In C++, you can represent colors using the RGB color model by defining them through the combination of red, green, and blue values, often utilized in graphics programming or user interface design.
Here's a code snippet demonstrating how to define and display a color in C++:
#include <iostream>
#include <string>
struct Color {
int red;
int green;
int blue;
};
void displayColor(const Color& color) {
std::cout << "Color(RGB): (" << color.red << ", " << color.green << ", " << color.blue << ")" << std::endl;
}
int main() {
Color myColor = {255, 0, 0}; // Red color
displayColor(myColor);
return 0;
}
Understanding C++ Color Codes
What are Color Codes?
Color codes are vital in specifying the color representation in programming. In C++, colors can be defined using various systems, including RGB (Red, Green, Blue), HEX (Hexadecimal), and CMYK (Cyan, Magenta, Yellow, Black) models. These codes allow developers to easily convey visual information through software applications, enhancing user experiences and improving UI designs.
Basic C++ Color Codes
C++ offers several predefined color codes, particularly when outputting colors to the console. ANSI color codes are commonly used for terminal applications. They are sequences of characters that change the text's foreground and background colors as seen in terminals that support ANSI.
Here’s an example code snippet demonstrating how to display colored text in the console using ANSI escape codes:
#include <iostream>
int main() {
std::cout << "\033[1;31mThis text is red!\033[0m" << std::endl;
std::cout << "\033[1;32mThis text is green!\033[0m" << std::endl;
return 0;
}
In the above example, `\033[1;31m` sets the text color to red and `\033[0m` resets it to the default color.
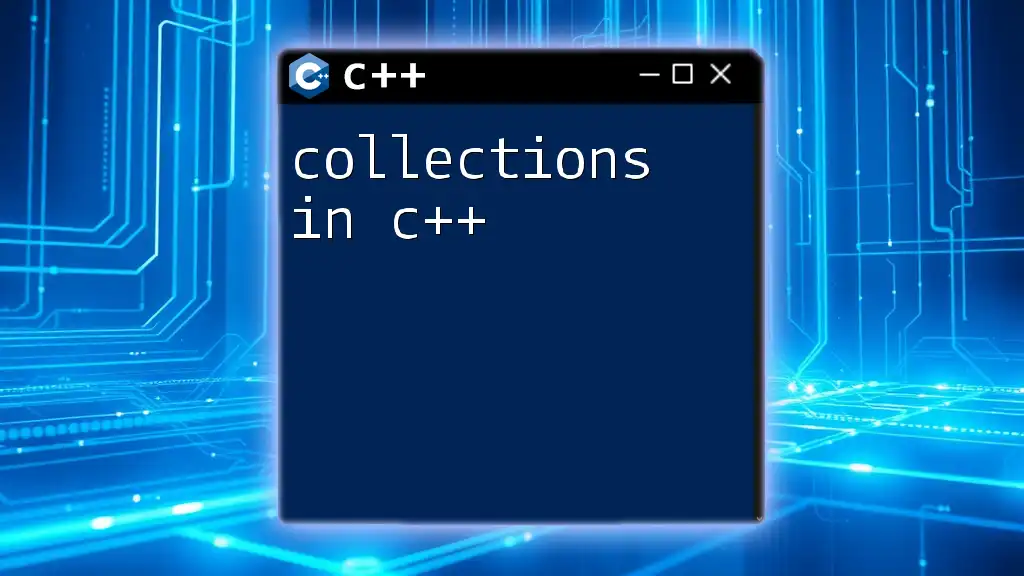
Implementing Colors in C++
Setting up the Environment
To work with colors in C++, ensure your programming environment supports output formatting. Libraries like ncurses can extend your capabilities for color management in console-based applications, allowing for a richer user interface.
Using RGB Color Model
The RGB color model lets you define colors based on the combination of red, green, and blue light. Each color’s intensity can range from 0 to 255. Below is an example that outputs a simple colored text in the console:
#include <iostream>
void printColor(int r, int g, int b) {
std::cout << "\033[38;2;" << r << ";" << g << ";" << b << "mThis text is in RGB color\033[0m" << std::endl;
}
int main() {
printColor(255, 0, 0); // Red
printColor(0, 255, 0); // Green
printColor(0, 0, 255); // Blue
return 0;
}
This code snippet allows for custom RGB colors by adjusting the red, green, and blue values passed to the function.
