Neovim is a powerful text editor that enhances the C++ programming experience by providing features like syntax highlighting and efficient navigation, allowing developers to edit and manage their code seamlessly.
Here's a simple example demonstrating how to include a header file in a C++ program using Neovim:
#include <iostream>
int main() {
std::cout << "Hello, C++ with Neovim!" << std::endl;
return 0;
}
What is Neovim?
Neovim is a modernized, extensible version of the original Vim text editor. Its purpose is to provide a welcoming and efficient environment for developers, enhancing the experience with various features unavailable in the classic version. One of the key differences between Neovim and traditional IDEs is its lightweight design that allows for rapid startup and quick navigation, making it a favorite among developers who appreciate speed and efficiency.
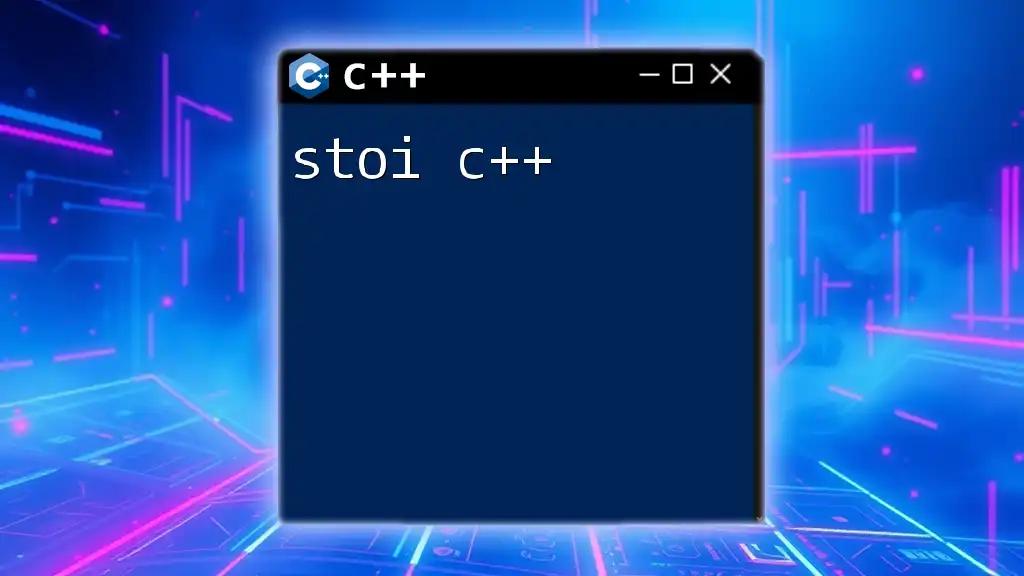
Why Choose Neovim for C++ Development?
Choosing Neovim for C++ development comes with numerous advantages:
- Lightweight and Fast: Neovim starts quickly and runs smoothly, making it ideal for rapid development cycles.
- Highly Customizable: With a plethora of plugins available, you can tailor Neovim to fit your specific workflow.
- Terminal Integration Options: Neovim operates well in terminal environments, enabling developers to switch seamlessly between coding and command-line tasks.
