In C++, tokens are the smallest elements of a program, such as keywords, identifiers, literals, and operators, which are combined to form expressions and statements.
Here's a simple code snippet showcasing different types of tokens:
int main() {
int x = 5; // Keyword (int), identifier (x), literal (5), operator (=)
if (x > 0) { // Keyword (if), operator (>)
std::cout << "Positive number"; // Identifier (std::cout), operator (<<)
}
return 0; // Keyword (return)
}
What is a Token?
A token is a fundamental element of your C++ code. During the compilation process, the C++ compiler breaks down the source code into these smaller building blocks known as tokens. Each token has a specific meaning and role in the overall structure of the program. Understanding tokens in C++ is essential for both beginners and experienced programmers, as they are the basic components that contribute to code readability and functionality.
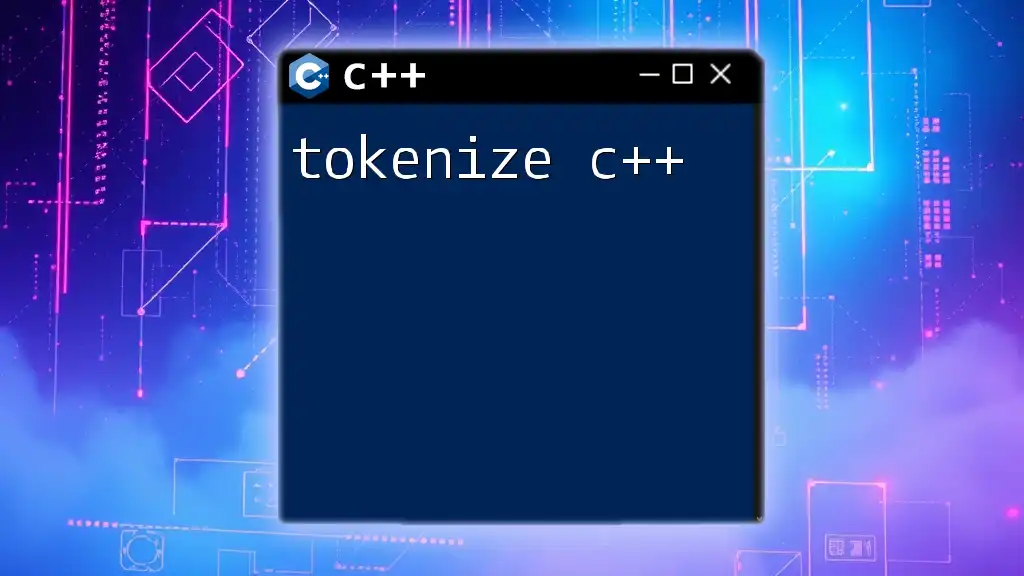
Types of C++ Tokens
Keywords
Keywords are reserved words in C++ that have special meanings. They are part of the syntax and cannot be used as identifiers. Examples include `int`, `return`, `if`, `else`, `while`, etc.
Here’s a simple code snippet that uses several keywords:
int main() {
if (true) {
return 0;
}
}
In this example, `int` and `return` are keywords that define the type of the main function and its return value, respectively.
Identifiers
Identifiers are names given to entities such as variables, functions, arrays, classes, etc., to differentiate them from one another. They are crucial for code organization and clarity.
When naming identifiers, keep the following rules in mind:
- Should only contain letters, digits, and underscores.
- Must begin with a letter or an underscore.
- Cannot be a keyword.
Example:
int myVariable;
In this case, `myVariable` is a valid identifier.
Literals
Literals are constant values that appear directly in your code, representing fixed data. There are several types of literals in C++:
- Integer literals: whole numbers, e.g., `42`.
- Floating-point literals: numbers with decimal points, e.g., `3.14`.
- Character literals: enclosed in single quotes, e.g., `'a'`.
- String literals: enclosed in double quotes, e.g., `"Hello, World!"`.
Example of different literals:
int num = 10; // Integer literal
float pi = 3.14f; // Floating-point literal
char letter = 'A'; // Character literal
std::string greeting = "Hi"; // String literal
Operators
Operators are special symbols that perform operations on operands. C++ includes several categories of operators, such as:
- Arithmetic operators (e.g., `+`, `-`, `*`, `/`)
- Relational operators (e.g., `==`, `!=`, `<`, `>`)
- Logical operators (e.g., `&&`, `||`, `!`)
Considering their use in a snippet:
int a = 5;
int b = 10;
int sum = a + b; // Using + (addition) operator
In this example, the `+` operator sums two integer values.