Utilizing HEX Color Codes
HEX color codes are another popular format for color representation. A HEX code is a 6-digit combination of numbers and letters defined with a `#` sign. Each pair of digits corresponds to the red, green, and blue components.
Converting a HEX color code to RGB can be done using the following function:
#include <iostream>
#include <sstream>
void hexToRGB(const std::string &hex) {
int r, g, b;
std::stringstream ss;
ss << std::hex << hex.substr(1); // Skip the '#' sign
ss >> r >> g >> b;
std::cout << "Red: " << r << " Green: " << g << " Blue: " << b << std::endl;
}
int main() {
hexToRGB("#FF5733"); // Example HEX color
return 0;
}
In this example, the function extracts the color components from a HEX code and outputs their corresponding RGB values.
Applying Colors to Graphics
C++ also allows for color application in graphics. Libraries such as SFML (Simple and Fast Multimedia Library) and SDL (Simple DirectMedia Layer) make it easy to incorporate colors into graphical applications. Here’s a basic example using SFML to draw colored shapes:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "Colored Shapes");
sf::CircleShape circle(50);
circle.setFillColor(sf::Color::Green);
circle.setPosition(200, 200);
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.draw(circle);
window.display();
}
return 0;
}
This code creates a window and draws a green circle, showcasing how to handle colors visually in a simple graphical application.
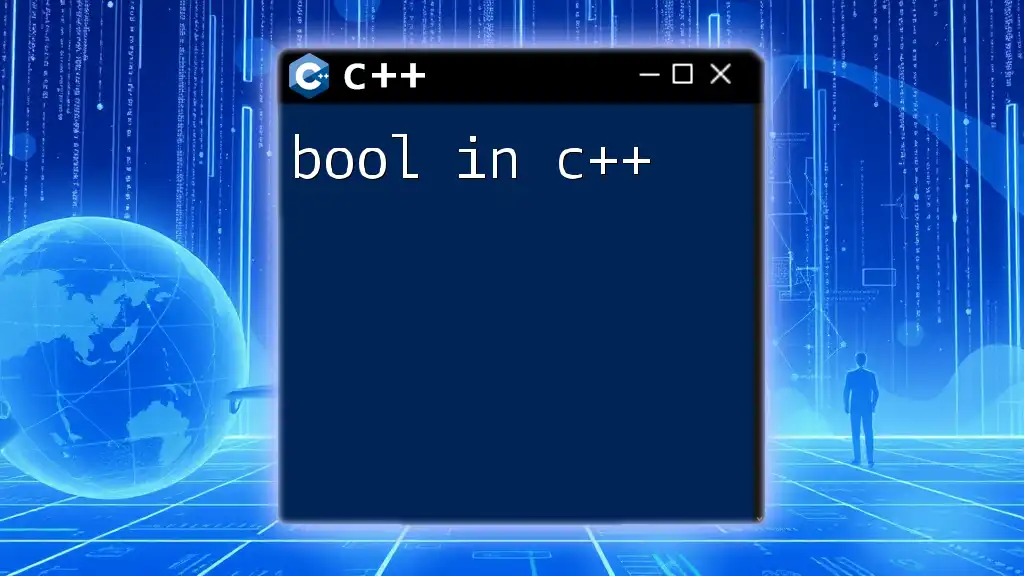
Creating Custom Colors in C++
Defining Custom Color Functions
Creating your own functions allows you to streamline color manipulations. For instance, you can define a function to create colors based on a specific formula:
#include <iostream>
struct Color {
int r, g, b;
};
Color createColor(int r, int g, int b) {
Color color = {r, g, b};
return color;
}
void printColor(const Color &color) {
std::cout << "RGB(" << color.r << ", " << color.g << ", " << color.b << ")" << std::endl;
}
int main() {
Color myColor = createColor(150, 75, 0);
printColor(myColor);
return 0;
}
This snippet defines a `Color` struct and functions for creating and printing a color, demonstrating modular coding and improving code readability.
Color Blending Techniques
Color blending involves combining two or more colors to create new shades. The blending formula can be as simple as averaging the RGB values of two colors.
