In C++, `cin` is used for input operations, allowing users to read data from the standard input stream.
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number; // Reads an integer from the user
cout << "You entered: " << number << endl;
return 0;
}
What is cin in C++?
cin is a fundamental object in C++ used for input operations. It is part of the iostream library, and its primary purpose is to read data from the standard input stream, usually the keyboard. In C++, cin helps facilitate user interaction by allowing programs to accept input during runtime.
When we refer to input streams in C++, cin represents the default input source. Unlike file streams, which are used to read data from files, cin directly interacts with the user, making it an essential tool for many applications.
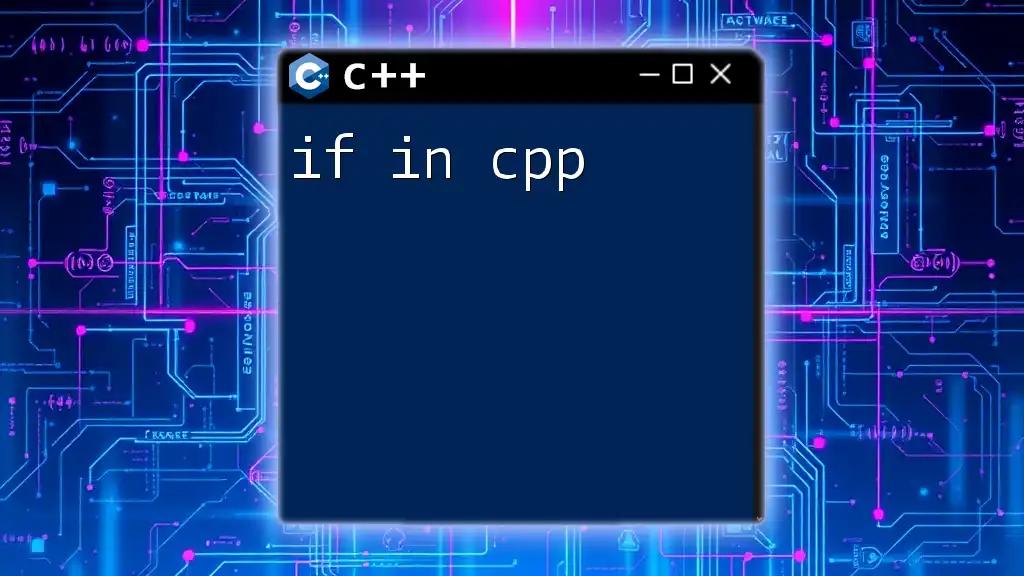
How to Use cin in C++
Using cin is straightforward, but knowing its syntax is crucial for effective implementation.
The basic syntax for using cin is:
cin >> variable;
Where `variable` can be of any input type, such as `int`, `float`, `string`, etc. Here’s a code snippet showcasing this basic usage:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "Your age is: " << age << endl;
return 0;
}
In this example, the program prompts the user for their age and then stores it in the variable `age`, echoing it back to the console.
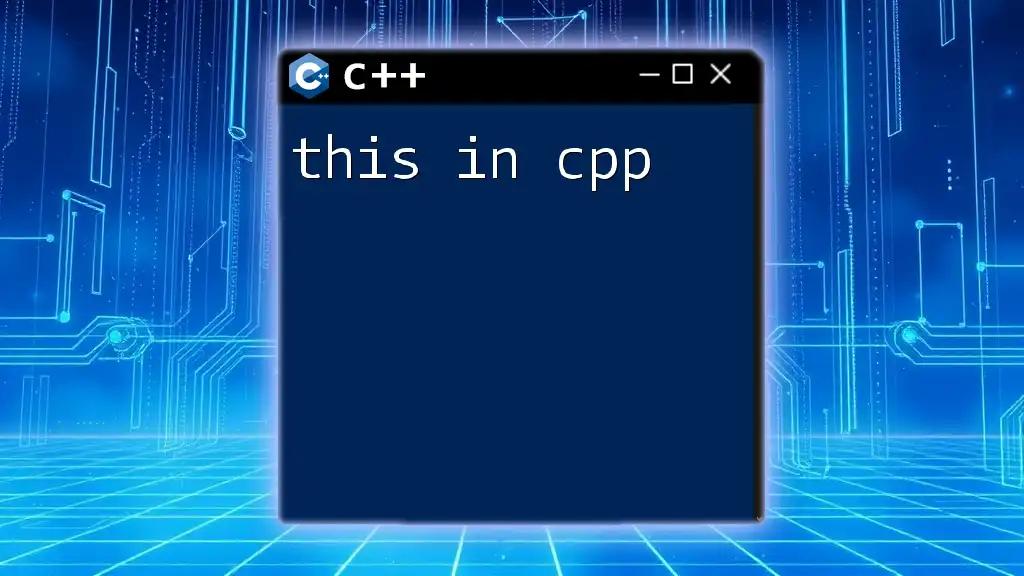
What Does cin Do in C++?
