Borland C++ is an early integrated development environment (IDE) and compiler for the C++ programming language, known for its ease of use and powerful features for building Windows applications.
Here's a simple example of a "Hello, World!" program in Borland C++:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Key Features of Borland C++
Borland C++ is known for its powerful Integrated Development Environment (IDE) and a host of advanced features that cater to both beginners and seasoned programmers.
Integrated Development Environment (IDE)
The Borland C++ IDE provides a user-friendly interface with an array of tools that make coding both intuitive and efficient. Key tools include:
- Code Editor Features: The editor includes syntax highlighting, code completion, and error detection, allowing programmers to spot mistakes as they code.
- Project Management: The IDE helps organize files and manage projects efficiently, enabling developers to focus on the code rather than on the project structure.
Compiler and Debugger
The built-in compiler is both fast and efficient, making it suitable for a variety of applications. The debugger enhances the development experience with features like:
- Breakpoints: Developers can pause execution at any point, making it easier to inspect variables and program state.
- Step-through Debugging: This feature allows for systematic examination of the code, making bugs easier to locate and fix.
Library Support
Borland C++ offers extensive library support, enabling developers to leverage existing code to accelerate their projects. Popular libraries include:
- The Standard Template Library (STL) for algorithms and data structures.
- Libraries for Windows API programming that allow for GUI development.
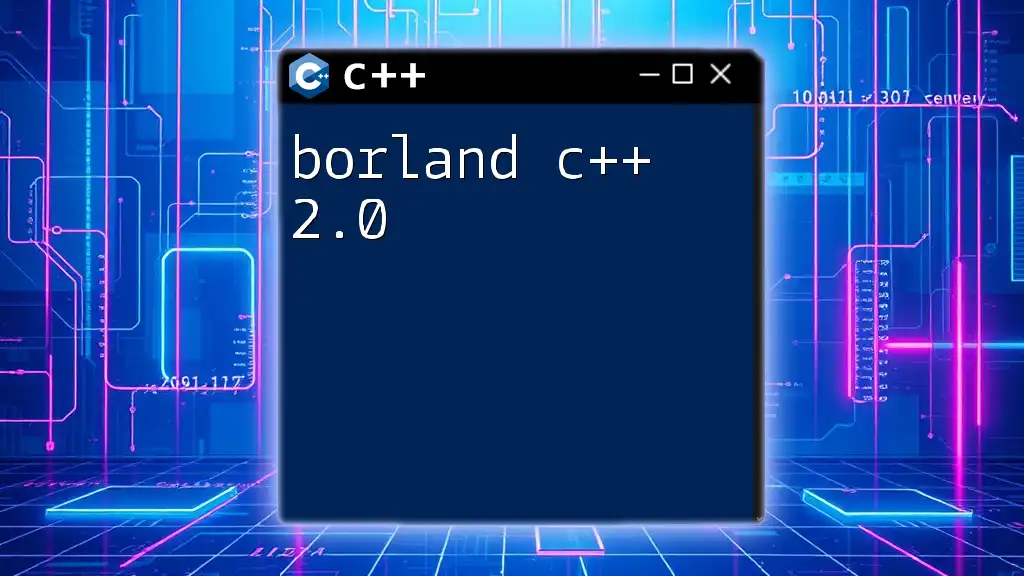
Getting Started with Borland C++
Installing Borland C++ Development Environment
To start using Borland C++, you'll first need to install the development environment. This involves:
- Downloading the installer from the official Borland website.
- Following the step-by-step instructions to complete the installation.
- Ensure your system meets the required specifications to run Borland C++ smoothly.
Creating Your First Project
Once installed, creating your first C++ project is straightforward:
- Launch the IDE and select the option to create a new project.
- Choose the type of project you want to create—either a console application or a GUI application.
- Set up the project properties to define settings like output directories and compiler options.
Understanding the Workspace
Familiarizing yourself with the workspace layout is crucial. Key components of the Borland C++ IDE workspace include:
- The Project Window: This shows all the files associated with your project.
- The Output Window: It displays compilation messages and errors, helping you track what’s happening with your code.
