The Snake Game in C++ is a simple console application that allows players to control a snake, collecting food while avoiding collisions with the game boundaries and itself.
Here's a basic code snippet for a simple Snake Game in C++:
#include <iostream>
#include <conio.h>
#include <windows.h> // For Sleep()
using namespace std;
bool gameOver;
const int width = 20;
const int height = 20;
int x, y, fruitX, fruitY, score;
int tailX[100], tailY[100];
int nTail;
enum eDirection { STOP = 0, LEFT, RIGHT, UP, DOWN };
eDirection dir;
void Setup() {
gameOver = false;
dir = STOP;
x = width / 2;
y = height / 2;
fruitX = rand() % width;
fruitY = rand() % height;
score = 0;
}
void Draw() {
system("cls");
for (int i = 0; i < width + 2; i++)
cout << "#";
cout << endl;
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
if (j == 0)
cout << "#";
if (i == y && j == x)
cout << "O"; // Snake head
else if (i == fruitY && j == fruitX)
cout << "F"; // Fruit
else {
bool print = false;
for (int k = 0; k < nTail; k++) {
if (tailX[k] == j && tailY[k] == i) {
cout << "o"; // Snake tail
print = true;
}
}
if (!print)
cout << " ";
}
if (j == width - 1)
cout << "#";
}
cout << endl;
}
for (int i = 0; i < width + 2; i++)
cout << "#";
cout << endl;
cout << "Score:" << score << endl;
}
void Input() {
if (_kbhit()) {
switch (_getch()) {
case 'a':
dir = LEFT;
break;
case 'd':
dir = RIGHT;
break;
case 'w':
dir = UP;
break;
case 's':
dir = DOWN;
break;
case 'x':
gameOver = true;
break;
}
}
}
void Logic() {
int prevX = tailX[0];
int prevY = tailY[0];
int prev2X, prev2Y;
tailX[0] = x;
tailY[0] = y;
for (int i = 1; i < nTail; i++) {
prev2X = tailX[i];
prev2Y = tailY[i];
tailX[i] = prevX;
tailY[i] = prevY;
prevX = prev2X;
prevY = prev2Y;
}
switch (dir) {
case LEFT:
x--;
break;
case RIGHT:
x++;
break;
case UP:
y--;
break;
case DOWN:
y++;
break;
default:
break;
}
if (x >= width) x = 0; else if (x < 0) x = width - 1;
if (y >= height) y = 0; else if (y < 0) y = height - 1;
for (int i = 0; i < nTail; i++)
if (tailX[i] == x && tailY[i] == y)
gameOver = true;
if (x == fruitX && y == fruitY) {
score += 10;
fruitX = rand() % width;
fruitY = rand() % height;
nTail++;
}
}
int main() {
Setup();
while (!gameOver) {
Draw();
Input();
Logic();
Sleep(10); // Sleep to control the speed of the game
}
return 0;
}
This code provides a foundational structure for a console-based Snake Game in C++, using simple graphics represented by ASCII characters.
Understanding the Basics of the Snake Game
Game Concept
The Snake Game is a classic arcade-style game where players control a snake that moves around the screen, consuming food to grow longer while avoiding collisions with itself and the game boundaries. The goal is simple: eat as much food as possible without crashing. This engaging and straightforward concept provides a perfect opportunity for learning C++ programming through game development, as it covers various programming concepts including control structures, data types, and object-oriented design.
Setting Up Your C++ Environment
Before diving into coding the snake game in C++, ensure your development environment is ready. Depending on your preference, you can choose from popular Integrated Development Environments (IDEs) such as Visual Studio, Code::Blocks, or CLion.
For a simple console-based game, the `<conio.h>` library is typically used for handling keyboard input and sometimes even for drawing characters. Ensure that the library is supported in your selected IDE. To begin, create a new C++ project and set up your main file where you will write your code.