Punctuation
Punctuation characters play crucial roles in defining the structure of C++ code. They are used to separate tokens and influence the way code is interpreted by the compiler. Common punctuation marks used in C++ include:
- Semicolon (`;`) - indicates the end of a statement.
- Comma (`,`) - separates items in a list.
- Parentheses (`(` and `)`) - used in functions and allocations.
- Curly braces (`{` and `}`) - define blocks of code.
Example demonstrating punctuation:
#include <iostream>
using namespace std;
int main() {
cout << "Welcome to C++!"; // Semicolon terminates the statement
return 0; // Another statement termination
}
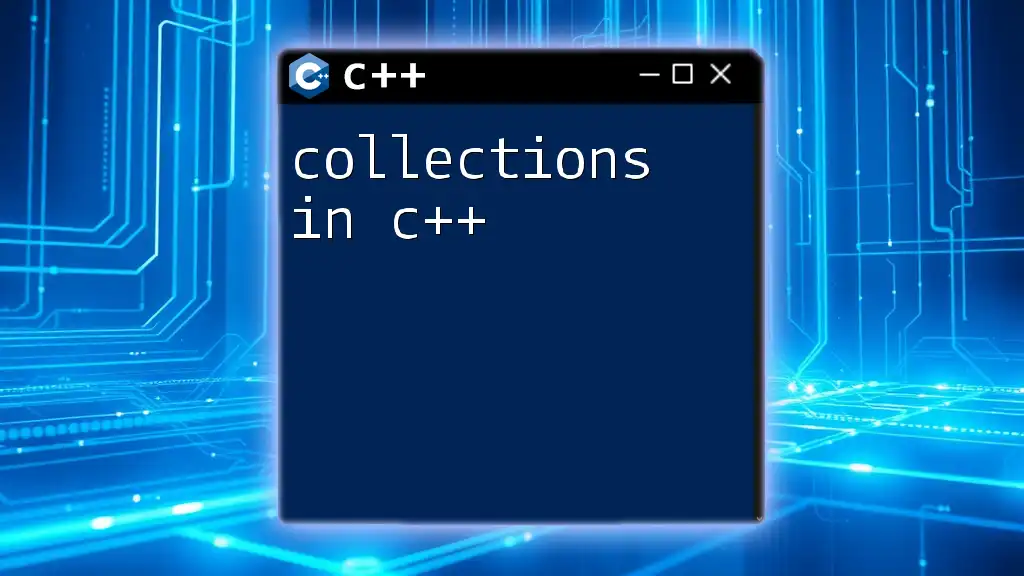
How Tokens are Processed
Tokens in C++ are processed during the compilation phase. The compilation of C++ code consists of several stages, with tokenization being one of the earliest. During this stage, a component called the lexer reads the source code and identifies each token based on predefined criteria.
Visualizing Tokens with an Example
Consider the following simple C++ code snippet:
int sum(int a, int b) {
return a + b;
}
Breaking it down into tokens yields:
- Keywords: `int` (function return type), `return`
- Identifiers: `sum`, `a`, `b`
- Operators: `+`
- Literals: (none in this case)
- Punctuation: `(`, `)`, `{`, `}`, `;`
Understanding these tokens aids in comprehending the structure and flow of the program, as each component plays a specific role.
