The `getch` function in C++ is used to capture a single character input from the user without waiting for the Enter key, making it useful for creating responsive console applications.
Here's a simple code snippet to demonstrate its usage:
#include <conio.h>
#include <iostream>
int main() {
std::cout << "Press any key to continue...";
getch(); // Waits for a key press
std::cout << "\nKey pressed! Exiting..." << std::endl;
return 0;
}
What is `getch`?
`getch` is a function found in the `<conio.h>` header file, primarily used in C and C++ programming. It stands for "get character" and serves the purpose of capturing a single character input from the keyboard without requiring the user to press the Enter key. This functionality makes `getch` especially valuable in scenarios where immediate keyboard feedback is essential, such as in games or interactive console applications.

History of `getch`
The `getch` function has its roots in early C programming, representing a fundamental method to interact with the console. Over the years, it has been adapted and used extensively in various applications, particularly in environments where quick response times to user input are critical. Despite its utility, `getch` remains primarily tied to the Windows platform, leading to some considerations regarding its portability across different operating systems.
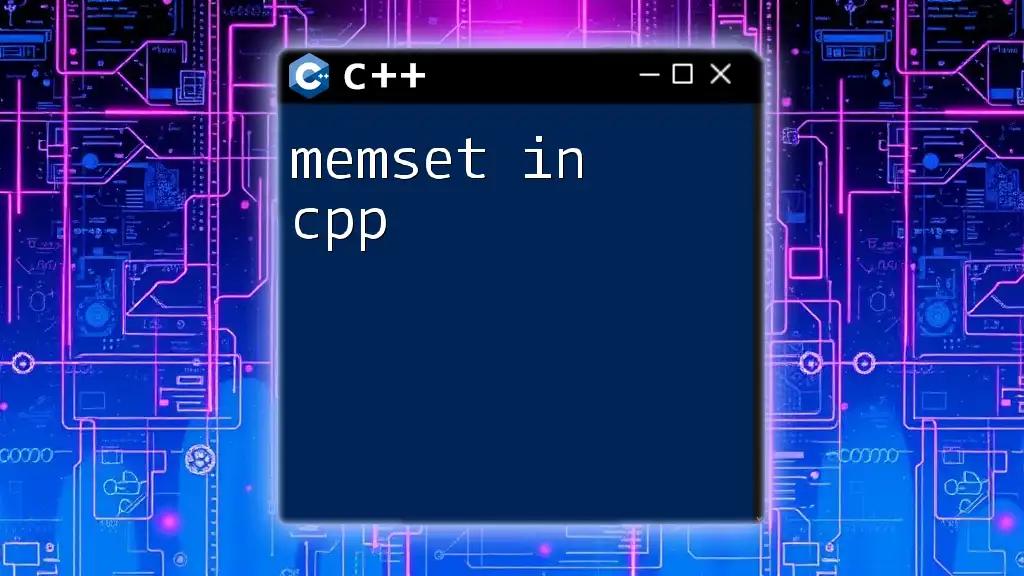
Understanding `getch` in C++
How `getch` Works
When a user presses a key, `getch` reads that key press from the keyboard buffer and returns the corresponding character. Unlike standard input methods such as `cin`, which require confirmation (i.e., hitting the Enter key), `getch` captures input immediately. Using `getch` allows developers to create interactive applications that respond to user input in real-time, providing a smoother experience.
Why Use `getch`?
Advantages of `getch`:
- Immediate input capture: `getch` allows for instantaneous response to key presses, making it ideal for scenarios where timing matters.
- No Enter key required: This functionality simplifies the user experience in console applications by removing the need for an additional confirmation step.
Situations where `getch` is most useful:
- In gaming applications, where player actions need to be detected without interruption.
- When creating command-line tools that require quick navigation or error handling.
