The `srand` function in C++ is used to seed the pseudo-random number generator to ensure that the sequence of random numbers generated by `rand` is different each time a program is run.
#include <cstdlib>
#include <ctime>
int main() {
srand(static_cast<unsigned int>(time(0))); // Seed the random number generator
int randomValue = rand(); // Generate a random number
return 0;
}
What is Random Number Generation in C++
Randomness in programming is a critical concept, particularly in fields like gaming, simulations, and statistical analysis. Random number generation (RNG) allows you to create values that exhibit unpredictability. This can enhance user experience in games, ensure unbiased outcomes in simulations, and add variability in scenarios where uniformity could skew results.
A pivotal aspect of RNG is the seed value. In computer science, a seed value initializes the random number generator, determining its starting point. By carefully choosing these seed values, developers can influence the sequence of numbers that the random number generator produces.
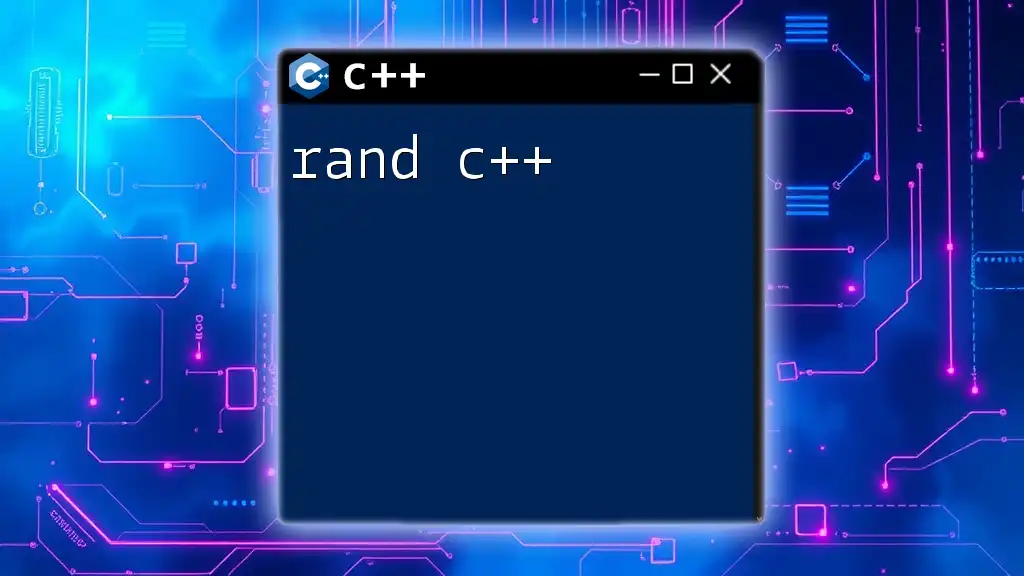
Understanding `srand` Function
Overview of `srand`
The function `srand` stands for “seed random” and plays an essential role in the initialization of the pseudo-random number generator used in C++. It ensures that the random numbers generated by the `rand` function start from a defined point, allowing for greater control over randomness.
The Syntax of `srand`
The syntax for using `srand` is straightforward:
void srand(unsigned int seed);
- Parameter: The `seed` is a value that the random number generator uses to begin generating the sequence of random numbers.
- Return Type: It does not return a value.
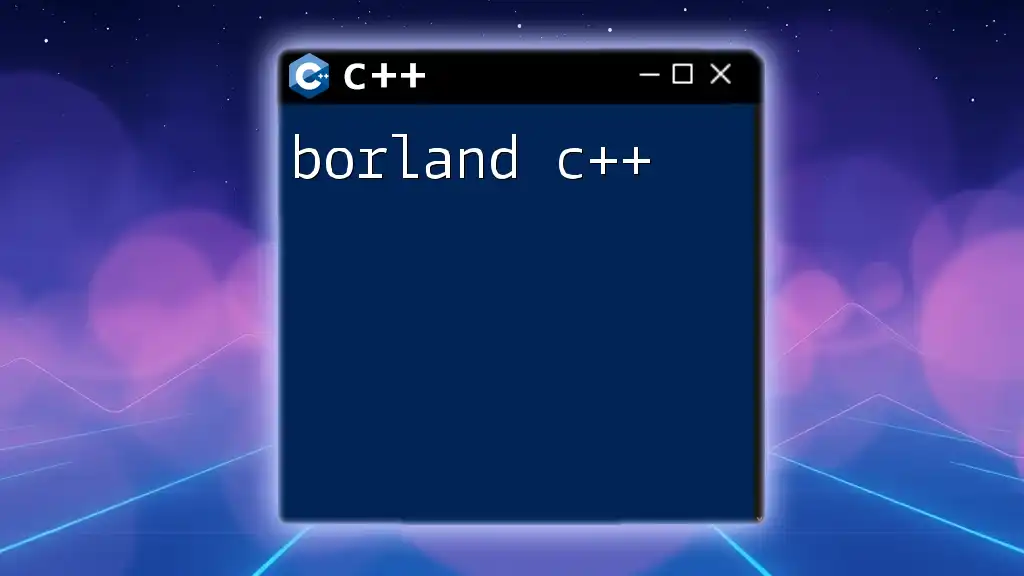
The Role of `srand` in Generating Random Numbers
Seeding the Random Number Generator
To effectively use `srand`, developers typically set the seed based on varying criteria—most commonly, the actual current time. This method guarantees a different starting point for every execution, thus leading to unique sequences of random numbers every time the program runs. Here’s an illustrative example:
#include <iostream>
#include <cstdlib> // for srand and rand
#include <ctime> // for time
int main() {
srand(static_cast<unsigned int>(time(0))); // Seed with current time
std::cout << rand() << std::endl; // Outputs the first random number
return 0;
}
In this example, using the `time(0)` function provides a continuously changing seed each second, leading to the generation of different random numbers across different executions of the program.