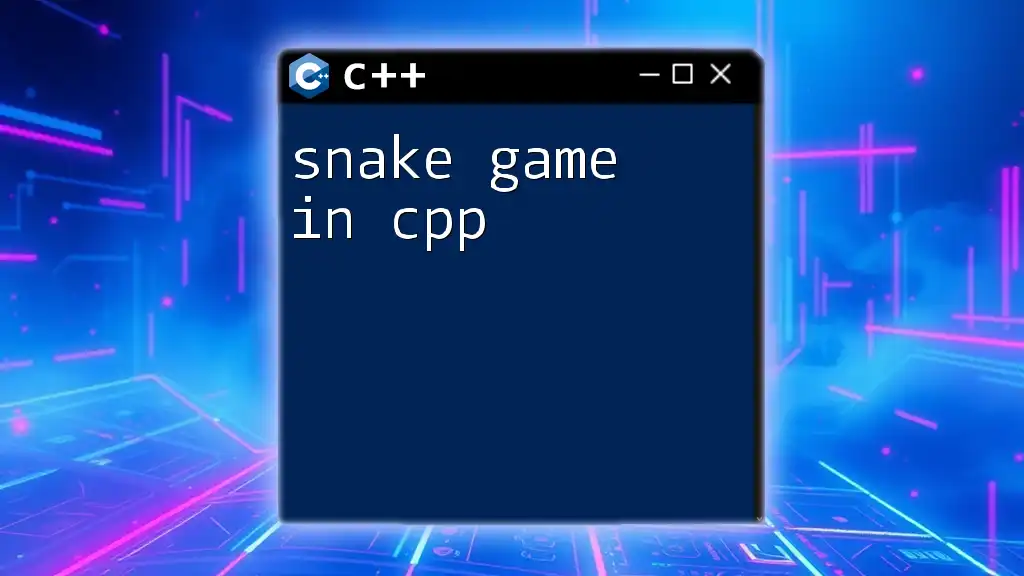
Game Development Fundamentals
Game Loop Structure
At the heart of every game lies the game loop, a continuous cycle that handles user input, processes game logic, and updates the display. This loop runs repetitively until the game ends. Here’s a basic skeleton of a game loop in C++:
while (gameIsRunning) {
// Handle input
// Update game state
// Render
}
This structure allows for a smooth gameplay experience as it ensures that player interactions are registered in real-time.
Handling User Input
User input is essential for controlling the snake's direction of movement. Using the `<conio.h>` library, you can capture key presses that dictate whether the snake moves up, down, left, or right. Here’s how you can implement input handling in your game:
if (_kbhit()) {
switch (_getch()) {
case 'w': // Move up
case 'a': // Move left
case 's': // Move down
case 'd': // Move right
}
}
By capturing keyboard events, you allow players to control their snake dynamically.
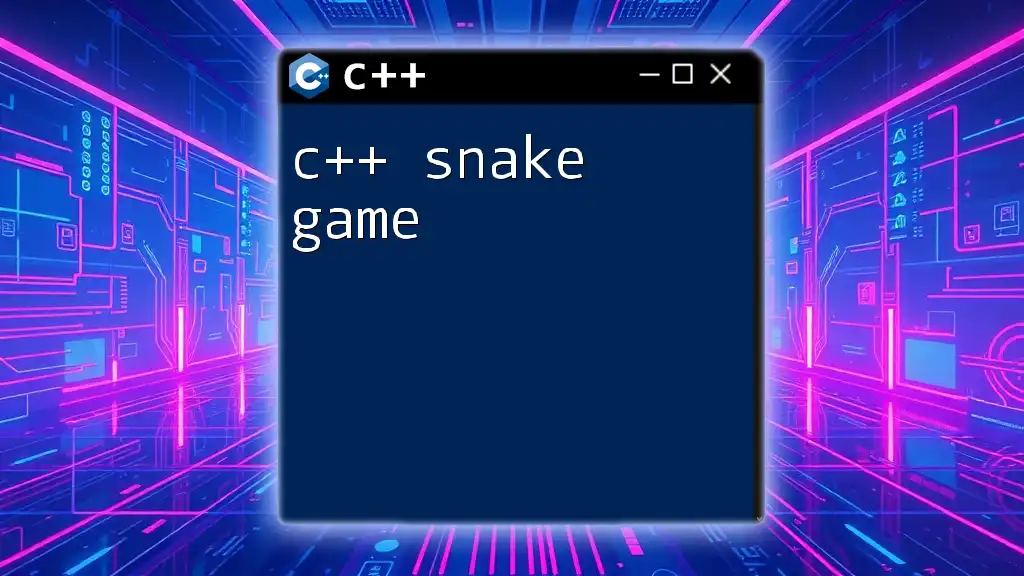
Game Components
Creating the Snake
In your implementation, the snake can be represented using a struct or a class that maintains its body as a series of positions. Leveraging a dynamic data structure such as a vector allows you to manage the snake's growth effectively. Here’s an example struct for the snake:
struct Position {
int x;
int y;
};
struct Snake {
std::vector<Position> body;
Direction currentDirection;
};
When the snake eats food, the body vector needs to expand to allow for growth, making this structure fundamental to gameplay.
