In C++, garbage collection is not built into the language but can be managed through manual memory management techniques, such as using smart pointers to automatically reclaim memory when it is no longer in use.
#include <iostream>
#include <memory>
int main() {
std::unique_ptr<int> ptr = std::make_unique<int>(42); // Automatic memory management
std::cout << *ptr << std::endl; // Outputs: 42
return 0; // Memory is automatically freed when ptr goes out of scope
}
Understanding Memory Management in C++
The Stack and the Heap
In C++, memory allocation occurs primarily in two areas: the stack and the heap. Understanding how these memory areas work is crucial for effective memory management.
-
Stack Memory: This is where local variables are stored, and it operates on a last-in-first-out (LIFO) principle. Memory allocation on the stack is efficient and automatically managed, which means that once a function call is completed, the memory it used is automatically freed. However, the size of stack memory is usually limited and can lead to stack overflow if too much memory is being used.
-
Heap Memory: Unlike stack memory, heap memory is managed manually. You allocate memory using operators like `new` and you must free it with `delete`. This allows for greater flexibility but increases the risk of memory leaks if you're not careful.
Manual vs. Automatic Memory Management
C++ is primarily a manual memory management language, meaning developers are responsible for handling memory allocation and deallocation. To allocate memory, you typically use:
int* ptr = new int; // Allocating memory on the heap
*ptr = 10; // Using the allocated memory
delete ptr; // Freeing the allocated memory
On the other hand, languages like Java and Python provide automatic memory management through built-in garbage collection mechanisms, easing some of the burdens on developers but also incorporating their own limitations.
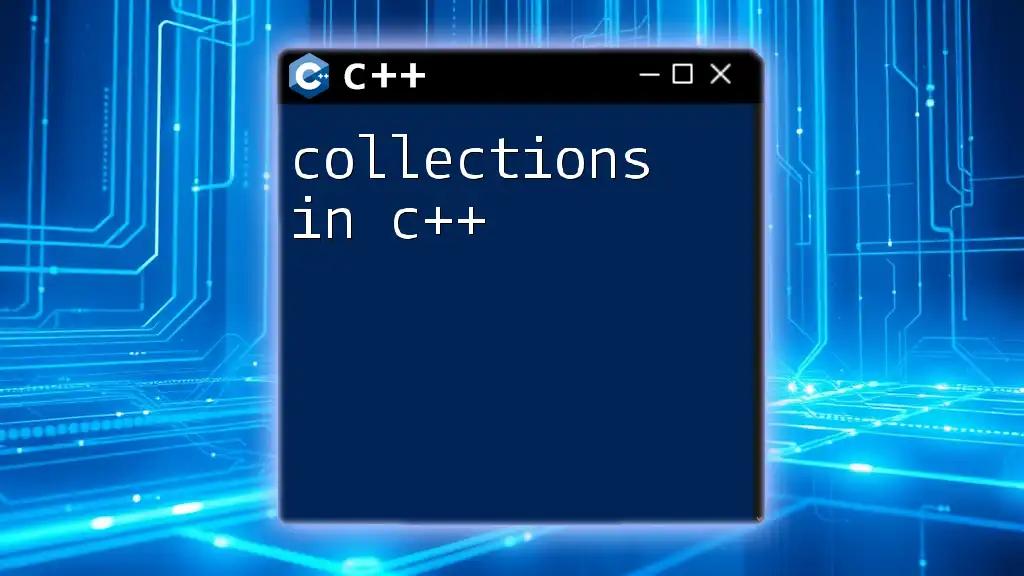
What is Garbage Collection?
How Garbage Collection Works
Garbage collection refers to the automatic process of reclaiming memory that is no longer in use, thus preventing memory leaks. It improves application stability and keeps memory consumption in check. There are two primary methods of garbage collection: reference counting and tracing garbage collection.
-
Reference Counting: This method involves tracking the number of references to each object in memory. When the count drops to zero, the memory can be freed.
class RefCounted { int count = 0; public: void addRef() { ++count; } void release() { if (--count == 0) delete this; } };
The key advantage here is simplicity; however, reference counting can struggle with circular references, where two objects reference each other, preventing their memory from being freed.
Limitations of Garbage Collection in C++
While garbage collection can be beneficial, it comes with its share of limitations:
- Performance Impact: Garbage collection pauses can affect performance, especially in real-time applications.
- Unpredictable Timing: The exact moment when garbage collection occurs can be unpredictable, which complicates resource management.
- Memory Leaks: Despite automated mechanisms, poorly designed programs can still suffer from memory leaks if they create reference loops or fail to release resources appropriately.

