A sentinel in C++ is a special value used to signify the end of a data set, often utilized in loops to control the number of iterations, ensuring efficient input handling without the need for a predefined size.
Here's a simple example using a sentinel value:
#include <iostream>
int main() {
int number, sum = 0;
std::cout << "Enter numbers to sum (enter -1 to stop):\n";
while (std::cin >> number && number != -1) {
sum += number;
}
std::cout << "Total sum: " << sum << std::endl;
return 0;
}
Understanding the Sentinel Value
Definition of a Sentinel Value
A sentinel value is a special value that is used to signify the end of a data structure or to indicate what constitutes valid input within an algorithm. Sentinels are particularly useful when trying to manage complex data flows, such as during iterations in loops or while processing arrays. Their primary function is to avoid unnecessary additional checks for conditionals that would otherwise complicate your code.
Characteristics of Sentinel Values
Sentinel values often possess unique properties that set them apart from the regular data in a data structure. Common examples include:
- Negative Numbers: For an array of positive integers, the value `-1` could act as a sentinel.
- NULL: In pointers, a NULL pointer may serve as an indicator that no valid object is assigned.
The key attribute of a sentinel is that it must be easily distinguishable from valid data.
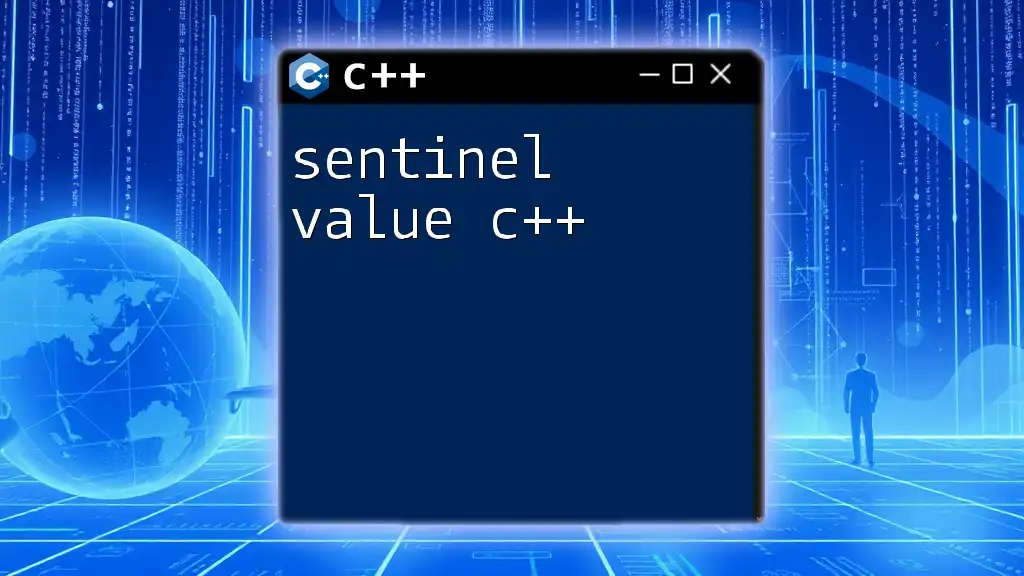
Benefits of Using Sentinel Values
Simplifying Control Structures
Sentinel values can significantly streamline loop and condition structures, providing a clear target that signifies termination. For example, instead of checking the size of an array in each iteration, you can rely on a sentinel value to conclude your loop.
int input;
while (std::cin >> input && input != -1) { // -1 as sentinel
// Process input
}
Here, the loop will continue until the user enters `-1`, simplifying the control structure and making the intent clear.
Avoiding Off-by-One Errors
One of the most common pitfalls in programming is the off-by-one error, where loops iterate one too many or one too few times. By using a sentinel value, you can circumvent this issue.
For example, when counting valid entries in a data series, a sentinel value can clearly delineate the end of the input:
int count = 0;
int num;
while (std::cin >> num && num != 0) { // 0 as a sentinel
count++;
}
This structure ensures that the loop halts precisely when the sentinel is encountered, effectively eliminating any potential off-by-one errors.
