In "C++ Without Fear," Brian Overland simplifies C++ programming concepts to empower learners to confidently write code with a clear understanding of fundamental commands and structures.
Here's a simple code snippet demonstrating basic input and output in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Without Fear
What Does "Without Fear" Mean?
Fear is a natural part of learning anything new, especially when it comes to programming languages like C++. Many beginners struggle with anxiety around syntax, concepts, and the overall complexity of C++. However, tackling these fears head-on is a crucial step toward mastering the language.
The phrase "Without Fear" encapsulates a mindset shift. It’s about recognizing that mistakes are part of the learning journey and that every programmer has faced challenges. Embracing challenges rather than shying away from them fosters a learning experience that is both enriching and fulfilling.
The Importance of a Strong Foundation
Basic Concepts of C++
Before diving into more complex C++ topics, it’s essential to establish a firm understanding of its core concepts, which serve as the building blocks for everything that follows.
Variables, Data Types, and Operators are the fundamentals. Variables are used to store data, and choosing the right data type is crucial for effective memory management and performance.
For example:
int age = 25; // Integer data type
float height = 5.9; // Float data type
char grade = 'A'; // Character data type
Control Structures such as `if`, `for`, and `while` guide the flow of your program. Understanding these will allow you to make decisions and iterate over data efficiently.
Here’s a simple program demonstrating conditional statements:
int num = 10;
if (num > 5) {
std::cout << "Number is greater than 5" << std::endl;
} else {
std::cout << "Number is 5 or less" << std::endl;
}
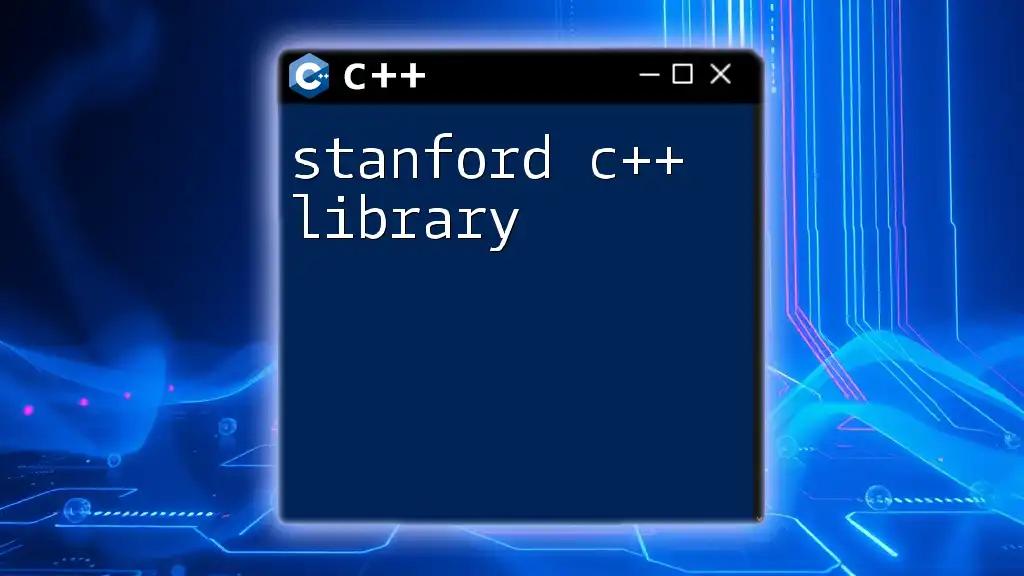
Brian Overland’s Approach to Learning C++
Key Principles of C++ Without Fear
Brian Overland emphasizes simplifying complex concepts to make them digestible. He advocates breaking down each topic into manageable parts, enabling learners to build their confidence progressively.
Hands-On Practice is another cornerstone of his approach. Theoretical knowledge without real-world application can lead to frustration. Putting concepts into practice through exercises is key to reinforcing what you've learned.
