R and C++ can work together to enhance data analysis and performance by allowing R to call C++ functions for computationally intensive tasks.
Here's a simple example of how to integrate R with C++ using the Rcpp package:
#include <Rcpp.h>
// [[Rcpp::export]]
double addNumbers(double a, double b) {
return a + b;
}
This snippet defines a C++ function that adds two numbers, which can be easily called from R.
Understanding R and C++
What is R?
R is a programming language and environment that excels in statistical computing and graphics. It is widely used by data scientists for data analysis, statistical modeling, and visualization. With its extensive libraries and packages, R provides powerful tools for tasks like regression analysis, time series analysis, and machine learning. The community around R is vibrant, contributing various packages and libraries that enhance its functionality and capabilities in handling diverse data challenges.
What is C++?
C++ is a flexible and powerful general-purpose programming language that builds on the foundations of C, adding features like object-oriented programming. Known for its speed and efficiency, C++ is widely utilized in applications where performance is critical, such as game development, real-time simulation, and systems-level programming. It enables developers to manage system resources and perform complex algorithms effectively, making it a preferred choice for applications requiring high-performance computing.
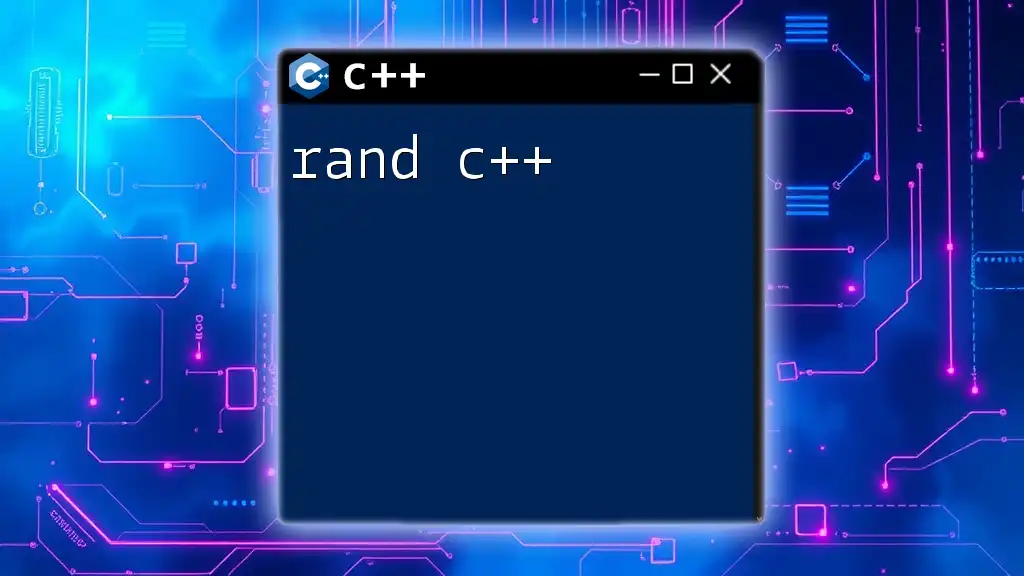
Benefits of Combining R and C++
Performance Enhancements
One of the primary advantages of integrating R and C++ is performance. C++ offers significantly faster execution times for computational tasks compared to R. For instance, when processing large datasets or performing complex calculations, C++ can execute operations in a fraction of the time it would take R alone. This efficiency is crucial for data scientists and analysts who increasingly deal with big data.
Extending R with C++
Integrating C++ allows R users to write custom functions tailored to specific computational tasks. C++ can handle computationally intensive operations that would be inefficient or slow in R. One popular way to facilitate this integration is through the Rcpp package, which acts as a bridge between R and C++. Rcpp makes it straightforward to write C++ functions and call them directly from R, enabling users to leverage the strengths of both languages.
