Recursion in C++ is a programming technique where a function calls itself to solve a problem by breaking it down into smaller, more manageable sub-problems.
#include <iostream>
using namespace std;
int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
int main() {
int number = 5;
cout << "Factorial of " << number << " is " << factorial(number) << endl;
return 0;
}
Understanding Recursion: An Overview
What is Recursion?
Recursion is a programming technique where a function calls itself in order to solve a problem. This method is particularly effective for problems that can be broken down into smaller, more manageable sub-problems. A common analogy for recursion involves navigating a maze — at each decision point, the solver explores one path at a time, retracing steps if necessary, until the exit is found.
Importance of Recursion in C++
Recursion plays a significant role in many applications of C++. It allows programmers to write cleaner, more readable code that can tackle complex problems. Recursive algorithms are often seen in scenarios such as:
- Tree traversals (depth-first search)
- Mathematical computations (factorials, Fibonacci numbers)
- Combinatorial problems (subset generation, permutations)
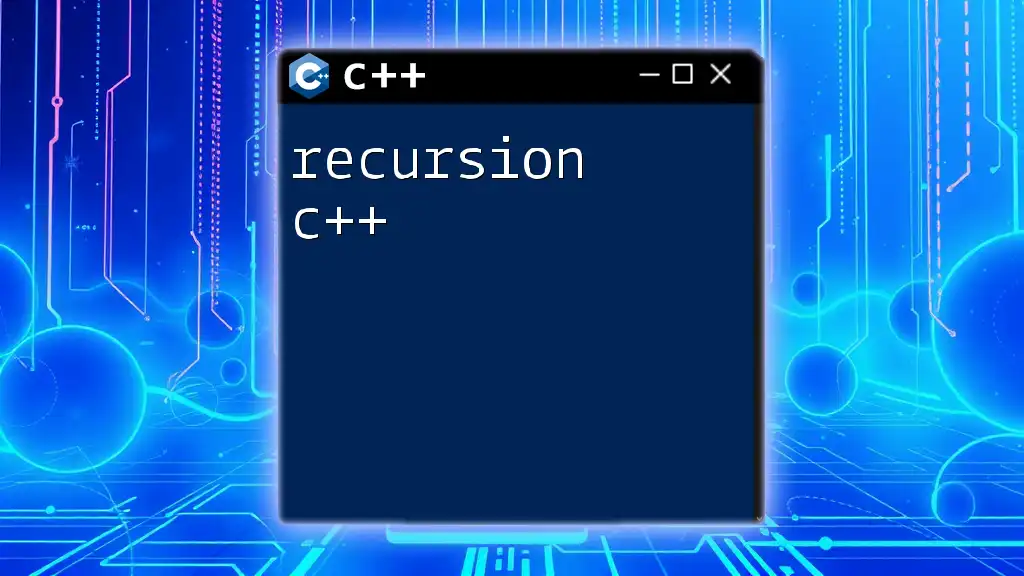
The Mechanics of Recursion
How Recursion Works
At its core, recursion relies on two fundamental concepts: base case and recursive case. The base case serves as a stopping condition for the recursive function, preventing it from calling itself indefinitely. On the other hand, the recursive case invokes the function again, narrowing down the problem with each call.
When a recursive function is executed, it utilizes stack memory. Each function call creates a new layer on the stack, preserving variables and state information. Once a base case is reached, the stack begins to unwind, returning control to the previous function calls.
Types of Recursive Functions
Direct Recursion
Direct recursion occurs when a function calls itself directly. This is the most common form found in recursive algorithms. For example, calculating the factorial of a number can be achieved through direct recursion.