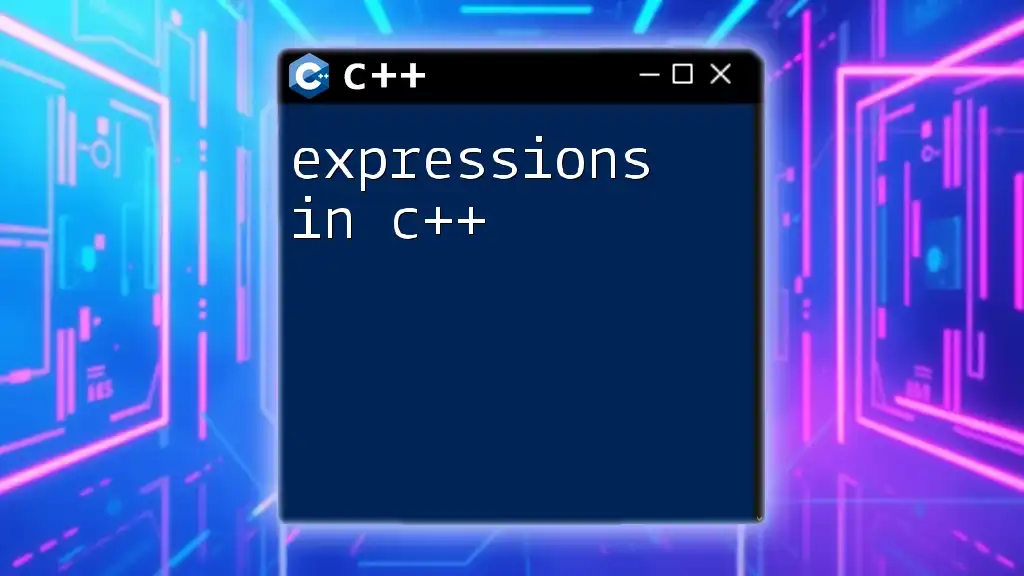
Best Practices for Using C++ Tokens
Naming Conventions for Identifiers
When creating identifiers, clarity and descriptiveness are paramount. Make use of meaningful names to improve readability. For instance, instead of using names like `x` or `y`, opt for names like `totalScore` or `userCount`.
Usage of Keywords
Avoid misuse of keywords; for example, do not attempt to redefine a keyword as an identifier. Misusing keywords can lead to confusion and compilation errors. Always refer to the C++ documentation if uncertain.
Effective Use of Operators and Punctuations
Use operators responsibly. Combining multiple operators in one expression can lead to confusion. Instead, break down complex operations into simpler parts. Proper use of punctuation can greatly enhance code readability.
Example of a well-structured arithmetic operation:
int totalItems = 5; // Using descriptive identifiers
int additionalItems = 10;
int finalCount = totalItems + additionalItems; // Clear and concise
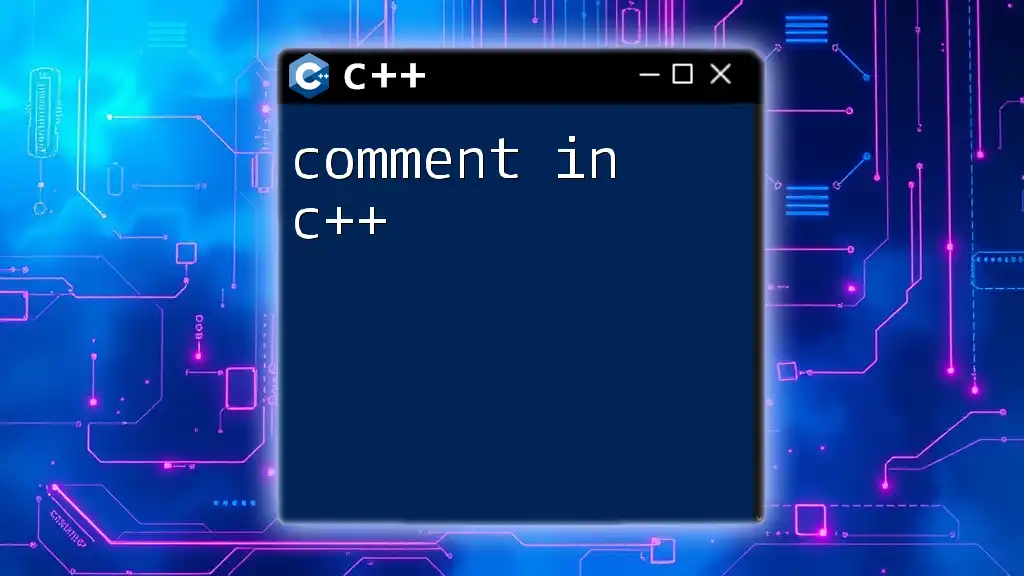
Common Errors Related to Tokens in C++
Compilation Errors due to Misused Tokens
Misusing tokens can lead to common compilation errors. A typical example is forgetting to terminate statements with a semicolon, leading to an error message that points to the next line as the problem.
Example of a common mistake:
int main()
return 0 // Missing semicolon
}
Tips for Avoiding Token-Related Mistakes
You can significantly reduce token-related errors by consistently reviewing your code. Look for tools that help catch semantic errors as well, such as linters, which can help streamline your writing process.