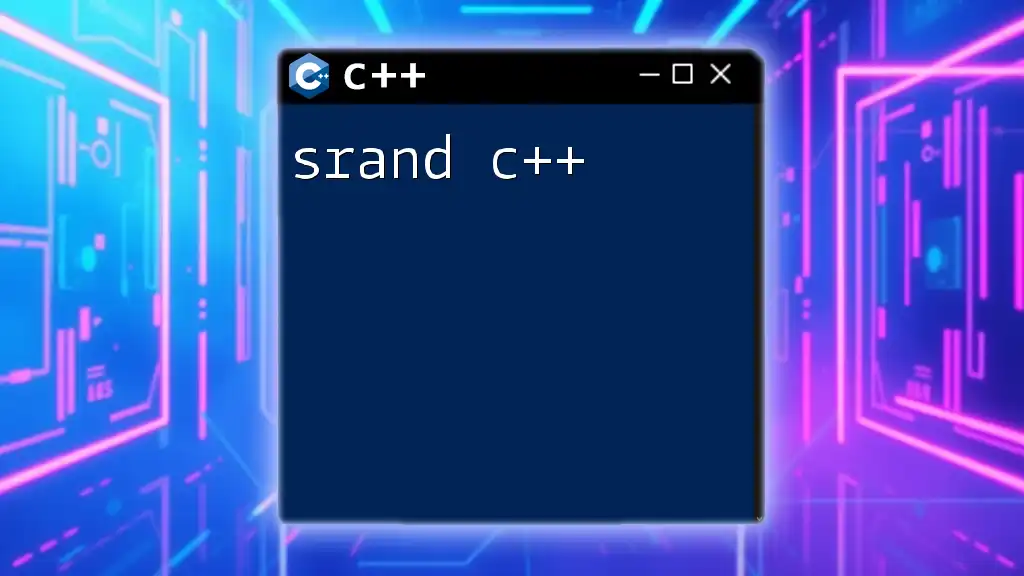
Setting Up Your Environment
Installing R and RTools
To get started with R and C++, the first step is to install R along with RTools for Windows users. For macOS and Linux, alternatives like Xcode and build-essential can be utilized. Installation guides are readily available on the official R website, ensuring you can set up your environment smoothly.
Installing Rcpp Package
Once R is installed, the next step is to install the Rcpp package, which will facilitate the integration of C++ into your R workflows. The installation can be completed with a simple command in R:
install.packages("Rcpp")
By executing this command, you'll gain access to Rcpp's functionalities, allowing you to start calling C++ code from R seamlessly.
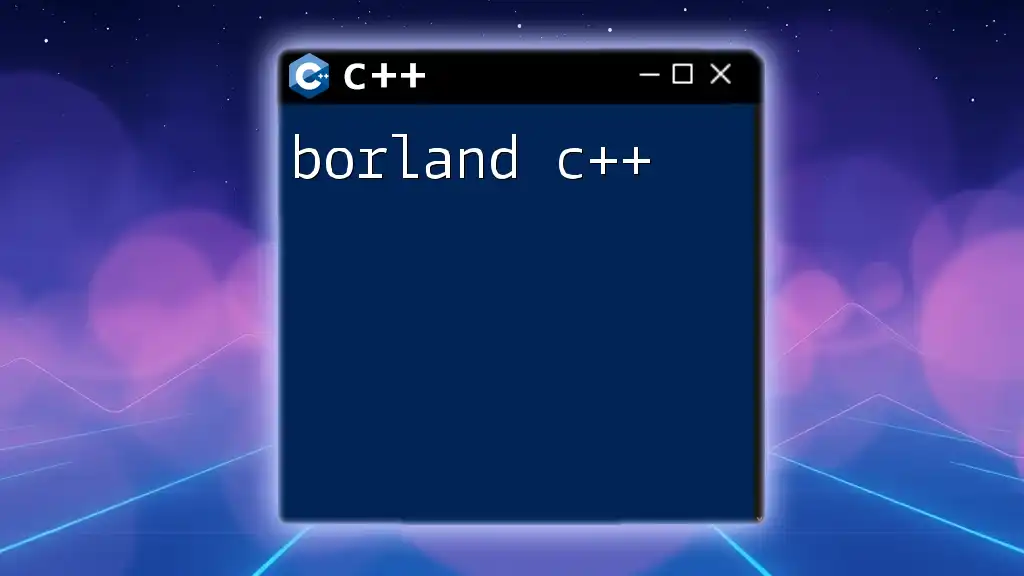
Writing Your First C++ Function in R
Creating a Simple C++ Function
Let’s walk through creating a basic C++ function that calculates the sum of an array of numbers. With Rcpp, writing C++ code that can be used in R is straightforward.
