`iomanip` in C++ is a header file that provides options for formatting output, such as setting the width, precision, and filling characters for output streams.
Here’s a code snippet demonstrating the use of `iomanip` to format output:
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159;
std::cout << std::fixed << std::setprecision(2) << "Value of Pi: " << pi << std::endl;
return 0;
}
What is iomanip?
The iomanip library in C++ stands for "input/output manipulator." It provides a suite of tools designed to manipulate data formatting in output streams (like `std::cout`) and input streams (like `std::cin`). This is incredibly important for presenting data in a user-friendly manner, especially when dealing with numerical values, aligning text, or handling decimal points.
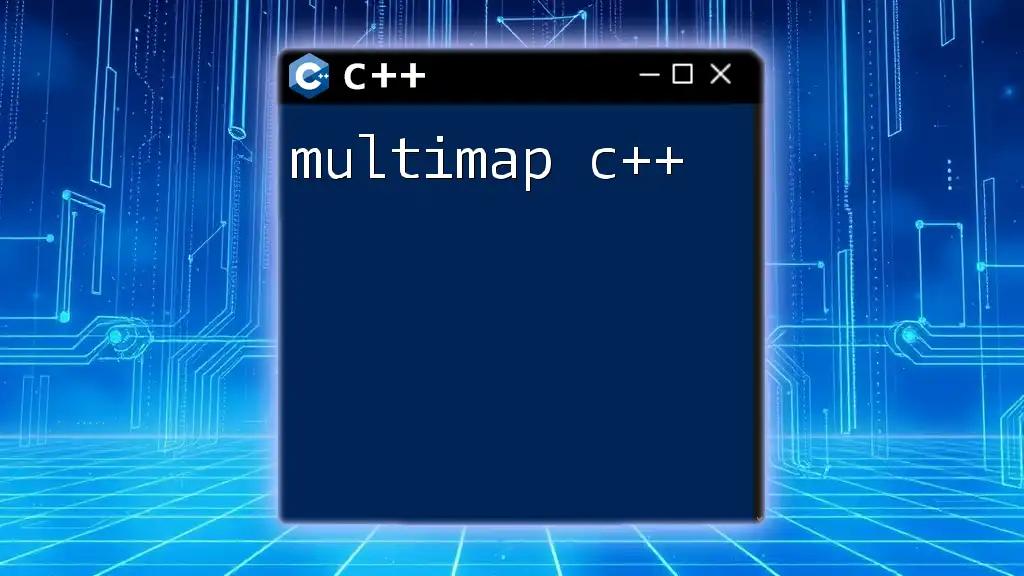
Understanding the Basics
What are Manipulators?
Manipulators in C++ are special functions or objects that can change the state of an input or output stream. They primarily serve to adjust the formatting of data being read or written. For instance, if you want to control how floating-point numbers are displayed (in fixed-point notation or scientific notation), you would use manipulators.
The Role of the iomanip Library
To utilize the features of the iomanip library, the first step is including it in your code with:
#include <iomanip>
This inclusion unlocks a variety of formatting capabilities that can enhance and refine your input and output operations significantly.
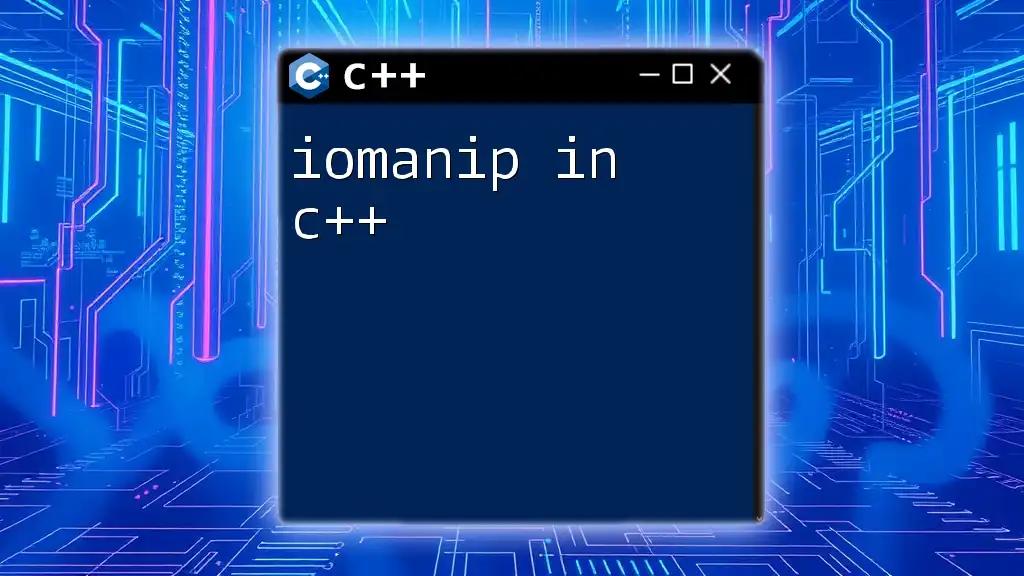
Essential iomanip Functions
Setting Output Precision
One of the most used manipulators in the iomanip c++ library is std::setprecision. This function allows developers to define the number of digits displayed after the decimal point when dealing with floating-point numbers. This is particularly useful for applications requiring high precision, such as scientific computations.