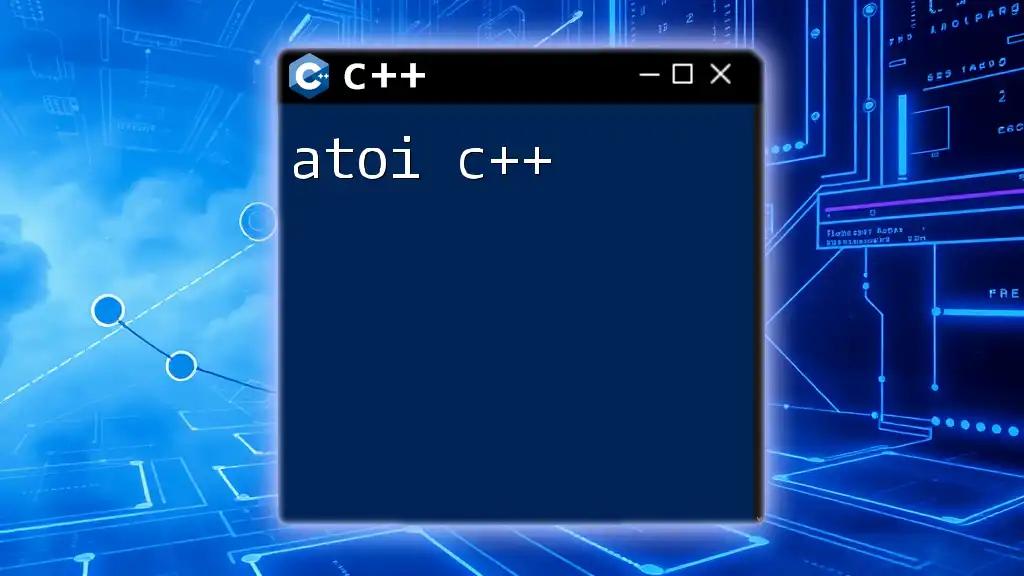
Setting Up Neovim for C++
Installing Neovim
To begin your journey with Neovim, the first step is downloading and installing it. Here’s how you can do this across different operating systems:
-
Linux: You can install Neovim using your package manager. For example, run the command:
sudo apt install neovim
-
macOS: Use Homebrew for installation:
brew install neovim
-
Windows: You can download pre-built binaries or use `scoop`:
scoop install neovim
Basic Configuration
After installing Neovim, it’s essential to configure it to suit your needs. The configuration file is located at `/.config/nvim/init.vim` or `/.config/nvim/init.lua` for Lua configuration. Here’s a simple example of what your `init.vim` might look like:
set nocompatible
set encoding=utf-8
set runtimepath+=~/.config/nvim
set number
syntax on
This configuration sets Neovim to use UTF-8 encoding, displays line numbers, and enables syntax highlighting, critical for coding in C++.
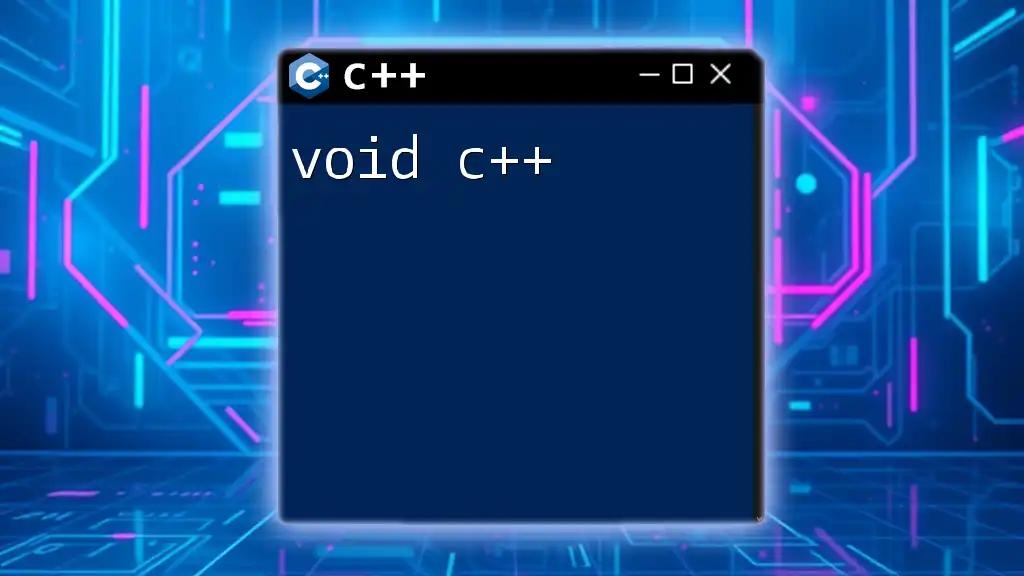
Essential Plugins for C++ Development
Package Management
Effective use of Neovim for C++ development heavily relies on plugins. The first step is to set up a plugin manager, with `vim-plug` being one of the most popular. To install `vim-plug`, add the following lines to your configuration file:
call plug#begin('~/.config/nvim/plugged')
call plug#end()
Recommended Plugins
-
LSP (Language Server Protocol): Language Server Protocol provides language-related features like autocompletion, go-to definitions, and more. For C++, consider using `coc.nvim` for a comprehensive experience.
