The `isnan` function in C++ is used to check if a given floating-point number is 'Not-a-Number' (NaN), returning `true` if it is and `false` otherwise.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <cmath>
int main() {
double value = std::nan(""); // Example of NaN
if (std::isnan(value)) {
std::cout << "The value is NaN" << std::endl;
} else {
std::cout << "The value is a valid number" << std::endl;
}
return 0;
}
What is isnan in C++?
The `isnan` function in C++ serves as a crucial tool for identifying situations where a floating-point value is Not a Number (NaN). Working with floating-point arithmetic can often lead to unexpected results due to operations that do not yield defined numerical results. This is where `isnan` steps in, allowing programmers to effectively check for NaN values that could otherwise lead to erroneous program behavior.

Why use isnan in C++?
Using `isnan` is essential in various scenarios, especially in mathematical computations. Here are some reasons why it is important:
-
Understanding Floating-Point Arithmetic: Floating-point types can represent real numbers but are prone to rounding errors and special values like infinity and NaN. Being aware of these issues is crucial for maintaining precision in calculations.
-
Common Scenarios for NaN: NaN can arise from multiple mathematical operations, including division by zero or invalid mathematical operations. Failure to handle these cases can lead to incorrect program outputs or crashes.
-
Importance in Mathematical Operations: Checking for NaN before performing operations helps prevent further errors in calculations that depend on the validity of numerical values.
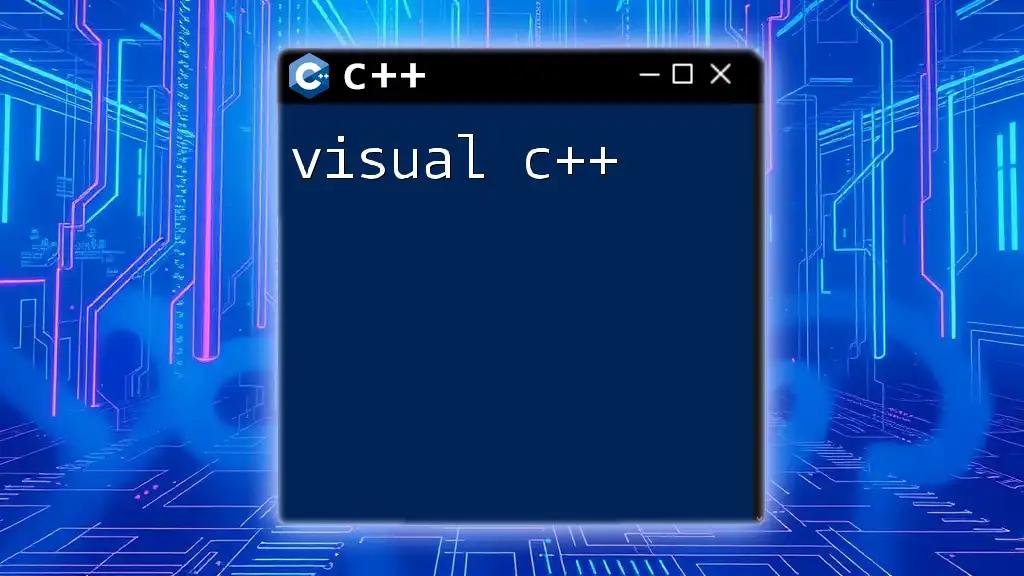
Understanding NaN (Not a Number)
What is NaN?
NaN is a special value in computing that represents undefined or unrepresentable values, especially in floating-point calculations. For instance, the result of dividing zero by zero is not a defined number; hence, it returns NaN instead.
How NaN is Generated
Several scenarios can lead to the generation of a NaN value:
-
Division by Zero: Attempting to divide a number by zero yields NaN.
-
Undefined Mathematical Operations: Operations like `sqrt(-1)` or `log(0)` can also result in NaN.
-
Invalid Inputs from Functions: Functions that fail to produce a valid number due to invalid inputs can return NaN as a result.
