C++ is a powerful programming language that allows developers to create complex games with high performance and control over system resources.
Here's a simple example of a C++ game loop that prints "Hello, Game!" every second:
#include <iostream>
#include <chrono>
#include <thread>
int main() {
while (true) {
std::cout << "Hello, Game!" << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(1));
}
return 0;
}
Understanding C++ in Game Development
When it comes to developing games, C++ is often regarded as one of the most powerful programming languages. Its ability to provide fine-grained control over system resources and efficient memory management is pivotal for performance-intensive applications like games. Moreover, C++ offers a rich set of features, such as object-oriented programming, which allows developers to create complex interactions and behaviors.
Numerous popular game engines utilize C++ for their core functionalities, including Unreal Engine and Unity (though Unity primarily uses C#). Understanding how these engines leverage C++ can provide valuable insights into creating high-performance games.

Getting Started with C++ for Game Development
Setting Up Your Development Environment
To begin your journey in game development with C++, setting up your development environment is crucial. The following IDEs are highly recommended for C++ programming:
- Visual Studio: A powerful IDE tailored for Windows environment, offering integrated debugging and extensive libraries.
- CLion: A cross-platform IDE that enhances productivity through intelligent coding assistance.
Moreover, using libraries like SFML (Simple and Fast Multimedia Library), SDL (Simple DirectMedia Layer), and OpenGL greatly facilitates game development by providing tools for graphics, audio, and input handling.
Basics of C++ Relevant to Games
Before diving into complex game development, you should grasp some foundational C++ concepts:
- Variables and Data Types: Understanding the types of data you're handling is essential.
- Control Structures: Conditional statements (if-else) and loops (for, while) will form the backbone of your game logic.
- Functions: They allow you to structure your code modularly.
As a quick example, you can define a simple function to move your character:
void moveCharacter(int x, int y) {
// Code to move character
}
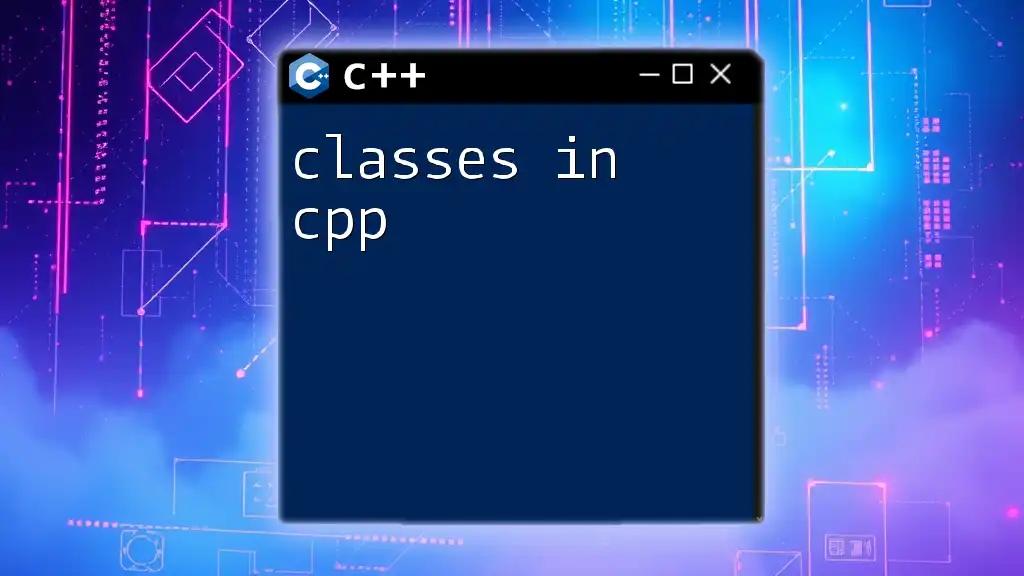
Core Concepts of Game Development
Game Loops and Timing
A game loop is central to any game; it manages the game's activities including user input, game updates, and rendering.
The basic structure of a game loop looks like this:
bool isRunning = true;
while (isRunning) {
processInput();
updateGame();
renderGame();
}
Key Takeaway: The game loop runs continuously, enabling real-time gameplay.
Entities and Game Objects
In game development, distinguishing between entities and game objects is critical. Entities are fundamental items in your game, while game objects typically encapsulate the entity's properties and behaviors.