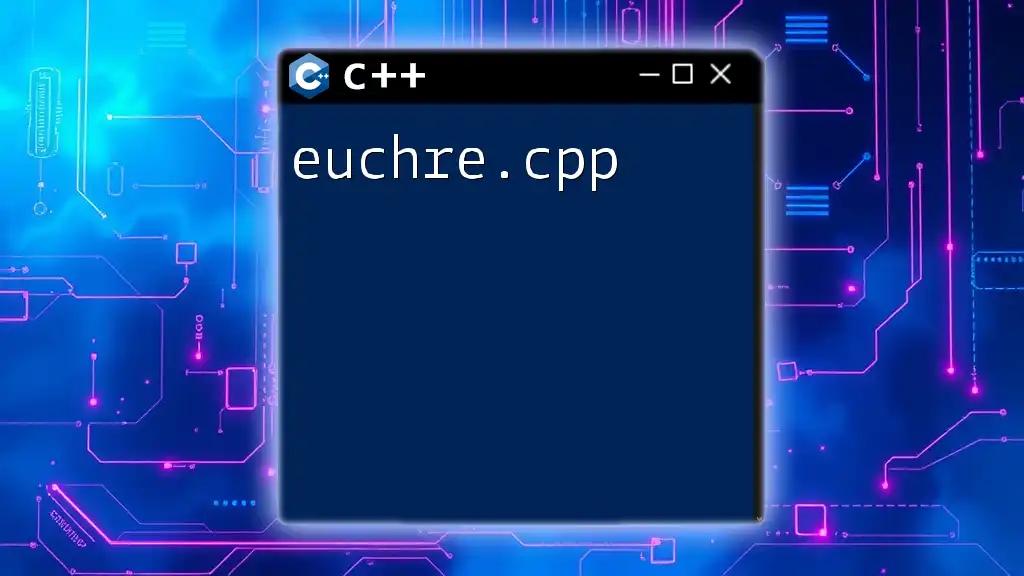
Implementing `getch` in Your C++ Programs
Including the Right Headers
To utilize `getch` in your program, you must include the `<conio.h>` header, which provides the necessary function declaration. It’s essential to note that `conio.h` may not be available on all compilers, particularly those used on Linux or macOS systems. Therefore, be mindful of the potential limitations when developing cross-platform applications.
Basic Syntax and Usage of `getch`
Here’s how you can use `getch` in its basic form:
#include <iostream>
#include <conio.h>
int main() {
std::cout << "Press any key to continue...";
getch(); // waits for a key press
return 0;
}
In this simple example, the program prompts the user with a message and then pauses execution until a key is pressed. This straightforward usage illustrates `getch`'s primary function effectively.
Handling Key Presses with `getch`
`getch` can also be used to detect specific key presses and respond accordingly. For instance, the following example checks for the Escape key press:
#include <iostream>
#include <conio.h>
int main() {
char ch;
std::cout << "Press 'Esc' to exit...\n";
while (true) {
ch = getch(); // capture input
if (ch == 27) { // ASCII code for 'Esc'
std::cout << "Exiting...\n";
break;
}
std::cout << "You pressed: " << ch << "\n";
}
return 0;
}
In this code, the program enters an infinite loop where it continuously waits for key presses. If the user presses the Escape key (ASCII code 27), the program exits. Any other key press will be displayed on the console, demonstrating how `getch` can facilitate more complex user interactions.

Important Considerations When Using `getch`
Limitations of `getch`
While `getch` is a powerful tool, it also has its limitations. Portability is one significant concern. As it is part of the `<conio.h>` library, which is primarily supported in Windows environments, using `getch` in cross-platform applications can lead to compatibility issues. If your program needs to run on various operating systems, you may consider alternative approaches.
Alternatives to `getch`
If you aim for broader compatibility, several libraries can offer similar functionalities:
- ncurses: A powerful library for text-user interfaces in Unix/Linux environments allows for non-blocking input and works similarly to `getch`.
- PDCurses: A derivative of `ncurses`, it offers a good level of compatibility for Windows systems.
Using these libraries can help ensure that your applications perform consistently across different platforms.
