Tokenizing in C++ refers to the process of splitting a string into individual components or tokens based on specified delimiters, often using the standard library functions. Here's a simple example to illustrate this:
#include <iostream>
#include <sstream>
#include <vector>
int main() {
std::string input = "hello,world,this,is,C++";
std::stringstream ss(input);
std::string token;
std::vector<std::string> tokens;
while (std::getline(ss, token, ',')) {
tokens.push_back(token);
}
for (const auto& str : tokens) {
std::cout << str << std::endl;
}
return 0;
}
What is Tokenization?
Tokenization is the process of dividing a string into smaller segments, known as tokens. In programming, tokens can be defined as meaningful sequences of characters, such as words separated by spaces or other delimiters. The importance of tokenization cannot be overstated; it forms the backbone of text processing, parsing, and data manipulation in various applications, including compilers, natural language processing, and even simple user input parsing.
Why Use Tokenization in C++?
Using tokenization in C++ offers numerous advantages. Key advantages include:
- Data Manipulation: Tokenization allows for effective parsing of input data, enabling developers to extract useful information from user inputs or files effortlessly.
- Flexible Processing: The ability to define custom delimiters when tokenizing strings gives developers the flexibility to process data in ways that standard methods may not support.
- Performance: Efficient tokenization can be critical in performance-sensitive applications where processing speed is crucial.
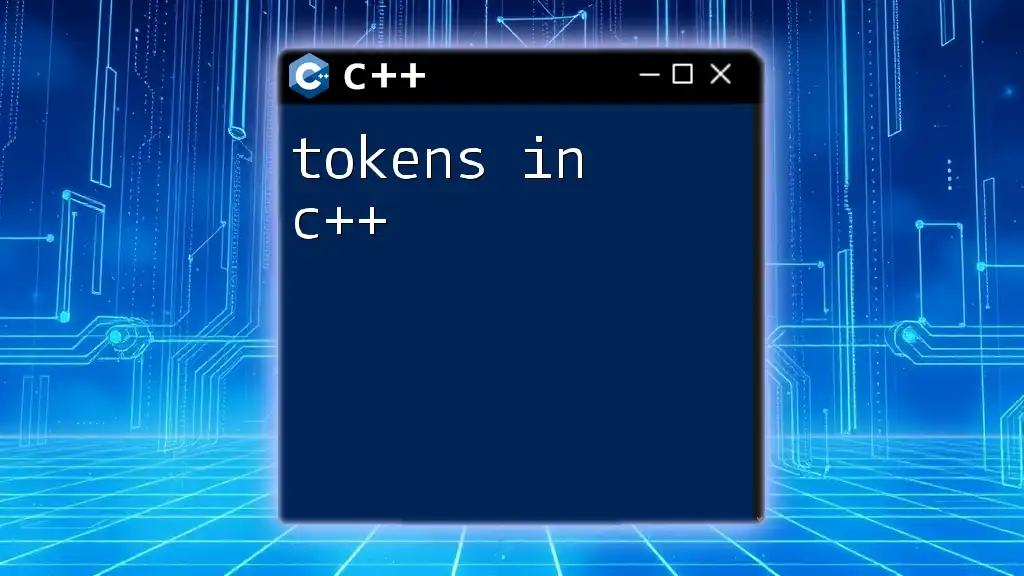
Understanding the C++ String Class
Overview of C++ Strings
C++ uses the `std::string` class as its primary means of handling strings. This class provides a robust set of functionalities for creating, manipulating, and destroying strings. Understanding the properties of the `std::string` class is essential for effective tokenization, as you will often work directly with string objects.
Characteristics of C++ Strings
C++ strings are dynamic in size and mutable, meaning they can be modified after their creation. This flexibility is vital when it comes to tokenization; you might need to modify the tokens or the original string depending on your application's requirements.
The memory management aspects of C++ strings also play a significant role in tokenization efficiency, making it crucial to understand how strings allocate and deallocate memory.