Consider a simple class design for a player character:
class Player {
public:
int health;
int xPosition;
int yPosition;
void move(int x, int y) {
xPosition += x;
yPosition += y;
}
};
This clean modular approach allows for extensive behavior definition down the line.
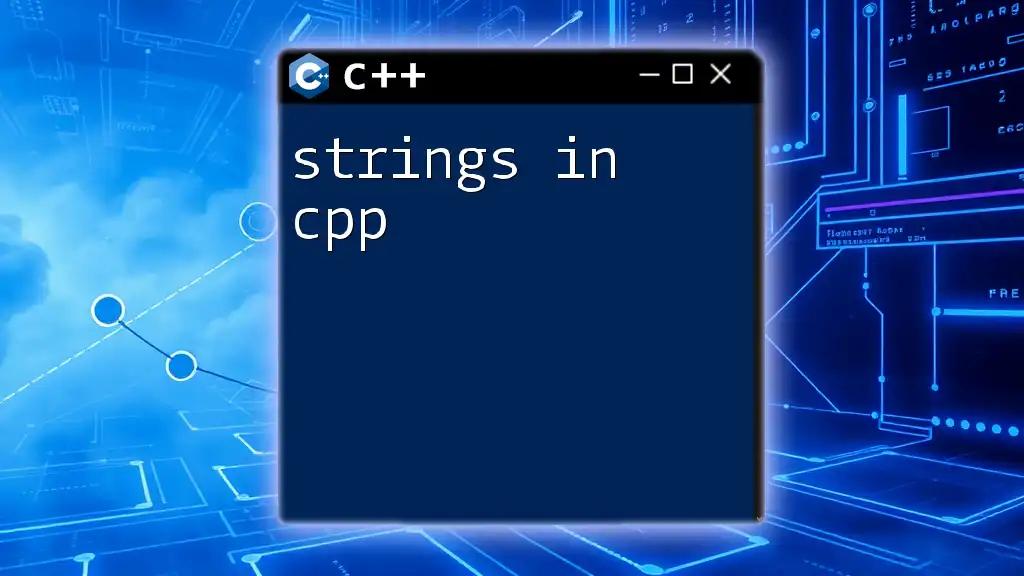
Graphics and User Interface
Rendering Graphics
To create visually appealing games, you need to handle graphics rendering. One of the most popular libraries for 2D graphics is SFML. Here's how to create a window using SFML:
sf::RenderWindow window(sf::VideoMode(800, 600), "Game Window");
You can utilize SFML to draw shapes and textures to the screen, enhancing the visual experience of your game.
Creating a Basic User Interface
A user-friendly UI is essential for player engagement. Using libraries like SFML, you can create text, buttons, and other interface elements easily. Here’s an example of rendering text on the screen:
sf::Text text;
text.setString("Hello, Player!");
text.setCharacterSize(24);
text.setFillColor(sf::Color::White);
// Render text in the window
window.draw(text);
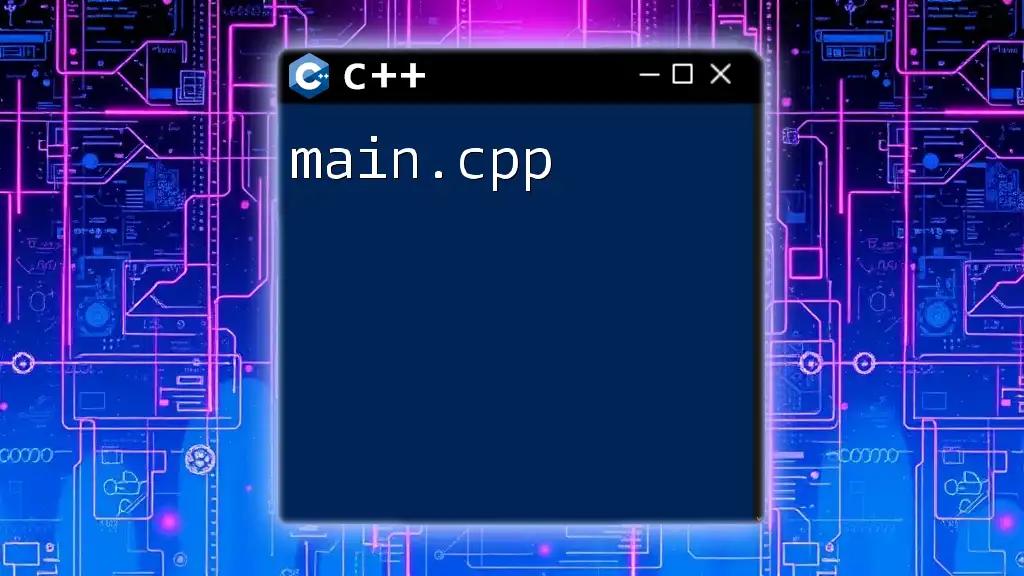
Sound and Music
Integrating Audio
Incorporating sound effects and music can significantly enhance the gaming experience. You can use libraries like FMOD or SDL_mixer to manage audio playback. Here is a pseudocode example for playing a sound:
// Pseudocode for playing a sound
sounds.load("soundfile.mp3");
sounds.play();
The auditory feedback will make your game more immersive.