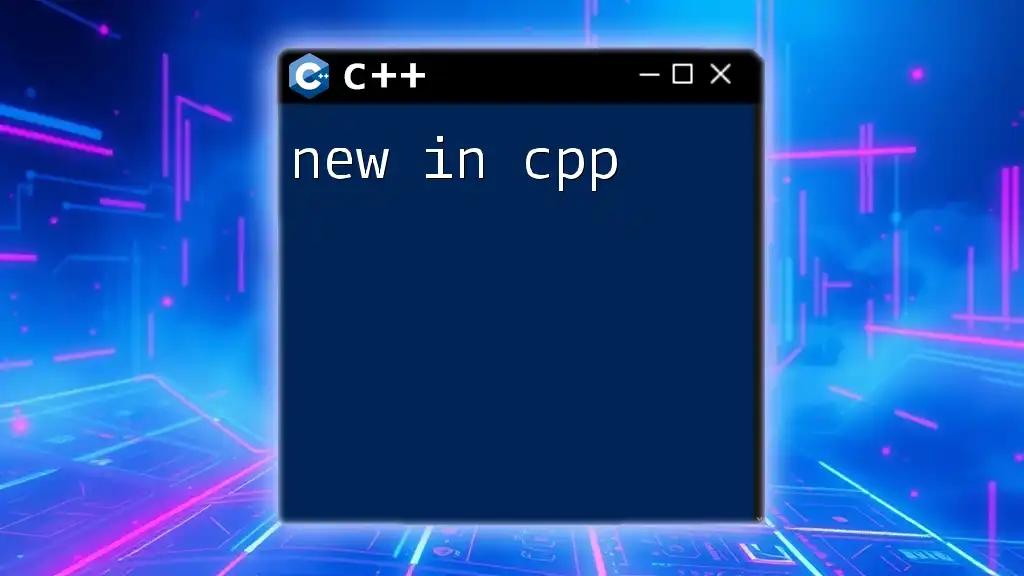
`getch` in Practical Applications
Games and Interactive Applications
`getch` is particularly suited for game development and interactive applications due to its ability to capture user input instantaneously. For instance, you can create a simple game mechanism where the player controls a character's movement through keyboard input. Here’s how it might look:
#include <iostream>
#include <conio.h>
int main() {
char ch;
std::cout << "Press 'a' to move left, 'd' to move right, or 'q' to quit.\n";
while (true) {
ch = getch();
if (ch == 'q') {
break;
} else if (ch == 'a') {
std::cout << "Moving left...\n";
} else if (ch == 'd') {
std::cout << "Moving right...\n";
}
}
return 0;
}
In this example, the program listens for the user to press either 'a', 'd', or 'q'. Based on the input, it generates a response in real time. This demonstrates how `getch` can make applications feel more interactive and dynamic, crucial in gaming environments.
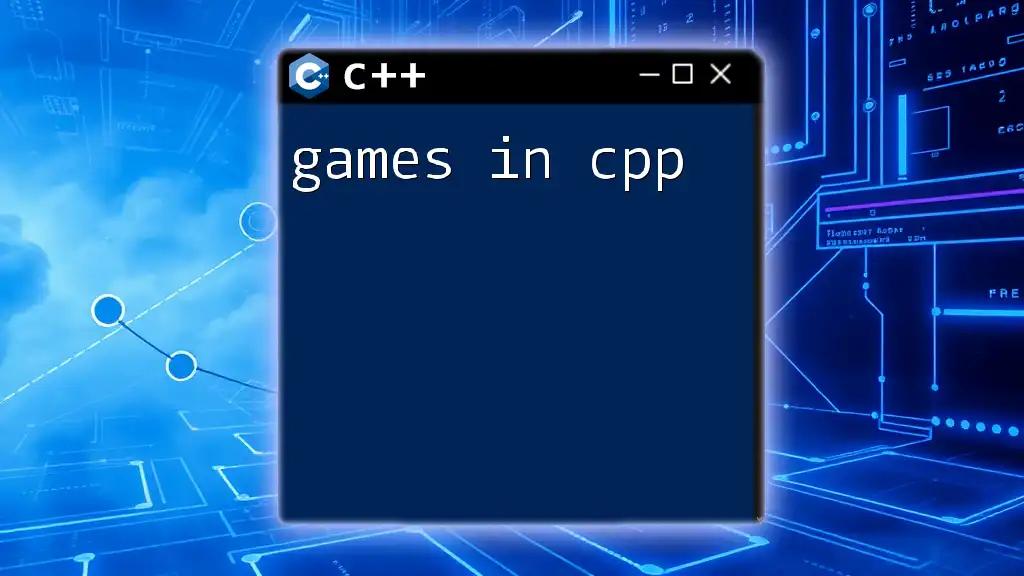
Conclusion
Summary of Key Points
This guide introduced the `getch` function in C++, elucidating its utility in capturing immediate user input. Key aspects discussed include the function’s history, implementation methods, and relevant code examples, showcasing its role in building interactive applications.
Further Learning Resources
For those interested in deepening their understanding of C++ programming and the `getch` function, consider exploring recommended textbooks, online courses, or community forums. Engaging with these resources can enhance your programming skills and expose you to new concepts and practices.

Call to Action
We encourage you to share your experiences using `getch` in your projects or any specific questions you have. Your feedback can guide future topics and assist others in maximizing their understanding of C++ and its unique functionalities.