An anonymous namespace in C++ is used to encapsulate identifiers so that they have internal linkage, meaning they are only accessible within the same translation unit.
namespace {
void myFunction() {
// This function can only be accessed within this translation unit
std::cout << "Hello from an anonymous namespace!" << std::endl;
}
}
int main() {
myFunction(); // This will work
return 0;
}
Understanding the Purpose of Anonymous Namespaces
What are Unnamed Namespaces?
An unnamed namespace is a special type of namespace in C++ that acts as a way to encapsulate various entities, like functions and variables, so that they are only accessible within the same translation unit. This means that the elements defined inside an anonymous namespace cannot be accessed from other files that are part of the same project, reducing the risk of name collisions.
In C++, when you declare a namespace without a name, it becomes anonymous. This is particularly useful in large codebases where many files might potentially define functions or variables with the same name. By leveraging anonymous namespaces, you can sidestep potential conflicts.
Why Use Anonymous Namespaces?
Encapsulation of Functions and Variables is one of the primary reasons to use anonymous namespaces. They allow for better organization of code by limiting the visibility of functions and variables to only those pieces of code that require them.
For example, consider the following implementation:
namespace {
void helperFunction() {
// This function is only accessible within this translation unit
std::cout << "This is a helper function." << std::endl;
}
}
In the example above, `helperFunction()` is not visible outside the file it's declared in, ensuring that it doesn't interfere with any other function named `helperFunction` in different files.
Preventing Name Collisions is another significant advantage of anonymous namespaces. In a larger codebase, where multiple developers might inadvertently use the same variable or function names, this feature helps prevent unexpected behavior due to naming conflicts.
Take a look at the following code snippet:
namespace {
int value = 10; // this variable is unique to this translation unit
}
// Other source files can define 'int value' without any conflict.
In this case, the variable `value` within the anonymous namespace will not interfere with similar declarations elsewhere in the project.

Syntax and How to Declare Anonymous Namespaces
Declaring an Anonymous Namespace
Declaring an anonymous namespace is quite straightforward. Simply wrap your code block inside the `namespace {}` construct. For instance, you might write:
namespace {
int localVar = 20; // This will not be accessible outside this translation unit
}
Scope and Visibility
An anonymous namespace provides local scope to the names defined within it. Unlike global variables, which can be accessed from anywhere in the program, variables and functions defined within an anonymous namespace are restricted to that specific file.
Consider this example, where the scope of a function is demonstrated:
namespace {
void funcA() {
// functionality here
std::cout << "funcA called." << std::endl;
}
}
void funcB() {
funcA(); // valid call, since funcA is within the same translation unit
}
// funcA is not accessible outside the anonymous namespace.
In this case, `funcA` is available to `funcB` because both are located in the same translation unit. However, any attempt to call `funcA` from another file would result in a linker error since it is encapsulated.