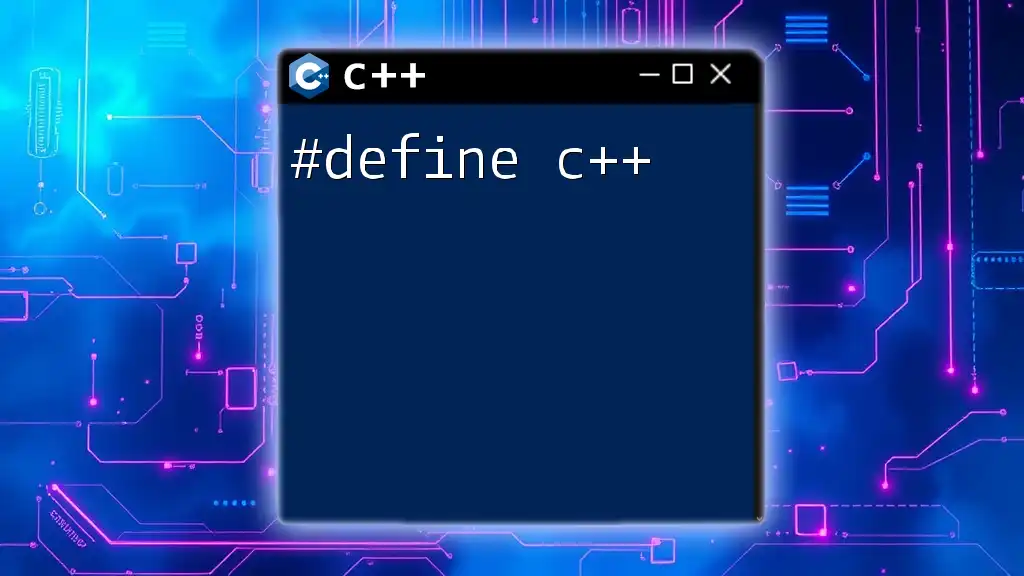
Tokenization Techniques in C++
Manual Tokenization Approach
Defining Tokens
In the context of C++, a token is commonly defined by certain delimiters—characters that separate different parts of a string. For example, in the sentence "Hello, world!", the comma and space serve as delimiters when breaking it into tokens.
Using Loops and Conditionals
A straightforward way to tokenize a string in C++ is by using loops and conditionals. Here’s a code snippet illustrating a simple manual tokenization method:
#include <iostream>
#include <string>
#include <vector>
std::vector<std::string> manualTokenize(const std::string &str, char delimiter) {
std::vector<std::string> tokens;
std::string token;
for (char ch : str) {
if (ch == delimiter) {
tokens.push_back(token);
token.clear();
} else {
token += ch;
}
}
if (!token.empty()) {
tokens.push_back(token);
}
return tokens;
}
In this example, we traverse each character in the string. When we encounter the specified delimiter, we push the current token into the `tokens` vector, and clear it to prepare for the next segment. This method is simple yet effective for basic tokenization.
Using the C++ Standard Library for Tokenization
The `std::istringstream` Class
An alternative approach to manual tokenization is leveraging the `std::istringstream` class from the C++ Standard Library. This class allows for treating a string as a stream, enabling easier extraction of tokens. Here’s how you can implement it:
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
std::vector<std::string> tokenizeString(const std::string &str, char delimiter) {
std::istringstream iss(str);
std::string token;
std::vector<std::string> tokens;
while (std::getline(iss, token, delimiter)) {
tokens.push_back(token);
}
return tokens;
}
This code snippet uses `std::getline` to read chunks of the string, splitting it by the specified delimiter. The result is a vector of tokens without manually tracking positions or indices.
C++ String Tokenizer Libraries
Overview of Popular Libraries
When performance and functionality are critical, leveraging established libraries can simplify string tokenization. One popular choice is Boost, a widely used C++ library known for its extensive functionalities, including robust string handling.
Example with Boost Tokenizer
The Boost Tokenizer library offers a streamlined approach to string tokenization. Here’s an example:
#include <iostream>
#include <boost/tokenizer.hpp>
void boostTokenizerExample(const std::string &str) {
typedef boost::tokenizer<boost::char_separator<char>> tokenizer;
boost::char_separator<char> sep(" ");
tokenizer tokens(str, sep);
for (const auto &token : tokens) {
std::cout << token << std::endl;
}
}
In this code, we define a char separator for spaces, which allows us to tokenize the input string efficiently. Boost handles the complexity of token management, making it an excellent choice for developers looking for simplicity and power.