At its core, cin functions by capturing user inputs and transferring them into specified variables. When input is provided, cin reads the data from the input buffer and attempts to convert it into the appropriate type.
This process includes several steps:
- Input capturing: cin retrieves data from the input stream.
- Type conversion: The data is then converted to the type of the variable it's assigned to.
- Buffering: The input is stored temporarily in a buffer until it is read.
Internally, cin uses buffering to optimize input reading, allowing it to handle large volumes of data efficiently. However, cin also maintains the state of the input stream, which can be essential for error handling.
When using cin, it’s important to understand how the input stream operates. If it encounters an incorrect type (e.g., entering letters when expecting a number), it can lead to input failure, disrupting the program's flow.

How to Use cin in C++ for Different Data Types
Using cin with Integer Input
To read an integer, you simply specify an integer variable. For instance:
int number;
cout << "Enter a number: ";
cin >> number;
This code asks the user for a number and stores the input in `number`.
Using cin with Floating-Point Input
When dealing with floating-point numbers, the approach remains the same. Here’s how to read a float:
float price;
cout << "Enter the price: ";
cin >> price;
This allows for decimal inputs and expands the program’s capability.
Using cin with String Input
Reading strings using cin differs slightly. By default, cin reads input only up to the first whitespace. For example:
string name;
cout << "Enter your name: ";
cin >> name;
If the user inputs "John Doe", only "John" is stored in `name`. This can be limiting if full names are expected.
Using cin with getline()
To read a full line of text, including spaces, getline() is preferred. Here’s how to implement it:
string fullName;
cout << "Enter your full name: ";
getline(cin, fullName);
This reads the entire line of input until the user presses Enter, making it suitable for phrases or names with spaces.
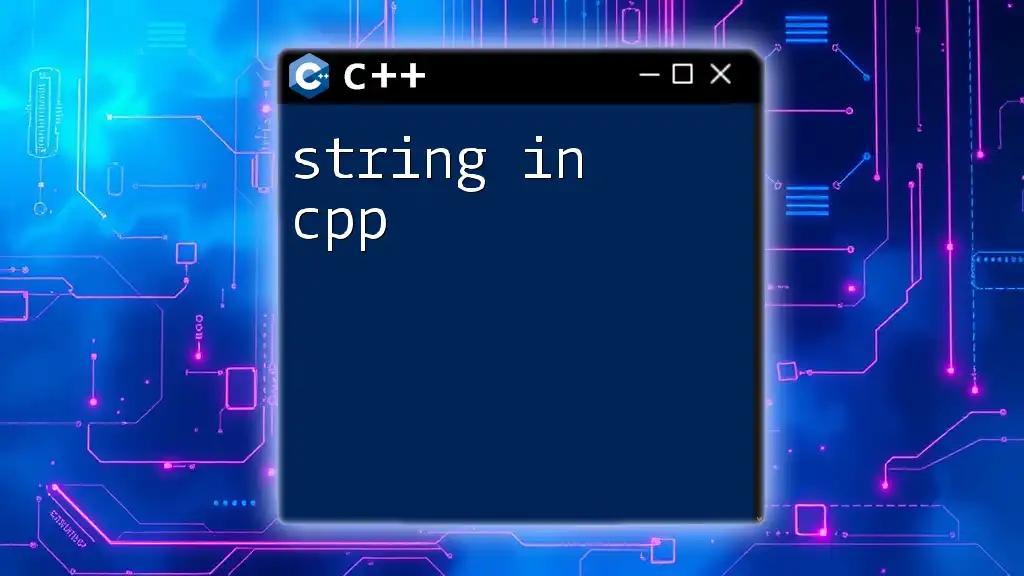
Common Issues and How to Fix Them
When working with cin, encountering issues is common, particularly with mismatched data types. Here’s how to handle them effectively:
- Input failure: If the user enters a value that cannot be converted to the required type (like letters for an integer), cin enters a fail state.
