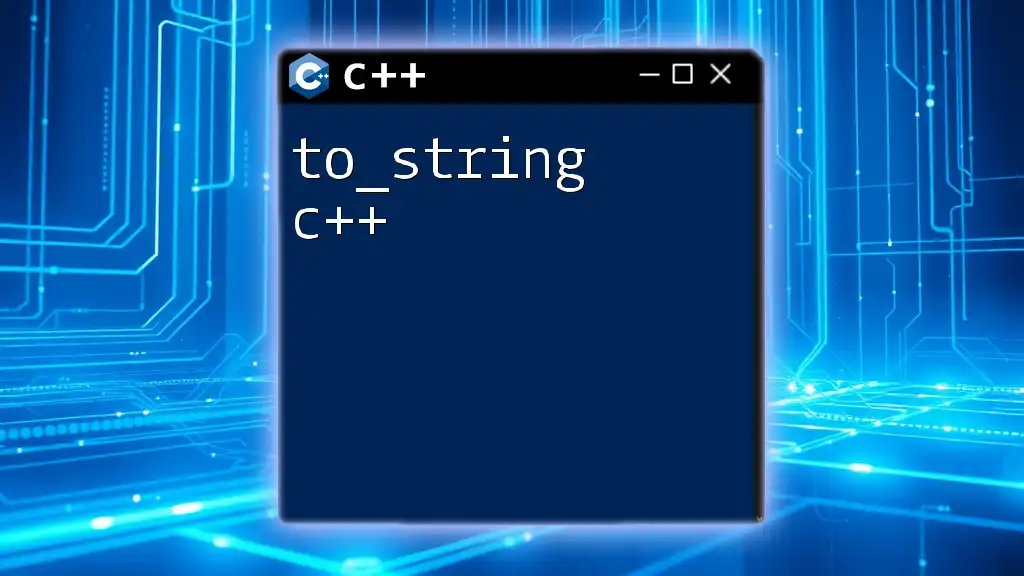
Conclusion
The `strftime` function is an integral part of date and time management in C++. It provides a simple yet powerful way to format time data for a variety of applications. By mastering its syntax and understanding its intricacies, developers can elevate their coding skills and improve user experience.
Further Reading and Resources
For those looking to delve deeper into the world of C++ datetime manipulation, consider browsing official documentation or additional programming tutorials on related libraries. These resources can significantly enhance your understanding and use of date and time functions in C++.
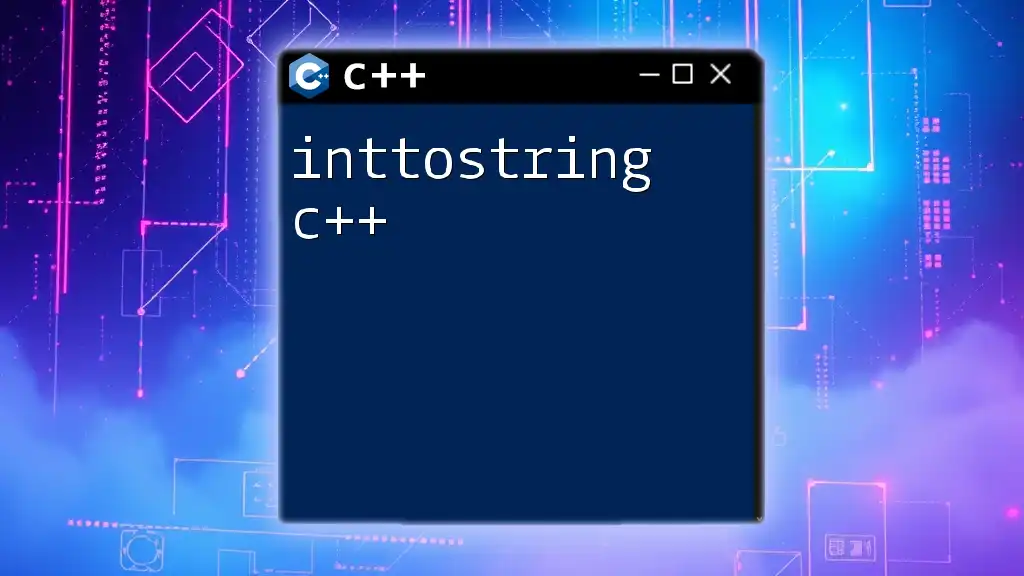
Frequently Asked Questions (FAQs)
What are some alternative methods to format time in C++?
Other options for time formatting include the use of the C++20 `<chrono>` library and third-party libraries like `Boost.Date_Time`, which provide more advanced features.
Can strftime handle timezone conversions?
The `strftime` function deals primarily with local times as defined by the system's settings. For timezone conversions, additional libraries or manual adjustments are typically necessary.
How does strftime differ from the new C++20 date libraries?
The C++20 date libraries offer more modern approaches to handling date and time, with improved handling of time zones, durations, and calendar systems compared to the traditional `strftime` approach.