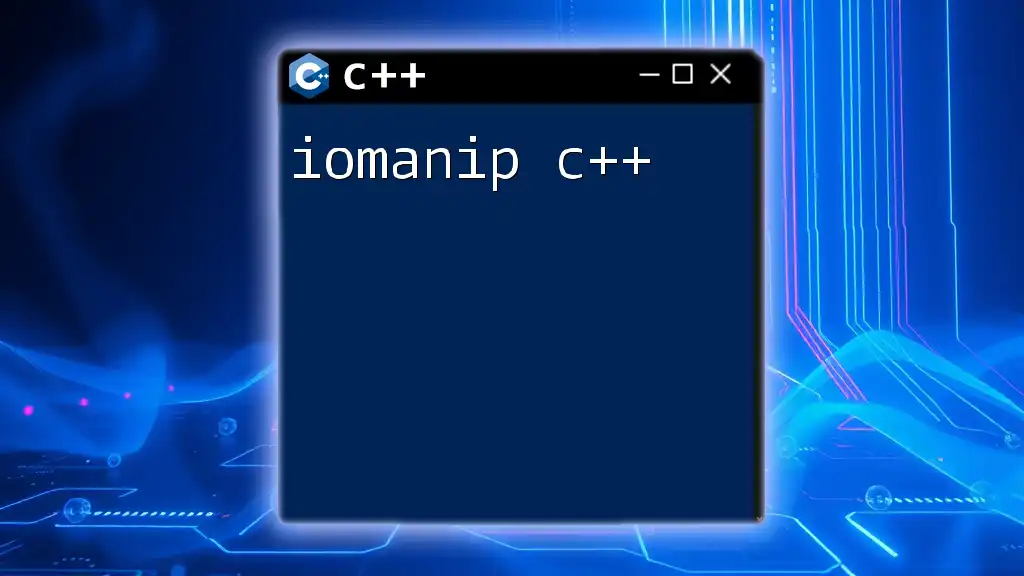
Advanced Topics in C++ String Tokenization
Custom Tokenizer Implementation
For specialized needs, creating a custom tokenizer class may be beneficial. A custom tokenizer can provide precisely defined behavior tailored to your specific requirements. Here’s a simple structure for such a class:
class CustomTokenizer {
public:
CustomTokenizer(const std::string &str, char delim) : str(str), delimiter(delim) {}
std::vector<std::string> tokenize() {
std::vector<std::string> tokens;
std::string token;
for (char ch : str) {
if (ch == delimiter) {
tokens.push_back(token);
token.clear();
} else {
token += ch;
}
}
if (!token.empty()) {
tokens.push_back(token);
}
return tokens;
}
private:
std::string str;
char delimiter;
};
This class encapsulates the tokenization logic, requiring only a string and a delimiter for instantiation, promoting ease of reuse and readability in your code.
Handling Edge Cases in Tokenization
Tokenization can encounter challenges, especially with edge cases such as:
- Empty Strings: Ensure your code can handle scenarios with no content gracefully.
- Multiple Delimiters: Design your tokenizer to manage consecutive delimiters, resulting in empty tokens.
Having a clear strategy to address these cases will help create robust tokenization logic that can withstand unexpected inputs.
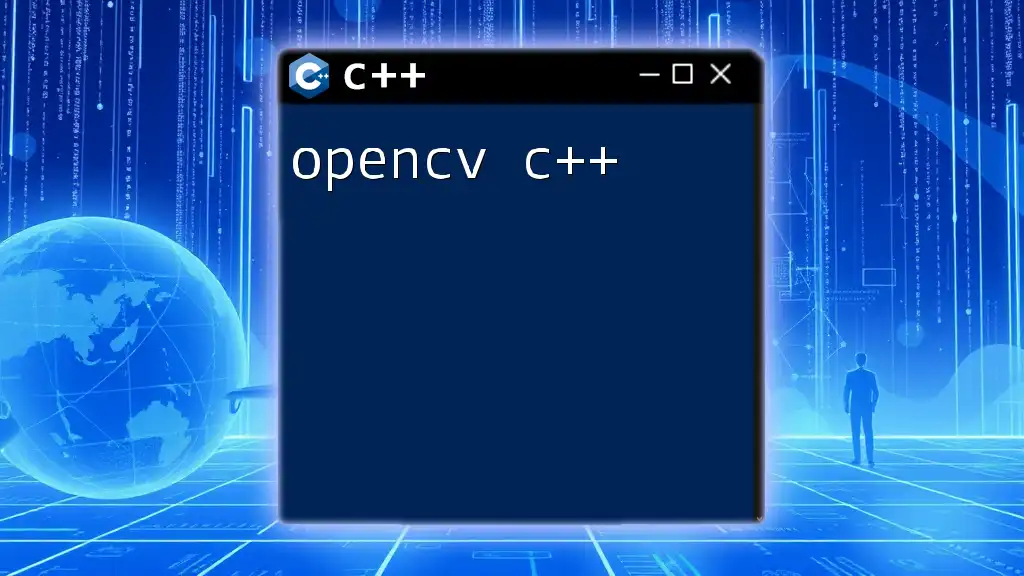
Common Pitfalls in Tokenizing Strings
Misunderstanding Delimiters
A common mistake when implementing tokenization is misjudging what delimiters should be used. Tokenization should be adaptable; sometimes, you may need multiple types of delimiters within your strings.