C++ Garbage Collection Mechanisms
Smart Pointers in C++
C++ offers smart pointers, which provide automated memory management to help mitigate many challenges associated with manual memory management.
-
unique_ptr: A smart pointer that ensures single ownership of an object. When the `unique_ptr` goes out of scope, the memory is automatically released.
std::unique_ptr<int> up(new int(10)); // Memory is automatically deleted when up goes out of scope
-
shared_ptr: This type of smart pointer allows multiple owners of a single resource. It uses a reference count to manage the lifetime of the resource.
std::shared_ptr<int> sp1(new int(10)); std::shared_ptr<int> sp2 = sp1; // Both sp1 and sp2 can access the resource
-
weak_ptr: This smart pointer prevents strong ownership from causing circular references. It allows access to the referenced object only as long as there is at least one `shared_ptr` in existence.
std::shared_ptr<Node> parent = std::make_shared<Node>("Parent"); std::weak_ptr<Node> child = parent; // Prevents strong circular reference
Third-party Libraries for Garbage Collection
There are external libraries that offer garbage collection features for C++. One popular choice is the Boehm-Demers-Weiser Garbage Collector. This provides a conservative garbage collection approach, allowing automatic memory management without significantly altering existing code.
Integration is straightforward:
#include <gc.h>
void* allocateMemory(size_t size) {
return GC_malloc(size); // Allocating memory using Boehm GC
}
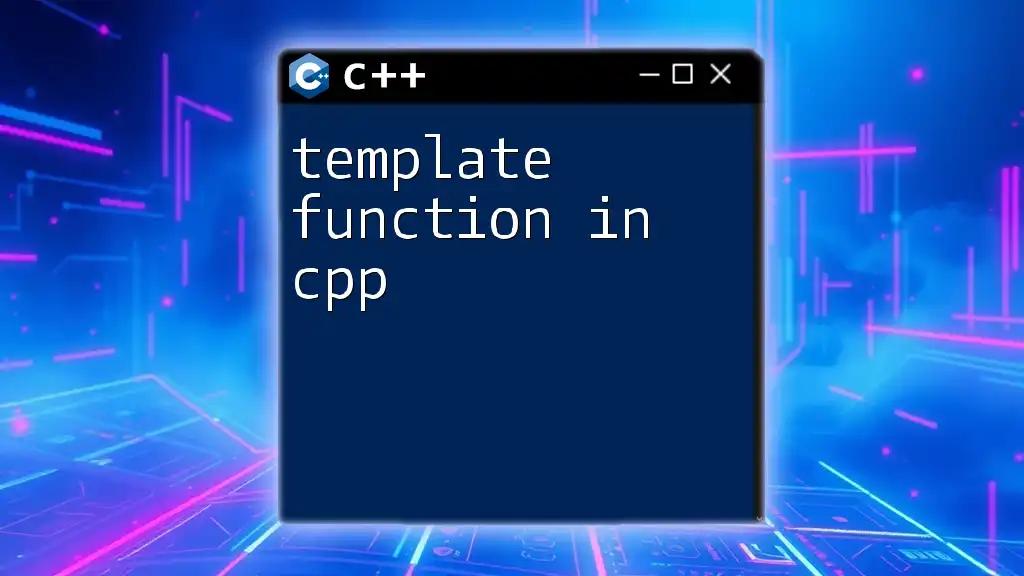
Best Practices for Memory Management in C++
Leveraging RAII (Resource Acquisition Is Initialization)
RAII is a design principle in C++ where resources are tied to object lifetime. It ensures that resources are released as soon as an object goes out of scope, effectively preventing memory leaks.
Minimizing Use of Raw Pointers
To optimize memory management, it is advisable to limit the use of raw pointers. Instead, leverage smart pointers whenever possible to reduce the margin for error and enhance safety. Avoiding raw pointers minimizes risks associated with memory management, including leaks, double deletions, and dangling pointers.

Conclusion
Understanding garbage collection in C++ is essential for effective memory management. By leveraging smart pointers, adhering to best practices, and accepting the limitations of manual management, developers can optimize their applications and ensure stability and performance. Proper memory management is not just a technical necessity; it shapes the overall robustness of your software. Emphasizing these principles will lead to cleaner, more maintainable code that allows your projects to thrive in the long run.

Further Reading and Resources
To continue your journey in mastering C++ memory management, refer to the following resources:
- C++ Reference Documentation
- Advanced C++ Programming Books
- Online courses on C++ Memory Management Techniques and Best Practices
Dive into these materials and reinforce your understanding of how effective garbage collection and memory management can elevate your C++ skills!