-
Syntax Highlighting and Formatting: Enhance your coding experience with plugins such as `vim-cpp-enhanced-highlight` for better syntax highlighting and `clang-format` for code formatting.
-
File Navigation and Search: Efficient file management is crucial. Utilize `fzf.vim` for fuzzy file searching and `nerdtree` for easy navigation through your project's directory structure. Here's a snippet to integrate `fzf.vim`:
Plug 'junegunn/fzf', { 'do': { -> fzf#install() } }
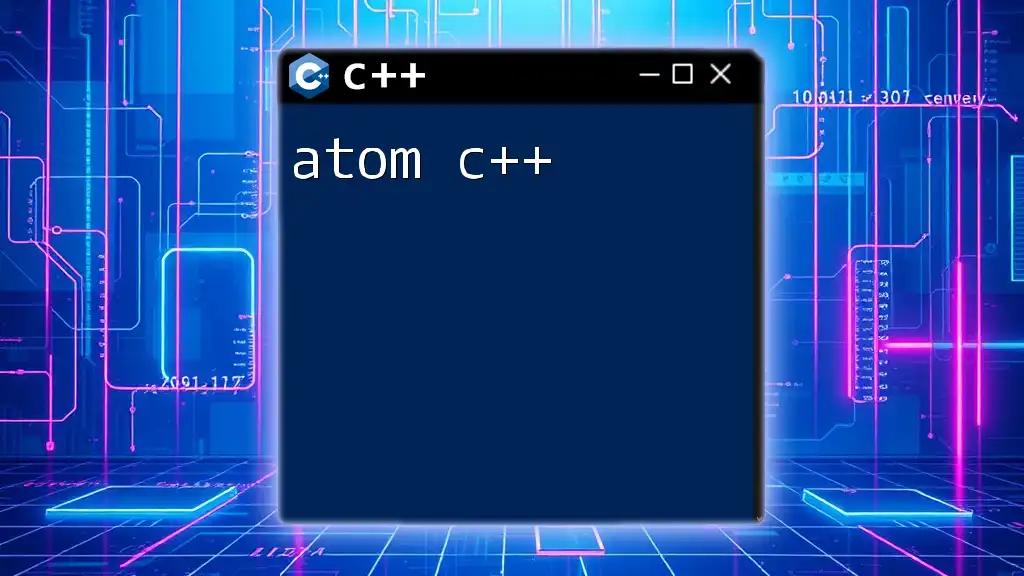
Coding in C++ with Neovim
Creating a C++ Project
When working on a C++ project, organization is key. A conventional directory structure enhances maintainability. Below is an example of a well-organized structure:
MyProject/
├── src/
├── include/
├── build/
└── main.cpp
Writing Your First C++ Program
Once your structure is in place, you can write your introductory C++ program. Here’s a simple 'Hello World' example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Compiling and Running Code
To compile your C++ program conveniently, you can use a `Makefile`. Below is a basic example for compiling your project:
all: main
main: main.o
g++ main.o -o main
main.o: main.cpp
g++ -c main.cpp
With this Makefile, you can simply run `make` in the terminal to compile your project.
Debugging in Neovim
Debugging adds another layer of efficiency to your development process. You can utilize `vimspector` which serves as a debugging interface. After installing, you can set breakpoints and inspect variables with simple commands tailored to your workflow.