Performance Issues
While tokenization can be fast, poorly designed algorithms or using inefficient methods may introduce significant performance costs. Always analyze and optimize your tokenization logic to ensure it doesn’t become a bottleneck in your application.
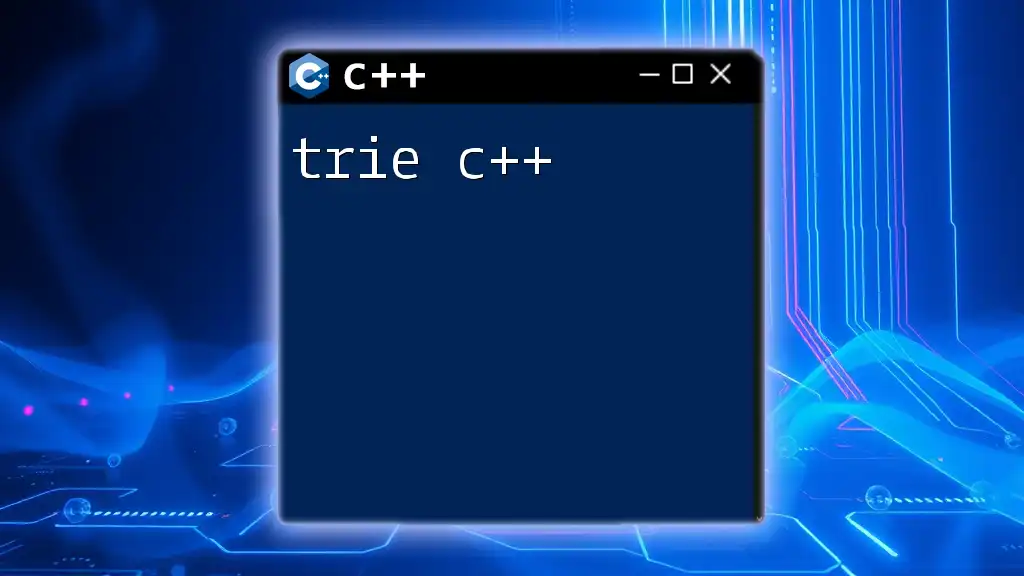
Conclusion on C++ String Tokenization
In summary, understanding how to effectively tokenize in C++ is crucial for any developer looking to manipulate strings and process data efficiently. From manual methods to leveraging the C++ Standard Library and popular libraries like Boost, you have various tools at your disposal to achieve effective string tokenization. Embrace these techniques, practice implementing them, and integrate them into your projects for enhanced functionality and user experience.
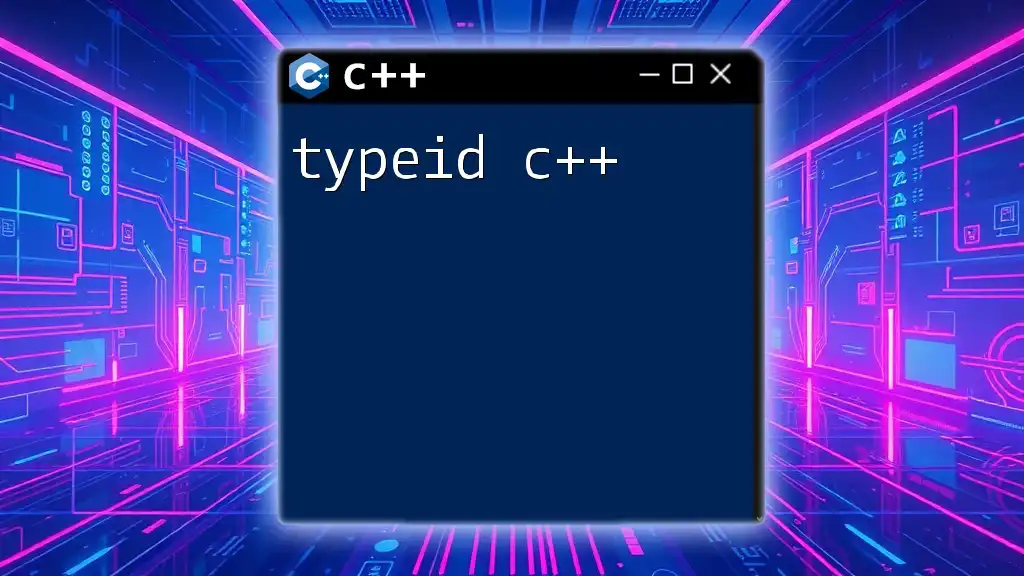
Additional Resources
To dive deeper into the world of C++ tokenization and string handling, consider exploring recommended books, articles, and engaging with community forums. Resources such as Stack Overflow and various C++ programming communities can provide valuable insights and answers to your tokenization challenges.