- Error handling: Use the following code to manage input failures:
if(cin.fail()) {
cin.clear(); // Clear the error state
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Ignore the invalid input
}
This code clears the fail state and discards invalid input, allowing the program to proceed smoothly without crashing.

Best Practices for Using cin in C++
To maximize the efficiency and reliability of using cin, consider the following best practices:
- Data type consistency: Ensure that the variable type matches the expected input.
- Input validation: Always validate user input to confirm it meets expectations (e.g., age should not be negative).
- User prompts: Clear and concise prompts make for a better user experience.
By adopting these practices, you reduce the chances of errors and enhance the program's robustness.
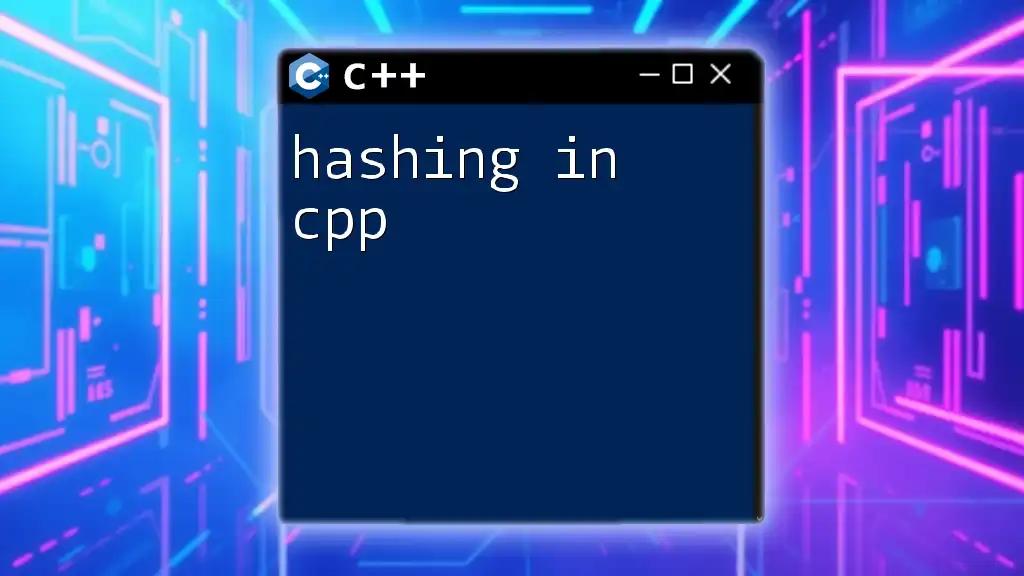
cin Example in C++
Here’s a comprehensive example that integrates various data types and error handling to illustrate a typical usage scenario for cin:
#include <iostream>
#include <limits>
#include <string>
using namespace std;
int main() {
int age;
float height;
string name;
// Prompt for name
cout << "Enter your name: ";
getline(cin, name);
// Prompt for age
while (true) {
cout << "Enter your age: ";
cin >> age;
if(cin.fail()) {
cin.clear(); // Clear the error
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Discard invalid input
cout << "Invalid input. Please enter a number." << endl;
} else {
break; // Valid input received
}
}
// Prompt for height
cout << "Enter your height in meters: ";
cin >> height;
// Output results
cout << "Name: " << name << ", Age: " << age << ", Height: " << height << "m" << endl;
return 0;
}
In this example, we gather a name, age, and height from the user, while also implementing error checking to ensure valid age input, showcasing the versatility of cin.

Conclusion
In summary, cin in C++ is a powerful tool that facilitates user input in a straightforward manner. Mastering its usage is crucial for any programmer looking to create interactive applications. By understanding cin's functions and applying best practices, you can handle user inputs with confidence and precision. As you continue to practice with various inputs and use cases, you’ll become more adept at utilizing cin effectively.