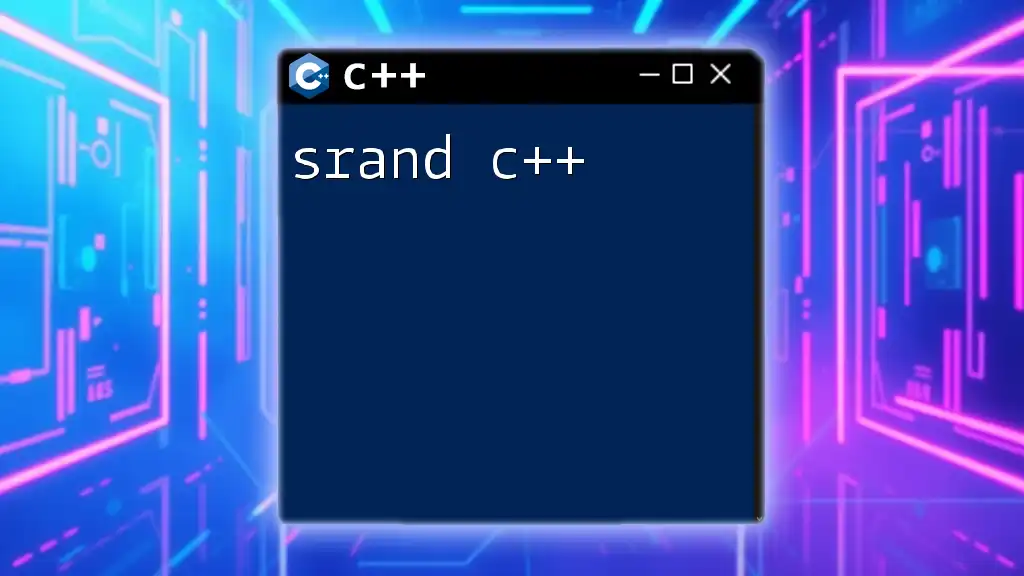
Basic Syntax and Program Structure in Borland C++
Understanding the basic syntax and structure of C++ is essential when working in Borland C++.
Overview of C++ Syntax
C++ syntax consists of a set of basic elements including variables, data types, and operators. For instance, declaring a variable and assigning a value is done like this:
int age = 25;
Writing Your First Program
To solidify your understanding, let's write a simple C++ program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This program does the following:
- Includes the iostream library for input and output.
- The `main` function serves as the entry point of the program.
- It outputs "Hello, World!" to the console.
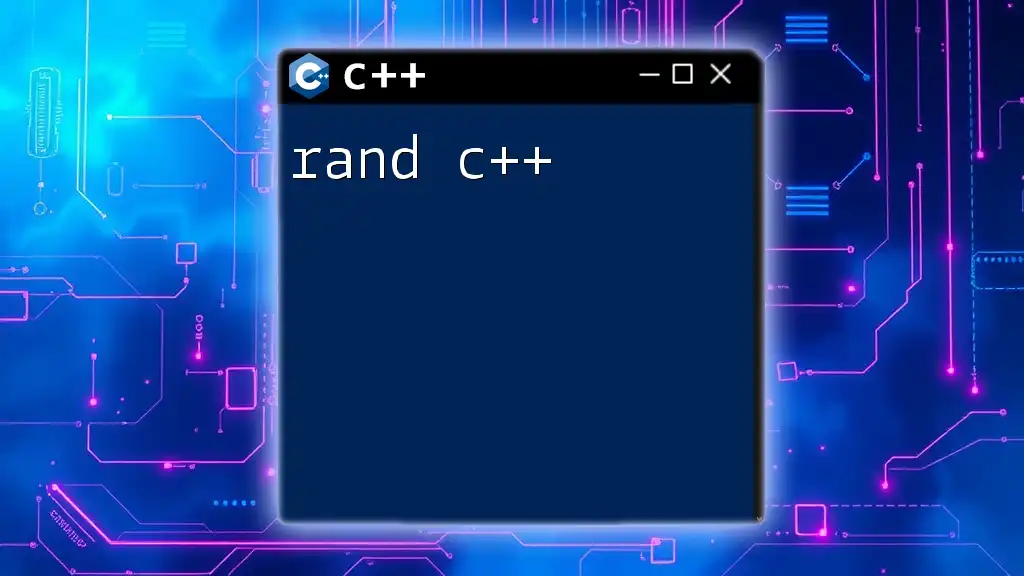
Advanced Features in Borland C++
Object-Oriented Programming
Borland C++ provides robust support for Object-Oriented Programming (OOP), enabling more structured and modular design of applications. Key concepts include:
- Classes and Objects: Define classes that encapsulate data and functions.
- Inheritance and Polymorphism: Extend functionality and create adaptable code.
Here is an example of a simple class in Borland C++:
class Dog {
public:
void bark() {
cout << "Woof!" << endl;
}
};
Template Programming
C++ templates allow for generic programming, which enhances code reusability. You can design functions and classes that operate with any data type. Here is an example of a template function:
template <typename T>
T add(T a, T b) {
return a + b;
}
This function can add integers, floats, or any other type that supports the addition operator.