Here’s an example blending two colors:
Color blendColors(const Color &c1, const Color &c2) {
Color blended;
blended.r = (c1.r + c2.r) / 2;
blended.g = (c1.g + c2.g) / 2;
blended.b = (c1.b + c2.b) / 2;
return blended;
}
int main() {
Color red = {255, 0, 0};
Color blue = {0, 0, 255};
Color purple = blendColors(red, blue);
printColor(purple); // Outputs RGB(127, 0, 127)
return 0;
}
In this example, the `blendColors` function takes two `Color` instances and averages their RGB values to create a blended color.
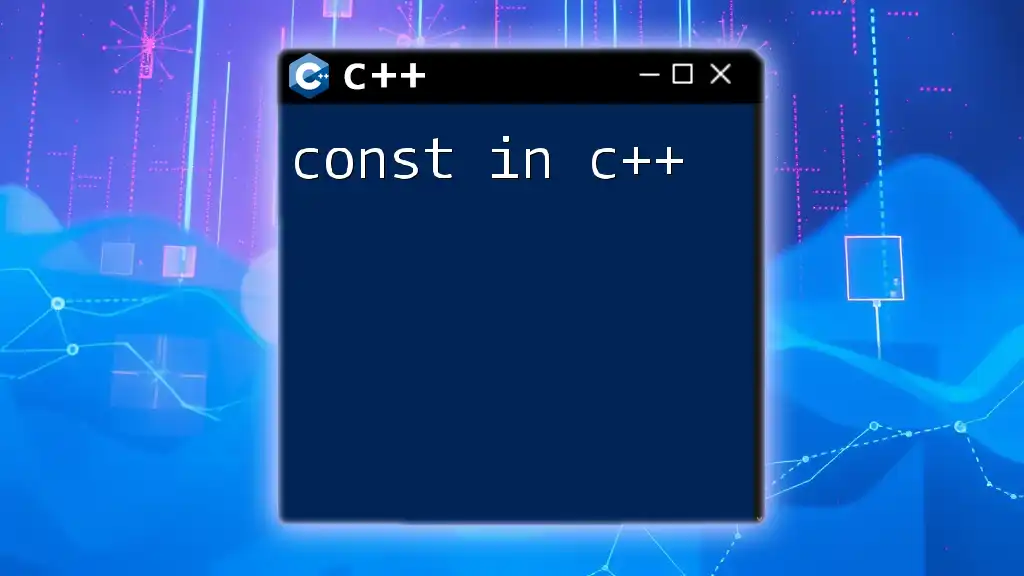
Advanced Color Management
Color Palettes in C++
Color palettes help manage colors for your applications and enhance overall design consistency. In C++, you can create an array or vector to store and manipulate these palettes easily.
Here’s an example of creating a simple color palette:
#include <iostream>
#include <vector>
struct Color {
int r, g, b;
};
void displayPalette(const std::vector<Color> &palette) {
for (const auto &color : palette) {
std::cout << "Color: RGB(" << color.r << ", " << color.g << ", " << color.b << ")" << std::endl;
}
}
int main() {
std::vector<Color> palette = {
{255, 0, 0}, // Red
{0, 255, 0}, // Green
{0, 0, 255}, // Blue
};
displayPalette(palette);
return 0;
}
This program defines a vector of `Color` instances representing a palette and displays each color.
Color Accessibility Considerations
Ensuring that your application is accessible for users with color vision deficiencies is crucial for broad usability. Use tools like contrast checkers to verify the visibility of your color combinations. Implementing contrasting colors can significantly enhance the readability of your applications.
Here’s a quick example demonstrating how to test color contrast:
bool isAccessible(const Color &bg, const Color &fg) {
int bgLuminance = 0.2126 * bg.r + 0.7152 * bg.g + 0.0722 * bg.b;
int fgLuminance = 0.2126 * fg.r + 0.7152 * fg.g + 0.0722 * fg.b;
return abs(bgLuminance - fgLuminance) > 128; // Basic contrast check
}
int main() {
Color background = {255, 255, 255}; // White
Color foreground = {0, 0, 0}; // Black
if (isAccessible(background, foreground)) {
std::cout << "The color combination is accessible." << std::endl;
} else {
std::cout << "Adjust your colors for better accessibility." << std::endl;
}
return 0;
}
This code provides a simple check for color contrast based on luminance and emphasizes the importance of color accessibility.