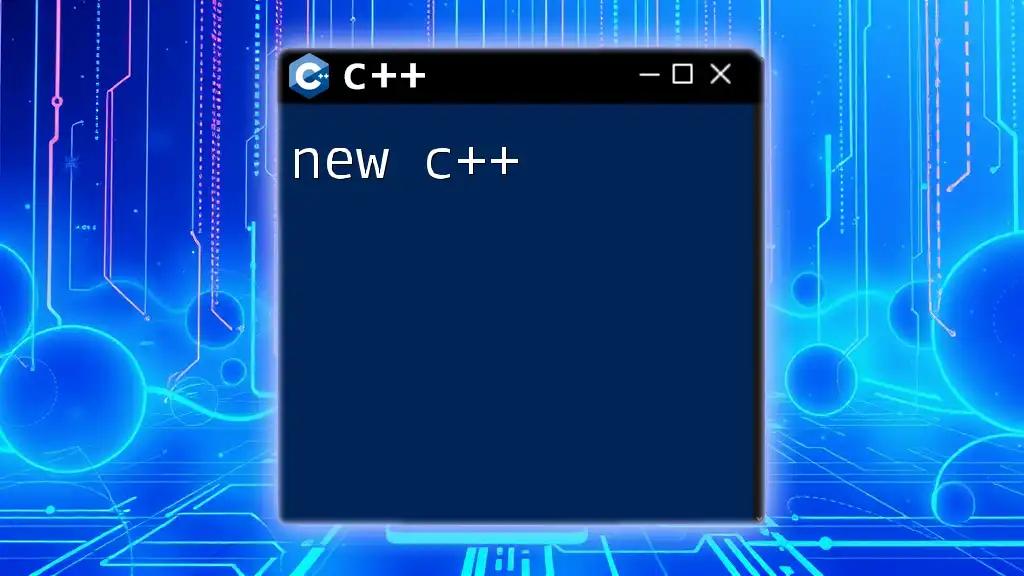
Advanced Features
Custom Keybindings
To optimize your workflow, consider creating custom key mappings. For instance, you can map a key to quickly run your C++ program after saving it:
nnoremap <leader>r :w<CR>:!./main<CR>
Snippets Management
Snippets can significantly speed up your coding process. Integrate snippet managers like `UltiSnips` or `LuaSnip` to handle C++ code snippets effectively. Here’s a basic configuration using `UltiSnips`:
Plug 'SirVer/ultisnips'
You can then create a snippet for commonly used C++ structures, allowing for quicker coding.
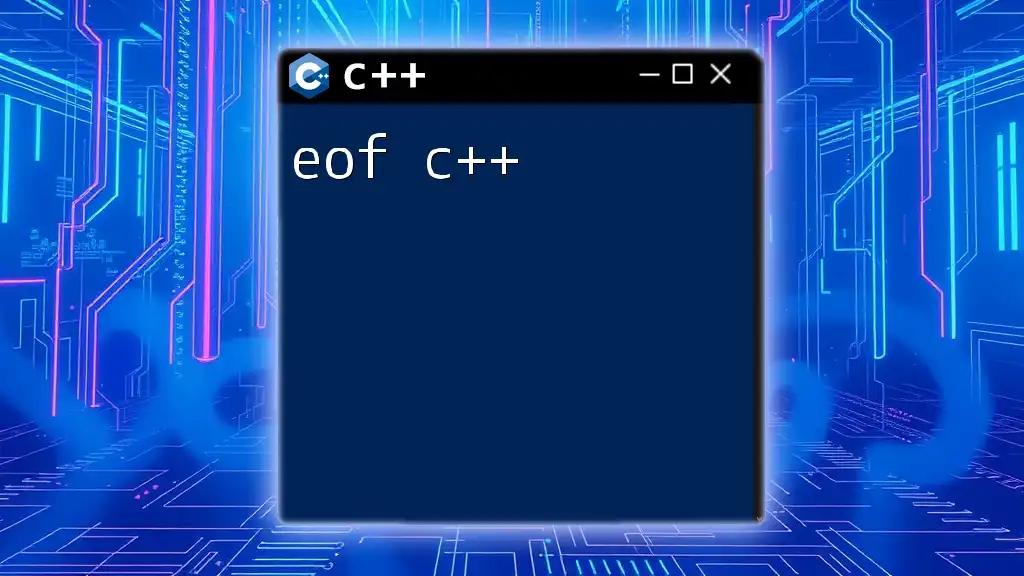
Conclusion
Using Neovim for C++ development offers a blend of efficiency and customizability. With its lightweight nature, advanced plugin support, and responsive environment, Neovim remains an excellent choice for developers looking to enhance their productivity.
Next Steps
Now that you have the basics of Neovim and C++ development at your fingertips, I encourage you to explore more plugins and custom configurations. Engaging with the community can also provide insights and tools that will further refine your development experience.