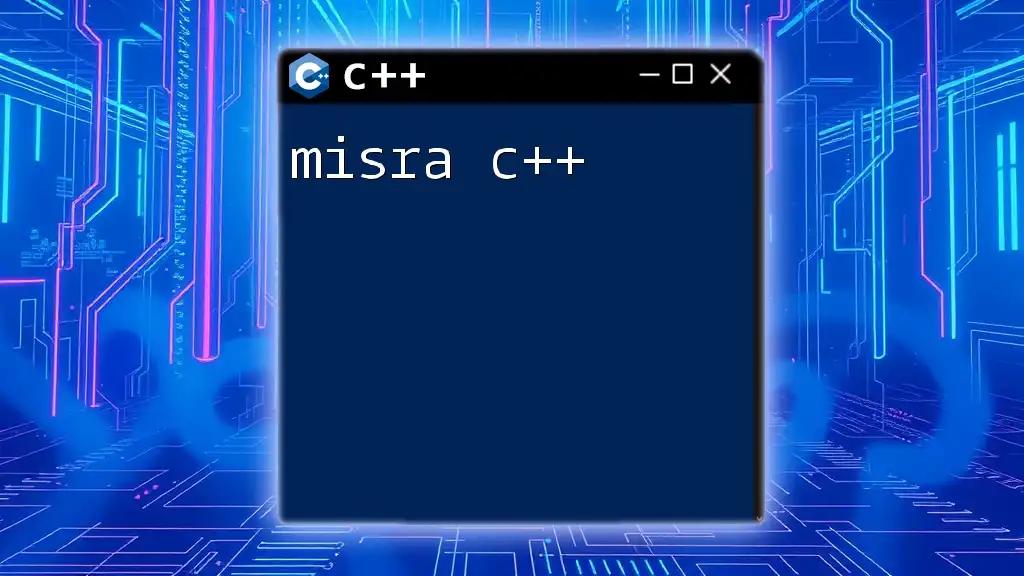
Using isnan in C++
Syntax of isnan
To utilize `isnan`, you must understand its syntax. The function prototype looks like this:
#include <cmath>
bool isnan(double value);
The function takes a single parameter — a `double` value — and returns `true` if the value is NaN; otherwise, it returns `false`.
Importing the Required Library
To use `isnan`, it is imperative to include the `cmath` library in your C++ program, as this library provides mathematical functions alongside `isnan`. Here’s how to include it:
#include <cmath>
Example of Using isnan
Here's a simple example that demonstrates the use of `isnan` to check for NaN values:
#include <iostream>
#include <cmath>
int main() {
double value = 0.0 / 0.0; // Generates NaN
if (std::isnan(value)) {
std::cout << "The value is NaN." << std::endl;
} else {
std::cout << "The value is valid." << std::endl;
}
return 0;
}
In this example, the program performs the division of zero by zero, which results in NaN. The `isnan` function is then utilized to confirm this value and outputs the result accordingly.