Here’s a simple C++ function to perform the calculation:
// Simple C++ function to calculate the sum of an array
#include <Rcpp.h>
using namespace Rcpp;
// [[Rcpp::export]]
double cpp_sum(NumericVector x) {
double sum = 0;
for(int i = 0; i < x.size(); i++) {
sum += x[i];
}
return sum;
}
Using the C++ Function in R
Once you've written your C++ function, you need to compile and call it from R. You can do this easily with the `sourceCPP` function from Rcpp. Here’s how to execute the function after saving it to a file:
Rcpp::sourceCPP("path/to/your/cpp_function.cpp")
cpp_sum(c(1, 2, 3, 4)) // Should return 10
This command compiles the C++ code and makes the function available in your R environment, where you can call it just like any native R function.
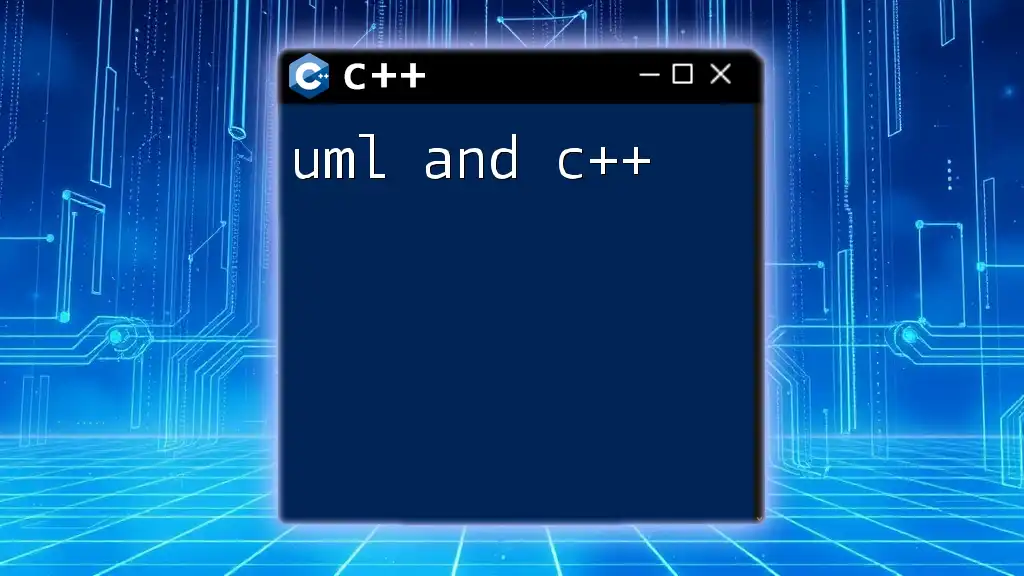
Advanced C++ Integration Techniques
Memory Management
When integrating C++ with R, understanding memory management is crucial. R has its own memory model that can be different from C++. It’s essential to avoid memory leaks by ensuring proper allocation and deallocation of memory in your C++ code. Using smart pointers in C++ can help manage memory automatically, reducing the risk of leaks.
Data Types and Structures
When working between R and C++, it's vital to match R's data types with their C++ counterparts. For example, R's NumericVector corresponds to `std::vector<double>` in C++. Understanding how to convert between these data types is key to successful integration, allowing for efficient data manipulation.
Creating R Packages with C++ Code
For those looking to extend their work further, creating R packages that include C++ code is a powerful approach. Tools like devtools alongside Rcpp can simplify this process, enabling you to encapsulate your functions into packages that can be easily shared and reused. The basic structure of an R package includes directories for R scripts, C++ code, and documentation, which you can set up using the `create` function from the devtools package.