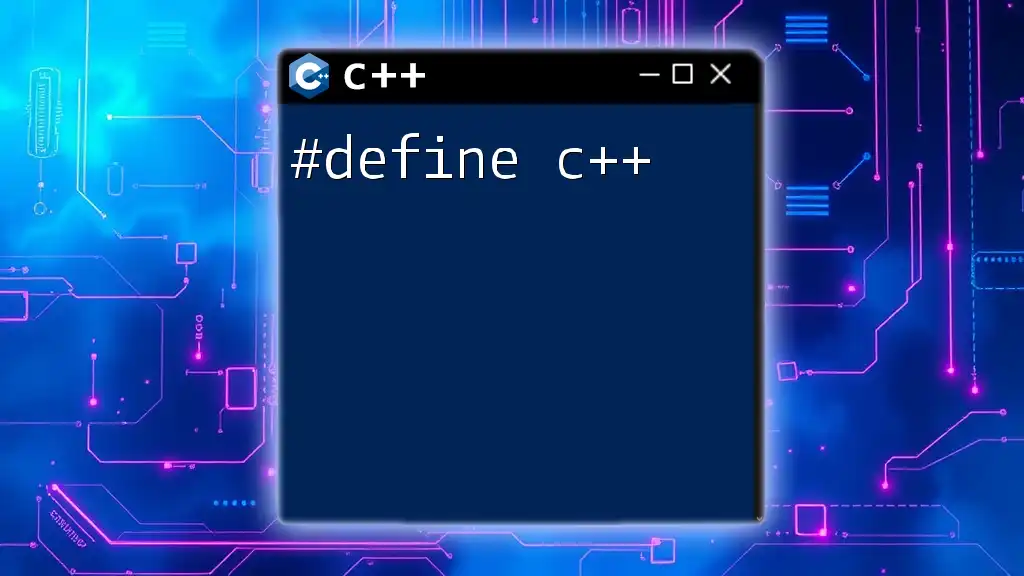
Common Use Cases for Sentinel Values in C++
Using Sentinel Values in Loops
One of the most ubiquitous scenarios for sentinel c++ programming is within loop structures. A practical example is finding the maximum value within a list of integers:
int maxValue = -1; // Sentinel value for empty input
for (int input; std::cin >> input;) {
if (input > maxValue) {
maxValue = input;
}
}
// Where for additional context, -1 signifies that no input has been provided.
In this case, `maxValue` starts as `-1`, signaling that no input has yet been processed. If the input stream yields no values, the maximum remains `-1`.
Using Sentinel Values in Arrays
Sentinel values are also highly effective within arrays. They can provide a clean way of iterating through an array without the need for explicit bounds checking:
int arr[] = {10, 20, 0, 30, 40}; // 0 as a sentinel
int i = 0;
while (arr[i] != 0) { // Process until we hit the sentinel
// Process arr[i]
i++;
}
In this example, `0` serves as a sentinel, indicating the termination of valid data in the array. The loop continues processing array elements until the sentinel is reached.
Using Sentinel Values in Functions
Sentinel values can also enhance functions designed to manipulate data collections. Here’s an example function that counts elements until a sentinel value is encountered:
int countUntilSentinel(const int* arr) {
int count = 0;
while (arr[count] != 0) { // 0 as sentinel
count++;
}
return count;
}
This function efficiently counts the number of elements preceding the sentinel value, demonstrating how a well-placed sentinel can keep your code concise and readable.
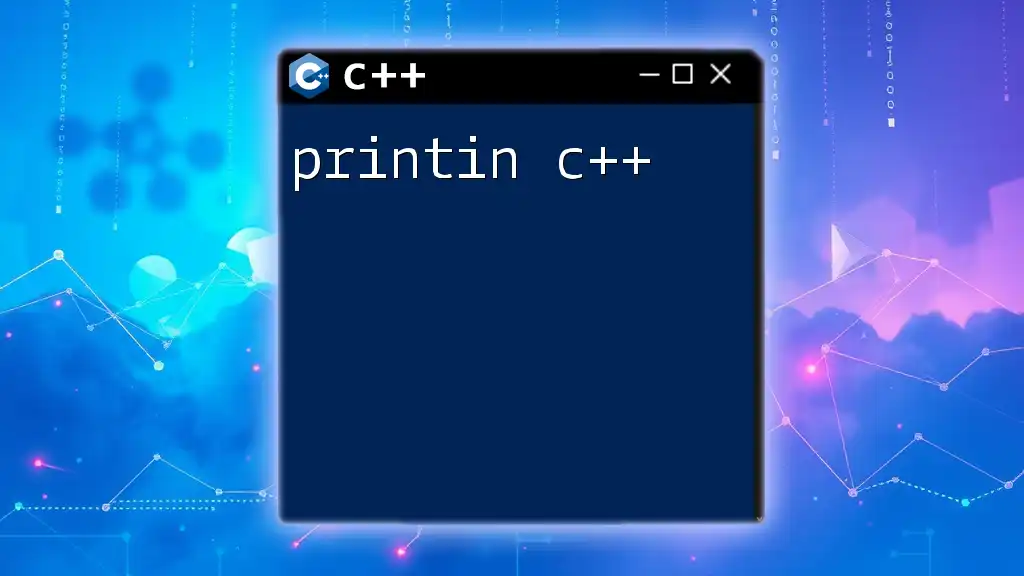
Best Practices for Implementing Sentinel Values
Choosing Appropriate Sentinel Values
Select sentinel values that are easy to identify and do not clash with valid data. For example, if you’re tracking ages, using `-1` as a sentinel is effective since no valid age can be negative. Careful selection prevents logical errors and enhances clarity.