For example:
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159;
std::cout << std::setprecision(3) << pi << std::endl; // Output: 3.14
return 0;
}
In this example, the output is restricted to three significant digits, demonstrating how precision can concisely communicate numerical data.
Controlling Width
Another valuable manipulator is std::setw. This function sets the width of the next output field, which is useful for creating well-aligned output when displaying tabular data.
Here’s how it works:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setw(5) << 42 << std::endl; // Right-aligns output
return 0;
}
In this code, the number `42` is displayed in a field that is five spaces wide, right-aligned by default. This is particularly beneficial when aligning columns of data, making it easier for users to read.
Formatting Output Alignment
The use of std::left, std::right, and std::internal manipulators allows for custom text alignment in your console output. This feature is essential when you want your output to maintain a specific format, improving readability.
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::left << std::setw(10) << "Left"
<< std::right << std::setw(10) << "Right" << std::endl;
return 0;
}
In this example, the text "Left" is positioned to the left of the field, while "Right" is right-aligned. This capability is vital when displaying labels or columns of data where alignment matters for easy user parsing.
Fixing Decimal Points
With the manipulators std::fixed and std::scientific, developers can control the format for floating-point numbers. The std::fixed manipulator ensures that numbers are printed in fixed-point notation.
Here is an example:
#include <iostream>
#include <iomanip>
int main() {
double num = 12345.6789;
std::cout << std::fixed << std::setprecision(2) << num << std::endl; // 12345.68
return 0;
}
In this snippet, the expected output is `12345.68`, where the number is rounded to two decimal places. std::scientific would change the output format to scientific notation, helping in situations involving extremely large or small numbers.
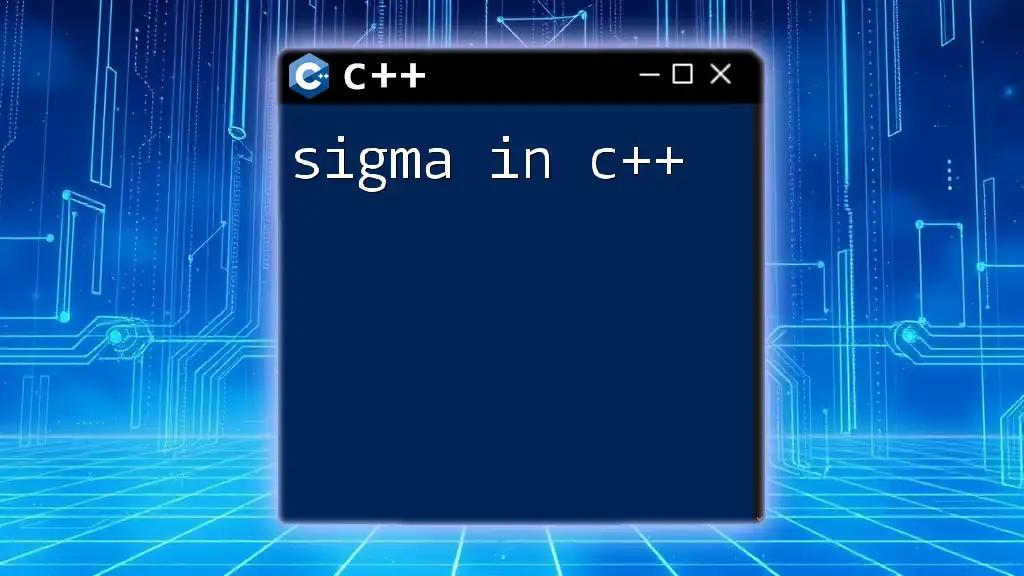
Advanced Usage of iomanip Functions
Mixing Manipulators for Custom Formatting
The true power of the iomanip c++ library comes alive when combining various manipulators. You can mix std::setw, std::setprecision, and others to create a tailored output format that meets specific needs.
#include <iostream>
#include <iomanip>
int main() {
double price = 19.99;
std::cout << "Price: " << std::fixed << std::setprecision(2)
<< std::setw(7) << price << std::endl; // Price: 19.99
return 0;
}
Here, the formatted output displays `Price: 19.99`, where the numerical value is precisely aligned and rounded to two decimal points, showing how these manipulators can intersect seamlessly for refined output.
Input Formatting with iomanip
Moreover, the iomanip library also allows manipulation during input operations. From handling decimal points to controlling the number format, you can ensure that your input validation is robust and straightforward.
For instance:
#include <iostream>
#include <iomanip>
int main() {
double value;
std::cout << "Enter a value: ";
std::cin >> std::fixed >> value; // input expects fixed decimal
std::cout << "You entered: " << value << std::endl;
return 0;
}
Utilizing std::fixed in the input stream, the code specifies that the input should be treated as a fixed-point decimal, ensuring consistency in user input expectations.