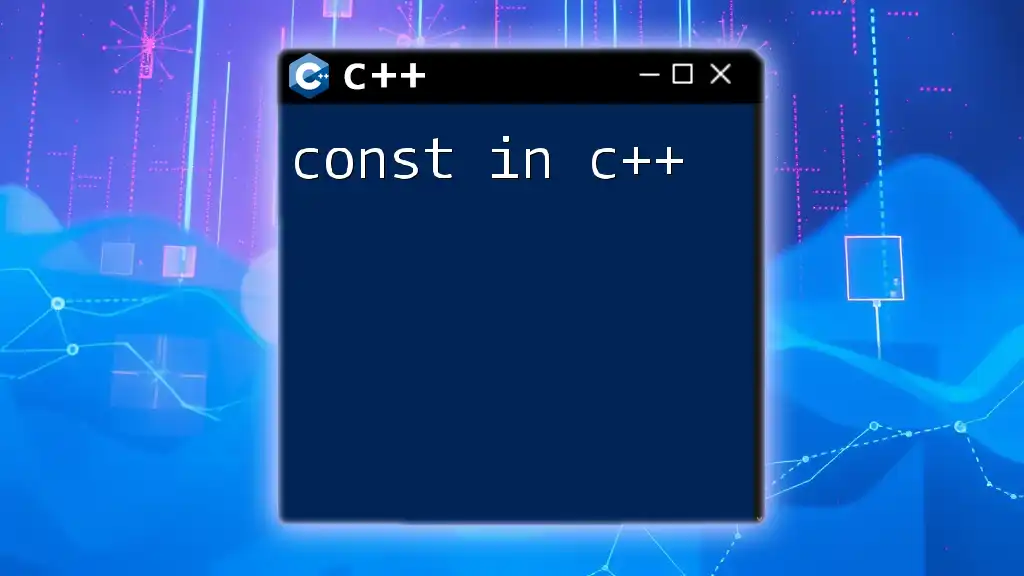
Conclusion
Understanding tokens in C++ is crucial to writing effective, readable, and functional code. By familiarizing yourself with the types of tokens, how they are processed, and best practices for their usage, you empower yourself as a programmer. Always remember that the clarity of your code directly correlates with the understandability and maintainability of your projects. Embrace the importance of tokens and see how they make your C++ programming journey more enriching.
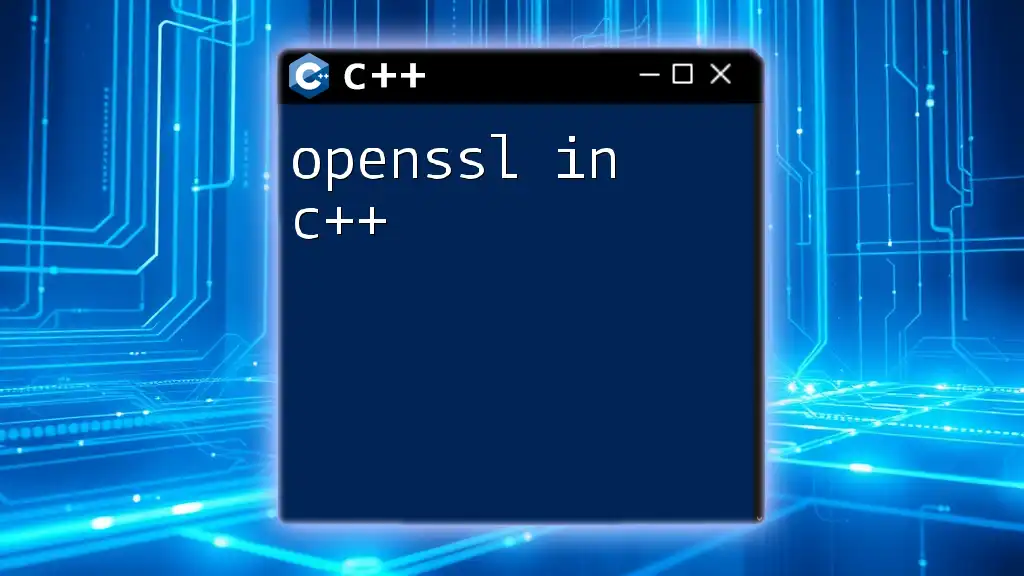
Additional Resources
To further enhance your understanding of tokens in C++, consider exploring recommended books, online courses, and engaging with community forums dedicated to C++ learning. These resources will provide you with invaluable insights and additional materials to elevate your coding skills!