Adding Food
The next important element is the food that the snake consumes. It is essential to generate food at random positions while making sure it doesn't spawn on the snake's body. Here’s a simple implementation for generating food:
Position generateFood(Snake& snake) {
Position food;
// Logic to avoid placing food on the snake's body
return food;
}
Implementing a method to check the validity of the food position is crucial to maintaining game integrity.
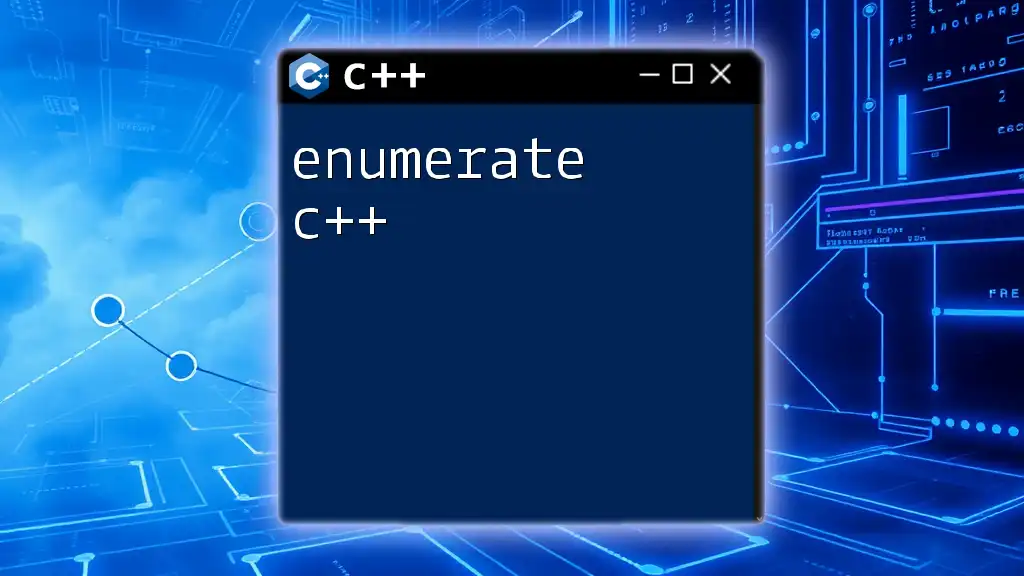
Game Logic
Collision Detection
One of the critical aspects of game design is collision detection. This logic checks if the snake collides with the screen boundaries or itself, leading to a game-over state. Here’s a basic collision detection function:
bool checkCollision(const Snake& snake) {
// Check if the head collides with walls or its body
}
This function plays a pivotal role in defining the end of the game, providing players with a satisfying challenge.