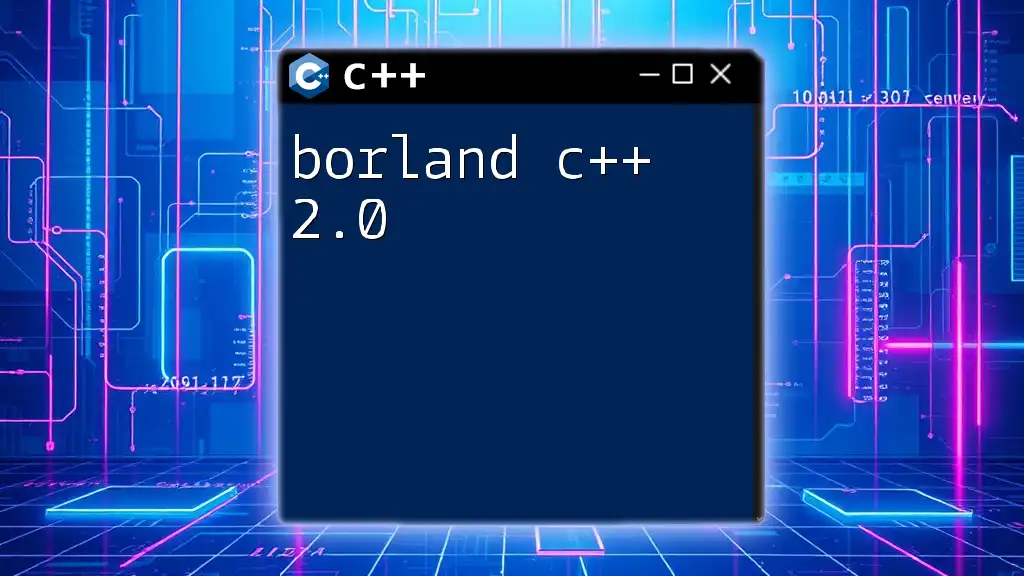
Common Challenges and Solutions
Debugging C++ Code in R
Debugging mixed-language code can pose challenges. Utilizing tools such as gdb for C++ debugging or R’s native debugging capabilities can provide insights into issues you may encounter. Common errors might include type mismatches or memory-related errors, which can usually be resolved through careful code inspection and employing debugging techniques.
Interfacing Between R and C++
You may face challenges while interfacing R and C++. For example, passing complex data structures may introduce compatibility issues. Having a deep understanding of how R handles data can help overcome these hurdles, ensuring you can effectively communicate between the two languages.
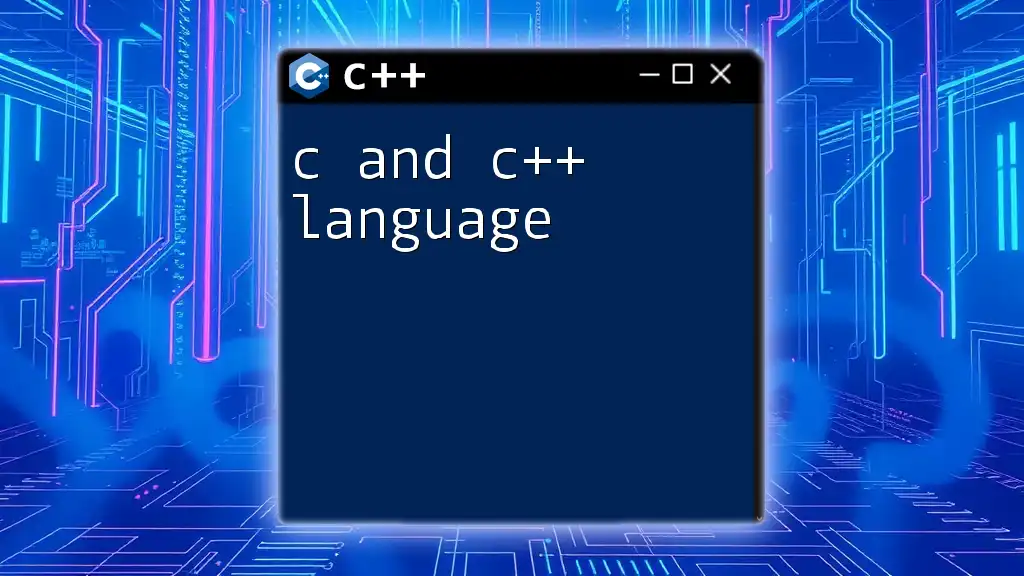
Case Studies: R and C++ in Action
Example 1: Machine Learning Model Training
Integrating C++ within R can significantly enhance the performance of machine learning algorithms. Suppose you are training a model on an enormous dataset; implementing the core algorithm in C++ can drastically reduce the computation time, allowing for more iterations and better optimization.
Example 2: Data Preprocessing
Using C++ for data preprocessing tasks can also enhance R's capabilities. For example, if you need to clean and transform vast amounts of data efficiently, implementing these algorithms in C++ allows you to take advantage of C++'s performance while still utilizing R's visualization and analysis tools.
