Namespaces in C++ are a declarative region that provides a scope to the identifiers (names of types, functions, variables, etc.) inside it, helping to prevent name conflicts in larger projects.
Here's a basic example of how to use namespaces in C++:
#include <iostream>
namespace MyNamespace {
void greet() {
std::cout << "Hello from MyNamespace!" << std::endl;
}
}
int main() {
MyNamespace::greet(); // Calling the function from MyNamespace
return 0;
}
What is a Namespace in C++?
A namespace in C++ is a declarative region that provides a scope to the identifiers (the names of types, functions, variables, etc.) inside it. It is a mechanism to avoid naming conflicts that can arise in larger codebases when different parts of a program have elements that share the same name. By organizing code into namespaces, developers gain a clearer structure, facilitating better maintenance and readability.
The fundamental purpose of a namespace is to manage the global namespace, which holds every identifier in a C++ program. Without namespaces, it can become chaotic, especially when integrating third-party libraries or user-defined functions. Namespaces allow for better organization of code logically and semantically.
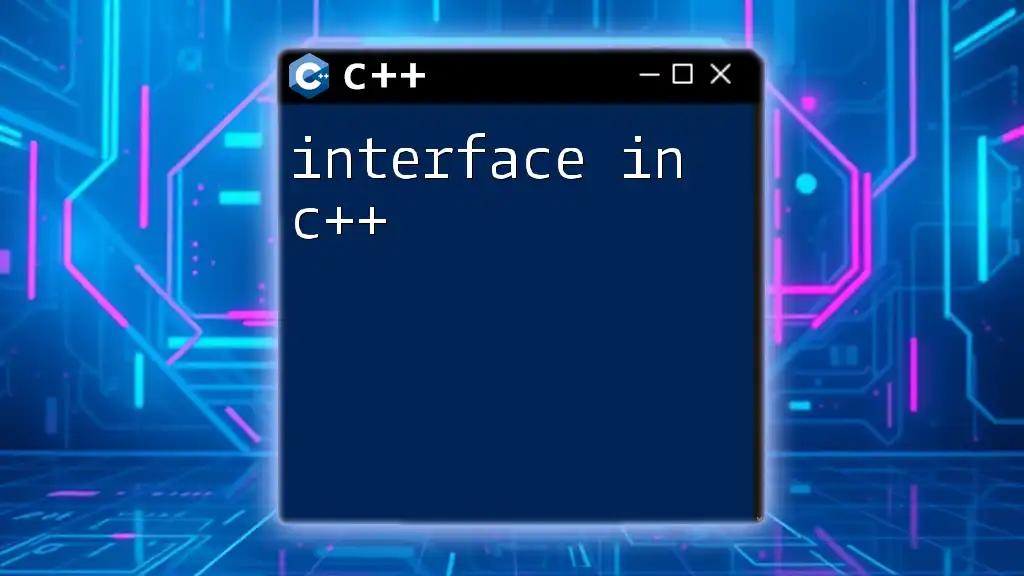
Types of Namespaces in C++
Standard Namespace
In C++, the std namespace is the default namespace used in the Standard Library. This includes a vast number of built-in functions, classes, and objects. To prevent potential conflicts in naming, the C++ standard library defines its components within the std namespace.
To make standard library features available without prefixing them with `std::`, you can use:
using namespace std;
This allows you to call functions more conveniently, but should be used judiciously, as it introduces all names from the namespace into the current scope.
User-defined Namespaces
You can create your own namespaces to encapsulate your functions and classes. This is particularly beneficial in larger applications or libraries to prevent naming conflicts.
Here’s a simple example of creating a user-defined namespace:
namespace MyNamespace {
void MyFunction() {
// code to do something
cout << "Hello from MyNamespace!" << endl;
}
}
In this example, we've created a function `MyFunction` inside `MyNamespace`, encapsulating its functionality and preventing conflicts with other similarly named functions.
Nested Namespaces
C++ allows for nested namespaces, which can further organize your code. A nested namespace is defined inside another namespace, allowing finer-grained control over naming.
For instance:
namespace Outer {
namespace Inner {
void innerFunction() {
// code for inner function
cout << "Hello from Outer::Inner!" << endl;
}
}
}
In this scenario, `innerFunction` is defined within `Inner`, which is itself within `Outer`, allowing clear hierarchical organization.

Declaring and Defining a Namespace in C++
The syntax for declaring a namespace is simple. You use the `namespace` keyword followed by the desired namespace name and then provide the scope of that namespace enclosed in curly braces.