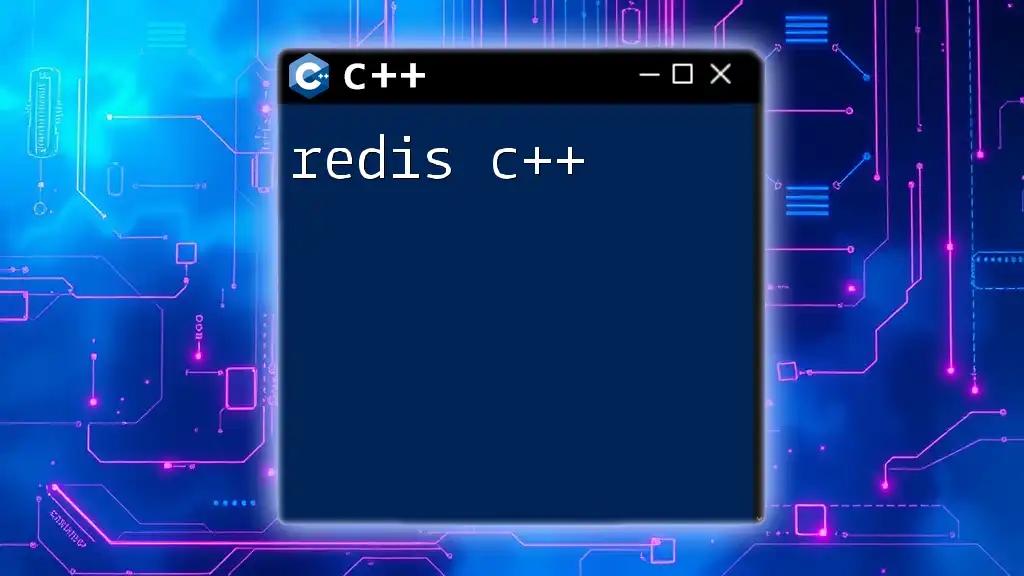
Additional Resources
To continue your journey, consider checking out the following documentation and resources for Neovim and C++ development:
- Neovim's official documentation
- Online C++ programming resources
- Community-driven forums for both Neovim and C++ development
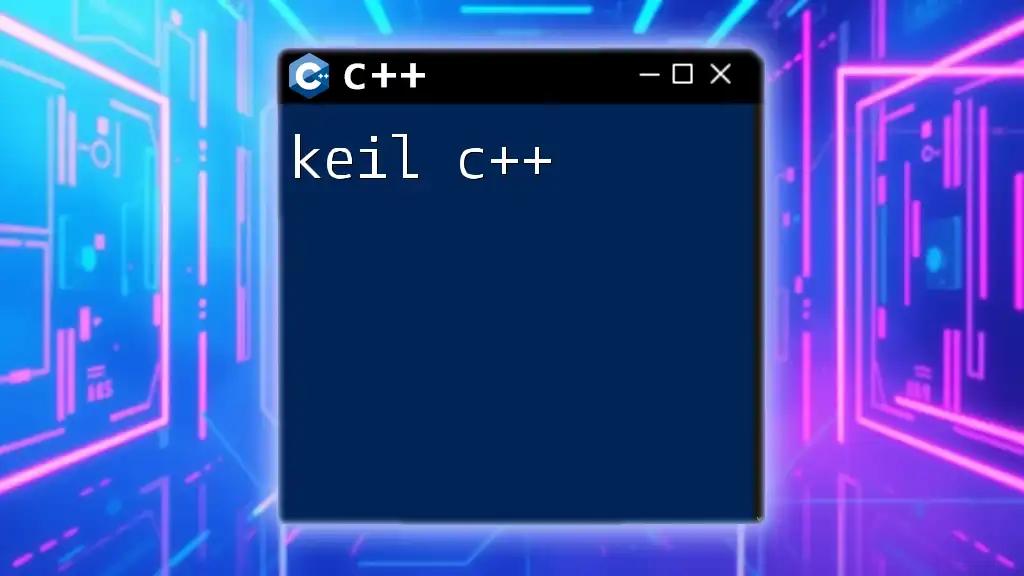
Call to Action
I invite you to engage with the Neovim and C++ communities. Share your experiences and learn from others to unlock even greater potential in your coding journey.