#include <iostream>
using namespace std;
int factorial(int n) {
if (n <= 1) {
return 1; // Base case
} else {
return n * factorial(n - 1); // Recursive case
}
}
Indirect Recursion
Indirect recursion takes place when a function calls another function, which in turn calls the first function back. This creates a cycle that can be just as effective. Here’s a simple example:
#include <iostream>
using namespace std;
void funcA(int n);
void funcB(int n);
void funcA(int n) {
if (n > 0) {
cout << n << " ";
funcB(n - 1); // Calls funcB
}
}
void funcB(int n) {
if (n > 0) {
cout << n << " ";
funcA(n - 1); // Calls funcA
}
}
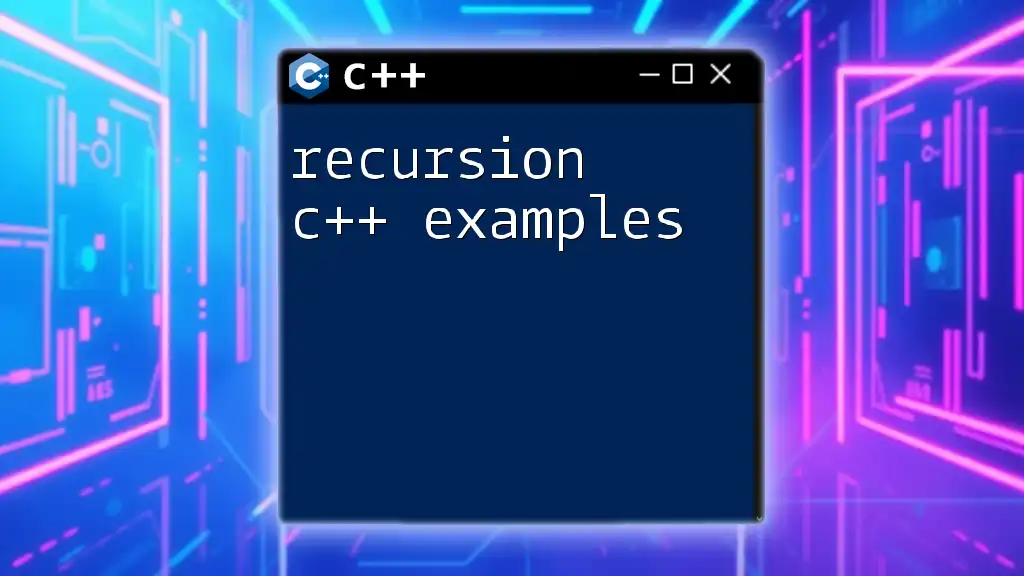
Writing a Recursive Function in C++
Structure of a Recursive Function
A recursive function typically consists of:
- A function signature that defines its parameters and return type.
- A base case to stop recursion.
- A recursive case to reduce the problem.
The following snippet showcases a basic factorial calculation as a recursive function, emphasizing the structure:
#include <iostream>
using namespace std;
int factorial(int n) {
if (n <= 1) {
return 1; // Base case
} else {
return n * factorial(n - 1); // Recursive case
}
}
In this example, the base case is when \( n \) is less than or equal to 1. Common mistakes in writing recursive functions include neglecting the base case or having poorly defined recursive cases, leading to infinite recursion.
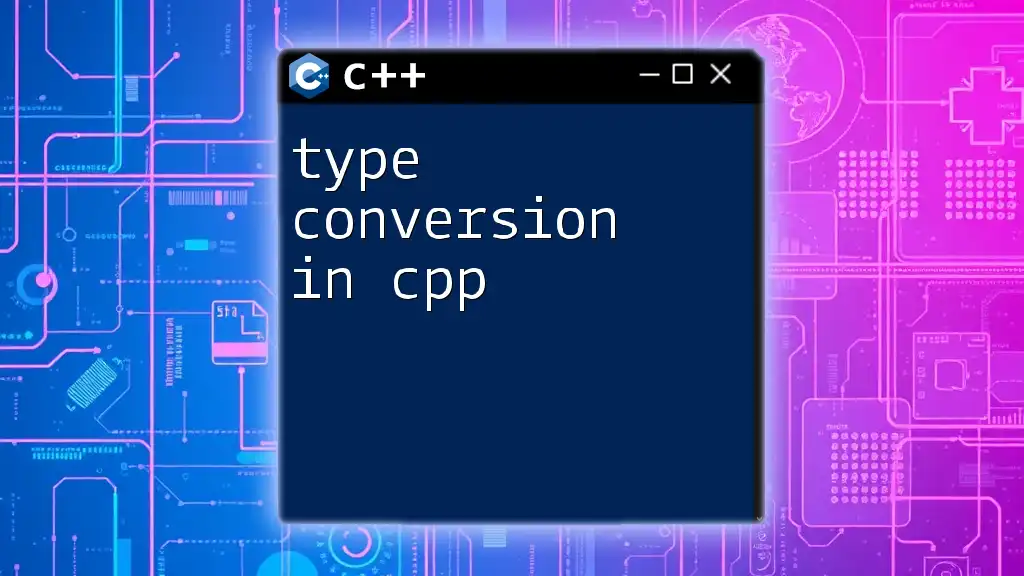
Common Algorithms Using Recursion in C++
Fibonacci Sequence
The Fibonacci sequence is a classic example that is often implemented using recursion, though it can be inefficient without optimization. Here’s how you can define the recursive function for Fibonacci:
#include <iostream>
using namespace std;
int fibonacci(int n) {
if (n <= 1) {
return n; // Base case
} else {
return fibonacci(n - 1) + fibonacci(n - 2); // Recursive case
}
}
This function breaks down larger Fibonacci calculations into smaller ones, but it can lead to redundant calculations. To improve efficiency, consider implementing memoization which stores previously calculated results.
