Collections in C++ are data structures that allow you to store and manage groups of elements, such as arrays, vectors, lists, and maps, enabling efficient data manipulation and retrieval.
#include <iostream>
#include <vector>
#include <map>
int main() {
// Using a vector to store a collection of integers
std::vector<int> numbers = {1, 2, 3, 4, 5};
// Using a map to store key-value pairs
std::map<std::string, int> ages = {{"Alice", 30}, {"Bob", 25}};
// Output the contents of the vector
std::cout << "Numbers: ";
for (int number : numbers) {
std::cout << number << " ";
}
std::cout << std::endl;
// Output the contents of the map
std::cout << "Ages: " << std::endl;
for (const auto& pair : ages) {
std::cout << pair.first << " is " << pair.second << " years old." << std::endl;
}
return 0;
}
Understanding Collections
Definition and Importance
Collections in C++ refer to a group of data structures that allow the grouping and manipulation of data types efficiently. They serve as the backbone of data management in most applications, offering a systematic way to store, retrieve, and handle multiple data items.
Using collections effectively can drastically improve the efficiency of your programs. They offer critical functionalities like searching, sorting, and data manipulation, helping developers manage complexity and increase productivity.
Types of Collections in C++
The Standard Template Library (STL) in C++ includes various collection types that are versatile and well-optimized for performance. Understanding each type of collection will help you choose the right one for your needs.
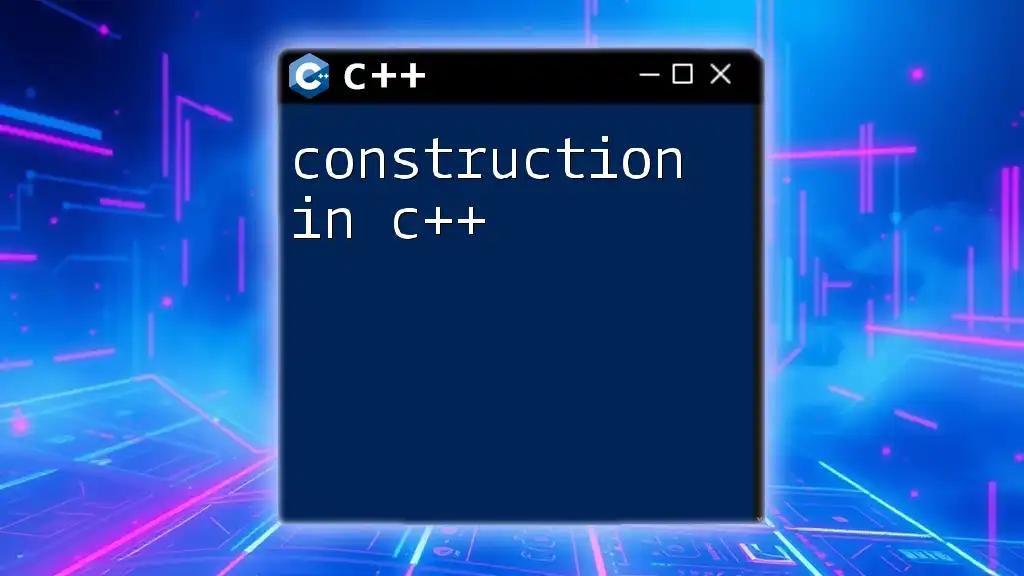
Overview of C++ Collection Types
Arrays
Arrays are one of the simplest forms of collections in C++. They are used to store fixed-size sequential collections of elements of the same type.
Key Characteristics:
- Fixed Size: The size of an array must be defined at compile time and cannot change.
- Homogeneous: All elements in an array are of the same type.
How to Use Arrays
To declare an array in C++, you specify the data type followed by the array name and size in square brackets:
int arr[5] = {10, 20, 30, 40, 50};
You can access elements using their index:
std::cout << arr[0]; // Outputs 10
Limitations: Arrays do not provide methods to change their size once created, making them less versatile than other collection types.
Vectors
In C++, vectors are dynamic arrays. Unlike regular arrays, vectors can resize themselves automatically when elements are added or removed, making them a crucial tool for developers.