Scoring System
Keeping track of the player's score adds an element of competition and goals for the player to strive towards. When the snake consumes food, the score should increment. Implementing a simple scoring system can resemble the following:
int score = 0;
if (snake.eatFood(food)) {
score++;
}
Displaying the score on the screen will keep players engaged and motivated to improve.
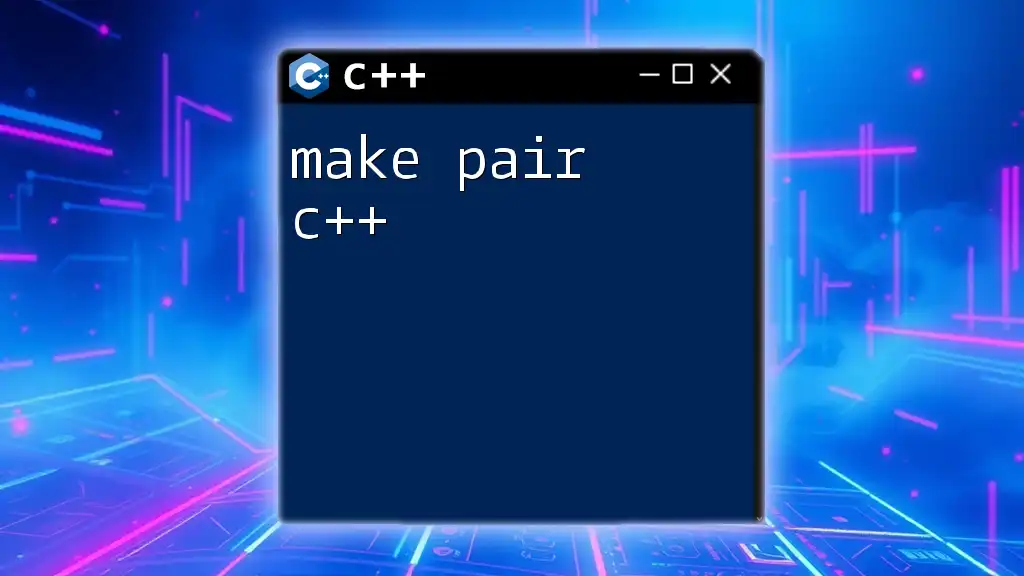
Rendering the Game
Drawing the Game Screen
Rendering the game visually is essential for engaging gameplay. In a console game, you can represent the snake and food using simple ASCII characters. Here’s a basic function to handle the visual representation:
void drawGame(const Snake& snake, const Position& food) {
// Clear screen and draw snake and food
}
This function will be called within the game loop to refresh the game state.
Updating the Display
In a console environment, you can clear the previous frame before rendering a new one. This could be accomplished with the following command:
system("cls"); // On Windows
By continuously updating the console output, you create a fluid gameplay experience.
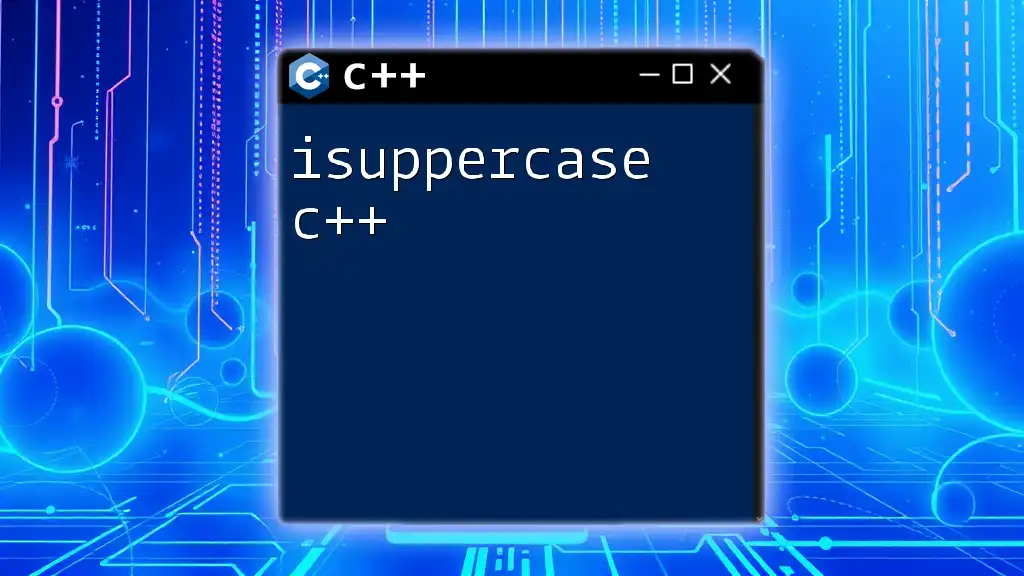
Putting It All Together
Code Structure Overview
Having discussed various components of the game, it's time to integrate them into a cohesive unit. The structure of your complete game might resemble:
int main() {
initializeGame();
while (gameIsRunning) {
handleInput();
updateGameState();
render();
}
return 0;
}
This main function orchestrates the flow of your game, integrating user inputs, game updates, and rendering.