Tower of Hanoi
The Tower of Hanoi is a well-known problem that is elegantly solved with recursion. The task is to move a stack of disks from one peg to another, obeying certain rules. Below is a recursive solution:
#include <iostream>
using namespace std;
void towerOfHanoi(int n, char source, char target, char auxiliary) {
if (n == 1) {
cout << "Move disk 1 from " << source << " to " << target << endl;
return;
}
towerOfHanoi(n - 1, source, auxiliary, target);
cout << "Move disk " << n << " from " << source << " to " << target << endl;
towerOfHanoi(n - 1, auxiliary, target, source);
}
This code illustrates how recursive calls enable the movement of disks while maintaining the rules of the game.
Depth-First Search (DFS) in Graphs
Recursion is essential in algorithms like Depth-First Search (DFS) for graph traversal. The following code snippet demonstrates a simple implementation:
#include <iostream>
#include <vector>
using namespace std;
void DFS(int v, vector<bool> &visited, const vector<vector<int>> &adj) {
visited[v] = true; // Mark the node as visited
cout << v << " "; // Print the node
for (int i : adj[v]) {
if (!visited[i]) {
DFS(i, visited, adj); // Recursive call for unvisited nodes
}
}
}
This function traverses through unvisited nodes in a depth-first manner, effectively employing recursion to explore all paths.
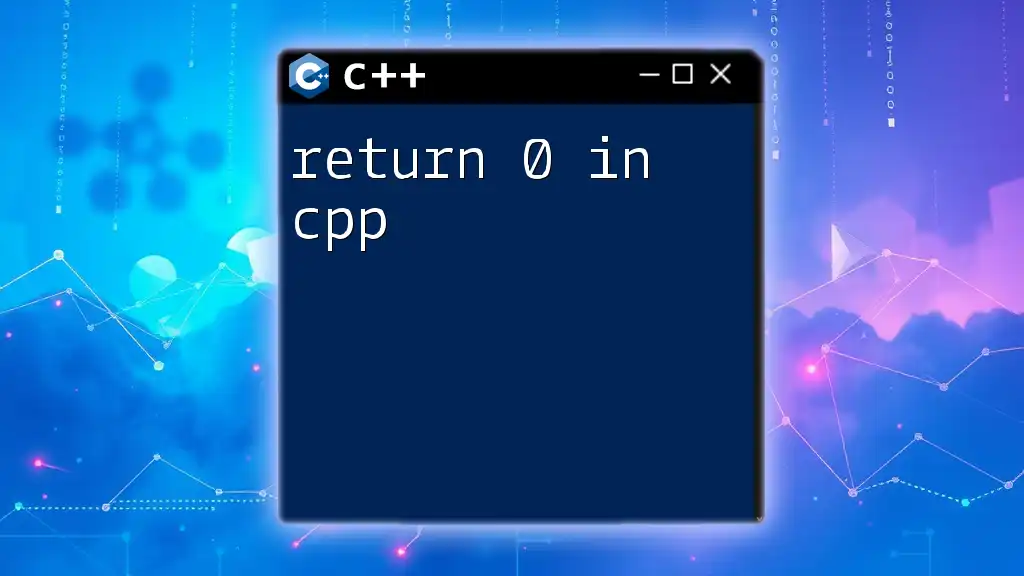
Advantages and Disadvantages of Recursion
Advantages
- Simplified Code: Recursion often leads to clearer and more concise code, particularly for complex problems.
- Intuitive Problem Solving: Recursive solutions closely mirror the problem structure, making them easier to understand.
Disadvantages
- Memory Consumption: Each function call requires stack memory, which can lead to high memory usage and potential stack overflow for deep recursion.
- Performance Impacts: Recursive functions can be slower due to multiple function calls, especially if optimal implementations (like memoization) aren’t utilized.