Developing a Simple Game in C++
Creating a simple game involves several components, which we'll illustrate through a basic 2D game development example.
Step-by-Step Guide: Creating a Basic 2D Game
- Game Concept: Decide on a straightforward game idea, such as a player dodging falling objects.
- Game Components: Set up player, enemies, and the game environment. Use C++ classes to manage each component effectively.
For instance, you can outline your enemy class:
class Enemy {
public:
int xPosition;
int yPosition;
void spawn(int x, int y) {
xPosition = x;
yPosition = y;
}
};
- Game Logic: Use the game loop to handle player movements and collisions.
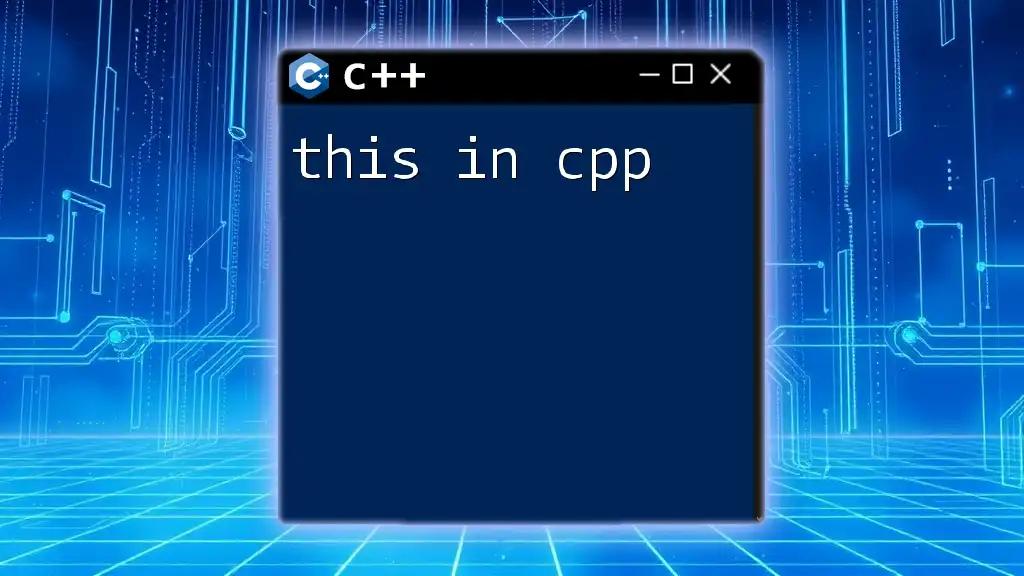
Multiplayer Gaming in C++
Networking Basics
With the rise of online gaming, understanding networking concepts is increasingly relevant. C++ can effectively manage networking tasks utilizing libraries such as ENet and Boost.Asio.
Here's a basic example of initiating a network connection:
// Basic pseudocode for initiating a network connection
connect(serverAddress, port);
This allows you to set up multiplayer functionalities within your game.