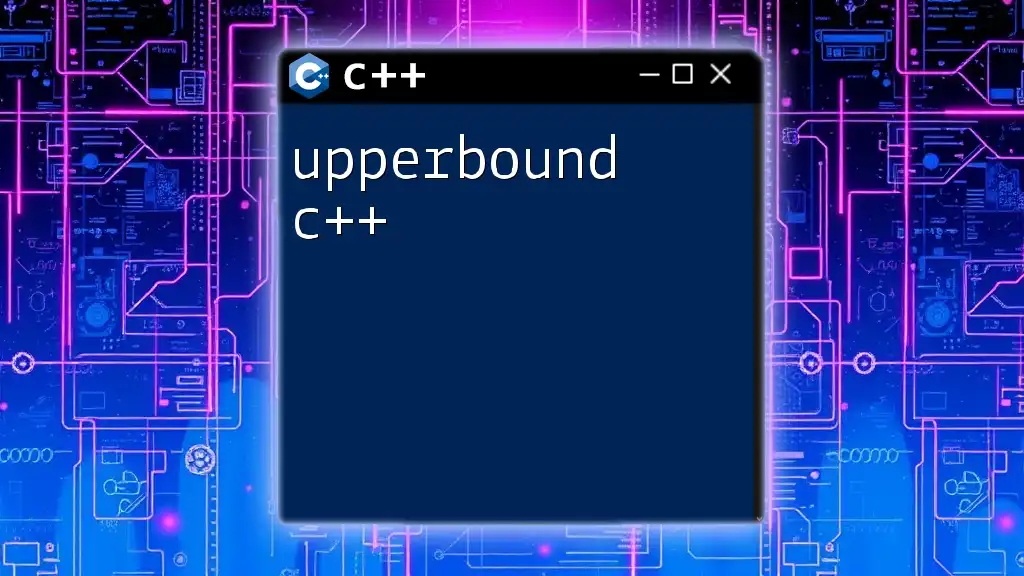
Debugging and Error Handling in Borland C++
Debugging Techniques
Debugging is an integral part of programming. Borland C++ offers several robust debugging techniques:
- Using Breakpoints: You can set breakpoints in your code to pause execution. This allows for inspection of variable values at specific points during runtime.
- Watch Variables: By using watch variables, you can keep track of how specific values change as your code executes.
Exception Handling in C++
Proper error handling ensures your application can manage unexpected issues gracefully. In C++, you can use `try`, `catch`, and `throw` statements for exception handling. Here’s an example:
try {
throw runtime_error("Error occurred");
} catch (runtime_error &e) {
cout << e.what() << endl;
}
This code snippet demonstrates how to throw and catch exceptions, providing a clear path for error management.
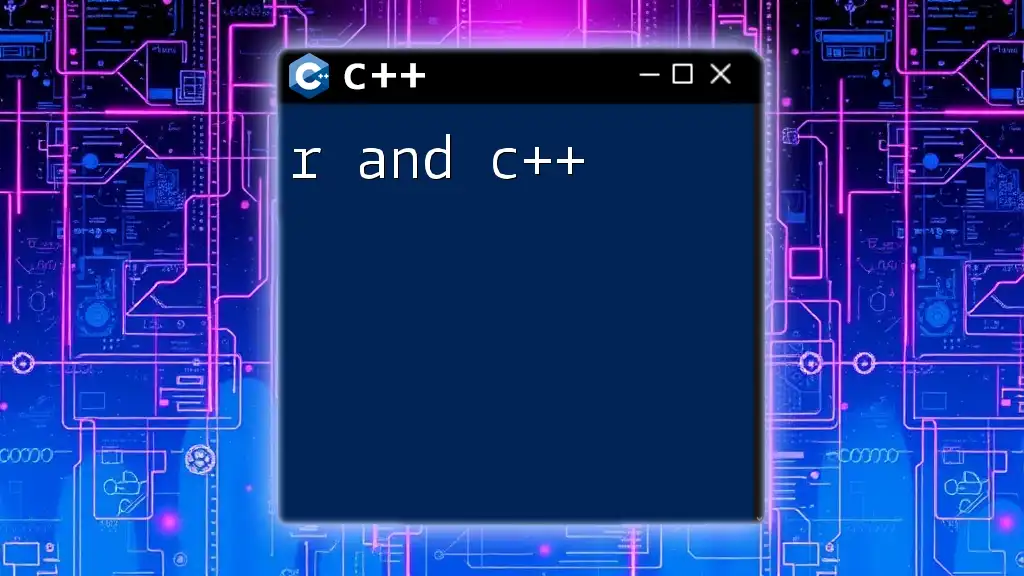
Best Practices for Writing C++ in Borland
Code Structure and Organization
Organizing your code sensibly is crucial for readability and maintainability. Consider dividing your code into modules, and use header files to declare functions.
Comments and Documentation
Effective comments and documentation enhance collaboration and make future updates easier. Use comments to explain complex sections of your code.
Performance Optimization
Optimizing your C++ code in Borland can lead to significant improvements in speed and efficiency. Use profiling tools to identify bottlenecks, and apply optimization techniques such as reducing memory usage and leveraging efficient algorithms.