For example, creating a simple calculator can help solidify your understanding of user input, loops, and functions:
#include <iostream>
using namespace std;
int main() {
char op;
float num1, num2;
cout << "Enter operator (+, -, *, /): ";
cin >> op;
cout << "Enter first number: ";
cin >> num1;
cout << "Enter second number: ";
cin >> num2;
switch(op) {
case '+':
cout << num1 + num2;
break;
case '-':
cout << num1 - num2;
break;
case '*':
cout << num1 * num2;
break;
case '/':
if(num2 != 0)
cout << num1 / num2;
else
cout << "Division by zero!";
break;
default:
cout << "Invalid operator!";
break;
}
return 0;
}
Essential Resources
To delve deeper into C++, one should explore various books and online courses. Brian Overland’s book, "C++ Without Fear," serves as an excellent guide for beginners looking to approach C++ without intimidation.
In addition to books, online platforms offer courses catering to various skill levels. Engaging with these resources can significantly enhance your understanding of C++ concepts.
Another vital component of learning is community and support. Joining forums, discussion groups, and finding mentorship can provide helpful insights and encouragement. Engaging with fellow learners fosters a collaborative environment where everyone can thrive.

Key Features of C++ to Embrace
Understanding Object-Oriented Programming
Object-Oriented Programming (OOP) is a fundamental aspect of C++. It revolves around the concept of classes and objects, which enable better data organization and modularity.
Classes serve as blueprints for creating objects. They encapsulate data and functions that operate on that data.
Here’s an example of a simple class definition:
class Car {
public:
string model;
int year;
void displayInfo() {
std::cout << "Model: " << model << ", Year: " << year << std::endl;
}
};
int main() {
Car myCar;
myCar.model = "Toyota";
myCar.year = 2022;
myCar.displayInfo();
return 0;
}
In this example, we define a class `Car` and implement a method to display its details. This encapsulation helps in managing complexity in larger programs.
Working with Standard Template Library (STL)
The Standard Template Library (STL) is an invaluable part of the C++ ecosystem. It provides a set of common data structures and algorithms that can save you time and effort, allowing you to focus on the logic of your programs.
Understanding and utilizing STL right from the beginning can drastically increase your productivity. For instance, using `vectors` for dynamic array management can optimize your code.
Here’s a quick code snippet showcasing how to create and manipulate a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.push_back(6); // Adding an element
for (int i : numbers) {
std::cout << i << " ";
}
}
The use of vectors here demonstrates how STL can simplify complex data manipulations, thus enabling you to write cleaner and more efficient code.
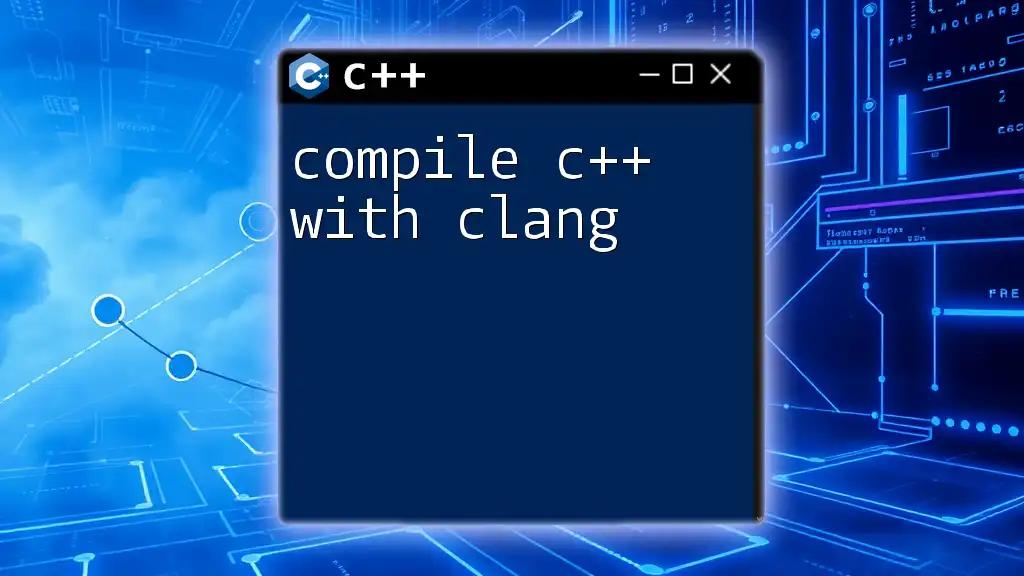
Overcoming Common Issues and Errors
Debugging in C++
Inevitably, every programmer faces errors and bugs in their code. Understanding how to debug effectively is crucial for every C++ learner. There are primarily two types of errors: compile-time errors, which occur during compilation, and run-time errors, which happen during the execution of the code.