Benefits of Using Vectors:
- Dynamic Size: Vectors can grow and shrink as needed.
- Standard Functionality: They come with built-in functions for ease of use.
Common Operations on Vectors
Creating a vector is straightforward using the `std::vector` template:
#include <vector>
std::vector<int> numbers;
numbers.push_back(10);
numbers.push_back(20);
You can iterate over a vector using a range-based for loop:
for(int num : numbers) {
std::cout << num << " "; // Outputs 10 20
}
Lists
Lists are another type of container in C++, structured as a doubly linked list. This means each element points to both the next and the previous elements.
Advantages of Lists over Arrays:
- Dynamic Size: Like vectors, lists can also expand and contract.
- Efficient Insertions/Deletions: Operations at both ends and in-between nodes are efficient.
Using Lists
You can include lists from the STL:
#include <list>
std::list<int> myList;
myList.push_back(5);
myList.push_front(1);
To iterate through a list, you can use iterators:
for(auto it = myList.begin(); it != myList.end(); ++it) {
std::cout << *it << " "; // Outputs 1 5
}
Deques
Deques (double-ended queues) are versatile collections that allow insertion and deletion from both ends.
When to Use Deques
Deques serve as a flexible alternative to both arrays and lists. They are particularly useful when you need to manipulate both ends of a collection frequently.
Basic Operations with Deques
#include <deque>
std::deque<int> myDeque;
myDeque.push_front(2);
myDeque.push_back(4);
You can access elements similarly to vectors:
std::cout << myDeque.front(); // Outputs 2
std::cout << myDeque.back(); // Outputs 4
Sets
Sets are collections that store unique elements; they automatically eliminate duplicates and maintain order.
When to Use Sets
Sets are particularly helpful when you need to manage unique items and perform efficient lookups.
Working with Sets
To use sets, include the appropriate header:
#include <set>
std::set<int> mySet;
mySet.insert(1);
mySet.insert(2);
mySet.insert(1); // Will not insert duplicate
You can iterate over a set like this:
for(int num : mySet) {
std::cout << num << " "; // Outputs 1 2
}
Maps
Maps are associative arrays that store key-value pairs. They function similarly to dictionaries in Python and allow fast access based on keys.
Use Cases for Maps
Maps are ideal for scenarios where you need to relate one piece of data to another, such as counting the occurrences of items.
Example of Map Operations
#include <map>
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
Accessing a value is as simple as:
std::cout << ageMap["Alice"]; // Outputs 30
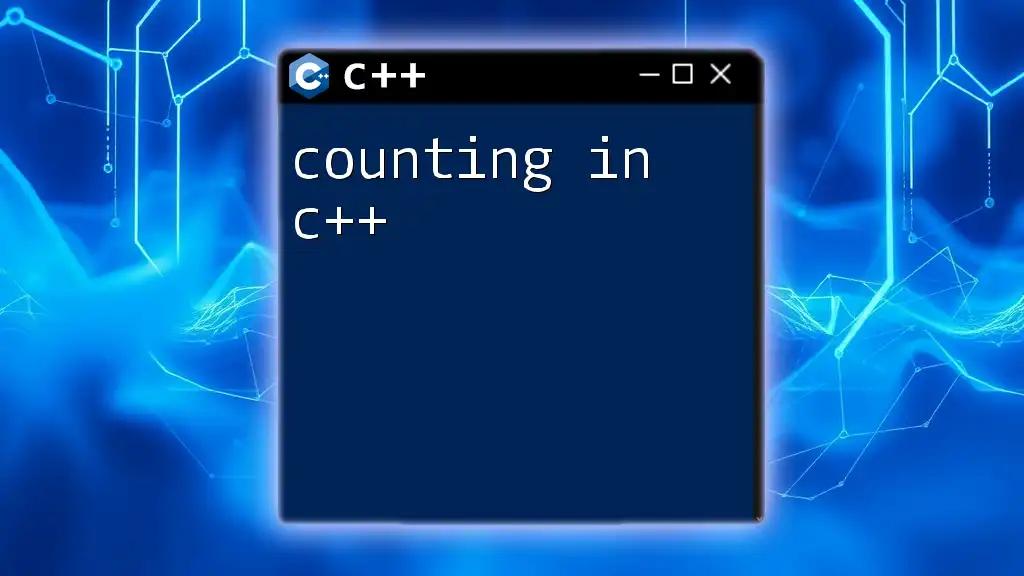
Advanced Collection Features
Iterators
Iterators are objects that provide a means to traverse collections. They abstract the details of how the collection stores data, allowing you to work seamlessly with varied types of collections.