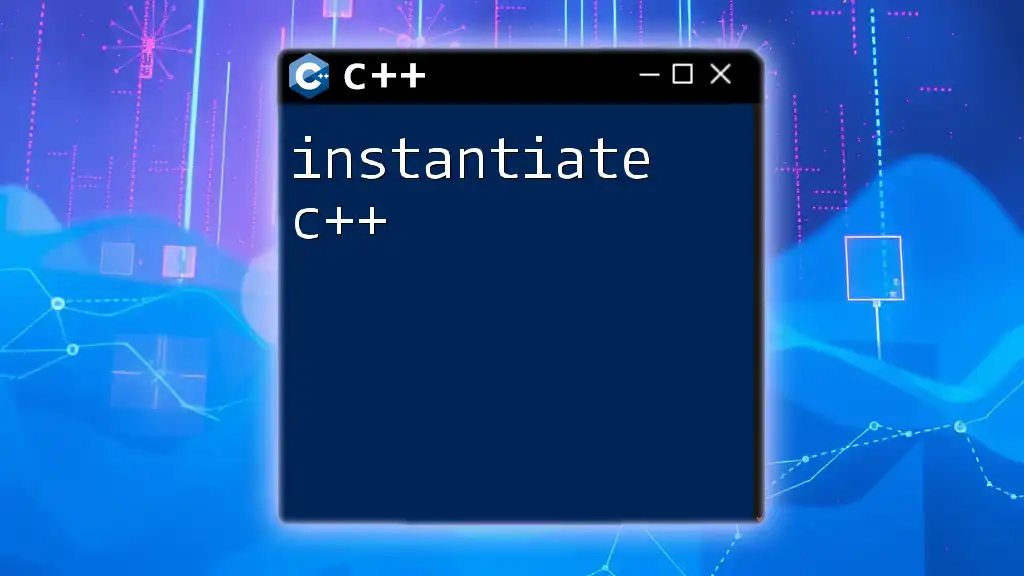
Expanding the Game
Adding Features
Once you have the fundamental game established, consider introducing additional features to enhance playability. This could include increasing the speed as the score rises, introducing obstacles, or providing power-ups. Each feature will not only improve gameplay but will also challenge your programming skills.
Optimizing the Code
Best coding practices play a vital role in creating an efficient game. Focus on organizing your code by dividing it into functions and classes. This approach not only makes your code cleaner but also more readable and maintainable in the long run.