Familiarizing yourself with debugging tools like GDB can help you identify and resolve issues quickly. Using breakpoints, watch expressions, and step execution will make the debugging process manageable, turning it from a daunting task into a systematic approach.
Avoiding Pitfalls
As you embark on your C++ journey, be aware of common mistakes that many beginners make. Memory leaks—failing to free dynamically allocated memory—can lead to significant performance issues.
Implementing best practices for writing clean code will also benefit you in the long run. Prioritize readability through consistent naming conventions and adequate comments. A well-documented codebase will not only serve as a reference for you but also helps others understand your thought process.
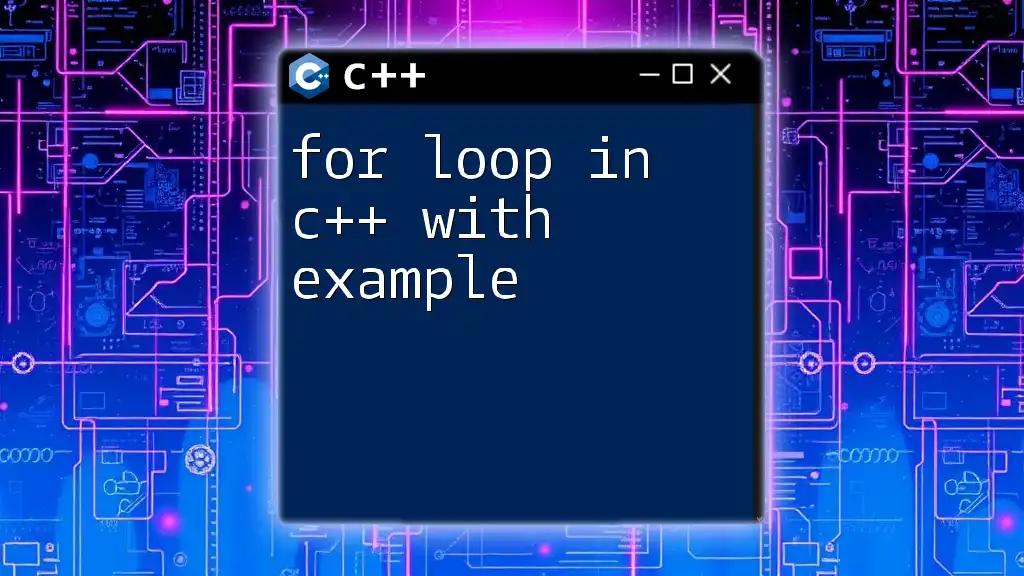
Conclusion
In summary, Brian Overland’s C++ Without Fear approach encourages learners to embrace challenges as part of their growth. By building a strong foundation, focusing on hands-on learning, and fostering a community of support, anyone can conquer their fears and become proficient in C++.
Every step taken in this journey is a step towards becoming a confident programmer. Take the first step, practice relentlessly, and remember: mistakes are simply opportunities for growth. The world of C++ awaits you!