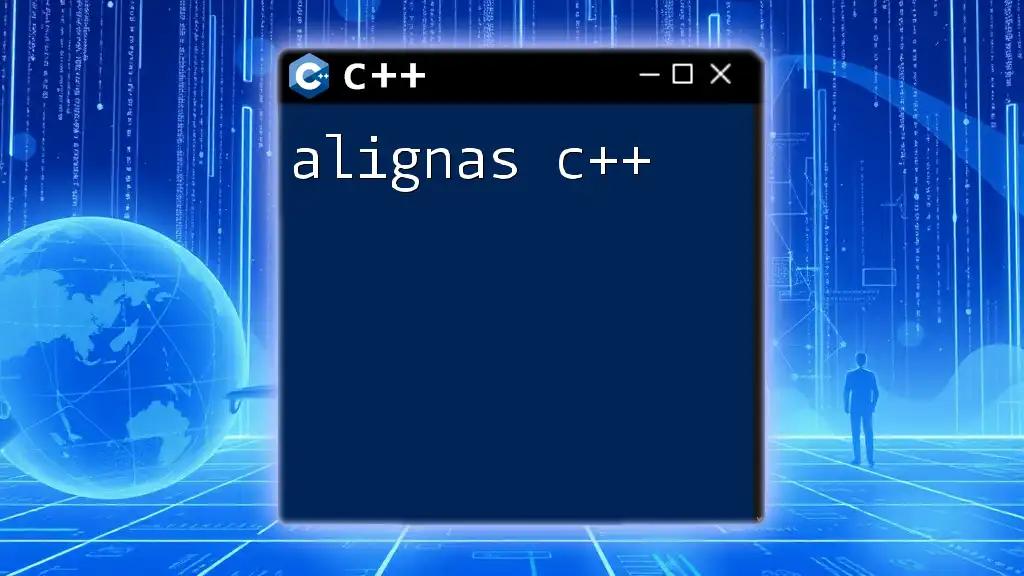
Handling NaN in C++
Common Mistakes when Using isnan
When using `isnan`, programmers often make several mistakes, such as:
-
Misinterpretation of NaN in Comparisons: NaN is not equal to itself, which can lead to frustrating logic errors if comparisons are improperly used. It’s better to use `isnan` instead of direct comparisons in such cases.
-
Using isnan Without Error Handling: Simply detecting NaN without a follow-up plan can result in more profound problems in the code. Having a coherent error-handling strategy is essential.
Best Practices for Checking NaN
To utilize `isnan` effectively in your code, consider these best practices:
-
Use isnan in Conditional Statements: Always wrap `isnan` checks within conditional statements. This ensures you can manage the flow of your program based on NaN checks.
-
Avoid Using Equality Comparisons with NaN: Instead of using comparison operators, always rely on `isnan` to determine if a floating-point number is NaN.
Contingency Operations on NaN
Dealing with NaN requires implementing alternative operations or fallbacks:
double safeDivision(double a, double b) {
if (b == 0) {
return 0; // Default value or error handling
}
return a / b;
}
In this `safeDivision` function, the implementation checks if the divisor is zero before proceeding, thus preventing the generation of NaN.
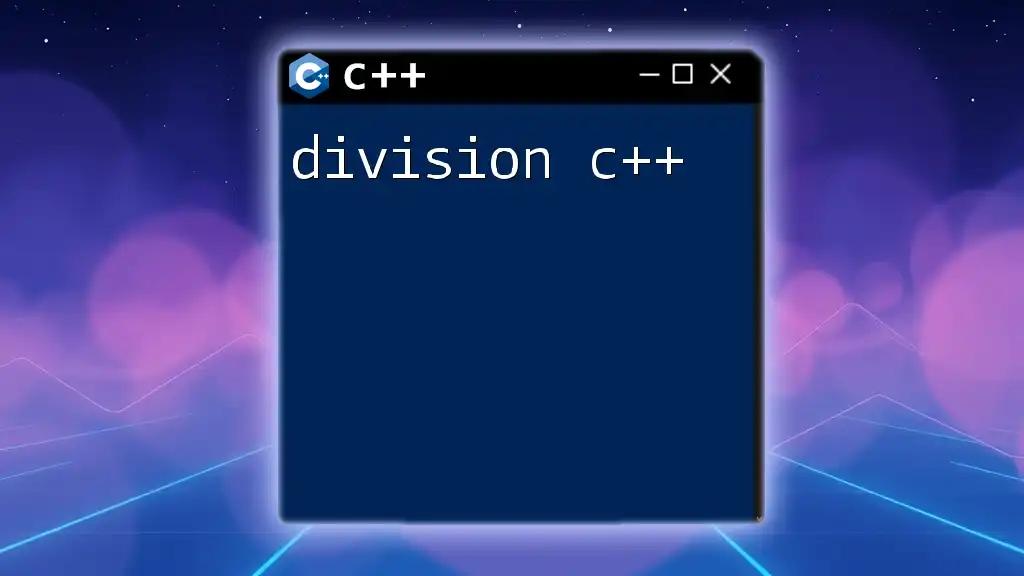
Performance Considerations
Is isnan Function Overhead
While `isnan` adds safety, it also introduces some performance overhead. When dealing with high-frequency computations, such as those in real-time simulations, this overhead might be a consideration. Employing `isnan` judiciously is key, especially where performance is critical.