Here’s a basic outline:
namespace YourNamespace {
// Declare functions or variables
int exampleNum;
void exampleFunction() {
// function implementation
}
}
This code declares a namespace `YourNamespace` which can hold variables, functions, and even other namespaces.

Accessing Elements in Namespaces
Using the Scope Resolution Operator
To access elements defined within a namespace, C++ uses the scope resolution operator (`::`). This operator tells the compiler which namespace to look into for the specific identifier.
For example, if we want to call `exampleFunction` from `YourNamespace`, we’d write:
YourNamespace::exampleFunction();
Using Statements
By using the statement:
using namespace YourNamespace;
You can bring all the identifiers from that namespace into the current scope, which makes calling elements much more straightforward:
exampleFunction(); // No need for YourNamespace:: prefix
However, while this simplifies access, it can also lead to ambiguity if two namespaces have overlapping identifiers.
Why Avoid Global Namespace Pollution
While convenient, overusing `using namespace` can lead to namespace pollution, where names collide, making the code less clear and harder to debug. It is often better to be explicit in your function calls or to only bring in specific identifiers using:
using YourNamespace::exampleFunction;
This approach keeps your global namespace clean while still allowing access to specific components from the desired namespace.
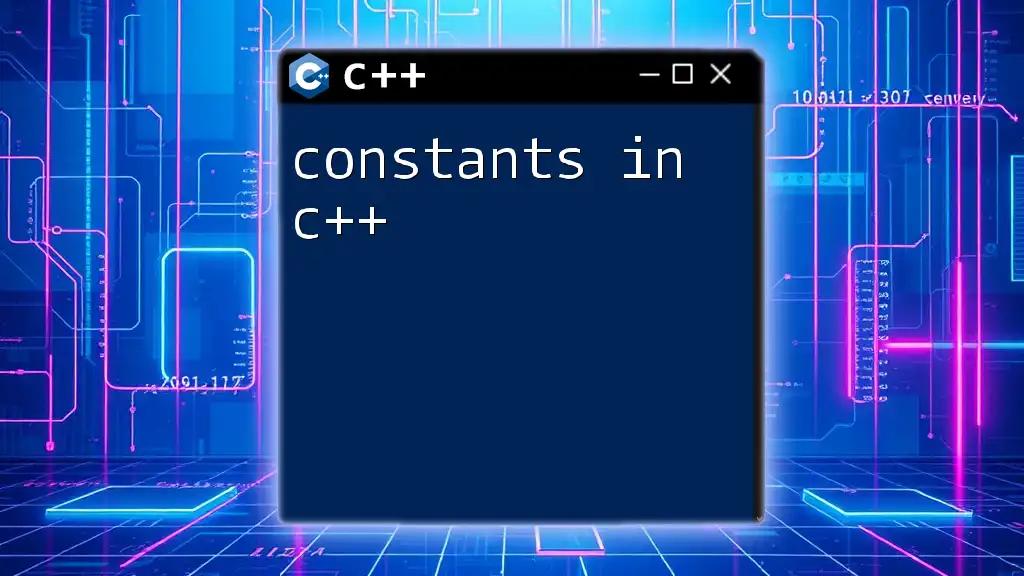
Best Practices with Namespaces in C++
- Keep namespaces small and relevant. This makes it easier for other programmers (or future you) to understand the context of functions and classes.
- Use namespaces to logically group related functionality. For example, if you have a library for mathematical operations, a `Math` namespace makes sense.
- Prefer nested namespaces to avoid clutter. If a namespace grows too large, consider splitting it into nested namespaces based on functionality.
- Be cautious with `using namespace`. Opt for explicit scoping unless simplicity is essential and the risks of conflicts are minimal.

Common Use Cases of Namespaces in C++
Namespaces in C++ come in handy in various scenarios:
- Library Development: When creating libraries, using namespaces prevents conflicts with the user’s identifiers.
- Collaboration: In team environments, different developers may define functions or classes with the same name. Namespaces help keep this organized.
- Code Organization: For larger projects, grouping logically related components into namespaces enhances code structure.

Conclusion
Understanding namespaces in C++ is essential for effective coding, especially as projects grow in complexity. They help prevent naming conflicts and structure your code better. The examples and practices outlined here provide a solid foundation for using namespaces effectively in your development work.
Now, it’s time to practice! Experiment with your own namespaces and see how they can enhance the organization and clarity of your code. For those interested in delving deeper, consider exploring more advanced namespace techniques and features in C++.