Documenting Sentinel Use
Code documentation is vital when employing sentinel values. Provide clear comments outlining the purpose and choice of sentinel values at the beginning of loops and functions. This practice helps other programmers (or your future self) understand your logic without ambiguity.
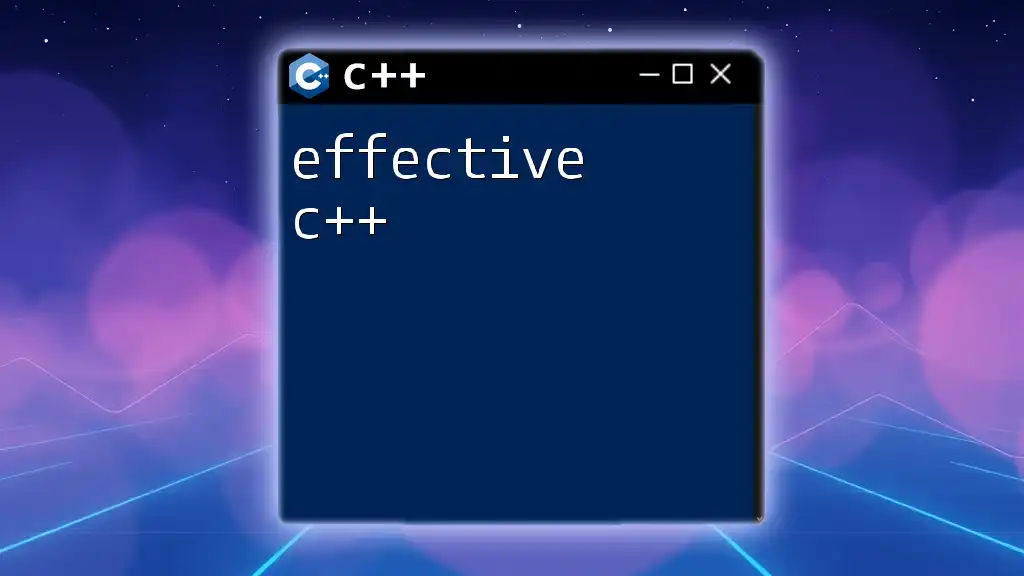
Challenges and Drawbacks of Sentinel Values
Potential for Confusion
While sentinels simplify some scenarios, they can introduce complexity when used improperly. If a sentinel is not clearly defined or becomes mixed with valid data, it can lead to unexpected results. An example might be a loop inadvertently including the sentinel value as legitimate input if logic isn’t clearly established.
Performance Implications
Although obfuscating logic isn't a frequent concern, performance can fluctuate when a program heavily relies on sentinel values. Ensure that the sentinel does not introduce overhead, particularly with larger datasets. Evaluate if they are the best fit for your specific cases compared to other structures like zero-terminated arrays or indexing approaches.
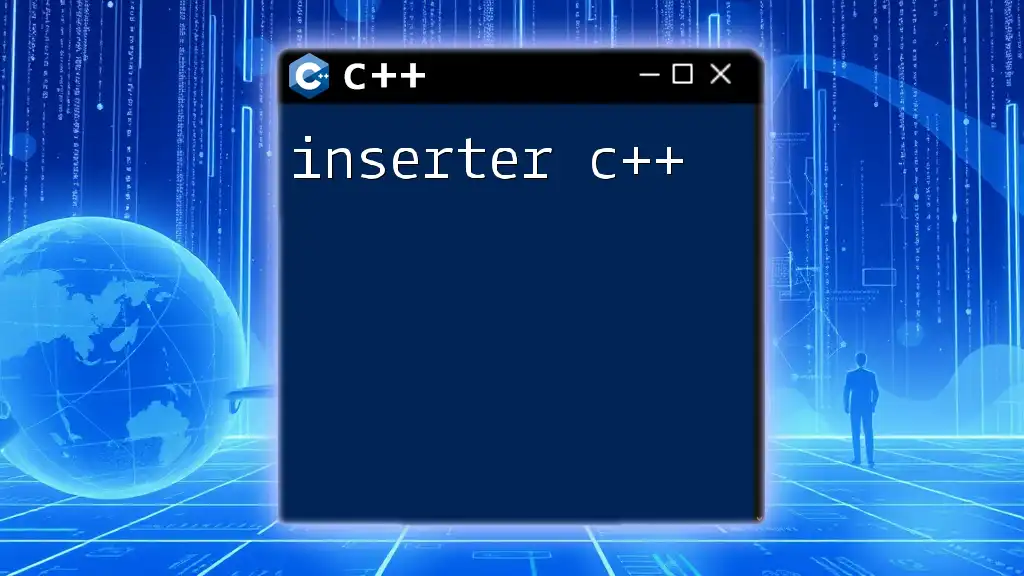
Conclusion
In summary, sentinel c++ offers significant advantages in streamlining control structures, aiding in error prevention, and improving code readability. While they come with challenges, carefully utilizing sentinel values can enhance your programming efficiency. Understanding when and how to implement sentinels is fundamental to writing robust C++ code.