When to Prioritize Performance Over Safety
In scenarios where performance is paramount, and input values can be controlled, developers might consider omitting `isnan` checks for critical paths. However, trades-offs in program safety and correctness must always be evaluated carefully.
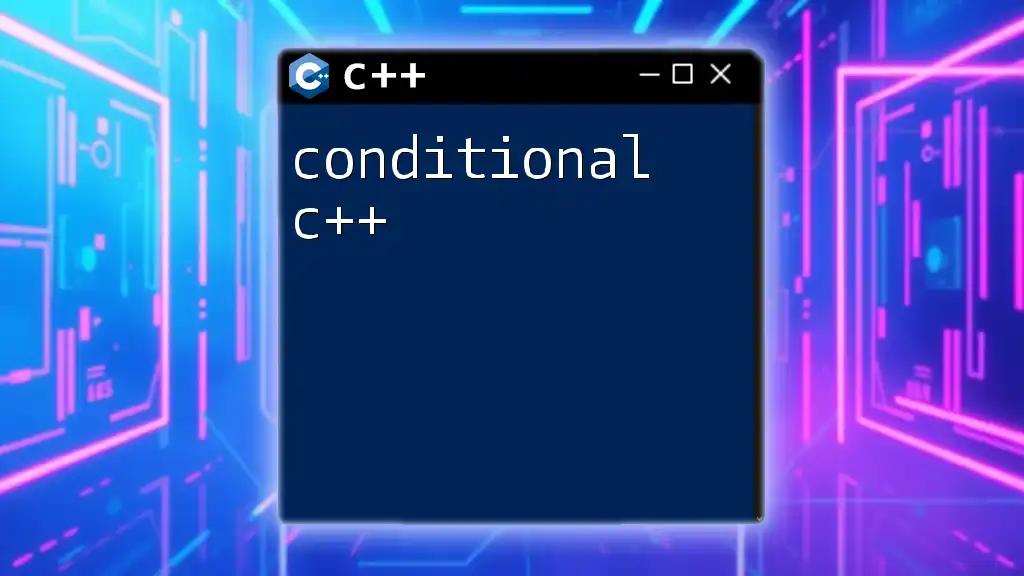
Real-world Applications of isnan
Use Cases in Data Science
In data analysis and science, NaN values are common in datasets. Using `isnan` allows data scientists to cleanse their data effectively, ensuring that only valid numerical entries are operated upon, which enhances the reliability of statistical analyses.
Use Cases in Graphics Programming
In graphics programming, NaN values can corrupt rendering calculations. For instance, when calculating vertex positions in 3D modeling, encountering NaN can lead to artifacts or unstable visuals on-screen. Utilizing `isnan` can help ensure visual integrity by managing these potential cases correspondingly.