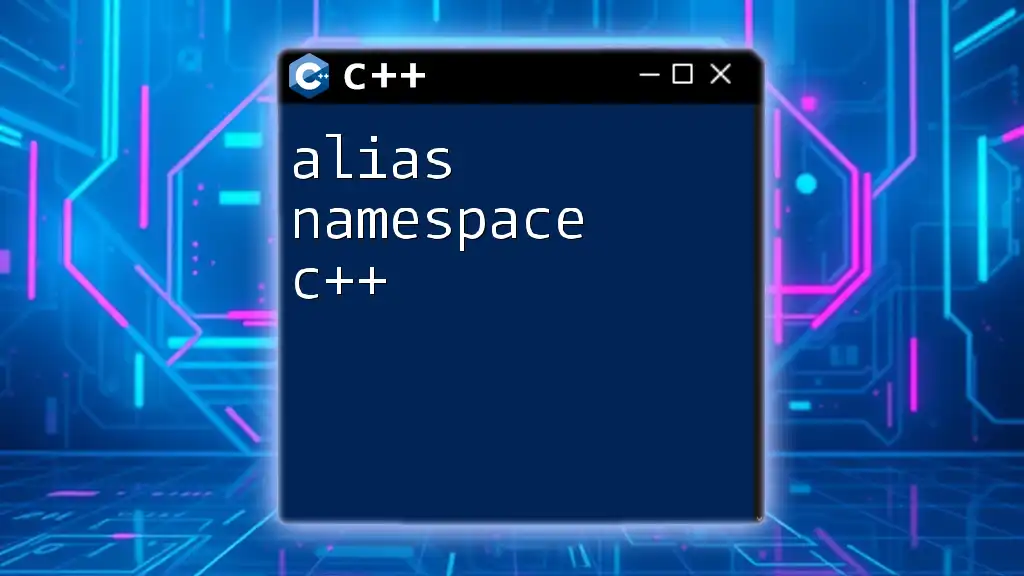
Conclusion
Through this snake game in C++, you have learned various crucial programming concepts, from managing user input to handling game logic and rendering graphics. With each component, you gain hands-on experience that will improve your coding proficiency. As you grow more comfortable with these concepts, challenge yourself to expand the game further, enhancing both your programming skills and your understanding of game development.
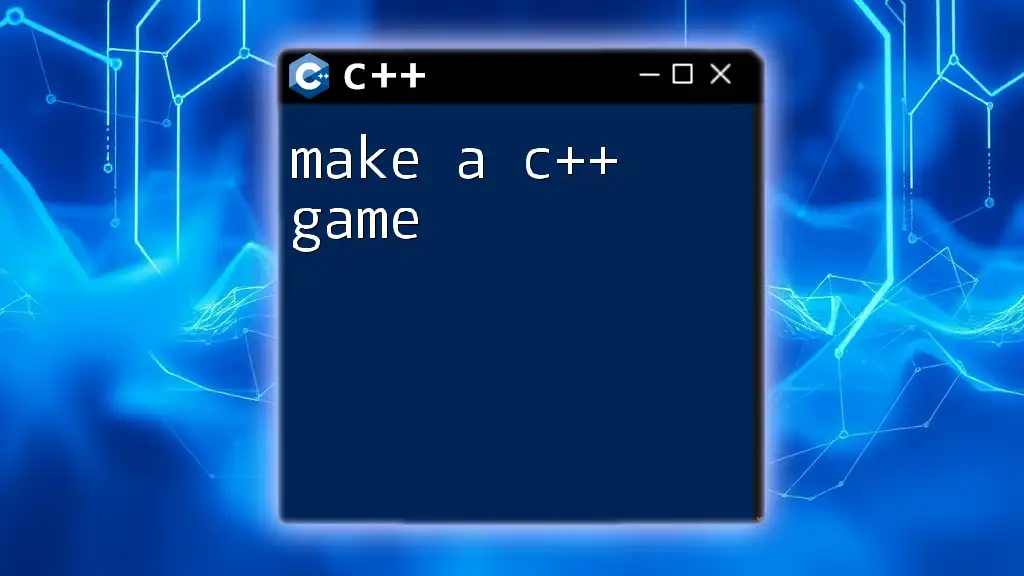
Additional Resources
To further aid your journey in mastering C++, consider exploring books focused on game programming and engaging with online forums or tutorials that cover advanced techniques and optimization strategies. Resources like Stack Overflow and GitHub can provide invaluable insights as you continue your coding adventures.