Repeated Executions and Randomness
It’s essential to note that if you run a program without reseeding `srand`, you will receive the same sequence of numbers each time. For example, if you were to initialize `srand(1);` and run the program multiple times, each execution would yield identical outputs from `rand()`. This behavior underscores the importance of using unique seed values to achieve true randomness.
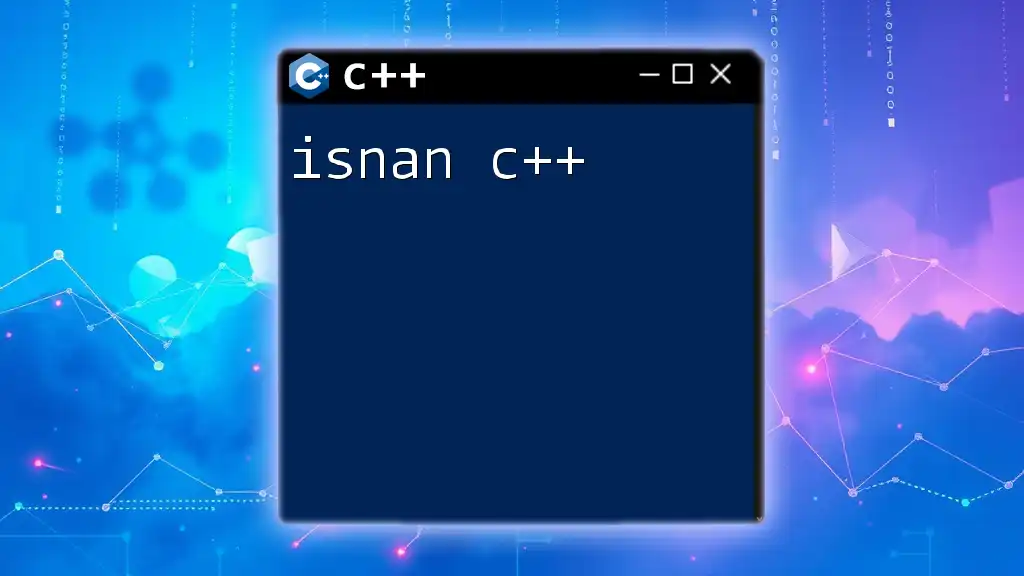
How to Use `srand` Effectively
Best Practices in Seeding
When using `srand`, the most effective approach is to utilize an ever-changing source like the system time. For instance:
srand(static_cast<unsigned int>(time(0))); // Dynamic seed
However, it is crucial to avoid static seeding, such as:
srand(1); // Weak seed choice
This choice results in predictable sequences, making the outputs less random.
Multiple Random Number Generators
When using more than one random number generator within a program, ensure that each has a unique seed. This is vital to prevent conflicts that can arise from sharing seed values between different instances. Separating the seeds can help maintain distinctive output across various random number generators.