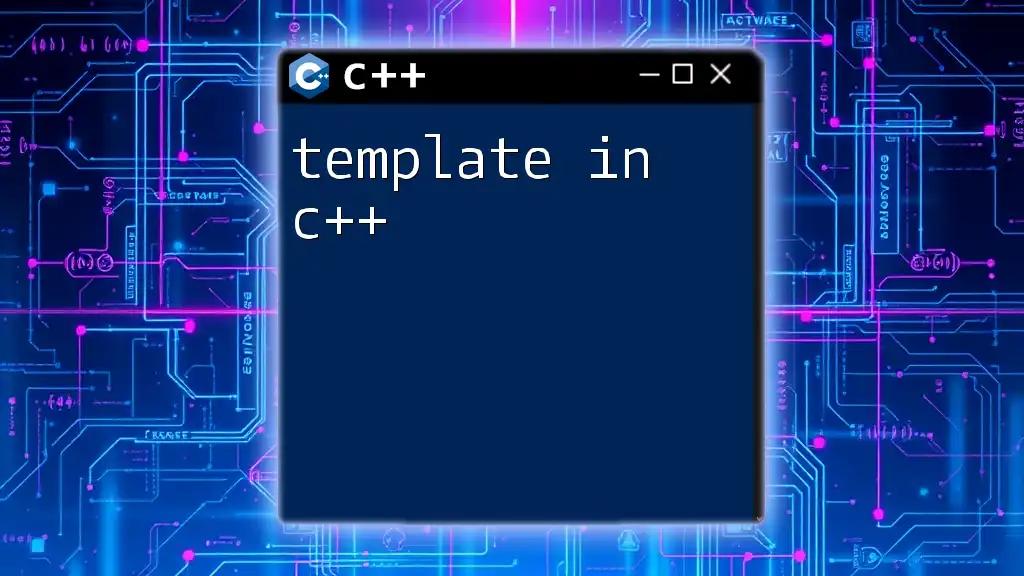
FAQs about Namespaces in C++
-
What is a namespace in C++? A namespace is a declarative region that helps encapsulate identifiers to prevent naming conflicts.
-
How do I declare a namespace in C++? Using the `namespace` keyword followed by the name and encapsulating the desired elements in curly braces.
-
What problems do namespaces solve? They organize code, prevent naming collisions, and improve overall code maintainability.
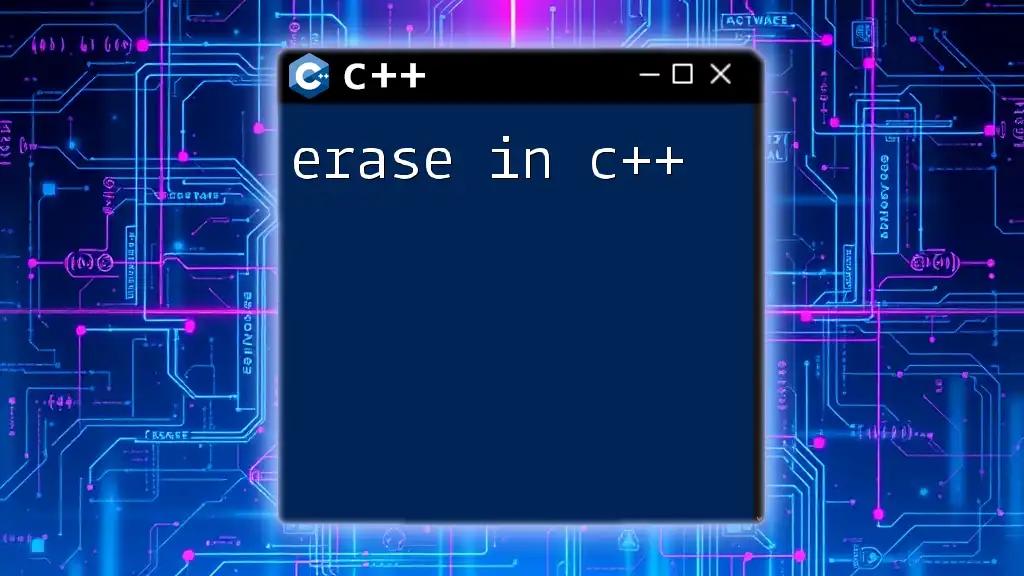
Additional Resources
For further reading, check out the official C++ documentation, tutorials, and tools that support namespace management in your development environment. Exploring these resources will deepen your understanding of namespaces and improve your C++ coding skills.