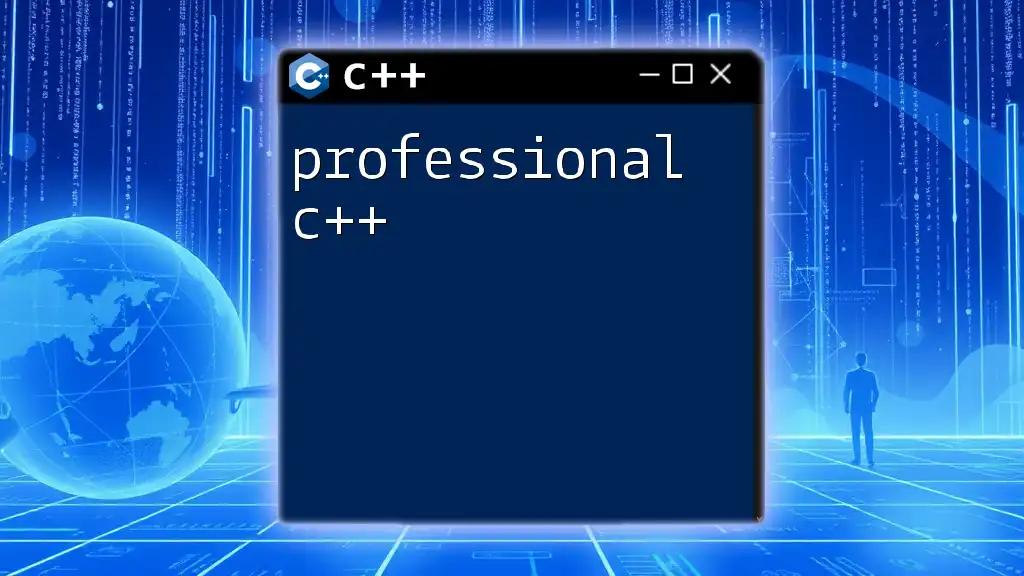
Conclusion
In summary, the `isnan` function in C++ is an invaluable tool when dealing with floating-point operations. It allows programmers to check for invalid numerical data efficiently, making their applications more robust and error-resistant. Understanding and applying `isnan` correctly can prevent many common pitfalls, ensuring more consistent and reliable program behavior. Now it is time to practice using this function and become proficient in utilizing the myriad of commands that C++ offers for efficient programming.
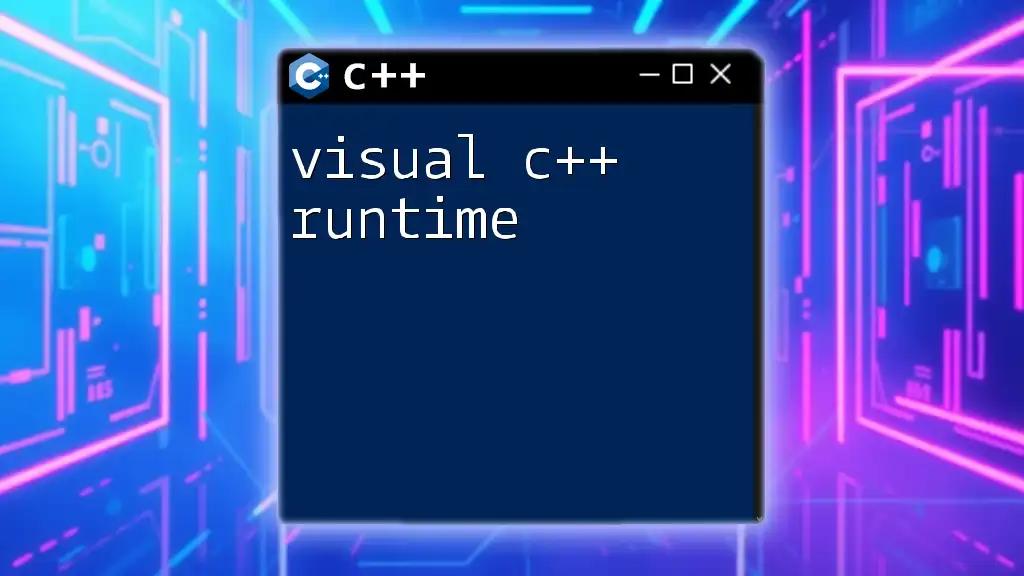
Additional Resources
To further enhance your understanding of `isnan` and related floating-point topics, consider exploring some of the recommended readings available. Also, if you have any questions about the nuances of `isnan` or its application, refer to the frequently asked questions section for more insights.