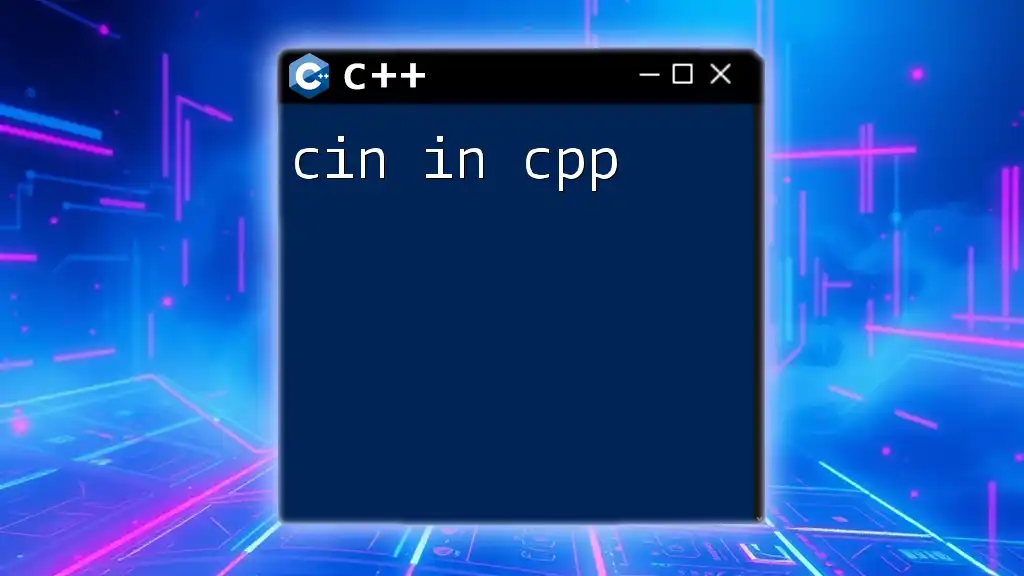
Best Practices for Recursion in C++
Tips for Writing Efficient Recursive Functions
To ensure your recursive functions are efficient, make sure to:
- Define Base Cases Clearly: Ensure that all possible routes lead to a base case to prevent infinite recursion.
- Optimize with Memoization: Avoid redundant calculations by storing previously computed results, particularly in problems like Fibonacci.
Debugging Recursive Functions
Debugging recursion can be tricky. Common pitfalls include infinite loops due to poorly defined base cases. Utilizing print statements to monitor the function's progress can provide insights into how each recursive call is processed.
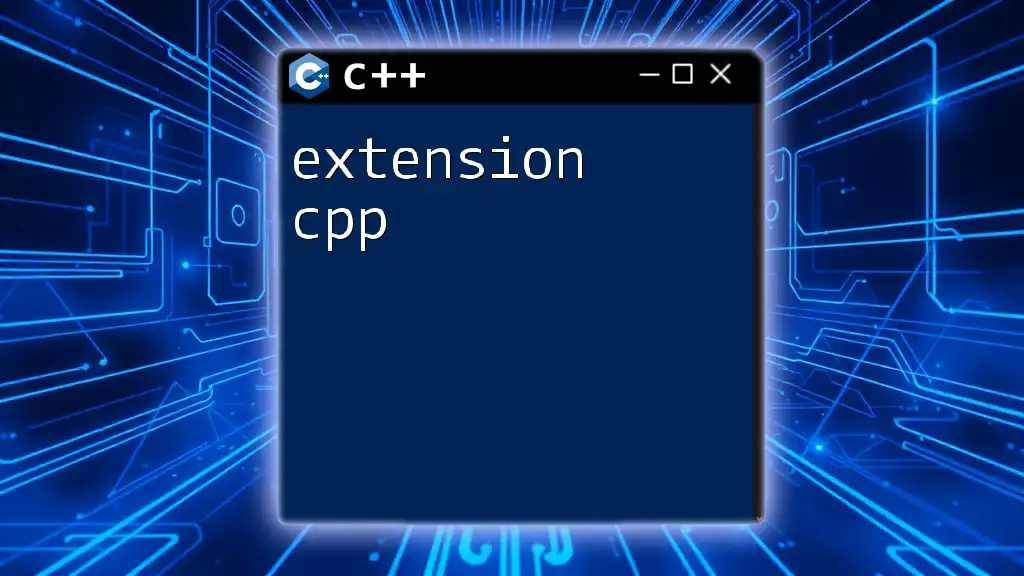
Conclusion
Recursion in C++ enables developers to tackle complex problems efficiently and elegantly. By understanding its mechanics, strengths, and weaknesses, programmers can harness the power of recursion to create robust algorithms. Practicing recursive problem solving is essential for mastering this technique and enhancing programming skills. Explore resources available online and practice regularly to improve your proficiency with recursion in C++.