For example, you can use iterators with vectors:
std::vector<int>::iterator it;
for (it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " ";
}
Algorithms with Collections
The STL provides various algorithms that can be applied to collections such as `sort`, `find`, and `accumulate`.
Example of Using Algorithms on Collections
Sorting a vector is straightforward:
#include <algorithm>
std::sort(numbers.begin(), numbers.end());

Best Practices for Using Collections
Choosing the Right Collection
Choosing the appropriate collection type can drastically affect the performance of your application. Consider aspects such as time complexity, memory usage, and the specific requirements of your project.
Performance Considerations
- Arrays: Fast access, but limited in flexibility.
- Vectors: Great for dynamic sizes and frequent insertions.
- Lists: Optimal for frequent insertions and deletions.
- Deques: Ideal when you need both ends modified.
- Sets: Best for unique collections with fast lookups.
- Maps: Perfect for key-value associations.
Managing Memory
C++ collections often handle memory automatically, especially with containers like vectors and lists. Understanding how each type manages memory (e.g., dynamic vs. static) is vital for optimizing performance and avoiding memory leaks.
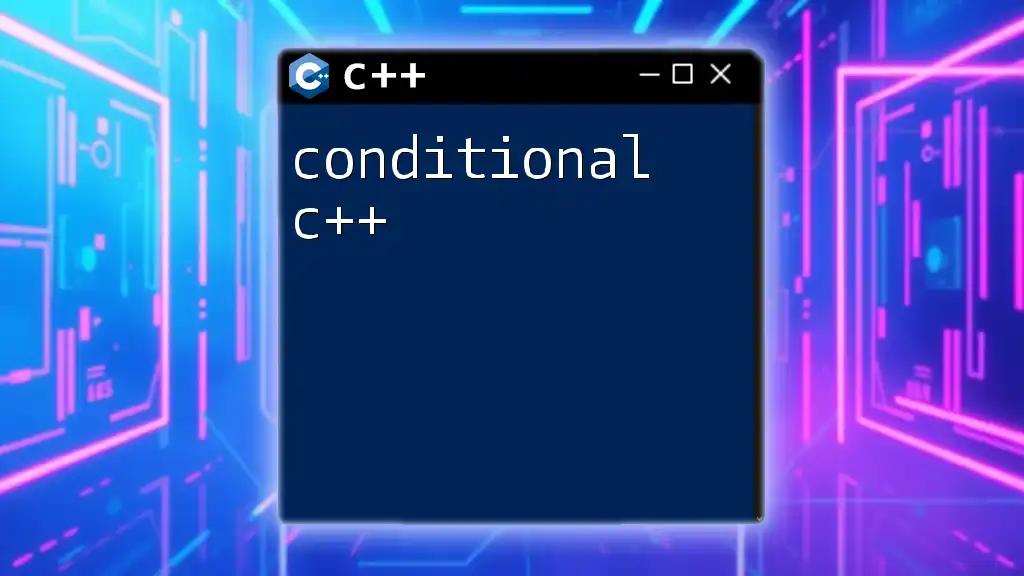
Conclusion
In conclusion, understanding collections in C++ is crucial for effective programming. By leveraging arrays, vectors, lists, deques, sets, and maps, you can manipulate data efficiently and build robust applications. Remember to choose the right collection type based on your specific needs and to practice implementing these concepts in your projects.

Additional Resources
For further learning, consider exploring books on C++ programming and engaging with online tutorials or communities. These resources can provide deeper insights and support as you continue your coding journey.