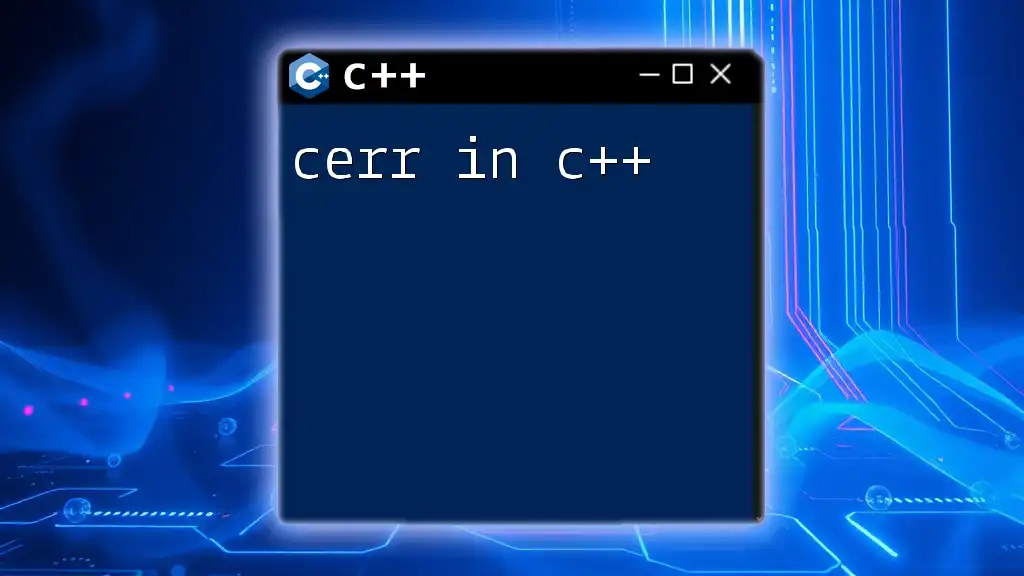
Conclusion
Exploring colors in C++ opens up a world of possibilities for enhancing user interfaces and creating visually appealing applications. Through understanding color codes, implementing them effectively, and considering accessibility, you can create dynamic and colorful applications that are both functional and engaging.
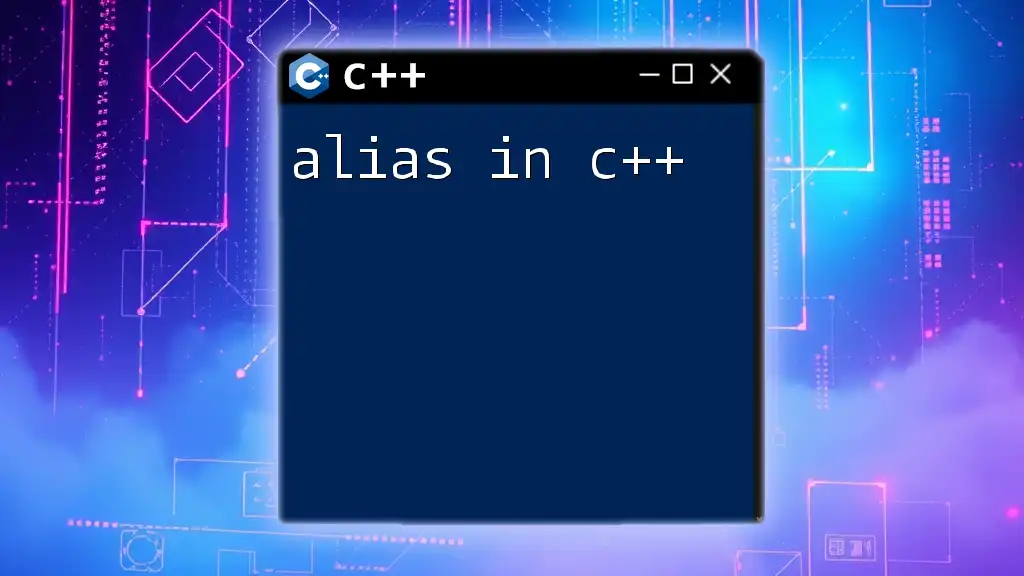
Further Reading and Resources
For further learning, explore graphics libraries like SFML and SDL. Books on UI/UX design can greatly enhance your understanding of effective color application. Online courses and community forums can also provide additional insights and troubleshooting assistance as you delve deeper into colors in C++.