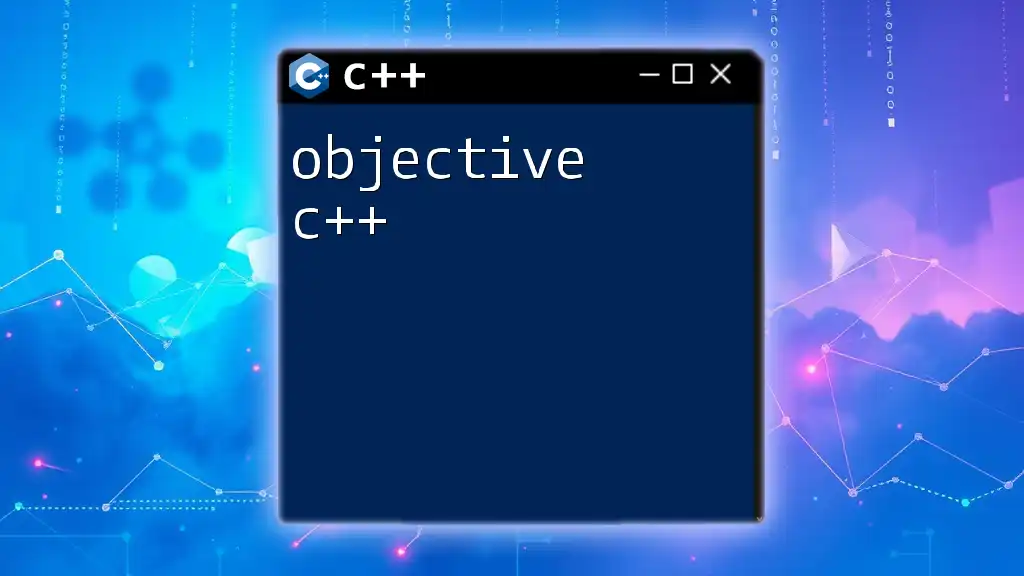
Additional Resources
For further exploration of programming best practices, consult online platforms, community forums, and documentation that delve deeper into C++ principles involving sentinel values and their optimal uses.
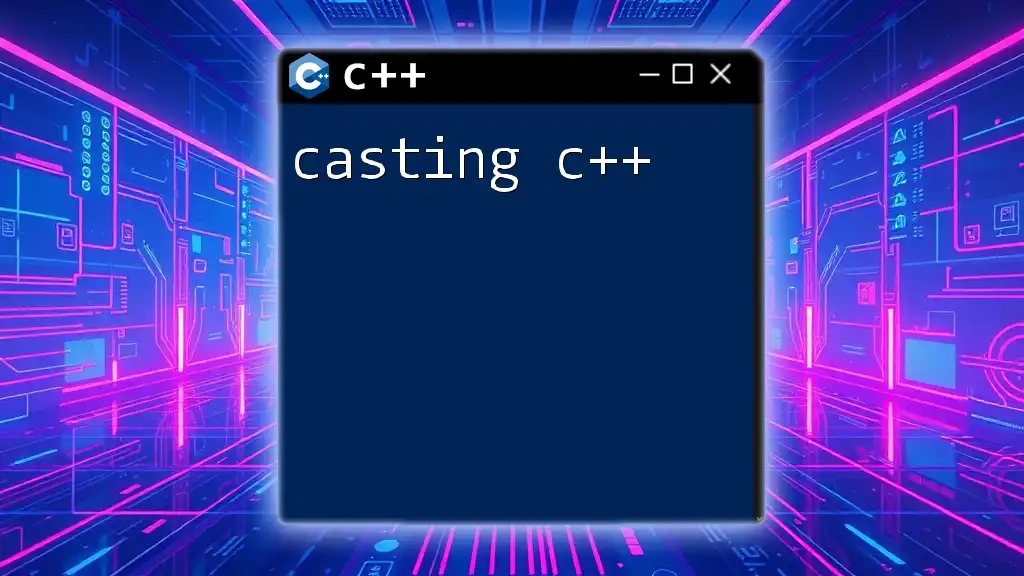
Call to Action
For more tutorials on C++ commands and practical coding strategies, be sure to check out our platform and embark on a journey of efficient learning!