Practical Examples of Anonymous Namespaces
Using Anonymous Namespaces in Header Files
When it comes to header files, it is generally considered best practice to avoid placing definitions within unnamed namespaces. However, if you must encapsulate functionality, be cautious and ensure that usage is limited to that header’s intended scope.
Here’s an example where an anonymous namespace is used in a header file for encapsulating a helper function:
// myFunctions.h
namespace {
void privateHelperFunction() {
std::cout << "This is a private helper function." << std::endl;
}
}
The `privateHelperFunction()` can now be accessed solely within the files that include `myFunctions.h`, preventing possible name conflicts.
Avoiding Multiple Definition Errors
In C++, when multiple source files contain the same definition, it can lead to multiple definition errors during linking. Utilizing anonymous namespaces can mitigate this issue.
Consider the following situation to illustrate how to avoid such conflicts:
namespace {
void utilizeFunction() {
// Implementation code
std::cout << "Utilize Function executed." << std::endl;
}
}
By encapsulating `utilizeFunction()` within an anonymous namespace, you ensure that the function can be defined in multiple source files without linking conflicts, as each definition is limited to its respective translation unit. Thus, even if another file has a function with the same name, they do not conflict.
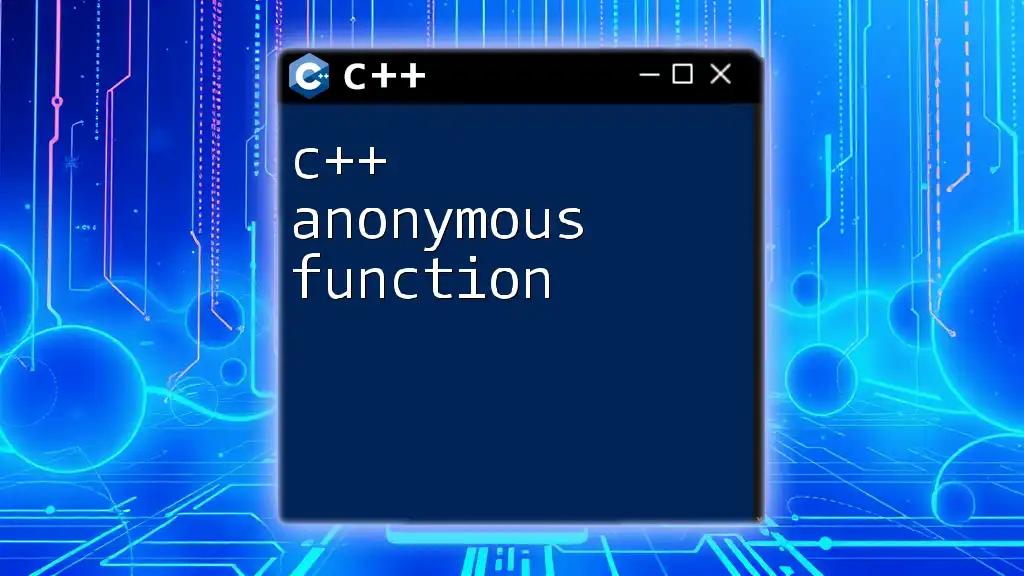
Best Practices for Using Anonymous Namespaces
When to Use Anonymous Namespaces
Anonymous namespaces should be used judiciously. They’re ideal in scenarios where a function or variable should only be used within a single translation unit. Focus on using them for helper functions or internal utility functions that don’t need to be exposed outside the file they are implemented in.
Managing Code Readability and Maintenance
While anonymous namespaces enhance encapsulation and help avoid naming conflicts, they can affect code readability if overused. It is essential to review your code regularly and maintain documentation that clarifies the role of functions and variables within anonymous namespaces.
A balance must be struck where clean code practices are upheld while still harnessing the benefits of anonymous namespaces. Understand your team’s collaboration workflow to ensure that everyone is aware of how and when to utilize these namespaces effectively.
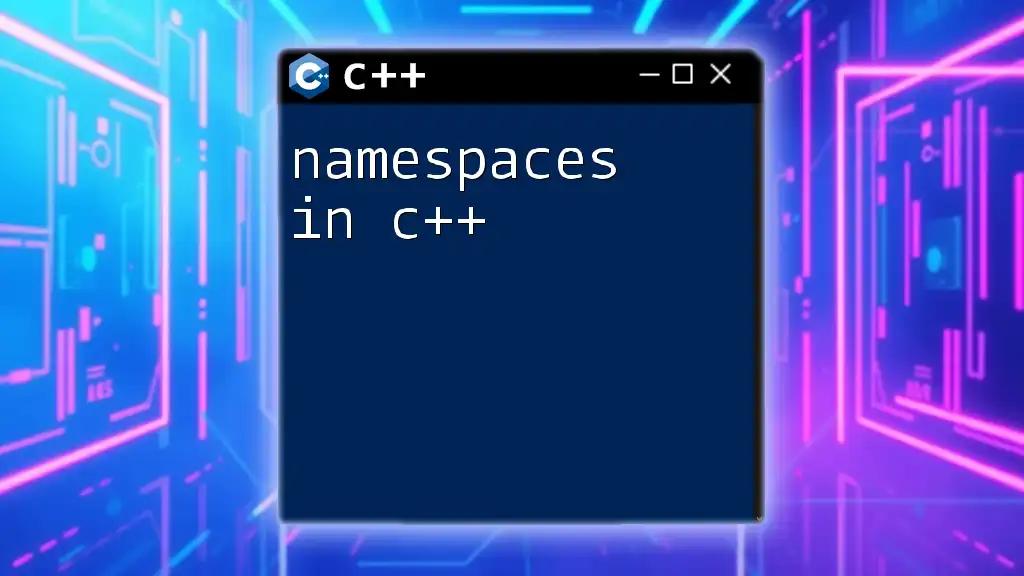
Conclusion
In summary, anonymous namespaces in C++ offer powerful capabilities for encapsulating functions and variables, thereby preventing name collisions and ensuring that your code remains clean and organized. By implementing best practices, you can wield the full potential of anonymous namespaces while enhancing the maintainability and readability of your codebase.
Take the time to experiment with anonymous namespaces in your projects, ensuring that you understand when and where they can be most beneficial. With a solid understanding of this C++ feature, you will be better equipped to write efficient and effective code that stands the test of time.

Additional Resources
For further exploration of C++ namespaces and their intricacies, consider diving into specialized readings, tutorials, and community forums online. Understanding the broader context of namespaces will significantly enhance your overall programming prowess in C++.