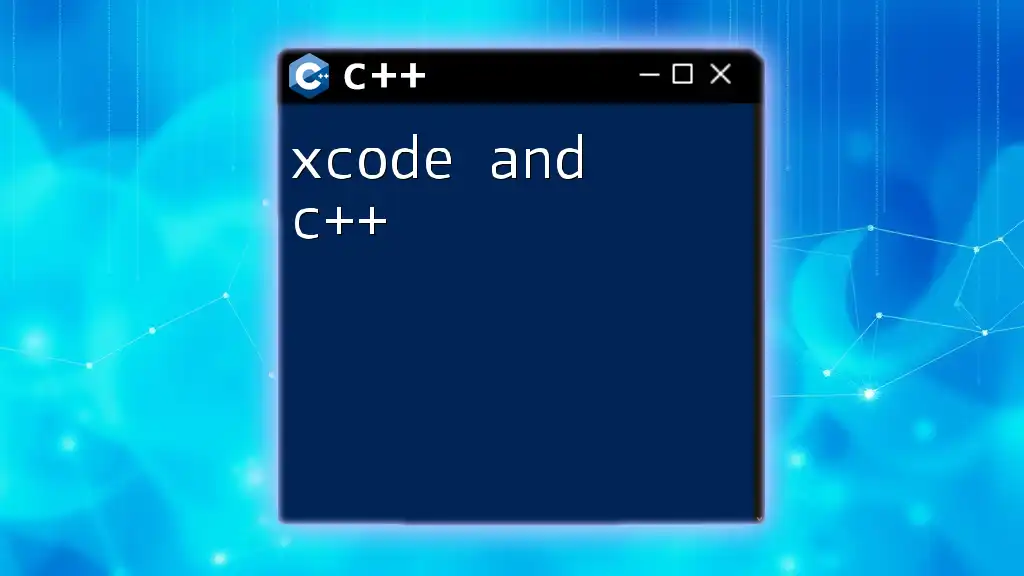
Conclusion
The integration of R and C++ offers significant advantages in performance and functionality, making it a valuable skill for data scientists and analysts. As both languages evolve, their interplay will become increasingly vital in addressing future data challenges. By leveraging the capabilities of both R and C++, you can enhance your data analysis processes and develop more powerful and efficient applications.
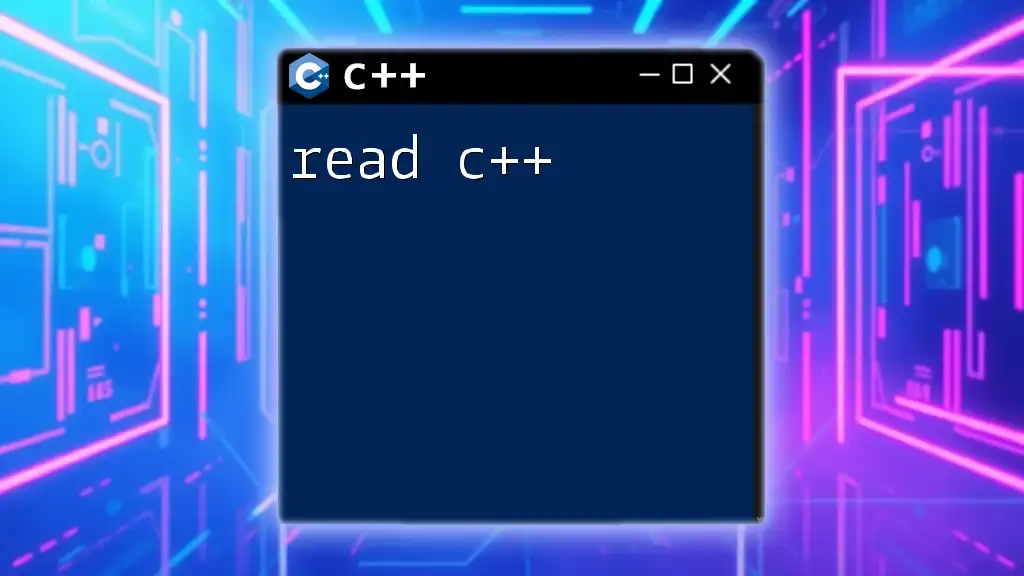
Call to Action
If you’re ready to dive deeper into the world of R and C++, numerous resources are available online, from tutorials to community forums. Don’t hesitate to experiment with integrating C++ into your R workflows, and feel free to share your experiences and questions in discussions with fellow learners!