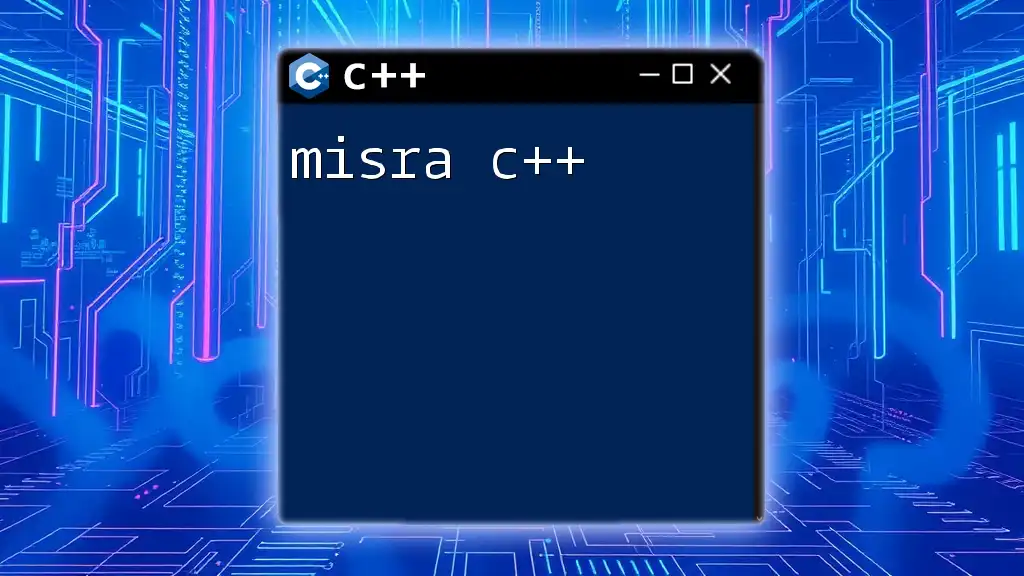
Functions Related to `srand`: Interaction with `rand`
Overview of `rand` Function
The `rand` function is the main function used to generate random numbers in C++. It provides integers within a fixed range (typically from 0 to `RAND_MAX`). Here’s a simple implementation:
std::cout << rand() % 100 << std::endl; // Generates a random number between 0 and 99
This example shows how to limit the range of random numbers generated by `rand`.
Compatibility with `srand`
The `srand` function directly sets the seed for the `rand` sequence. This close-knit relationship allows developers to control randomness based on their specific applications. Running a loop that generates multiple random numbers can illustrate this synergy beautifully:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
srand(static_cast<unsigned int>(time(0))); // Dynamic seeding
for (int i = 0; i < 5; ++i) {
std::cout << rand() % 100 << std::endl; // Outputs different random numbers on each run
}
return 0;
}
Each time you execute this code, you will likely receive a fresh sequence of random numbers ranging from 0 to 99.
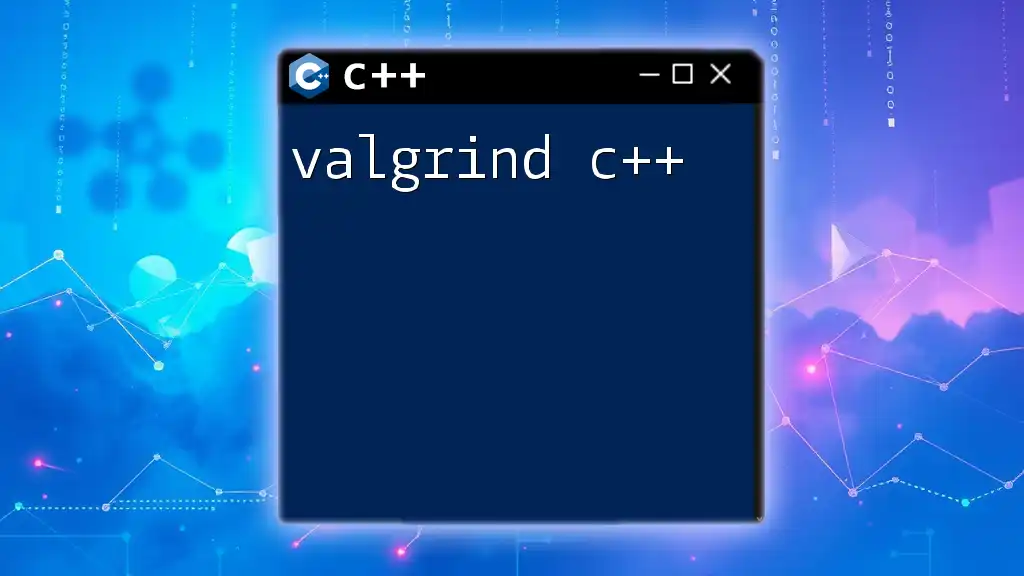
Common Mistakes to Avoid When Using `srand`
Seeding Multiple Times
A common pitfall when using `srand` is reseeding multiple times within a program, particularly in loops. Reseeding with `srand(time(0));` inside a loop can lead to generating the same random value if the loop executes quickly enough (i.e., during the same second). It’s best to seed once at the beginning of the program, as demonstrated earlier.
Forgetting to Include Headers
For using `srand` and `rand`, it is vital to include the appropriate headers:
#include <cstdlib>
#include <ctime>
Failing to include these can lead to compile-time errors:
// Uncommenting this will lead to errors
// srand(time(0));
// std::cout << rand();
Conclusion
The `srand` function is a vital component in generating random numbers in C++. By understanding its role, properly utilizing it with the `rand` function, and avoiding common pitfalls, developers can harness the power of randomness for a variety of applications.
Embrace experimentation with `srand` and witness how it can enhance your C++ projects, particularly in game development and simulations. To gain an even deeper understanding of random number generation, explore additional resources that dive into advanced topics and techniques!