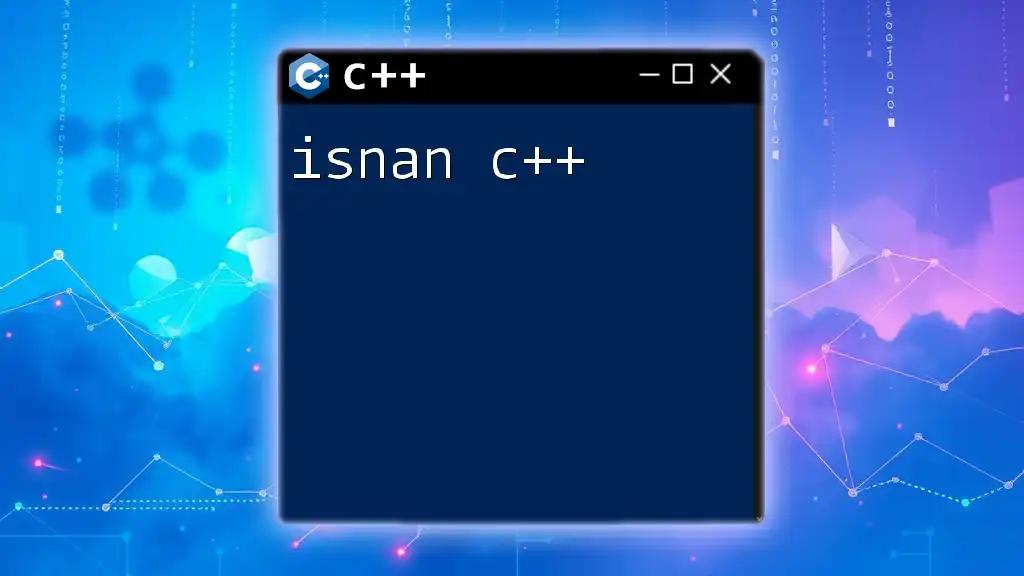
Best Practices for Using iomanip
Common Mistakes to Avoid
When using the iomanip library, it's easy to overlook the importance of manipulating the state of streams correctly. Beginners may forget to apply manipulators immediately or get confused between fixed and scientific formatting.
- Always remember to manipulate either `std::cout` or `std::cin` immediately after the respective object rather than applying them elsewhere.
- Check whether your manipulations are affecting the intended line of output—confusion often arises when you inadvertently affect formatting across multiple lines.
Performance Considerations
While the iomanip library is incredibly useful, excessively chaining manipulators can have performance implications, especially in tight loops or performance-critical applications. To mitigate this:
- Minimize the use of manipulators in performance-sensitive parts of your code, and consider directly formatting strings or outputs where necessary.
- If performance is crucial, opt for formatted streams only when required after necessary calculations, rather than in every instance of output.
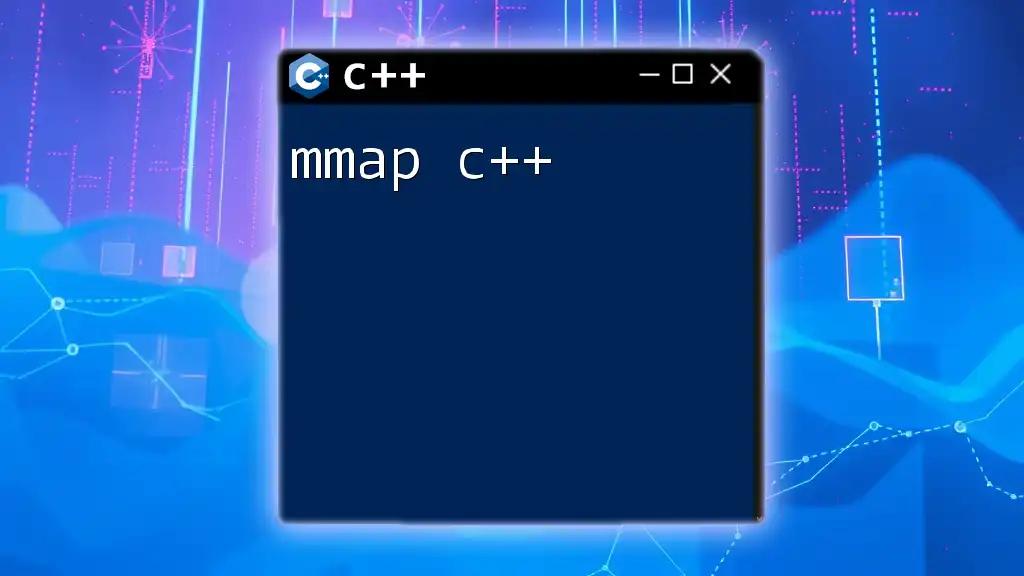
Conclusion
The iomanip c++ library stands as an essential tool for any C++ programmer, providing flexible options to manipulate input and output for better readability and user experience. By utilizing manipulators like std::setprecision, std::setw, std::fixed, and aligning text output, you can significantly improve the clarity of data presentation. Don't hesitate to dive deeper and experiment with these functions to suit your specific needs.