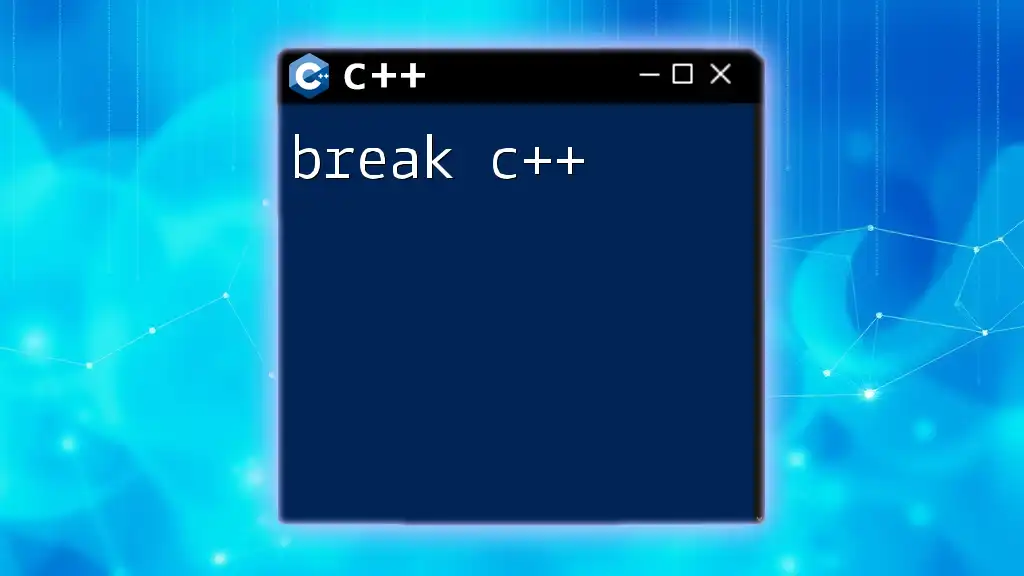
Common Issues and Troubleshooting
Common Errors in Borland C++
Even experienced developers encounter issues. Familiarize yourself with common compilation and runtime errors, such as:
- Syntax errors.
- Linker errors due to missing files.
- Runtime errors due to logic issues.
Community Resources
Engaging with the community can provide valuable insights. Explore forums and online resources to share experiences, ask for help, and learn best practices.
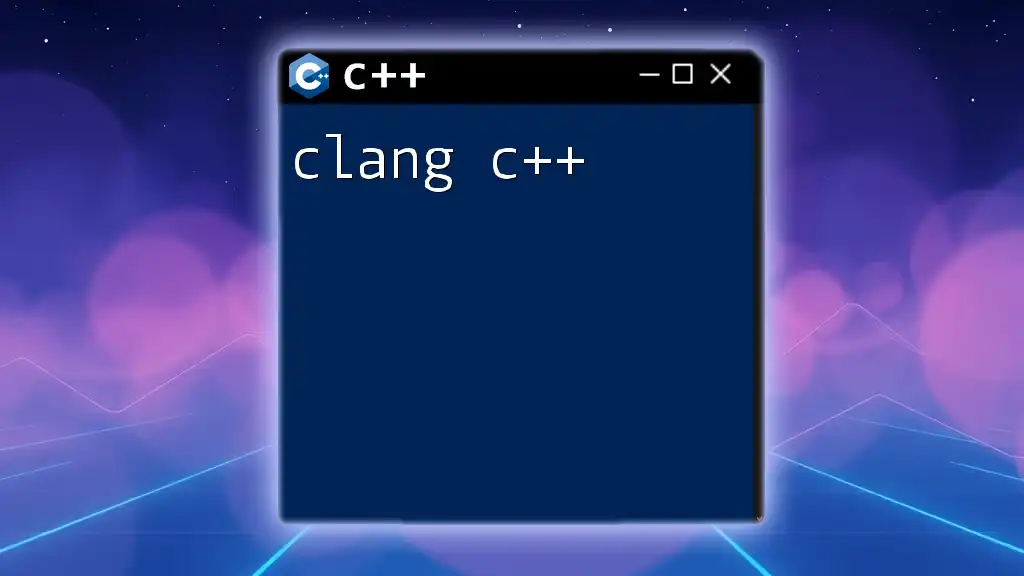
Conclusion
Borland C++ remains a powerful tool for developers, providing a rich environment that supports both basic and advanced programming concepts. By mastering its features—from project setup to object-oriented programming—you can enhance your coding proficiency and tackle increasingly complex projects.
Additional Resources
To further your learning, consider books and online courses focused on Borland C++. Utilize the official Borland C++ documentation and engage with online communities to stay updated and expand your knowledge.
Embrace the journey of learning Borland C++; the benefits are manifold, from writing efficient code to building robust applications. Happy coding!