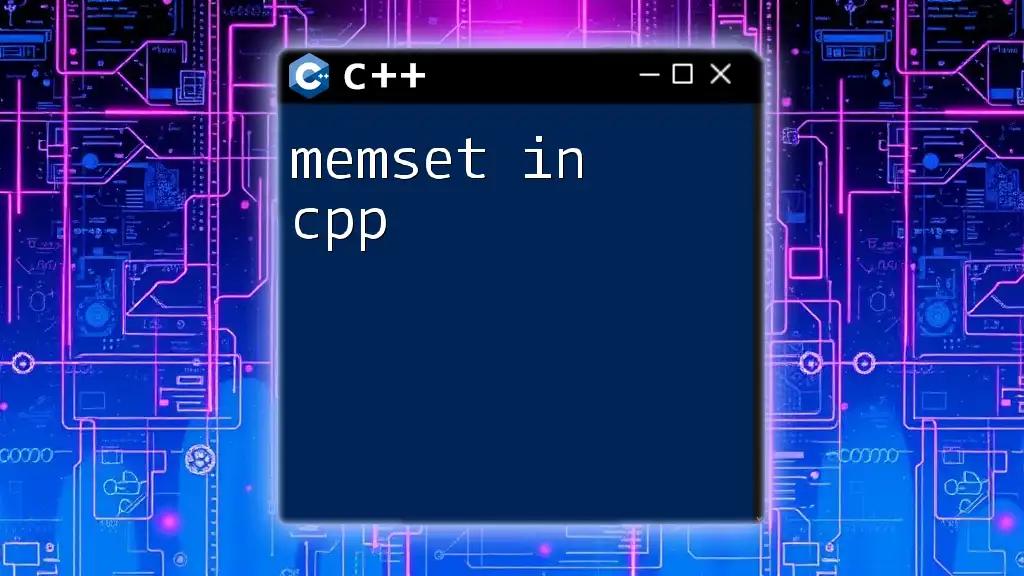
Debugging and Testing Games
Common Bugs and How to Fix Them
Expect to encounter various bugs in game development, ranging from graphical glitches to unexpected crashes. Familiarizing yourself with debugging tools can streamline this process. Tools within your IDE, such as breakpoints and memory leak detection, will be invaluable.
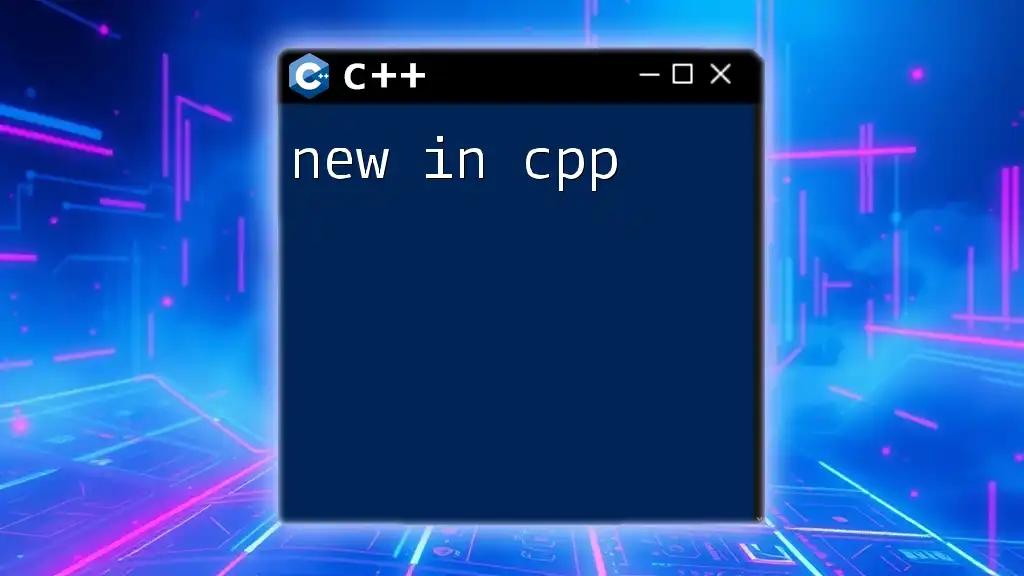
Optimizing Performance
Performance Tips for C++ Games
Optimizing your game is crucial for ensuring a smooth gameplay experience. Here are some effective strategies:
- Memory Management: Use smart pointers and avoid memory leaks.
- Optimize Graphics: Implement efficient rendering techniques, such as sprite batching and culling.
By employing these methods, your game will not only run smoothly but also be more responsive to player actions.

Resources and Communities
Learning More about C++ Game Development
To further enhance your skills, consider diving into recommended books and online courses focused on C++ game development. Engaging with the developer community via forums and Discord can provide support and guidance.

Conclusion
Embarking on the journey of developing games in C++ can be challenging yet profoundly rewarding. The rich features of C++ paired with continuous learning and practice will empower you to create engaging and performance-driven games. Stay curious and keep pushing the boundaries of your creativity and technical skills!